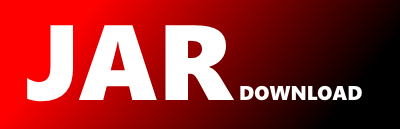
org.tensorflow.framework.FunctionDefOrBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/framework/function.proto
package org.tensorflow.framework;
public interface FunctionDefOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.FunctionDef)
com.google.protobuf.MessageOrBuilder {
/**
*
* The definition of the function's name, arguments, return values,
* attrs etc.
*
*
* .tensorflow.OpDef signature = 1;
*/
boolean hasSignature();
/**
*
* The definition of the function's name, arguments, return values,
* attrs etc.
*
*
* .tensorflow.OpDef signature = 1;
*/
org.tensorflow.framework.OpDef getSignature();
/**
*
* The definition of the function's name, arguments, return values,
* attrs etc.
*
*
* .tensorflow.OpDef signature = 1;
*/
org.tensorflow.framework.OpDefOrBuilder getSignatureOrBuilder();
/**
*
* Attributes specific to this function definition.
*
*
* map<string, .tensorflow.AttrValue> attr = 5;
*/
int getAttrCount();
/**
*
* Attributes specific to this function definition.
*
*
* map<string, .tensorflow.AttrValue> attr = 5;
*/
boolean containsAttr(
java.lang.String key);
/**
* Use {@link #getAttrMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getAttr();
/**
*
* Attributes specific to this function definition.
*
*
* map<string, .tensorflow.AttrValue> attr = 5;
*/
java.util.Map
getAttrMap();
/**
*
* Attributes specific to this function definition.
*
*
* map<string, .tensorflow.AttrValue> attr = 5;
*/
org.tensorflow.framework.AttrValue getAttrOrDefault(
java.lang.String key,
org.tensorflow.framework.AttrValue defaultValue);
/**
*
* Attributes specific to this function definition.
*
*
* map<string, .tensorflow.AttrValue> attr = 5;
*/
org.tensorflow.framework.AttrValue getAttrOrThrow(
java.lang.String key);
/**
* map<uint32, .tensorflow.FunctionDef.ArgAttrs> arg_attr = 7;
*/
int getArgAttrCount();
/**
* map<uint32, .tensorflow.FunctionDef.ArgAttrs> arg_attr = 7;
*/
boolean containsArgAttr(
int key);
/**
* Use {@link #getArgAttrMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getArgAttr();
/**
* map<uint32, .tensorflow.FunctionDef.ArgAttrs> arg_attr = 7;
*/
java.util.Map
getArgAttrMap();
/**
* map<uint32, .tensorflow.FunctionDef.ArgAttrs> arg_attr = 7;
*/
org.tensorflow.framework.FunctionDef.ArgAttrs getArgAttrOrDefault(
int key,
org.tensorflow.framework.FunctionDef.ArgAttrs defaultValue);
/**
* map<uint32, .tensorflow.FunctionDef.ArgAttrs> arg_attr = 7;
*/
org.tensorflow.framework.FunctionDef.ArgAttrs getArgAttrOrThrow(
int key);
/**
*
* By convention, "op" in node_def is resolved by consulting with a
* user-defined library first. If not resolved, "func" is assumed to
* be a builtin op.
*
*
* repeated .tensorflow.NodeDef node_def = 3;
*/
java.util.List
getNodeDefList();
/**
*
* By convention, "op" in node_def is resolved by consulting with a
* user-defined library first. If not resolved, "func" is assumed to
* be a builtin op.
*
*
* repeated .tensorflow.NodeDef node_def = 3;
*/
org.tensorflow.framework.NodeDef getNodeDef(int index);
/**
*
* By convention, "op" in node_def is resolved by consulting with a
* user-defined library first. If not resolved, "func" is assumed to
* be a builtin op.
*
*
* repeated .tensorflow.NodeDef node_def = 3;
*/
int getNodeDefCount();
/**
*
* By convention, "op" in node_def is resolved by consulting with a
* user-defined library first. If not resolved, "func" is assumed to
* be a builtin op.
*
*
* repeated .tensorflow.NodeDef node_def = 3;
*/
java.util.List extends org.tensorflow.framework.NodeDefOrBuilder>
getNodeDefOrBuilderList();
/**
*
* By convention, "op" in node_def is resolved by consulting with a
* user-defined library first. If not resolved, "func" is assumed to
* be a builtin op.
*
*
* repeated .tensorflow.NodeDef node_def = 3;
*/
org.tensorflow.framework.NodeDefOrBuilder getNodeDefOrBuilder(
int index);
/**
*
* A mapping from the output arg names from `signature` to the
* outputs from `node_def` that should be returned by the function.
*
*
* map<string, string> ret = 4;
*/
int getRetCount();
/**
*
* A mapping from the output arg names from `signature` to the
* outputs from `node_def` that should be returned by the function.
*
*
* map<string, string> ret = 4;
*/
boolean containsRet(
java.lang.String key);
/**
* Use {@link #getRetMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getRet();
/**
*
* A mapping from the output arg names from `signature` to the
* outputs from `node_def` that should be returned by the function.
*
*
* map<string, string> ret = 4;
*/
java.util.Map
getRetMap();
/**
*
* A mapping from the output arg names from `signature` to the
* outputs from `node_def` that should be returned by the function.
*
*
* map<string, string> ret = 4;
*/
java.lang.String getRetOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* A mapping from the output arg names from `signature` to the
* outputs from `node_def` that should be returned by the function.
*
*
* map<string, string> ret = 4;
*/
java.lang.String getRetOrThrow(
java.lang.String key);
/**
*
* A mapping from control output names from `signature` to node names in
* `node_def` which should be control outputs of this function.
*
*
* map<string, string> control_ret = 6;
*/
int getControlRetCount();
/**
*
* A mapping from control output names from `signature` to node names in
* `node_def` which should be control outputs of this function.
*
*
* map<string, string> control_ret = 6;
*/
boolean containsControlRet(
java.lang.String key);
/**
* Use {@link #getControlRetMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getControlRet();
/**
*
* A mapping from control output names from `signature` to node names in
* `node_def` which should be control outputs of this function.
*
*
* map<string, string> control_ret = 6;
*/
java.util.Map
getControlRetMap();
/**
*
* A mapping from control output names from `signature` to node names in
* `node_def` which should be control outputs of this function.
*
*
* map<string, string> control_ret = 6;
*/
java.lang.String getControlRetOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* A mapping from control output names from `signature` to node names in
* `node_def` which should be control outputs of this function.
*
*
* map<string, string> control_ret = 6;
*/
java.lang.String getControlRetOrThrow(
java.lang.String key);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy