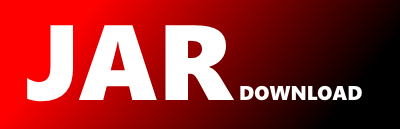
org.telegram.telegrambots.meta.generics.TelegramClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of telegrambots-meta Show documentation
Show all versions of telegrambots-meta Show documentation
Easy to use library to create Telegram Bots
The newest version!
package org.telegram.telegrambots.meta.generics;
import org.telegram.telegrambots.meta.api.methods.botapimethods.BotApiMethod;
import org.telegram.telegrambots.meta.api.methods.groupadministration.SetChatPhoto;
import org.telegram.telegrambots.meta.api.methods.send.SendAnimation;
import org.telegram.telegrambots.meta.api.methods.send.SendAudio;
import org.telegram.telegrambots.meta.api.methods.send.SendDocument;
import org.telegram.telegrambots.meta.api.methods.send.SendMediaGroup;
import org.telegram.telegrambots.meta.api.methods.send.SendPaidMedia;
import org.telegram.telegrambots.meta.api.methods.send.SendPhoto;
import org.telegram.telegrambots.meta.api.methods.send.SendSticker;
import org.telegram.telegrambots.meta.api.methods.send.SendVideo;
import org.telegram.telegrambots.meta.api.methods.send.SendVideoNote;
import org.telegram.telegrambots.meta.api.methods.send.SendVoice;
import org.telegram.telegrambots.meta.api.methods.stickers.AddStickerToSet;
import org.telegram.telegrambots.meta.api.methods.stickers.CreateNewStickerSet;
import org.telegram.telegrambots.meta.api.methods.stickers.ReplaceStickerInSet;
import org.telegram.telegrambots.meta.api.methods.stickers.SetStickerSetThumbnail;
import org.telegram.telegrambots.meta.api.methods.stickers.UploadStickerFile;
import org.telegram.telegrambots.meta.api.methods.updates.SetWebhook;
import org.telegram.telegrambots.meta.api.methods.updatingmessages.EditMessageMedia;
import org.telegram.telegrambots.meta.api.objects.File;
import org.telegram.telegrambots.meta.api.objects.message.Message;
import org.telegram.telegrambots.meta.exceptions.TelegramApiException;
import java.io.InputStream;
import java.io.Serializable;
import java.util.List;
import java.util.concurrent.CompletableFuture;
public interface TelegramClient {
> CompletableFuture executeAsync(Method method) throws TelegramApiException;
> T execute(Method method) throws TelegramApiException;
// Specific Send Requests
Message execute(SendDocument sendDocument) throws TelegramApiException;
Message execute(SendPhoto sendPhoto) throws TelegramApiException;
Boolean execute(SetWebhook setWebhook) throws TelegramApiException;
Message execute(SendVideo sendVideo) throws TelegramApiException;
Message execute(SendVideoNote sendVideoNote) throws TelegramApiException;
Message execute(SendSticker sendSticker) throws TelegramApiException;
/**
* Sends a file using Send Audio method
* @param sendAudio Information to send
* @return If success, the sent Message is returned
* @throws TelegramApiException If there is any error sending the audio
* @see https://core.telegram.org/bots/api#sendaudio)
*/
Message execute(SendAudio sendAudio) throws TelegramApiException;
/**
* Sends a voice note using Send Voice method
* For this to work, your audio must be in an .ogg file encoded with OPUS
* @param sendVoice Information to send
* @return If success, the sent Message is returned
* @throws TelegramApiException If there is any error sending the audio
* @see https://core.telegram.org/bots/api#sendvoice)
*/
Message execute(SendVoice sendVoice) throws TelegramApiException;
/**
* Send a media group
* @return If success, list of generated messages
* @throws TelegramApiException If there is any error sending the media group
* @see https://core.telegram.org/bots/api#sendMediaGroup
*/
List execute(SendMediaGroup sendMediaGroup) throws TelegramApiException;
/**
* Send a paid media
* @return If success, list of generated messages
* @throws TelegramApiException If there is any error sending the media group
* @see https://core.telegram.org/bots/api#sendMediaGroup
*/
List execute(SendPaidMedia sendPaidMedia) throws TelegramApiException;
/**
* Set chat profile photo
* @param setChatPhoto Information to set the photo
* @return If success, true is returned
* @throws TelegramApiException If there is any error setting the photo.
* @see https://core.telegram.org/bots/api#setChatPhoto
*/
Boolean execute(SetChatPhoto setChatPhoto) throws TelegramApiException;
/**
* Adds a new sticker to a set
* @param addStickerToSet Information of the sticker to set
* @return If success, true is returned
* @throws TelegramApiException If there is any error adding the sticker to the set
* @see https://core.telegram.org/bots/api#addStickerToSet
*/
Boolean execute(AddStickerToSet addStickerToSet) throws TelegramApiException;
/**
* Replace a sticker in a set
* @param replaceStickerInSet Information of the sticker to set
* @return If success, true is returned
* @throws TelegramApiException If there is any error adding the sticker to the set
* @see https://core.telegram.org/bots/api#replaceStickerInSet
*/
Boolean execute(ReplaceStickerInSet replaceStickerInSet) throws TelegramApiException;
/**
* Set sticker set thumb
* @param setStickerSetThumbnail Information of the sticker to set
* @return If success, true is returned
* @throws TelegramApiException If there is any error setting the thumb to the set
* @see https://core.telegram.org/bots/api#setStickerSetThumbail
*/
Boolean execute(SetStickerSetThumbnail setStickerSetThumbnail) throws TelegramApiException;
/**
* Creates a new sticker set ≈
* @param createNewStickerSet Information of the sticker set to create
* @return If success, true is returned
* @throws TelegramApiException If there is any error creating the new sticker set
* @see https://core.telegram.org/bots/api#setStickerSetThumbail
*/
Boolean execute(CreateNewStickerSet createNewStickerSet) throws TelegramApiException;
/**
* Upload a new file as sticker
* @param uploadStickerFile Information of the file to upload as sticker
* @return If success, true is returned
* @throws TelegramApiException If there is any error uploading the new file
* @see https://core.telegram.org/bots/api#uploadStickerFile
*/
File execute(UploadStickerFile uploadStickerFile) throws TelegramApiException;
/**
* Edit media in a message
* @param editMessageMedia Information of the new media
* @return If the edited message is not an inline message, the edited Message is returned, otherwise True is returned
* @throws TelegramApiException If there is any error editing the media
*/
Serializable execute(EditMessageMedia editMessageMedia) throws TelegramApiException;
java.io.File downloadFile(File file) throws TelegramApiException;
@SuppressWarnings("unused")
default java.io.File downloadFile(String filePath) throws TelegramApiException {
return downloadFile(new File(null, null, null, filePath));
}
InputStream downloadFileAsStream(File file) throws TelegramApiException;
@SuppressWarnings("unused")
default InputStream downloadFileAsStream(String filePath) throws TelegramApiException {
return downloadFileAsStream(new File(null, null, null, filePath));
}
/**
* Send animation
* @param sendAnimation Information of the animation
* @return Sent message
* @throws TelegramApiException If there is any error sending animation
*/
Message execute(SendAnimation sendAnimation) throws TelegramApiException;
CompletableFuture executeAsync(SendDocument sendDocument);
CompletableFuture executeAsync(SendPhoto sendPhoto);
CompletableFuture executeAsync(SetWebhook setWebhook);
CompletableFuture executeAsync(SendVideo sendVideo);
CompletableFuture executeAsync(SendVideoNote sendVideoNote);
CompletableFuture executeAsync(SendSticker sendSticker);
/**
* Sends a file using the Send Audio method
* @param sendAudio Information to send
* @return If success, the sent Message is returned
*/
CompletableFuture executeAsync(SendAudio sendAudio);
/**
* Sends a voice note using the Send Voice method
* For this to work, your audio must be in an .ogg file encoded with OPUS
* @param sendVoice Information to send
* @return If success, the sent Message is returned
*/
CompletableFuture executeAsync(SendVoice sendVoice);
/**
* Send a media group
* @return If success, list of generated messages
* @see https://core.telegram.org/bots/api#sendMediaGroup
*/
CompletableFuture> executeAsync(SendMediaGroup sendMediaGroup);
/**
* Send a paid media
* @return If success, list of generated messages
* @see https://core.telegram.org/bots/api#sendMediaGroup
*/
CompletableFuture> executeAsync(SendPaidMedia sendPaidMedia);
/**
* Set chat profile photo
* @param setChatPhoto Information to set the photo
* @return If success, true is returned
* @see https://core.telegram.org/bots/api#setChatPhoto
*/
CompletableFuture executeAsync(SetChatPhoto setChatPhoto);
/**
* Adds a new sticker to a set
* @param addStickerToSet Information of the sticker to set
* @return If success, true is returned
* @see https://core.telegram.org/bots/api#addStickerToSet
*/
CompletableFuture executeAsync(AddStickerToSet addStickerToSet);
/**
* Replace a sticker in a set
* @param replaceStickerInSet Information of the sticker to set
* @return If success, true is returned
* @see https://core.telegram.org/bots/api#replaceStickerInSet
*/
CompletableFuture executeAsync(ReplaceStickerInSet replaceStickerInSet);
/**
* Set sticker set thumb
* @param setStickerSetThumbnail Information of the sticker to set
* @return If success, true is returned
* @see https://core.telegram.org/bots/api#setStickerSetThumbail
*/
CompletableFuture executeAsync(SetStickerSetThumbnail setStickerSetThumbnail);
/**
* Creates a new sticker set
* @param createNewStickerSet Information of the sticker set to create
* @return If success, true is returned
* @see https://core.telegram.org/bots/api#createNewStickerSet
*/
CompletableFuture executeAsync(CreateNewStickerSet createNewStickerSet);
/**
* Upload a new file as sticker
* @param uploadStickerFile Information of the file to upload as sticker
* @return If success, true is returned
* @see https://core.telegram.org/bots/api#uploadStickerFile
*/
CompletableFuture executeAsync(UploadStickerFile uploadStickerFile);
/**
* Edit media in a message
* @param editMessageMedia Information of the new media
* @return If the edited message is not an inline message, the edited Message is returned, otherwise True is returned
*/
CompletableFuture executeAsync(EditMessageMedia editMessageMedia);
/**
* Send animation
* @param sendAnimation Information of the animation
* @return Sent message
*/
CompletableFuture executeAsync(SendAnimation sendAnimation);
CompletableFuture downloadFileAsync(File file);
@SuppressWarnings("unused")
default CompletableFuture downloadFileAsync(String filePath) {
return downloadFileAsync(new File(null, null, null, filePath));
}
CompletableFuture downloadFileAsStreamAsync(File file);
@SuppressWarnings("unused")
default CompletableFuture downloadFileAsStreamAsync(String filePath) {
return downloadFileAsStreamAsync(new File(null, null, null, filePath));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy