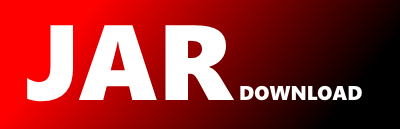
tensorflow.DevicePropertiesProtos Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/device_properties.proto
package tensorflow;
public final class DevicePropertiesProtos {
private DevicePropertiesProtos() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface DevicePropertiesOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.DeviceProperties)
com.google.protobuf.MessageOrBuilder {
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
java.lang.String getType();
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
com.google.protobuf.ByteString
getTypeBytes();
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
java.lang.String getVendor();
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
com.google.protobuf.ByteString
getVendorBytes();
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
java.lang.String getModel();
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
com.google.protobuf.ByteString
getModelBytes();
/**
*
* Core Frequency in Mhz
*
*
* int64 frequency = 4;
*/
long getFrequency();
/**
*
* Number of cores
*
*
* int64 num_cores = 5;
*/
long getNumCores();
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
int getEnvironmentCount();
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
boolean containsEnvironment(
java.lang.String key);
/**
* Use {@link #getEnvironmentMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getEnvironment();
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
java.util.Map
getEnvironmentMap();
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
java.lang.String getEnvironmentOrDefault(
java.lang.String key,
java.lang.String defaultValue);
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
java.lang.String getEnvironmentOrThrow(
java.lang.String key);
/**
*
* Number of registers per core.
*
*
* int64 num_registers = 7;
*/
long getNumRegisters();
/**
*
* L1 cache size in bytes
*
*
* int64 l1_cache_size = 8;
*/
long getL1CacheSize();
/**
*
* L2 cache size in bytes
*
*
* int64 l2_cache_size = 9;
*/
long getL2CacheSize();
/**
*
* L3 cache size in bytes
*
*
* int64 l3_cache_size = 10;
*/
long getL3CacheSize();
/**
*
* Shared memory size per multiprocessor in bytes. This field is
* applicable to GPUs only.
*
*
* int64 shared_memory_size_per_multiprocessor = 11;
*/
long getSharedMemorySizePerMultiprocessor();
/**
*
* Memory size in bytes
*
*
* int64 memory_size = 12;
*/
long getMemorySize();
/**
*
* Memory bandwidth in KB/s
*
*
* int64 bandwidth = 13;
*/
long getBandwidth();
}
/**
* Protobuf type {@code tensorflow.DeviceProperties}
*/
public static final class DeviceProperties extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.DeviceProperties)
DevicePropertiesOrBuilder {
private static final long serialVersionUID = 0L;
// Use DeviceProperties.newBuilder() to construct.
private DeviceProperties(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private DeviceProperties() {
type_ = "";
vendor_ = "";
model_ = "";
frequency_ = 0L;
numCores_ = 0L;
numRegisters_ = 0L;
l1CacheSize_ = 0L;
l2CacheSize_ = 0L;
l3CacheSize_ = 0L;
sharedMemorySizePerMultiprocessor_ = 0L;
memorySize_ = 0L;
bandwidth_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private DeviceProperties(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
type_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
vendor_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
model_ = s;
break;
}
case 32: {
frequency_ = input.readInt64();
break;
}
case 40: {
numCores_ = input.readInt64();
break;
}
case 50: {
if (!((mutable_bitField0_ & 0x00000020) == 0x00000020)) {
environment_ = com.google.protobuf.MapField.newMapField(
EnvironmentDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000020;
}
com.google.protobuf.MapEntry
environment__ = input.readMessage(
EnvironmentDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
environment_.getMutableMap().put(
environment__.getKey(), environment__.getValue());
break;
}
case 56: {
numRegisters_ = input.readInt64();
break;
}
case 64: {
l1CacheSize_ = input.readInt64();
break;
}
case 72: {
l2CacheSize_ = input.readInt64();
break;
}
case 80: {
l3CacheSize_ = input.readInt64();
break;
}
case 88: {
sharedMemorySizePerMultiprocessor_ = input.readInt64();
break;
}
case 96: {
memorySize_ = input.readInt64();
break;
}
case 104: {
bandwidth_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_DeviceProperties_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetEnvironment();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_DeviceProperties_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.DevicePropertiesProtos.DeviceProperties.class, tensorflow.DevicePropertiesProtos.DeviceProperties.Builder.class);
}
private int bitField0_;
public static final int TYPE_FIELD_NUMBER = 1;
private volatile java.lang.Object type_;
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
}
}
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VENDOR_FIELD_NUMBER = 2;
private volatile java.lang.Object vendor_;
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
public java.lang.String getVendor() {
java.lang.Object ref = vendor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendor_ = s;
return s;
}
}
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
public com.google.protobuf.ByteString
getVendorBytes() {
java.lang.Object ref = vendor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MODEL_FIELD_NUMBER = 3;
private volatile java.lang.Object model_;
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
model_ = s;
return s;
}
}
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FREQUENCY_FIELD_NUMBER = 4;
private long frequency_;
/**
*
* Core Frequency in Mhz
*
*
* int64 frequency = 4;
*/
public long getFrequency() {
return frequency_;
}
public static final int NUM_CORES_FIELD_NUMBER = 5;
private long numCores_;
/**
*
* Number of cores
*
*
* int64 num_cores = 5;
*/
public long getNumCores() {
return numCores_;
}
public static final int ENVIRONMENT_FIELD_NUMBER = 6;
private static final class EnvironmentDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, java.lang.String> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.DevicePropertiesProtos.internal_static_tensorflow_DeviceProperties_EnvironmentEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.STRING,
"");
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> environment_;
private com.google.protobuf.MapField
internalGetEnvironment() {
if (environment_ == null) {
return com.google.protobuf.MapField.emptyMapField(
EnvironmentDefaultEntryHolder.defaultEntry);
}
return environment_;
}
public int getEnvironmentCount() {
return internalGetEnvironment().getMap().size();
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public boolean containsEnvironment(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetEnvironment().getMap().containsKey(key);
}
/**
* Use {@link #getEnvironmentMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getEnvironment() {
return getEnvironmentMap();
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public java.util.Map getEnvironmentMap() {
return internalGetEnvironment().getMap();
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public java.lang.String getEnvironmentOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetEnvironment().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public java.lang.String getEnvironmentOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetEnvironment().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public static final int NUM_REGISTERS_FIELD_NUMBER = 7;
private long numRegisters_;
/**
*
* Number of registers per core.
*
*
* int64 num_registers = 7;
*/
public long getNumRegisters() {
return numRegisters_;
}
public static final int L1_CACHE_SIZE_FIELD_NUMBER = 8;
private long l1CacheSize_;
/**
*
* L1 cache size in bytes
*
*
* int64 l1_cache_size = 8;
*/
public long getL1CacheSize() {
return l1CacheSize_;
}
public static final int L2_CACHE_SIZE_FIELD_NUMBER = 9;
private long l2CacheSize_;
/**
*
* L2 cache size in bytes
*
*
* int64 l2_cache_size = 9;
*/
public long getL2CacheSize() {
return l2CacheSize_;
}
public static final int L3_CACHE_SIZE_FIELD_NUMBER = 10;
private long l3CacheSize_;
/**
*
* L3 cache size in bytes
*
*
* int64 l3_cache_size = 10;
*/
public long getL3CacheSize() {
return l3CacheSize_;
}
public static final int SHARED_MEMORY_SIZE_PER_MULTIPROCESSOR_FIELD_NUMBER = 11;
private long sharedMemorySizePerMultiprocessor_;
/**
*
* Shared memory size per multiprocessor in bytes. This field is
* applicable to GPUs only.
*
*
* int64 shared_memory_size_per_multiprocessor = 11;
*/
public long getSharedMemorySizePerMultiprocessor() {
return sharedMemorySizePerMultiprocessor_;
}
public static final int MEMORY_SIZE_FIELD_NUMBER = 12;
private long memorySize_;
/**
*
* Memory size in bytes
*
*
* int64 memory_size = 12;
*/
public long getMemorySize() {
return memorySize_;
}
public static final int BANDWIDTH_FIELD_NUMBER = 13;
private long bandwidth_;
/**
*
* Memory bandwidth in KB/s
*
*
* int64 bandwidth = 13;
*/
public long getBandwidth() {
return bandwidth_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTypeBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, type_);
}
if (!getVendorBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, vendor_);
}
if (!getModelBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, model_);
}
if (frequency_ != 0L) {
output.writeInt64(4, frequency_);
}
if (numCores_ != 0L) {
output.writeInt64(5, numCores_);
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetEnvironment(),
EnvironmentDefaultEntryHolder.defaultEntry,
6);
if (numRegisters_ != 0L) {
output.writeInt64(7, numRegisters_);
}
if (l1CacheSize_ != 0L) {
output.writeInt64(8, l1CacheSize_);
}
if (l2CacheSize_ != 0L) {
output.writeInt64(9, l2CacheSize_);
}
if (l3CacheSize_ != 0L) {
output.writeInt64(10, l3CacheSize_);
}
if (sharedMemorySizePerMultiprocessor_ != 0L) {
output.writeInt64(11, sharedMemorySizePerMultiprocessor_);
}
if (memorySize_ != 0L) {
output.writeInt64(12, memorySize_);
}
if (bandwidth_ != 0L) {
output.writeInt64(13, bandwidth_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTypeBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, type_);
}
if (!getVendorBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, vendor_);
}
if (!getModelBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, model_);
}
if (frequency_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(4, frequency_);
}
if (numCores_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(5, numCores_);
}
for (java.util.Map.Entry entry
: internalGetEnvironment().getMap().entrySet()) {
com.google.protobuf.MapEntry
environment__ = EnvironmentDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, environment__);
}
if (numRegisters_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(7, numRegisters_);
}
if (l1CacheSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(8, l1CacheSize_);
}
if (l2CacheSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(9, l2CacheSize_);
}
if (l3CacheSize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(10, l3CacheSize_);
}
if (sharedMemorySizePerMultiprocessor_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(11, sharedMemorySizePerMultiprocessor_);
}
if (memorySize_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(12, memorySize_);
}
if (bandwidth_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(13, bandwidth_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.DevicePropertiesProtos.DeviceProperties)) {
return super.equals(obj);
}
tensorflow.DevicePropertiesProtos.DeviceProperties other = (tensorflow.DevicePropertiesProtos.DeviceProperties) obj;
boolean result = true;
result = result && getType()
.equals(other.getType());
result = result && getVendor()
.equals(other.getVendor());
result = result && getModel()
.equals(other.getModel());
result = result && (getFrequency()
== other.getFrequency());
result = result && (getNumCores()
== other.getNumCores());
result = result && internalGetEnvironment().equals(
other.internalGetEnvironment());
result = result && (getNumRegisters()
== other.getNumRegisters());
result = result && (getL1CacheSize()
== other.getL1CacheSize());
result = result && (getL2CacheSize()
== other.getL2CacheSize());
result = result && (getL3CacheSize()
== other.getL3CacheSize());
result = result && (getSharedMemorySizePerMultiprocessor()
== other.getSharedMemorySizePerMultiprocessor());
result = result && (getMemorySize()
== other.getMemorySize());
result = result && (getBandwidth()
== other.getBandwidth());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + getType().hashCode();
hash = (37 * hash) + VENDOR_FIELD_NUMBER;
hash = (53 * hash) + getVendor().hashCode();
hash = (37 * hash) + MODEL_FIELD_NUMBER;
hash = (53 * hash) + getModel().hashCode();
hash = (37 * hash) + FREQUENCY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getFrequency());
hash = (37 * hash) + NUM_CORES_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumCores());
if (!internalGetEnvironment().getMap().isEmpty()) {
hash = (37 * hash) + ENVIRONMENT_FIELD_NUMBER;
hash = (53 * hash) + internalGetEnvironment().hashCode();
}
hash = (37 * hash) + NUM_REGISTERS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getNumRegisters());
hash = (37 * hash) + L1_CACHE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getL1CacheSize());
hash = (37 * hash) + L2_CACHE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getL2CacheSize());
hash = (37 * hash) + L3_CACHE_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getL3CacheSize());
hash = (37 * hash) + SHARED_MEMORY_SIZE_PER_MULTIPROCESSOR_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSharedMemorySizePerMultiprocessor());
hash = (37 * hash) + MEMORY_SIZE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMemorySize());
hash = (37 * hash) + BANDWIDTH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBandwidth());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.DevicePropertiesProtos.DeviceProperties prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.DeviceProperties}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.DeviceProperties)
tensorflow.DevicePropertiesProtos.DevicePropertiesOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_DeviceProperties_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 6:
return internalGetEnvironment();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 6:
return internalGetMutableEnvironment();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_DeviceProperties_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.DevicePropertiesProtos.DeviceProperties.class, tensorflow.DevicePropertiesProtos.DeviceProperties.Builder.class);
}
// Construct using tensorflow.DevicePropertiesProtos.DeviceProperties.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
type_ = "";
vendor_ = "";
model_ = "";
frequency_ = 0L;
numCores_ = 0L;
internalGetMutableEnvironment().clear();
numRegisters_ = 0L;
l1CacheSize_ = 0L;
l2CacheSize_ = 0L;
l3CacheSize_ = 0L;
sharedMemorySizePerMultiprocessor_ = 0L;
memorySize_ = 0L;
bandwidth_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_DeviceProperties_descriptor;
}
public tensorflow.DevicePropertiesProtos.DeviceProperties getDefaultInstanceForType() {
return tensorflow.DevicePropertiesProtos.DeviceProperties.getDefaultInstance();
}
public tensorflow.DevicePropertiesProtos.DeviceProperties build() {
tensorflow.DevicePropertiesProtos.DeviceProperties result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.DevicePropertiesProtos.DeviceProperties buildPartial() {
tensorflow.DevicePropertiesProtos.DeviceProperties result = new tensorflow.DevicePropertiesProtos.DeviceProperties(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.type_ = type_;
result.vendor_ = vendor_;
result.model_ = model_;
result.frequency_ = frequency_;
result.numCores_ = numCores_;
result.environment_ = internalGetEnvironment();
result.environment_.makeImmutable();
result.numRegisters_ = numRegisters_;
result.l1CacheSize_ = l1CacheSize_;
result.l2CacheSize_ = l2CacheSize_;
result.l3CacheSize_ = l3CacheSize_;
result.sharedMemorySizePerMultiprocessor_ = sharedMemorySizePerMultiprocessor_;
result.memorySize_ = memorySize_;
result.bandwidth_ = bandwidth_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.DevicePropertiesProtos.DeviceProperties) {
return mergeFrom((tensorflow.DevicePropertiesProtos.DeviceProperties)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.DevicePropertiesProtos.DeviceProperties other) {
if (other == tensorflow.DevicePropertiesProtos.DeviceProperties.getDefaultInstance()) return this;
if (!other.getType().isEmpty()) {
type_ = other.type_;
onChanged();
}
if (!other.getVendor().isEmpty()) {
vendor_ = other.vendor_;
onChanged();
}
if (!other.getModel().isEmpty()) {
model_ = other.model_;
onChanged();
}
if (other.getFrequency() != 0L) {
setFrequency(other.getFrequency());
}
if (other.getNumCores() != 0L) {
setNumCores(other.getNumCores());
}
internalGetMutableEnvironment().mergeFrom(
other.internalGetEnvironment());
if (other.getNumRegisters() != 0L) {
setNumRegisters(other.getNumRegisters());
}
if (other.getL1CacheSize() != 0L) {
setL1CacheSize(other.getL1CacheSize());
}
if (other.getL2CacheSize() != 0L) {
setL2CacheSize(other.getL2CacheSize());
}
if (other.getL3CacheSize() != 0L) {
setL3CacheSize(other.getL3CacheSize());
}
if (other.getSharedMemorySizePerMultiprocessor() != 0L) {
setSharedMemorySizePerMultiprocessor(other.getSharedMemorySizePerMultiprocessor());
}
if (other.getMemorySize() != 0L) {
setMemorySize(other.getMemorySize());
}
if (other.getBandwidth() != 0L) {
setBandwidth(other.getBandwidth());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.DevicePropertiesProtos.DeviceProperties parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.DevicePropertiesProtos.DeviceProperties) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object type_ = "";
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
public java.lang.String getType() {
java.lang.Object ref = type_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
type_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
public com.google.protobuf.ByteString
getTypeBytes() {
java.lang.Object ref = type_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
type_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
public Builder setType(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
type_ = value;
onChanged();
return this;
}
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
public Builder clearType() {
type_ = getDefaultInstance().getType();
onChanged();
return this;
}
/**
*
* Device type (CPU, GPU, ...)
*
*
* string type = 1;
*/
public Builder setTypeBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
type_ = value;
onChanged();
return this;
}
private java.lang.Object vendor_ = "";
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
public java.lang.String getVendor() {
java.lang.Object ref = vendor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
public com.google.protobuf.ByteString
getVendorBytes() {
java.lang.Object ref = vendor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
public Builder setVendor(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
vendor_ = value;
onChanged();
return this;
}
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
public Builder clearVendor() {
vendor_ = getDefaultInstance().getVendor();
onChanged();
return this;
}
/**
*
* Vendor (Intel, nvidia, ...)
*
*
* string vendor = 2;
*/
public Builder setVendorBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
vendor_ = value;
onChanged();
return this;
}
private java.lang.Object model_ = "";
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
public java.lang.String getModel() {
java.lang.Object ref = model_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
model_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
public com.google.protobuf.ByteString
getModelBytes() {
java.lang.Object ref = model_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
model_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
public Builder setModel(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
model_ = value;
onChanged();
return this;
}
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
public Builder clearModel() {
model_ = getDefaultInstance().getModel();
onChanged();
return this;
}
/**
*
* Model (Haswell, K40, ...)
*
*
* string model = 3;
*/
public Builder setModelBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
model_ = value;
onChanged();
return this;
}
private long frequency_ ;
/**
*
* Core Frequency in Mhz
*
*
* int64 frequency = 4;
*/
public long getFrequency() {
return frequency_;
}
/**
*
* Core Frequency in Mhz
*
*
* int64 frequency = 4;
*/
public Builder setFrequency(long value) {
frequency_ = value;
onChanged();
return this;
}
/**
*
* Core Frequency in Mhz
*
*
* int64 frequency = 4;
*/
public Builder clearFrequency() {
frequency_ = 0L;
onChanged();
return this;
}
private long numCores_ ;
/**
*
* Number of cores
*
*
* int64 num_cores = 5;
*/
public long getNumCores() {
return numCores_;
}
/**
*
* Number of cores
*
*
* int64 num_cores = 5;
*/
public Builder setNumCores(long value) {
numCores_ = value;
onChanged();
return this;
}
/**
*
* Number of cores
*
*
* int64 num_cores = 5;
*/
public Builder clearNumCores() {
numCores_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.MapField<
java.lang.String, java.lang.String> environment_;
private com.google.protobuf.MapField
internalGetEnvironment() {
if (environment_ == null) {
return com.google.protobuf.MapField.emptyMapField(
EnvironmentDefaultEntryHolder.defaultEntry);
}
return environment_;
}
private com.google.protobuf.MapField
internalGetMutableEnvironment() {
onChanged();;
if (environment_ == null) {
environment_ = com.google.protobuf.MapField.newMapField(
EnvironmentDefaultEntryHolder.defaultEntry);
}
if (!environment_.isMutable()) {
environment_ = environment_.copy();
}
return environment_;
}
public int getEnvironmentCount() {
return internalGetEnvironment().getMap().size();
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public boolean containsEnvironment(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetEnvironment().getMap().containsKey(key);
}
/**
* Use {@link #getEnvironmentMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getEnvironment() {
return getEnvironmentMap();
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public java.util.Map getEnvironmentMap() {
return internalGetEnvironment().getMap();
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public java.lang.String getEnvironmentOrDefault(
java.lang.String key,
java.lang.String defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetEnvironment().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public java.lang.String getEnvironmentOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetEnvironment().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearEnvironment() {
internalGetMutableEnvironment().getMutableMap()
.clear();
return this;
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public Builder removeEnvironment(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableEnvironment().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableEnvironment() {
return internalGetMutableEnvironment().getMutableMap();
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public Builder putEnvironment(
java.lang.String key,
java.lang.String value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableEnvironment().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Version of the tools and libraries used with this device (e.g. gcc 4.9,
* cudnn 5.1)
*
*
* map<string, string> environment = 6;
*/
public Builder putAllEnvironment(
java.util.Map values) {
internalGetMutableEnvironment().getMutableMap()
.putAll(values);
return this;
}
private long numRegisters_ ;
/**
*
* Number of registers per core.
*
*
* int64 num_registers = 7;
*/
public long getNumRegisters() {
return numRegisters_;
}
/**
*
* Number of registers per core.
*
*
* int64 num_registers = 7;
*/
public Builder setNumRegisters(long value) {
numRegisters_ = value;
onChanged();
return this;
}
/**
*
* Number of registers per core.
*
*
* int64 num_registers = 7;
*/
public Builder clearNumRegisters() {
numRegisters_ = 0L;
onChanged();
return this;
}
private long l1CacheSize_ ;
/**
*
* L1 cache size in bytes
*
*
* int64 l1_cache_size = 8;
*/
public long getL1CacheSize() {
return l1CacheSize_;
}
/**
*
* L1 cache size in bytes
*
*
* int64 l1_cache_size = 8;
*/
public Builder setL1CacheSize(long value) {
l1CacheSize_ = value;
onChanged();
return this;
}
/**
*
* L1 cache size in bytes
*
*
* int64 l1_cache_size = 8;
*/
public Builder clearL1CacheSize() {
l1CacheSize_ = 0L;
onChanged();
return this;
}
private long l2CacheSize_ ;
/**
*
* L2 cache size in bytes
*
*
* int64 l2_cache_size = 9;
*/
public long getL2CacheSize() {
return l2CacheSize_;
}
/**
*
* L2 cache size in bytes
*
*
* int64 l2_cache_size = 9;
*/
public Builder setL2CacheSize(long value) {
l2CacheSize_ = value;
onChanged();
return this;
}
/**
*
* L2 cache size in bytes
*
*
* int64 l2_cache_size = 9;
*/
public Builder clearL2CacheSize() {
l2CacheSize_ = 0L;
onChanged();
return this;
}
private long l3CacheSize_ ;
/**
*
* L3 cache size in bytes
*
*
* int64 l3_cache_size = 10;
*/
public long getL3CacheSize() {
return l3CacheSize_;
}
/**
*
* L3 cache size in bytes
*
*
* int64 l3_cache_size = 10;
*/
public Builder setL3CacheSize(long value) {
l3CacheSize_ = value;
onChanged();
return this;
}
/**
*
* L3 cache size in bytes
*
*
* int64 l3_cache_size = 10;
*/
public Builder clearL3CacheSize() {
l3CacheSize_ = 0L;
onChanged();
return this;
}
private long sharedMemorySizePerMultiprocessor_ ;
/**
*
* Shared memory size per multiprocessor in bytes. This field is
* applicable to GPUs only.
*
*
* int64 shared_memory_size_per_multiprocessor = 11;
*/
public long getSharedMemorySizePerMultiprocessor() {
return sharedMemorySizePerMultiprocessor_;
}
/**
*
* Shared memory size per multiprocessor in bytes. This field is
* applicable to GPUs only.
*
*
* int64 shared_memory_size_per_multiprocessor = 11;
*/
public Builder setSharedMemorySizePerMultiprocessor(long value) {
sharedMemorySizePerMultiprocessor_ = value;
onChanged();
return this;
}
/**
*
* Shared memory size per multiprocessor in bytes. This field is
* applicable to GPUs only.
*
*
* int64 shared_memory_size_per_multiprocessor = 11;
*/
public Builder clearSharedMemorySizePerMultiprocessor() {
sharedMemorySizePerMultiprocessor_ = 0L;
onChanged();
return this;
}
private long memorySize_ ;
/**
*
* Memory size in bytes
*
*
* int64 memory_size = 12;
*/
public long getMemorySize() {
return memorySize_;
}
/**
*
* Memory size in bytes
*
*
* int64 memory_size = 12;
*/
public Builder setMemorySize(long value) {
memorySize_ = value;
onChanged();
return this;
}
/**
*
* Memory size in bytes
*
*
* int64 memory_size = 12;
*/
public Builder clearMemorySize() {
memorySize_ = 0L;
onChanged();
return this;
}
private long bandwidth_ ;
/**
*
* Memory bandwidth in KB/s
*
*
* int64 bandwidth = 13;
*/
public long getBandwidth() {
return bandwidth_;
}
/**
*
* Memory bandwidth in KB/s
*
*
* int64 bandwidth = 13;
*/
public Builder setBandwidth(long value) {
bandwidth_ = value;
onChanged();
return this;
}
/**
*
* Memory bandwidth in KB/s
*
*
* int64 bandwidth = 13;
*/
public Builder clearBandwidth() {
bandwidth_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.DeviceProperties)
}
// @@protoc_insertion_point(class_scope:tensorflow.DeviceProperties)
private static final tensorflow.DevicePropertiesProtos.DeviceProperties DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.DevicePropertiesProtos.DeviceProperties();
}
public static tensorflow.DevicePropertiesProtos.DeviceProperties getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public DeviceProperties parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new DeviceProperties(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.DevicePropertiesProtos.DeviceProperties getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface NamedDeviceOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.NamedDevice)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
*/
java.lang.String getName();
/**
* string name = 1;
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* .tensorflow.DeviceProperties properties = 2;
*/
boolean hasProperties();
/**
* .tensorflow.DeviceProperties properties = 2;
*/
tensorflow.DevicePropertiesProtos.DeviceProperties getProperties();
/**
* .tensorflow.DeviceProperties properties = 2;
*/
tensorflow.DevicePropertiesProtos.DevicePropertiesOrBuilder getPropertiesOrBuilder();
}
/**
* Protobuf type {@code tensorflow.NamedDevice}
*/
public static final class NamedDevice extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.NamedDevice)
NamedDeviceOrBuilder {
private static final long serialVersionUID = 0L;
// Use NamedDevice.newBuilder() to construct.
private NamedDevice(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private NamedDevice() {
name_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private NamedDevice(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
tensorflow.DevicePropertiesProtos.DeviceProperties.Builder subBuilder = null;
if (properties_ != null) {
subBuilder = properties_.toBuilder();
}
properties_ = input.readMessage(tensorflow.DevicePropertiesProtos.DeviceProperties.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(properties_);
properties_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_NamedDevice_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_NamedDevice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.DevicePropertiesProtos.NamedDevice.class, tensorflow.DevicePropertiesProtos.NamedDevice.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PROPERTIES_FIELD_NUMBER = 2;
private tensorflow.DevicePropertiesProtos.DeviceProperties properties_;
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public boolean hasProperties() {
return properties_ != null;
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public tensorflow.DevicePropertiesProtos.DeviceProperties getProperties() {
return properties_ == null ? tensorflow.DevicePropertiesProtos.DeviceProperties.getDefaultInstance() : properties_;
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public tensorflow.DevicePropertiesProtos.DevicePropertiesOrBuilder getPropertiesOrBuilder() {
return getProperties();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (properties_ != null) {
output.writeMessage(2, getProperties());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (properties_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getProperties());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.DevicePropertiesProtos.NamedDevice)) {
return super.equals(obj);
}
tensorflow.DevicePropertiesProtos.NamedDevice other = (tensorflow.DevicePropertiesProtos.NamedDevice) obj;
boolean result = true;
result = result && getName()
.equals(other.getName());
result = result && (hasProperties() == other.hasProperties());
if (hasProperties()) {
result = result && getProperties()
.equals(other.getProperties());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (hasProperties()) {
hash = (37 * hash) + PROPERTIES_FIELD_NUMBER;
hash = (53 * hash) + getProperties().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.DevicePropertiesProtos.NamedDevice parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.DevicePropertiesProtos.NamedDevice prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.NamedDevice}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.NamedDevice)
tensorflow.DevicePropertiesProtos.NamedDeviceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_NamedDevice_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_NamedDevice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.DevicePropertiesProtos.NamedDevice.class, tensorflow.DevicePropertiesProtos.NamedDevice.Builder.class);
}
// Construct using tensorflow.DevicePropertiesProtos.NamedDevice.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
name_ = "";
if (propertiesBuilder_ == null) {
properties_ = null;
} else {
properties_ = null;
propertiesBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.DevicePropertiesProtos.internal_static_tensorflow_NamedDevice_descriptor;
}
public tensorflow.DevicePropertiesProtos.NamedDevice getDefaultInstanceForType() {
return tensorflow.DevicePropertiesProtos.NamedDevice.getDefaultInstance();
}
public tensorflow.DevicePropertiesProtos.NamedDevice build() {
tensorflow.DevicePropertiesProtos.NamedDevice result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.DevicePropertiesProtos.NamedDevice buildPartial() {
tensorflow.DevicePropertiesProtos.NamedDevice result = new tensorflow.DevicePropertiesProtos.NamedDevice(this);
result.name_ = name_;
if (propertiesBuilder_ == null) {
result.properties_ = properties_;
} else {
result.properties_ = propertiesBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.DevicePropertiesProtos.NamedDevice) {
return mergeFrom((tensorflow.DevicePropertiesProtos.NamedDevice)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.DevicePropertiesProtos.NamedDevice other) {
if (other == tensorflow.DevicePropertiesProtos.NamedDevice.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.hasProperties()) {
mergeProperties(other.getProperties());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.DevicePropertiesProtos.NamedDevice parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.DevicePropertiesProtos.NamedDevice) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private tensorflow.DevicePropertiesProtos.DeviceProperties properties_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.DevicePropertiesProtos.DeviceProperties, tensorflow.DevicePropertiesProtos.DeviceProperties.Builder, tensorflow.DevicePropertiesProtos.DevicePropertiesOrBuilder> propertiesBuilder_;
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public boolean hasProperties() {
return propertiesBuilder_ != null || properties_ != null;
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public tensorflow.DevicePropertiesProtos.DeviceProperties getProperties() {
if (propertiesBuilder_ == null) {
return properties_ == null ? tensorflow.DevicePropertiesProtos.DeviceProperties.getDefaultInstance() : properties_;
} else {
return propertiesBuilder_.getMessage();
}
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public Builder setProperties(tensorflow.DevicePropertiesProtos.DeviceProperties value) {
if (propertiesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
properties_ = value;
onChanged();
} else {
propertiesBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public Builder setProperties(
tensorflow.DevicePropertiesProtos.DeviceProperties.Builder builderForValue) {
if (propertiesBuilder_ == null) {
properties_ = builderForValue.build();
onChanged();
} else {
propertiesBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public Builder mergeProperties(tensorflow.DevicePropertiesProtos.DeviceProperties value) {
if (propertiesBuilder_ == null) {
if (properties_ != null) {
properties_ =
tensorflow.DevicePropertiesProtos.DeviceProperties.newBuilder(properties_).mergeFrom(value).buildPartial();
} else {
properties_ = value;
}
onChanged();
} else {
propertiesBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public Builder clearProperties() {
if (propertiesBuilder_ == null) {
properties_ = null;
onChanged();
} else {
properties_ = null;
propertiesBuilder_ = null;
}
return this;
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public tensorflow.DevicePropertiesProtos.DeviceProperties.Builder getPropertiesBuilder() {
onChanged();
return getPropertiesFieldBuilder().getBuilder();
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
public tensorflow.DevicePropertiesProtos.DevicePropertiesOrBuilder getPropertiesOrBuilder() {
if (propertiesBuilder_ != null) {
return propertiesBuilder_.getMessageOrBuilder();
} else {
return properties_ == null ?
tensorflow.DevicePropertiesProtos.DeviceProperties.getDefaultInstance() : properties_;
}
}
/**
* .tensorflow.DeviceProperties properties = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.DevicePropertiesProtos.DeviceProperties, tensorflow.DevicePropertiesProtos.DeviceProperties.Builder, tensorflow.DevicePropertiesProtos.DevicePropertiesOrBuilder>
getPropertiesFieldBuilder() {
if (propertiesBuilder_ == null) {
propertiesBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.DevicePropertiesProtos.DeviceProperties, tensorflow.DevicePropertiesProtos.DeviceProperties.Builder, tensorflow.DevicePropertiesProtos.DevicePropertiesOrBuilder>(
getProperties(),
getParentForChildren(),
isClean());
properties_ = null;
}
return propertiesBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.NamedDevice)
}
// @@protoc_insertion_point(class_scope:tensorflow.NamedDevice)
private static final tensorflow.DevicePropertiesProtos.NamedDevice DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.DevicePropertiesProtos.NamedDevice();
}
public static tensorflow.DevicePropertiesProtos.NamedDevice getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public NamedDevice parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new NamedDevice(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.DevicePropertiesProtos.NamedDevice getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_DeviceProperties_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_DeviceProperties_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_DeviceProperties_EnvironmentEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_DeviceProperties_EnvironmentEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_NamedDevice_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_NamedDevice_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n0tensorflow/core/protobuf/device_proper" +
"ties.proto\022\ntensorflow\"\220\003\n\020DevicePropert" +
"ies\022\014\n\004type\030\001 \001(\t\022\016\n\006vendor\030\002 \001(\t\022\r\n\005mod" +
"el\030\003 \001(\t\022\021\n\tfrequency\030\004 \001(\003\022\021\n\tnum_cores" +
"\030\005 \001(\003\022B\n\013environment\030\006 \003(\0132-.tensorflow" +
".DeviceProperties.EnvironmentEntry\022\025\n\rnu" +
"m_registers\030\007 \001(\003\022\025\n\rl1_cache_size\030\010 \001(\003" +
"\022\025\n\rl2_cache_size\030\t \001(\003\022\025\n\rl3_cache_size" +
"\030\n \001(\003\022-\n%shared_memory_size_per_multipr" +
"ocessor\030\013 \001(\003\022\023\n\013memory_size\030\014 \001(\003\022\021\n\tba" +
"ndwidth\030\r \001(\003\0322\n\020EnvironmentEntry\022\013\n\003key" +
"\030\001 \001(\t\022\r\n\005value\030\002 \001(\t:\0028\001\"M\n\013NamedDevice" +
"\022\014\n\004name\030\001 \001(\t\0220\n\nproperties\030\002 \001(\0132\034.ten" +
"sorflow.DevicePropertiesBYB\026DeviceProper" +
"tiesProtosZ
© 2015 - 2025 Weber Informatics LLC | Privacy Policy