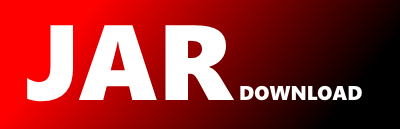
org.tensorflow.framework.RunOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/config.proto
package org.tensorflow.framework;
/**
*
* Options for a single Run() call.
*
*
* Protobuf type {@code tensorflow.RunOptions}
*/
public final class RunOptions extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.RunOptions)
RunOptionsOrBuilder {
private static final long serialVersionUID = 0L;
// Use RunOptions.newBuilder() to construct.
private RunOptions(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RunOptions() {
traceLevel_ = 0;
timeoutInMs_ = 0L;
interOpThreadPool_ = 0;
outputPartitionGraphs_ = false;
reportTensorAllocationsUponOom_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RunOptions(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
traceLevel_ = rawValue;
break;
}
case 16: {
timeoutInMs_ = input.readInt64();
break;
}
case 24: {
interOpThreadPool_ = input.readInt32();
break;
}
case 40: {
outputPartitionGraphs_ = input.readBool();
break;
}
case 50: {
org.tensorflow.framework.DebugOptions.Builder subBuilder = null;
if (debugOptions_ != null) {
subBuilder = debugOptions_.toBuilder();
}
debugOptions_ = input.readMessage(org.tensorflow.framework.DebugOptions.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(debugOptions_);
debugOptions_ = subBuilder.buildPartial();
}
break;
}
case 56: {
reportTensorAllocationsUponOom_ = input.readBool();
break;
}
case 66: {
org.tensorflow.framework.RunOptions.Experimental.Builder subBuilder = null;
if (experimental_ != null) {
subBuilder = experimental_.toBuilder();
}
experimental_ = input.readMessage(org.tensorflow.framework.RunOptions.Experimental.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(experimental_);
experimental_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RunOptions.class, org.tensorflow.framework.RunOptions.Builder.class);
}
/**
*
* TODO(pbar) Turn this into a TraceOptions proto which allows
* tracing to be controlled in a more orthogonal manner?
*
*
* Protobuf enum {@code tensorflow.RunOptions.TraceLevel}
*/
public enum TraceLevel
implements com.google.protobuf.ProtocolMessageEnum {
/**
* NO_TRACE = 0;
*/
NO_TRACE(0),
/**
* SOFTWARE_TRACE = 1;
*/
SOFTWARE_TRACE(1),
/**
* HARDWARE_TRACE = 2;
*/
HARDWARE_TRACE(2),
/**
* FULL_TRACE = 3;
*/
FULL_TRACE(3),
UNRECOGNIZED(-1),
;
/**
* NO_TRACE = 0;
*/
public static final int NO_TRACE_VALUE = 0;
/**
* SOFTWARE_TRACE = 1;
*/
public static final int SOFTWARE_TRACE_VALUE = 1;
/**
* HARDWARE_TRACE = 2;
*/
public static final int HARDWARE_TRACE_VALUE = 2;
/**
* FULL_TRACE = 3;
*/
public static final int FULL_TRACE_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static TraceLevel valueOf(int value) {
return forNumber(value);
}
public static TraceLevel forNumber(int value) {
switch (value) {
case 0: return NO_TRACE;
case 1: return SOFTWARE_TRACE;
case 2: return HARDWARE_TRACE;
case 3: return FULL_TRACE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
TraceLevel> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public TraceLevel findValueByNumber(int number) {
return TraceLevel.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tensorflow.framework.RunOptions.getDescriptor().getEnumTypes().get(0);
}
private static final TraceLevel[] VALUES = values();
public static TraceLevel valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private TraceLevel(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.RunOptions.TraceLevel)
}
public interface ExperimentalOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.RunOptions.Experimental)
com.google.protobuf.MessageOrBuilder {
/**
*
* If non-zero, declares that this graph is going to use collective
* ops and must synchronize step_ids with any other graph with this
* same group_key value (in a distributed computation where tasks
* run disjoint graphs).
*
*
* int64 collective_graph_key = 1;
*/
long getCollectiveGraphKey();
/**
*
* If true, then operations (using the inter-op pool) across all
* session::run() calls will be centrally scheduled, optimizing for (median
* and tail) latency.
* Consider using this option for CPU-bound workloads like inference.
*
*
* bool use_run_handler_pool = 2;
*/
boolean getUseRunHandlerPool();
}
/**
*
* Everything inside Experimental is subject to change and is not subject
* to API stability guarantees in
* https://www.tensorflow.org/guide/version_compat.
*
*
* Protobuf type {@code tensorflow.RunOptions.Experimental}
*/
public static final class Experimental extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.RunOptions.Experimental)
ExperimentalOrBuilder {
private static final long serialVersionUID = 0L;
// Use Experimental.newBuilder() to construct.
private Experimental(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Experimental() {
collectiveGraphKey_ = 0L;
useRunHandlerPool_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Experimental(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
collectiveGraphKey_ = input.readInt64();
break;
}
case 16: {
useRunHandlerPool_ = input.readBool();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_Experimental_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_Experimental_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RunOptions.Experimental.class, org.tensorflow.framework.RunOptions.Experimental.Builder.class);
}
public static final int COLLECTIVE_GRAPH_KEY_FIELD_NUMBER = 1;
private long collectiveGraphKey_;
/**
*
* If non-zero, declares that this graph is going to use collective
* ops and must synchronize step_ids with any other graph with this
* same group_key value (in a distributed computation where tasks
* run disjoint graphs).
*
*
* int64 collective_graph_key = 1;
*/
public long getCollectiveGraphKey() {
return collectiveGraphKey_;
}
public static final int USE_RUN_HANDLER_POOL_FIELD_NUMBER = 2;
private boolean useRunHandlerPool_;
/**
*
* If true, then operations (using the inter-op pool) across all
* session::run() calls will be centrally scheduled, optimizing for (median
* and tail) latency.
* Consider using this option for CPU-bound workloads like inference.
*
*
* bool use_run_handler_pool = 2;
*/
public boolean getUseRunHandlerPool() {
return useRunHandlerPool_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (collectiveGraphKey_ != 0L) {
output.writeInt64(1, collectiveGraphKey_);
}
if (useRunHandlerPool_ != false) {
output.writeBool(2, useRunHandlerPool_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (collectiveGraphKey_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(1, collectiveGraphKey_);
}
if (useRunHandlerPool_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, useRunHandlerPool_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.RunOptions.Experimental)) {
return super.equals(obj);
}
org.tensorflow.framework.RunOptions.Experimental other = (org.tensorflow.framework.RunOptions.Experimental) obj;
boolean result = true;
result = result && (getCollectiveGraphKey()
== other.getCollectiveGraphKey());
result = result && (getUseRunHandlerPool()
== other.getUseRunHandlerPool());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + COLLECTIVE_GRAPH_KEY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCollectiveGraphKey());
hash = (37 * hash) + USE_RUN_HANDLER_POOL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseRunHandlerPool());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions.Experimental parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RunOptions.Experimental parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RunOptions.Experimental parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.RunOptions.Experimental prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Everything inside Experimental is subject to change and is not subject
* to API stability guarantees in
* https://www.tensorflow.org/guide/version_compat.
*
*
* Protobuf type {@code tensorflow.RunOptions.Experimental}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.RunOptions.Experimental)
org.tensorflow.framework.RunOptions.ExperimentalOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_Experimental_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_Experimental_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RunOptions.Experimental.class, org.tensorflow.framework.RunOptions.Experimental.Builder.class);
}
// Construct using org.tensorflow.framework.RunOptions.Experimental.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
collectiveGraphKey_ = 0L;
useRunHandlerPool_ = false;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_Experimental_descriptor;
}
public org.tensorflow.framework.RunOptions.Experimental getDefaultInstanceForType() {
return org.tensorflow.framework.RunOptions.Experimental.getDefaultInstance();
}
public org.tensorflow.framework.RunOptions.Experimental build() {
org.tensorflow.framework.RunOptions.Experimental result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.RunOptions.Experimental buildPartial() {
org.tensorflow.framework.RunOptions.Experimental result = new org.tensorflow.framework.RunOptions.Experimental(this);
result.collectiveGraphKey_ = collectiveGraphKey_;
result.useRunHandlerPool_ = useRunHandlerPool_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.RunOptions.Experimental) {
return mergeFrom((org.tensorflow.framework.RunOptions.Experimental)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.RunOptions.Experimental other) {
if (other == org.tensorflow.framework.RunOptions.Experimental.getDefaultInstance()) return this;
if (other.getCollectiveGraphKey() != 0L) {
setCollectiveGraphKey(other.getCollectiveGraphKey());
}
if (other.getUseRunHandlerPool() != false) {
setUseRunHandlerPool(other.getUseRunHandlerPool());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.RunOptions.Experimental parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.RunOptions.Experimental) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private long collectiveGraphKey_ ;
/**
*
* If non-zero, declares that this graph is going to use collective
* ops and must synchronize step_ids with any other graph with this
* same group_key value (in a distributed computation where tasks
* run disjoint graphs).
*
*
* int64 collective_graph_key = 1;
*/
public long getCollectiveGraphKey() {
return collectiveGraphKey_;
}
/**
*
* If non-zero, declares that this graph is going to use collective
* ops and must synchronize step_ids with any other graph with this
* same group_key value (in a distributed computation where tasks
* run disjoint graphs).
*
*
* int64 collective_graph_key = 1;
*/
public Builder setCollectiveGraphKey(long value) {
collectiveGraphKey_ = value;
onChanged();
return this;
}
/**
*
* If non-zero, declares that this graph is going to use collective
* ops and must synchronize step_ids with any other graph with this
* same group_key value (in a distributed computation where tasks
* run disjoint graphs).
*
*
* int64 collective_graph_key = 1;
*/
public Builder clearCollectiveGraphKey() {
collectiveGraphKey_ = 0L;
onChanged();
return this;
}
private boolean useRunHandlerPool_ ;
/**
*
* If true, then operations (using the inter-op pool) across all
* session::run() calls will be centrally scheduled, optimizing for (median
* and tail) latency.
* Consider using this option for CPU-bound workloads like inference.
*
*
* bool use_run_handler_pool = 2;
*/
public boolean getUseRunHandlerPool() {
return useRunHandlerPool_;
}
/**
*
* If true, then operations (using the inter-op pool) across all
* session::run() calls will be centrally scheduled, optimizing for (median
* and tail) latency.
* Consider using this option for CPU-bound workloads like inference.
*
*
* bool use_run_handler_pool = 2;
*/
public Builder setUseRunHandlerPool(boolean value) {
useRunHandlerPool_ = value;
onChanged();
return this;
}
/**
*
* If true, then operations (using the inter-op pool) across all
* session::run() calls will be centrally scheduled, optimizing for (median
* and tail) latency.
* Consider using this option for CPU-bound workloads like inference.
*
*
* bool use_run_handler_pool = 2;
*/
public Builder clearUseRunHandlerPool() {
useRunHandlerPool_ = false;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.RunOptions.Experimental)
}
// @@protoc_insertion_point(class_scope:tensorflow.RunOptions.Experimental)
private static final org.tensorflow.framework.RunOptions.Experimental DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.RunOptions.Experimental();
}
public static org.tensorflow.framework.RunOptions.Experimental getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public Experimental parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Experimental(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.RunOptions.Experimental getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int TRACE_LEVEL_FIELD_NUMBER = 1;
private int traceLevel_;
/**
* .tensorflow.RunOptions.TraceLevel trace_level = 1;
*/
public int getTraceLevelValue() {
return traceLevel_;
}
/**
* .tensorflow.RunOptions.TraceLevel trace_level = 1;
*/
public org.tensorflow.framework.RunOptions.TraceLevel getTraceLevel() {
org.tensorflow.framework.RunOptions.TraceLevel result = org.tensorflow.framework.RunOptions.TraceLevel.valueOf(traceLevel_);
return result == null ? org.tensorflow.framework.RunOptions.TraceLevel.UNRECOGNIZED : result;
}
public static final int TIMEOUT_IN_MS_FIELD_NUMBER = 2;
private long timeoutInMs_;
/**
*
* Time to wait for operation to complete in milliseconds.
*
*
* int64 timeout_in_ms = 2;
*/
public long getTimeoutInMs() {
return timeoutInMs_;
}
public static final int INTER_OP_THREAD_POOL_FIELD_NUMBER = 3;
private int interOpThreadPool_;
/**
*
* The thread pool to use, if session_inter_op_thread_pool is configured.
* To use the caller thread set this to -1 - this uses the caller thread
* to execute Session::Run() and thus avoids a context switch. Using the
* caller thread to execute Session::Run() should be done ONLY for simple
* graphs, where the overhead of an additional context switch is
* comparable with the overhead of Session::Run().
*
*
* int32 inter_op_thread_pool = 3;
*/
public int getInterOpThreadPool() {
return interOpThreadPool_;
}
public static final int OUTPUT_PARTITION_GRAPHS_FIELD_NUMBER = 5;
private boolean outputPartitionGraphs_;
/**
*
* Whether the partition graph(s) executed by the executor(s) should be
* outputted via RunMetadata.
*
*
* bool output_partition_graphs = 5;
*/
public boolean getOutputPartitionGraphs() {
return outputPartitionGraphs_;
}
public static final int DEBUG_OPTIONS_FIELD_NUMBER = 6;
private org.tensorflow.framework.DebugOptions debugOptions_;
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public boolean hasDebugOptions() {
return debugOptions_ != null;
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public org.tensorflow.framework.DebugOptions getDebugOptions() {
return debugOptions_ == null ? org.tensorflow.framework.DebugOptions.getDefaultInstance() : debugOptions_;
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public org.tensorflow.framework.DebugOptionsOrBuilder getDebugOptionsOrBuilder() {
return getDebugOptions();
}
public static final int REPORT_TENSOR_ALLOCATIONS_UPON_OOM_FIELD_NUMBER = 7;
private boolean reportTensorAllocationsUponOom_;
/**
*
* When enabled, causes tensor allocation information to be included in
* the error message when the Run() call fails because the allocator ran
* out of memory (OOM).
* Enabling this option can slow down the Run() call.
*
*
* bool report_tensor_allocations_upon_oom = 7;
*/
public boolean getReportTensorAllocationsUponOom() {
return reportTensorAllocationsUponOom_;
}
public static final int EXPERIMENTAL_FIELD_NUMBER = 8;
private org.tensorflow.framework.RunOptions.Experimental experimental_;
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public boolean hasExperimental() {
return experimental_ != null;
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public org.tensorflow.framework.RunOptions.Experimental getExperimental() {
return experimental_ == null ? org.tensorflow.framework.RunOptions.Experimental.getDefaultInstance() : experimental_;
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public org.tensorflow.framework.RunOptions.ExperimentalOrBuilder getExperimentalOrBuilder() {
return getExperimental();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (traceLevel_ != org.tensorflow.framework.RunOptions.TraceLevel.NO_TRACE.getNumber()) {
output.writeEnum(1, traceLevel_);
}
if (timeoutInMs_ != 0L) {
output.writeInt64(2, timeoutInMs_);
}
if (interOpThreadPool_ != 0) {
output.writeInt32(3, interOpThreadPool_);
}
if (outputPartitionGraphs_ != false) {
output.writeBool(5, outputPartitionGraphs_);
}
if (debugOptions_ != null) {
output.writeMessage(6, getDebugOptions());
}
if (reportTensorAllocationsUponOom_ != false) {
output.writeBool(7, reportTensorAllocationsUponOom_);
}
if (experimental_ != null) {
output.writeMessage(8, getExperimental());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (traceLevel_ != org.tensorflow.framework.RunOptions.TraceLevel.NO_TRACE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, traceLevel_);
}
if (timeoutInMs_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(2, timeoutInMs_);
}
if (interOpThreadPool_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(3, interOpThreadPool_);
}
if (outputPartitionGraphs_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, outputPartitionGraphs_);
}
if (debugOptions_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, getDebugOptions());
}
if (reportTensorAllocationsUponOom_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(7, reportTensorAllocationsUponOom_);
}
if (experimental_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, getExperimental());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.framework.RunOptions)) {
return super.equals(obj);
}
org.tensorflow.framework.RunOptions other = (org.tensorflow.framework.RunOptions) obj;
boolean result = true;
result = result && traceLevel_ == other.traceLevel_;
result = result && (getTimeoutInMs()
== other.getTimeoutInMs());
result = result && (getInterOpThreadPool()
== other.getInterOpThreadPool());
result = result && (getOutputPartitionGraphs()
== other.getOutputPartitionGraphs());
result = result && (hasDebugOptions() == other.hasDebugOptions());
if (hasDebugOptions()) {
result = result && getDebugOptions()
.equals(other.getDebugOptions());
}
result = result && (getReportTensorAllocationsUponOom()
== other.getReportTensorAllocationsUponOom());
result = result && (hasExperimental() == other.hasExperimental());
if (hasExperimental()) {
result = result && getExperimental()
.equals(other.getExperimental());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TRACE_LEVEL_FIELD_NUMBER;
hash = (53 * hash) + traceLevel_;
hash = (37 * hash) + TIMEOUT_IN_MS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeoutInMs());
hash = (37 * hash) + INTER_OP_THREAD_POOL_FIELD_NUMBER;
hash = (53 * hash) + getInterOpThreadPool();
hash = (37 * hash) + OUTPUT_PARTITION_GRAPHS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOutputPartitionGraphs());
if (hasDebugOptions()) {
hash = (37 * hash) + DEBUG_OPTIONS_FIELD_NUMBER;
hash = (53 * hash) + getDebugOptions().hashCode();
}
hash = (37 * hash) + REPORT_TENSOR_ALLOCATIONS_UPON_OOM_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getReportTensorAllocationsUponOom());
if (hasExperimental()) {
hash = (37 * hash) + EXPERIMENTAL_FIELD_NUMBER;
hash = (53 * hash) + getExperimental().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.framework.RunOptions parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RunOptions parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RunOptions parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.framework.RunOptions parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RunOptions parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RunOptions parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.framework.RunOptions parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.framework.RunOptions parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.framework.RunOptions prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Options for a single Run() call.
*
*
* Protobuf type {@code tensorflow.RunOptions}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.RunOptions)
org.tensorflow.framework.RunOptionsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.framework.RunOptions.class, org.tensorflow.framework.RunOptions.Builder.class);
}
// Construct using org.tensorflow.framework.RunOptions.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
traceLevel_ = 0;
timeoutInMs_ = 0L;
interOpThreadPool_ = 0;
outputPartitionGraphs_ = false;
if (debugOptionsBuilder_ == null) {
debugOptions_ = null;
} else {
debugOptions_ = null;
debugOptionsBuilder_ = null;
}
reportTensorAllocationsUponOom_ = false;
if (experimentalBuilder_ == null) {
experimental_ = null;
} else {
experimental_ = null;
experimentalBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.framework.ConfigProtos.internal_static_tensorflow_RunOptions_descriptor;
}
public org.tensorflow.framework.RunOptions getDefaultInstanceForType() {
return org.tensorflow.framework.RunOptions.getDefaultInstance();
}
public org.tensorflow.framework.RunOptions build() {
org.tensorflow.framework.RunOptions result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.framework.RunOptions buildPartial() {
org.tensorflow.framework.RunOptions result = new org.tensorflow.framework.RunOptions(this);
result.traceLevel_ = traceLevel_;
result.timeoutInMs_ = timeoutInMs_;
result.interOpThreadPool_ = interOpThreadPool_;
result.outputPartitionGraphs_ = outputPartitionGraphs_;
if (debugOptionsBuilder_ == null) {
result.debugOptions_ = debugOptions_;
} else {
result.debugOptions_ = debugOptionsBuilder_.build();
}
result.reportTensorAllocationsUponOom_ = reportTensorAllocationsUponOom_;
if (experimentalBuilder_ == null) {
result.experimental_ = experimental_;
} else {
result.experimental_ = experimentalBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.framework.RunOptions) {
return mergeFrom((org.tensorflow.framework.RunOptions)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.framework.RunOptions other) {
if (other == org.tensorflow.framework.RunOptions.getDefaultInstance()) return this;
if (other.traceLevel_ != 0) {
setTraceLevelValue(other.getTraceLevelValue());
}
if (other.getTimeoutInMs() != 0L) {
setTimeoutInMs(other.getTimeoutInMs());
}
if (other.getInterOpThreadPool() != 0) {
setInterOpThreadPool(other.getInterOpThreadPool());
}
if (other.getOutputPartitionGraphs() != false) {
setOutputPartitionGraphs(other.getOutputPartitionGraphs());
}
if (other.hasDebugOptions()) {
mergeDebugOptions(other.getDebugOptions());
}
if (other.getReportTensorAllocationsUponOom() != false) {
setReportTensorAllocationsUponOom(other.getReportTensorAllocationsUponOom());
}
if (other.hasExperimental()) {
mergeExperimental(other.getExperimental());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.framework.RunOptions parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.framework.RunOptions) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int traceLevel_ = 0;
/**
* .tensorflow.RunOptions.TraceLevel trace_level = 1;
*/
public int getTraceLevelValue() {
return traceLevel_;
}
/**
* .tensorflow.RunOptions.TraceLevel trace_level = 1;
*/
public Builder setTraceLevelValue(int value) {
traceLevel_ = value;
onChanged();
return this;
}
/**
* .tensorflow.RunOptions.TraceLevel trace_level = 1;
*/
public org.tensorflow.framework.RunOptions.TraceLevel getTraceLevel() {
org.tensorflow.framework.RunOptions.TraceLevel result = org.tensorflow.framework.RunOptions.TraceLevel.valueOf(traceLevel_);
return result == null ? org.tensorflow.framework.RunOptions.TraceLevel.UNRECOGNIZED : result;
}
/**
* .tensorflow.RunOptions.TraceLevel trace_level = 1;
*/
public Builder setTraceLevel(org.tensorflow.framework.RunOptions.TraceLevel value) {
if (value == null) {
throw new NullPointerException();
}
traceLevel_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.RunOptions.TraceLevel trace_level = 1;
*/
public Builder clearTraceLevel() {
traceLevel_ = 0;
onChanged();
return this;
}
private long timeoutInMs_ ;
/**
*
* Time to wait for operation to complete in milliseconds.
*
*
* int64 timeout_in_ms = 2;
*/
public long getTimeoutInMs() {
return timeoutInMs_;
}
/**
*
* Time to wait for operation to complete in milliseconds.
*
*
* int64 timeout_in_ms = 2;
*/
public Builder setTimeoutInMs(long value) {
timeoutInMs_ = value;
onChanged();
return this;
}
/**
*
* Time to wait for operation to complete in milliseconds.
*
*
* int64 timeout_in_ms = 2;
*/
public Builder clearTimeoutInMs() {
timeoutInMs_ = 0L;
onChanged();
return this;
}
private int interOpThreadPool_ ;
/**
*
* The thread pool to use, if session_inter_op_thread_pool is configured.
* To use the caller thread set this to -1 - this uses the caller thread
* to execute Session::Run() and thus avoids a context switch. Using the
* caller thread to execute Session::Run() should be done ONLY for simple
* graphs, where the overhead of an additional context switch is
* comparable with the overhead of Session::Run().
*
*
* int32 inter_op_thread_pool = 3;
*/
public int getInterOpThreadPool() {
return interOpThreadPool_;
}
/**
*
* The thread pool to use, if session_inter_op_thread_pool is configured.
* To use the caller thread set this to -1 - this uses the caller thread
* to execute Session::Run() and thus avoids a context switch. Using the
* caller thread to execute Session::Run() should be done ONLY for simple
* graphs, where the overhead of an additional context switch is
* comparable with the overhead of Session::Run().
*
*
* int32 inter_op_thread_pool = 3;
*/
public Builder setInterOpThreadPool(int value) {
interOpThreadPool_ = value;
onChanged();
return this;
}
/**
*
* The thread pool to use, if session_inter_op_thread_pool is configured.
* To use the caller thread set this to -1 - this uses the caller thread
* to execute Session::Run() and thus avoids a context switch. Using the
* caller thread to execute Session::Run() should be done ONLY for simple
* graphs, where the overhead of an additional context switch is
* comparable with the overhead of Session::Run().
*
*
* int32 inter_op_thread_pool = 3;
*/
public Builder clearInterOpThreadPool() {
interOpThreadPool_ = 0;
onChanged();
return this;
}
private boolean outputPartitionGraphs_ ;
/**
*
* Whether the partition graph(s) executed by the executor(s) should be
* outputted via RunMetadata.
*
*
* bool output_partition_graphs = 5;
*/
public boolean getOutputPartitionGraphs() {
return outputPartitionGraphs_;
}
/**
*
* Whether the partition graph(s) executed by the executor(s) should be
* outputted via RunMetadata.
*
*
* bool output_partition_graphs = 5;
*/
public Builder setOutputPartitionGraphs(boolean value) {
outputPartitionGraphs_ = value;
onChanged();
return this;
}
/**
*
* Whether the partition graph(s) executed by the executor(s) should be
* outputted via RunMetadata.
*
*
* bool output_partition_graphs = 5;
*/
public Builder clearOutputPartitionGraphs() {
outputPartitionGraphs_ = false;
onChanged();
return this;
}
private org.tensorflow.framework.DebugOptions debugOptions_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.DebugOptions, org.tensorflow.framework.DebugOptions.Builder, org.tensorflow.framework.DebugOptionsOrBuilder> debugOptionsBuilder_;
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public boolean hasDebugOptions() {
return debugOptionsBuilder_ != null || debugOptions_ != null;
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public org.tensorflow.framework.DebugOptions getDebugOptions() {
if (debugOptionsBuilder_ == null) {
return debugOptions_ == null ? org.tensorflow.framework.DebugOptions.getDefaultInstance() : debugOptions_;
} else {
return debugOptionsBuilder_.getMessage();
}
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public Builder setDebugOptions(org.tensorflow.framework.DebugOptions value) {
if (debugOptionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
debugOptions_ = value;
onChanged();
} else {
debugOptionsBuilder_.setMessage(value);
}
return this;
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public Builder setDebugOptions(
org.tensorflow.framework.DebugOptions.Builder builderForValue) {
if (debugOptionsBuilder_ == null) {
debugOptions_ = builderForValue.build();
onChanged();
} else {
debugOptionsBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public Builder mergeDebugOptions(org.tensorflow.framework.DebugOptions value) {
if (debugOptionsBuilder_ == null) {
if (debugOptions_ != null) {
debugOptions_ =
org.tensorflow.framework.DebugOptions.newBuilder(debugOptions_).mergeFrom(value).buildPartial();
} else {
debugOptions_ = value;
}
onChanged();
} else {
debugOptionsBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public Builder clearDebugOptions() {
if (debugOptionsBuilder_ == null) {
debugOptions_ = null;
onChanged();
} else {
debugOptions_ = null;
debugOptionsBuilder_ = null;
}
return this;
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public org.tensorflow.framework.DebugOptions.Builder getDebugOptionsBuilder() {
onChanged();
return getDebugOptionsFieldBuilder().getBuilder();
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
public org.tensorflow.framework.DebugOptionsOrBuilder getDebugOptionsOrBuilder() {
if (debugOptionsBuilder_ != null) {
return debugOptionsBuilder_.getMessageOrBuilder();
} else {
return debugOptions_ == null ?
org.tensorflow.framework.DebugOptions.getDefaultInstance() : debugOptions_;
}
}
/**
*
* EXPERIMENTAL. Options used to initialize DebuggerState, if enabled.
*
*
* .tensorflow.DebugOptions debug_options = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.DebugOptions, org.tensorflow.framework.DebugOptions.Builder, org.tensorflow.framework.DebugOptionsOrBuilder>
getDebugOptionsFieldBuilder() {
if (debugOptionsBuilder_ == null) {
debugOptionsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.DebugOptions, org.tensorflow.framework.DebugOptions.Builder, org.tensorflow.framework.DebugOptionsOrBuilder>(
getDebugOptions(),
getParentForChildren(),
isClean());
debugOptions_ = null;
}
return debugOptionsBuilder_;
}
private boolean reportTensorAllocationsUponOom_ ;
/**
*
* When enabled, causes tensor allocation information to be included in
* the error message when the Run() call fails because the allocator ran
* out of memory (OOM).
* Enabling this option can slow down the Run() call.
*
*
* bool report_tensor_allocations_upon_oom = 7;
*/
public boolean getReportTensorAllocationsUponOom() {
return reportTensorAllocationsUponOom_;
}
/**
*
* When enabled, causes tensor allocation information to be included in
* the error message when the Run() call fails because the allocator ran
* out of memory (OOM).
* Enabling this option can slow down the Run() call.
*
*
* bool report_tensor_allocations_upon_oom = 7;
*/
public Builder setReportTensorAllocationsUponOom(boolean value) {
reportTensorAllocationsUponOom_ = value;
onChanged();
return this;
}
/**
*
* When enabled, causes tensor allocation information to be included in
* the error message when the Run() call fails because the allocator ran
* out of memory (OOM).
* Enabling this option can slow down the Run() call.
*
*
* bool report_tensor_allocations_upon_oom = 7;
*/
public Builder clearReportTensorAllocationsUponOom() {
reportTensorAllocationsUponOom_ = false;
onChanged();
return this;
}
private org.tensorflow.framework.RunOptions.Experimental experimental_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.RunOptions.Experimental, org.tensorflow.framework.RunOptions.Experimental.Builder, org.tensorflow.framework.RunOptions.ExperimentalOrBuilder> experimentalBuilder_;
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public boolean hasExperimental() {
return experimentalBuilder_ != null || experimental_ != null;
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public org.tensorflow.framework.RunOptions.Experimental getExperimental() {
if (experimentalBuilder_ == null) {
return experimental_ == null ? org.tensorflow.framework.RunOptions.Experimental.getDefaultInstance() : experimental_;
} else {
return experimentalBuilder_.getMessage();
}
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public Builder setExperimental(org.tensorflow.framework.RunOptions.Experimental value) {
if (experimentalBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
experimental_ = value;
onChanged();
} else {
experimentalBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public Builder setExperimental(
org.tensorflow.framework.RunOptions.Experimental.Builder builderForValue) {
if (experimentalBuilder_ == null) {
experimental_ = builderForValue.build();
onChanged();
} else {
experimentalBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public Builder mergeExperimental(org.tensorflow.framework.RunOptions.Experimental value) {
if (experimentalBuilder_ == null) {
if (experimental_ != null) {
experimental_ =
org.tensorflow.framework.RunOptions.Experimental.newBuilder(experimental_).mergeFrom(value).buildPartial();
} else {
experimental_ = value;
}
onChanged();
} else {
experimentalBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public Builder clearExperimental() {
if (experimentalBuilder_ == null) {
experimental_ = null;
onChanged();
} else {
experimental_ = null;
experimentalBuilder_ = null;
}
return this;
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public org.tensorflow.framework.RunOptions.Experimental.Builder getExperimentalBuilder() {
onChanged();
return getExperimentalFieldBuilder().getBuilder();
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
public org.tensorflow.framework.RunOptions.ExperimentalOrBuilder getExperimentalOrBuilder() {
if (experimentalBuilder_ != null) {
return experimentalBuilder_.getMessageOrBuilder();
} else {
return experimental_ == null ?
org.tensorflow.framework.RunOptions.Experimental.getDefaultInstance() : experimental_;
}
}
/**
* .tensorflow.RunOptions.Experimental experimental = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.RunOptions.Experimental, org.tensorflow.framework.RunOptions.Experimental.Builder, org.tensorflow.framework.RunOptions.ExperimentalOrBuilder>
getExperimentalFieldBuilder() {
if (experimentalBuilder_ == null) {
experimentalBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.RunOptions.Experimental, org.tensorflow.framework.RunOptions.Experimental.Builder, org.tensorflow.framework.RunOptions.ExperimentalOrBuilder>(
getExperimental(),
getParentForChildren(),
isClean());
experimental_ = null;
}
return experimentalBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.RunOptions)
}
// @@protoc_insertion_point(class_scope:tensorflow.RunOptions)
private static final org.tensorflow.framework.RunOptions DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.framework.RunOptions();
}
public static org.tensorflow.framework.RunOptions getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public RunOptions parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RunOptions(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.framework.RunOptions getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy