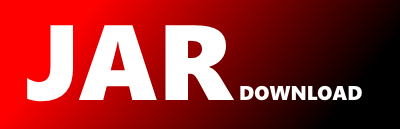
org.teamapps.dto.UiValidationRule Maven / Gradle / Ivy
package org.teamapps.dto;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Set;
import java.util.function.Supplier;
import java.util.stream.Collectors;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import com.fasterxml.jackson.annotation.JsonTypeInfo;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.databind.annotation.JsonTypeIdResolver;
/**
* THIS IS GENERATED CODE!
* PLEASE DO NOT MODIFY - ALL YOUR WORK WOULD BE LOST!
*/
@JsonTypeInfo(use = JsonTypeInfo.Id.CUSTOM, property = "_type")
@JsonTypeIdResolver(TeamAppsJacksonTypeIdResolver.class)
@JsonInclude(JsonInclude.Include.NON_NULL)
public class UiValidationRule extends UiMatcherRule implements UiObject {
public enum DisplayType {
POPUP_WINDOW, NOTIFICATION, INLINE;
@com.fasterxml.jackson.annotation.JsonValue
public int jsonValue() {
return ordinal();
}
}
protected DisplayType displayType = DisplayType.INLINE;
protected String errorMessage;
protected boolean markFieldsThatRuleMatches = true;
protected boolean checkOnFieldUpdate = true;
/**
* @deprecated Only for Jackson deserialization. Use the other constructor instead.
*/
@Deprecated
public UiValidationRule() {
// default constructor for Jackson
}
public UiValidationRule(List valueMatcher, String errorMessage) {
super(valueMatcher);
this.errorMessage = errorMessage;
}
@com.fasterxml.jackson.annotation.JsonIgnore
public UiObjectType getUiObjectType() {
return UiObjectType.UI_VALIDATION_RULE;
}
@SuppressWarnings("unchecked")
public String toString() {
return new StringBuilder(getClass().getSimpleName()).append(": ")
.append("executeRuleIfAnyMatcherMatches=" + executeRuleIfAnyMatcherMatches).append(", ")
.append("displayType=" + displayType).append(", ")
.append("errorMessage=" + errorMessage).append(", ")
.append("markFieldsThatRuleMatches=" + markFieldsThatRuleMatches).append(", ")
.append("checkOnFieldUpdate=" + checkOnFieldUpdate).append(", ")
.append(valueMatcher != null ? "valueMatcher={" + valueMatcher.toString() + "}" : "")
.toString();
}
@com.fasterxml.jackson.annotation.JsonGetter("displayType")
public DisplayType getDisplayType() {
return displayType;
}
@com.fasterxml.jackson.annotation.JsonGetter("errorMessage")
public String getErrorMessage() {
return errorMessage;
}
@com.fasterxml.jackson.annotation.JsonGetter("markFieldsThatRuleMatches")
public boolean getMarkFieldsThatRuleMatches() {
return markFieldsThatRuleMatches;
}
@com.fasterxml.jackson.annotation.JsonGetter("checkOnFieldUpdate")
public boolean getCheckOnFieldUpdate() {
return checkOnFieldUpdate;
}
@com.fasterxml.jackson.annotation.JsonSetter("executeRuleIfAnyMatcherMatches")
public UiValidationRule setExecuteRuleIfAnyMatcherMatches(boolean executeRuleIfAnyMatcherMatches) {
this.executeRuleIfAnyMatcherMatches = executeRuleIfAnyMatcherMatches;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("displayType")
public UiValidationRule setDisplayType(DisplayType displayType) {
this.displayType = displayType;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("markFieldsThatRuleMatches")
public UiValidationRule setMarkFieldsThatRuleMatches(boolean markFieldsThatRuleMatches) {
this.markFieldsThatRuleMatches = markFieldsThatRuleMatches;
return this;
}
@com.fasterxml.jackson.annotation.JsonSetter("checkOnFieldUpdate")
public UiValidationRule setCheckOnFieldUpdate(boolean checkOnFieldUpdate) {
this.checkOnFieldUpdate = checkOnFieldUpdate;
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy