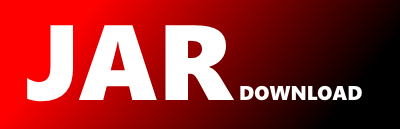
org.teiid.adminapi.AdminObject Maven / Gradle / Ivy
/*
* Copyright Red Hat, Inc. and/or its affiliates
* and other contributors as indicated by the @author tags and
* the COPYRIGHT.txt file distributed with this work.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.teiid.adminapi;
import java.io.Serializable;
import java.util.Properties;
/**
* Base interface of client side admin objects. Specifies behaviors and properties common to all administrative objects.
*
* Unique identifiers are available for all AdminObject
s and their forms are specific to each object. See
* the javadoc on the individual object for its particular identifier pattern required.
*
*
* This interface need not be used directly by clients except when coding to constants.
*
*
* @since 4.3
*/
public interface AdminObject extends Serializable {
/**
* The character that delimits the atomic components of the identifier.
* @see #DELIMITER
*/
public static final char DELIMITER_CHAR = '|';
/**
* The character (as a String
) that delimits the atomic components of the identifier.
*
* It is strongly advisable to write admin client code using this DELIMITER
* rather than hard-coding a delimiter character in admin code. Doing this eliminates the possibility
* of admin client code breaking if/when the delimiter character must be changed.
*/
public static final String DELIMITER = new String(new char[] {DELIMITER_CHAR});
/**
* The delimiter character as a String
escaped.
* @see #DELIMITER
*/
public static final String ESCAPED_DELIMITER = "\\" + DELIMITER; //$NON-NLS-1$
/**
* The wildcard character (as a String
) that can be used in may identifier patterns
* to indicate "anything" or, more specifically, replace "zero or more"
* identifier components.
*
* It is strongly advisable to write admin client code using this WILDCARD
* rather than hard-coding a wildcard character in admin code. Doing this eliminates the possibility
* of admin client code breaking if/when the wildcard character must be changed.
*/
public static final String WILDCARD = "*"; //$NON-NLS-1$
/**
* The wildcard character as a String
escaped.
* @see #WILDCARD
*/
public static final String ESCAPED_WILDCARD = "\\" + WILDCARD; //$NON-NLS-1$
/**
* Get the name for this AdminObject, usually the last component of the identifier.
*
* @return String Name
* @since 4.3
*/
String getName();
/**
* Get the Configuration Properties that defines this process
*
* @return Properties
* @since 4.3
*/
Properties getProperties();
/**
* Searches for the property with the specified key in this Admin Object. If the key is not found the method returns
* null
.
*
* @param name
* the property key.
* @return the value in this Admin Object with the specified key value.
* @since 4.3
*/
String getPropertyValue(String name);
}