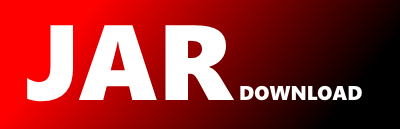
org.teamapps.dto.UiConfiguration Maven / Gradle / Ivy
package org.teamapps.dto;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
/**
* THIS IS GENERATED CODE!
* PLEASE DO NOT MODIFY - ALL YOUR WORK WOULD BE LOST!
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.CUSTOM, property = "_type")
@JsonTypeIdResolver(TeamAppsJacksonTypeIdResolver.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class UiConfiguration implements UiObject {
protected String isoLanguage = "en";
protected String timeZoneId = "Europe/Berlin";
protected String dateFormat = "yyyy-MM-dd";
protected String timeFormat = "HH:mm";
protected UiWeekDay firstDayOfWeek = UiWeekDay.MONDAY;
protected String decimalSeparator = ".";
protected String thousandsSeparator = "";
protected String themeClassName = null;
protected boolean optimizedForTouch = false;
protected String iconPath = "icons";
public UiConfiguration() {
}
@com.fasterxml.jackson.annotation.JsonIgnore
public UiObjectType getUiObjectType() {
return UiObjectType.UI_CONFIGURATION;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("timeZoneId=" + timeZoneId).append(", ")
.append("themeClassName=" + themeClassName).append(", ")
.append("isoLanguage=" + isoLanguage).append(", ")
.append("dateFormat=" + dateFormat).append(", ")
.append("timeFormat=" + timeFormat).append(", ")
.append("firstDayOfWeek=" + firstDayOfWeek).append(", ")
.append("decimalSeparator=" + decimalSeparator).append(", ")
.append("thousandsSeparator=" + thousandsSeparator).append(", ")
.append("optimizedForTouch=" + optimizedForTouch).append(", ")
.append("iconPath=" + iconPath)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("isoLanguage")
public String getIsoLanguage() {
return isoLanguage;
}
@com.fasterxml.jackson.annotation.JsonGetter("timeZoneId")
public String getTimeZoneId() {
return timeZoneId;
}
@com.fasterxml.jackson.annotation.JsonGetter("dateFormat")
public String getDateFormat() {
return dateFormat;
}
@com.fasterxml.jackson.annotation.JsonGetter("timeFormat")
public String getTimeFormat() {
return timeFormat;
}
@com.fasterxml.jackson.annotation.JsonGetter("firstDayOfWeek")
public UiWeekDay getFirstDayOfWeek() {
return firstDayOfWeek;
}
@com.fasterxml.jackson.annotation.JsonGetter("decimalSeparator")
public String getDecimalSeparator() {
return decimalSeparator;
}
@com.fasterxml.jackson.annotation.JsonGetter("thousandsSeparator")
public String getThousandsSeparator() {
return thousandsSeparator;
}
@com.fasterxml.jackson.annotation.JsonGetter("themeClassName")
public String getThemeClassName() {
return themeClassName;
}
@com.fasterxml.jackson.annotation.JsonGetter("optimizedForTouch")
public boolean getOptimizedForTouch() {
return optimizedForTouch;
}
@com.fasterxml.jackson.annotation.JsonGetter("iconPath")
public String getIconPath() {
return iconPath;
}
@com.fasterxml.jackson.annotation.JsonSetter("isoLanguage")
public UiConfiguration setIsoLanguage(String isoLanguage) {
this.isoLanguage = isoLanguage;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("timeZoneId")
public UiConfiguration setTimeZoneId(String timeZoneId) {
this.timeZoneId = timeZoneId;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("dateFormat")
public UiConfiguration setDateFormat(String dateFormat) {
this.dateFormat = dateFormat;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("timeFormat")
public UiConfiguration setTimeFormat(String timeFormat) {
this.timeFormat = timeFormat;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("firstDayOfWeek")
public UiConfiguration setFirstDayOfWeek(UiWeekDay firstDayOfWeek) {
this.firstDayOfWeek = firstDayOfWeek;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("decimalSeparator")
public UiConfiguration setDecimalSeparator(String decimalSeparator) {
this.decimalSeparator = decimalSeparator;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("thousandsSeparator")
public UiConfiguration setThousandsSeparator(String thousandsSeparator) {
this.thousandsSeparator = thousandsSeparator;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("themeClassName")
public UiConfiguration setThemeClassName(String themeClassName) {
this.themeClassName = themeClassName;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("optimizedForTouch")
public UiConfiguration setOptimizedForTouch(boolean optimizedForTouch) {
this.optimizedForTouch = optimizedForTouch;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("iconPath")
public UiConfiguration setIconPath(String iconPath) {
this.iconPath = iconPath;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy