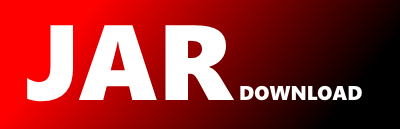
org.teamapps.dto.UiTree Maven / Gradle / Ivy
The newest version!
package org.teamapps.dto;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
/**
* THIS IS GENERATED CODE!
* PLEASE DO NOT MODIFY - ALL YOUR WORK WOULD BE LOST!
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.CUSTOM, property = "_type", defaultImpl = UiTree.class)
public class UiTree extends UiComponent implements UiObject {
protected Map templates;
protected String defaultTemplateId = null;
protected List initialData;
protected Integer selectedNodeId;
protected boolean animate;
protected boolean showExpanders = true;
protected boolean openOnSelection = false;
protected boolean enforceSingleExpandedPath = false;
protected int indentation = 15;
public UiTree() {
super();
}
@com.fasterxml.jackson.annotation.JsonIgnore
public UiObjectType getUiObjectType() {
return UiObjectType.UI_TREE;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("id=" + id).append(", ")
.append("debuggingId=" + debuggingId).append(", ")
.append("defaultTemplateId=" + defaultTemplateId).append(", ")
.append("selectedNodeId=" + selectedNodeId).append(", ")
.append("classNamesBySelector=" + classNamesBySelector).append(", ")
.append("visible=" + visible).append(", ")
.append("stylesBySelector=" + stylesBySelector).append(", ")
.append("attributesBySelector=" + attributesBySelector).append(", ")
.append(templates != null ? "templates={" + templates.toString() + "}" : "").append(", ")
.append("animate=" + animate).append(", ")
.append("showExpanders=" + showExpanders).append(", ")
.append("openOnSelection=" + openOnSelection).append(", ")
.append("enforceSingleExpandedPath=" + enforceSingleExpandedPath).append(", ")
.append("indentation=" + indentation).append(", ")
.append(initialData != null ? "initialData={" + initialData.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("templates")
public Map getTemplates() {
return templates;
}
@com.fasterxml.jackson.annotation.JsonGetter("defaultTemplateId")
public String getDefaultTemplateId() {
return defaultTemplateId;
}
@com.fasterxml.jackson.annotation.JsonGetter("initialData")
public List getInitialData() {
return initialData;
}
@com.fasterxml.jackson.annotation.JsonGetter("selectedNodeId")
public Integer getSelectedNodeId() {
return selectedNodeId;
}
@com.fasterxml.jackson.annotation.JsonGetter("animate")
public boolean getAnimate() {
return animate;
}
@com.fasterxml.jackson.annotation.JsonGetter("showExpanders")
public boolean getShowExpanders() {
return showExpanders;
}
@com.fasterxml.jackson.annotation.JsonGetter("openOnSelection")
public boolean getOpenOnSelection() {
return openOnSelection;
}
@com.fasterxml.jackson.annotation.JsonGetter("enforceSingleExpandedPath")
public boolean getEnforceSingleExpandedPath() {
return enforceSingleExpandedPath;
}
@com.fasterxml.jackson.annotation.JsonGetter("indentation")
public int getIndentation() {
return indentation;
}
@com.fasterxml.jackson.annotation.JsonSetter("id")
public UiTree setId(String id) {
this.id = id;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("debuggingId")
public UiTree setDebuggingId(String debuggingId) {
this.debuggingId = debuggingId;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("visible")
public UiTree setVisible(boolean visible) {
this.visible = visible;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("stylesBySelector")
public UiTree setStylesBySelector(Map> stylesBySelector) {
this.stylesBySelector = stylesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("classNamesBySelector")
public UiTree setClassNamesBySelector(Map> classNamesBySelector) {
this.classNamesBySelector = classNamesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("attributesBySelector")
public UiTree setAttributesBySelector(Map> attributesBySelector) {
this.attributesBySelector = attributesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("templates")
public UiTree setTemplates(Map templates) {
this.templates = templates;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("defaultTemplateId")
public UiTree setDefaultTemplateId(String defaultTemplateId) {
this.defaultTemplateId = defaultTemplateId;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("initialData")
public UiTree setInitialData(List initialData) {
this.initialData = initialData;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("selectedNodeId")
public UiTree setSelectedNodeId(Integer selectedNodeId) {
this.selectedNodeId = selectedNodeId;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("animate")
public UiTree setAnimate(boolean animate) {
this.animate = animate;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("showExpanders")
public UiTree setShowExpanders(boolean showExpanders) {
this.showExpanders = showExpanders;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("openOnSelection")
public UiTree setOpenOnSelection(boolean openOnSelection) {
this.openOnSelection = openOnSelection;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("enforceSingleExpandedPath")
public UiTree setEnforceSingleExpandedPath(boolean enforceSingleExpandedPath) {
this.enforceSingleExpandedPath = enforceSingleExpandedPath;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("indentation")
public UiTree setIndentation(int indentation) {
this.indentation = indentation;
return this;
}
public static class TextInputEvent implements UiEvent {
@UiComponentId protected String componentId;
protected String text;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public TextInputEvent() {
// default constructor for Jackson
}
public TextInputEvent(String componentId, String text) {
this.componentId = componentId;
this.text = text;
}
public UiEventType getUiEventType() {
return UiEventType.UI_TREE_TEXT_INPUT;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("text=" + text)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("text")
public String getText() {
return text;
}
}
public static class NodeSelectedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int nodeId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public NodeSelectedEvent() {
// default constructor for Jackson
}
public NodeSelectedEvent(String componentId, int nodeId) {
this.componentId = componentId;
this.nodeId = nodeId;
}
public UiEventType getUiEventType() {
return UiEventType.UI_TREE_NODE_SELECTED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("nodeId=" + nodeId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodeId")
public int getNodeId() {
return nodeId;
}
}
public static class NodeExpansionChangedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int nodeId;
protected boolean expanded;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public NodeExpansionChangedEvent() {
// default constructor for Jackson
}
public NodeExpansionChangedEvent(String componentId, int nodeId, boolean expanded) {
this.componentId = componentId;
this.nodeId = nodeId;
this.expanded = expanded;
}
public UiEventType getUiEventType() {
return UiEventType.UI_TREE_NODE_EXPANSION_CHANGED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("nodeId=" + nodeId).append(", ")
.append("expanded=" + expanded)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodeId")
public int getNodeId() {
return nodeId;
}
@com.fasterxml.jackson.annotation.JsonGetter("expanded")
public boolean getExpanded() {
return expanded;
}
}
public static class RequestTreeDataEvent implements UiEvent {
@UiComponentId protected String componentId;
protected int parentNodeId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public RequestTreeDataEvent() {
// default constructor for Jackson
}
public RequestTreeDataEvent(String componentId, int parentNodeId) {
this.componentId = componentId;
this.parentNodeId = parentNodeId;
}
public UiEventType getUiEventType() {
return UiEventType.UI_TREE_REQUEST_TREE_DATA;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("parentNodeId=" + parentNodeId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("parentNodeId")
public int getParentNodeId() {
return parentNodeId;
}
}
public static class ReplaceDataCommand implements UiCommand {
@UiComponentId protected String componentId;
protected List nodes;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public ReplaceDataCommand() {
// default constructor for Jackson
}
public ReplaceDataCommand(String componentId, List nodes) {
this.componentId = componentId;
this.nodes = nodes;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append(nodes != null ? "nodes={" + nodes.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodes")
public List getNodes() {
return nodes;
}
}
public static class BulkUpdateCommand implements UiCommand {
@UiComponentId protected String componentId;
protected List nodesToBeRemoved;
protected List nodesToBeAdded;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public BulkUpdateCommand() {
// default constructor for Jackson
}
public BulkUpdateCommand(String componentId, List nodesToBeRemoved, List nodesToBeAdded) {
this.componentId = componentId;
this.nodesToBeRemoved = nodesToBeRemoved;
this.nodesToBeAdded = nodesToBeAdded;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("nodesToBeRemoved=" + nodesToBeRemoved).append(", ")
.append(nodesToBeAdded != null ? "nodesToBeAdded={" + nodesToBeAdded.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodesToBeRemoved")
public List getNodesToBeRemoved() {
return nodesToBeRemoved;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodesToBeAdded")
public List getNodesToBeAdded() {
return nodesToBeAdded;
}
}
public static class SetSelectedNodeCommand implements UiCommand {
@UiComponentId protected String componentId;
protected Integer recordId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetSelectedNodeCommand() {
// default constructor for Jackson
}
public SetSelectedNodeCommand(String componentId, Integer recordId) {
this.componentId = componentId;
this.recordId = recordId;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("recordId=" + recordId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("recordId")
public Integer getRecordId() {
return recordId;
}
}
public static class RegisterTemplateCommand implements UiCommand {
@UiComponentId protected String componentId;
protected String id;
protected UiTemplate template;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public RegisterTemplateCommand() {
// default constructor for Jackson
}
public RegisterTemplateCommand(String componentId, String id, UiTemplate template) {
this.componentId = componentId;
this.id = id;
this.template = template;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("id=" + id).append(", ")
.append("componentId=" + componentId).append(", ")
.append(template != null ? "template={" + template.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("id")
public String getId() {
return id;
}
@com.fasterxml.jackson.annotation.JsonGetter("template")
public UiTemplate getTemplate() {
return template;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy