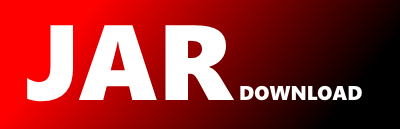
bdi.glue.http.common.HttpRequestBuilder Maven / Gradle / Ivy
package bdi.glue.http.common;
import java.util.ArrayList;
import java.util.List;
/**
* @author @aloyer
*/
public class HttpRequestBuilder {
private String methodAsString;
private String requestPath;
private final List headers = new ArrayList<>();
private final List cookies = new ArrayList<>();
private String body;
private String contentType;
private String username;
private String password;
private final List parameters = new ArrayList<>();
private final List cookiesToRemove = new ArrayList<>();
public HttpRequestBuilder method(String methodAsString) {
this.methodAsString = methodAsString;
return this;
}
public HttpRequestBuilder cookie(String cookieName, String cookieValue) {
this.cookies.add(new CookieImpl(cookieName, cookieValue));
return this;
}
public List getCookies() {
return cookies;
}
public HttpRequestBuilder header(Header header) {
this.headers.add(header);
return this;
}
public HttpRequestBuilder headers(Header... headers) {
for (Header header : headers) {
header(header);
}
return this;
}
public HttpRequestBuilder headers(Iterable headers) {
for (Header header : headers) {
header(header);
}
return this;
}
public String getMethodAsString() {
return methodAsString;
}
public HttpRequestBuilder requestPath(String requestPath) {
this.requestPath = requestPath;
return this;
}
public String getRequestPath() {
return requestPath;
}
public List getHeaders() {
return headers;
}
public HttpRequestBuilder body(String body) {
this.body = body;
return this;
}
public String getBody() {
return body;
}
public HttpRequestBuilder contentType(String contentType) {
this.contentType = contentType;
return this;
}
public String getContentType() {
return contentType;
}
public HttpRequestBuilder basicAuthCredentials(String username, String password) {
this.username = username;
this.password = password;
return this;
}
public String getUsername() {
return username;
}
public String getPassword() {
return password;
}
public HttpRequestBuilder parameters(List parameters) {
this.parameters.addAll(parameters);
return this;
}
public List getParameters() {
return parameters;
}
public void cookieToRemove(String cookieName) {
this.cookiesToRemove.add(cookieName);
}
public List getCookiesToRemove() {
return cookiesToRemove;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy