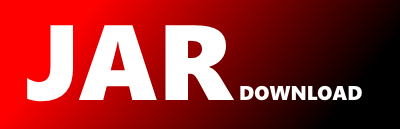
org.teatrove.teatools.MethodDescriptionBeanInfo Maven / Gradle / Ivy
The newest version!
/*
* MethodDescriptionBeanInfo.java
*
* BeanDoc generated on Fri Mar 16 at 12:39:55 EDT 02012.
*
* See MethodDescription.java for copyright information.
*/
package org.teatrove.teatools;
import java.beans.*;
import java.lang.reflect.*;
import java.util.Vector;
/**
* The BeanInfo class describing MethodDescription.
*
* @author Mark Masse
*/
public class MethodDescriptionBeanInfo extends SimpleBeanInfo {
/** BeanDescriptor returned by the getBeanDescriptor
method */
private BeanDescriptor mBeanDescriptor;
/** BeanInfo array returned by the getAdditionalBeanInfo
method */
private BeanInfo[] mAdditionalBeanInfo;
/** MethodDescriptor array returned by the
getMethodDescriptors
method */
private MethodDescriptor[] mMethodDescriptors;
/** PropertyDescriptor array returned by the
getPropertyDescriptors
method */
private PropertyDescriptor[] mPropertyDescriptors;
/** The index of the default property */
private int mDefaultPropertyIndex;
/**
* Creates a new MethodDescriptionBeanInfo.
*/
public MethodDescriptionBeanInfo() {
mDefaultPropertyIndex = -1;
}
/**
* Returns a BeanDescriptor that describes MethodDescription.
*/
public BeanDescriptor getBeanDescriptor() {
if (mBeanDescriptor == null) {
mBeanDescriptor = createBeanDescriptor();
}
return mBeanDescriptor;
}
/**
* Returns a BeanInfo array containing BeanInfo object's for:
* org.teatrove.teatools.FeatureDescription
*/
public BeanInfo[] getAdditionalBeanInfo() {
if (mAdditionalBeanInfo == null) {
mAdditionalBeanInfo = createAdditionalBeanInfo();
}
return mAdditionalBeanInfo;
}
/**
* Returns MethodDescriptors that describe the methods of
* MethodDescription. Call the
* getAdditionalBeanInfo
method to get the
* method descriptors of the superclass and interfaces.
*/
public MethodDescriptor[] getMethodDescriptors() {
if (mMethodDescriptors == null) {
mMethodDescriptors = createMethodDescriptors();
}
return mMethodDescriptors;
}
/**
* Returns a PropertyDescriptor array that describes the properties of
* MethodDescription.
*/
public PropertyDescriptor[] getPropertyDescriptors() {
if (mPropertyDescriptors == null) {
mPropertyDescriptors = createPropertyDescriptors();
}
return mPropertyDescriptors;
}
/**
* Return the index of the default property.
*/
public int getDefaultPropertyIndex() {
return mDefaultPropertyIndex;
}
//
// Non-public interface
//
// Create the bean descriptor for the bean.
private BeanDescriptor createBeanDescriptor() {
BeanDescriptor bd =
new BeanDescriptor(MethodDescription.class);
bd.setName("MethodDescription");
bd.setDisplayName("MethodDescription");
bd.setShortDescription("Wrapper for a MethodDescriptor object.");
bd.setValue("BeanDoc", "4.1.2");
return bd;
}
// Create the additional bean info objects for the bean.
private BeanInfo[] createAdditionalBeanInfo() {
Vector bis = new Vector();
BeanInfo bi = null;
bi = null;
try {
// Get the BeanInfo for the superclass
bi = Introspector.getBeanInfo(org.teatrove.teatools.FeatureDescription.class);
}
catch (Throwable t) {
t.printStackTrace();
}
if (bi != null) {
bis.addElement(bi);
}
BeanInfo[] additionalBeanInfo = null;
if (bis.size() > 0) {
additionalBeanInfo = new BeanInfo[bis.size()];
bis.copyInto(additionalBeanInfo);
}
return additionalBeanInfo;
}
// Create the method descriptor objects for the bean.
private MethodDescriptor[] createMethodDescriptors() {
Class[] paramTypes = null;
int paramIndex = 0;
ParameterDescriptor[] paramDescriptors = null;
ParameterDescriptor pd = null;
Vector mds = new Vector();
Method m = null;
MethodDescriptor md = null;
//
// getAcceptsSubstitution
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getAcceptsSubstitution", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getAcceptsSubstitution");
md.setDisplayName("getAcceptsSubstitution");
md.setShortDescription("Returns true if the specified method accepts a \n Substitution
as its last parameter.");
mds.addElement(md);
}
//
// getFeatureDescriptor
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getFeatureDescriptor", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getFeatureDescriptor");
md.setDisplayName("getFeatureDescriptor");
md.setShortDescription("Returns the FeatureDescriptor that is wrapped by this \n FeatureDescription");
mds.addElement(md);
}
//
// getLongFormat
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getLongFormat", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getLongFormat");
md.setDisplayName("getLongFormat");
md.setShortDescription("Returns a long format String for this FeatureDescription");
mds.addElement(md);
}
//
// getMethod
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getMethod", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getMethod");
md.setDisplayName("getMethod");
md.setShortDescription("Returns the Method");
mds.addElement(md);
}
//
// getMethodDescriptor
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getMethodDescriptor", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getMethodDescriptor");
md.setDisplayName("getMethodDescriptor");
md.setShortDescription("Returns the MethodDescriptor");
mds.addElement(md);
}
//
// getModifiers
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getModifiers", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getModifiers");
md.setDisplayName("getModifiers");
md.setShortDescription("Returns a Modifiers instance that can be used to check the type\'s\n modifiers.");
mds.addElement(md);
}
//
// getParameters
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getParameters", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getParameters");
md.setDisplayName("getParameters");
md.setShortDescription("Returns the method\'s parameters");
mds.addElement(md);
}
//
// getReturnType
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getReturnType", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getReturnType");
md.setDisplayName("getReturnType");
md.setShortDescription("Returns the method\'s return type");
mds.addElement(md);
}
//
// getShortFormat
//
paramTypes = new Class[0];
paramDescriptors = new ParameterDescriptor[0];
paramIndex = 0;
m = null;
try {
m = MethodDescription.class.getMethod("getShortFormat", paramTypes);
}
catch (Throwable t) {
t.printStackTrace();
}
if (m != null) {
md = null;
if (paramDescriptors != null) {
md = new MethodDescriptor(m, paramDescriptors);
}
else {
md = new MethodDescriptor(m);
}
md.setName("getShortFormat");
md.setDisplayName("getShortFormat");
md.setShortDescription("Returns a short format String for this FeatureDescription");
mds.addElement(md);
}
MethodDescriptor[] methodDescriptors =
new MethodDescriptor[mds.size()];
mds.copyInto(methodDescriptors);
return methodDescriptors;
}
// Create the property descriptor objects for the bean.
private PropertyDescriptor[] createPropertyDescriptors() {
PropertyDescriptor[] propertyDescriptors = new PropertyDescriptor[9];
PropertyDescriptor pd = null;
int propertyIndex = 0;
pd = null;
try {
pd = new PropertyDescriptor("acceptsSubstitution", MethodDescription.class, "getAcceptsSubstitution", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("acceptsSubstitution");
pd.setShortDescription("True if the specified method accepts a \n Substitution
as its last parameter.");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("featureDescriptor", MethodDescription.class, "getFeatureDescriptor", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("featureDescriptor");
pd.setShortDescription("");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("longFormat", MethodDescription.class, "getLongFormat", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("longFormat");
pd.setShortDescription("");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("method", MethodDescription.class, "getMethod", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("method");
pd.setShortDescription("The Method");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("methodDescriptor", MethodDescription.class, "getMethodDescriptor", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("methodDescriptor");
pd.setShortDescription("The MethodDescriptor");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("modifiers", MethodDescription.class, "getModifiers", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("modifiers");
pd.setShortDescription("A Modifiers instance that can be used to check the type\'s\n modifiers.");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("parameters", MethodDescription.class, "getParameters", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("parameters");
pd.setShortDescription("The method\'s parameters");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("returnType", MethodDescription.class, "getReturnType", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("returnType");
pd.setShortDescription("The method\'s return type");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
pd = null;
try {
pd = new PropertyDescriptor("shortFormat", MethodDescription.class, "getShortFormat", null);
}
catch (Throwable t) {
t.printStackTrace();
}
if (pd != null) {
pd.setDisplayName("shortFormat");
pd.setShortDescription("");
propertyDescriptors[propertyIndex] = pd;
propertyIndex++;
}
if (propertyDescriptors.length == 0) {
propertyDescriptors = null;
}
return propertyDescriptors;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy