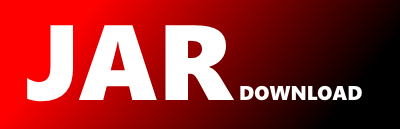
org.telegram.api.updates.difference.TLAbsDifference Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of telegramapi Show documentation
Show all versions of telegramapi Show documentation
Java library to create Telegram Clients
package org.telegram.api.updates.difference;
import org.telegram.api.chat.TLAbsChat;
import org.telegram.api.encrypted.message.TLAbsEncryptedMessage;
import org.telegram.api.message.TLAbsMessage;
import org.telegram.api.update.TLAbsUpdate;
import org.telegram.api.user.TLAbsUser;
import org.telegram.tl.TLObject;
import org.telegram.tl.TLVector;
/**
* The type TL abs difference.
*/
public abstract class TLAbsDifference extends TLObject {
/**
* The Date.
*/
protected int date;
/**
* The Seq.
*/
protected int seq;
/**
* The New messages.
*/
protected TLVector newMessages = new TLVector<>();
/**
* The New encrypted messages.
*/
protected TLVector newEncryptedMessages = new TLVector<>();
/**
* The Other updates.
*/
protected TLVector otherUpdates = new TLVector<>();
/**
* The Chats.
*/
protected TLVector chats = new TLVector<>();
/**
* The Users.
*/
protected TLVector users = new TLVector<>();
/**
* Instantiates a new TL abs difference.
*/
protected TLAbsDifference() {
super();
}
/**
* Gets date.
*
* @return the date
*/
public int getDate() {
return this.date;
}
/**
* Sets date.
*
* @param date the date
*/
public void setDate(int date) {
this.date = date;
}
/**
* Gets seq.
*
* @return the seq
*/
public int getSeq() {
return this.seq;
}
/**
* Sets seq.
*
* @param seq the seq
*/
public void setSeq(int seq) {
this.seq = seq;
}
/**
* Gets new messages.
*
* @return the new messages
*/
public TLVector getNewMessages() {
return this.newMessages;
}
/**
* Sets new messages.
*
* @param newMessages the new messages
*/
public void setNewMessages(TLVector newMessages) {
this.newMessages = newMessages;
}
/**
* Gets new encrypted messages.
*
* @return the new encrypted messages
*/
public TLVector getNewEncryptedMessages() {
return this.newEncryptedMessages;
}
/**
* Sets new encrypted messages.
*
* @param newEncryptedMessages the new encrypted messages
*/
public void setNewEncryptedMessages(TLVector newEncryptedMessages) {
this.newEncryptedMessages = newEncryptedMessages;
}
/**
* Gets other updates.
*
* @return the other updates
*/
public TLVector getOtherUpdates() {
return this.otherUpdates;
}
/**
* Sets other updates.
*
* @param otherUpdates the other updates
*/
public void setOtherUpdates(TLVector otherUpdates) {
this.otherUpdates = otherUpdates;
}
/**
* Gets chats.
*
* @return the chats
*/
public TLVector getChats() {
return this.chats;
}
/**
* Sets chats.
*
* @param chats the chats
*/
public void setChats(TLVector chats) {
this.chats = chats;
}
/**
* Gets users.
*
* @return the users
*/
public TLVector getUsers() {
return this.users;
}
/**
* Sets users.
*
* @param users the users
*/
public void setUsers(TLVector users) {
this.users = users;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy