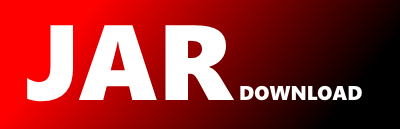
org.tensorflow.framework.GraphTransferInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/framework/graph_transfer_info.proto
package org.tensorflow.framework;
/**
*
* Protocol buffer representing a handle to a tensorflow resource. Handles are
* not valid across executions, but can be serialized back and forth from within
* a single run.
*
*
* Protobuf type {@code tensorflow.GraphTransferInfo}
*/
public final class GraphTransferInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.GraphTransferInfo)
GraphTransferInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use GraphTransferInfo.newBuilder() to construct.
private GraphTransferInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GraphTransferInfo() {
nodeInfo_ = java.util.Collections.emptyList();
constNodeInfo_ = java.util.Collections.emptyList();
nodeInputInfo_ = java.util.Collections.emptyList();
nodeOutputInfo_ = java.util.Collections.emptyList();
graphInputNodeInfo_ = java.util.Collections.emptyList();
graphOutputNodeInfo_ = java.util.Collections.emptyList();
destination_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GraphTransferInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
nodeInfo_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
nodeInfo_.add(
input.readMessage(org.tensorflow.framework.GraphTransferNodeInfo.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
constNodeInfo_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
constNodeInfo_.add(
input.readMessage(org.tensorflow.framework.GraphTransferConstNodeInfo.parser(), extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
nodeInputInfo_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000004;
}
nodeInputInfo_.add(
input.readMessage(org.tensorflow.framework.GraphTransferNodeInputInfo.parser(), extensionRegistry));
break;
}
case 34: {
if (!((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
nodeOutputInfo_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000008;
}
nodeOutputInfo_.add(
input.readMessage(org.tensorflow.framework.GraphTransferNodeOutputInfo.parser(), extensionRegistry));
break;
}
case 42: {
if (!((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
graphInputNodeInfo_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000010;
}
graphInputNodeInfo_.add(
input.readMessage(org.tensorflow.framework.GraphTransferGraphInputNodeInfo.parser(), extensionRegistry));
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
graphOutputNodeInfo_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
graphOutputNodeInfo_.add(
input.readMessage(org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.parser(), extensionRegistry));
break;
}
case 56: {
int rawValue = input.readEnum();
destination_ = rawValue;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
nodeInfo_ = java.util.Collections.unmodifiableList(nodeInfo_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
constNodeInfo_ = java.util.Collections.unmodifiableList(constNodeInfo_);
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
nodeInputInfo_ = java.util.Collections.unmodifiableList(nodeInputInfo_);
}
if (((mutable_bitField0_ & 0x00000008) == 0x00000008)) {
nodeOutputInfo_ = java.util.Collections.unmodifiableList(nodeOutputInfo_);
}
if (((mutable_bitField0_ & 0x00000010) == 0x00000010)) {
graphInputNodeInfo_ = java.util.Collections.unmodifiableList(graphInputNodeInfo_);
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
graphOutputNodeInfo_ = java.util.Collections.unmodifiableList(graphOutputNodeInfo_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphTransferInfoProto.internal_static_tensorflow_GraphTransferInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphTransferInfoProto.internal_static_tensorflow_GraphTransferInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphTransferInfo.class, org.tensorflow.framework.GraphTransferInfo.Builder.class);
}
/**
* Protobuf enum {@code tensorflow.GraphTransferInfo.Destination}
*/
public enum Destination
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NOP = 0;
*/
NOP(0),
/**
* HEXAGON = 1;
*/
HEXAGON(1),
UNRECOGNIZED(-1),
;
/**
* NOP = 0;
*/
public static final int NOP_VALUE = 0;
/**
* HEXAGON = 1;
*/
public static final int HEXAGON_VALUE = 1;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Destination valueOf(int value) {
return forNumber(value);
}
public static Destination forNumber(int value) {
switch (value) {
case 0: return NOP;
case 1: return HEXAGON;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Destination> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Destination findValueByNumber(int number) {
return Destination.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tensorflow.framework.GraphTransferInfo.getDescriptor().getEnumTypes().get(0);
}
private static final Destination[] VALUES = values();
public static Destination valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Destination(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.GraphTransferInfo.Destination)
}
private int bitField0_;
public static final int NODE_INFO_FIELD_NUMBER = 1;
private java.util.List nodeInfo_;
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public java.util.List getNodeInfoList() {
return nodeInfo_;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferNodeInfoOrBuilder>
getNodeInfoOrBuilderList() {
return nodeInfo_;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public int getNodeInfoCount() {
return nodeInfo_.size();
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public org.tensorflow.framework.GraphTransferNodeInfo getNodeInfo(int index) {
return nodeInfo_.get(index);
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public org.tensorflow.framework.GraphTransferNodeInfoOrBuilder getNodeInfoOrBuilder(
int index) {
return nodeInfo_.get(index);
}
public static final int CONST_NODE_INFO_FIELD_NUMBER = 2;
private java.util.List constNodeInfo_;
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public java.util.List getConstNodeInfoList() {
return constNodeInfo_;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferConstNodeInfoOrBuilder>
getConstNodeInfoOrBuilderList() {
return constNodeInfo_;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public int getConstNodeInfoCount() {
return constNodeInfo_.size();
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public org.tensorflow.framework.GraphTransferConstNodeInfo getConstNodeInfo(int index) {
return constNodeInfo_.get(index);
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public org.tensorflow.framework.GraphTransferConstNodeInfoOrBuilder getConstNodeInfoOrBuilder(
int index) {
return constNodeInfo_.get(index);
}
public static final int NODE_INPUT_INFO_FIELD_NUMBER = 3;
private java.util.List nodeInputInfo_;
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public java.util.List getNodeInputInfoList() {
return nodeInputInfo_;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferNodeInputInfoOrBuilder>
getNodeInputInfoOrBuilderList() {
return nodeInputInfo_;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public int getNodeInputInfoCount() {
return nodeInputInfo_.size();
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public org.tensorflow.framework.GraphTransferNodeInputInfo getNodeInputInfo(int index) {
return nodeInputInfo_.get(index);
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public org.tensorflow.framework.GraphTransferNodeInputInfoOrBuilder getNodeInputInfoOrBuilder(
int index) {
return nodeInputInfo_.get(index);
}
public static final int NODE_OUTPUT_INFO_FIELD_NUMBER = 4;
private java.util.List nodeOutputInfo_;
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public java.util.List getNodeOutputInfoList() {
return nodeOutputInfo_;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferNodeOutputInfoOrBuilder>
getNodeOutputInfoOrBuilderList() {
return nodeOutputInfo_;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public int getNodeOutputInfoCount() {
return nodeOutputInfo_.size();
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public org.tensorflow.framework.GraphTransferNodeOutputInfo getNodeOutputInfo(int index) {
return nodeOutputInfo_.get(index);
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public org.tensorflow.framework.GraphTransferNodeOutputInfoOrBuilder getNodeOutputInfoOrBuilder(
int index) {
return nodeOutputInfo_.get(index);
}
public static final int GRAPH_INPUT_NODE_INFO_FIELD_NUMBER = 5;
private java.util.List graphInputNodeInfo_;
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public java.util.List getGraphInputNodeInfoList() {
return graphInputNodeInfo_;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferGraphInputNodeInfoOrBuilder>
getGraphInputNodeInfoOrBuilderList() {
return graphInputNodeInfo_;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public int getGraphInputNodeInfoCount() {
return graphInputNodeInfo_.size();
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public org.tensorflow.framework.GraphTransferGraphInputNodeInfo getGraphInputNodeInfo(int index) {
return graphInputNodeInfo_.get(index);
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public org.tensorflow.framework.GraphTransferGraphInputNodeInfoOrBuilder getGraphInputNodeInfoOrBuilder(
int index) {
return graphInputNodeInfo_.get(index);
}
public static final int GRAPH_OUTPUT_NODE_INFO_FIELD_NUMBER = 6;
private java.util.List graphOutputNodeInfo_;
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public java.util.List getGraphOutputNodeInfoList() {
return graphOutputNodeInfo_;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferGraphOutputNodeInfoOrBuilder>
getGraphOutputNodeInfoOrBuilderList() {
return graphOutputNodeInfo_;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public int getGraphOutputNodeInfoCount() {
return graphOutputNodeInfo_.size();
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public org.tensorflow.framework.GraphTransferGraphOutputNodeInfo getGraphOutputNodeInfo(int index) {
return graphOutputNodeInfo_.get(index);
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public org.tensorflow.framework.GraphTransferGraphOutputNodeInfoOrBuilder getGraphOutputNodeInfoOrBuilder(
int index) {
return graphOutputNodeInfo_.get(index);
}
public static final int DESTINATION_FIELD_NUMBER = 7;
private int destination_;
/**
*
* Destination of graph transfer
*
*
* .tensorflow.GraphTransferInfo.Destination destination = 7;
*/
public int getDestinationValue() {
return destination_;
}
/**
*
* Destination of graph transfer
*
*
* .tensorflow.GraphTransferInfo.Destination destination = 7;
*/
public org.tensorflow.framework.GraphTransferInfo.Destination getDestination() {
org.tensorflow.framework.GraphTransferInfo.Destination result = org.tensorflow.framework.GraphTransferInfo.Destination.valueOf(destination_);
return result == null ? org.tensorflow.framework.GraphTransferInfo.Destination.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < nodeInfo_.size(); i++) {
output.writeMessage(1, nodeInfo_.get(i));
}
for (int i = 0; i < constNodeInfo_.size(); i++) {
output.writeMessage(2, constNodeInfo_.get(i));
}
for (int i = 0; i < nodeInputInfo_.size(); i++) {
output.writeMessage(3, nodeInputInfo_.get(i));
}
for (int i = 0; i < nodeOutputInfo_.size(); i++) {
output.writeMessage(4, nodeOutputInfo_.get(i));
}
for (int i = 0; i < graphInputNodeInfo_.size(); i++) {
output.writeMessage(5, graphInputNodeInfo_.get(i));
}
for (int i = 0; i < graphOutputNodeInfo_.size(); i++) {
output.writeMessage(6, graphOutputNodeInfo_.get(i));
}
if (destination_ != org.tensorflow.framework.GraphTransferInfo.Destination.NOP.getNumber()) {
output.writeEnum(7, destination_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < nodeInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, nodeInfo_.get(i));
}
for (int i = 0; i < constNodeInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, constNodeInfo_.get(i));
}
for (int i = 0; i < nodeInputInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, nodeInputInfo_.get(i));
}
for (int i = 0; i < nodeOutputInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, nodeOutputInfo_.get(i));
}
for (int i = 0; i < graphInputNodeInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, graphInputNodeInfo_.get(i));
}
for (int i = 0; i < graphOutputNodeInfo_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, graphOutputNodeInfo_.get(i));
}
if (destination_ != org.tensorflow.framework.GraphTransferInfo.Destination.NOP.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, destination_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.GraphTransferInfo)) {
return super.equals(obj);
}
org.tensorflow.framework.GraphTransferInfo other = (org.tensorflow.framework.GraphTransferInfo) obj;
boolean result = true;
result = result && getNodeInfoList()
.equals(other.getNodeInfoList());
result = result && getConstNodeInfoList()
.equals(other.getConstNodeInfoList());
result = result && getNodeInputInfoList()
.equals(other.getNodeInputInfoList());
result = result && getNodeOutputInfoList()
.equals(other.getNodeOutputInfoList());
result = result && getGraphInputNodeInfoList()
.equals(other.getGraphInputNodeInfoList());
result = result && getGraphOutputNodeInfoList()
.equals(other.getGraphOutputNodeInfoList());
result = result && destination_ == other.destination_;
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNodeInfoCount() > 0) {
hash = (37 * hash) + NODE_INFO_FIELD_NUMBER;
hash = (53 * hash) + getNodeInfoList().hashCode();
}
if (getConstNodeInfoCount() > 0) {
hash = (37 * hash) + CONST_NODE_INFO_FIELD_NUMBER;
hash = (53 * hash) + getConstNodeInfoList().hashCode();
}
if (getNodeInputInfoCount() > 0) {
hash = (37 * hash) + NODE_INPUT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getNodeInputInfoList().hashCode();
}
if (getNodeOutputInfoCount() > 0) {
hash = (37 * hash) + NODE_OUTPUT_INFO_FIELD_NUMBER;
hash = (53 * hash) + getNodeOutputInfoList().hashCode();
}
if (getGraphInputNodeInfoCount() > 0) {
hash = (37 * hash) + GRAPH_INPUT_NODE_INFO_FIELD_NUMBER;
hash = (53 * hash) + getGraphInputNodeInfoList().hashCode();
}
if (getGraphOutputNodeInfoCount() > 0) {
hash = (37 * hash) + GRAPH_OUTPUT_NODE_INFO_FIELD_NUMBER;
hash = (53 * hash) + getGraphOutputNodeInfoList().hashCode();
}
hash = (37 * hash) + DESTINATION_FIELD_NUMBER;
hash = (53 * hash) + destination_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphTransferInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphTransferInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphTransferInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.GraphTransferInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Protocol buffer representing a handle to a tensorflow resource. Handles are
* not valid across executions, but can be serialized back and forth from within
* a single run.
*
*
* Protobuf type {@code tensorflow.GraphTransferInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.GraphTransferInfo)
org.tensorflow.framework.GraphTransferInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphTransferInfoProto.internal_static_tensorflow_GraphTransferInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphTransferInfoProto.internal_static_tensorflow_GraphTransferInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphTransferInfo.class, org.tensorflow.framework.GraphTransferInfo.Builder.class);
}
// Construct using org.tensorflow.framework.GraphTransferInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNodeInfoFieldBuilder();
getConstNodeInfoFieldBuilder();
getNodeInputInfoFieldBuilder();
getNodeOutputInfoFieldBuilder();
getGraphInputNodeInfoFieldBuilder();
getGraphOutputNodeInfoFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (nodeInfoBuilder_ == null) {
nodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
nodeInfoBuilder_.clear();
}
if (constNodeInfoBuilder_ == null) {
constNodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
constNodeInfoBuilder_.clear();
}
if (nodeInputInfoBuilder_ == null) {
nodeInputInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
} else {
nodeInputInfoBuilder_.clear();
}
if (nodeOutputInfoBuilder_ == null) {
nodeOutputInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
} else {
nodeOutputInfoBuilder_.clear();
}
if (graphInputNodeInfoBuilder_ == null) {
graphInputNodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
} else {
graphInputNodeInfoBuilder_.clear();
}
if (graphOutputNodeInfoBuilder_ == null) {
graphOutputNodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
graphOutputNodeInfoBuilder_.clear();
}
destination_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.GraphTransferInfoProto.internal_static_tensorflow_GraphTransferInfo_descriptor;
}
public org.tensorflow.framework.GraphTransferInfo getDefaultInstanceForType() {
return org.tensorflow.framework.GraphTransferInfo.getDefaultInstance();
}
public org.tensorflow.framework.GraphTransferInfo build() {
org.tensorflow.framework.GraphTransferInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.GraphTransferInfo buildPartial() {
org.tensorflow.framework.GraphTransferInfo result = new org.tensorflow.framework.GraphTransferInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (nodeInfoBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
nodeInfo_ = java.util.Collections.unmodifiableList(nodeInfo_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.nodeInfo_ = nodeInfo_;
} else {
result.nodeInfo_ = nodeInfoBuilder_.build();
}
if (constNodeInfoBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
constNodeInfo_ = java.util.Collections.unmodifiableList(constNodeInfo_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.constNodeInfo_ = constNodeInfo_;
} else {
result.constNodeInfo_ = constNodeInfoBuilder_.build();
}
if (nodeInputInfoBuilder_ == null) {
if (((bitField0_ & 0x00000004) == 0x00000004)) {
nodeInputInfo_ = java.util.Collections.unmodifiableList(nodeInputInfo_);
bitField0_ = (bitField0_ & ~0x00000004);
}
result.nodeInputInfo_ = nodeInputInfo_;
} else {
result.nodeInputInfo_ = nodeInputInfoBuilder_.build();
}
if (nodeOutputInfoBuilder_ == null) {
if (((bitField0_ & 0x00000008) == 0x00000008)) {
nodeOutputInfo_ = java.util.Collections.unmodifiableList(nodeOutputInfo_);
bitField0_ = (bitField0_ & ~0x00000008);
}
result.nodeOutputInfo_ = nodeOutputInfo_;
} else {
result.nodeOutputInfo_ = nodeOutputInfoBuilder_.build();
}
if (graphInputNodeInfoBuilder_ == null) {
if (((bitField0_ & 0x00000010) == 0x00000010)) {
graphInputNodeInfo_ = java.util.Collections.unmodifiableList(graphInputNodeInfo_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.graphInputNodeInfo_ = graphInputNodeInfo_;
} else {
result.graphInputNodeInfo_ = graphInputNodeInfoBuilder_.build();
}
if (graphOutputNodeInfoBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
graphOutputNodeInfo_ = java.util.Collections.unmodifiableList(graphOutputNodeInfo_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.graphOutputNodeInfo_ = graphOutputNodeInfo_;
} else {
result.graphOutputNodeInfo_ = graphOutputNodeInfoBuilder_.build();
}
result.destination_ = destination_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.GraphTransferInfo) {
return mergeFrom((org.tensorflow.framework.GraphTransferInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.GraphTransferInfo other) {
if (other == org.tensorflow.framework.GraphTransferInfo.getDefaultInstance()) return this;
if (nodeInfoBuilder_ == null) {
if (!other.nodeInfo_.isEmpty()) {
if (nodeInfo_.isEmpty()) {
nodeInfo_ = other.nodeInfo_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNodeInfoIsMutable();
nodeInfo_.addAll(other.nodeInfo_);
}
onChanged();
}
} else {
if (!other.nodeInfo_.isEmpty()) {
if (nodeInfoBuilder_.isEmpty()) {
nodeInfoBuilder_.dispose();
nodeInfoBuilder_ = null;
nodeInfo_ = other.nodeInfo_;
bitField0_ = (bitField0_ & ~0x00000001);
nodeInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNodeInfoFieldBuilder() : null;
} else {
nodeInfoBuilder_.addAllMessages(other.nodeInfo_);
}
}
}
if (constNodeInfoBuilder_ == null) {
if (!other.constNodeInfo_.isEmpty()) {
if (constNodeInfo_.isEmpty()) {
constNodeInfo_ = other.constNodeInfo_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureConstNodeInfoIsMutable();
constNodeInfo_.addAll(other.constNodeInfo_);
}
onChanged();
}
} else {
if (!other.constNodeInfo_.isEmpty()) {
if (constNodeInfoBuilder_.isEmpty()) {
constNodeInfoBuilder_.dispose();
constNodeInfoBuilder_ = null;
constNodeInfo_ = other.constNodeInfo_;
bitField0_ = (bitField0_ & ~0x00000002);
constNodeInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getConstNodeInfoFieldBuilder() : null;
} else {
constNodeInfoBuilder_.addAllMessages(other.constNodeInfo_);
}
}
}
if (nodeInputInfoBuilder_ == null) {
if (!other.nodeInputInfo_.isEmpty()) {
if (nodeInputInfo_.isEmpty()) {
nodeInputInfo_ = other.nodeInputInfo_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureNodeInputInfoIsMutable();
nodeInputInfo_.addAll(other.nodeInputInfo_);
}
onChanged();
}
} else {
if (!other.nodeInputInfo_.isEmpty()) {
if (nodeInputInfoBuilder_.isEmpty()) {
nodeInputInfoBuilder_.dispose();
nodeInputInfoBuilder_ = null;
nodeInputInfo_ = other.nodeInputInfo_;
bitField0_ = (bitField0_ & ~0x00000004);
nodeInputInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNodeInputInfoFieldBuilder() : null;
} else {
nodeInputInfoBuilder_.addAllMessages(other.nodeInputInfo_);
}
}
}
if (nodeOutputInfoBuilder_ == null) {
if (!other.nodeOutputInfo_.isEmpty()) {
if (nodeOutputInfo_.isEmpty()) {
nodeOutputInfo_ = other.nodeOutputInfo_;
bitField0_ = (bitField0_ & ~0x00000008);
} else {
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.addAll(other.nodeOutputInfo_);
}
onChanged();
}
} else {
if (!other.nodeOutputInfo_.isEmpty()) {
if (nodeOutputInfoBuilder_.isEmpty()) {
nodeOutputInfoBuilder_.dispose();
nodeOutputInfoBuilder_ = null;
nodeOutputInfo_ = other.nodeOutputInfo_;
bitField0_ = (bitField0_ & ~0x00000008);
nodeOutputInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNodeOutputInfoFieldBuilder() : null;
} else {
nodeOutputInfoBuilder_.addAllMessages(other.nodeOutputInfo_);
}
}
}
if (graphInputNodeInfoBuilder_ == null) {
if (!other.graphInputNodeInfo_.isEmpty()) {
if (graphInputNodeInfo_.isEmpty()) {
graphInputNodeInfo_ = other.graphInputNodeInfo_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.addAll(other.graphInputNodeInfo_);
}
onChanged();
}
} else {
if (!other.graphInputNodeInfo_.isEmpty()) {
if (graphInputNodeInfoBuilder_.isEmpty()) {
graphInputNodeInfoBuilder_.dispose();
graphInputNodeInfoBuilder_ = null;
graphInputNodeInfo_ = other.graphInputNodeInfo_;
bitField0_ = (bitField0_ & ~0x00000010);
graphInputNodeInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getGraphInputNodeInfoFieldBuilder() : null;
} else {
graphInputNodeInfoBuilder_.addAllMessages(other.graphInputNodeInfo_);
}
}
}
if (graphOutputNodeInfoBuilder_ == null) {
if (!other.graphOutputNodeInfo_.isEmpty()) {
if (graphOutputNodeInfo_.isEmpty()) {
graphOutputNodeInfo_ = other.graphOutputNodeInfo_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.addAll(other.graphOutputNodeInfo_);
}
onChanged();
}
} else {
if (!other.graphOutputNodeInfo_.isEmpty()) {
if (graphOutputNodeInfoBuilder_.isEmpty()) {
graphOutputNodeInfoBuilder_.dispose();
graphOutputNodeInfoBuilder_ = null;
graphOutputNodeInfo_ = other.graphOutputNodeInfo_;
bitField0_ = (bitField0_ & ~0x00000020);
graphOutputNodeInfoBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getGraphOutputNodeInfoFieldBuilder() : null;
} else {
graphOutputNodeInfoBuilder_.addAllMessages(other.graphOutputNodeInfo_);
}
}
}
if (other.destination_ != 0) {
setDestinationValue(other.getDestinationValue());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.GraphTransferInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.GraphTransferInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List nodeInfo_ =
java.util.Collections.emptyList();
private void ensureNodeInfoIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
nodeInfo_ = new java.util.ArrayList(nodeInfo_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeInfo, org.tensorflow.framework.GraphTransferNodeInfo.Builder, org.tensorflow.framework.GraphTransferNodeInfoOrBuilder> nodeInfoBuilder_;
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public java.util.List getNodeInfoList() {
if (nodeInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(nodeInfo_);
} else {
return nodeInfoBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public int getNodeInfoCount() {
if (nodeInfoBuilder_ == null) {
return nodeInfo_.size();
} else {
return nodeInfoBuilder_.getCount();
}
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public org.tensorflow.framework.GraphTransferNodeInfo getNodeInfo(int index) {
if (nodeInfoBuilder_ == null) {
return nodeInfo_.get(index);
} else {
return nodeInfoBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder setNodeInfo(
int index, org.tensorflow.framework.GraphTransferNodeInfo value) {
if (nodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeInfoIsMutable();
nodeInfo_.set(index, value);
onChanged();
} else {
nodeInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder setNodeInfo(
int index, org.tensorflow.framework.GraphTransferNodeInfo.Builder builderForValue) {
if (nodeInfoBuilder_ == null) {
ensureNodeInfoIsMutable();
nodeInfo_.set(index, builderForValue.build());
onChanged();
} else {
nodeInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder addNodeInfo(org.tensorflow.framework.GraphTransferNodeInfo value) {
if (nodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeInfoIsMutable();
nodeInfo_.add(value);
onChanged();
} else {
nodeInfoBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder addNodeInfo(
int index, org.tensorflow.framework.GraphTransferNodeInfo value) {
if (nodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeInfoIsMutable();
nodeInfo_.add(index, value);
onChanged();
} else {
nodeInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder addNodeInfo(
org.tensorflow.framework.GraphTransferNodeInfo.Builder builderForValue) {
if (nodeInfoBuilder_ == null) {
ensureNodeInfoIsMutable();
nodeInfo_.add(builderForValue.build());
onChanged();
} else {
nodeInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder addNodeInfo(
int index, org.tensorflow.framework.GraphTransferNodeInfo.Builder builderForValue) {
if (nodeInfoBuilder_ == null) {
ensureNodeInfoIsMutable();
nodeInfo_.add(index, builderForValue.build());
onChanged();
} else {
nodeInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder addAllNodeInfo(
java.lang.Iterable extends org.tensorflow.framework.GraphTransferNodeInfo> values) {
if (nodeInfoBuilder_ == null) {
ensureNodeInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nodeInfo_);
onChanged();
} else {
nodeInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder clearNodeInfo() {
if (nodeInfoBuilder_ == null) {
nodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
nodeInfoBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public Builder removeNodeInfo(int index) {
if (nodeInfoBuilder_ == null) {
ensureNodeInfoIsMutable();
nodeInfo_.remove(index);
onChanged();
} else {
nodeInfoBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public org.tensorflow.framework.GraphTransferNodeInfo.Builder getNodeInfoBuilder(
int index) {
return getNodeInfoFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public org.tensorflow.framework.GraphTransferNodeInfoOrBuilder getNodeInfoOrBuilder(
int index) {
if (nodeInfoBuilder_ == null) {
return nodeInfo_.get(index); } else {
return nodeInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferNodeInfoOrBuilder>
getNodeInfoOrBuilderList() {
if (nodeInfoBuilder_ != null) {
return nodeInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nodeInfo_);
}
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public org.tensorflow.framework.GraphTransferNodeInfo.Builder addNodeInfoBuilder() {
return getNodeInfoFieldBuilder().addBuilder(
org.tensorflow.framework.GraphTransferNodeInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public org.tensorflow.framework.GraphTransferNodeInfo.Builder addNodeInfoBuilder(
int index) {
return getNodeInfoFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphTransferNodeInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferNodeInfo node_info = 1;
*/
public java.util.List
getNodeInfoBuilderList() {
return getNodeInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeInfo, org.tensorflow.framework.GraphTransferNodeInfo.Builder, org.tensorflow.framework.GraphTransferNodeInfoOrBuilder>
getNodeInfoFieldBuilder() {
if (nodeInfoBuilder_ == null) {
nodeInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeInfo, org.tensorflow.framework.GraphTransferNodeInfo.Builder, org.tensorflow.framework.GraphTransferNodeInfoOrBuilder>(
nodeInfo_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
nodeInfo_ = null;
}
return nodeInfoBuilder_;
}
private java.util.List constNodeInfo_ =
java.util.Collections.emptyList();
private void ensureConstNodeInfoIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
constNodeInfo_ = new java.util.ArrayList(constNodeInfo_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferConstNodeInfo, org.tensorflow.framework.GraphTransferConstNodeInfo.Builder, org.tensorflow.framework.GraphTransferConstNodeInfoOrBuilder> constNodeInfoBuilder_;
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public java.util.List getConstNodeInfoList() {
if (constNodeInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(constNodeInfo_);
} else {
return constNodeInfoBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public int getConstNodeInfoCount() {
if (constNodeInfoBuilder_ == null) {
return constNodeInfo_.size();
} else {
return constNodeInfoBuilder_.getCount();
}
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public org.tensorflow.framework.GraphTransferConstNodeInfo getConstNodeInfo(int index) {
if (constNodeInfoBuilder_ == null) {
return constNodeInfo_.get(index);
} else {
return constNodeInfoBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder setConstNodeInfo(
int index, org.tensorflow.framework.GraphTransferConstNodeInfo value) {
if (constNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConstNodeInfoIsMutable();
constNodeInfo_.set(index, value);
onChanged();
} else {
constNodeInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder setConstNodeInfo(
int index, org.tensorflow.framework.GraphTransferConstNodeInfo.Builder builderForValue) {
if (constNodeInfoBuilder_ == null) {
ensureConstNodeInfoIsMutable();
constNodeInfo_.set(index, builderForValue.build());
onChanged();
} else {
constNodeInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder addConstNodeInfo(org.tensorflow.framework.GraphTransferConstNodeInfo value) {
if (constNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConstNodeInfoIsMutable();
constNodeInfo_.add(value);
onChanged();
} else {
constNodeInfoBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder addConstNodeInfo(
int index, org.tensorflow.framework.GraphTransferConstNodeInfo value) {
if (constNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConstNodeInfoIsMutable();
constNodeInfo_.add(index, value);
onChanged();
} else {
constNodeInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder addConstNodeInfo(
org.tensorflow.framework.GraphTransferConstNodeInfo.Builder builderForValue) {
if (constNodeInfoBuilder_ == null) {
ensureConstNodeInfoIsMutable();
constNodeInfo_.add(builderForValue.build());
onChanged();
} else {
constNodeInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder addConstNodeInfo(
int index, org.tensorflow.framework.GraphTransferConstNodeInfo.Builder builderForValue) {
if (constNodeInfoBuilder_ == null) {
ensureConstNodeInfoIsMutable();
constNodeInfo_.add(index, builderForValue.build());
onChanged();
} else {
constNodeInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder addAllConstNodeInfo(
java.lang.Iterable extends org.tensorflow.framework.GraphTransferConstNodeInfo> values) {
if (constNodeInfoBuilder_ == null) {
ensureConstNodeInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, constNodeInfo_);
onChanged();
} else {
constNodeInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder clearConstNodeInfo() {
if (constNodeInfoBuilder_ == null) {
constNodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
constNodeInfoBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public Builder removeConstNodeInfo(int index) {
if (constNodeInfoBuilder_ == null) {
ensureConstNodeInfoIsMutable();
constNodeInfo_.remove(index);
onChanged();
} else {
constNodeInfoBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public org.tensorflow.framework.GraphTransferConstNodeInfo.Builder getConstNodeInfoBuilder(
int index) {
return getConstNodeInfoFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public org.tensorflow.framework.GraphTransferConstNodeInfoOrBuilder getConstNodeInfoOrBuilder(
int index) {
if (constNodeInfoBuilder_ == null) {
return constNodeInfo_.get(index); } else {
return constNodeInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferConstNodeInfoOrBuilder>
getConstNodeInfoOrBuilderList() {
if (constNodeInfoBuilder_ != null) {
return constNodeInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(constNodeInfo_);
}
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public org.tensorflow.framework.GraphTransferConstNodeInfo.Builder addConstNodeInfoBuilder() {
return getConstNodeInfoFieldBuilder().addBuilder(
org.tensorflow.framework.GraphTransferConstNodeInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public org.tensorflow.framework.GraphTransferConstNodeInfo.Builder addConstNodeInfoBuilder(
int index) {
return getConstNodeInfoFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphTransferConstNodeInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferConstNodeInfo const_node_info = 2;
*/
public java.util.List
getConstNodeInfoBuilderList() {
return getConstNodeInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferConstNodeInfo, org.tensorflow.framework.GraphTransferConstNodeInfo.Builder, org.tensorflow.framework.GraphTransferConstNodeInfoOrBuilder>
getConstNodeInfoFieldBuilder() {
if (constNodeInfoBuilder_ == null) {
constNodeInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferConstNodeInfo, org.tensorflow.framework.GraphTransferConstNodeInfo.Builder, org.tensorflow.framework.GraphTransferConstNodeInfoOrBuilder>(
constNodeInfo_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
constNodeInfo_ = null;
}
return constNodeInfoBuilder_;
}
private java.util.List nodeInputInfo_ =
java.util.Collections.emptyList();
private void ensureNodeInputInfoIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
nodeInputInfo_ = new java.util.ArrayList(nodeInputInfo_);
bitField0_ |= 0x00000004;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeInputInfo, org.tensorflow.framework.GraphTransferNodeInputInfo.Builder, org.tensorflow.framework.GraphTransferNodeInputInfoOrBuilder> nodeInputInfoBuilder_;
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public java.util.List getNodeInputInfoList() {
if (nodeInputInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(nodeInputInfo_);
} else {
return nodeInputInfoBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public int getNodeInputInfoCount() {
if (nodeInputInfoBuilder_ == null) {
return nodeInputInfo_.size();
} else {
return nodeInputInfoBuilder_.getCount();
}
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public org.tensorflow.framework.GraphTransferNodeInputInfo getNodeInputInfo(int index) {
if (nodeInputInfoBuilder_ == null) {
return nodeInputInfo_.get(index);
} else {
return nodeInputInfoBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder setNodeInputInfo(
int index, org.tensorflow.framework.GraphTransferNodeInputInfo value) {
if (nodeInputInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeInputInfoIsMutable();
nodeInputInfo_.set(index, value);
onChanged();
} else {
nodeInputInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder setNodeInputInfo(
int index, org.tensorflow.framework.GraphTransferNodeInputInfo.Builder builderForValue) {
if (nodeInputInfoBuilder_ == null) {
ensureNodeInputInfoIsMutable();
nodeInputInfo_.set(index, builderForValue.build());
onChanged();
} else {
nodeInputInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder addNodeInputInfo(org.tensorflow.framework.GraphTransferNodeInputInfo value) {
if (nodeInputInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeInputInfoIsMutable();
nodeInputInfo_.add(value);
onChanged();
} else {
nodeInputInfoBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder addNodeInputInfo(
int index, org.tensorflow.framework.GraphTransferNodeInputInfo value) {
if (nodeInputInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeInputInfoIsMutable();
nodeInputInfo_.add(index, value);
onChanged();
} else {
nodeInputInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder addNodeInputInfo(
org.tensorflow.framework.GraphTransferNodeInputInfo.Builder builderForValue) {
if (nodeInputInfoBuilder_ == null) {
ensureNodeInputInfoIsMutable();
nodeInputInfo_.add(builderForValue.build());
onChanged();
} else {
nodeInputInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder addNodeInputInfo(
int index, org.tensorflow.framework.GraphTransferNodeInputInfo.Builder builderForValue) {
if (nodeInputInfoBuilder_ == null) {
ensureNodeInputInfoIsMutable();
nodeInputInfo_.add(index, builderForValue.build());
onChanged();
} else {
nodeInputInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder addAllNodeInputInfo(
java.lang.Iterable extends org.tensorflow.framework.GraphTransferNodeInputInfo> values) {
if (nodeInputInfoBuilder_ == null) {
ensureNodeInputInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nodeInputInfo_);
onChanged();
} else {
nodeInputInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder clearNodeInputInfo() {
if (nodeInputInfoBuilder_ == null) {
nodeInputInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
} else {
nodeInputInfoBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public Builder removeNodeInputInfo(int index) {
if (nodeInputInfoBuilder_ == null) {
ensureNodeInputInfoIsMutable();
nodeInputInfo_.remove(index);
onChanged();
} else {
nodeInputInfoBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public org.tensorflow.framework.GraphTransferNodeInputInfo.Builder getNodeInputInfoBuilder(
int index) {
return getNodeInputInfoFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public org.tensorflow.framework.GraphTransferNodeInputInfoOrBuilder getNodeInputInfoOrBuilder(
int index) {
if (nodeInputInfoBuilder_ == null) {
return nodeInputInfo_.get(index); } else {
return nodeInputInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferNodeInputInfoOrBuilder>
getNodeInputInfoOrBuilderList() {
if (nodeInputInfoBuilder_ != null) {
return nodeInputInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nodeInputInfo_);
}
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public org.tensorflow.framework.GraphTransferNodeInputInfo.Builder addNodeInputInfoBuilder() {
return getNodeInputInfoFieldBuilder().addBuilder(
org.tensorflow.framework.GraphTransferNodeInputInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public org.tensorflow.framework.GraphTransferNodeInputInfo.Builder addNodeInputInfoBuilder(
int index) {
return getNodeInputInfoFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphTransferNodeInputInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferNodeInputInfo node_input_info = 3;
*/
public java.util.List
getNodeInputInfoBuilderList() {
return getNodeInputInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeInputInfo, org.tensorflow.framework.GraphTransferNodeInputInfo.Builder, org.tensorflow.framework.GraphTransferNodeInputInfoOrBuilder>
getNodeInputInfoFieldBuilder() {
if (nodeInputInfoBuilder_ == null) {
nodeInputInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeInputInfo, org.tensorflow.framework.GraphTransferNodeInputInfo.Builder, org.tensorflow.framework.GraphTransferNodeInputInfoOrBuilder>(
nodeInputInfo_,
((bitField0_ & 0x00000004) == 0x00000004),
getParentForChildren(),
isClean());
nodeInputInfo_ = null;
}
return nodeInputInfoBuilder_;
}
private java.util.List nodeOutputInfo_ =
java.util.Collections.emptyList();
private void ensureNodeOutputInfoIsMutable() {
if (!((bitField0_ & 0x00000008) == 0x00000008)) {
nodeOutputInfo_ = new java.util.ArrayList(nodeOutputInfo_);
bitField0_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeOutputInfo, org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder, org.tensorflow.framework.GraphTransferNodeOutputInfoOrBuilder> nodeOutputInfoBuilder_;
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public java.util.List getNodeOutputInfoList() {
if (nodeOutputInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(nodeOutputInfo_);
} else {
return nodeOutputInfoBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public int getNodeOutputInfoCount() {
if (nodeOutputInfoBuilder_ == null) {
return nodeOutputInfo_.size();
} else {
return nodeOutputInfoBuilder_.getCount();
}
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public org.tensorflow.framework.GraphTransferNodeOutputInfo getNodeOutputInfo(int index) {
if (nodeOutputInfoBuilder_ == null) {
return nodeOutputInfo_.get(index);
} else {
return nodeOutputInfoBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder setNodeOutputInfo(
int index, org.tensorflow.framework.GraphTransferNodeOutputInfo value) {
if (nodeOutputInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.set(index, value);
onChanged();
} else {
nodeOutputInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder setNodeOutputInfo(
int index, org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder builderForValue) {
if (nodeOutputInfoBuilder_ == null) {
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.set(index, builderForValue.build());
onChanged();
} else {
nodeOutputInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder addNodeOutputInfo(org.tensorflow.framework.GraphTransferNodeOutputInfo value) {
if (nodeOutputInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.add(value);
onChanged();
} else {
nodeOutputInfoBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder addNodeOutputInfo(
int index, org.tensorflow.framework.GraphTransferNodeOutputInfo value) {
if (nodeOutputInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.add(index, value);
onChanged();
} else {
nodeOutputInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder addNodeOutputInfo(
org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder builderForValue) {
if (nodeOutputInfoBuilder_ == null) {
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.add(builderForValue.build());
onChanged();
} else {
nodeOutputInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder addNodeOutputInfo(
int index, org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder builderForValue) {
if (nodeOutputInfoBuilder_ == null) {
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.add(index, builderForValue.build());
onChanged();
} else {
nodeOutputInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder addAllNodeOutputInfo(
java.lang.Iterable extends org.tensorflow.framework.GraphTransferNodeOutputInfo> values) {
if (nodeOutputInfoBuilder_ == null) {
ensureNodeOutputInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nodeOutputInfo_);
onChanged();
} else {
nodeOutputInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder clearNodeOutputInfo() {
if (nodeOutputInfoBuilder_ == null) {
nodeOutputInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
} else {
nodeOutputInfoBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public Builder removeNodeOutputInfo(int index) {
if (nodeOutputInfoBuilder_ == null) {
ensureNodeOutputInfoIsMutable();
nodeOutputInfo_.remove(index);
onChanged();
} else {
nodeOutputInfoBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder getNodeOutputInfoBuilder(
int index) {
return getNodeOutputInfoFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public org.tensorflow.framework.GraphTransferNodeOutputInfoOrBuilder getNodeOutputInfoOrBuilder(
int index) {
if (nodeOutputInfoBuilder_ == null) {
return nodeOutputInfo_.get(index); } else {
return nodeOutputInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferNodeOutputInfoOrBuilder>
getNodeOutputInfoOrBuilderList() {
if (nodeOutputInfoBuilder_ != null) {
return nodeOutputInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nodeOutputInfo_);
}
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder addNodeOutputInfoBuilder() {
return getNodeOutputInfoFieldBuilder().addBuilder(
org.tensorflow.framework.GraphTransferNodeOutputInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder addNodeOutputInfoBuilder(
int index) {
return getNodeOutputInfoFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphTransferNodeOutputInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferNodeOutputInfo node_output_info = 4;
*/
public java.util.List
getNodeOutputInfoBuilderList() {
return getNodeOutputInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeOutputInfo, org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder, org.tensorflow.framework.GraphTransferNodeOutputInfoOrBuilder>
getNodeOutputInfoFieldBuilder() {
if (nodeOutputInfoBuilder_ == null) {
nodeOutputInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferNodeOutputInfo, org.tensorflow.framework.GraphTransferNodeOutputInfo.Builder, org.tensorflow.framework.GraphTransferNodeOutputInfoOrBuilder>(
nodeOutputInfo_,
((bitField0_ & 0x00000008) == 0x00000008),
getParentForChildren(),
isClean());
nodeOutputInfo_ = null;
}
return nodeOutputInfoBuilder_;
}
private java.util.List graphInputNodeInfo_ =
java.util.Collections.emptyList();
private void ensureGraphInputNodeInfoIsMutable() {
if (!((bitField0_ & 0x00000010) == 0x00000010)) {
graphInputNodeInfo_ = new java.util.ArrayList(graphInputNodeInfo_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferGraphInputNodeInfo, org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder, org.tensorflow.framework.GraphTransferGraphInputNodeInfoOrBuilder> graphInputNodeInfoBuilder_;
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public java.util.List getGraphInputNodeInfoList() {
if (graphInputNodeInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(graphInputNodeInfo_);
} else {
return graphInputNodeInfoBuilder_.getMessageList();
}
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public int getGraphInputNodeInfoCount() {
if (graphInputNodeInfoBuilder_ == null) {
return graphInputNodeInfo_.size();
} else {
return graphInputNodeInfoBuilder_.getCount();
}
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public org.tensorflow.framework.GraphTransferGraphInputNodeInfo getGraphInputNodeInfo(int index) {
if (graphInputNodeInfoBuilder_ == null) {
return graphInputNodeInfo_.get(index);
} else {
return graphInputNodeInfoBuilder_.getMessage(index);
}
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder setGraphInputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphInputNodeInfo value) {
if (graphInputNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.set(index, value);
onChanged();
} else {
graphInputNodeInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder setGraphInputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder builderForValue) {
if (graphInputNodeInfoBuilder_ == null) {
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.set(index, builderForValue.build());
onChanged();
} else {
graphInputNodeInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder addGraphInputNodeInfo(org.tensorflow.framework.GraphTransferGraphInputNodeInfo value) {
if (graphInputNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.add(value);
onChanged();
} else {
graphInputNodeInfoBuilder_.addMessage(value);
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder addGraphInputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphInputNodeInfo value) {
if (graphInputNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.add(index, value);
onChanged();
} else {
graphInputNodeInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder addGraphInputNodeInfo(
org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder builderForValue) {
if (graphInputNodeInfoBuilder_ == null) {
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.add(builderForValue.build());
onChanged();
} else {
graphInputNodeInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder addGraphInputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder builderForValue) {
if (graphInputNodeInfoBuilder_ == null) {
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.add(index, builderForValue.build());
onChanged();
} else {
graphInputNodeInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder addAllGraphInputNodeInfo(
java.lang.Iterable extends org.tensorflow.framework.GraphTransferGraphInputNodeInfo> values) {
if (graphInputNodeInfoBuilder_ == null) {
ensureGraphInputNodeInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, graphInputNodeInfo_);
onChanged();
} else {
graphInputNodeInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder clearGraphInputNodeInfo() {
if (graphInputNodeInfoBuilder_ == null) {
graphInputNodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
graphInputNodeInfoBuilder_.clear();
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public Builder removeGraphInputNodeInfo(int index) {
if (graphInputNodeInfoBuilder_ == null) {
ensureGraphInputNodeInfoIsMutable();
graphInputNodeInfo_.remove(index);
onChanged();
} else {
graphInputNodeInfoBuilder_.remove(index);
}
return this;
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder getGraphInputNodeInfoBuilder(
int index) {
return getGraphInputNodeInfoFieldBuilder().getBuilder(index);
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public org.tensorflow.framework.GraphTransferGraphInputNodeInfoOrBuilder getGraphInputNodeInfoOrBuilder(
int index) {
if (graphInputNodeInfoBuilder_ == null) {
return graphInputNodeInfo_.get(index); } else {
return graphInputNodeInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferGraphInputNodeInfoOrBuilder>
getGraphInputNodeInfoOrBuilderList() {
if (graphInputNodeInfoBuilder_ != null) {
return graphInputNodeInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(graphInputNodeInfo_);
}
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder addGraphInputNodeInfoBuilder() {
return getGraphInputNodeInfoFieldBuilder().addBuilder(
org.tensorflow.framework.GraphTransferGraphInputNodeInfo.getDefaultInstance());
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder addGraphInputNodeInfoBuilder(
int index) {
return getGraphInputNodeInfoFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphTransferGraphInputNodeInfo.getDefaultInstance());
}
/**
*
* Input Node parameters of transferred graph
*
*
* repeated .tensorflow.GraphTransferGraphInputNodeInfo graph_input_node_info = 5;
*/
public java.util.List
getGraphInputNodeInfoBuilderList() {
return getGraphInputNodeInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferGraphInputNodeInfo, org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder, org.tensorflow.framework.GraphTransferGraphInputNodeInfoOrBuilder>
getGraphInputNodeInfoFieldBuilder() {
if (graphInputNodeInfoBuilder_ == null) {
graphInputNodeInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferGraphInputNodeInfo, org.tensorflow.framework.GraphTransferGraphInputNodeInfo.Builder, org.tensorflow.framework.GraphTransferGraphInputNodeInfoOrBuilder>(
graphInputNodeInfo_,
((bitField0_ & 0x00000010) == 0x00000010),
getParentForChildren(),
isClean());
graphInputNodeInfo_ = null;
}
return graphInputNodeInfoBuilder_;
}
private java.util.List graphOutputNodeInfo_ =
java.util.Collections.emptyList();
private void ensureGraphOutputNodeInfoIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
graphOutputNodeInfo_ = new java.util.ArrayList(graphOutputNodeInfo_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferGraphOutputNodeInfo, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder, org.tensorflow.framework.GraphTransferGraphOutputNodeInfoOrBuilder> graphOutputNodeInfoBuilder_;
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public java.util.List getGraphOutputNodeInfoList() {
if (graphOutputNodeInfoBuilder_ == null) {
return java.util.Collections.unmodifiableList(graphOutputNodeInfo_);
} else {
return graphOutputNodeInfoBuilder_.getMessageList();
}
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public int getGraphOutputNodeInfoCount() {
if (graphOutputNodeInfoBuilder_ == null) {
return graphOutputNodeInfo_.size();
} else {
return graphOutputNodeInfoBuilder_.getCount();
}
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public org.tensorflow.framework.GraphTransferGraphOutputNodeInfo getGraphOutputNodeInfo(int index) {
if (graphOutputNodeInfoBuilder_ == null) {
return graphOutputNodeInfo_.get(index);
} else {
return graphOutputNodeInfoBuilder_.getMessage(index);
}
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder setGraphOutputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo value) {
if (graphOutputNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.set(index, value);
onChanged();
} else {
graphOutputNodeInfoBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder setGraphOutputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder builderForValue) {
if (graphOutputNodeInfoBuilder_ == null) {
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.set(index, builderForValue.build());
onChanged();
} else {
graphOutputNodeInfoBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder addGraphOutputNodeInfo(org.tensorflow.framework.GraphTransferGraphOutputNodeInfo value) {
if (graphOutputNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.add(value);
onChanged();
} else {
graphOutputNodeInfoBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder addGraphOutputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo value) {
if (graphOutputNodeInfoBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.add(index, value);
onChanged();
} else {
graphOutputNodeInfoBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder addGraphOutputNodeInfo(
org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder builderForValue) {
if (graphOutputNodeInfoBuilder_ == null) {
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.add(builderForValue.build());
onChanged();
} else {
graphOutputNodeInfoBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder addGraphOutputNodeInfo(
int index, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder builderForValue) {
if (graphOutputNodeInfoBuilder_ == null) {
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.add(index, builderForValue.build());
onChanged();
} else {
graphOutputNodeInfoBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder addAllGraphOutputNodeInfo(
java.lang.Iterable extends org.tensorflow.framework.GraphTransferGraphOutputNodeInfo> values) {
if (graphOutputNodeInfoBuilder_ == null) {
ensureGraphOutputNodeInfoIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, graphOutputNodeInfo_);
onChanged();
} else {
graphOutputNodeInfoBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder clearGraphOutputNodeInfo() {
if (graphOutputNodeInfoBuilder_ == null) {
graphOutputNodeInfo_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
graphOutputNodeInfoBuilder_.clear();
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public Builder removeGraphOutputNodeInfo(int index) {
if (graphOutputNodeInfoBuilder_ == null) {
ensureGraphOutputNodeInfoIsMutable();
graphOutputNodeInfo_.remove(index);
onChanged();
} else {
graphOutputNodeInfoBuilder_.remove(index);
}
return this;
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder getGraphOutputNodeInfoBuilder(
int index) {
return getGraphOutputNodeInfoFieldBuilder().getBuilder(index);
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public org.tensorflow.framework.GraphTransferGraphOutputNodeInfoOrBuilder getGraphOutputNodeInfoOrBuilder(
int index) {
if (graphOutputNodeInfoBuilder_ == null) {
return graphOutputNodeInfo_.get(index); } else {
return graphOutputNodeInfoBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public java.util.List extends org.tensorflow.framework.GraphTransferGraphOutputNodeInfoOrBuilder>
getGraphOutputNodeInfoOrBuilderList() {
if (graphOutputNodeInfoBuilder_ != null) {
return graphOutputNodeInfoBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(graphOutputNodeInfo_);
}
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder addGraphOutputNodeInfoBuilder() {
return getGraphOutputNodeInfoFieldBuilder().addBuilder(
org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder addGraphOutputNodeInfoBuilder(
int index) {
return getGraphOutputNodeInfoFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.getDefaultInstance());
}
/**
* repeated .tensorflow.GraphTransferGraphOutputNodeInfo graph_output_node_info = 6;
*/
public java.util.List
getGraphOutputNodeInfoBuilderList() {
return getGraphOutputNodeInfoFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferGraphOutputNodeInfo, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder, org.tensorflow.framework.GraphTransferGraphOutputNodeInfoOrBuilder>
getGraphOutputNodeInfoFieldBuilder() {
if (graphOutputNodeInfoBuilder_ == null) {
graphOutputNodeInfoBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphTransferGraphOutputNodeInfo, org.tensorflow.framework.GraphTransferGraphOutputNodeInfo.Builder, org.tensorflow.framework.GraphTransferGraphOutputNodeInfoOrBuilder>(
graphOutputNodeInfo_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
graphOutputNodeInfo_ = null;
}
return graphOutputNodeInfoBuilder_;
}
private int destination_ = 0;
/**
*
* Destination of graph transfer
*
*
* .tensorflow.GraphTransferInfo.Destination destination = 7;
*/
public int getDestinationValue() {
return destination_;
}
/**
*
* Destination of graph transfer
*
*
* .tensorflow.GraphTransferInfo.Destination destination = 7;
*/
public Builder setDestinationValue(int value) {
destination_ = value;
onChanged();
return this;
}
/**
*
* Destination of graph transfer
*
*
* .tensorflow.GraphTransferInfo.Destination destination = 7;
*/
public org.tensorflow.framework.GraphTransferInfo.Destination getDestination() {
org.tensorflow.framework.GraphTransferInfo.Destination result = org.tensorflow.framework.GraphTransferInfo.Destination.valueOf(destination_);
return result == null ? org.tensorflow.framework.GraphTransferInfo.Destination.UNRECOGNIZED : result;
}
/**
*
* Destination of graph transfer
*
*
* .tensorflow.GraphTransferInfo.Destination destination = 7;
*/
public Builder setDestination(org.tensorflow.framework.GraphTransferInfo.Destination value) {
if (value == null) {
throw new NullPointerException();
}
destination_ = value.getNumber();
onChanged();
return this;
}
/**
*
* Destination of graph transfer
*
*
* .tensorflow.GraphTransferInfo.Destination destination = 7;
*/
public Builder clearDestination() {
destination_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.GraphTransferInfo)
}
// @@protoc_insertion_point(class_scope:tensorflow.GraphTransferInfo)
private static final org.tensorflow.framework.GraphTransferInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.GraphTransferInfo();
}
public static org.tensorflow.framework.GraphTransferInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GraphTransferInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GraphTransferInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.GraphTransferInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy