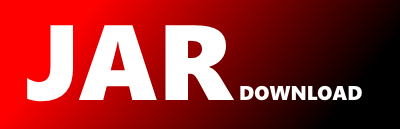
org.tensorflow.framework.RemoteFusedGraphExecuteInfo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/framework/remote_fused_graph_execute_info.proto
package org.tensorflow.framework;
/**
*
* Protocol buffer representing a handle to a tensorflow resource. Handles are
* not valid across executions, but can be serialized back and forth from within
* a single run.
*
*
* Protobuf type {@code tensorflow.RemoteFusedGraphExecuteInfo}
*/
public final class RemoteFusedGraphExecuteInfo extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.RemoteFusedGraphExecuteInfo)
RemoteFusedGraphExecuteInfoOrBuilder {
private static final long serialVersionUID = 0L;
// Use RemoteFusedGraphExecuteInfo.newBuilder() to construct.
private RemoteFusedGraphExecuteInfo(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RemoteFusedGraphExecuteInfo() {
graphInputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
graphOutputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
executorName_ = "";
serializedExecutorParameters_ = com.google.protobuf.ByteString.EMPTY;
defaultGraphInputTensorShape_ = java.util.Collections.emptyList();
defaultGraphOutputTensorShape_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RemoteFusedGraphExecuteInfo(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
org.tensorflow.framework.GraphDef.Builder subBuilder = null;
if (remoteGraph_ != null) {
subBuilder = remoteGraph_.toBuilder();
}
remoteGraph_ = input.readMessage(org.tensorflow.framework.GraphDef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(remoteGraph_);
remoteGraph_ = subBuilder.buildPartial();
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
graphInputNodeName_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
graphInputNodeName_.add(s);
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
graphOutputNodeName_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000004;
}
graphOutputNodeName_.add(s);
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
executorName_ = s;
break;
}
case 42: {
serializedExecutorParameters_ = input.readBytes();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
defaultGraphInputTensorShape_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000020;
}
defaultGraphInputTensorShape_.add(
input.readMessage(org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.parser(), extensionRegistry));
break;
}
case 58: {
if (!((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
defaultGraphOutputTensorShape_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000040;
}
defaultGraphOutputTensorShape_.add(
input.readMessage(org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
graphInputNodeName_ = graphInputNodeName_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000004) == 0x00000004)) {
graphOutputNodeName_ = graphOutputNodeName_.getUnmodifiableView();
}
if (((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
defaultGraphInputTensorShape_ = java.util.Collections.unmodifiableList(defaultGraphInputTensorShape_);
}
if (((mutable_bitField0_ & 0x00000040) == 0x00000040)) {
defaultGraphOutputTensorShape_ = java.util.Collections.unmodifiableList(defaultGraphOutputTensorShape_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.class, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.Builder.class);
}
public interface TensorShapeTypeProtoOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto)
com.google.protobuf.MessageOrBuilder {
/**
* .tensorflow.DataType dtype = 1;
*/
int getDtypeValue();
/**
* .tensorflow.DataType dtype = 1;
*/
org.tensorflow.framework.DataType getDtype();
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
boolean hasShape();
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
org.tensorflow.framework.TensorShapeProto getShape();
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
org.tensorflow.framework.TensorShapeProtoOrBuilder getShapeOrBuilder();
}
/**
* Protobuf type {@code tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto}
*/
public static final class TensorShapeTypeProto extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto)
TensorShapeTypeProtoOrBuilder {
private static final long serialVersionUID = 0L;
// Use TensorShapeTypeProto.newBuilder() to construct.
private TensorShapeTypeProto(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private TensorShapeTypeProto() {
dtype_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private TensorShapeTypeProto(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
dtype_ = rawValue;
break;
}
case 18: {
org.tensorflow.framework.TensorShapeProto.Builder subBuilder = null;
if (shape_ != null) {
subBuilder = shape_.toBuilder();
}
shape_ = input.readMessage(org.tensorflow.framework.TensorShapeProto.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(shape_);
shape_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_TensorShapeTypeProto_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_TensorShapeTypeProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.class, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder.class);
}
public static final int DTYPE_FIELD_NUMBER = 1;
private int dtype_;
/**
* .tensorflow.DataType dtype = 1;
*/
public int getDtypeValue() {
return dtype_;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public org.tensorflow.framework.DataType getDtype() {
org.tensorflow.framework.DataType result = org.tensorflow.framework.DataType.valueOf(dtype_);
return result == null ? org.tensorflow.framework.DataType.UNRECOGNIZED : result;
}
public static final int SHAPE_FIELD_NUMBER = 2;
private org.tensorflow.framework.TensorShapeProto shape_;
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public boolean hasShape() {
return shape_ != null;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProto getShape() {
return shape_ == null ? org.tensorflow.framework.TensorShapeProto.getDefaultInstance() : shape_;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProtoOrBuilder getShapeOrBuilder() {
return getShape();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (dtype_ != org.tensorflow.framework.DataType.DT_INVALID.getNumber()) {
output.writeEnum(1, dtype_);
}
if (shape_ != null) {
output.writeMessage(2, getShape());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (dtype_ != org.tensorflow.framework.DataType.DT_INVALID.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, dtype_);
}
if (shape_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getShape());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto)) {
return super.equals(obj);
}
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto other = (org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto) obj;
boolean result = true;
result = result && dtype_ == other.dtype_;
result = result && (hasShape() == other.hasShape());
if (hasShape()) {
result = result && getShape()
.equals(other.getShape());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DTYPE_FIELD_NUMBER;
hash = (53 * hash) + dtype_;
if (hasShape()) {
hash = (37 * hash) + SHAPE_FIELD_NUMBER;
hash = (53 * hash) + getShape().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto)
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_TensorShapeTypeProto_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_TensorShapeTypeProto_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.class, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder.class);
}
// Construct using org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
dtype_ = 0;
if (shapeBuilder_ == null) {
shape_ = null;
} else {
shape_ = null;
shapeBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_TensorShapeTypeProto_descriptor;
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto getDefaultInstanceForType() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.getDefaultInstance();
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto build() {
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto buildPartial() {
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto result = new org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto(this);
result.dtype_ = dtype_;
if (shapeBuilder_ == null) {
result.shape_ = shape_;
} else {
result.shape_ = shapeBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto) {
return mergeFrom((org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto other) {
if (other == org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.getDefaultInstance()) return this;
if (other.dtype_ != 0) {
setDtypeValue(other.getDtypeValue());
}
if (other.hasShape()) {
mergeShape(other.getShape());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int dtype_ = 0;
/**
* .tensorflow.DataType dtype = 1;
*/
public int getDtypeValue() {
return dtype_;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public Builder setDtypeValue(int value) {
dtype_ = value;
onChanged();
return this;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public org.tensorflow.framework.DataType getDtype() {
org.tensorflow.framework.DataType result = org.tensorflow.framework.DataType.valueOf(dtype_);
return result == null ? org.tensorflow.framework.DataType.UNRECOGNIZED : result;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public Builder setDtype(org.tensorflow.framework.DataType value) {
if (value == null) {
throw new NullPointerException();
}
dtype_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public Builder clearDtype() {
dtype_ = 0;
onChanged();
return this;
}
private org.tensorflow.framework.TensorShapeProto shape_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.TensorShapeProto, org.tensorflow.framework.TensorShapeProto.Builder, org.tensorflow.framework.TensorShapeProtoOrBuilder> shapeBuilder_;
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public boolean hasShape() {
return shapeBuilder_ != null || shape_ != null;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProto getShape() {
if (shapeBuilder_ == null) {
return shape_ == null ? org.tensorflow.framework.TensorShapeProto.getDefaultInstance() : shape_;
} else {
return shapeBuilder_.getMessage();
}
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder setShape(org.tensorflow.framework.TensorShapeProto value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shape_ = value;
onChanged();
} else {
shapeBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder setShape(
org.tensorflow.framework.TensorShapeProto.Builder builderForValue) {
if (shapeBuilder_ == null) {
shape_ = builderForValue.build();
onChanged();
} else {
shapeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder mergeShape(org.tensorflow.framework.TensorShapeProto value) {
if (shapeBuilder_ == null) {
if (shape_ != null) {
shape_ =
org.tensorflow.framework.TensorShapeProto.newBuilder(shape_).mergeFrom(value).buildPartial();
} else {
shape_ = value;
}
onChanged();
} else {
shapeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder clearShape() {
if (shapeBuilder_ == null) {
shape_ = null;
onChanged();
} else {
shape_ = null;
shapeBuilder_ = null;
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProto.Builder getShapeBuilder() {
onChanged();
return getShapeFieldBuilder().getBuilder();
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProtoOrBuilder getShapeOrBuilder() {
if (shapeBuilder_ != null) {
return shapeBuilder_.getMessageOrBuilder();
} else {
return shape_ == null ?
org.tensorflow.framework.TensorShapeProto.getDefaultInstance() : shape_;
}
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.TensorShapeProto, org.tensorflow.framework.TensorShapeProto.Builder, org.tensorflow.framework.TensorShapeProtoOrBuilder>
getShapeFieldBuilder() {
if (shapeBuilder_ == null) {
shapeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.TensorShapeProto, org.tensorflow.framework.TensorShapeProto.Builder, org.tensorflow.framework.TensorShapeProtoOrBuilder>(
getShape(),
getParentForChildren(),
isClean());
shape_ = null;
}
return shapeBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto)
}
// @@protoc_insertion_point(class_scope:tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto)
private static final org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto();
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public TensorShapeTypeProto parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new TensorShapeTypeProto(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int REMOTE_GRAPH_FIELD_NUMBER = 1;
private org.tensorflow.framework.GraphDef remoteGraph_;
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public boolean hasRemoteGraph() {
return remoteGraph_ != null;
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public org.tensorflow.framework.GraphDef getRemoteGraph() {
return remoteGraph_ == null ? org.tensorflow.framework.GraphDef.getDefaultInstance() : remoteGraph_;
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public org.tensorflow.framework.GraphDefOrBuilder getRemoteGraphOrBuilder() {
return getRemoteGraph();
}
public static final int GRAPH_INPUT_NODE_NAME_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList graphInputNodeName_;
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public com.google.protobuf.ProtocolStringList
getGraphInputNodeNameList() {
return graphInputNodeName_;
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public int getGraphInputNodeNameCount() {
return graphInputNodeName_.size();
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public java.lang.String getGraphInputNodeName(int index) {
return graphInputNodeName_.get(index);
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public com.google.protobuf.ByteString
getGraphInputNodeNameBytes(int index) {
return graphInputNodeName_.getByteString(index);
}
public static final int GRAPH_OUTPUT_NODE_NAME_FIELD_NUMBER = 3;
private com.google.protobuf.LazyStringList graphOutputNodeName_;
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public com.google.protobuf.ProtocolStringList
getGraphOutputNodeNameList() {
return graphOutputNodeName_;
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public int getGraphOutputNodeNameCount() {
return graphOutputNodeName_.size();
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public java.lang.String getGraphOutputNodeName(int index) {
return graphOutputNodeName_.get(index);
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public com.google.protobuf.ByteString
getGraphOutputNodeNameBytes(int index) {
return graphOutputNodeName_.getByteString(index);
}
public static final int EXECUTOR_NAME_FIELD_NUMBER = 4;
private volatile java.lang.Object executorName_;
/**
*
* Executor's name
*
*
* string executor_name = 4;
*/
public java.lang.String getExecutorName() {
java.lang.Object ref = executorName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executorName_ = s;
return s;
}
}
/**
*
* Executor's name
*
*
* string executor_name = 4;
*/
public com.google.protobuf.ByteString
getExecutorNameBytes() {
java.lang.Object ref = executorName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERIALIZED_EXECUTOR_PARAMETERS_FIELD_NUMBER = 5;
private com.google.protobuf.ByteString serializedExecutorParameters_;
/**
*
* Optional: Parameters given to the executor
*
*
* bytes serialized_executor_parameters = 5;
*/
public com.google.protobuf.ByteString getSerializedExecutorParameters() {
return serializedExecutorParameters_;
}
public static final int DEFAULT_GRAPH_INPUT_TENSOR_SHAPE_FIELD_NUMBER = 6;
private java.util.List defaultGraphInputTensorShape_;
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public java.util.List getDefaultGraphInputTensorShapeList() {
return defaultGraphInputTensorShape_;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public java.util.List extends org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>
getDefaultGraphInputTensorShapeOrBuilderList() {
return defaultGraphInputTensorShape_;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public int getDefaultGraphInputTensorShapeCount() {
return defaultGraphInputTensorShape_.size();
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto getDefaultGraphInputTensorShape(int index) {
return defaultGraphInputTensorShape_.get(index);
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder getDefaultGraphInputTensorShapeOrBuilder(
int index) {
return defaultGraphInputTensorShape_.get(index);
}
public static final int DEFAULT_GRAPH_OUTPUT_TENSOR_SHAPE_FIELD_NUMBER = 7;
private java.util.List defaultGraphOutputTensorShape_;
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public java.util.List getDefaultGraphOutputTensorShapeList() {
return defaultGraphOutputTensorShape_;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public java.util.List extends org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>
getDefaultGraphOutputTensorShapeOrBuilderList() {
return defaultGraphOutputTensorShape_;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public int getDefaultGraphOutputTensorShapeCount() {
return defaultGraphOutputTensorShape_.size();
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto getDefaultGraphOutputTensorShape(int index) {
return defaultGraphOutputTensorShape_.get(index);
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder getDefaultGraphOutputTensorShapeOrBuilder(
int index) {
return defaultGraphOutputTensorShape_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (remoteGraph_ != null) {
output.writeMessage(1, getRemoteGraph());
}
for (int i = 0; i < graphInputNodeName_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, graphInputNodeName_.getRaw(i));
}
for (int i = 0; i < graphOutputNodeName_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, graphOutputNodeName_.getRaw(i));
}
if (!getExecutorNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, executorName_);
}
if (!serializedExecutorParameters_.isEmpty()) {
output.writeBytes(5, serializedExecutorParameters_);
}
for (int i = 0; i < defaultGraphInputTensorShape_.size(); i++) {
output.writeMessage(6, defaultGraphInputTensorShape_.get(i));
}
for (int i = 0; i < defaultGraphOutputTensorShape_.size(); i++) {
output.writeMessage(7, defaultGraphOutputTensorShape_.get(i));
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (remoteGraph_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getRemoteGraph());
}
{
int dataSize = 0;
for (int i = 0; i < graphInputNodeName_.size(); i++) {
dataSize += computeStringSizeNoTag(graphInputNodeName_.getRaw(i));
}
size += dataSize;
size += 1 * getGraphInputNodeNameList().size();
}
{
int dataSize = 0;
for (int i = 0; i < graphOutputNodeName_.size(); i++) {
dataSize += computeStringSizeNoTag(graphOutputNodeName_.getRaw(i));
}
size += dataSize;
size += 1 * getGraphOutputNodeNameList().size();
}
if (!getExecutorNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, executorName_);
}
if (!serializedExecutorParameters_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(5, serializedExecutorParameters_);
}
for (int i = 0; i < defaultGraphInputTensorShape_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, defaultGraphInputTensorShape_.get(i));
}
for (int i = 0; i < defaultGraphOutputTensorShape_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, defaultGraphOutputTensorShape_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.RemoteFusedGraphExecuteInfo)) {
return super.equals(obj);
}
org.tensorflow.framework.RemoteFusedGraphExecuteInfo other = (org.tensorflow.framework.RemoteFusedGraphExecuteInfo) obj;
boolean result = true;
result = result && (hasRemoteGraph() == other.hasRemoteGraph());
if (hasRemoteGraph()) {
result = result && getRemoteGraph()
.equals(other.getRemoteGraph());
}
result = result && getGraphInputNodeNameList()
.equals(other.getGraphInputNodeNameList());
result = result && getGraphOutputNodeNameList()
.equals(other.getGraphOutputNodeNameList());
result = result && getExecutorName()
.equals(other.getExecutorName());
result = result && getSerializedExecutorParameters()
.equals(other.getSerializedExecutorParameters());
result = result && getDefaultGraphInputTensorShapeList()
.equals(other.getDefaultGraphInputTensorShapeList());
result = result && getDefaultGraphOutputTensorShapeList()
.equals(other.getDefaultGraphOutputTensorShapeList());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasRemoteGraph()) {
hash = (37 * hash) + REMOTE_GRAPH_FIELD_NUMBER;
hash = (53 * hash) + getRemoteGraph().hashCode();
}
if (getGraphInputNodeNameCount() > 0) {
hash = (37 * hash) + GRAPH_INPUT_NODE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getGraphInputNodeNameList().hashCode();
}
if (getGraphOutputNodeNameCount() > 0) {
hash = (37 * hash) + GRAPH_OUTPUT_NODE_NAME_FIELD_NUMBER;
hash = (53 * hash) + getGraphOutputNodeNameList().hashCode();
}
hash = (37 * hash) + EXECUTOR_NAME_FIELD_NUMBER;
hash = (53 * hash) + getExecutorName().hashCode();
hash = (37 * hash) + SERIALIZED_EXECUTOR_PARAMETERS_FIELD_NUMBER;
hash = (53 * hash) + getSerializedExecutorParameters().hashCode();
if (getDefaultGraphInputTensorShapeCount() > 0) {
hash = (37 * hash) + DEFAULT_GRAPH_INPUT_TENSOR_SHAPE_FIELD_NUMBER;
hash = (53 * hash) + getDefaultGraphInputTensorShapeList().hashCode();
}
if (getDefaultGraphOutputTensorShapeCount() > 0) {
hash = (37 * hash) + DEFAULT_GRAPH_OUTPUT_TENSOR_SHAPE_FIELD_NUMBER;
hash = (53 * hash) + getDefaultGraphOutputTensorShapeList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.RemoteFusedGraphExecuteInfo prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Protocol buffer representing a handle to a tensorflow resource. Handles are
* not valid across executions, but can be serialized back and forth from within
* a single run.
*
*
* Protobuf type {@code tensorflow.RemoteFusedGraphExecuteInfo}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.RemoteFusedGraphExecuteInfo)
org.tensorflow.framework.RemoteFusedGraphExecuteInfoOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.class, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.Builder.class);
}
// Construct using org.tensorflow.framework.RemoteFusedGraphExecuteInfo.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getDefaultGraphInputTensorShapeFieldBuilder();
getDefaultGraphOutputTensorShapeFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (remoteGraphBuilder_ == null) {
remoteGraph_ = null;
} else {
remoteGraph_ = null;
remoteGraphBuilder_ = null;
}
graphInputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
graphOutputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
executorName_ = "";
serializedExecutorParameters_ = com.google.protobuf.ByteString.EMPTY;
if (defaultGraphInputTensorShapeBuilder_ == null) {
defaultGraphInputTensorShape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
} else {
defaultGraphInputTensorShapeBuilder_.clear();
}
if (defaultGraphOutputTensorShapeBuilder_ == null) {
defaultGraphOutputTensorShape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
} else {
defaultGraphOutputTensorShapeBuilder_.clear();
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfoProto.internal_static_tensorflow_RemoteFusedGraphExecuteInfo_descriptor;
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo getDefaultInstanceForType() {
return org.tensorflow.framework.RemoteFusedGraphExecuteInfo.getDefaultInstance();
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo build() {
org.tensorflow.framework.RemoteFusedGraphExecuteInfo result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo buildPartial() {
org.tensorflow.framework.RemoteFusedGraphExecuteInfo result = new org.tensorflow.framework.RemoteFusedGraphExecuteInfo(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (remoteGraphBuilder_ == null) {
result.remoteGraph_ = remoteGraph_;
} else {
result.remoteGraph_ = remoteGraphBuilder_.build();
}
if (((bitField0_ & 0x00000002) == 0x00000002)) {
graphInputNodeName_ = graphInputNodeName_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.graphInputNodeName_ = graphInputNodeName_;
if (((bitField0_ & 0x00000004) == 0x00000004)) {
graphOutputNodeName_ = graphOutputNodeName_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.graphOutputNodeName_ = graphOutputNodeName_;
result.executorName_ = executorName_;
result.serializedExecutorParameters_ = serializedExecutorParameters_;
if (defaultGraphInputTensorShapeBuilder_ == null) {
if (((bitField0_ & 0x00000020) == 0x00000020)) {
defaultGraphInputTensorShape_ = java.util.Collections.unmodifiableList(defaultGraphInputTensorShape_);
bitField0_ = (bitField0_ & ~0x00000020);
}
result.defaultGraphInputTensorShape_ = defaultGraphInputTensorShape_;
} else {
result.defaultGraphInputTensorShape_ = defaultGraphInputTensorShapeBuilder_.build();
}
if (defaultGraphOutputTensorShapeBuilder_ == null) {
if (((bitField0_ & 0x00000040) == 0x00000040)) {
defaultGraphOutputTensorShape_ = java.util.Collections.unmodifiableList(defaultGraphOutputTensorShape_);
bitField0_ = (bitField0_ & ~0x00000040);
}
result.defaultGraphOutputTensorShape_ = defaultGraphOutputTensorShape_;
} else {
result.defaultGraphOutputTensorShape_ = defaultGraphOutputTensorShapeBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.RemoteFusedGraphExecuteInfo) {
return mergeFrom((org.tensorflow.framework.RemoteFusedGraphExecuteInfo)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.RemoteFusedGraphExecuteInfo other) {
if (other == org.tensorflow.framework.RemoteFusedGraphExecuteInfo.getDefaultInstance()) return this;
if (other.hasRemoteGraph()) {
mergeRemoteGraph(other.getRemoteGraph());
}
if (!other.graphInputNodeName_.isEmpty()) {
if (graphInputNodeName_.isEmpty()) {
graphInputNodeName_ = other.graphInputNodeName_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureGraphInputNodeNameIsMutable();
graphInputNodeName_.addAll(other.graphInputNodeName_);
}
onChanged();
}
if (!other.graphOutputNodeName_.isEmpty()) {
if (graphOutputNodeName_.isEmpty()) {
graphOutputNodeName_ = other.graphOutputNodeName_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureGraphOutputNodeNameIsMutable();
graphOutputNodeName_.addAll(other.graphOutputNodeName_);
}
onChanged();
}
if (!other.getExecutorName().isEmpty()) {
executorName_ = other.executorName_;
onChanged();
}
if (other.getSerializedExecutorParameters() != com.google.protobuf.ByteString.EMPTY) {
setSerializedExecutorParameters(other.getSerializedExecutorParameters());
}
if (defaultGraphInputTensorShapeBuilder_ == null) {
if (!other.defaultGraphInputTensorShape_.isEmpty()) {
if (defaultGraphInputTensorShape_.isEmpty()) {
defaultGraphInputTensorShape_ = other.defaultGraphInputTensorShape_;
bitField0_ = (bitField0_ & ~0x00000020);
} else {
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.addAll(other.defaultGraphInputTensorShape_);
}
onChanged();
}
} else {
if (!other.defaultGraphInputTensorShape_.isEmpty()) {
if (defaultGraphInputTensorShapeBuilder_.isEmpty()) {
defaultGraphInputTensorShapeBuilder_.dispose();
defaultGraphInputTensorShapeBuilder_ = null;
defaultGraphInputTensorShape_ = other.defaultGraphInputTensorShape_;
bitField0_ = (bitField0_ & ~0x00000020);
defaultGraphInputTensorShapeBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDefaultGraphInputTensorShapeFieldBuilder() : null;
} else {
defaultGraphInputTensorShapeBuilder_.addAllMessages(other.defaultGraphInputTensorShape_);
}
}
}
if (defaultGraphOutputTensorShapeBuilder_ == null) {
if (!other.defaultGraphOutputTensorShape_.isEmpty()) {
if (defaultGraphOutputTensorShape_.isEmpty()) {
defaultGraphOutputTensorShape_ = other.defaultGraphOutputTensorShape_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.addAll(other.defaultGraphOutputTensorShape_);
}
onChanged();
}
} else {
if (!other.defaultGraphOutputTensorShape_.isEmpty()) {
if (defaultGraphOutputTensorShapeBuilder_.isEmpty()) {
defaultGraphOutputTensorShapeBuilder_.dispose();
defaultGraphOutputTensorShapeBuilder_ = null;
defaultGraphOutputTensorShape_ = other.defaultGraphOutputTensorShape_;
bitField0_ = (bitField0_ & ~0x00000040);
defaultGraphOutputTensorShapeBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getDefaultGraphOutputTensorShapeFieldBuilder() : null;
} else {
defaultGraphOutputTensorShapeBuilder_.addAllMessages(other.defaultGraphOutputTensorShape_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.RemoteFusedGraphExecuteInfo parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.RemoteFusedGraphExecuteInfo) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private org.tensorflow.framework.GraphDef remoteGraph_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.GraphDef, org.tensorflow.framework.GraphDef.Builder, org.tensorflow.framework.GraphDefOrBuilder> remoteGraphBuilder_;
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public boolean hasRemoteGraph() {
return remoteGraphBuilder_ != null || remoteGraph_ != null;
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public org.tensorflow.framework.GraphDef getRemoteGraph() {
if (remoteGraphBuilder_ == null) {
return remoteGraph_ == null ? org.tensorflow.framework.GraphDef.getDefaultInstance() : remoteGraph_;
} else {
return remoteGraphBuilder_.getMessage();
}
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public Builder setRemoteGraph(org.tensorflow.framework.GraphDef value) {
if (remoteGraphBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
remoteGraph_ = value;
onChanged();
} else {
remoteGraphBuilder_.setMessage(value);
}
return this;
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public Builder setRemoteGraph(
org.tensorflow.framework.GraphDef.Builder builderForValue) {
if (remoteGraphBuilder_ == null) {
remoteGraph_ = builderForValue.build();
onChanged();
} else {
remoteGraphBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public Builder mergeRemoteGraph(org.tensorflow.framework.GraphDef value) {
if (remoteGraphBuilder_ == null) {
if (remoteGraph_ != null) {
remoteGraph_ =
org.tensorflow.framework.GraphDef.newBuilder(remoteGraph_).mergeFrom(value).buildPartial();
} else {
remoteGraph_ = value;
}
onChanged();
} else {
remoteGraphBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public Builder clearRemoteGraph() {
if (remoteGraphBuilder_ == null) {
remoteGraph_ = null;
onChanged();
} else {
remoteGraph_ = null;
remoteGraphBuilder_ = null;
}
return this;
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public org.tensorflow.framework.GraphDef.Builder getRemoteGraphBuilder() {
onChanged();
return getRemoteGraphFieldBuilder().getBuilder();
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
public org.tensorflow.framework.GraphDefOrBuilder getRemoteGraphOrBuilder() {
if (remoteGraphBuilder_ != null) {
return remoteGraphBuilder_.getMessageOrBuilder();
} else {
return remoteGraph_ == null ?
org.tensorflow.framework.GraphDef.getDefaultInstance() : remoteGraph_;
}
}
/**
*
* Definition of remote graph
*
*
* .tensorflow.GraphDef remote_graph = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.GraphDef, org.tensorflow.framework.GraphDef.Builder, org.tensorflow.framework.GraphDefOrBuilder>
getRemoteGraphFieldBuilder() {
if (remoteGraphBuilder_ == null) {
remoteGraphBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.GraphDef, org.tensorflow.framework.GraphDef.Builder, org.tensorflow.framework.GraphDefOrBuilder>(
getRemoteGraph(),
getParentForChildren(),
isClean());
remoteGraph_ = null;
}
return remoteGraphBuilder_;
}
private com.google.protobuf.LazyStringList graphInputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureGraphInputNodeNameIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
graphInputNodeName_ = new com.google.protobuf.LazyStringArrayList(graphInputNodeName_);
bitField0_ |= 0x00000002;
}
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public com.google.protobuf.ProtocolStringList
getGraphInputNodeNameList() {
return graphInputNodeName_.getUnmodifiableView();
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public int getGraphInputNodeNameCount() {
return graphInputNodeName_.size();
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public java.lang.String getGraphInputNodeName(int index) {
return graphInputNodeName_.get(index);
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public com.google.protobuf.ByteString
getGraphInputNodeNameBytes(int index) {
return graphInputNodeName_.getByteString(index);
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public Builder setGraphInputNodeName(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphInputNodeNameIsMutable();
graphInputNodeName_.set(index, value);
onChanged();
return this;
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public Builder addGraphInputNodeName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphInputNodeNameIsMutable();
graphInputNodeName_.add(value);
onChanged();
return this;
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public Builder addAllGraphInputNodeName(
java.lang.Iterable values) {
ensureGraphInputNodeNameIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, graphInputNodeName_);
onChanged();
return this;
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public Builder clearGraphInputNodeName() {
graphInputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* Remote fused graph input node name
*
*
* repeated string graph_input_node_name = 2;
*/
public Builder addGraphInputNodeNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureGraphInputNodeNameIsMutable();
graphInputNodeName_.add(value);
onChanged();
return this;
}
private com.google.protobuf.LazyStringList graphOutputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureGraphOutputNodeNameIsMutable() {
if (!((bitField0_ & 0x00000004) == 0x00000004)) {
graphOutputNodeName_ = new com.google.protobuf.LazyStringArrayList(graphOutputNodeName_);
bitField0_ |= 0x00000004;
}
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public com.google.protobuf.ProtocolStringList
getGraphOutputNodeNameList() {
return graphOutputNodeName_.getUnmodifiableView();
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public int getGraphOutputNodeNameCount() {
return graphOutputNodeName_.size();
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public java.lang.String getGraphOutputNodeName(int index) {
return graphOutputNodeName_.get(index);
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public com.google.protobuf.ByteString
getGraphOutputNodeNameBytes(int index) {
return graphOutputNodeName_.getByteString(index);
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public Builder setGraphOutputNodeName(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphOutputNodeNameIsMutable();
graphOutputNodeName_.set(index, value);
onChanged();
return this;
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public Builder addGraphOutputNodeName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureGraphOutputNodeNameIsMutable();
graphOutputNodeName_.add(value);
onChanged();
return this;
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public Builder addAllGraphOutputNodeName(
java.lang.Iterable values) {
ensureGraphOutputNodeNameIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, graphOutputNodeName_);
onChanged();
return this;
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public Builder clearGraphOutputNodeName() {
graphOutputNodeName_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* Remote fused graph output node name
*
*
* repeated string graph_output_node_name = 3;
*/
public Builder addGraphOutputNodeNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureGraphOutputNodeNameIsMutable();
graphOutputNodeName_.add(value);
onChanged();
return this;
}
private java.lang.Object executorName_ = "";
/**
*
* Executor's name
*
*
* string executor_name = 4;
*/
public java.lang.String getExecutorName() {
java.lang.Object ref = executorName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
executorName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Executor's name
*
*
* string executor_name = 4;
*/
public com.google.protobuf.ByteString
getExecutorNameBytes() {
java.lang.Object ref = executorName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
executorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Executor's name
*
*
* string executor_name = 4;
*/
public Builder setExecutorName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
executorName_ = value;
onChanged();
return this;
}
/**
*
* Executor's name
*
*
* string executor_name = 4;
*/
public Builder clearExecutorName() {
executorName_ = getDefaultInstance().getExecutorName();
onChanged();
return this;
}
/**
*
* Executor's name
*
*
* string executor_name = 4;
*/
public Builder setExecutorNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
executorName_ = value;
onChanged();
return this;
}
private com.google.protobuf.ByteString serializedExecutorParameters_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Optional: Parameters given to the executor
*
*
* bytes serialized_executor_parameters = 5;
*/
public com.google.protobuf.ByteString getSerializedExecutorParameters() {
return serializedExecutorParameters_;
}
/**
*
* Optional: Parameters given to the executor
*
*
* bytes serialized_executor_parameters = 5;
*/
public Builder setSerializedExecutorParameters(com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
serializedExecutorParameters_ = value;
onChanged();
return this;
}
/**
*
* Optional: Parameters given to the executor
*
*
* bytes serialized_executor_parameters = 5;
*/
public Builder clearSerializedExecutorParameters() {
serializedExecutorParameters_ = getDefaultInstance().getSerializedExecutorParameters();
onChanged();
return this;
}
private java.util.List defaultGraphInputTensorShape_ =
java.util.Collections.emptyList();
private void ensureDefaultGraphInputTensorShapeIsMutable() {
if (!((bitField0_ & 0x00000020) == 0x00000020)) {
defaultGraphInputTensorShape_ = new java.util.ArrayList(defaultGraphInputTensorShape_);
bitField0_ |= 0x00000020;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder> defaultGraphInputTensorShapeBuilder_;
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public java.util.List getDefaultGraphInputTensorShapeList() {
if (defaultGraphInputTensorShapeBuilder_ == null) {
return java.util.Collections.unmodifiableList(defaultGraphInputTensorShape_);
} else {
return defaultGraphInputTensorShapeBuilder_.getMessageList();
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public int getDefaultGraphInputTensorShapeCount() {
if (defaultGraphInputTensorShapeBuilder_ == null) {
return defaultGraphInputTensorShape_.size();
} else {
return defaultGraphInputTensorShapeBuilder_.getCount();
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto getDefaultGraphInputTensorShape(int index) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
return defaultGraphInputTensorShape_.get(index);
} else {
return defaultGraphInputTensorShapeBuilder_.getMessage(index);
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder setDefaultGraphInputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto value) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.set(index, value);
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder setDefaultGraphInputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder builderForValue) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.set(index, builderForValue.build());
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder addDefaultGraphInputTensorShape(org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto value) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.add(value);
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.addMessage(value);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder addDefaultGraphInputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto value) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.add(index, value);
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder addDefaultGraphInputTensorShape(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder builderForValue) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.add(builderForValue.build());
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder addDefaultGraphInputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder builderForValue) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.add(index, builderForValue.build());
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder addAllDefaultGraphInputTensorShape(
java.lang.Iterable extends org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto> values) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
ensureDefaultGraphInputTensorShapeIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, defaultGraphInputTensorShape_);
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder clearDefaultGraphInputTensorShape() {
if (defaultGraphInputTensorShapeBuilder_ == null) {
defaultGraphInputTensorShape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.clear();
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public Builder removeDefaultGraphInputTensorShape(int index) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
ensureDefaultGraphInputTensorShapeIsMutable();
defaultGraphInputTensorShape_.remove(index);
onChanged();
} else {
defaultGraphInputTensorShapeBuilder_.remove(index);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder getDefaultGraphInputTensorShapeBuilder(
int index) {
return getDefaultGraphInputTensorShapeFieldBuilder().getBuilder(index);
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder getDefaultGraphInputTensorShapeOrBuilder(
int index) {
if (defaultGraphInputTensorShapeBuilder_ == null) {
return defaultGraphInputTensorShape_.get(index); } else {
return defaultGraphInputTensorShapeBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public java.util.List extends org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>
getDefaultGraphInputTensorShapeOrBuilderList() {
if (defaultGraphInputTensorShapeBuilder_ != null) {
return defaultGraphInputTensorShapeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(defaultGraphInputTensorShape_);
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder addDefaultGraphInputTensorShapeBuilder() {
return getDefaultGraphInputTensorShapeFieldBuilder().addBuilder(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.getDefaultInstance());
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder addDefaultGraphInputTensorShapeBuilder(
int index) {
return getDefaultGraphInputTensorShapeFieldBuilder().addBuilder(
index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.getDefaultInstance());
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_input_tensor_shape = 6;
*/
public java.util.List
getDefaultGraphInputTensorShapeBuilderList() {
return getDefaultGraphInputTensorShapeFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>
getDefaultGraphInputTensorShapeFieldBuilder() {
if (defaultGraphInputTensorShapeBuilder_ == null) {
defaultGraphInputTensorShapeBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>(
defaultGraphInputTensorShape_,
((bitField0_ & 0x00000020) == 0x00000020),
getParentForChildren(),
isClean());
defaultGraphInputTensorShape_ = null;
}
return defaultGraphInputTensorShapeBuilder_;
}
private java.util.List defaultGraphOutputTensorShape_ =
java.util.Collections.emptyList();
private void ensureDefaultGraphOutputTensorShapeIsMutable() {
if (!((bitField0_ & 0x00000040) == 0x00000040)) {
defaultGraphOutputTensorShape_ = new java.util.ArrayList(defaultGraphOutputTensorShape_);
bitField0_ |= 0x00000040;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder> defaultGraphOutputTensorShapeBuilder_;
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public java.util.List getDefaultGraphOutputTensorShapeList() {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
return java.util.Collections.unmodifiableList(defaultGraphOutputTensorShape_);
} else {
return defaultGraphOutputTensorShapeBuilder_.getMessageList();
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public int getDefaultGraphOutputTensorShapeCount() {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
return defaultGraphOutputTensorShape_.size();
} else {
return defaultGraphOutputTensorShapeBuilder_.getCount();
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto getDefaultGraphOutputTensorShape(int index) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
return defaultGraphOutputTensorShape_.get(index);
} else {
return defaultGraphOutputTensorShapeBuilder_.getMessage(index);
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder setDefaultGraphOutputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto value) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.set(index, value);
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder setDefaultGraphOutputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder builderForValue) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.set(index, builderForValue.build());
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder addDefaultGraphOutputTensorShape(org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto value) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.add(value);
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.addMessage(value);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder addDefaultGraphOutputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto value) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.add(index, value);
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder addDefaultGraphOutputTensorShape(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder builderForValue) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.add(builderForValue.build());
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder addDefaultGraphOutputTensorShape(
int index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder builderForValue) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.add(index, builderForValue.build());
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder addAllDefaultGraphOutputTensorShape(
java.lang.Iterable extends org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto> values) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
ensureDefaultGraphOutputTensorShapeIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, defaultGraphOutputTensorShape_);
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder clearDefaultGraphOutputTensorShape() {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
defaultGraphOutputTensorShape_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.clear();
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public Builder removeDefaultGraphOutputTensorShape(int index) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
ensureDefaultGraphOutputTensorShapeIsMutable();
defaultGraphOutputTensorShape_.remove(index);
onChanged();
} else {
defaultGraphOutputTensorShapeBuilder_.remove(index);
}
return this;
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder getDefaultGraphOutputTensorShapeBuilder(
int index) {
return getDefaultGraphOutputTensorShapeFieldBuilder().getBuilder(index);
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder getDefaultGraphOutputTensorShapeOrBuilder(
int index) {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
return defaultGraphOutputTensorShape_.get(index); } else {
return defaultGraphOutputTensorShapeBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public java.util.List extends org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>
getDefaultGraphOutputTensorShapeOrBuilderList() {
if (defaultGraphOutputTensorShapeBuilder_ != null) {
return defaultGraphOutputTensorShapeBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(defaultGraphOutputTensorShape_);
}
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder addDefaultGraphOutputTensorShapeBuilder() {
return getDefaultGraphOutputTensorShapeFieldBuilder().addBuilder(
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.getDefaultInstance());
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder addDefaultGraphOutputTensorShapeBuilder(
int index) {
return getDefaultGraphOutputTensorShapeFieldBuilder().addBuilder(
index, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.getDefaultInstance());
}
/**
*
* Optional: Default graph input tensor shape used to allocate memory
* before executing op
* TODO(satok): Remote output tensor shape once shape information is stored
* in NodeDef
*
*
* repeated .tensorflow.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto default_graph_output_tensor_shape = 7;
*/
public java.util.List
getDefaultGraphOutputTensorShapeBuilderList() {
return getDefaultGraphOutputTensorShapeFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>
getDefaultGraphOutputTensorShapeFieldBuilder() {
if (defaultGraphOutputTensorShapeBuilder_ == null) {
defaultGraphOutputTensorShapeBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProto.Builder, org.tensorflow.framework.RemoteFusedGraphExecuteInfo.TensorShapeTypeProtoOrBuilder>(
defaultGraphOutputTensorShape_,
((bitField0_ & 0x00000040) == 0x00000040),
getParentForChildren(),
isClean());
defaultGraphOutputTensorShape_ = null;
}
return defaultGraphOutputTensorShapeBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.RemoteFusedGraphExecuteInfo)
}
// @@protoc_insertion_point(class_scope:tensorflow.RemoteFusedGraphExecuteInfo)
private static final org.tensorflow.framework.RemoteFusedGraphExecuteInfo DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.RemoteFusedGraphExecuteInfo();
}
public static org.tensorflow.framework.RemoteFusedGraphExecuteInfo getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RemoteFusedGraphExecuteInfo parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RemoteFusedGraphExecuteInfo(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.RemoteFusedGraphExecuteInfo getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy