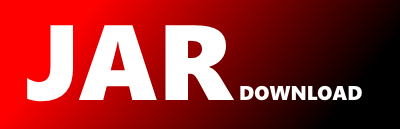
org.teamapps.dto.UiNetworkGraph Maven / Gradle / Ivy
package org.teamapps.dto;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
/**
* THIS IS GENERATED CODE!
* PLEASE DO NOT MODIFY - ALL YOUR WORK WOULD BE LOST!
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.CUSTOM, property = "_type")
@JsonTypeIdResolver(TeamAppsJacksonTypeIdResolver.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class UiNetworkGraph extends UiComponent implements UiObject {
protected List nodes;
protected List links;
protected List images;
protected float gravity = 0.1f;
protected float theta = 0.3f;
protected float alpha = 0.1f;
protected int charge = -300;
protected int distance = 30;
protected String highlightColor;
protected int animationDuration = 1000;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public UiNetworkGraph() {
// default constructor for Jackson
}
public UiNetworkGraph(List nodes, List links, List images) {
super();
this.nodes = nodes;
this.links = links;
this.images = images;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public UiObjectType getUiObjectType() {
return UiObjectType.UI_NETWORK_GRAPH;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("id=" + id).append(", ")
.append("visible=" + visible).append(", ")
.append("stylesBySelector=" + stylesBySelector).append(", ")
.append("gravity=" + gravity).append(", ")
.append("theta=" + theta).append(", ")
.append("alpha=" + alpha).append(", ")
.append("charge=" + charge).append(", ")
.append("distance=" + distance).append(", ")
.append("highlightColor=" + highlightColor).append(", ")
.append("animationDuration=" + animationDuration).append(", ")
.append(nodes != null ? "nodes={" + nodes.toString() + "}" : "").append(", ")
.append(links != null ? "links={" + links.toString() + "}" : "").append(", ")
.append(images != null ? "images={" + images.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("nodes")
public List getNodes() {
return nodes;
}
@com.fasterxml.jackson.annotation.JsonGetter("links")
public List getLinks() {
return links;
}
@com.fasterxml.jackson.annotation.JsonGetter("images")
public List getImages() {
return images;
}
@com.fasterxml.jackson.annotation.JsonGetter("gravity")
public float getGravity() {
return gravity;
}
@com.fasterxml.jackson.annotation.JsonGetter("theta")
public float getTheta() {
return theta;
}
@com.fasterxml.jackson.annotation.JsonGetter("alpha")
public float getAlpha() {
return alpha;
}
@com.fasterxml.jackson.annotation.JsonGetter("charge")
public int getCharge() {
return charge;
}
@com.fasterxml.jackson.annotation.JsonGetter("distance")
public int getDistance() {
return distance;
}
@com.fasterxml.jackson.annotation.JsonGetter("highlightColor")
public String getHighlightColor() {
return highlightColor;
}
@com.fasterxml.jackson.annotation.JsonGetter("animationDuration")
public int getAnimationDuration() {
return animationDuration;
}
@com.fasterxml.jackson.annotation.JsonSetter("id")
public UiNetworkGraph setId(String id) {
this.id = id;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("visible")
public UiNetworkGraph setVisible(boolean visible) {
this.visible = visible;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("stylesBySelector")
public UiNetworkGraph setStylesBySelector(Map> stylesBySelector) {
this.stylesBySelector = stylesBySelector;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("gravity")
public UiNetworkGraph setGravity(float gravity) {
this.gravity = gravity;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("theta")
public UiNetworkGraph setTheta(float theta) {
this.theta = theta;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("alpha")
public UiNetworkGraph setAlpha(float alpha) {
this.alpha = alpha;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("charge")
public UiNetworkGraph setCharge(int charge) {
this.charge = charge;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("distance")
public UiNetworkGraph setDistance(int distance) {
this.distance = distance;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("highlightColor")
public UiNetworkGraph setHighlightColor(String highlightColor) {
this.highlightColor = highlightColor;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("animationDuration")
public UiNetworkGraph setAnimationDuration(int animationDuration) {
this.animationDuration = animationDuration;
return this;
}
public static class NodeClickedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected String nodeId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public NodeClickedEvent() {
// default constructor for Jackson
}
public NodeClickedEvent(String componentId, String nodeId) {
this.componentId = componentId;
this.nodeId = nodeId;
}
public UiEventType getUiEventType() {
return UiEventType.UI_NETWORK_GRAPH_NODE_CLICKED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("nodeId=" + nodeId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodeId")
public String getNodeId() {
return nodeId;
}
}
public static class NodeExpandedOrCollapsedEvent implements UiEvent {
@UiComponentId protected String componentId;
protected String nodeId;
protected boolean expanded;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public NodeExpandedOrCollapsedEvent() {
// default constructor for Jackson
}
public NodeExpandedOrCollapsedEvent(String componentId, String nodeId, boolean expanded) {
this.componentId = componentId;
this.nodeId = nodeId;
this.expanded = expanded;
}
public UiEventType getUiEventType() {
return UiEventType.UI_NETWORK_GRAPH_NODE_EXPANDED_OR_COLLAPSED;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("nodeId=" + nodeId).append(", ")
.append("expanded=" + expanded)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodeId")
public String getNodeId() {
return nodeId;
}
@com.fasterxml.jackson.annotation.JsonGetter("expanded")
public boolean getExpanded() {
return expanded;
}
}
public static class SetZoomFactorCommand implements UiCommand {
@UiComponentId protected String componentId;
protected float zoomFactor;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetZoomFactorCommand() {
// default constructor for Jackson
}
public SetZoomFactorCommand(String componentId, float zoomFactor) {
this.componentId = componentId;
this.zoomFactor = zoomFactor;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("zoomFactor=" + zoomFactor)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("zoomFactor")
public float getZoomFactor() {
return zoomFactor;
}
}
public static class SetGravityCommand implements UiCommand {
@UiComponentId protected String componentId;
protected float gravity;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetGravityCommand() {
// default constructor for Jackson
}
public SetGravityCommand(String componentId, float gravity) {
this.componentId = componentId;
this.gravity = gravity;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("gravity=" + gravity)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("gravity")
public float getGravity() {
return gravity;
}
}
public static class SetChargeCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int charge;
protected boolean overrideNodeCharge;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetChargeCommand() {
// default constructor for Jackson
}
public SetChargeCommand(String componentId, int charge, boolean overrideNodeCharge) {
this.componentId = componentId;
this.charge = charge;
this.overrideNodeCharge = overrideNodeCharge;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("charge=" + charge).append(", ")
.append("overrideNodeCharge=" + overrideNodeCharge)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("charge")
public int getCharge() {
return charge;
}
@com.fasterxml.jackson.annotation.JsonGetter("overrideNodeCharge")
public boolean getOverrideNodeCharge() {
return overrideNodeCharge;
}
}
public static class SetDistanceCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int distance;
protected boolean overrideLinkDistance;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public SetDistanceCommand() {
// default constructor for Jackson
}
public SetDistanceCommand(String componentId, int distance, boolean overrideLinkDistance) {
this.componentId = componentId;
this.distance = distance;
this.overrideLinkDistance = overrideLinkDistance;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("distance=" + distance).append(", ")
.append("overrideLinkDistance=" + overrideLinkDistance)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("distance")
public int getDistance() {
return distance;
}
@com.fasterxml.jackson.annotation.JsonGetter("overrideLinkDistance")
public boolean getOverrideLinkDistance() {
return overrideLinkDistance;
}
}
public static class ZoomAllNodesIntoViewCommand implements UiCommand {
@UiComponentId protected String componentId;
protected int animationDuration;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public ZoomAllNodesIntoViewCommand() {
// default constructor for Jackson
}
public ZoomAllNodesIntoViewCommand(String componentId, int animationDuration) {
this.componentId = componentId;
this.animationDuration = animationDuration;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("animationDuration=" + animationDuration)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("animationDuration")
public int getAnimationDuration() {
return animationDuration;
}
}
public static class AddNodesAndLinksCommand implements UiCommand {
@UiComponentId protected String componentId;
protected List nodes;
protected List links;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public AddNodesAndLinksCommand() {
// default constructor for Jackson
}
public AddNodesAndLinksCommand(String componentId, List nodes, List links) {
this.componentId = componentId;
this.nodes = nodes;
this.links = links;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append(nodes != null ? "nodes={" + nodes.toString() + "}" : "").append(", ")
.append(links != null ? "links={" + links.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodes")
public List getNodes() {
return nodes;
}
@com.fasterxml.jackson.annotation.JsonGetter("links")
public List getLinks() {
return links;
}
}
public static class RemoveNodesAndLinksCommand implements UiCommand {
@UiComponentId protected String componentId;
protected List nodeIds;
protected Map> linksBySourceNodeId;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public RemoveNodesAndLinksCommand() {
// default constructor for Jackson
}
public RemoveNodesAndLinksCommand(String componentId, List nodeIds, Map> linksBySourceNodeId) {
this.componentId = componentId;
this.nodeIds = nodeIds;
this.linksBySourceNodeId = linksBySourceNodeId;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("componentId=" + componentId).append(", ")
.append("nodeIds=" + nodeIds).append(", ")
.append("linksBySourceNodeId=" + linksBySourceNodeId)
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("componentId")
public String getComponentId() {
return componentId;
}
@com.fasterxml.jackson.annotation.JsonGetter("nodeIds")
public List getNodeIds() {
return nodeIds;
}
@com.fasterxml.jackson.annotation.JsonGetter("linksBySourceNodeId")
public Map> getLinksBySourceNodeId() {
return linksBySourceNodeId;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy