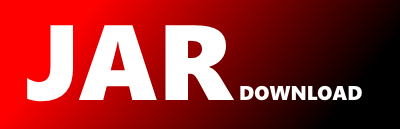
org.tensorflow.op.Ops Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of libtensorflow Show documentation
Show all versions of libtensorflow Show documentation
Pure-Java code for the TensorFlow machine intelligence library.
The newest version!
package org.tensorflow.op;
import java.nio.ByteBuffer;
import java.nio.DoubleBuffer;
import java.nio.FloatBuffer;
import java.nio.IntBuffer;
import java.nio.LongBuffer;
import java.nio.charset.Charset;
import java.util.List;
import org.tensorflow.EagerSession;
import org.tensorflow.ExecutionEnvironment;
import org.tensorflow.Operand;
import org.tensorflow.Shape;
import org.tensorflow.op.core.Abort;
import org.tensorflow.op.core.All;
import org.tensorflow.op.core.Any;
import org.tensorflow.op.core.AssertThat;
import org.tensorflow.op.core.Assign;
import org.tensorflow.op.core.AssignAdd;
import org.tensorflow.op.core.AssignAddVariableOp;
import org.tensorflow.op.core.AssignSub;
import org.tensorflow.op.core.AssignSubVariableOp;
import org.tensorflow.op.core.AssignVariableOp;
import org.tensorflow.op.core.Barrier;
import org.tensorflow.op.core.BarrierClose;
import org.tensorflow.op.core.BarrierIncompleteSize;
import org.tensorflow.op.core.BarrierInsertMany;
import org.tensorflow.op.core.BarrierReadySize;
import org.tensorflow.op.core.BarrierTakeMany;
import org.tensorflow.op.core.Batch;
import org.tensorflow.op.core.BatchMatMulV2;
import org.tensorflow.op.core.BatchToSpace;
import org.tensorflow.op.core.BatchToSpaceNd;
import org.tensorflow.op.core.Bitcast;
import org.tensorflow.op.core.BroadcastDynamicShape;
import org.tensorflow.op.core.BroadcastTo;
import org.tensorflow.op.core.Bucketize;
import org.tensorflow.op.core.ClipByValue;
import org.tensorflow.op.core.CombinedNonMaxSuppression;
import org.tensorflow.op.core.Concat;
import org.tensorflow.op.core.Constant;
import org.tensorflow.op.core.ConsumeMutexLock;
import org.tensorflow.op.core.ControlTrigger;
import org.tensorflow.op.core.CountUpTo;
import org.tensorflow.op.core.CudnnRNNCanonicalToParamsV2;
import org.tensorflow.op.core.CudnnRNNParamsToCanonicalV2;
import org.tensorflow.op.core.DecodePaddedRaw;
import org.tensorflow.op.core.DeepCopy;
import org.tensorflow.op.core.DeleteSessionTensor;
import org.tensorflow.op.core.DestroyResourceOp;
import org.tensorflow.op.core.DestroyTemporaryVariable;
import org.tensorflow.op.core.DrawBoundingBoxesV2;
import org.tensorflow.op.core.DynamicPartition;
import org.tensorflow.op.core.DynamicStitch;
import org.tensorflow.op.core.EditDistance;
import org.tensorflow.op.core.Einsum;
import org.tensorflow.op.core.Empty;
import org.tensorflow.op.core.EmptyTensorList;
import org.tensorflow.op.core.EnsureShape;
import org.tensorflow.op.core.EuclideanNorm;
import org.tensorflow.op.core.ExpandDims;
import org.tensorflow.op.core.ExtractVolumePatches;
import org.tensorflow.op.core.Fill;
import org.tensorflow.op.core.Fingerprint;
import org.tensorflow.op.core.FusedBatchNormGradV3;
import org.tensorflow.op.core.FusedBatchNormV3;
import org.tensorflow.op.core.Gather;
import org.tensorflow.op.core.GatherNd;
import org.tensorflow.op.core.GcsConfigureBlockCache;
import org.tensorflow.op.core.GcsConfigureCredentials;
import org.tensorflow.op.core.GenerateBigQueryReaderPartitions;
import org.tensorflow.op.core.GetSessionHandle;
import org.tensorflow.op.core.GetSessionTensor;
import org.tensorflow.op.core.Gradients;
import org.tensorflow.op.core.GuaranteeConst;
import org.tensorflow.op.core.HashTable;
import org.tensorflow.op.core.HistogramFixedWidth;
import org.tensorflow.op.core.Identity;
import org.tensorflow.op.core.IdentityN;
import org.tensorflow.op.core.ImmutableConst;
import org.tensorflow.op.core.InitializeTable;
import org.tensorflow.op.core.InitializeTableFromTextFile;
import org.tensorflow.op.core.InplaceAdd;
import org.tensorflow.op.core.InplaceSub;
import org.tensorflow.op.core.InplaceUpdate;
import org.tensorflow.op.core.IsVariableInitialized;
import org.tensorflow.op.core.LinSpace;
import org.tensorflow.op.core.LookupTableExport;
import org.tensorflow.op.core.LookupTableFind;
import org.tensorflow.op.core.LookupTableImport;
import org.tensorflow.op.core.LookupTableInsert;
import org.tensorflow.op.core.LookupTableSize;
import org.tensorflow.op.core.LoopCond;
import org.tensorflow.op.core.Lu;
import org.tensorflow.op.core.MapClear;
import org.tensorflow.op.core.MapIncompleteSize;
import org.tensorflow.op.core.MapPeek;
import org.tensorflow.op.core.MapSize;
import org.tensorflow.op.core.MapStage;
import org.tensorflow.op.core.MapUnstage;
import org.tensorflow.op.core.MapUnstageNoKey;
import org.tensorflow.op.core.MatrixDiagPartV2;
import org.tensorflow.op.core.MatrixDiagV2;
import org.tensorflow.op.core.MatrixSetDiagV2;
import org.tensorflow.op.core.Max;
import org.tensorflow.op.core.Merge;
import org.tensorflow.op.core.Min;
import org.tensorflow.op.core.MirrorPad;
import org.tensorflow.op.core.MulNoNan;
import org.tensorflow.op.core.MutableDenseHashTable;
import org.tensorflow.op.core.MutableHashTable;
import org.tensorflow.op.core.MutableHashTableOfTensors;
import org.tensorflow.op.core.Mutex;
import org.tensorflow.op.core.MutexLock;
import org.tensorflow.op.core.NextAfter;
import org.tensorflow.op.core.NextIteration;
import org.tensorflow.op.core.NoOp;
import org.tensorflow.op.core.NonMaxSuppressionV5;
import org.tensorflow.op.core.OneHot;
import org.tensorflow.op.core.OnesLike;
import org.tensorflow.op.core.OrderedMapClear;
import org.tensorflow.op.core.OrderedMapIncompleteSize;
import org.tensorflow.op.core.OrderedMapPeek;
import org.tensorflow.op.core.OrderedMapSize;
import org.tensorflow.op.core.OrderedMapStage;
import org.tensorflow.op.core.OrderedMapUnstage;
import org.tensorflow.op.core.OrderedMapUnstageNoKey;
import org.tensorflow.op.core.Pad;
import org.tensorflow.op.core.ParallelConcat;
import org.tensorflow.op.core.ParallelDynamicStitch;
import org.tensorflow.op.core.Placeholder;
import org.tensorflow.op.core.PlaceholderWithDefault;
import org.tensorflow.op.core.Print;
import org.tensorflow.op.core.Prod;
import org.tensorflow.op.core.QuantizedConcat;
import org.tensorflow.op.core.QuantizedReshape;
import org.tensorflow.op.core.Range;
import org.tensorflow.op.core.Rank;
import org.tensorflow.op.core.ReadVariableOp;
import org.tensorflow.op.core.ReduceAll;
import org.tensorflow.op.core.ReduceAny;
import org.tensorflow.op.core.ReduceMax;
import org.tensorflow.op.core.ReduceMin;
import org.tensorflow.op.core.ReduceProd;
import org.tensorflow.op.core.ReduceSum;
import org.tensorflow.op.core.RefNextIteration;
import org.tensorflow.op.core.RefSelect;
import org.tensorflow.op.core.RefSwitch;
import org.tensorflow.op.core.RemoteFusedGraphExecute;
import org.tensorflow.op.core.Reshape;
import org.tensorflow.op.core.ResourceApplyAdamWithAmsgrad;
import org.tensorflow.op.core.ResourceApplyKerasMomentum;
import org.tensorflow.op.core.ResourceCountUpTo;
import org.tensorflow.op.core.ResourceGather;
import org.tensorflow.op.core.ResourceGatherNd;
import org.tensorflow.op.core.ResourceScatterAdd;
import org.tensorflow.op.core.ResourceScatterDiv;
import org.tensorflow.op.core.ResourceScatterMax;
import org.tensorflow.op.core.ResourceScatterMin;
import org.tensorflow.op.core.ResourceScatterMul;
import org.tensorflow.op.core.ResourceScatterNdAdd;
import org.tensorflow.op.core.ResourceScatterNdSub;
import org.tensorflow.op.core.ResourceScatterNdUpdate;
import org.tensorflow.op.core.ResourceScatterSub;
import org.tensorflow.op.core.ResourceScatterUpdate;
import org.tensorflow.op.core.ResourceSparseApplyKerasMomentum;
import org.tensorflow.op.core.ResourceStridedSliceAssign;
import org.tensorflow.op.core.Reverse;
import org.tensorflow.op.core.ReverseSequence;
import org.tensorflow.op.core.Roll;
import org.tensorflow.op.core.Rpc;
import org.tensorflow.op.core.ScaleAndTranslate;
import org.tensorflow.op.core.ScatterAdd;
import org.tensorflow.op.core.ScatterDiv;
import org.tensorflow.op.core.ScatterMax;
import org.tensorflow.op.core.ScatterMin;
import org.tensorflow.op.core.ScatterMul;
import org.tensorflow.op.core.ScatterNd;
import org.tensorflow.op.core.ScatterNdAdd;
import org.tensorflow.op.core.ScatterNdNonAliasingAdd;
import org.tensorflow.op.core.ScatterNdSub;
import org.tensorflow.op.core.ScatterNdUpdate;
import org.tensorflow.op.core.ScatterSub;
import org.tensorflow.op.core.ScatterUpdate;
import org.tensorflow.op.core.SelectV2;
import org.tensorflow.op.core.SetDiff1d;
import org.tensorflow.op.core.SetSize;
import org.tensorflow.op.core.ShapeN;
import org.tensorflow.op.core.Size;
import org.tensorflow.op.core.Skipgram;
import org.tensorflow.op.core.Slice;
import org.tensorflow.op.core.Snapshot;
import org.tensorflow.op.core.SpaceToBatchNd;
import org.tensorflow.op.core.Split;
import org.tensorflow.op.core.SplitV;
import org.tensorflow.op.core.Squeeze;
import org.tensorflow.op.core.Stack;
import org.tensorflow.op.core.Stage;
import org.tensorflow.op.core.StageClear;
import org.tensorflow.op.core.StagePeek;
import org.tensorflow.op.core.StageSize;
import org.tensorflow.op.core.StatefulRandomBinomial;
import org.tensorflow.op.core.StatefulStandardNormal;
import org.tensorflow.op.core.StatefulStandardNormalV2;
import org.tensorflow.op.core.StopGradient;
import org.tensorflow.op.core.StridedSlice;
import org.tensorflow.op.core.StridedSliceAssign;
import org.tensorflow.op.core.StridedSliceGrad;
import org.tensorflow.op.core.StringLower;
import org.tensorflow.op.core.StringNGrams;
import org.tensorflow.op.core.StringUpper;
import org.tensorflow.op.core.Sum;
import org.tensorflow.op.core.SwitchCond;
import org.tensorflow.op.core.TemporaryVariable;
import org.tensorflow.op.core.TensorArray;
import org.tensorflow.op.core.TensorArrayClose;
import org.tensorflow.op.core.TensorArrayConcat;
import org.tensorflow.op.core.TensorArrayGather;
import org.tensorflow.op.core.TensorArrayGrad;
import org.tensorflow.op.core.TensorArrayGradWithShape;
import org.tensorflow.op.core.TensorArrayPack;
import org.tensorflow.op.core.TensorArrayRead;
import org.tensorflow.op.core.TensorArrayScatter;
import org.tensorflow.op.core.TensorArraySize;
import org.tensorflow.op.core.TensorArraySplit;
import org.tensorflow.op.core.TensorArrayUnpack;
import org.tensorflow.op.core.TensorArrayWrite;
import org.tensorflow.op.core.TensorListConcat;
import org.tensorflow.op.core.TensorListConcatLists;
import org.tensorflow.op.core.TensorListConcatV2;
import org.tensorflow.op.core.TensorListElementShape;
import org.tensorflow.op.core.TensorListFromTensor;
import org.tensorflow.op.core.TensorListGather;
import org.tensorflow.op.core.TensorListGetItem;
import org.tensorflow.op.core.TensorListLength;
import org.tensorflow.op.core.TensorListPopBack;
import org.tensorflow.op.core.TensorListPushBack;
import org.tensorflow.op.core.TensorListPushBackBatch;
import org.tensorflow.op.core.TensorListReserve;
import org.tensorflow.op.core.TensorListResize;
import org.tensorflow.op.core.TensorListScatter;
import org.tensorflow.op.core.TensorListScatterIntoExistingList;
import org.tensorflow.op.core.TensorListScatterV2;
import org.tensorflow.op.core.TensorListSetItem;
import org.tensorflow.op.core.TensorListSplit;
import org.tensorflow.op.core.TensorListStack;
import org.tensorflow.op.core.TensorScatterAdd;
import org.tensorflow.op.core.TensorScatterSub;
import org.tensorflow.op.core.TensorScatterUpdate;
import org.tensorflow.op.core.TensorStridedSliceUpdate;
import org.tensorflow.op.core.Tile;
import org.tensorflow.op.core.Timestamp;
import org.tensorflow.op.core.TryRpc;
import org.tensorflow.op.core.Unbatch;
import org.tensorflow.op.core.UnbatchGrad;
import org.tensorflow.op.core.Unique;
import org.tensorflow.op.core.UniqueWithCounts;
import org.tensorflow.op.core.UnravelIndex;
import org.tensorflow.op.core.UnsortedSegmentJoin;
import org.tensorflow.op.core.Unstack;
import org.tensorflow.op.core.Unstage;
import org.tensorflow.op.core.VarHandleOp;
import org.tensorflow.op.core.VarIsInitializedOp;
import org.tensorflow.op.core.Variable;
import org.tensorflow.op.core.VariableShape;
import org.tensorflow.op.core.Where;
import org.tensorflow.op.core.Where3;
import org.tensorflow.op.core.Zeros;
import org.tensorflow.op.core.ZerosLike;
/**
* An API for building operations as {@link Op Op}s
*
* Any operation wrapper found in the classpath properly annotated as an{@link org.tensorflow.op.annotation.Operator @Operator} is exposed
* by this API or one of its subgroup.
*
Example usage:
*
{@code
* try (Graph g = new Graph()) {
* Ops ops = Ops.create(g);
* // Operations are typed classes with convenience
* // builders in Ops.
* Constant three = ops.constant(3);
* // Single-result operations implement the Operand
* // interface, so this works too.
* Operand four = ops.constant(4);
* // Most builders are found within a group, and accept
* // Operand types as operands
* Operand nine = ops.math.add(four, ops.constant(5));
* // Multi-result operations however offer methods to
* // select a particular result for use.
* Operand result =
* ops.math.add(ops.unique(s, a).y(), b);
* // Optional attributes
* ops.linalg.matMul(a, b, MatMul.transposeA(true));
* // Naming operators
* ops.withName("foo").constant(5); // name "foo"
* // Names can exist in a hierarchy
* Ops sub = ops.withSubScope("sub");
* sub.withName("bar").constant(4); // "sub/bar"
* }
* }
*/
public final class Ops {
private final Scope scope;
public final NnOps nn;
public final SummaryOps summary;
public final ImageOps image;
public final DataOps data;
public final IoOps io;
public final DtypesOps dtypes;
public final LinalgOps linalg;
public final RandomOps random;
public final StringsOps strings;
public final SparseOps sparse;
public final BitwiseOps bitwise;
public final AudioOps audio;
public final MathOps math;
public final SignalOps signal;
public final QuantizationOps quantization;
public final TrainOps train;
private Ops(Scope scope) {
this.scope = scope;
nn = new NnOps(scope);
summary = new SummaryOps(scope);
image = new ImageOps(scope);
data = new DataOps(scope);
io = new IoOps(scope);
dtypes = new DtypesOps(scope);
linalg = new LinalgOps(scope);
random = new RandomOps(scope);
strings = new StringsOps(scope);
sparse = new SparseOps(scope);
bitwise = new BitwiseOps(scope);
audio = new AudioOps(scope);
math = new MathOps(scope);
signal = new SignalOps(scope);
quantization = new QuantizationOps(scope);
train = new TrainOps(scope);
}
/**
* Builds an {@link ReduceAll} operation
*
* @param input The tensor to reduce.
* @param axis The dimensions to reduce. Must be in the range
* @param options carries optional attributes values
* @return a new instance of ReduceAll
* @see org.tensorflow.op.core.ReduceAll
*/
public ReduceAll reduceAll(Operand input, Operand axis,
ReduceAll.Options... options) {
return ReduceAll.create(scope, input, axis, options);
}
/**
* Builds an {@link MutexLock} operation
*
* @param mutex The mutex resource to lock.
* @return a new instance of MutexLock
* @see org.tensorflow.op.core.MutexLock
*/
public MutexLock mutexLock(Operand> mutex) {
return MutexLock.create(scope, mutex);
}
/**
* Builds an {@link Sum} operation
*
* @param input The tensor to reduce.
* @param axis The dimensions to reduce. Must be in the range
* @param options carries optional attributes values
* @return a new instance of Sum
* @see org.tensorflow.op.core.Sum
*/
public Sum sum(Operand input, Operand axis,
Sum.Options... options) {
return Sum.create(scope, input, axis, options);
}
/**
* Builds an {@link Bucketize} operation
*
* @param input Any shape of Tensor contains with int or float type.
* @param boundaries A sorted list of floats gives the boundary of the buckets.
* @return a new instance of Bucketize
* @see org.tensorflow.op.core.Bucketize
*/
public Bucketize bucketize(Operand input, List boundaries) {
return Bucketize.create(scope, input, boundaries);
}
/**
* Builds an {@link TensorListGather} operation
*
* @param inputHandle
* @param indices
* @param elementShape
* @param elementDtype
* @return a new instance of TensorListGather
* @see org.tensorflow.op.core.TensorListGather
*/
public TensorListGather tensorListGather(Operand> inputHandle, Operand indices,
Operand elementShape, Class elementDtype) {
return TensorListGather.create(scope, inputHandle, indices, elementShape, elementDtype);
}
/**
* Builds an {@link ResourceGather} operation
*
* @param resource
* @param indices
* @param dtype
* @param options carries optional attributes values
* @return a new instance of ResourceGather
* @see org.tensorflow.op.core.ResourceGather
*/
public ResourceGather resourceGather(Operand> resource,
Operand indices, Class dtype, ResourceGather.Options... options) {
return ResourceGather.create(scope, resource, indices, dtype, options);
}
/**
* Builds an {@link Constant} operation
*
* @param shape the tensor shape.
* @param data a buffer containing the tensor data.
* @return a float constant
* @throws IllegalArgumentException If the tensor shape is not compatible with the buffer
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(long[] shape, FloatBuffer data) {
return Constant.create(scope, shape, data);
}
/**
* Builds an {@link ScatterMin} operation
*
* @param ref Should be from a `Variable` node.
* @param indices A tensor of indices into the first dimension of `ref`.
* @param updates A tensor of updated values to reduce into `ref`.
* @param options carries optional attributes values
* @return a new instance of ScatterMin
* @see org.tensorflow.op.core.ScatterMin
*/
public ScatterMin scatterMin(Operand ref,
Operand indices, Operand updates, ScatterMin.Options... options) {
return ScatterMin.create(scope, ref, indices, updates, options);
}
/**
* Builds an {@link Constant} operation
*
* @param data The value to put into the new constant.
* @return a float constant
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(float data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link HistogramFixedWidth} operation
*
* @param values Numeric `Tensor`.
* @param valueRange Shape [2] `Tensor` of same `dtype` as `values`.
* @param nbins Scalar `int32 Tensor`. Number of histogram bins.
* @param dtype
* @return a new instance of HistogramFixedWidth
* @see org.tensorflow.op.core.HistogramFixedWidth
*/
public HistogramFixedWidth histogramFixedWidth(Operand values,
Operand valueRange, Operand nbins, Class dtype) {
return HistogramFixedWidth.create(scope, values, valueRange, nbins, dtype);
}
/**
* Builds an {@link HistogramFixedWidth} operation
*
* @param values Numeric `Tensor`.
* @param valueRange Shape [2] `Tensor` of same `dtype` as `values`.
* @param nbins Scalar `int32 Tensor`. Number of histogram bins.
* @return a new instance of HistogramFixedWidth
* @see org.tensorflow.op.core.HistogramFixedWidth
*/
public HistogramFixedWidth histogramFixedWidth(Operand values,
Operand valueRange, Operand nbins) {
return HistogramFixedWidth.create(scope, values, valueRange, nbins);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. String elements are
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(byte[] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link InplaceAdd} operation
*
* @param x A `Tensor` of type T.
* @param i A vector. Indices into the left-most dimension of `x`.
* @param v A `Tensor` of type T. Same dimension sizes as x except the first dimension, which must be the same as i's size.
* @return a new instance of InplaceAdd
* @see org.tensorflow.op.core.InplaceAdd
*/
public InplaceAdd inplaceAdd(Operand x, Operand i, Operand v) {
return InplaceAdd.create(scope, x, i, v);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(int[][][] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link Min} operation
*
* @param input The tensor to reduce.
* @param axis The dimensions to reduce. Must be in the range
* @param options carries optional attributes values
* @return a new instance of Min
* @see org.tensorflow.op.core.Min
*/
public Min min(Operand input, Operand axis,
Min.Options... options) {
return Min.create(scope, input, axis, options);
}
/**
* Builds an {@link TensorArraySplit} operation
*
* @param handle The handle to a TensorArray.
* @param value The concatenated tensor to write to the TensorArray.
* @param lengths The vector of lengths, how to split the rows of value into the
* @param flowIn A float scalar that enforces proper chaining of operations.
* @return a new instance of TensorArraySplit
* @see org.tensorflow.op.core.TensorArraySplit
*/
public TensorArraySplit tensorArraySplit(Operand> handle, Operand value,
Operand lengths, Operand flowIn) {
return TensorArraySplit.create(scope, handle, value, lengths, flowIn);
}
/**
* Builds an {@link VarHandleOp} operation
*
* @param dtype the type of this variable. Must agree with the dtypes
* @param shape The (possibly partially specified) shape of this variable.
* @param options carries optional attributes values
* @return a new instance of VarHandleOp
* @see org.tensorflow.op.core.VarHandleOp
*/
public VarHandleOp varHandleOp(Class dtype, Shape shape, VarHandleOp.Options... options) {
return VarHandleOp.create(scope, dtype, shape, options);
}
/**
* Builds an {@link MapIncompleteSize} operation
*
* @param dtypes
* @param options carries optional attributes values
* @return a new instance of MapIncompleteSize
* @see org.tensorflow.op.core.MapIncompleteSize
*/
public MapIncompleteSize mapIncompleteSize(List> dtypes,
MapIncompleteSize.Options... options) {
return MapIncompleteSize.create(scope, dtypes, options);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(boolean[][][][][][] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link ScatterDiv} operation
*
* @param ref Should be from a `Variable` node.
* @param indices A tensor of indices into the first dimension of `ref`.
* @param updates A tensor of values that `ref` is divided by.
* @param options carries optional attributes values
* @return a new instance of ScatterDiv
* @see org.tensorflow.op.core.ScatterDiv
*/
public ScatterDiv scatterDiv(Operand ref, Operand indices,
Operand updates, ScatterDiv.Options... options) {
return ScatterDiv.create(scope, ref, indices, updates, options);
}
/**
* Builds an {@link TensorListConcatLists} operation
*
* @param inputA
* @param inputB
* @param elementDtype
* @return a new instance of TensorListConcatLists
* @see org.tensorflow.op.core.TensorListConcatLists
*/
public TensorListConcatLists tensorListConcatLists(Operand> inputA, Operand> inputB,
Class elementDtype) {
return TensorListConcatLists.create(scope, inputA, inputB, elementDtype);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(int[] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link FusedBatchNormV3} operation
*
* @param x A 4D Tensor for input data.
* @param scale A 1D Tensor for scaling factor, to scale the normalized x.
* @param offset A 1D Tensor for offset, to shift to the normalized x.
* @param mean A 1D Tensor for population mean. Used for inference only;
* @param variance A 1D Tensor for population variance. Used for inference only;
* @param options carries optional attributes values
* @return a new instance of FusedBatchNormV3
* @see org.tensorflow.op.core.FusedBatchNormV3
*/
public FusedBatchNormV3 fusedBatchNormV3(Operand x,
Operand scale, Operand offset, Operand mean, Operand variance,
FusedBatchNormV3.Options... options) {
return FusedBatchNormV3.create(scope, x, scale, offset, mean, variance, options);
}
/**
* Builds an {@link TensorArrayWrite} operation
*
* @param handle The handle to a TensorArray.
* @param index The position to write to inside the TensorArray.
* @param value The tensor to write to the TensorArray.
* @param flowIn A float scalar that enforces proper chaining of operations.
* @return a new instance of TensorArrayWrite
* @see org.tensorflow.op.core.TensorArrayWrite
*/
public TensorArrayWrite tensorArrayWrite(Operand> handle, Operand index,
Operand value, Operand flowIn) {
return TensorArrayWrite.create(scope, handle, index, value, flowIn);
}
/**
* Builds an {@link Unstage} operation
*
* @param dtypes
* @param options carries optional attributes values
* @return a new instance of Unstage
* @see org.tensorflow.op.core.Unstage
*/
public Unstage unstage(List> dtypes, Unstage.Options... options) {
return Unstage.create(scope, dtypes, options);
}
/**
* Builds an {@link ResourceScatterMin} operation
*
* @param resource Should be from a `Variable` node.
* @param indices A tensor of indices into the first dimension of `ref`.
* @param updates A tensor of updated values to add to `ref`.
* @return a new instance of ResourceScatterMin
* @see org.tensorflow.op.core.ResourceScatterMin
*/
public ResourceScatterMin resourceScatterMin(Operand> resource,
Operand indices, Operand updates) {
return ResourceScatterMin.create(scope, resource, indices, updates);
}
/**
* Builds an {@link DestroyTemporaryVariable} operation
*
* @param ref A reference to the temporary variable tensor.
* @param varName Name of the temporary variable, usually the name of the matching
* @return a new instance of DestroyTemporaryVariable
* @see org.tensorflow.op.core.DestroyTemporaryVariable
*/
public DestroyTemporaryVariable destroyTemporaryVariable(Operand ref, String varName) {
return DestroyTemporaryVariable.create(scope, ref, varName);
}
/**
* Builds an {@link RemoteFusedGraphExecute} operation
*
* @param inputs Arbitrary number of tensors with arbitrary data types
* @param Toutputs
* @param serializedRemoteFusedGraphExecuteInfo Serialized protocol buffer
* @return a new instance of RemoteFusedGraphExecute
* @see org.tensorflow.op.core.RemoteFusedGraphExecute
*/
public RemoteFusedGraphExecute remoteFusedGraphExecute(Iterable> inputs,
List> Toutputs, String serializedRemoteFusedGraphExecuteInfo) {
return RemoteFusedGraphExecute.create(scope, inputs, Toutputs, serializedRemoteFusedGraphExecuteInfo);
}
/**
* Builds an {@link Prod} operation
*
* @param input The tensor to reduce.
* @param axis The dimensions to reduce. Must be in the range
* @param options carries optional attributes values
* @return a new instance of Prod
* @see org.tensorflow.op.core.Prod
*/
public Prod prod(Operand input, Operand axis,
Prod.Options... options) {
return Prod.create(scope, input, axis, options);
}
/**
* Builds an {@link Fingerprint} operation
*
* @param data Must have rank 1 or higher.
* @param method Fingerprint method used by this op. Currently available method is
* @return a new instance of Fingerprint
* @see org.tensorflow.op.core.Fingerprint
*/
public Fingerprint fingerprint(Operand data, Operand method) {
return Fingerprint.create(scope, data, method);
}
/**
* Builds an {@link Reverse} operation
*
* @param tensor Up to 8-D.
* @param axis 1-D. The indices of the dimensions to reverse. Must be in the range
* @return a new instance of Reverse
* @see org.tensorflow.op.core.Reverse
*/
public Reverse reverse(Operand tensor, Operand axis) {
return Reverse.create(scope, tensor, axis);
}
/**
* Builds an {@link BarrierInsertMany} operation
*
* @param handle The handle to a barrier.
* @param keys A one-dimensional tensor of keys, with length n.
* @param values An any-dimensional tensor of values, which are associated with the
* @param componentIndex The component of the barrier elements that is being assigned.
* @return a new instance of BarrierInsertMany
* @see org.tensorflow.op.core.BarrierInsertMany
*/
public BarrierInsertMany barrierInsertMany(Operand handle, Operand keys,
Operand values, Long componentIndex) {
return BarrierInsertMany.create(scope, handle, keys, values, componentIndex);
}
/**
* Builds an {@link PlaceholderWithDefault} operation
*
* @param input The default value to produce when `output` is not fed.
* @param shape The (possibly partial) shape of the tensor.
* @return a new instance of PlaceholderWithDefault
* @see org.tensorflow.op.core.PlaceholderWithDefault
*/
public PlaceholderWithDefault placeholderWithDefault(Operand input, Shape shape) {
return PlaceholderWithDefault.create(scope, input, shape);
}
/**
* Builds an {@link StridedSliceAssign} operation
*
* @param ref
* @param begin
* @param end
* @param strides
* @param value
* @param options carries optional attributes values
* @return a new instance of StridedSliceAssign
* @see org.tensorflow.op.core.StridedSliceAssign
*/
public StridedSliceAssign stridedSliceAssign(Operand ref,
Operand begin, Operand end, Operand strides, Operand value,
StridedSliceAssign.Options... options) {
return StridedSliceAssign.create(scope, ref, begin, end, strides, value, options);
}
/**
* Builds an {@link MatrixDiagPartV2} operation
*
* @param input Rank `r` tensor where `r >= 2`.
* @param k Diagonal offset(s). Positive value means superdiagonal, 0 refers to the main
* @param paddingValue The value to fill the area outside the specified diagonal band with.
* @return a new instance of MatrixDiagPartV2
* @see org.tensorflow.op.core.MatrixDiagPartV2
*/
public MatrixDiagPartV2 matrixDiagPartV2(Operand input, Operand k,
Operand paddingValue) {
return MatrixDiagPartV2.create(scope, input, k, paddingValue);
}
/**
* Builds an {@link TensorListReserve} operation
*
* @param elementShape
* @param numElements
* @param elementDtype
* @return a new instance of TensorListReserve
* @see org.tensorflow.op.core.TensorListReserve
*/
public TensorListReserve tensorListReserve(Operand elementShape,
Operand numElements, Class elementDtype) {
return TensorListReserve.create(scope, elementShape, numElements, elementDtype);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(float[][] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link EuclideanNorm} operation
*
* @param input The tensor to reduce.
* @param axis The dimensions to reduce. Must be in the range
* @param options carries optional attributes values
* @return a new instance of EuclideanNorm
* @see org.tensorflow.op.core.EuclideanNorm
*/
public EuclideanNorm euclideanNorm(Operand input, Operand axis,
EuclideanNorm.Options... options) {
return EuclideanNorm.create(scope, input, axis, options);
}
/**
* Builds an {@link Constant} operation
*
* @param shape the tensor shape.
* @param data a buffer containing the tensor data.
* @return a double constant
* @throws IllegalArgumentException If the tensor shape is not compatible with the buffer
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(long[] shape, DoubleBuffer data) {
return Constant.create(scope, shape, data);
}
/**
* Builds an {@link Assign} operation
*
* @param ref Should be from a `Variable` node. May be uninitialized.
* @param value The value to be assigned to the variable.
* @param options carries optional attributes values
* @return a new instance of Assign
* @see org.tensorflow.op.core.Assign
*/
public Assign assign(Operand ref, Operand value, Assign.Options... options) {
return Assign.create(scope, ref, value, options);
}
/**
* Builds an {@link LookupTableInsert} operation
*
* @param tableHandle Handle to the table.
* @param keys Any shape. Keys to look up.
* @param values Values to associate with keys.
* @return a new instance of LookupTableInsert
* @see org.tensorflow.op.core.LookupTableInsert
*/
public LookupTableInsert lookupTableInsert(Operand> tableHandle, Operand keys,
Operand values) {
return LookupTableInsert.create(scope, tableHandle, keys, values);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(float[] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link ReduceSum} operation
*
* @param input The tensor to reduce.
* @param axis The dimensions to reduce. Must be in the range
* @param options carries optional attributes values
* @return a new instance of ReduceSum
* @see org.tensorflow.op.core.ReduceSum
*/
public ReduceSum reduceSum(Operand input, Operand axis,
ReduceSum.Options... options) {
return ReduceSum.create(scope, input, axis, options);
}
/**
* Builds an {@link Size} operation
*
* @param input
* @param outType
* @return a new instance of Size
* @see org.tensorflow.op.core.Size
*/
public Size size(Operand input, Class outType) {
return Size.create(scope, input, outType);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(boolean[][][][] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link ResourceScatterMul} operation
*
* @param resource Should be from a `Variable` node.
* @param indices A tensor of indices into the first dimension of `ref`.
* @param updates A tensor of updated values to add to `ref`.
* @return a new instance of ResourceScatterMul
* @see org.tensorflow.op.core.ResourceScatterMul
*/
public ResourceScatterMul resourceScatterMul(Operand> resource,
Operand indices, Operand updates) {
return ResourceScatterMul.create(scope, resource, indices, updates);
}
/**
* Builds an {@link ResourceGatherNd} operation
*
* @param resource
* @param indices
* @param dtype
* @return a new instance of ResourceGatherNd
* @see org.tensorflow.op.core.ResourceGatherNd
*/
public ResourceGatherNd resourceGatherNd(Operand> resource,
Operand indices, Class dtype) {
return ResourceGatherNd.create(scope, resource, indices, dtype);
}
/**
* Builds an {@link GetSessionTensor} operation
*
* @param handle The handle for a tensor stored in the session state.
* @param dtype The type of the output value.
* @return a new instance of GetSessionTensor
* @see org.tensorflow.op.core.GetSessionTensor
*/
public GetSessionTensor getSessionTensor(Operand handle, Class dtype) {
return GetSessionTensor.create(scope, handle, dtype);
}
/**
* Builds an {@link AssignAdd} operation
*
* @param ref Should be from a `Variable` node.
* @param value The value to be added to the variable.
* @param options carries optional attributes values
* @return a new instance of AssignAdd
* @see org.tensorflow.op.core.AssignAdd
*/
public AssignAdd assignAdd(Operand ref, Operand value,
AssignAdd.Options... options) {
return AssignAdd.create(scope, ref, value, options);
}
/**
* Builds an {@link TensorListSetItem} operation
*
* @param inputHandle
* @param index
* @param item
* @return a new instance of TensorListSetItem
* @see org.tensorflow.op.core.TensorListSetItem
*/
public TensorListSetItem tensorListSetItem(Operand> inputHandle, Operand index,
Operand item) {
return TensorListSetItem.create(scope, inputHandle, index, item);
}
/**
* Builds an {@link MutableHashTable} operation
*
* @param keyDtype Type of the table keys.
* @param valueDtype Type of the table values.
* @param options carries optional attributes values
* @return a new instance of MutableHashTable
* @see org.tensorflow.op.core.MutableHashTable
*/
public MutableHashTable mutableHashTable(Class keyDtype, Class valueDtype,
MutableHashTable.Options... options) {
return MutableHashTable.create(scope, keyDtype, valueDtype, options);
}
/**
* Builds an {@link TensorListScatterIntoExistingList} operation
*
* @param inputHandle
* @param tensor
* @param indices
* @return a new instance of TensorListScatterIntoExistingList
* @see org.tensorflow.op.core.TensorListScatterIntoExistingList
*/
public TensorListScatterIntoExistingList tensorListScatterIntoExistingList(Operand> inputHandle,
Operand tensor, Operand indices) {
return TensorListScatterIntoExistingList.create(scope, inputHandle, tensor, indices);
}
/**
* Builds an {@link EditDistance} operation
*
* @param hypothesisIndices The indices of the hypothesis list SparseTensor.
* @param hypothesisValues The values of the hypothesis list SparseTensor.
* @param hypothesisShape The shape of the hypothesis list SparseTensor.
* @param truthIndices The indices of the truth list SparseTensor.
* @param truthValues The values of the truth list SparseTensor.
* @param truthShape truth indices, vector.
* @param options carries optional attributes values
* @return a new instance of EditDistance
* @see org.tensorflow.op.core.EditDistance
*/
public EditDistance editDistance(Operand hypothesisIndices, Operand hypothesisValues,
Operand hypothesisShape, Operand truthIndices, Operand truthValues,
Operand truthShape, EditDistance.Options... options) {
return EditDistance.create(scope, hypothesisIndices, hypothesisValues, hypothesisShape, truthIndices, truthValues, truthShape, options);
}
/**
* Builds an {@link ResourceApplyAdamWithAmsgrad} operation
*
* @param var Should be from a Variable().
* @param m Should be from a Variable().
* @param v Should be from a Variable().
* @param vhat Should be from a Variable().
* @param beta1Power Must be a scalar.
* @param beta2Power Must be a scalar.
* @param lr Scaling factor. Must be a scalar.
* @param beta1 Momentum factor. Must be a scalar.
* @param beta2 Momentum factor. Must be a scalar.
* @param epsilon Ridge term. Must be a scalar.
* @param grad The gradient.
* @param options carries optional attributes values
* @return a new instance of ResourceApplyAdamWithAmsgrad
* @see org.tensorflow.op.core.ResourceApplyAdamWithAmsgrad
*/
public ResourceApplyAdamWithAmsgrad resourceApplyAdamWithAmsgrad(Operand> var, Operand> m,
Operand> v, Operand> vhat, Operand beta1Power, Operand beta2Power, Operand lr,
Operand beta1, Operand beta2, Operand epsilon, Operand grad,
ResourceApplyAdamWithAmsgrad.Options... options) {
return ResourceApplyAdamWithAmsgrad.create(scope, var, m, v, vhat, beta1Power, beta2Power, lr, beta1, beta2, epsilon, grad, options);
}
/**
* Builds an {@link MirrorPad} operation
*
* @param input The input tensor to be padded.
* @param paddings A two-column matrix specifying the padding sizes. The number of
* @param mode Either `REFLECT` or `SYMMETRIC`. In reflect mode the padded regions
* @return a new instance of MirrorPad
* @see org.tensorflow.op.core.MirrorPad
*/
public MirrorPad mirrorPad(Operand input, Operand paddings,
String mode) {
return MirrorPad.create(scope, input, paddings, mode);
}
/**
* Builds an {@link Size} operation
*
* @param input
* @return a new instance of Size
* @see org.tensorflow.op.core.Size
*/
public Size size(Operand input) {
return Size.create(scope, input);
}
/**
* Builds an {@link Constant} operation
*
* @param object a Java object representing the constant.
* @return a constant of type `type`
* @see org.tensorflow.Tensor#create(Object) Tensor.create
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(Object object, Class type) {
return Constant.create(scope, object, type);
}
/**
* Builds an {@link OrderedMapPeek} operation
*
* @param key
* @param indices
* @param dtypes
* @param options carries optional attributes values
* @return a new instance of OrderedMapPeek
* @see org.tensorflow.op.core.OrderedMapPeek
*/
public OrderedMapPeek orderedMapPeek(Operand key, Operand indices,
List> dtypes, OrderedMapPeek.Options... options) {
return OrderedMapPeek.create(scope, key, indices, dtypes, options);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. String elements are
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(byte[][][] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link ResourceStridedSliceAssign} operation
*
* @param ref
* @param begin
* @param end
* @param strides
* @param value
* @param options carries optional attributes values
* @return a new instance of ResourceStridedSliceAssign
* @see org.tensorflow.op.core.ResourceStridedSliceAssign
*/
public ResourceStridedSliceAssign resourceStridedSliceAssign(Operand> ref,
Operand begin, Operand end, Operand strides, Operand value,
ResourceStridedSliceAssign.Options... options) {
return ResourceStridedSliceAssign.create(scope, ref, begin, end, strides, value, options);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(int[][][][] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link TensorListStack} operation
*
* @param inputHandle
* @param elementShape
* @param elementDtype
* @param options carries optional attributes values
* @return a new instance of TensorListStack
* @see org.tensorflow.op.core.TensorListStack
*/
public TensorListStack tensorListStack(Operand> inputHandle,
Operand elementShape, Class elementDtype, TensorListStack.Options... options) {
return TensorListStack.create(scope, inputHandle, elementShape, elementDtype, options);
}
/**
* Builds an {@link StatefulStandardNormal} operation
*
* @param resource The handle of the resource variable that stores the state of the RNG.
* @param shape The shape of the output tensor.
* @return a new instance of StatefulStandardNormal
* @see org.tensorflow.op.core.StatefulStandardNormal
*/
public StatefulStandardNormal statefulStandardNormal(Operand> resource,
Operand shape) {
return StatefulStandardNormal.create(scope, resource, shape);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(double[] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link Lu} operation
*
* @param input A tensor of shape `[..., M, M]` whose inner-most 2 dimensions form matrices of
* @return a new instance of Lu
* @see org.tensorflow.op.core.Lu
*/
public Lu lu(Operand input) {
return Lu.create(scope, input);
}
/**
* Builds an {@link OneHot} operation
*
* @param indices A tensor of indices.
* @param depth A scalar defining the depth of the one hot dimension.
* @param onValue A scalar defining the value to fill in output when `indices[j] = i`.
* @param offValue A scalar defining the value to fill in output when `indices[j] != i`.
* @param options carries optional attributes values
* @return a new instance of OneHot
* @see org.tensorflow.op.core.OneHot
*/
public OneHot oneHot(Operand indices, Operand depth,
Operand onValue, Operand offValue, OneHot.Options... options) {
return OneHot.create(scope, indices, depth, onValue, offValue, options);
}
/**
* Builds an {@link DeleteSessionTensor} operation
*
* @param handle The handle for a tensor stored in the session state.
* @return a new instance of DeleteSessionTensor
* @see org.tensorflow.op.core.DeleteSessionTensor
*/
public DeleteSessionTensor deleteSessionTensor(Operand handle) {
return DeleteSessionTensor.create(scope, handle);
}
/**
* Builds an {@link Gradients} operation
*
* @param y output of the function to derive
* @param x inputs of the function for which partial derivatives are computed
* @param options carries optional attributes values
* @return a new instance of {@code Gradients}
* @throws IllegalArgumentException if execution environment is not a graph
* @see org.tensorflow.op.core.Gradients
*/
public Gradients gradients(Operand> y, Iterable extends Operand>> x,
Gradients.Options... options) {
return Gradients.create(scope, y, x, options);
}
/**
* Builds an {@link UnsortedSegmentJoin} operation
*
* @param inputs The input to be joined.
* @param segmentIds A tensor whose shape is a prefix of data.shape. Negative segment ids are not
* @param numSegments A scalar.
* @param options carries optional attributes values
* @return a new instance of UnsortedSegmentJoin
* @see org.tensorflow.op.core.UnsortedSegmentJoin
*/
public UnsortedSegmentJoin unsortedSegmentJoin(Operand inputs,
Operand segmentIds, Operand numSegments, UnsortedSegmentJoin.Options... options) {
return UnsortedSegmentJoin.create(scope, inputs, segmentIds, numSegments, options);
}
/**
* Builds an {@link ResourceScatterNdSub} operation
*
* @param ref A resource handle. Must be from a VarHandleOp.
* @param indices A Tensor. Must be one of the following types: int32, int64.
* @param updates A Tensor. Must have the same type as ref. A tensor of
* @param options carries optional attributes values
* @return a new instance of ResourceScatterNdSub
* @see org.tensorflow.op.core.ResourceScatterNdSub
*/
public ResourceScatterNdSub resourceScatterNdSub(Operand> ref,
Operand indices, Operand updates, ResourceScatterNdSub.Options... options) {
return ResourceScatterNdSub.create(scope, ref, indices, updates, options);
}
/**
* Builds an {@link ResourceScatterNdUpdate} operation
*
* @param ref A resource handle. Must be from a VarHandleOp.
* @param indices A Tensor. Must be one of the following types: int32, int64.
* @param updates A Tensor. Must have the same type as ref. A tensor of updated
* @param options carries optional attributes values
* @return a new instance of ResourceScatterNdUpdate
* @see org.tensorflow.op.core.ResourceScatterNdUpdate
*/
public ResourceScatterNdUpdate resourceScatterNdUpdate(Operand> ref,
Operand indices, Operand updates, ResourceScatterNdUpdate.Options... options) {
return ResourceScatterNdUpdate.create(scope, ref, indices, updates, options);
}
/**
* Builds an {@link Constant} operation
*
* @param data An array containing the values to put into the new constant. The dimensions of the
* @see org.tensorflow.op.core.Constant
*/
public Constant constant(float[][][][][][] data) {
return Constant.create(scope, data);
}
/**
* Builds an {@link CombinedNonMaxSuppression} operation
*
* @param boxes A 4-D float tensor of shape `[batch_size, num_boxes, q, 4]`. If `q` is 1 then
* @param scores A 3-D float tensor of shape `[batch_size, num_boxes, num_classes]`
* @param maxOutputSizePerClass A scalar integer tensor representing the maximum number of
* @param maxTotalSize A scalar representing maximum number of boxes retained over all classes.
* @param iouThreshold A 0-D float tensor representing the threshold for deciding whether
* @param scoreThreshold A 0-D float tensor representing the threshold for deciding when to remove
* @param options carries optional attributes values
* @return a new instance of CombinedNonMaxSuppression
* @see org.tensorflow.op.core.CombinedNonMaxSuppression
*/
public CombinedNonMaxSuppression combinedNonMaxSuppression(Operand boxes,
Operand scores, Operand maxOutputSizePerClass, Operand maxTotalSize,
Operand iouThreshold, Operand scoreThreshold,
CombinedNonMaxSuppression.Options... options) {
return CombinedNonMaxSuppression.create(scope, boxes, scores, maxOutputSizePerClass, maxTotalSize, iouThreshold, scoreThreshold, options);
}
/**
* Builds an {@link LinSpace} operation
*
* @param start 0-D tensor. First entry in the range.
* @param stop 0-D tensor. Last entry in the range.
* @param num 0-D tensor. Number of values to generate.
* @return a new instance of LinSpace
* @see org.tensorflow.op.core.LinSpace
*/
public LinSpace linSpace(Operand start,
Operand stop, Operand num) {
return LinSpace.create(scope, start, stop, num);
}
/**
* Builds an {@link StringNGrams} operation
*
* @param data The values tensor of the ragged string tensor to make ngrams out of. Must be a
* @param dataSplits The splits tensor of the ragged string tensor to make ngrams out of.
* @param separator The string to append between elements of the token. Use "" for no separator.
* @param ngramWidths The sizes of the ngrams to create.
* @param leftPad The string to use to pad the left side of the ngram sequence. Only used if
* @param rightPad The string to use to pad the right side of the ngram sequence. Only used if
* @param padWidth The number of padding elements to add to each side of each
* @param preserveShortSequences
* @return a new instance of StringNGrams
* @see org.tensorflow.op.core.StringNGrams
*/
public StringNGrams stringNGrams(Operand data,
Operand dataSplits, String separator, List ngramWidths, String leftPad,
String rightPad, Long padWidth, Boolean preserveShortSequences) {
return StringNGrams.create(scope, data, dataSplits, separator, ngramWidths, leftPad, rightPad, padWidth, preserveShortSequences);
}
/**
* Builds an {@link ZerosLike} operation
*
* @param x a tensor of type T.
* @return a new instance of ZerosLike
* @see org.tensorflow.op.core.ZerosLike
*/
public ZerosLike zerosLike(Operand x) {
return ZerosLike.create(scope, x);
}
/**
* Builds an {@link InplaceSub} operation
*
* @param x A `Tensor` of type T.
* @param i A vector. Indices into the left-most dimension of `x`.
* @param v A `Tensor` of type T. Same dimension sizes as x except the first dimension, which must be the same as i's size.
* @return a new instance of InplaceSub
* @see org.tensorflow.op.core.InplaceSub
*/
public InplaceSub inplaceSub(Operand