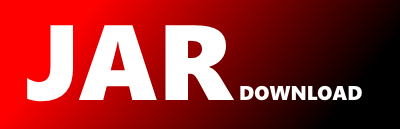
org.tensorflow.framework.GraphDebugInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/graph_debug_info.proto
package org.tensorflow.framework;
/**
* Protobuf type {@code tensorflow.GraphDebugInfo}
*/
public final class GraphDebugInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.GraphDebugInfo)
GraphDebugInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use GraphDebugInfo.newBuilder() to construct.
private GraphDebugInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private GraphDebugInfo() {
files_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private GraphDebugInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
files_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
files_.add(s);
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
traces_ = com.google.protobuf.MapField.newMapField(
TracesDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
traces__ = input.readMessage(
TracesDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
traces_.getMutableMap().put(
traces__.getKey(), traces__.getValue());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
files_ = files_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetTraces();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphDebugInfo.class, org.tensorflow.framework.GraphDebugInfo.Builder.class);
}
public interface FileLineColOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.GraphDebugInfo.FileLineCol)
com.google.protobuf.MessageOrBuilder {
/**
*
* File name index, which can be used to retrieve the file name string from
* `files`. The value should be between 0 and (len(files)-1)
*
*
* int32 file_index = 1;
*/
int getFileIndex();
/**
*
* Line number in the file.
*
*
* int32 line = 2;
*/
int getLine();
/**
*
* Col number in the file line.
*
*
* int32 col = 3;
*/
int getCol();
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
java.lang.String getFunc();
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
com.google.protobuf.ByteString
getFuncBytes();
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
java.lang.String getCode();
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
com.google.protobuf.ByteString
getCodeBytes();
}
/**
*
* This represents a file/line location in the source code.
*
*
* Protobuf type {@code tensorflow.GraphDebugInfo.FileLineCol}
*/
public static final class FileLineCol extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.GraphDebugInfo.FileLineCol)
FileLineColOrBuilder {
private static final long serialVersionUID = 0L;
// Use FileLineCol.newBuilder() to construct.
private FileLineCol(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FileLineCol() {
fileIndex_ = 0;
line_ = 0;
col_ = 0;
func_ = "";
code_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FileLineCol(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
fileIndex_ = input.readInt32();
break;
}
case 16: {
line_ = input.readInt32();
break;
}
case 24: {
col_ = input.readInt32();
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
func_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
code_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_FileLineCol_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_FileLineCol_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphDebugInfo.FileLineCol.class, org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder.class);
}
public static final int FILE_INDEX_FIELD_NUMBER = 1;
private int fileIndex_;
/**
*
* File name index, which can be used to retrieve the file name string from
* `files`. The value should be between 0 and (len(files)-1)
*
*
* int32 file_index = 1;
*/
public int getFileIndex() {
return fileIndex_;
}
public static final int LINE_FIELD_NUMBER = 2;
private int line_;
/**
*
* Line number in the file.
*
*
* int32 line = 2;
*/
public int getLine() {
return line_;
}
public static final int COL_FIELD_NUMBER = 3;
private int col_;
/**
*
* Col number in the file line.
*
*
* int32 col = 3;
*/
public int getCol() {
return col_;
}
public static final int FUNC_FIELD_NUMBER = 4;
private volatile java.lang.Object func_;
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
public java.lang.String getFunc() {
java.lang.Object ref = func_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
func_ = s;
return s;
}
}
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
public com.google.protobuf.ByteString
getFuncBytes() {
java.lang.Object ref = func_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
func_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CODE_FIELD_NUMBER = 5;
private volatile java.lang.Object code_;
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
public java.lang.String getCode() {
java.lang.Object ref = code_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
code_ = s;
return s;
}
}
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
public com.google.protobuf.ByteString
getCodeBytes() {
java.lang.Object ref = code_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
code_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (fileIndex_ != 0) {
output.writeInt32(1, fileIndex_);
}
if (line_ != 0) {
output.writeInt32(2, line_);
}
if (col_ != 0) {
output.writeInt32(3, col_);
}
if (!getFuncBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, func_);
}
if (!getCodeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, code_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (fileIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, fileIndex_);
}
if (line_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(2, line_);
}
if (col_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, col_);
}
if (!getFuncBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, func_);
}
if (!getCodeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, code_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.GraphDebugInfo.FileLineCol)) {
return super.equals(obj);
}
org.tensorflow.framework.GraphDebugInfo.FileLineCol other = (org.tensorflow.framework.GraphDebugInfo.FileLineCol) obj;
boolean result = true;
result = result && (getFileIndex()
== other.getFileIndex());
result = result && (getLine()
== other.getLine());
result = result && (getCol()
== other.getCol());
result = result && getFunc()
.equals(other.getFunc());
result = result && getCode()
.equals(other.getCode());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FILE_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getFileIndex();
hash = (37 * hash) + LINE_FIELD_NUMBER;
hash = (53 * hash) + getLine();
hash = (37 * hash) + COL_FIELD_NUMBER;
hash = (53 * hash) + getCol();
hash = (37 * hash) + FUNC_FIELD_NUMBER;
hash = (53 * hash) + getFunc().hashCode();
hash = (37 * hash) + CODE_FIELD_NUMBER;
hash = (53 * hash) + getCode().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.GraphDebugInfo.FileLineCol prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This represents a file/line location in the source code.
*
*
* Protobuf type {@code tensorflow.GraphDebugInfo.FileLineCol}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.GraphDebugInfo.FileLineCol)
org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_FileLineCol_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_FileLineCol_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphDebugInfo.FileLineCol.class, org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder.class);
}
// Construct using org.tensorflow.framework.GraphDebugInfo.FileLineCol.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
fileIndex_ = 0;
line_ = 0;
col_ = 0;
func_ = "";
code_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_FileLineCol_descriptor;
}
public org.tensorflow.framework.GraphDebugInfo.FileLineCol getDefaultInstanceForType() {
return org.tensorflow.framework.GraphDebugInfo.FileLineCol.getDefaultInstance();
}
public org.tensorflow.framework.GraphDebugInfo.FileLineCol build() {
org.tensorflow.framework.GraphDebugInfo.FileLineCol result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.GraphDebugInfo.FileLineCol buildPartial() {
org.tensorflow.framework.GraphDebugInfo.FileLineCol result = new org.tensorflow.framework.GraphDebugInfo.FileLineCol(this);
result.fileIndex_ = fileIndex_;
result.line_ = line_;
result.col_ = col_;
result.func_ = func_;
result.code_ = code_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.GraphDebugInfo.FileLineCol) {
return mergeFrom((org.tensorflow.framework.GraphDebugInfo.FileLineCol)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.GraphDebugInfo.FileLineCol other) {
if (other == org.tensorflow.framework.GraphDebugInfo.FileLineCol.getDefaultInstance()) return this;
if (other.getFileIndex() != 0) {
setFileIndex(other.getFileIndex());
}
if (other.getLine() != 0) {
setLine(other.getLine());
}
if (other.getCol() != 0) {
setCol(other.getCol());
}
if (!other.getFunc().isEmpty()) {
func_ = other.func_;
onChanged();
}
if (!other.getCode().isEmpty()) {
code_ = other.code_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.GraphDebugInfo.FileLineCol parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.GraphDebugInfo.FileLineCol) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int fileIndex_ ;
/**
*
* File name index, which can be used to retrieve the file name string from
* `files`. The value should be between 0 and (len(files)-1)
*
*
* int32 file_index = 1;
*/
public int getFileIndex() {
return fileIndex_;
}
/**
*
* File name index, which can be used to retrieve the file name string from
* `files`. The value should be between 0 and (len(files)-1)
*
*
* int32 file_index = 1;
*/
public Builder setFileIndex(int value) {
fileIndex_ = value;
onChanged();
return this;
}
/**
*
* File name index, which can be used to retrieve the file name string from
* `files`. The value should be between 0 and (len(files)-1)
*
*
* int32 file_index = 1;
*/
public Builder clearFileIndex() {
fileIndex_ = 0;
onChanged();
return this;
}
private int line_ ;
/**
*
* Line number in the file.
*
*
* int32 line = 2;
*/
public int getLine() {
return line_;
}
/**
*
* Line number in the file.
*
*
* int32 line = 2;
*/
public Builder setLine(int value) {
line_ = value;
onChanged();
return this;
}
/**
*
* Line number in the file.
*
*
* int32 line = 2;
*/
public Builder clearLine() {
line_ = 0;
onChanged();
return this;
}
private int col_ ;
/**
*
* Col number in the file line.
*
*
* int32 col = 3;
*/
public int getCol() {
return col_;
}
/**
*
* Col number in the file line.
*
*
* int32 col = 3;
*/
public Builder setCol(int value) {
col_ = value;
onChanged();
return this;
}
/**
*
* Col number in the file line.
*
*
* int32 col = 3;
*/
public Builder clearCol() {
col_ = 0;
onChanged();
return this;
}
private java.lang.Object func_ = "";
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
public java.lang.String getFunc() {
java.lang.Object ref = func_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
func_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
public com.google.protobuf.ByteString
getFuncBytes() {
java.lang.Object ref = func_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
func_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
public Builder setFunc(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
func_ = value;
onChanged();
return this;
}
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
public Builder clearFunc() {
func_ = getDefaultInstance().getFunc();
onChanged();
return this;
}
/**
*
* Name of function contains the file line.
*
*
* string func = 4;
*/
public Builder setFuncBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
func_ = value;
onChanged();
return this;
}
private java.lang.Object code_ = "";
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
public java.lang.String getCode() {
java.lang.Object ref = code_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
code_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
public com.google.protobuf.ByteString
getCodeBytes() {
java.lang.Object ref = code_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
code_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
public Builder setCode(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
code_ = value;
onChanged();
return this;
}
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
public Builder clearCode() {
code_ = getDefaultInstance().getCode();
onChanged();
return this;
}
/**
*
* Source code contained in this file line.
*
*
* string code = 5;
*/
public Builder setCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
code_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.GraphDebugInfo.FileLineCol)
}
// @@protoc_insertion_point(class_scope:tensorflow.GraphDebugInfo.FileLineCol)
private static final org.tensorflow.framework.GraphDebugInfo.FileLineCol DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.GraphDebugInfo.FileLineCol();
}
public static org.tensorflow.framework.GraphDebugInfo.FileLineCol getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FileLineCol parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FileLineCol(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.GraphDebugInfo.FileLineCol getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface StackTraceOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.GraphDebugInfo.StackTrace)
com.google.protobuf.MessageOrBuilder {
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
java.util.List
getFileLineColsList();
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
org.tensorflow.framework.GraphDebugInfo.FileLineCol getFileLineCols(int index);
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
int getFileLineColsCount();
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
java.util.List extends org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder>
getFileLineColsOrBuilderList();
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder getFileLineColsOrBuilder(
int index);
}
/**
*
* This represents a stack trace which is a ordered list of `FileLineCol`.
*
*
* Protobuf type {@code tensorflow.GraphDebugInfo.StackTrace}
*/
public static final class StackTrace extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.GraphDebugInfo.StackTrace)
StackTraceOrBuilder {
private static final long serialVersionUID = 0L;
// Use StackTrace.newBuilder() to construct.
private StackTrace(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private StackTrace() {
fileLineCols_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private StackTrace(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
fileLineCols_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
fileLineCols_.add(
input.readMessage(org.tensorflow.framework.GraphDebugInfo.FileLineCol.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
fileLineCols_ = java.util.Collections.unmodifiableList(fileLineCols_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_StackTrace_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_StackTrace_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphDebugInfo.StackTrace.class, org.tensorflow.framework.GraphDebugInfo.StackTrace.Builder.class);
}
public static final int FILE_LINE_COLS_FIELD_NUMBER = 1;
private java.util.List fileLineCols_;
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public java.util.List getFileLineColsList() {
return fileLineCols_;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public java.util.List extends org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder>
getFileLineColsOrBuilderList() {
return fileLineCols_;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public int getFileLineColsCount() {
return fileLineCols_.size();
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public org.tensorflow.framework.GraphDebugInfo.FileLineCol getFileLineCols(int index) {
return fileLineCols_.get(index);
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder getFileLineColsOrBuilder(
int index) {
return fileLineCols_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < fileLineCols_.size(); i++) {
output.writeMessage(1, fileLineCols_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < fileLineCols_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, fileLineCols_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.GraphDebugInfo.StackTrace)) {
return super.equals(obj);
}
org.tensorflow.framework.GraphDebugInfo.StackTrace other = (org.tensorflow.framework.GraphDebugInfo.StackTrace) obj;
boolean result = true;
result = result && getFileLineColsList()
.equals(other.getFileLineColsList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFileLineColsCount() > 0) {
hash = (37 * hash) + FILE_LINE_COLS_FIELD_NUMBER;
hash = (53 * hash) + getFileLineColsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.GraphDebugInfo.StackTrace prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* This represents a stack trace which is a ordered list of `FileLineCol`.
*
*
* Protobuf type {@code tensorflow.GraphDebugInfo.StackTrace}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.GraphDebugInfo.StackTrace)
org.tensorflow.framework.GraphDebugInfo.StackTraceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_StackTrace_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_StackTrace_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphDebugInfo.StackTrace.class, org.tensorflow.framework.GraphDebugInfo.StackTrace.Builder.class);
}
// Construct using org.tensorflow.framework.GraphDebugInfo.StackTrace.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getFileLineColsFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (fileLineColsBuilder_ == null) {
fileLineCols_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
fileLineColsBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_StackTrace_descriptor;
}
public org.tensorflow.framework.GraphDebugInfo.StackTrace getDefaultInstanceForType() {
return org.tensorflow.framework.GraphDebugInfo.StackTrace.getDefaultInstance();
}
public org.tensorflow.framework.GraphDebugInfo.StackTrace build() {
org.tensorflow.framework.GraphDebugInfo.StackTrace result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.GraphDebugInfo.StackTrace buildPartial() {
org.tensorflow.framework.GraphDebugInfo.StackTrace result = new org.tensorflow.framework.GraphDebugInfo.StackTrace(this);
int from_bitField0_ = bitField0_;
if (fileLineColsBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
fileLineCols_ = java.util.Collections.unmodifiableList(fileLineCols_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.fileLineCols_ = fileLineCols_;
} else {
result.fileLineCols_ = fileLineColsBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.GraphDebugInfo.StackTrace) {
return mergeFrom((org.tensorflow.framework.GraphDebugInfo.StackTrace)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.GraphDebugInfo.StackTrace other) {
if (other == org.tensorflow.framework.GraphDebugInfo.StackTrace.getDefaultInstance()) return this;
if (fileLineColsBuilder_ == null) {
if (!other.fileLineCols_.isEmpty()) {
if (fileLineCols_.isEmpty()) {
fileLineCols_ = other.fileLineCols_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFileLineColsIsMutable();
fileLineCols_.addAll(other.fileLineCols_);
}
onChanged();
}
} else {
if (!other.fileLineCols_.isEmpty()) {
if (fileLineColsBuilder_.isEmpty()) {
fileLineColsBuilder_.dispose();
fileLineColsBuilder_ = null;
fileLineCols_ = other.fileLineCols_;
bitField0_ = (bitField0_ & ~0x00000001);
fileLineColsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getFileLineColsFieldBuilder() : null;
} else {
fileLineColsBuilder_.addAllMessages(other.fileLineCols_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.GraphDebugInfo.StackTrace parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.GraphDebugInfo.StackTrace) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List fileLineCols_ =
java.util.Collections.emptyList();
private void ensureFileLineColsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
fileLineCols_ = new java.util.ArrayList(fileLineCols_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphDebugInfo.FileLineCol, org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder, org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder> fileLineColsBuilder_;
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public java.util.List getFileLineColsList() {
if (fileLineColsBuilder_ == null) {
return java.util.Collections.unmodifiableList(fileLineCols_);
} else {
return fileLineColsBuilder_.getMessageList();
}
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public int getFileLineColsCount() {
if (fileLineColsBuilder_ == null) {
return fileLineCols_.size();
} else {
return fileLineColsBuilder_.getCount();
}
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public org.tensorflow.framework.GraphDebugInfo.FileLineCol getFileLineCols(int index) {
if (fileLineColsBuilder_ == null) {
return fileLineCols_.get(index);
} else {
return fileLineColsBuilder_.getMessage(index);
}
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder setFileLineCols(
int index, org.tensorflow.framework.GraphDebugInfo.FileLineCol value) {
if (fileLineColsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFileLineColsIsMutable();
fileLineCols_.set(index, value);
onChanged();
} else {
fileLineColsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder setFileLineCols(
int index, org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder builderForValue) {
if (fileLineColsBuilder_ == null) {
ensureFileLineColsIsMutable();
fileLineCols_.set(index, builderForValue.build());
onChanged();
} else {
fileLineColsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder addFileLineCols(org.tensorflow.framework.GraphDebugInfo.FileLineCol value) {
if (fileLineColsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFileLineColsIsMutable();
fileLineCols_.add(value);
onChanged();
} else {
fileLineColsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder addFileLineCols(
int index, org.tensorflow.framework.GraphDebugInfo.FileLineCol value) {
if (fileLineColsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureFileLineColsIsMutable();
fileLineCols_.add(index, value);
onChanged();
} else {
fileLineColsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder addFileLineCols(
org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder builderForValue) {
if (fileLineColsBuilder_ == null) {
ensureFileLineColsIsMutable();
fileLineCols_.add(builderForValue.build());
onChanged();
} else {
fileLineColsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder addFileLineCols(
int index, org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder builderForValue) {
if (fileLineColsBuilder_ == null) {
ensureFileLineColsIsMutable();
fileLineCols_.add(index, builderForValue.build());
onChanged();
} else {
fileLineColsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder addAllFileLineCols(
java.lang.Iterable extends org.tensorflow.framework.GraphDebugInfo.FileLineCol> values) {
if (fileLineColsBuilder_ == null) {
ensureFileLineColsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, fileLineCols_);
onChanged();
} else {
fileLineColsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder clearFileLineCols() {
if (fileLineColsBuilder_ == null) {
fileLineCols_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
fileLineColsBuilder_.clear();
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public Builder removeFileLineCols(int index) {
if (fileLineColsBuilder_ == null) {
ensureFileLineColsIsMutable();
fileLineCols_.remove(index);
onChanged();
} else {
fileLineColsBuilder_.remove(index);
}
return this;
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder getFileLineColsBuilder(
int index) {
return getFileLineColsFieldBuilder().getBuilder(index);
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder getFileLineColsOrBuilder(
int index) {
if (fileLineColsBuilder_ == null) {
return fileLineCols_.get(index); } else {
return fileLineColsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public java.util.List extends org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder>
getFileLineColsOrBuilderList() {
if (fileLineColsBuilder_ != null) {
return fileLineColsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(fileLineCols_);
}
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder addFileLineColsBuilder() {
return getFileLineColsFieldBuilder().addBuilder(
org.tensorflow.framework.GraphDebugInfo.FileLineCol.getDefaultInstance());
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder addFileLineColsBuilder(
int index) {
return getFileLineColsFieldBuilder().addBuilder(
index, org.tensorflow.framework.GraphDebugInfo.FileLineCol.getDefaultInstance());
}
/**
*
* Each line in the stack trace.
*
*
* repeated .tensorflow.GraphDebugInfo.FileLineCol file_line_cols = 1;
*/
public java.util.List
getFileLineColsBuilderList() {
return getFileLineColsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphDebugInfo.FileLineCol, org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder, org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder>
getFileLineColsFieldBuilder() {
if (fileLineColsBuilder_ == null) {
fileLineColsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.GraphDebugInfo.FileLineCol, org.tensorflow.framework.GraphDebugInfo.FileLineCol.Builder, org.tensorflow.framework.GraphDebugInfo.FileLineColOrBuilder>(
fileLineCols_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
fileLineCols_ = null;
}
return fileLineColsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.GraphDebugInfo.StackTrace)
}
// @@protoc_insertion_point(class_scope:tensorflow.GraphDebugInfo.StackTrace)
private static final org.tensorflow.framework.GraphDebugInfo.StackTrace DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.GraphDebugInfo.StackTrace();
}
public static org.tensorflow.framework.GraphDebugInfo.StackTrace getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public StackTrace parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new StackTrace(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.GraphDebugInfo.StackTrace getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int FILES_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList files_;
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public com.google.protobuf.ProtocolStringList
getFilesList() {
return files_;
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public int getFilesCount() {
return files_.size();
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public java.lang.String getFiles(int index) {
return files_.get(index);
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public com.google.protobuf.ByteString
getFilesBytes(int index) {
return files_.getByteString(index);
}
public static final int TRACES_FIELD_NUMBER = 2;
private static final class TracesDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, org.tensorflow.framework.GraphDebugInfo.StackTrace> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_TracesEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
org.tensorflow.framework.GraphDebugInfo.StackTrace.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, org.tensorflow.framework.GraphDebugInfo.StackTrace> traces_;
private com.google.protobuf.MapField
internalGetTraces() {
if (traces_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TracesDefaultEntryHolder.defaultEntry);
}
return traces_;
}
public int getTracesCount() {
return internalGetTraces().getMap().size();
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public boolean containsTraces(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetTraces().getMap().containsKey(key);
}
/**
* Use {@link #getTracesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getTraces() {
return getTracesMap();
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public java.util.Map getTracesMap() {
return internalGetTraces().getMap();
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public org.tensorflow.framework.GraphDebugInfo.StackTrace getTracesOrDefault(
java.lang.String key,
org.tensorflow.framework.GraphDebugInfo.StackTrace defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetTraces().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public org.tensorflow.framework.GraphDebugInfo.StackTrace getTracesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetTraces().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < files_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, files_.getRaw(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetTraces(),
TracesDefaultEntryHolder.defaultEntry,
2);
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < files_.size(); i++) {
dataSize += computeStringSizeNoTag(files_.getRaw(i));
}
size += dataSize;
size += 1 * getFilesList().size();
}
for (java.util.Map.Entry entry
: internalGetTraces().getMap().entrySet()) {
com.google.protobuf.MapEntry
traces__ = TracesDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, traces__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.GraphDebugInfo)) {
return super.equals(obj);
}
org.tensorflow.framework.GraphDebugInfo other = (org.tensorflow.framework.GraphDebugInfo) obj;
boolean result = true;
result = result && getFilesList()
.equals(other.getFilesList());
result = result && internalGetTraces().equals(
other.internalGetTraces());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getFilesCount() > 0) {
hash = (37 * hash) + FILES_FIELD_NUMBER;
hash = (53 * hash) + getFilesList().hashCode();
}
if (!internalGetTraces().getMap().isEmpty()) {
hash = (37 * hash) + TRACES_FIELD_NUMBER;
hash = (53 * hash) + internalGetTraces().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.GraphDebugInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.GraphDebugInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.GraphDebugInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.GraphDebugInfo)
org.tensorflow.framework.GraphDebugInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetTraces();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableTraces();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.GraphDebugInfo.class, org.tensorflow.framework.GraphDebugInfo.Builder.class);
}
// Construct using org.tensorflow.framework.GraphDebugInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
files_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
internalGetMutableTraces().clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.GraphDebugInfoProtos.internal_static_tensorflow_GraphDebugInfo_descriptor;
}
public org.tensorflow.framework.GraphDebugInfo getDefaultInstanceForType() {
return org.tensorflow.framework.GraphDebugInfo.getDefaultInstance();
}
public org.tensorflow.framework.GraphDebugInfo build() {
org.tensorflow.framework.GraphDebugInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.GraphDebugInfo buildPartial() {
org.tensorflow.framework.GraphDebugInfo result = new org.tensorflow.framework.GraphDebugInfo(this);
int from_bitField0_ = bitField0_;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
files_ = files_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.files_ = files_;
result.traces_ = internalGetTraces();
result.traces_.makeImmutable();
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.GraphDebugInfo) {
return mergeFrom((org.tensorflow.framework.GraphDebugInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.GraphDebugInfo other) {
if (other == org.tensorflow.framework.GraphDebugInfo.getDefaultInstance()) return this;
if (!other.files_.isEmpty()) {
if (files_.isEmpty()) {
files_ = other.files_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureFilesIsMutable();
files_.addAll(other.files_);
}
onChanged();
}
internalGetMutableTraces().mergeFrom(
other.internalGetTraces());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.GraphDebugInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.GraphDebugInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList files_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureFilesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
files_ = new com.google.protobuf.LazyStringArrayList(files_);
bitField0_ |= 0x00000001;
}
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public com.google.protobuf.ProtocolStringList
getFilesList() {
return files_.getUnmodifiableView();
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public int getFilesCount() {
return files_.size();
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public java.lang.String getFiles(int index) {
return files_.get(index);
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public com.google.protobuf.ByteString
getFilesBytes(int index) {
return files_.getByteString(index);
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public Builder setFiles(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.set(index, value);
onChanged();
return this;
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public Builder addFiles(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureFilesIsMutable();
files_.add(value);
onChanged();
return this;
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public Builder addAllFiles(
java.lang.Iterable values) {
ensureFilesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, files_);
onChanged();
return this;
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public Builder clearFiles() {
files_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* This stores all the source code file names and can be indexed by the
* `file_index`.
*
*
* repeated string files = 1;
*/
public Builder addFilesBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureFilesIsMutable();
files_.add(value);
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, org.tensorflow.framework.GraphDebugInfo.StackTrace> traces_;
private com.google.protobuf.MapField
internalGetTraces() {
if (traces_ == null) {
return com.google.protobuf.MapField.emptyMapField(
TracesDefaultEntryHolder.defaultEntry);
}
return traces_;
}
private com.google.protobuf.MapField
internalGetMutableTraces() {
onChanged();;
if (traces_ == null) {
traces_ = com.google.protobuf.MapField.newMapField(
TracesDefaultEntryHolder.defaultEntry);
}
if (!traces_.isMutable()) {
traces_ = traces_.copy();
}
return traces_;
}
public int getTracesCount() {
return internalGetTraces().getMap().size();
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public boolean containsTraces(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetTraces().getMap().containsKey(key);
}
/**
* Use {@link #getTracesMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getTraces() {
return getTracesMap();
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public java.util.Map getTracesMap() {
return internalGetTraces().getMap();
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public org.tensorflow.framework.GraphDebugInfo.StackTrace getTracesOrDefault(
java.lang.String key,
org.tensorflow.framework.GraphDebugInfo.StackTrace defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetTraces().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public org.tensorflow.framework.GraphDebugInfo.StackTrace getTracesOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetTraces().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearTraces() {
internalGetMutableTraces().getMutableMap()
.clear();
return this;
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public Builder removeTraces(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableTraces().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableTraces() {
return internalGetMutableTraces().getMutableMap();
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public Builder putTraces(
java.lang.String key,
org.tensorflow.framework.GraphDebugInfo.StackTrace value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableTraces().getMutableMap()
.put(key, value);
return this;
}
/**
*
* This maps a node name to a stack trace in the source code.
*
*
* map<string, .tensorflow.GraphDebugInfo.StackTrace> traces = 2;
*/
public Builder putAllTraces(
java.util.Map values) {
internalGetMutableTraces().getMutableMap()
.putAll(values);
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.GraphDebugInfo)
}
// @@protoc_insertion_point(class_scope:tensorflow.GraphDebugInfo)
private static final org.tensorflow.framework.GraphDebugInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.GraphDebugInfo();
}
public static org.tensorflow.framework.GraphDebugInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public GraphDebugInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new GraphDebugInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.GraphDebugInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy