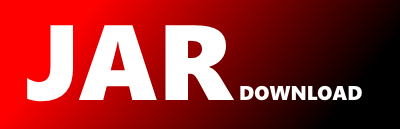
org.teasoft.bee.osql.api.MoreTable Maven / Gradle / Ivy
Show all versions of bee Show documentation
/*
* Copyright 2016-2023 the original author.All rights reserved.
* Kingstar([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.teasoft.bee.osql.api;
import java.util.List;
import org.teasoft.bee.osql.CommOperate;
/**
* More table select(multi-table query).
*
* Multi table Select/Update/Insert/Delete.
* @since 2.4.0
*
* In sharding mode, the main table and sub-table need map to same dataSource.
*
* example1:
public class Orders{
private Long id;
private String userid;
private String name;
private BigDecimal total;
private Timestamp createtime;
private String remark;
private String sequence;
//@JoinTable(mainField="userid", subField="username")
//@JoinTable(mainField="userid", subField="username", joinType=JoinType.LEFT_JOIN) //ok //... from orders left join user on orders.userid=user.username where ...
@JoinTable(mainField="userid,name", subField="username,name", joinType=JoinType.JOIN)
//@JoinTable(mainField="userid", subField="username",subAlias="myuser" , joinType=JoinType.FULL_JOIN)
//@JoinTable()
private User user;
// ... get,set methods.
}
public class User {
private Long id;
private String email;
private String lastName;
private String name;
private String password;
private String username;
private Timestamp createtime;
// ... get,set methods.
}
public class MoreTableExam {
public static void main(String[] args) {
MoreTable moreTable=BeeFactory.getHoneyFactory().getMoreTable();
Orders orders1=new Orders();
orders1.setUserid("bee");
orders1.setName("Bee(ORM Framework)");
User user=new User();
user.setEmail("[email protected]");
orders1.setUser(user);
List list1 =moreTable.select(orders1); //select
// List list1 =moreTable.select(orders1,0,10); //select,paging
//... process list1
}
}
--------------------------------
example2: List type sub entity field
@Entity("Clazz")
public class Clazz0 implements Serializable {
private static final long serialVersionUID = 1591972382398L;
private Integer id;
private String classname;
private String place;
private String teachername;
private String remark;
@JoinTable(mainField="id", subField="classno", joinType=JoinType.LEFT_JOIN,subClass="Student")
private List studentList=new ArrayList<>();
//subClass="Student", if sub Entity and main Entity in the same package, can use class name only.
//full like, subClass="org.teasoft.exam.bee.osql.entity.Student")
//... get,set method
}
public class Student implements Serializable {
private static final long serialVersionUID = 1591622324231L;
private Integer id;
private Integer sid;
private String name;
private Integer age;
private Boolean sex;
private String majorid;
private Boolean flag;
private Integer classno;
//... get,set method
}
public static void main(String[] args) {
MoreTable moreTable = BeeFactoryHelper.getMoreTable();
Clazz0 c0=new Clazz0();
List list0=moreTable.select(c0);
Printer.printList(list0); //print list
}
* @author Kingstar
* @since 1.7
*/
public interface MoreTable extends CommOperate {
/**
* According to entity object select records from database.
* @param entity table's entity(do not allow null).
* entity corresponding to table and can not be null.
* If the field value is not null and not empty string as filter condition,
* the operator is equal sign.eg:field=value
* @return list can contain more than one entity
*/
public List select(T entity);
/**
* According to entity object select records from database.
* @param entity table's entity(do not allow null).
* entity corresponding to table and can not be null.
* If the field value is not null and not empty string as filter condition,
* the operator is equal sign.eg:field=value
* @param start Start index,min value is 0 or 1(eg:MySQL is 0,Oracle is 1).
* @param size Fetch result size (>0).
* @return list can contain more than one entity
*/
public List select(T entity, int start, int size);
/**
* Select the records according to entity and condition.
* @param entity table's entity(do not allow null).
* @param condition If the field of entity is not null or empty,
*
it will be translate to field=value.Other can define with condition.
* @return list can contain more than one entity
*/
public List select(T entity, Condition condition);
/**
* set dynamic parameter for dynamic table & entity name
*
This method is called earlier than the select methods.
* @param para parameter name
* @param value parameter value
* @return MoreTable
* @since 1.9
*/
public MoreTable setDynamicParameter(String para, String value);
/**
* insert entity. support oneToOne/oneToMany.
* If the primary key of the main table is used for the foreign key of the sub table, it will be automatically filled in.
* The multiple tables modify is done by calling suidRich, so Interceptor use suidRich's.
* @param entity table's entity(do not allow null).
* @return returns the number of affected records in the main table.
* @since 2.1.8
*/
public int insert(T entity);
/**
* Update the main entity (main table) and the sub-entity (sub table) that meet the conditions.
* This method uses the primary key as the filtering condition to determine the entity,
* so the primary key field of the entity must not be empty.
* When all the foreign key corresponding fields are set in the main table, the sub table will be automatically
* updated in association.
* If not all the foreign key corresponding fields are set in the main table, the sub table will also be automatically
* updated in association if all the foreign key values are set in the sub table; otherwise, it will not be updated.
* The multiple tables modify is done by calling suidRich, so Interceptor use suidRich's.
* @param entity
* @return returns the number of affected records in the main table.
* @since 2.1.8
*/
public int update(T entity);
/**
* Delete the main entity (main table) and the sub-entity (sub table) that meet the conditions.
* When all the foreign key corresponding fields are set in the main table, the sub table will be automatically updated
* in association.
* If not all the foreign key corresponding fields are set in the main table, the sub table will also be automatically
* updated in association if all the foreign key values are set in the sub table; otherwise, it will not be updated.
* The multiple tables modify is done by calling suidRich, so Interceptor use suidRich's.
* @param entity
* @return returns the number of affected records in the main table.
* @since 2.1.8
*/
public int delete(T entity);
/**
* Select result with one function,just select one function.
* eg select max users id:
* String maxUsersId= moreTable.selectWithFun(new Users(),BF.getCondition().selectFun(FunctionType.MAX, "users.id"));
* @param entity table's entity(do not allow null).
* @param condition Condition instance.
*
here will ignore the condition's selectFun method.
* @return one function result.
*
If the result set of statistics is empty,the count return 0,the other return empty string.
* @since 2.4.0
*/
public String selectWithFun(T entity, Condition condition);
/**
* total number of statistical records.
* @param entity table's entity(do not allow null).
* @return total number of records that satisfy the condition.
* @since 2.4.0
*/
public int count(T entity);
/**
* total number of statistical records.
* @param entity table's entity(do not allow null).
* @param condition Condition as filter the record.
* @return total number of records that satisfy the condition.
* @since 2.4.0
*/
public int count(T entity, Condition condition);
/**
* Instead of returning data in a entity structure, it uses a character array in List.
* @param entity table's entity(do not allow null).
* @param condition Condition as filter the record.
* @return list can contain more than one record with String array struct.
* @since 2.4.0
*/
public List selectString(T entity, Condition condition);
/**
* Select and return data in Json format according to entity object.
* @param entity table's entity(do not allow null).
* @param condition Condition as filter the record.
* @return Json string, it transform from list which can contain more than one entity.
* @since 2.4.0
*/
public String selectJson(T entity, Condition condition);
}