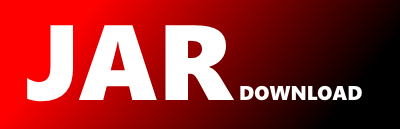
org.tensorflow.framework.CondContextDef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/control_flow.proto
package org.tensorflow.framework;
/**
*
* Protocol buffer representing a CondContext object.
*
*
* Protobuf type {@code tensorflow.CondContextDef}
*/
public final class CondContextDef extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.CondContextDef)
CondContextDefOrBuilder {
private static final long serialVersionUID = 0L;
// Use CondContextDef.newBuilder() to construct.
private CondContextDef(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CondContextDef() {
contextName_ = "";
predName_ = "";
pivotName_ = "";
branch_ = 0;
nestedContexts_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CondContextDef(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
contextName_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
predName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
pivotName_ = s;
break;
}
case 32: {
branch_ = input.readInt32();
break;
}
case 42: {
org.tensorflow.framework.ValuesDef.Builder subBuilder = null;
if (valuesDef_ != null) {
subBuilder = valuesDef_.toBuilder();
}
valuesDef_ = input.readMessage(org.tensorflow.framework.ValuesDef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(valuesDef_);
valuesDef_ = subBuilder.buildPartial();
}
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
nestedContexts_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
nestedContexts_.add(
input.readMessage(org.tensorflow.framework.ControlFlowContextDef.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
nestedContexts_ = java.util.Collections.unmodifiableList(nestedContexts_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.ControlFlowProtos.internal_static_tensorflow_CondContextDef_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.ControlFlowProtos.internal_static_tensorflow_CondContextDef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.CondContextDef.class, org.tensorflow.framework.CondContextDef.Builder.class);
}
private int bitField0_;
public static final int CONTEXT_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object contextName_;
/**
*
* Name of the context.
*
*
* string context_name = 1;
*/
public java.lang.String getContextName() {
java.lang.Object ref = contextName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contextName_ = s;
return s;
}
}
/**
*
* Name of the context.
*
*
* string context_name = 1;
*/
public com.google.protobuf.ByteString
getContextNameBytes() {
java.lang.Object ref = contextName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contextName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PRED_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object predName_;
/**
*
* Name of the pred tensor.
*
*
* string pred_name = 2;
*/
public java.lang.String getPredName() {
java.lang.Object ref = predName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
predName_ = s;
return s;
}
}
/**
*
* Name of the pred tensor.
*
*
* string pred_name = 2;
*/
public com.google.protobuf.ByteString
getPredNameBytes() {
java.lang.Object ref = predName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
predName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PIVOT_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object pivotName_;
/**
*
* Name of the pivot tensor.
*
*
* string pivot_name = 3;
*/
public java.lang.String getPivotName() {
java.lang.Object ref = pivotName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pivotName_ = s;
return s;
}
}
/**
*
* Name of the pivot tensor.
*
*
* string pivot_name = 3;
*/
public com.google.protobuf.ByteString
getPivotNameBytes() {
java.lang.Object ref = pivotName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pivotName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BRANCH_FIELD_NUMBER = 4;
private int branch_;
/**
*
* Branch prediction. 0 or 1.
*
*
* int32 branch = 4;
*/
public int getBranch() {
return branch_;
}
public static final int VALUES_DEF_FIELD_NUMBER = 5;
private org.tensorflow.framework.ValuesDef valuesDef_;
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public boolean hasValuesDef() {
return valuesDef_ != null;
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public org.tensorflow.framework.ValuesDef getValuesDef() {
return valuesDef_ == null ? org.tensorflow.framework.ValuesDef.getDefaultInstance() : valuesDef_;
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public org.tensorflow.framework.ValuesDefOrBuilder getValuesDefOrBuilder() {
return getValuesDef();
}
public static final int NESTED_CONTEXTS_FIELD_NUMBER = 6;
private java.util.List nestedContexts_;
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public java.util.List getNestedContextsList() {
return nestedContexts_;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public java.util.List extends org.tensorflow.framework.ControlFlowContextDefOrBuilder>
getNestedContextsOrBuilderList() {
return nestedContexts_;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public int getNestedContextsCount() {
return nestedContexts_.size();
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public org.tensorflow.framework.ControlFlowContextDef getNestedContexts(int index) {
return nestedContexts_.get(index);
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public org.tensorflow.framework.ControlFlowContextDefOrBuilder getNestedContextsOrBuilder(
int index) {
return nestedContexts_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getContextNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, contextName_);
}
if (!getPredNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, predName_);
}
if (!getPivotNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, pivotName_);
}
if (branch_ != 0) {
output.writeInt32(4, branch_);
}
if (valuesDef_ != null) {
output.writeMessage(5, getValuesDef());
}
for (int i = 0; i < nestedContexts_.size(); i++) {
output.writeMessage(6, nestedContexts_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getContextNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, contextName_);
}
if (!getPredNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, predName_);
}
if (!getPivotNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, pivotName_);
}
if (branch_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, branch_);
}
if (valuesDef_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getValuesDef());
}
for (int i = 0; i < nestedContexts_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, nestedContexts_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.CondContextDef)) {
return super.equals(obj);
}
org.tensorflow.framework.CondContextDef other = (org.tensorflow.framework.CondContextDef) obj;
boolean result = true;
result = result && getContextName()
.equals(other.getContextName());
result = result && getPredName()
.equals(other.getPredName());
result = result && getPivotName()
.equals(other.getPivotName());
result = result && (getBranch()
== other.getBranch());
result = result && (hasValuesDef() == other.hasValuesDef());
if (hasValuesDef()) {
result = result && getValuesDef()
.equals(other.getValuesDef());
}
result = result && getNestedContextsList()
.equals(other.getNestedContextsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONTEXT_NAME_FIELD_NUMBER;
hash = (53 * hash) + getContextName().hashCode();
hash = (37 * hash) + PRED_NAME_FIELD_NUMBER;
hash = (53 * hash) + getPredName().hashCode();
hash = (37 * hash) + PIVOT_NAME_FIELD_NUMBER;
hash = (53 * hash) + getPivotName().hashCode();
hash = (37 * hash) + BRANCH_FIELD_NUMBER;
hash = (53 * hash) + getBranch();
if (hasValuesDef()) {
hash = (37 * hash) + VALUES_DEF_FIELD_NUMBER;
hash = (53 * hash) + getValuesDef().hashCode();
}
if (getNestedContextsCount() > 0) {
hash = (37 * hash) + NESTED_CONTEXTS_FIELD_NUMBER;
hash = (53 * hash) + getNestedContextsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.CondContextDef parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.CondContextDef parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.CondContextDef parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.CondContextDef parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.CondContextDef parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.CondContextDef parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.CondContextDef parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.CondContextDef parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.CondContextDef parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.CondContextDef parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.CondContextDef parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.CondContextDef parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.CondContextDef prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Protocol buffer representing a CondContext object.
*
*
* Protobuf type {@code tensorflow.CondContextDef}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.CondContextDef)
org.tensorflow.framework.CondContextDefOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.ControlFlowProtos.internal_static_tensorflow_CondContextDef_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.ControlFlowProtos.internal_static_tensorflow_CondContextDef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.CondContextDef.class, org.tensorflow.framework.CondContextDef.Builder.class);
}
// Construct using org.tensorflow.framework.CondContextDef.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNestedContextsFieldBuilder();
}
}
public Builder clear() {
super.clear();
contextName_ = "";
predName_ = "";
pivotName_ = "";
branch_ = 0;
if (valuesDefBuilder_ == null) {
valuesDef_ = null;
} else {
valuesDef_ = null;
valuesDefBuilder_ = null;
}
if (nestedContextsBuilder_ == null) {
nestedContexts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
nestedContextsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.ControlFlowProtos.internal_static_tensorflow_CondContextDef_descriptor;
}
public org.tensorflow.framework.CondContextDef getDefaultInstanceForType() {
return org.tensorflow.framework.CondContextDef.getDefaultInstance();
}
public org.tensorflow.framework.CondContextDef build() {
org.tensorflow.framework.CondContextDef result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.CondContextDef buildPartial() {
org.tensorflow.framework.CondContextDef result = new org.tensorflow.framework.CondContextDef(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.contextName_ = contextName_;
result.predName_ = predName_;
result.pivotName_ = pivotName_;
result.branch_ = branch_;
if (valuesDefBuilder_ == null) {
result.valuesDef_ = valuesDef_;
} else {
result.valuesDef_ = valuesDefBuilder_.build();
}
if (nestedContextsBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
nestedContexts_ = java.util.Collections.unmodifiableList(nestedContexts_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.nestedContexts_ = nestedContexts_;
} else {
result.nestedContexts_ = nestedContextsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.CondContextDef) {
return mergeFrom((org.tensorflow.framework.CondContextDef)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.CondContextDef other) {
if (other == org.tensorflow.framework.CondContextDef.getDefaultInstance()) return this;
if (!other.getContextName().isEmpty()) {
contextName_ = other.contextName_;
onChanged();
}
if (!other.getPredName().isEmpty()) {
predName_ = other.predName_;
onChanged();
}
if (!other.getPivotName().isEmpty()) {
pivotName_ = other.pivotName_;
onChanged();
}
if (other.getBranch() != 0) {
setBranch(other.getBranch());
}
if (other.hasValuesDef()) {
mergeValuesDef(other.getValuesDef());
}
if (nestedContextsBuilder_ == null) {
if (!other.nestedContexts_.isEmpty()) {
if (nestedContexts_.isEmpty()) {
nestedContexts_ = other.nestedContexts_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureNestedContextsIsMutable();
nestedContexts_.addAll(other.nestedContexts_);
}
onChanged();
}
} else {
if (!other.nestedContexts_.isEmpty()) {
if (nestedContextsBuilder_.isEmpty()) {
nestedContextsBuilder_.dispose();
nestedContextsBuilder_ = null;
nestedContexts_ = other.nestedContexts_;
bitField0_ = (bitField0_ & ~0x00000020);
nestedContextsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNestedContextsFieldBuilder() : null;
} else {
nestedContextsBuilder_.addAllMessages(other.nestedContexts_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.CondContextDef parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.CondContextDef) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object contextName_ = "";
/**
*
* Name of the context.
*
*
* string context_name = 1;
*/
public java.lang.String getContextName() {
java.lang.Object ref = contextName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
contextName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the context.
*
*
* string context_name = 1;
*/
public com.google.protobuf.ByteString
getContextNameBytes() {
java.lang.Object ref = contextName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
contextName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the context.
*
*
* string context_name = 1;
*/
public Builder setContextName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
contextName_ = value;
onChanged();
return this;
}
/**
*
* Name of the context.
*
*
* string context_name = 1;
*/
public Builder clearContextName() {
contextName_ = getDefaultInstance().getContextName();
onChanged();
return this;
}
/**
*
* Name of the context.
*
*
* string context_name = 1;
*/
public Builder setContextNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
contextName_ = value;
onChanged();
return this;
}
private java.lang.Object predName_ = "";
/**
*
* Name of the pred tensor.
*
*
* string pred_name = 2;
*/
public java.lang.String getPredName() {
java.lang.Object ref = predName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
predName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the pred tensor.
*
*
* string pred_name = 2;
*/
public com.google.protobuf.ByteString
getPredNameBytes() {
java.lang.Object ref = predName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
predName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the pred tensor.
*
*
* string pred_name = 2;
*/
public Builder setPredName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
predName_ = value;
onChanged();
return this;
}
/**
*
* Name of the pred tensor.
*
*
* string pred_name = 2;
*/
public Builder clearPredName() {
predName_ = getDefaultInstance().getPredName();
onChanged();
return this;
}
/**
*
* Name of the pred tensor.
*
*
* string pred_name = 2;
*/
public Builder setPredNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
predName_ = value;
onChanged();
return this;
}
private java.lang.Object pivotName_ = "";
/**
*
* Name of the pivot tensor.
*
*
* string pivot_name = 3;
*/
public java.lang.String getPivotName() {
java.lang.Object ref = pivotName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
pivotName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of the pivot tensor.
*
*
* string pivot_name = 3;
*/
public com.google.protobuf.ByteString
getPivotNameBytes() {
java.lang.Object ref = pivotName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
pivotName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of the pivot tensor.
*
*
* string pivot_name = 3;
*/
public Builder setPivotName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
pivotName_ = value;
onChanged();
return this;
}
/**
*
* Name of the pivot tensor.
*
*
* string pivot_name = 3;
*/
public Builder clearPivotName() {
pivotName_ = getDefaultInstance().getPivotName();
onChanged();
return this;
}
/**
*
* Name of the pivot tensor.
*
*
* string pivot_name = 3;
*/
public Builder setPivotNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
pivotName_ = value;
onChanged();
return this;
}
private int branch_ ;
/**
*
* Branch prediction. 0 or 1.
*
*
* int32 branch = 4;
*/
public int getBranch() {
return branch_;
}
/**
*
* Branch prediction. 0 or 1.
*
*
* int32 branch = 4;
*/
public Builder setBranch(int value) {
branch_ = value;
onChanged();
return this;
}
/**
*
* Branch prediction. 0 or 1.
*
*
* int32 branch = 4;
*/
public Builder clearBranch() {
branch_ = 0;
onChanged();
return this;
}
private org.tensorflow.framework.ValuesDef valuesDef_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.ValuesDef, org.tensorflow.framework.ValuesDef.Builder, org.tensorflow.framework.ValuesDefOrBuilder> valuesDefBuilder_;
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public boolean hasValuesDef() {
return valuesDefBuilder_ != null || valuesDef_ != null;
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public org.tensorflow.framework.ValuesDef getValuesDef() {
if (valuesDefBuilder_ == null) {
return valuesDef_ == null ? org.tensorflow.framework.ValuesDef.getDefaultInstance() : valuesDef_;
} else {
return valuesDefBuilder_.getMessage();
}
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public Builder setValuesDef(org.tensorflow.framework.ValuesDef value) {
if (valuesDefBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
valuesDef_ = value;
onChanged();
} else {
valuesDefBuilder_.setMessage(value);
}
return this;
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public Builder setValuesDef(
org.tensorflow.framework.ValuesDef.Builder builderForValue) {
if (valuesDefBuilder_ == null) {
valuesDef_ = builderForValue.build();
onChanged();
} else {
valuesDefBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public Builder mergeValuesDef(org.tensorflow.framework.ValuesDef value) {
if (valuesDefBuilder_ == null) {
if (valuesDef_ != null) {
valuesDef_ =
org.tensorflow.framework.ValuesDef.newBuilder(valuesDef_).mergeFrom(value).buildPartial();
} else {
valuesDef_ = value;
}
onChanged();
} else {
valuesDefBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public Builder clearValuesDef() {
if (valuesDefBuilder_ == null) {
valuesDef_ = null;
onChanged();
} else {
valuesDef_ = null;
valuesDefBuilder_ = null;
}
return this;
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public org.tensorflow.framework.ValuesDef.Builder getValuesDefBuilder() {
onChanged();
return getValuesDefFieldBuilder().getBuilder();
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
public org.tensorflow.framework.ValuesDefOrBuilder getValuesDefOrBuilder() {
if (valuesDefBuilder_ != null) {
return valuesDefBuilder_.getMessageOrBuilder();
} else {
return valuesDef_ == null ?
org.tensorflow.framework.ValuesDef.getDefaultInstance() : valuesDef_;
}
}
/**
*
* Values and external values in control flow context.
*
*
* .tensorflow.ValuesDef values_def = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.ValuesDef, org.tensorflow.framework.ValuesDef.Builder, org.tensorflow.framework.ValuesDefOrBuilder>
getValuesDefFieldBuilder() {
if (valuesDefBuilder_ == null) {
valuesDefBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.ValuesDef, org.tensorflow.framework.ValuesDef.Builder, org.tensorflow.framework.ValuesDefOrBuilder>(
getValuesDef(),
getParentForChildren(),
isClean());
valuesDef_ = null;
}
return valuesDefBuilder_;
}
private java.util.List nestedContexts_ =
java.util.Collections.emptyList();
private void ensureNestedContextsIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
nestedContexts_ = new java.util.ArrayList(nestedContexts_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.ControlFlowContextDef, org.tensorflow.framework.ControlFlowContextDef.Builder, org.tensorflow.framework.ControlFlowContextDefOrBuilder> nestedContextsBuilder_;
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public java.util.List getNestedContextsList() {
if (nestedContextsBuilder_ == null) {
return java.util.Collections.unmodifiableList(nestedContexts_);
} else {
return nestedContextsBuilder_.getMessageList();
}
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public int getNestedContextsCount() {
if (nestedContextsBuilder_ == null) {
return nestedContexts_.size();
} else {
return nestedContextsBuilder_.getCount();
}
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public org.tensorflow.framework.ControlFlowContextDef getNestedContexts(int index) {
if (nestedContextsBuilder_ == null) {
return nestedContexts_.get(index);
} else {
return nestedContextsBuilder_.getMessage(index);
}
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder setNestedContexts(
int index, org.tensorflow.framework.ControlFlowContextDef value) {
if (nestedContextsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNestedContextsIsMutable();
nestedContexts_.set(index, value);
onChanged();
} else {
nestedContextsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder setNestedContexts(
int index, org.tensorflow.framework.ControlFlowContextDef.Builder builderForValue) {
if (nestedContextsBuilder_ == null) {
ensureNestedContextsIsMutable();
nestedContexts_.set(index, builderForValue.build());
onChanged();
} else {
nestedContextsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder addNestedContexts(org.tensorflow.framework.ControlFlowContextDef value) {
if (nestedContextsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNestedContextsIsMutable();
nestedContexts_.add(value);
onChanged();
} else {
nestedContextsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder addNestedContexts(
int index, org.tensorflow.framework.ControlFlowContextDef value) {
if (nestedContextsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNestedContextsIsMutable();
nestedContexts_.add(index, value);
onChanged();
} else {
nestedContextsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder addNestedContexts(
org.tensorflow.framework.ControlFlowContextDef.Builder builderForValue) {
if (nestedContextsBuilder_ == null) {
ensureNestedContextsIsMutable();
nestedContexts_.add(builderForValue.build());
onChanged();
} else {
nestedContextsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder addNestedContexts(
int index, org.tensorflow.framework.ControlFlowContextDef.Builder builderForValue) {
if (nestedContextsBuilder_ == null) {
ensureNestedContextsIsMutable();
nestedContexts_.add(index, builderForValue.build());
onChanged();
} else {
nestedContextsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder addAllNestedContexts(
java.lang.Iterable extends org.tensorflow.framework.ControlFlowContextDef> values) {
if (nestedContextsBuilder_ == null) {
ensureNestedContextsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nestedContexts_);
onChanged();
} else {
nestedContextsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder clearNestedContexts() {
if (nestedContextsBuilder_ == null) {
nestedContexts_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
nestedContextsBuilder_.clear();
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public Builder removeNestedContexts(int index) {
if (nestedContextsBuilder_ == null) {
ensureNestedContextsIsMutable();
nestedContexts_.remove(index);
onChanged();
} else {
nestedContextsBuilder_.remove(index);
}
return this;
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public org.tensorflow.framework.ControlFlowContextDef.Builder getNestedContextsBuilder(
int index) {
return getNestedContextsFieldBuilder().getBuilder(index);
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public org.tensorflow.framework.ControlFlowContextDefOrBuilder getNestedContextsOrBuilder(
int index) {
if (nestedContextsBuilder_ == null) {
return nestedContexts_.get(index); } else {
return nestedContextsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public java.util.List extends org.tensorflow.framework.ControlFlowContextDefOrBuilder>
getNestedContextsOrBuilderList() {
if (nestedContextsBuilder_ != null) {
return nestedContextsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nestedContexts_);
}
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public org.tensorflow.framework.ControlFlowContextDef.Builder addNestedContextsBuilder() {
return getNestedContextsFieldBuilder().addBuilder(
org.tensorflow.framework.ControlFlowContextDef.getDefaultInstance());
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public org.tensorflow.framework.ControlFlowContextDef.Builder addNestedContextsBuilder(
int index) {
return getNestedContextsFieldBuilder().addBuilder(
index, org.tensorflow.framework.ControlFlowContextDef.getDefaultInstance());
}
/**
*
* Contexts contained inside this context (e.g. nested conds).
*
*
* repeated .tensorflow.ControlFlowContextDef nested_contexts = 6;
*/
public java.util.List
getNestedContextsBuilderList() {
return getNestedContextsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.ControlFlowContextDef, org.tensorflow.framework.ControlFlowContextDef.Builder, org.tensorflow.framework.ControlFlowContextDefOrBuilder>
getNestedContextsFieldBuilder() {
if (nestedContextsBuilder_ == null) {
nestedContextsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.ControlFlowContextDef, org.tensorflow.framework.ControlFlowContextDef.Builder, org.tensorflow.framework.ControlFlowContextDefOrBuilder>(
nestedContexts_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
nestedContexts_ = null;
}
return nestedContextsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.CondContextDef)
}
// @@protoc_insertion_point(class_scope:tensorflow.CondContextDef)
private static final org.tensorflow.framework.CondContextDef DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.CondContextDef();
}
public static org.tensorflow.framework.CondContextDef getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public CondContextDef parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CondContextDef(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.CondContextDef getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy