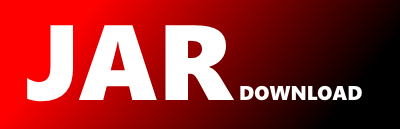
bdi.glue.jdbc.common.Rows Maven / Gradle / Ivy
package bdi.glue.jdbc.common;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
/**
* @author @aloyer
*/
public class Rows {
private Column[] columns;
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy