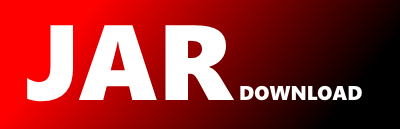
org.tensorflow.op.BitwiseOps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libtensorflow Show documentation
Show all versions of libtensorflow Show documentation
Pure-Java code for the TensorFlow machine intelligence library.
The newest version!
package org.tensorflow.op;
import org.tensorflow.Operand;
import org.tensorflow.op.bitwise.BitwiseAnd;
import org.tensorflow.op.bitwise.BitwiseOr;
import org.tensorflow.op.bitwise.BitwiseXor;
import org.tensorflow.op.bitwise.Invert;
import org.tensorflow.op.bitwise.LeftShift;
import org.tensorflow.op.bitwise.RightShift;
/**
* An API for building {@code bitwise} operations as {@link Op Op}s
*
* @see {@link Ops}
*/
public final class BitwiseOps {
private final Scope scope;
BitwiseOps(Scope scope) {
this.scope = scope;
}
/**
* Builds an {@link Invert} operation
*
* @param x
* @return a new instance of Invert
* @see org.tensorflow.op.bitwise.Invert
*/
public Invert invert(Operand x) {
return Invert.create(scope, x);
}
/**
* Builds an {@link LeftShift} operation
*
* @param x
* @param y
* @return a new instance of LeftShift
* @see org.tensorflow.op.bitwise.LeftShift
*/
public LeftShift leftShift(Operand x, Operand y) {
return LeftShift.create(scope, x, y);
}
/**
* Builds an {@link BitwiseAnd} operation
*
* @param x
* @param y
* @return a new instance of BitwiseAnd
* @see org.tensorflow.op.bitwise.BitwiseAnd
*/
public BitwiseAnd bitwiseAnd(Operand x, Operand y) {
return BitwiseAnd.create(scope, x, y);
}
/**
* Builds an {@link BitwiseXor} operation
*
* @param x
* @param y
* @return a new instance of BitwiseXor
* @see org.tensorflow.op.bitwise.BitwiseXor
*/
public BitwiseXor bitwiseXor(Operand x, Operand y) {
return BitwiseXor.create(scope, x, y);
}
/**
* Builds an {@link BitwiseOr} operation
*
* @param x
* @param y
* @return a new instance of BitwiseOr
* @see org.tensorflow.op.bitwise.BitwiseOr
*/
public BitwiseOr bitwiseOr(Operand x, Operand y) {
return BitwiseOr.create(scope, x, y);
}
/**
* Builds an {@link RightShift} operation
*
* @param x
* @param y
* @return a new instance of RightShift
* @see org.tensorflow.op.bitwise.RightShift
*/
public RightShift rightShift(Operand x, Operand y) {
return RightShift.create(scope, x, y);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy