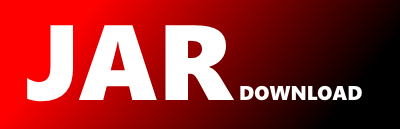
tensorflow.SavedObjectGraphOuterClass Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/saved_object_graph.proto
package tensorflow;
public final class SavedObjectGraphOuterClass {
private SavedObjectGraphOuterClass() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface SavedObjectGraphOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedObjectGraph)
com.google.protobuf.MessageOrBuilder {
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
java.util.List
getNodesList();
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
tensorflow.SavedObjectGraphOuterClass.SavedObject getNodes(int index);
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
int getNodesCount();
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
java.util.List extends tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder>
getNodesOrBuilderList();
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder getNodesOrBuilder(
int index);
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
int getConcreteFunctionsCount();
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
boolean containsConcreteFunctions(
java.lang.String key);
/**
* Use {@link #getConcreteFunctionsMap()} instead.
*/
@java.lang.Deprecated
java.util.Map
getConcreteFunctions();
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
java.util.Map
getConcreteFunctionsMap();
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getConcreteFunctionsOrDefault(
java.lang.String key,
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction defaultValue);
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getConcreteFunctionsOrThrow(
java.lang.String key);
}
/**
* Protobuf type {@code tensorflow.SavedObjectGraph}
*/
public static final class SavedObjectGraph extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedObjectGraph)
SavedObjectGraphOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedObjectGraph.newBuilder() to construct.
private SavedObjectGraph(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedObjectGraph() {
nodes_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedObjectGraph(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
nodes_.add(
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedObject.parser(), extensionRegistry));
break;
}
case 18: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
concreteFunctions_ = com.google.protobuf.MapField.newMapField(
ConcreteFunctionsDefaultEntryHolder.defaultEntry);
mutable_bitField0_ |= 0x00000002;
}
com.google.protobuf.MapEntry
concreteFunctions__ = input.readMessage(
ConcreteFunctionsDefaultEntryHolder.defaultEntry.getParserForType(), extensionRegistry);
concreteFunctions_.getMutableMap().put(
concreteFunctions__.getKey(), concreteFunctions__.getValue());
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = java.util.Collections.unmodifiableList(nodes_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObjectGraph_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetConcreteFunctions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObjectGraph_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph.class, tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph.Builder.class);
}
public static final int NODES_FIELD_NUMBER = 1;
private java.util.List nodes_;
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public java.util.List getNodesList() {
return nodes_;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public java.util.List extends tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder>
getNodesOrBuilderList() {
return nodes_;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public int getNodesCount() {
return nodes_.size();
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedObject getNodes(int index) {
return nodes_.get(index);
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder getNodesOrBuilder(
int index) {
return nodes_.get(index);
}
public static final int CONCRETE_FUNCTIONS_FIELD_NUMBER = 2;
private static final class ConcreteFunctionsDefaultEntryHolder {
static final com.google.protobuf.MapEntry<
java.lang.String, tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction> defaultEntry =
com.google.protobuf.MapEntry
.newDefaultInstance(
tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObjectGraph_ConcreteFunctionsEntry_descriptor,
com.google.protobuf.WireFormat.FieldType.STRING,
"",
com.google.protobuf.WireFormat.FieldType.MESSAGE,
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.getDefaultInstance());
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction> concreteFunctions_;
private com.google.protobuf.MapField
internalGetConcreteFunctions() {
if (concreteFunctions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ConcreteFunctionsDefaultEntryHolder.defaultEntry);
}
return concreteFunctions_;
}
public int getConcreteFunctionsCount() {
return internalGetConcreteFunctions().getMap().size();
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public boolean containsConcreteFunctions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetConcreteFunctions().getMap().containsKey(key);
}
/**
* Use {@link #getConcreteFunctionsMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getConcreteFunctions() {
return getConcreteFunctionsMap();
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public java.util.Map getConcreteFunctionsMap() {
return internalGetConcreteFunctions().getMap();
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getConcreteFunctionsOrDefault(
java.lang.String key,
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetConcreteFunctions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getConcreteFunctionsOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetConcreteFunctions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < nodes_.size(); i++) {
output.writeMessage(1, nodes_.get(i));
}
com.google.protobuf.GeneratedMessageV3
.serializeStringMapTo(
output,
internalGetConcreteFunctions(),
ConcreteFunctionsDefaultEntryHolder.defaultEntry,
2);
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < nodes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, nodes_.get(i));
}
for (java.util.Map.Entry entry
: internalGetConcreteFunctions().getMap().entrySet()) {
com.google.protobuf.MapEntry
concreteFunctions__ = ConcreteFunctionsDefaultEntryHolder.defaultEntry.newBuilderForType()
.setKey(entry.getKey())
.setValue(entry.getValue())
.build();
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, concreteFunctions__);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph other = (tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph) obj;
boolean result = true;
result = result && getNodesList()
.equals(other.getNodesList());
result = result && internalGetConcreteFunctions().equals(
other.internalGetConcreteFunctions());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getNodesCount() > 0) {
hash = (37 * hash) + NODES_FIELD_NUMBER;
hash = (53 * hash) + getNodesList().hashCode();
}
if (!internalGetConcreteFunctions().getMap().isEmpty()) {
hash = (37 * hash) + CONCRETE_FUNCTIONS_FIELD_NUMBER;
hash = (53 * hash) + internalGetConcreteFunctions().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.SavedObjectGraph}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedObjectGraph)
tensorflow.SavedObjectGraphOuterClass.SavedObjectGraphOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObjectGraph_descriptor;
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMapField(
int number) {
switch (number) {
case 2:
return internalGetConcreteFunctions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
@SuppressWarnings({"rawtypes"})
protected com.google.protobuf.MapField internalGetMutableMapField(
int number) {
switch (number) {
case 2:
return internalGetMutableConcreteFunctions();
default:
throw new RuntimeException(
"Invalid map field number: " + number);
}
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObjectGraph_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph.class, tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getNodesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (nodesBuilder_ == null) {
nodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
nodesBuilder_.clear();
}
internalGetMutableConcreteFunctions().clear();
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObjectGraph_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph build() {
tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph result = new tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph(this);
int from_bitField0_ = bitField0_;
if (nodesBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = java.util.Collections.unmodifiableList(nodes_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.nodes_ = nodes_;
} else {
result.nodes_ = nodesBuilder_.build();
}
result.concreteFunctions_ = internalGetConcreteFunctions();
result.concreteFunctions_.makeImmutable();
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph.getDefaultInstance()) return this;
if (nodesBuilder_ == null) {
if (!other.nodes_.isEmpty()) {
if (nodes_.isEmpty()) {
nodes_ = other.nodes_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureNodesIsMutable();
nodes_.addAll(other.nodes_);
}
onChanged();
}
} else {
if (!other.nodes_.isEmpty()) {
if (nodesBuilder_.isEmpty()) {
nodesBuilder_.dispose();
nodesBuilder_ = null;
nodes_ = other.nodes_;
bitField0_ = (bitField0_ & ~0x00000001);
nodesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getNodesFieldBuilder() : null;
} else {
nodesBuilder_.addAllMessages(other.nodes_);
}
}
}
internalGetMutableConcreteFunctions().mergeFrom(
other.internalGetConcreteFunctions());
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List nodes_ =
java.util.Collections.emptyList();
private void ensureNodesIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
nodes_ = new java.util.ArrayList(nodes_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedObject, tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder, tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder> nodesBuilder_;
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public java.util.List getNodesList() {
if (nodesBuilder_ == null) {
return java.util.Collections.unmodifiableList(nodes_);
} else {
return nodesBuilder_.getMessageList();
}
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public int getNodesCount() {
if (nodesBuilder_ == null) {
return nodes_.size();
} else {
return nodesBuilder_.getCount();
}
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedObject getNodes(int index) {
if (nodesBuilder_ == null) {
return nodes_.get(index);
} else {
return nodesBuilder_.getMessage(index);
}
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder setNodes(
int index, tensorflow.SavedObjectGraphOuterClass.SavedObject value) {
if (nodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.set(index, value);
onChanged();
} else {
nodesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder setNodes(
int index, tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder builderForValue) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.set(index, builderForValue.build());
onChanged();
} else {
nodesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder addNodes(tensorflow.SavedObjectGraphOuterClass.SavedObject value) {
if (nodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.add(value);
onChanged();
} else {
nodesBuilder_.addMessage(value);
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder addNodes(
int index, tensorflow.SavedObjectGraphOuterClass.SavedObject value) {
if (nodesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureNodesIsMutable();
nodes_.add(index, value);
onChanged();
} else {
nodesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder addNodes(
tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder builderForValue) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.add(builderForValue.build());
onChanged();
} else {
nodesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder addNodes(
int index, tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder builderForValue) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.add(index, builderForValue.build());
onChanged();
} else {
nodesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder addAllNodes(
java.lang.Iterable extends tensorflow.SavedObjectGraphOuterClass.SavedObject> values) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, nodes_);
onChanged();
} else {
nodesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder clearNodes() {
if (nodesBuilder_ == null) {
nodes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
nodesBuilder_.clear();
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public Builder removeNodes(int index) {
if (nodesBuilder_ == null) {
ensureNodesIsMutable();
nodes_.remove(index);
onChanged();
} else {
nodesBuilder_.remove(index);
}
return this;
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder getNodesBuilder(
int index) {
return getNodesFieldBuilder().getBuilder(index);
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder getNodesOrBuilder(
int index) {
if (nodesBuilder_ == null) {
return nodes_.get(index); } else {
return nodesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public java.util.List extends tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder>
getNodesOrBuilderList() {
if (nodesBuilder_ != null) {
return nodesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(nodes_);
}
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder addNodesBuilder() {
return getNodesFieldBuilder().addBuilder(
tensorflow.SavedObjectGraphOuterClass.SavedObject.getDefaultInstance());
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder addNodesBuilder(
int index) {
return getNodesFieldBuilder().addBuilder(
index, tensorflow.SavedObjectGraphOuterClass.SavedObject.getDefaultInstance());
}
/**
*
* Flattened list of objects in the object graph.
* The position of the object in this list indicates its id.
* Nodes[0] is considered the root node.
*
*
* repeated .tensorflow.SavedObject nodes = 1;
*/
public java.util.List
getNodesBuilderList() {
return getNodesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedObject, tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder, tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder>
getNodesFieldBuilder() {
if (nodesBuilder_ == null) {
nodesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedObject, tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder, tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder>(
nodes_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
nodes_ = null;
}
return nodesBuilder_;
}
private com.google.protobuf.MapField<
java.lang.String, tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction> concreteFunctions_;
private com.google.protobuf.MapField
internalGetConcreteFunctions() {
if (concreteFunctions_ == null) {
return com.google.protobuf.MapField.emptyMapField(
ConcreteFunctionsDefaultEntryHolder.defaultEntry);
}
return concreteFunctions_;
}
private com.google.protobuf.MapField
internalGetMutableConcreteFunctions() {
onChanged();;
if (concreteFunctions_ == null) {
concreteFunctions_ = com.google.protobuf.MapField.newMapField(
ConcreteFunctionsDefaultEntryHolder.defaultEntry);
}
if (!concreteFunctions_.isMutable()) {
concreteFunctions_ = concreteFunctions_.copy();
}
return concreteFunctions_;
}
public int getConcreteFunctionsCount() {
return internalGetConcreteFunctions().getMap().size();
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public boolean containsConcreteFunctions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
return internalGetConcreteFunctions().getMap().containsKey(key);
}
/**
* Use {@link #getConcreteFunctionsMap()} instead.
*/
@java.lang.Deprecated
public java.util.Map getConcreteFunctions() {
return getConcreteFunctionsMap();
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public java.util.Map getConcreteFunctionsMap() {
return internalGetConcreteFunctions().getMap();
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getConcreteFunctionsOrDefault(
java.lang.String key,
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction defaultValue) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetConcreteFunctions().getMap();
return map.containsKey(key) ? map.get(key) : defaultValue;
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getConcreteFunctionsOrThrow(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
java.util.Map map =
internalGetConcreteFunctions().getMap();
if (!map.containsKey(key)) {
throw new java.lang.IllegalArgumentException();
}
return map.get(key);
}
public Builder clearConcreteFunctions() {
internalGetMutableConcreteFunctions().getMutableMap()
.clear();
return this;
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public Builder removeConcreteFunctions(
java.lang.String key) {
if (key == null) { throw new java.lang.NullPointerException(); }
internalGetMutableConcreteFunctions().getMutableMap()
.remove(key);
return this;
}
/**
* Use alternate mutation accessors instead.
*/
@java.lang.Deprecated
public java.util.Map
getMutableConcreteFunctions() {
return internalGetMutableConcreteFunctions().getMutableMap();
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public Builder putConcreteFunctions(
java.lang.String key,
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction value) {
if (key == null) { throw new java.lang.NullPointerException(); }
if (value == null) { throw new java.lang.NullPointerException(); }
internalGetMutableConcreteFunctions().getMutableMap()
.put(key, value);
return this;
}
/**
*
* Information about captures and output structures in concrete functions.
* Referenced from SavedBareConcreteFunction and SavedFunction.
*
*
* map<string, .tensorflow.SavedConcreteFunction> concrete_functions = 2;
*/
public Builder putAllConcreteFunctions(
java.util.Map values) {
internalGetMutableConcreteFunctions().getMutableMap()
.putAll(values);
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedObjectGraph)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedObjectGraph)
private static final tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedObjectGraph parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedObjectGraph(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedObjectGraph getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedObjectOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedObject)
com.google.protobuf.MessageOrBuilder {
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
java.util.List
getChildrenList();
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference getChildren(int index);
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
int getChildrenCount();
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
java.util.List extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder>
getChildrenOrBuilderList();
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder getChildrenOrBuilder(
int index);
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
java.util.List
getSlotVariablesList();
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference getSlotVariables(int index);
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
int getSlotVariablesCount();
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
java.util.List extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder>
getSlotVariablesOrBuilderList();
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder getSlotVariablesOrBuilder(
int index);
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
boolean hasUserObject();
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
tensorflow.SavedObjectGraphOuterClass.SavedUserObject getUserObject();
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
tensorflow.SavedObjectGraphOuterClass.SavedUserObjectOrBuilder getUserObjectOrBuilder();
/**
* .tensorflow.SavedAsset asset = 5;
*/
boolean hasAsset();
/**
* .tensorflow.SavedAsset asset = 5;
*/
tensorflow.SavedObjectGraphOuterClass.SavedAsset getAsset();
/**
* .tensorflow.SavedAsset asset = 5;
*/
tensorflow.SavedObjectGraphOuterClass.SavedAssetOrBuilder getAssetOrBuilder();
/**
* .tensorflow.SavedFunction function = 6;
*/
boolean hasFunction();
/**
* .tensorflow.SavedFunction function = 6;
*/
tensorflow.SavedObjectGraphOuterClass.SavedFunction getFunction();
/**
* .tensorflow.SavedFunction function = 6;
*/
tensorflow.SavedObjectGraphOuterClass.SavedFunctionOrBuilder getFunctionOrBuilder();
/**
* .tensorflow.SavedVariable variable = 7;
*/
boolean hasVariable();
/**
* .tensorflow.SavedVariable variable = 7;
*/
tensorflow.SavedObjectGraphOuterClass.SavedVariable getVariable();
/**
* .tensorflow.SavedVariable variable = 7;
*/
tensorflow.SavedObjectGraphOuterClass.SavedVariableOrBuilder getVariableOrBuilder();
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
boolean hasBareConcreteFunction();
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction getBareConcreteFunction();
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunctionOrBuilder getBareConcreteFunctionOrBuilder();
/**
* .tensorflow.SavedConstant constant = 9;
*/
boolean hasConstant();
/**
* .tensorflow.SavedConstant constant = 9;
*/
tensorflow.SavedObjectGraphOuterClass.SavedConstant getConstant();
/**
* .tensorflow.SavedConstant constant = 9;
*/
tensorflow.SavedObjectGraphOuterClass.SavedConstantOrBuilder getConstantOrBuilder();
/**
* .tensorflow.SavedResource resource = 10;
*/
boolean hasResource();
/**
* .tensorflow.SavedResource resource = 10;
*/
tensorflow.SavedObjectGraphOuterClass.SavedResource getResource();
/**
* .tensorflow.SavedResource resource = 10;
*/
tensorflow.SavedObjectGraphOuterClass.SavedResourceOrBuilder getResourceOrBuilder();
public tensorflow.SavedObjectGraphOuterClass.SavedObject.KindCase getKindCase();
}
/**
* Protobuf type {@code tensorflow.SavedObject}
*/
public static final class SavedObject extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedObject)
SavedObjectOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedObject.newBuilder() to construct.
private SavedObject(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedObject() {
children_ = java.util.Collections.emptyList();
slotVariables_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedObject(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
children_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
children_.add(
input.readMessage(tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.parser(), extensionRegistry));
break;
}
case 26: {
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
slotVariables_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000002;
}
slotVariables_.add(
input.readMessage(tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.parser(), extensionRegistry));
break;
}
case 34: {
tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder subBuilder = null;
if (kindCase_ == 4) {
subBuilder = ((tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_).toBuilder();
}
kind_ =
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedUserObject.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_);
kind_ = subBuilder.buildPartial();
}
kindCase_ = 4;
break;
}
case 42: {
tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder subBuilder = null;
if (kindCase_ == 5) {
subBuilder = ((tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_).toBuilder();
}
kind_ =
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedAsset.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_);
kind_ = subBuilder.buildPartial();
}
kindCase_ = 5;
break;
}
case 50: {
tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder subBuilder = null;
if (kindCase_ == 6) {
subBuilder = ((tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_).toBuilder();
}
kind_ =
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedFunction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_);
kind_ = subBuilder.buildPartial();
}
kindCase_ = 6;
break;
}
case 58: {
tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder subBuilder = null;
if (kindCase_ == 7) {
subBuilder = ((tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_).toBuilder();
}
kind_ =
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedVariable.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_);
kind_ = subBuilder.buildPartial();
}
kindCase_ = 7;
break;
}
case 66: {
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder subBuilder = null;
if (kindCase_ == 8) {
subBuilder = ((tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_).toBuilder();
}
kind_ =
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_);
kind_ = subBuilder.buildPartial();
}
kindCase_ = 8;
break;
}
case 74: {
tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder subBuilder = null;
if (kindCase_ == 9) {
subBuilder = ((tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_).toBuilder();
}
kind_ =
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedConstant.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_);
kind_ = subBuilder.buildPartial();
}
kindCase_ = 9;
break;
}
case 82: {
tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder subBuilder = null;
if (kindCase_ == 10) {
subBuilder = ((tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_).toBuilder();
}
kind_ =
input.readMessage(tensorflow.SavedObjectGraphOuterClass.SavedResource.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_);
kind_ = subBuilder.buildPartial();
}
kindCase_ = 10;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
children_ = java.util.Collections.unmodifiableList(children_);
}
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
slotVariables_ = java.util.Collections.unmodifiableList(slotVariables_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObject_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedObject.class, tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder.class);
}
private int bitField0_;
private int kindCase_ = 0;
private java.lang.Object kind_;
public enum KindCase
implements com.google.protobuf.Internal.EnumLite {
USER_OBJECT(4),
ASSET(5),
FUNCTION(6),
VARIABLE(7),
BARE_CONCRETE_FUNCTION(8),
CONSTANT(9),
RESOURCE(10),
KIND_NOT_SET(0);
private final int value;
private KindCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static KindCase valueOf(int value) {
return forNumber(value);
}
public static KindCase forNumber(int value) {
switch (value) {
case 4: return USER_OBJECT;
case 5: return ASSET;
case 6: return FUNCTION;
case 7: return VARIABLE;
case 8: return BARE_CONCRETE_FUNCTION;
case 9: return CONSTANT;
case 10: return RESOURCE;
case 0: return KIND_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public KindCase
getKindCase() {
return KindCase.forNumber(
kindCase_);
}
public static final int CHILDREN_FIELD_NUMBER = 1;
private java.util.List children_;
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public java.util.List getChildrenList() {
return children_;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public java.util.List extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder>
getChildrenOrBuilderList() {
return children_;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public int getChildrenCount() {
return children_.size();
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference getChildren(int index) {
return children_.get(index);
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder getChildrenOrBuilder(
int index) {
return children_.get(index);
}
public static final int SLOT_VARIABLES_FIELD_NUMBER = 3;
private java.util.List slotVariables_;
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public java.util.List getSlotVariablesList() {
return slotVariables_;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public java.util.List extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder>
getSlotVariablesOrBuilderList() {
return slotVariables_;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public int getSlotVariablesCount() {
return slotVariables_.size();
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference getSlotVariables(int index) {
return slotVariables_.get(index);
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder getSlotVariablesOrBuilder(
int index) {
return slotVariables_.get(index);
}
public static final int USER_OBJECT_FIELD_NUMBER = 4;
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public boolean hasUserObject() {
return kindCase_ == 4;
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedUserObject getUserObject() {
if (kindCase_ == 4) {
return (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance();
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedUserObjectOrBuilder getUserObjectOrBuilder() {
if (kindCase_ == 4) {
return (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance();
}
public static final int ASSET_FIELD_NUMBER = 5;
/**
* .tensorflow.SavedAsset asset = 5;
*/
public boolean hasAsset() {
return kindCase_ == 5;
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedAsset getAsset() {
if (kindCase_ == 5) {
return (tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance();
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedAssetOrBuilder getAssetOrBuilder() {
if (kindCase_ == 5) {
return (tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance();
}
public static final int FUNCTION_FIELD_NUMBER = 6;
/**
* .tensorflow.SavedFunction function = 6;
*/
public boolean hasFunction() {
return kindCase_ == 6;
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedFunction getFunction() {
if (kindCase_ == 6) {
return (tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance();
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedFunctionOrBuilder getFunctionOrBuilder() {
if (kindCase_ == 6) {
return (tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance();
}
public static final int VARIABLE_FIELD_NUMBER = 7;
/**
* .tensorflow.SavedVariable variable = 7;
*/
public boolean hasVariable() {
return kindCase_ == 7;
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedVariable getVariable() {
if (kindCase_ == 7) {
return (tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance();
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedVariableOrBuilder getVariableOrBuilder() {
if (kindCase_ == 7) {
return (tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance();
}
public static final int BARE_CONCRETE_FUNCTION_FIELD_NUMBER = 8;
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public boolean hasBareConcreteFunction() {
return kindCase_ == 8;
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction getBareConcreteFunction() {
if (kindCase_ == 8) {
return (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance();
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunctionOrBuilder getBareConcreteFunctionOrBuilder() {
if (kindCase_ == 8) {
return (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance();
}
public static final int CONSTANT_FIELD_NUMBER = 9;
/**
* .tensorflow.SavedConstant constant = 9;
*/
public boolean hasConstant() {
return kindCase_ == 9;
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConstant getConstant() {
if (kindCase_ == 9) {
return (tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance();
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConstantOrBuilder getConstantOrBuilder() {
if (kindCase_ == 9) {
return (tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance();
}
public static final int RESOURCE_FIELD_NUMBER = 10;
/**
* .tensorflow.SavedResource resource = 10;
*/
public boolean hasResource() {
return kindCase_ == 10;
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedResource getResource() {
if (kindCase_ == 10) {
return (tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance();
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedResourceOrBuilder getResourceOrBuilder() {
if (kindCase_ == 10) {
return (tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < children_.size(); i++) {
output.writeMessage(1, children_.get(i));
}
for (int i = 0; i < slotVariables_.size(); i++) {
output.writeMessage(3, slotVariables_.get(i));
}
if (kindCase_ == 4) {
output.writeMessage(4, (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_);
}
if (kindCase_ == 5) {
output.writeMessage(5, (tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_);
}
if (kindCase_ == 6) {
output.writeMessage(6, (tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_);
}
if (kindCase_ == 7) {
output.writeMessage(7, (tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_);
}
if (kindCase_ == 8) {
output.writeMessage(8, (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_);
}
if (kindCase_ == 9) {
output.writeMessage(9, (tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_);
}
if (kindCase_ == 10) {
output.writeMessage(10, (tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < children_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, children_.get(i));
}
for (int i = 0; i < slotVariables_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, slotVariables_.get(i));
}
if (kindCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_);
}
if (kindCase_ == 5) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, (tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_);
}
if (kindCase_ == 6) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(6, (tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_);
}
if (kindCase_ == 7) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(7, (tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_);
}
if (kindCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_);
}
if (kindCase_ == 9) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(9, (tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_);
}
if (kindCase_ == 10) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(10, (tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedObject)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedObject other = (tensorflow.SavedObjectGraphOuterClass.SavedObject) obj;
boolean result = true;
result = result && getChildrenList()
.equals(other.getChildrenList());
result = result && getSlotVariablesList()
.equals(other.getSlotVariablesList());
result = result && getKindCase().equals(
other.getKindCase());
if (!result) return false;
switch (kindCase_) {
case 4:
result = result && getUserObject()
.equals(other.getUserObject());
break;
case 5:
result = result && getAsset()
.equals(other.getAsset());
break;
case 6:
result = result && getFunction()
.equals(other.getFunction());
break;
case 7:
result = result && getVariable()
.equals(other.getVariable());
break;
case 8:
result = result && getBareConcreteFunction()
.equals(other.getBareConcreteFunction());
break;
case 9:
result = result && getConstant()
.equals(other.getConstant());
break;
case 10:
result = result && getResource()
.equals(other.getResource());
break;
case 0:
default:
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getChildrenCount() > 0) {
hash = (37 * hash) + CHILDREN_FIELD_NUMBER;
hash = (53 * hash) + getChildrenList().hashCode();
}
if (getSlotVariablesCount() > 0) {
hash = (37 * hash) + SLOT_VARIABLES_FIELD_NUMBER;
hash = (53 * hash) + getSlotVariablesList().hashCode();
}
switch (kindCase_) {
case 4:
hash = (37 * hash) + USER_OBJECT_FIELD_NUMBER;
hash = (53 * hash) + getUserObject().hashCode();
break;
case 5:
hash = (37 * hash) + ASSET_FIELD_NUMBER;
hash = (53 * hash) + getAsset().hashCode();
break;
case 6:
hash = (37 * hash) + FUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getFunction().hashCode();
break;
case 7:
hash = (37 * hash) + VARIABLE_FIELD_NUMBER;
hash = (53 * hash) + getVariable().hashCode();
break;
case 8:
hash = (37 * hash) + BARE_CONCRETE_FUNCTION_FIELD_NUMBER;
hash = (53 * hash) + getBareConcreteFunction().hashCode();
break;
case 9:
hash = (37 * hash) + CONSTANT_FIELD_NUMBER;
hash = (53 * hash) + getConstant().hashCode();
break;
case 10:
hash = (37 * hash) + RESOURCE_FIELD_NUMBER;
hash = (53 * hash) + getResource().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedObject prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.SavedObject}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedObject)
tensorflow.SavedObjectGraphOuterClass.SavedObjectOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObject_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedObject.class, tensorflow.SavedObjectGraphOuterClass.SavedObject.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedObject.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getChildrenFieldBuilder();
getSlotVariablesFieldBuilder();
}
}
public Builder clear() {
super.clear();
if (childrenBuilder_ == null) {
children_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
childrenBuilder_.clear();
}
if (slotVariablesBuilder_ == null) {
slotVariables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
} else {
slotVariablesBuilder_.clear();
}
kindCase_ = 0;
kind_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedObject_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedObject getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedObject.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedObject build() {
tensorflow.SavedObjectGraphOuterClass.SavedObject result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedObject buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedObject result = new tensorflow.SavedObjectGraphOuterClass.SavedObject(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (childrenBuilder_ == null) {
if (((bitField0_ & 0x00000001) == 0x00000001)) {
children_ = java.util.Collections.unmodifiableList(children_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.children_ = children_;
} else {
result.children_ = childrenBuilder_.build();
}
if (slotVariablesBuilder_ == null) {
if (((bitField0_ & 0x00000002) == 0x00000002)) {
slotVariables_ = java.util.Collections.unmodifiableList(slotVariables_);
bitField0_ = (bitField0_ & ~0x00000002);
}
result.slotVariables_ = slotVariables_;
} else {
result.slotVariables_ = slotVariablesBuilder_.build();
}
if (kindCase_ == 4) {
if (userObjectBuilder_ == null) {
result.kind_ = kind_;
} else {
result.kind_ = userObjectBuilder_.build();
}
}
if (kindCase_ == 5) {
if (assetBuilder_ == null) {
result.kind_ = kind_;
} else {
result.kind_ = assetBuilder_.build();
}
}
if (kindCase_ == 6) {
if (functionBuilder_ == null) {
result.kind_ = kind_;
} else {
result.kind_ = functionBuilder_.build();
}
}
if (kindCase_ == 7) {
if (variableBuilder_ == null) {
result.kind_ = kind_;
} else {
result.kind_ = variableBuilder_.build();
}
}
if (kindCase_ == 8) {
if (bareConcreteFunctionBuilder_ == null) {
result.kind_ = kind_;
} else {
result.kind_ = bareConcreteFunctionBuilder_.build();
}
}
if (kindCase_ == 9) {
if (constantBuilder_ == null) {
result.kind_ = kind_;
} else {
result.kind_ = constantBuilder_.build();
}
}
if (kindCase_ == 10) {
if (resourceBuilder_ == null) {
result.kind_ = kind_;
} else {
result.kind_ = resourceBuilder_.build();
}
}
result.bitField0_ = to_bitField0_;
result.kindCase_ = kindCase_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedObject) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedObject)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedObject other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedObject.getDefaultInstance()) return this;
if (childrenBuilder_ == null) {
if (!other.children_.isEmpty()) {
if (children_.isEmpty()) {
children_ = other.children_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureChildrenIsMutable();
children_.addAll(other.children_);
}
onChanged();
}
} else {
if (!other.children_.isEmpty()) {
if (childrenBuilder_.isEmpty()) {
childrenBuilder_.dispose();
childrenBuilder_ = null;
children_ = other.children_;
bitField0_ = (bitField0_ & ~0x00000001);
childrenBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getChildrenFieldBuilder() : null;
} else {
childrenBuilder_.addAllMessages(other.children_);
}
}
}
if (slotVariablesBuilder_ == null) {
if (!other.slotVariables_.isEmpty()) {
if (slotVariables_.isEmpty()) {
slotVariables_ = other.slotVariables_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureSlotVariablesIsMutable();
slotVariables_.addAll(other.slotVariables_);
}
onChanged();
}
} else {
if (!other.slotVariables_.isEmpty()) {
if (slotVariablesBuilder_.isEmpty()) {
slotVariablesBuilder_.dispose();
slotVariablesBuilder_ = null;
slotVariables_ = other.slotVariables_;
bitField0_ = (bitField0_ & ~0x00000002);
slotVariablesBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getSlotVariablesFieldBuilder() : null;
} else {
slotVariablesBuilder_.addAllMessages(other.slotVariables_);
}
}
}
switch (other.getKindCase()) {
case USER_OBJECT: {
mergeUserObject(other.getUserObject());
break;
}
case ASSET: {
mergeAsset(other.getAsset());
break;
}
case FUNCTION: {
mergeFunction(other.getFunction());
break;
}
case VARIABLE: {
mergeVariable(other.getVariable());
break;
}
case BARE_CONCRETE_FUNCTION: {
mergeBareConcreteFunction(other.getBareConcreteFunction());
break;
}
case CONSTANT: {
mergeConstant(other.getConstant());
break;
}
case RESOURCE: {
mergeResource(other.getResource());
break;
}
case KIND_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedObject parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedObject) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int kindCase_ = 0;
private java.lang.Object kind_;
public KindCase
getKindCase() {
return KindCase.forNumber(
kindCase_);
}
public Builder clearKind() {
kindCase_ = 0;
kind_ = null;
onChanged();
return this;
}
private int bitField0_;
private java.util.List children_ =
java.util.Collections.emptyList();
private void ensureChildrenIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
children_ = new java.util.ArrayList(children_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder> childrenBuilder_;
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public java.util.List getChildrenList() {
if (childrenBuilder_ == null) {
return java.util.Collections.unmodifiableList(children_);
} else {
return childrenBuilder_.getMessageList();
}
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public int getChildrenCount() {
if (childrenBuilder_ == null) {
return children_.size();
} else {
return childrenBuilder_.getCount();
}
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference getChildren(int index) {
if (childrenBuilder_ == null) {
return children_.get(index);
} else {
return childrenBuilder_.getMessage(index);
}
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder setChildren(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference value) {
if (childrenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChildrenIsMutable();
children_.set(index, value);
onChanged();
} else {
childrenBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder setChildren(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder builderForValue) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.set(index, builderForValue.build());
onChanged();
} else {
childrenBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder addChildren(tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference value) {
if (childrenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChildrenIsMutable();
children_.add(value);
onChanged();
} else {
childrenBuilder_.addMessage(value);
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder addChildren(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference value) {
if (childrenBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureChildrenIsMutable();
children_.add(index, value);
onChanged();
} else {
childrenBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder addChildren(
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder builderForValue) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.add(builderForValue.build());
onChanged();
} else {
childrenBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder addChildren(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder builderForValue) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.add(index, builderForValue.build());
onChanged();
} else {
childrenBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder addAllChildren(
java.lang.Iterable extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference> values) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, children_);
onChanged();
} else {
childrenBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder clearChildren() {
if (childrenBuilder_ == null) {
children_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
childrenBuilder_.clear();
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public Builder removeChildren(int index) {
if (childrenBuilder_ == null) {
ensureChildrenIsMutable();
children_.remove(index);
onChanged();
} else {
childrenBuilder_.remove(index);
}
return this;
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder getChildrenBuilder(
int index) {
return getChildrenFieldBuilder().getBuilder(index);
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder getChildrenOrBuilder(
int index) {
if (childrenBuilder_ == null) {
return children_.get(index); } else {
return childrenBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public java.util.List extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder>
getChildrenOrBuilderList() {
if (childrenBuilder_ != null) {
return childrenBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(children_);
}
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder addChildrenBuilder() {
return getChildrenFieldBuilder().addBuilder(
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.getDefaultInstance());
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder addChildrenBuilder(
int index) {
return getChildrenFieldBuilder().addBuilder(
index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.getDefaultInstance());
}
/**
*
* Objects which this object depends on: named edges in the dependency
* graph.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.ObjectReference children = 1;
*/
public java.util.List
getChildrenBuilderList() {
return getChildrenFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder>
getChildrenFieldBuilder() {
if (childrenBuilder_ == null) {
childrenBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReference.Builder, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.ObjectReferenceOrBuilder>(
children_,
((bitField0_ & 0x00000001) == 0x00000001),
getParentForChildren(),
isClean());
children_ = null;
}
return childrenBuilder_;
}
private java.util.List slotVariables_ =
java.util.Collections.emptyList();
private void ensureSlotVariablesIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
slotVariables_ = new java.util.ArrayList(slotVariables_);
bitField0_ |= 0x00000002;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder> slotVariablesBuilder_;
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public java.util.List getSlotVariablesList() {
if (slotVariablesBuilder_ == null) {
return java.util.Collections.unmodifiableList(slotVariables_);
} else {
return slotVariablesBuilder_.getMessageList();
}
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public int getSlotVariablesCount() {
if (slotVariablesBuilder_ == null) {
return slotVariables_.size();
} else {
return slotVariablesBuilder_.getCount();
}
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference getSlotVariables(int index) {
if (slotVariablesBuilder_ == null) {
return slotVariables_.get(index);
} else {
return slotVariablesBuilder_.getMessage(index);
}
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder setSlotVariables(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference value) {
if (slotVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSlotVariablesIsMutable();
slotVariables_.set(index, value);
onChanged();
} else {
slotVariablesBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder setSlotVariables(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder builderForValue) {
if (slotVariablesBuilder_ == null) {
ensureSlotVariablesIsMutable();
slotVariables_.set(index, builderForValue.build());
onChanged();
} else {
slotVariablesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder addSlotVariables(tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference value) {
if (slotVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSlotVariablesIsMutable();
slotVariables_.add(value);
onChanged();
} else {
slotVariablesBuilder_.addMessage(value);
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder addSlotVariables(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference value) {
if (slotVariablesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSlotVariablesIsMutable();
slotVariables_.add(index, value);
onChanged();
} else {
slotVariablesBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder addSlotVariables(
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder builderForValue) {
if (slotVariablesBuilder_ == null) {
ensureSlotVariablesIsMutable();
slotVariables_.add(builderForValue.build());
onChanged();
} else {
slotVariablesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder addSlotVariables(
int index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder builderForValue) {
if (slotVariablesBuilder_ == null) {
ensureSlotVariablesIsMutable();
slotVariables_.add(index, builderForValue.build());
onChanged();
} else {
slotVariablesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder addAllSlotVariables(
java.lang.Iterable extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference> values) {
if (slotVariablesBuilder_ == null) {
ensureSlotVariablesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, slotVariables_);
onChanged();
} else {
slotVariablesBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder clearSlotVariables() {
if (slotVariablesBuilder_ == null) {
slotVariables_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
} else {
slotVariablesBuilder_.clear();
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public Builder removeSlotVariables(int index) {
if (slotVariablesBuilder_ == null) {
ensureSlotVariablesIsMutable();
slotVariables_.remove(index);
onChanged();
} else {
slotVariablesBuilder_.remove(index);
}
return this;
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder getSlotVariablesBuilder(
int index) {
return getSlotVariablesFieldBuilder().getBuilder(index);
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder getSlotVariablesOrBuilder(
int index) {
if (slotVariablesBuilder_ == null) {
return slotVariables_.get(index); } else {
return slotVariablesBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public java.util.List extends tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder>
getSlotVariablesOrBuilderList() {
if (slotVariablesBuilder_ != null) {
return slotVariablesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(slotVariables_);
}
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder addSlotVariablesBuilder() {
return getSlotVariablesFieldBuilder().addBuilder(
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.getDefaultInstance());
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder addSlotVariablesBuilder(
int index) {
return getSlotVariablesFieldBuilder().addBuilder(
index, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.getDefaultInstance());
}
/**
*
* Slot variables owned by this object. This describes the three-way
* (optimizer, variable, slot variable) relationship; none of the three
* depend on the others directly.
* Note: currently only valid if kind == "user_object".
*
*
* repeated .tensorflow.TrackableObjectGraph.TrackableObject.SlotVariableReference slot_variables = 3;
*/
public java.util.List
getSlotVariablesBuilderList() {
return getSlotVariablesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder>
getSlotVariablesFieldBuilder() {
if (slotVariablesBuilder_ == null) {
slotVariablesBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReference.Builder, tensorflow.TrackableObjectGraphOuterClass.TrackableObjectGraph.TrackableObject.SlotVariableReferenceOrBuilder>(
slotVariables_,
((bitField0_ & 0x00000002) == 0x00000002),
getParentForChildren(),
isClean());
slotVariables_ = null;
}
return slotVariablesBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedUserObject, tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder, tensorflow.SavedObjectGraphOuterClass.SavedUserObjectOrBuilder> userObjectBuilder_;
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public boolean hasUserObject() {
return kindCase_ == 4;
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedUserObject getUserObject() {
if (userObjectBuilder_ == null) {
if (kindCase_ == 4) {
return (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance();
} else {
if (kindCase_ == 4) {
return userObjectBuilder_.getMessage();
}
return tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance();
}
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public Builder setUserObject(tensorflow.SavedObjectGraphOuterClass.SavedUserObject value) {
if (userObjectBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
onChanged();
} else {
userObjectBuilder_.setMessage(value);
}
kindCase_ = 4;
return this;
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public Builder setUserObject(
tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder builderForValue) {
if (userObjectBuilder_ == null) {
kind_ = builderForValue.build();
onChanged();
} else {
userObjectBuilder_.setMessage(builderForValue.build());
}
kindCase_ = 4;
return this;
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public Builder mergeUserObject(tensorflow.SavedObjectGraphOuterClass.SavedUserObject value) {
if (userObjectBuilder_ == null) {
if (kindCase_ == 4 &&
kind_ != tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance()) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedUserObject.newBuilder((tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_)
.mergeFrom(value).buildPartial();
} else {
kind_ = value;
}
onChanged();
} else {
if (kindCase_ == 4) {
userObjectBuilder_.mergeFrom(value);
}
userObjectBuilder_.setMessage(value);
}
kindCase_ = 4;
return this;
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public Builder clearUserObject() {
if (userObjectBuilder_ == null) {
if (kindCase_ == 4) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
} else {
if (kindCase_ == 4) {
kindCase_ = 0;
kind_ = null;
}
userObjectBuilder_.clear();
}
return this;
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder getUserObjectBuilder() {
return getUserObjectFieldBuilder().getBuilder();
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedUserObjectOrBuilder getUserObjectOrBuilder() {
if ((kindCase_ == 4) && (userObjectBuilder_ != null)) {
return userObjectBuilder_.getMessageOrBuilder();
} else {
if (kindCase_ == 4) {
return (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance();
}
}
/**
* .tensorflow.SavedUserObject user_object = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedUserObject, tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder, tensorflow.SavedObjectGraphOuterClass.SavedUserObjectOrBuilder>
getUserObjectFieldBuilder() {
if (userObjectBuilder_ == null) {
if (!(kindCase_ == 4)) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance();
}
userObjectBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedUserObject, tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder, tensorflow.SavedObjectGraphOuterClass.SavedUserObjectOrBuilder>(
(tensorflow.SavedObjectGraphOuterClass.SavedUserObject) kind_,
getParentForChildren(),
isClean());
kind_ = null;
}
kindCase_ = 4;
onChanged();;
return userObjectBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedAsset, tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder, tensorflow.SavedObjectGraphOuterClass.SavedAssetOrBuilder> assetBuilder_;
/**
* .tensorflow.SavedAsset asset = 5;
*/
public boolean hasAsset() {
return kindCase_ == 5;
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedAsset getAsset() {
if (assetBuilder_ == null) {
if (kindCase_ == 5) {
return (tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance();
} else {
if (kindCase_ == 5) {
return assetBuilder_.getMessage();
}
return tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance();
}
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public Builder setAsset(tensorflow.SavedObjectGraphOuterClass.SavedAsset value) {
if (assetBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
onChanged();
} else {
assetBuilder_.setMessage(value);
}
kindCase_ = 5;
return this;
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public Builder setAsset(
tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder builderForValue) {
if (assetBuilder_ == null) {
kind_ = builderForValue.build();
onChanged();
} else {
assetBuilder_.setMessage(builderForValue.build());
}
kindCase_ = 5;
return this;
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public Builder mergeAsset(tensorflow.SavedObjectGraphOuterClass.SavedAsset value) {
if (assetBuilder_ == null) {
if (kindCase_ == 5 &&
kind_ != tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance()) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedAsset.newBuilder((tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_)
.mergeFrom(value).buildPartial();
} else {
kind_ = value;
}
onChanged();
} else {
if (kindCase_ == 5) {
assetBuilder_.mergeFrom(value);
}
assetBuilder_.setMessage(value);
}
kindCase_ = 5;
return this;
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public Builder clearAsset() {
if (assetBuilder_ == null) {
if (kindCase_ == 5) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
} else {
if (kindCase_ == 5) {
kindCase_ = 0;
kind_ = null;
}
assetBuilder_.clear();
}
return this;
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder getAssetBuilder() {
return getAssetFieldBuilder().getBuilder();
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedAssetOrBuilder getAssetOrBuilder() {
if ((kindCase_ == 5) && (assetBuilder_ != null)) {
return assetBuilder_.getMessageOrBuilder();
} else {
if (kindCase_ == 5) {
return (tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance();
}
}
/**
* .tensorflow.SavedAsset asset = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedAsset, tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder, tensorflow.SavedObjectGraphOuterClass.SavedAssetOrBuilder>
getAssetFieldBuilder() {
if (assetBuilder_ == null) {
if (!(kindCase_ == 5)) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance();
}
assetBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedAsset, tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder, tensorflow.SavedObjectGraphOuterClass.SavedAssetOrBuilder>(
(tensorflow.SavedObjectGraphOuterClass.SavedAsset) kind_,
getParentForChildren(),
isClean());
kind_ = null;
}
kindCase_ = 5;
onChanged();;
return assetBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedFunction, tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder, tensorflow.SavedObjectGraphOuterClass.SavedFunctionOrBuilder> functionBuilder_;
/**
* .tensorflow.SavedFunction function = 6;
*/
public boolean hasFunction() {
return kindCase_ == 6;
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedFunction getFunction() {
if (functionBuilder_ == null) {
if (kindCase_ == 6) {
return (tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance();
} else {
if (kindCase_ == 6) {
return functionBuilder_.getMessage();
}
return tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance();
}
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public Builder setFunction(tensorflow.SavedObjectGraphOuterClass.SavedFunction value) {
if (functionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
onChanged();
} else {
functionBuilder_.setMessage(value);
}
kindCase_ = 6;
return this;
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public Builder setFunction(
tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder builderForValue) {
if (functionBuilder_ == null) {
kind_ = builderForValue.build();
onChanged();
} else {
functionBuilder_.setMessage(builderForValue.build());
}
kindCase_ = 6;
return this;
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public Builder mergeFunction(tensorflow.SavedObjectGraphOuterClass.SavedFunction value) {
if (functionBuilder_ == null) {
if (kindCase_ == 6 &&
kind_ != tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance()) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedFunction.newBuilder((tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_)
.mergeFrom(value).buildPartial();
} else {
kind_ = value;
}
onChanged();
} else {
if (kindCase_ == 6) {
functionBuilder_.mergeFrom(value);
}
functionBuilder_.setMessage(value);
}
kindCase_ = 6;
return this;
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public Builder clearFunction() {
if (functionBuilder_ == null) {
if (kindCase_ == 6) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
} else {
if (kindCase_ == 6) {
kindCase_ = 0;
kind_ = null;
}
functionBuilder_.clear();
}
return this;
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder getFunctionBuilder() {
return getFunctionFieldBuilder().getBuilder();
}
/**
* .tensorflow.SavedFunction function = 6;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedFunctionOrBuilder getFunctionOrBuilder() {
if ((kindCase_ == 6) && (functionBuilder_ != null)) {
return functionBuilder_.getMessageOrBuilder();
} else {
if (kindCase_ == 6) {
return (tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance();
}
}
/**
* .tensorflow.SavedFunction function = 6;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedFunction, tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder, tensorflow.SavedObjectGraphOuterClass.SavedFunctionOrBuilder>
getFunctionFieldBuilder() {
if (functionBuilder_ == null) {
if (!(kindCase_ == 6)) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance();
}
functionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedFunction, tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder, tensorflow.SavedObjectGraphOuterClass.SavedFunctionOrBuilder>(
(tensorflow.SavedObjectGraphOuterClass.SavedFunction) kind_,
getParentForChildren(),
isClean());
kind_ = null;
}
kindCase_ = 6;
onChanged();;
return functionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedVariable, tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder, tensorflow.SavedObjectGraphOuterClass.SavedVariableOrBuilder> variableBuilder_;
/**
* .tensorflow.SavedVariable variable = 7;
*/
public boolean hasVariable() {
return kindCase_ == 7;
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedVariable getVariable() {
if (variableBuilder_ == null) {
if (kindCase_ == 7) {
return (tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance();
} else {
if (kindCase_ == 7) {
return variableBuilder_.getMessage();
}
return tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance();
}
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public Builder setVariable(tensorflow.SavedObjectGraphOuterClass.SavedVariable value) {
if (variableBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
onChanged();
} else {
variableBuilder_.setMessage(value);
}
kindCase_ = 7;
return this;
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public Builder setVariable(
tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder builderForValue) {
if (variableBuilder_ == null) {
kind_ = builderForValue.build();
onChanged();
} else {
variableBuilder_.setMessage(builderForValue.build());
}
kindCase_ = 7;
return this;
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public Builder mergeVariable(tensorflow.SavedObjectGraphOuterClass.SavedVariable value) {
if (variableBuilder_ == null) {
if (kindCase_ == 7 &&
kind_ != tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance()) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedVariable.newBuilder((tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_)
.mergeFrom(value).buildPartial();
} else {
kind_ = value;
}
onChanged();
} else {
if (kindCase_ == 7) {
variableBuilder_.mergeFrom(value);
}
variableBuilder_.setMessage(value);
}
kindCase_ = 7;
return this;
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public Builder clearVariable() {
if (variableBuilder_ == null) {
if (kindCase_ == 7) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
} else {
if (kindCase_ == 7) {
kindCase_ = 0;
kind_ = null;
}
variableBuilder_.clear();
}
return this;
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder getVariableBuilder() {
return getVariableFieldBuilder().getBuilder();
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedVariableOrBuilder getVariableOrBuilder() {
if ((kindCase_ == 7) && (variableBuilder_ != null)) {
return variableBuilder_.getMessageOrBuilder();
} else {
if (kindCase_ == 7) {
return (tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance();
}
}
/**
* .tensorflow.SavedVariable variable = 7;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedVariable, tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder, tensorflow.SavedObjectGraphOuterClass.SavedVariableOrBuilder>
getVariableFieldBuilder() {
if (variableBuilder_ == null) {
if (!(kindCase_ == 7)) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance();
}
variableBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedVariable, tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder, tensorflow.SavedObjectGraphOuterClass.SavedVariableOrBuilder>(
(tensorflow.SavedObjectGraphOuterClass.SavedVariable) kind_,
getParentForChildren(),
isClean());
kind_ = null;
}
kindCase_ = 7;
onChanged();;
return variableBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunctionOrBuilder> bareConcreteFunctionBuilder_;
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public boolean hasBareConcreteFunction() {
return kindCase_ == 8;
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction getBareConcreteFunction() {
if (bareConcreteFunctionBuilder_ == null) {
if (kindCase_ == 8) {
return (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance();
} else {
if (kindCase_ == 8) {
return bareConcreteFunctionBuilder_.getMessage();
}
return tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance();
}
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public Builder setBareConcreteFunction(tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction value) {
if (bareConcreteFunctionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
onChanged();
} else {
bareConcreteFunctionBuilder_.setMessage(value);
}
kindCase_ = 8;
return this;
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public Builder setBareConcreteFunction(
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder builderForValue) {
if (bareConcreteFunctionBuilder_ == null) {
kind_ = builderForValue.build();
onChanged();
} else {
bareConcreteFunctionBuilder_.setMessage(builderForValue.build());
}
kindCase_ = 8;
return this;
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public Builder mergeBareConcreteFunction(tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction value) {
if (bareConcreteFunctionBuilder_ == null) {
if (kindCase_ == 8 &&
kind_ != tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance()) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.newBuilder((tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_)
.mergeFrom(value).buildPartial();
} else {
kind_ = value;
}
onChanged();
} else {
if (kindCase_ == 8) {
bareConcreteFunctionBuilder_.mergeFrom(value);
}
bareConcreteFunctionBuilder_.setMessage(value);
}
kindCase_ = 8;
return this;
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public Builder clearBareConcreteFunction() {
if (bareConcreteFunctionBuilder_ == null) {
if (kindCase_ == 8) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
} else {
if (kindCase_ == 8) {
kindCase_ = 0;
kind_ = null;
}
bareConcreteFunctionBuilder_.clear();
}
return this;
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder getBareConcreteFunctionBuilder() {
return getBareConcreteFunctionFieldBuilder().getBuilder();
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunctionOrBuilder getBareConcreteFunctionOrBuilder() {
if ((kindCase_ == 8) && (bareConcreteFunctionBuilder_ != null)) {
return bareConcreteFunctionBuilder_.getMessageOrBuilder();
} else {
if (kindCase_ == 8) {
return (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance();
}
}
/**
* .tensorflow.SavedBareConcreteFunction bare_concrete_function = 8;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunctionOrBuilder>
getBareConcreteFunctionFieldBuilder() {
if (bareConcreteFunctionBuilder_ == null) {
if (!(kindCase_ == 8)) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance();
}
bareConcreteFunctionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunctionOrBuilder>(
(tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) kind_,
getParentForChildren(),
isClean());
kind_ = null;
}
kindCase_ = 8;
onChanged();;
return bareConcreteFunctionBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedConstant, tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder, tensorflow.SavedObjectGraphOuterClass.SavedConstantOrBuilder> constantBuilder_;
/**
* .tensorflow.SavedConstant constant = 9;
*/
public boolean hasConstant() {
return kindCase_ == 9;
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConstant getConstant() {
if (constantBuilder_ == null) {
if (kindCase_ == 9) {
return (tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance();
} else {
if (kindCase_ == 9) {
return constantBuilder_.getMessage();
}
return tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance();
}
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public Builder setConstant(tensorflow.SavedObjectGraphOuterClass.SavedConstant value) {
if (constantBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
onChanged();
} else {
constantBuilder_.setMessage(value);
}
kindCase_ = 9;
return this;
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public Builder setConstant(
tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder builderForValue) {
if (constantBuilder_ == null) {
kind_ = builderForValue.build();
onChanged();
} else {
constantBuilder_.setMessage(builderForValue.build());
}
kindCase_ = 9;
return this;
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public Builder mergeConstant(tensorflow.SavedObjectGraphOuterClass.SavedConstant value) {
if (constantBuilder_ == null) {
if (kindCase_ == 9 &&
kind_ != tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance()) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedConstant.newBuilder((tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_)
.mergeFrom(value).buildPartial();
} else {
kind_ = value;
}
onChanged();
} else {
if (kindCase_ == 9) {
constantBuilder_.mergeFrom(value);
}
constantBuilder_.setMessage(value);
}
kindCase_ = 9;
return this;
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public Builder clearConstant() {
if (constantBuilder_ == null) {
if (kindCase_ == 9) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
} else {
if (kindCase_ == 9) {
kindCase_ = 0;
kind_ = null;
}
constantBuilder_.clear();
}
return this;
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder getConstantBuilder() {
return getConstantFieldBuilder().getBuilder();
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedConstantOrBuilder getConstantOrBuilder() {
if ((kindCase_ == 9) && (constantBuilder_ != null)) {
return constantBuilder_.getMessageOrBuilder();
} else {
if (kindCase_ == 9) {
return (tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance();
}
}
/**
* .tensorflow.SavedConstant constant = 9;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedConstant, tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder, tensorflow.SavedObjectGraphOuterClass.SavedConstantOrBuilder>
getConstantFieldBuilder() {
if (constantBuilder_ == null) {
if (!(kindCase_ == 9)) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance();
}
constantBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedConstant, tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder, tensorflow.SavedObjectGraphOuterClass.SavedConstantOrBuilder>(
(tensorflow.SavedObjectGraphOuterClass.SavedConstant) kind_,
getParentForChildren(),
isClean());
kind_ = null;
}
kindCase_ = 9;
onChanged();;
return constantBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedResource, tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder, tensorflow.SavedObjectGraphOuterClass.SavedResourceOrBuilder> resourceBuilder_;
/**
* .tensorflow.SavedResource resource = 10;
*/
public boolean hasResource() {
return kindCase_ == 10;
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedResource getResource() {
if (resourceBuilder_ == null) {
if (kindCase_ == 10) {
return (tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance();
} else {
if (kindCase_ == 10) {
return resourceBuilder_.getMessage();
}
return tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance();
}
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public Builder setResource(tensorflow.SavedObjectGraphOuterClass.SavedResource value) {
if (resourceBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
kind_ = value;
onChanged();
} else {
resourceBuilder_.setMessage(value);
}
kindCase_ = 10;
return this;
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public Builder setResource(
tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder builderForValue) {
if (resourceBuilder_ == null) {
kind_ = builderForValue.build();
onChanged();
} else {
resourceBuilder_.setMessage(builderForValue.build());
}
kindCase_ = 10;
return this;
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public Builder mergeResource(tensorflow.SavedObjectGraphOuterClass.SavedResource value) {
if (resourceBuilder_ == null) {
if (kindCase_ == 10 &&
kind_ != tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance()) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedResource.newBuilder((tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_)
.mergeFrom(value).buildPartial();
} else {
kind_ = value;
}
onChanged();
} else {
if (kindCase_ == 10) {
resourceBuilder_.mergeFrom(value);
}
resourceBuilder_.setMessage(value);
}
kindCase_ = 10;
return this;
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public Builder clearResource() {
if (resourceBuilder_ == null) {
if (kindCase_ == 10) {
kindCase_ = 0;
kind_ = null;
onChanged();
}
} else {
if (kindCase_ == 10) {
kindCase_ = 0;
kind_ = null;
}
resourceBuilder_.clear();
}
return this;
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder getResourceBuilder() {
return getResourceFieldBuilder().getBuilder();
}
/**
* .tensorflow.SavedResource resource = 10;
*/
public tensorflow.SavedObjectGraphOuterClass.SavedResourceOrBuilder getResourceOrBuilder() {
if ((kindCase_ == 10) && (resourceBuilder_ != null)) {
return resourceBuilder_.getMessageOrBuilder();
} else {
if (kindCase_ == 10) {
return (tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_;
}
return tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance();
}
}
/**
* .tensorflow.SavedResource resource = 10;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedResource, tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder, tensorflow.SavedObjectGraphOuterClass.SavedResourceOrBuilder>
getResourceFieldBuilder() {
if (resourceBuilder_ == null) {
if (!(kindCase_ == 10)) {
kind_ = tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance();
}
resourceBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.SavedResource, tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder, tensorflow.SavedObjectGraphOuterClass.SavedResourceOrBuilder>(
(tensorflow.SavedObjectGraphOuterClass.SavedResource) kind_,
getParentForChildren(),
isClean());
kind_ = null;
}
kindCase_ = 10;
onChanged();;
return resourceBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedObject)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedObject)
private static final tensorflow.SavedObjectGraphOuterClass.SavedObject DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedObject();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedObject getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedObject parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedObject(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedObject getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedUserObjectOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedUserObject)
com.google.protobuf.MessageOrBuilder {
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
java.lang.String getIdentifier();
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
com.google.protobuf.ByteString
getIdentifierBytes();
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
boolean hasVersion();
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
org.tensorflow.framework.VersionDef getVersion();
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
org.tensorflow.framework.VersionDefOrBuilder getVersionOrBuilder();
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
java.lang.String getMetadata();
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
com.google.protobuf.ByteString
getMetadataBytes();
}
/**
*
* A SavedUserObject is an object (in the object-oriented language of the
* TensorFlow program) of some user- or framework-defined class other than
* those handled specifically by the other kinds of SavedObjects.
* This object cannot be evaluated as a tensor, and therefore cannot be bound
* to an input of a function.
*
*
* Protobuf type {@code tensorflow.SavedUserObject}
*/
public static final class SavedUserObject extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedUserObject)
SavedUserObjectOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedUserObject.newBuilder() to construct.
private SavedUserObject(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedUserObject() {
identifier_ = "";
metadata_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedUserObject(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
identifier_ = s;
break;
}
case 18: {
org.tensorflow.framework.VersionDef.Builder subBuilder = null;
if (version_ != null) {
subBuilder = version_.toBuilder();
}
version_ = input.readMessage(org.tensorflow.framework.VersionDef.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(version_);
version_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
metadata_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedUserObject_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedUserObject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedUserObject.class, tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder.class);
}
public static final int IDENTIFIER_FIELD_NUMBER = 1;
private volatile java.lang.Object identifier_;
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
public java.lang.String getIdentifier() {
java.lang.Object ref = identifier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identifier_ = s;
return s;
}
}
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
public com.google.protobuf.ByteString
getIdentifierBytes() {
java.lang.Object ref = identifier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VERSION_FIELD_NUMBER = 2;
private org.tensorflow.framework.VersionDef version_;
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public boolean hasVersion() {
return version_ != null;
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public org.tensorflow.framework.VersionDef getVersion() {
return version_ == null ? org.tensorflow.framework.VersionDef.getDefaultInstance() : version_;
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public org.tensorflow.framework.VersionDefOrBuilder getVersionOrBuilder() {
return getVersion();
}
public static final int METADATA_FIELD_NUMBER = 3;
private volatile java.lang.Object metadata_;
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
public java.lang.String getMetadata() {
java.lang.Object ref = metadata_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
metadata_ = s;
return s;
}
}
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
public com.google.protobuf.ByteString
getMetadataBytes() {
java.lang.Object ref = metadata_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
metadata_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdentifierBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, identifier_);
}
if (version_ != null) {
output.writeMessage(2, getVersion());
}
if (!getMetadataBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, metadata_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdentifierBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, identifier_);
}
if (version_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getVersion());
}
if (!getMetadataBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, metadata_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedUserObject)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedUserObject other = (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) obj;
boolean result = true;
result = result && getIdentifier()
.equals(other.getIdentifier());
result = result && (hasVersion() == other.hasVersion());
if (hasVersion()) {
result = result && getVersion()
.equals(other.getVersion());
}
result = result && getMetadata()
.equals(other.getMetadata());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + IDENTIFIER_FIELD_NUMBER;
hash = (53 * hash) + getIdentifier().hashCode();
if (hasVersion()) {
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + getVersion().hashCode();
}
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + getMetadata().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedUserObject prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A SavedUserObject is an object (in the object-oriented language of the
* TensorFlow program) of some user- or framework-defined class other than
* those handled specifically by the other kinds of SavedObjects.
* This object cannot be evaluated as a tensor, and therefore cannot be bound
* to an input of a function.
*
*
* Protobuf type {@code tensorflow.SavedUserObject}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedUserObject)
tensorflow.SavedObjectGraphOuterClass.SavedUserObjectOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedUserObject_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedUserObject_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedUserObject.class, tensorflow.SavedObjectGraphOuterClass.SavedUserObject.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedUserObject.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
identifier_ = "";
if (versionBuilder_ == null) {
version_ = null;
} else {
version_ = null;
versionBuilder_ = null;
}
metadata_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedUserObject_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedUserObject getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedUserObject build() {
tensorflow.SavedObjectGraphOuterClass.SavedUserObject result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedUserObject buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedUserObject result = new tensorflow.SavedObjectGraphOuterClass.SavedUserObject(this);
result.identifier_ = identifier_;
if (versionBuilder_ == null) {
result.version_ = version_;
} else {
result.version_ = versionBuilder_.build();
}
result.metadata_ = metadata_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedUserObject) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedUserObject)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedUserObject other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedUserObject.getDefaultInstance()) return this;
if (!other.getIdentifier().isEmpty()) {
identifier_ = other.identifier_;
onChanged();
}
if (other.hasVersion()) {
mergeVersion(other.getVersion());
}
if (!other.getMetadata().isEmpty()) {
metadata_ = other.metadata_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedUserObject parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedUserObject) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object identifier_ = "";
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
public java.lang.String getIdentifier() {
java.lang.Object ref = identifier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
identifier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
public com.google.protobuf.ByteString
getIdentifierBytes() {
java.lang.Object ref = identifier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
identifier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
public Builder setIdentifier(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
identifier_ = value;
onChanged();
return this;
}
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
public Builder clearIdentifier() {
identifier_ = getDefaultInstance().getIdentifier();
onChanged();
return this;
}
/**
*
* Corresponds to a registration of the type to use in the loading program.
*
*
* string identifier = 1;
*/
public Builder setIdentifierBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
identifier_ = value;
onChanged();
return this;
}
private org.tensorflow.framework.VersionDef version_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.VersionDef, org.tensorflow.framework.VersionDef.Builder, org.tensorflow.framework.VersionDefOrBuilder> versionBuilder_;
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public boolean hasVersion() {
return versionBuilder_ != null || version_ != null;
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public org.tensorflow.framework.VersionDef getVersion() {
if (versionBuilder_ == null) {
return version_ == null ? org.tensorflow.framework.VersionDef.getDefaultInstance() : version_;
} else {
return versionBuilder_.getMessage();
}
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public Builder setVersion(org.tensorflow.framework.VersionDef value) {
if (versionBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
version_ = value;
onChanged();
} else {
versionBuilder_.setMessage(value);
}
return this;
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public Builder setVersion(
org.tensorflow.framework.VersionDef.Builder builderForValue) {
if (versionBuilder_ == null) {
version_ = builderForValue.build();
onChanged();
} else {
versionBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public Builder mergeVersion(org.tensorflow.framework.VersionDef value) {
if (versionBuilder_ == null) {
if (version_ != null) {
version_ =
org.tensorflow.framework.VersionDef.newBuilder(version_).mergeFrom(value).buildPartial();
} else {
version_ = value;
}
onChanged();
} else {
versionBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public Builder clearVersion() {
if (versionBuilder_ == null) {
version_ = null;
onChanged();
} else {
version_ = null;
versionBuilder_ = null;
}
return this;
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public org.tensorflow.framework.VersionDef.Builder getVersionBuilder() {
onChanged();
return getVersionFieldBuilder().getBuilder();
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
public org.tensorflow.framework.VersionDefOrBuilder getVersionOrBuilder() {
if (versionBuilder_ != null) {
return versionBuilder_.getMessageOrBuilder();
} else {
return version_ == null ?
org.tensorflow.framework.VersionDef.getDefaultInstance() : version_;
}
}
/**
*
* Version information from the producer of this SavedUserObject.
*
*
* .tensorflow.VersionDef version = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.VersionDef, org.tensorflow.framework.VersionDef.Builder, org.tensorflow.framework.VersionDefOrBuilder>
getVersionFieldBuilder() {
if (versionBuilder_ == null) {
versionBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.VersionDef, org.tensorflow.framework.VersionDef.Builder, org.tensorflow.framework.VersionDefOrBuilder>(
getVersion(),
getParentForChildren(),
isClean());
version_ = null;
}
return versionBuilder_;
}
private java.lang.Object metadata_ = "";
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
public java.lang.String getMetadata() {
java.lang.Object ref = metadata_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
metadata_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
public com.google.protobuf.ByteString
getMetadataBytes() {
java.lang.Object ref = metadata_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
metadata_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
public Builder setMetadata(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
metadata_ = value;
onChanged();
return this;
}
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
public Builder clearMetadata() {
metadata_ = getDefaultInstance().getMetadata();
onChanged();
return this;
}
/**
*
* Initialization-related metadata.
*
*
* string metadata = 3;
*/
public Builder setMetadataBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
metadata_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedUserObject)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedUserObject)
private static final tensorflow.SavedObjectGraphOuterClass.SavedUserObject DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedUserObject();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedUserObject getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedUserObject parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedUserObject(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedUserObject getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedAssetOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedAsset)
com.google.protobuf.MessageOrBuilder {
/**
*
* Index into `MetaGraphDef.asset_file_def[]` that describes the Asset.
* Only the field `AssetFileDef.filename` is used. Other fields, such as
* `AssetFileDef.tensor_info`, MUST be ignored.
*
*
* int32 asset_file_def_index = 1;
*/
int getAssetFileDefIndex();
}
/**
*
* A SavedAsset points to an asset in the MetaGraph.
* When bound to a function this object evaluates to a tensor with the absolute
* filename. Users should not depend on a particular part of the filename to
* remain stable (e.g. basename could be changed).
*
*
* Protobuf type {@code tensorflow.SavedAsset}
*/
public static final class SavedAsset extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedAsset)
SavedAssetOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedAsset.newBuilder() to construct.
private SavedAsset(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedAsset() {
assetFileDefIndex_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedAsset(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
assetFileDefIndex_ = input.readInt32();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedAsset_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedAsset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedAsset.class, tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder.class);
}
public static final int ASSET_FILE_DEF_INDEX_FIELD_NUMBER = 1;
private int assetFileDefIndex_;
/**
*
* Index into `MetaGraphDef.asset_file_def[]` that describes the Asset.
* Only the field `AssetFileDef.filename` is used. Other fields, such as
* `AssetFileDef.tensor_info`, MUST be ignored.
*
*
* int32 asset_file_def_index = 1;
*/
public int getAssetFileDefIndex() {
return assetFileDefIndex_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (assetFileDefIndex_ != 0) {
output.writeInt32(1, assetFileDefIndex_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (assetFileDefIndex_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(1, assetFileDefIndex_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedAsset)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedAsset other = (tensorflow.SavedObjectGraphOuterClass.SavedAsset) obj;
boolean result = true;
result = result && (getAssetFileDefIndex()
== other.getAssetFileDefIndex());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ASSET_FILE_DEF_INDEX_FIELD_NUMBER;
hash = (53 * hash) + getAssetFileDefIndex();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedAsset prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A SavedAsset points to an asset in the MetaGraph.
* When bound to a function this object evaluates to a tensor with the absolute
* filename. Users should not depend on a particular part of the filename to
* remain stable (e.g. basename could be changed).
*
*
* Protobuf type {@code tensorflow.SavedAsset}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedAsset)
tensorflow.SavedObjectGraphOuterClass.SavedAssetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedAsset_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedAsset_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedAsset.class, tensorflow.SavedObjectGraphOuterClass.SavedAsset.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedAsset.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
assetFileDefIndex_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedAsset_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedAsset getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedAsset build() {
tensorflow.SavedObjectGraphOuterClass.SavedAsset result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedAsset buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedAsset result = new tensorflow.SavedObjectGraphOuterClass.SavedAsset(this);
result.assetFileDefIndex_ = assetFileDefIndex_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedAsset) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedAsset)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedAsset other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedAsset.getDefaultInstance()) return this;
if (other.getAssetFileDefIndex() != 0) {
setAssetFileDefIndex(other.getAssetFileDefIndex());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedAsset parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedAsset) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int assetFileDefIndex_ ;
/**
*
* Index into `MetaGraphDef.asset_file_def[]` that describes the Asset.
* Only the field `AssetFileDef.filename` is used. Other fields, such as
* `AssetFileDef.tensor_info`, MUST be ignored.
*
*
* int32 asset_file_def_index = 1;
*/
public int getAssetFileDefIndex() {
return assetFileDefIndex_;
}
/**
*
* Index into `MetaGraphDef.asset_file_def[]` that describes the Asset.
* Only the field `AssetFileDef.filename` is used. Other fields, such as
* `AssetFileDef.tensor_info`, MUST be ignored.
*
*
* int32 asset_file_def_index = 1;
*/
public Builder setAssetFileDefIndex(int value) {
assetFileDefIndex_ = value;
onChanged();
return this;
}
/**
*
* Index into `MetaGraphDef.asset_file_def[]` that describes the Asset.
* Only the field `AssetFileDef.filename` is used. Other fields, such as
* `AssetFileDef.tensor_info`, MUST be ignored.
*
*
* int32 asset_file_def_index = 1;
*/
public Builder clearAssetFileDefIndex() {
assetFileDefIndex_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedAsset)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedAsset)
private static final tensorflow.SavedObjectGraphOuterClass.SavedAsset DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedAsset();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedAsset getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedAsset parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedAsset(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedAsset getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedFunctionOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedFunction)
com.google.protobuf.MessageOrBuilder {
/**
* repeated string concrete_functions = 1;
*/
java.util.List
getConcreteFunctionsList();
/**
* repeated string concrete_functions = 1;
*/
int getConcreteFunctionsCount();
/**
* repeated string concrete_functions = 1;
*/
java.lang.String getConcreteFunctions(int index);
/**
* repeated string concrete_functions = 1;
*/
com.google.protobuf.ByteString
getConcreteFunctionsBytes(int index);
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
boolean hasFunctionSpec();
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
tensorflow.SavedObjectGraphOuterClass.FunctionSpec getFunctionSpec();
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
tensorflow.SavedObjectGraphOuterClass.FunctionSpecOrBuilder getFunctionSpecOrBuilder();
}
/**
*
* A function with multiple signatures, possibly with non-Tensor arguments.
*
*
* Protobuf type {@code tensorflow.SavedFunction}
*/
public static final class SavedFunction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedFunction)
SavedFunctionOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedFunction.newBuilder() to construct.
private SavedFunction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedFunction() {
concreteFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedFunction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
concreteFunctions_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
concreteFunctions_.add(s);
break;
}
case 18: {
tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder subBuilder = null;
if (functionSpec_ != null) {
subBuilder = functionSpec_.toBuilder();
}
functionSpec_ = input.readMessage(tensorflow.SavedObjectGraphOuterClass.FunctionSpec.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(functionSpec_);
functionSpec_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
concreteFunctions_ = concreteFunctions_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedFunction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedFunction.class, tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder.class);
}
private int bitField0_;
public static final int CONCRETE_FUNCTIONS_FIELD_NUMBER = 1;
private com.google.protobuf.LazyStringList concreteFunctions_;
/**
* repeated string concrete_functions = 1;
*/
public com.google.protobuf.ProtocolStringList
getConcreteFunctionsList() {
return concreteFunctions_;
}
/**
* repeated string concrete_functions = 1;
*/
public int getConcreteFunctionsCount() {
return concreteFunctions_.size();
}
/**
* repeated string concrete_functions = 1;
*/
public java.lang.String getConcreteFunctions(int index) {
return concreteFunctions_.get(index);
}
/**
* repeated string concrete_functions = 1;
*/
public com.google.protobuf.ByteString
getConcreteFunctionsBytes(int index) {
return concreteFunctions_.getByteString(index);
}
public static final int FUNCTION_SPEC_FIELD_NUMBER = 2;
private tensorflow.SavedObjectGraphOuterClass.FunctionSpec functionSpec_;
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public boolean hasFunctionSpec() {
return functionSpec_ != null;
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.FunctionSpec getFunctionSpec() {
return functionSpec_ == null ? tensorflow.SavedObjectGraphOuterClass.FunctionSpec.getDefaultInstance() : functionSpec_;
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.FunctionSpecOrBuilder getFunctionSpecOrBuilder() {
return getFunctionSpec();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < concreteFunctions_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, concreteFunctions_.getRaw(i));
}
if (functionSpec_ != null) {
output.writeMessage(2, getFunctionSpec());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < concreteFunctions_.size(); i++) {
dataSize += computeStringSizeNoTag(concreteFunctions_.getRaw(i));
}
size += dataSize;
size += 1 * getConcreteFunctionsList().size();
}
if (functionSpec_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getFunctionSpec());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedFunction)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedFunction other = (tensorflow.SavedObjectGraphOuterClass.SavedFunction) obj;
boolean result = true;
result = result && getConcreteFunctionsList()
.equals(other.getConcreteFunctionsList());
result = result && (hasFunctionSpec() == other.hasFunctionSpec());
if (hasFunctionSpec()) {
result = result && getFunctionSpec()
.equals(other.getFunctionSpec());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConcreteFunctionsCount() > 0) {
hash = (37 * hash) + CONCRETE_FUNCTIONS_FIELD_NUMBER;
hash = (53 * hash) + getConcreteFunctionsList().hashCode();
}
if (hasFunctionSpec()) {
hash = (37 * hash) + FUNCTION_SPEC_FIELD_NUMBER;
hash = (53 * hash) + getFunctionSpec().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedFunction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A function with multiple signatures, possibly with non-Tensor arguments.
*
*
* Protobuf type {@code tensorflow.SavedFunction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedFunction)
tensorflow.SavedObjectGraphOuterClass.SavedFunctionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedFunction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedFunction.class, tensorflow.SavedObjectGraphOuterClass.SavedFunction.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedFunction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
concreteFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
if (functionSpecBuilder_ == null) {
functionSpec_ = null;
} else {
functionSpec_ = null;
functionSpecBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedFunction_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedFunction getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedFunction build() {
tensorflow.SavedObjectGraphOuterClass.SavedFunction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedFunction buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedFunction result = new tensorflow.SavedObjectGraphOuterClass.SavedFunction(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
concreteFunctions_ = concreteFunctions_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.concreteFunctions_ = concreteFunctions_;
if (functionSpecBuilder_ == null) {
result.functionSpec_ = functionSpec_;
} else {
result.functionSpec_ = functionSpecBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedFunction) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedFunction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedFunction other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedFunction.getDefaultInstance()) return this;
if (!other.concreteFunctions_.isEmpty()) {
if (concreteFunctions_.isEmpty()) {
concreteFunctions_ = other.concreteFunctions_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConcreteFunctionsIsMutable();
concreteFunctions_.addAll(other.concreteFunctions_);
}
onChanged();
}
if (other.hasFunctionSpec()) {
mergeFunctionSpec(other.getFunctionSpec());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedFunction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedFunction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private com.google.protobuf.LazyStringList concreteFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureConcreteFunctionsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
concreteFunctions_ = new com.google.protobuf.LazyStringArrayList(concreteFunctions_);
bitField0_ |= 0x00000001;
}
}
/**
* repeated string concrete_functions = 1;
*/
public com.google.protobuf.ProtocolStringList
getConcreteFunctionsList() {
return concreteFunctions_.getUnmodifiableView();
}
/**
* repeated string concrete_functions = 1;
*/
public int getConcreteFunctionsCount() {
return concreteFunctions_.size();
}
/**
* repeated string concrete_functions = 1;
*/
public java.lang.String getConcreteFunctions(int index) {
return concreteFunctions_.get(index);
}
/**
* repeated string concrete_functions = 1;
*/
public com.google.protobuf.ByteString
getConcreteFunctionsBytes(int index) {
return concreteFunctions_.getByteString(index);
}
/**
* repeated string concrete_functions = 1;
*/
public Builder setConcreteFunctions(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConcreteFunctionsIsMutable();
concreteFunctions_.set(index, value);
onChanged();
return this;
}
/**
* repeated string concrete_functions = 1;
*/
public Builder addConcreteFunctions(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureConcreteFunctionsIsMutable();
concreteFunctions_.add(value);
onChanged();
return this;
}
/**
* repeated string concrete_functions = 1;
*/
public Builder addAllConcreteFunctions(
java.lang.Iterable values) {
ensureConcreteFunctionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, concreteFunctions_);
onChanged();
return this;
}
/**
* repeated string concrete_functions = 1;
*/
public Builder clearConcreteFunctions() {
concreteFunctions_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* repeated string concrete_functions = 1;
*/
public Builder addConcreteFunctionsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureConcreteFunctionsIsMutable();
concreteFunctions_.add(value);
onChanged();
return this;
}
private tensorflow.SavedObjectGraphOuterClass.FunctionSpec functionSpec_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.FunctionSpec, tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder, tensorflow.SavedObjectGraphOuterClass.FunctionSpecOrBuilder> functionSpecBuilder_;
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public boolean hasFunctionSpec() {
return functionSpecBuilder_ != null || functionSpec_ != null;
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.FunctionSpec getFunctionSpec() {
if (functionSpecBuilder_ == null) {
return functionSpec_ == null ? tensorflow.SavedObjectGraphOuterClass.FunctionSpec.getDefaultInstance() : functionSpec_;
} else {
return functionSpecBuilder_.getMessage();
}
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public Builder setFunctionSpec(tensorflow.SavedObjectGraphOuterClass.FunctionSpec value) {
if (functionSpecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
functionSpec_ = value;
onChanged();
} else {
functionSpecBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public Builder setFunctionSpec(
tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder builderForValue) {
if (functionSpecBuilder_ == null) {
functionSpec_ = builderForValue.build();
onChanged();
} else {
functionSpecBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public Builder mergeFunctionSpec(tensorflow.SavedObjectGraphOuterClass.FunctionSpec value) {
if (functionSpecBuilder_ == null) {
if (functionSpec_ != null) {
functionSpec_ =
tensorflow.SavedObjectGraphOuterClass.FunctionSpec.newBuilder(functionSpec_).mergeFrom(value).buildPartial();
} else {
functionSpec_ = value;
}
onChanged();
} else {
functionSpecBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public Builder clearFunctionSpec() {
if (functionSpecBuilder_ == null) {
functionSpec_ = null;
onChanged();
} else {
functionSpec_ = null;
functionSpecBuilder_ = null;
}
return this;
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder getFunctionSpecBuilder() {
onChanged();
return getFunctionSpecFieldBuilder().getBuilder();
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
public tensorflow.SavedObjectGraphOuterClass.FunctionSpecOrBuilder getFunctionSpecOrBuilder() {
if (functionSpecBuilder_ != null) {
return functionSpecBuilder_.getMessageOrBuilder();
} else {
return functionSpec_ == null ?
tensorflow.SavedObjectGraphOuterClass.FunctionSpec.getDefaultInstance() : functionSpec_;
}
}
/**
* .tensorflow.FunctionSpec function_spec = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.FunctionSpec, tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder, tensorflow.SavedObjectGraphOuterClass.FunctionSpecOrBuilder>
getFunctionSpecFieldBuilder() {
if (functionSpecBuilder_ == null) {
functionSpecBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.SavedObjectGraphOuterClass.FunctionSpec, tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder, tensorflow.SavedObjectGraphOuterClass.FunctionSpecOrBuilder>(
getFunctionSpec(),
getParentForChildren(),
isClean());
functionSpec_ = null;
}
return functionSpecBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedFunction)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedFunction)
private static final tensorflow.SavedObjectGraphOuterClass.SavedFunction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedFunction();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedFunction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedFunction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedFunction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedFunction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedConcreteFunctionOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedConcreteFunction)
com.google.protobuf.MessageOrBuilder {
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
java.util.List getBoundInputsList();
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
int getBoundInputsCount();
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
int getBoundInputs(int index);
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
boolean hasCanonicalizedInputSignature();
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
tensorflow.Struct.StructuredValue getCanonicalizedInputSignature();
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
tensorflow.Struct.StructuredValueOrBuilder getCanonicalizedInputSignatureOrBuilder();
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
boolean hasOutputSignature();
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
tensorflow.Struct.StructuredValue getOutputSignature();
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
tensorflow.Struct.StructuredValueOrBuilder getOutputSignatureOrBuilder();
}
/**
*
* Stores low-level information about a concrete function. Referenced in either
* a SavedFunction or a SavedBareConcreteFunction.
*
*
* Protobuf type {@code tensorflow.SavedConcreteFunction}
*/
public static final class SavedConcreteFunction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedConcreteFunction)
SavedConcreteFunctionOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedConcreteFunction.newBuilder() to construct.
private SavedConcreteFunction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedConcreteFunction() {
boundInputs_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedConcreteFunction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 16: {
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
boundInputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
boundInputs_.add(input.readInt32());
break;
}
case 18: {
int length = input.readRawVarint32();
int limit = input.pushLimit(length);
if (!((mutable_bitField0_ & 0x00000001) == 0x00000001) && input.getBytesUntilLimit() > 0) {
boundInputs_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
while (input.getBytesUntilLimit() > 0) {
boundInputs_.add(input.readInt32());
}
input.popLimit(limit);
break;
}
case 26: {
tensorflow.Struct.StructuredValue.Builder subBuilder = null;
if (canonicalizedInputSignature_ != null) {
subBuilder = canonicalizedInputSignature_.toBuilder();
}
canonicalizedInputSignature_ = input.readMessage(tensorflow.Struct.StructuredValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(canonicalizedInputSignature_);
canonicalizedInputSignature_ = subBuilder.buildPartial();
}
break;
}
case 34: {
tensorflow.Struct.StructuredValue.Builder subBuilder = null;
if (outputSignature_ != null) {
subBuilder = outputSignature_.toBuilder();
}
outputSignature_ = input.readMessage(tensorflow.Struct.StructuredValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(outputSignature_);
outputSignature_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) == 0x00000001)) {
boundInputs_ = java.util.Collections.unmodifiableList(boundInputs_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConcreteFunction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConcreteFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.class, tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.Builder.class);
}
private int bitField0_;
public static final int BOUND_INPUTS_FIELD_NUMBER = 2;
private java.util.List boundInputs_;
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public java.util.List
getBoundInputsList() {
return boundInputs_;
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public int getBoundInputsCount() {
return boundInputs_.size();
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public int getBoundInputs(int index) {
return boundInputs_.get(index);
}
private int boundInputsMemoizedSerializedSize = -1;
public static final int CANONICALIZED_INPUT_SIGNATURE_FIELD_NUMBER = 3;
private tensorflow.Struct.StructuredValue canonicalizedInputSignature_;
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public boolean hasCanonicalizedInputSignature() {
return canonicalizedInputSignature_ != null;
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public tensorflow.Struct.StructuredValue getCanonicalizedInputSignature() {
return canonicalizedInputSignature_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : canonicalizedInputSignature_;
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public tensorflow.Struct.StructuredValueOrBuilder getCanonicalizedInputSignatureOrBuilder() {
return getCanonicalizedInputSignature();
}
public static final int OUTPUT_SIGNATURE_FIELD_NUMBER = 4;
private tensorflow.Struct.StructuredValue outputSignature_;
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public boolean hasOutputSignature() {
return outputSignature_ != null;
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public tensorflow.Struct.StructuredValue getOutputSignature() {
return outputSignature_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : outputSignature_;
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public tensorflow.Struct.StructuredValueOrBuilder getOutputSignatureOrBuilder() {
return getOutputSignature();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (getBoundInputsList().size() > 0) {
output.writeUInt32NoTag(18);
output.writeUInt32NoTag(boundInputsMemoizedSerializedSize);
}
for (int i = 0; i < boundInputs_.size(); i++) {
output.writeInt32NoTag(boundInputs_.get(i));
}
if (canonicalizedInputSignature_ != null) {
output.writeMessage(3, getCanonicalizedInputSignature());
}
if (outputSignature_ != null) {
output.writeMessage(4, getOutputSignature());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
{
int dataSize = 0;
for (int i = 0; i < boundInputs_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(boundInputs_.get(i));
}
size += dataSize;
if (!getBoundInputsList().isEmpty()) {
size += 1;
size += com.google.protobuf.CodedOutputStream
.computeInt32SizeNoTag(dataSize);
}
boundInputsMemoizedSerializedSize = dataSize;
}
if (canonicalizedInputSignature_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getCanonicalizedInputSignature());
}
if (outputSignature_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getOutputSignature());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction other = (tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction) obj;
boolean result = true;
result = result && getBoundInputsList()
.equals(other.getBoundInputsList());
result = result && (hasCanonicalizedInputSignature() == other.hasCanonicalizedInputSignature());
if (hasCanonicalizedInputSignature()) {
result = result && getCanonicalizedInputSignature()
.equals(other.getCanonicalizedInputSignature());
}
result = result && (hasOutputSignature() == other.hasOutputSignature());
if (hasOutputSignature()) {
result = result && getOutputSignature()
.equals(other.getOutputSignature());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getBoundInputsCount() > 0) {
hash = (37 * hash) + BOUND_INPUTS_FIELD_NUMBER;
hash = (53 * hash) + getBoundInputsList().hashCode();
}
if (hasCanonicalizedInputSignature()) {
hash = (37 * hash) + CANONICALIZED_INPUT_SIGNATURE_FIELD_NUMBER;
hash = (53 * hash) + getCanonicalizedInputSignature().hashCode();
}
if (hasOutputSignature()) {
hash = (37 * hash) + OUTPUT_SIGNATURE_FIELD_NUMBER;
hash = (53 * hash) + getOutputSignature().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Stores low-level information about a concrete function. Referenced in either
* a SavedFunction or a SavedBareConcreteFunction.
*
*
* Protobuf type {@code tensorflow.SavedConcreteFunction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedConcreteFunction)
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunctionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConcreteFunction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConcreteFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.class, tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
boundInputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
if (canonicalizedInputSignatureBuilder_ == null) {
canonicalizedInputSignature_ = null;
} else {
canonicalizedInputSignature_ = null;
canonicalizedInputSignatureBuilder_ = null;
}
if (outputSignatureBuilder_ == null) {
outputSignature_ = null;
} else {
outputSignature_ = null;
outputSignatureBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConcreteFunction_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction build() {
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction result = new tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (((bitField0_ & 0x00000001) == 0x00000001)) {
boundInputs_ = java.util.Collections.unmodifiableList(boundInputs_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.boundInputs_ = boundInputs_;
if (canonicalizedInputSignatureBuilder_ == null) {
result.canonicalizedInputSignature_ = canonicalizedInputSignature_;
} else {
result.canonicalizedInputSignature_ = canonicalizedInputSignatureBuilder_.build();
}
if (outputSignatureBuilder_ == null) {
result.outputSignature_ = outputSignature_;
} else {
result.outputSignature_ = outputSignatureBuilder_.build();
}
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction.getDefaultInstance()) return this;
if (!other.boundInputs_.isEmpty()) {
if (boundInputs_.isEmpty()) {
boundInputs_ = other.boundInputs_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureBoundInputsIsMutable();
boundInputs_.addAll(other.boundInputs_);
}
onChanged();
}
if (other.hasCanonicalizedInputSignature()) {
mergeCanonicalizedInputSignature(other.getCanonicalizedInputSignature());
}
if (other.hasOutputSignature()) {
mergeOutputSignature(other.getOutputSignature());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List boundInputs_ = java.util.Collections.emptyList();
private void ensureBoundInputsIsMutable() {
if (!((bitField0_ & 0x00000001) == 0x00000001)) {
boundInputs_ = new java.util.ArrayList(boundInputs_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public java.util.List
getBoundInputsList() {
return java.util.Collections.unmodifiableList(boundInputs_);
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public int getBoundInputsCount() {
return boundInputs_.size();
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public int getBoundInputs(int index) {
return boundInputs_.get(index);
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public Builder setBoundInputs(
int index, int value) {
ensureBoundInputsIsMutable();
boundInputs_.set(index, value);
onChanged();
return this;
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public Builder addBoundInputs(int value) {
ensureBoundInputsIsMutable();
boundInputs_.add(value);
onChanged();
return this;
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public Builder addAllBoundInputs(
java.lang.Iterable extends java.lang.Integer> values) {
ensureBoundInputsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, boundInputs_);
onChanged();
return this;
}
/**
*
* Bound inputs to the function. The SavedObjects identified by the node ids
* given here are appended as extra inputs to the caller-supplied inputs.
* The only types of SavedObjects valid here are SavedVariable, SavedResource
* and SavedAsset.
*
*
* repeated int32 bound_inputs = 2;
*/
public Builder clearBoundInputs() {
boundInputs_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
private tensorflow.Struct.StructuredValue canonicalizedInputSignature_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder> canonicalizedInputSignatureBuilder_;
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public boolean hasCanonicalizedInputSignature() {
return canonicalizedInputSignatureBuilder_ != null || canonicalizedInputSignature_ != null;
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public tensorflow.Struct.StructuredValue getCanonicalizedInputSignature() {
if (canonicalizedInputSignatureBuilder_ == null) {
return canonicalizedInputSignature_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : canonicalizedInputSignature_;
} else {
return canonicalizedInputSignatureBuilder_.getMessage();
}
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public Builder setCanonicalizedInputSignature(tensorflow.Struct.StructuredValue value) {
if (canonicalizedInputSignatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
canonicalizedInputSignature_ = value;
onChanged();
} else {
canonicalizedInputSignatureBuilder_.setMessage(value);
}
return this;
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public Builder setCanonicalizedInputSignature(
tensorflow.Struct.StructuredValue.Builder builderForValue) {
if (canonicalizedInputSignatureBuilder_ == null) {
canonicalizedInputSignature_ = builderForValue.build();
onChanged();
} else {
canonicalizedInputSignatureBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public Builder mergeCanonicalizedInputSignature(tensorflow.Struct.StructuredValue value) {
if (canonicalizedInputSignatureBuilder_ == null) {
if (canonicalizedInputSignature_ != null) {
canonicalizedInputSignature_ =
tensorflow.Struct.StructuredValue.newBuilder(canonicalizedInputSignature_).mergeFrom(value).buildPartial();
} else {
canonicalizedInputSignature_ = value;
}
onChanged();
} else {
canonicalizedInputSignatureBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public Builder clearCanonicalizedInputSignature() {
if (canonicalizedInputSignatureBuilder_ == null) {
canonicalizedInputSignature_ = null;
onChanged();
} else {
canonicalizedInputSignature_ = null;
canonicalizedInputSignatureBuilder_ = null;
}
return this;
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public tensorflow.Struct.StructuredValue.Builder getCanonicalizedInputSignatureBuilder() {
onChanged();
return getCanonicalizedInputSignatureFieldBuilder().getBuilder();
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
public tensorflow.Struct.StructuredValueOrBuilder getCanonicalizedInputSignatureOrBuilder() {
if (canonicalizedInputSignatureBuilder_ != null) {
return canonicalizedInputSignatureBuilder_.getMessageOrBuilder();
} else {
return canonicalizedInputSignature_ == null ?
tensorflow.Struct.StructuredValue.getDefaultInstance() : canonicalizedInputSignature_;
}
}
/**
*
* Input in canonicalized form that was received to create this concrete
* function.
*
*
* .tensorflow.StructuredValue canonicalized_input_signature = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>
getCanonicalizedInputSignatureFieldBuilder() {
if (canonicalizedInputSignatureBuilder_ == null) {
canonicalizedInputSignatureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>(
getCanonicalizedInputSignature(),
getParentForChildren(),
isClean());
canonicalizedInputSignature_ = null;
}
return canonicalizedInputSignatureBuilder_;
}
private tensorflow.Struct.StructuredValue outputSignature_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder> outputSignatureBuilder_;
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public boolean hasOutputSignature() {
return outputSignatureBuilder_ != null || outputSignature_ != null;
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public tensorflow.Struct.StructuredValue getOutputSignature() {
if (outputSignatureBuilder_ == null) {
return outputSignature_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : outputSignature_;
} else {
return outputSignatureBuilder_.getMessage();
}
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public Builder setOutputSignature(tensorflow.Struct.StructuredValue value) {
if (outputSignatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
outputSignature_ = value;
onChanged();
} else {
outputSignatureBuilder_.setMessage(value);
}
return this;
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public Builder setOutputSignature(
tensorflow.Struct.StructuredValue.Builder builderForValue) {
if (outputSignatureBuilder_ == null) {
outputSignature_ = builderForValue.build();
onChanged();
} else {
outputSignatureBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public Builder mergeOutputSignature(tensorflow.Struct.StructuredValue value) {
if (outputSignatureBuilder_ == null) {
if (outputSignature_ != null) {
outputSignature_ =
tensorflow.Struct.StructuredValue.newBuilder(outputSignature_).mergeFrom(value).buildPartial();
} else {
outputSignature_ = value;
}
onChanged();
} else {
outputSignatureBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public Builder clearOutputSignature() {
if (outputSignatureBuilder_ == null) {
outputSignature_ = null;
onChanged();
} else {
outputSignature_ = null;
outputSignatureBuilder_ = null;
}
return this;
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public tensorflow.Struct.StructuredValue.Builder getOutputSignatureBuilder() {
onChanged();
return getOutputSignatureFieldBuilder().getBuilder();
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
public tensorflow.Struct.StructuredValueOrBuilder getOutputSignatureOrBuilder() {
if (outputSignatureBuilder_ != null) {
return outputSignatureBuilder_.getMessageOrBuilder();
} else {
return outputSignature_ == null ?
tensorflow.Struct.StructuredValue.getDefaultInstance() : outputSignature_;
}
}
/**
*
* Output that was the return value of this function after replacing all
* Tensors with TensorSpecs. This can be an arbitrary nested function and will
* be used to reconstruct the full structure from pure tensors.
*
*
* .tensorflow.StructuredValue output_signature = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>
getOutputSignatureFieldBuilder() {
if (outputSignatureBuilder_ == null) {
outputSignatureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>(
getOutputSignature(),
getParentForChildren(),
isClean());
outputSignature_ = null;
}
return outputSignatureBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedConcreteFunction)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedConcreteFunction)
private static final tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedConcreteFunction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedConcreteFunction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedConcreteFunction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedBareConcreteFunctionOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedBareConcreteFunction)
com.google.protobuf.MessageOrBuilder {
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
java.lang.String getConcreteFunctionName();
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
com.google.protobuf.ByteString
getConcreteFunctionNameBytes();
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
java.util.List
getArgumentKeywordsList();
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
int getArgumentKeywordsCount();
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
java.lang.String getArgumentKeywords(int index);
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
com.google.protobuf.ByteString
getArgumentKeywordsBytes(int index);
/**
*
* The prefix of `argument_keywords` which may be identified by position.
*
*
* int64 allowed_positional_arguments = 3;
*/
long getAllowedPositionalArguments();
}
/**
* Protobuf type {@code tensorflow.SavedBareConcreteFunction}
*/
public static final class SavedBareConcreteFunction extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedBareConcreteFunction)
SavedBareConcreteFunctionOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedBareConcreteFunction.newBuilder() to construct.
private SavedBareConcreteFunction(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedBareConcreteFunction() {
concreteFunctionName_ = "";
argumentKeywords_ = com.google.protobuf.LazyStringArrayList.EMPTY;
allowedPositionalArguments_ = 0L;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedBareConcreteFunction(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
concreteFunctionName_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
argumentKeywords_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000002;
}
argumentKeywords_.add(s);
break;
}
case 24: {
allowedPositionalArguments_ = input.readInt64();
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000002) == 0x00000002)) {
argumentKeywords_ = argumentKeywords_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedBareConcreteFunction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedBareConcreteFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.class, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder.class);
}
private int bitField0_;
public static final int CONCRETE_FUNCTION_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object concreteFunctionName_;
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
public java.lang.String getConcreteFunctionName() {
java.lang.Object ref = concreteFunctionName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
concreteFunctionName_ = s;
return s;
}
}
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
public com.google.protobuf.ByteString
getConcreteFunctionNameBytes() {
java.lang.Object ref = concreteFunctionName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
concreteFunctionName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ARGUMENT_KEYWORDS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList argumentKeywords_;
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public com.google.protobuf.ProtocolStringList
getArgumentKeywordsList() {
return argumentKeywords_;
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public int getArgumentKeywordsCount() {
return argumentKeywords_.size();
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public java.lang.String getArgumentKeywords(int index) {
return argumentKeywords_.get(index);
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public com.google.protobuf.ByteString
getArgumentKeywordsBytes(int index) {
return argumentKeywords_.getByteString(index);
}
public static final int ALLOWED_POSITIONAL_ARGUMENTS_FIELD_NUMBER = 3;
private long allowedPositionalArguments_;
/**
*
* The prefix of `argument_keywords` which may be identified by position.
*
*
* int64 allowed_positional_arguments = 3;
*/
public long getAllowedPositionalArguments() {
return allowedPositionalArguments_;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getConcreteFunctionNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, concreteFunctionName_);
}
for (int i = 0; i < argumentKeywords_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, argumentKeywords_.getRaw(i));
}
if (allowedPositionalArguments_ != 0L) {
output.writeInt64(3, allowedPositionalArguments_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getConcreteFunctionNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, concreteFunctionName_);
}
{
int dataSize = 0;
for (int i = 0; i < argumentKeywords_.size(); i++) {
dataSize += computeStringSizeNoTag(argumentKeywords_.getRaw(i));
}
size += dataSize;
size += 1 * getArgumentKeywordsList().size();
}
if (allowedPositionalArguments_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeInt64Size(3, allowedPositionalArguments_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction other = (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) obj;
boolean result = true;
result = result && getConcreteFunctionName()
.equals(other.getConcreteFunctionName());
result = result && getArgumentKeywordsList()
.equals(other.getArgumentKeywordsList());
result = result && (getAllowedPositionalArguments()
== other.getAllowedPositionalArguments());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CONCRETE_FUNCTION_NAME_FIELD_NUMBER;
hash = (53 * hash) + getConcreteFunctionName().hashCode();
if (getArgumentKeywordsCount() > 0) {
hash = (37 * hash) + ARGUMENT_KEYWORDS_FIELD_NUMBER;
hash = (53 * hash) + getArgumentKeywordsList().hashCode();
}
hash = (37 * hash) + ALLOWED_POSITIONAL_ARGUMENTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getAllowedPositionalArguments());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.SavedBareConcreteFunction}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedBareConcreteFunction)
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunctionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedBareConcreteFunction_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedBareConcreteFunction_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.class, tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
concreteFunctionName_ = "";
argumentKeywords_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
allowedPositionalArguments_ = 0L;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedBareConcreteFunction_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction build() {
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction result = new tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
result.concreteFunctionName_ = concreteFunctionName_;
if (((bitField0_ & 0x00000002) == 0x00000002)) {
argumentKeywords_ = argumentKeywords_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000002);
}
result.argumentKeywords_ = argumentKeywords_;
result.allowedPositionalArguments_ = allowedPositionalArguments_;
result.bitField0_ = to_bitField0_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction.getDefaultInstance()) return this;
if (!other.getConcreteFunctionName().isEmpty()) {
concreteFunctionName_ = other.concreteFunctionName_;
onChanged();
}
if (!other.argumentKeywords_.isEmpty()) {
if (argumentKeywords_.isEmpty()) {
argumentKeywords_ = other.argumentKeywords_;
bitField0_ = (bitField0_ & ~0x00000002);
} else {
ensureArgumentKeywordsIsMutable();
argumentKeywords_.addAll(other.argumentKeywords_);
}
onChanged();
}
if (other.getAllowedPositionalArguments() != 0L) {
setAllowedPositionalArguments(other.getAllowedPositionalArguments());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object concreteFunctionName_ = "";
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
public java.lang.String getConcreteFunctionName() {
java.lang.Object ref = concreteFunctionName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
concreteFunctionName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
public com.google.protobuf.ByteString
getConcreteFunctionNameBytes() {
java.lang.Object ref = concreteFunctionName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
concreteFunctionName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
public Builder setConcreteFunctionName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
concreteFunctionName_ = value;
onChanged();
return this;
}
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
public Builder clearConcreteFunctionName() {
concreteFunctionName_ = getDefaultInstance().getConcreteFunctionName();
onChanged();
return this;
}
/**
*
* Identifies a SavedConcreteFunction.
*
*
* string concrete_function_name = 1;
*/
public Builder setConcreteFunctionNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
concreteFunctionName_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList argumentKeywords_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureArgumentKeywordsIsMutable() {
if (!((bitField0_ & 0x00000002) == 0x00000002)) {
argumentKeywords_ = new com.google.protobuf.LazyStringArrayList(argumentKeywords_);
bitField0_ |= 0x00000002;
}
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public com.google.protobuf.ProtocolStringList
getArgumentKeywordsList() {
return argumentKeywords_.getUnmodifiableView();
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public int getArgumentKeywordsCount() {
return argumentKeywords_.size();
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public java.lang.String getArgumentKeywords(int index) {
return argumentKeywords_.get(index);
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public com.google.protobuf.ByteString
getArgumentKeywordsBytes(int index) {
return argumentKeywords_.getByteString(index);
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public Builder setArgumentKeywords(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentKeywordsIsMutable();
argumentKeywords_.set(index, value);
onChanged();
return this;
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public Builder addArgumentKeywords(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureArgumentKeywordsIsMutable();
argumentKeywords_.add(value);
onChanged();
return this;
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public Builder addAllArgumentKeywords(
java.lang.Iterable values) {
ensureArgumentKeywordsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, argumentKeywords_);
onChanged();
return this;
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public Builder clearArgumentKeywords() {
argumentKeywords_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* A sequence of unique strings, one per Tensor argument.
*
*
* repeated string argument_keywords = 2;
*/
public Builder addArgumentKeywordsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureArgumentKeywordsIsMutable();
argumentKeywords_.add(value);
onChanged();
return this;
}
private long allowedPositionalArguments_ ;
/**
*
* The prefix of `argument_keywords` which may be identified by position.
*
*
* int64 allowed_positional_arguments = 3;
*/
public long getAllowedPositionalArguments() {
return allowedPositionalArguments_;
}
/**
*
* The prefix of `argument_keywords` which may be identified by position.
*
*
* int64 allowed_positional_arguments = 3;
*/
public Builder setAllowedPositionalArguments(long value) {
allowedPositionalArguments_ = value;
onChanged();
return this;
}
/**
*
* The prefix of `argument_keywords` which may be identified by position.
*
*
* int64 allowed_positional_arguments = 3;
*/
public Builder clearAllowedPositionalArguments() {
allowedPositionalArguments_ = 0L;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedBareConcreteFunction)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedBareConcreteFunction)
private static final tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedBareConcreteFunction parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedBareConcreteFunction(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedBareConcreteFunction getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedConstantOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedConstant)
com.google.protobuf.MessageOrBuilder {
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
java.lang.String getOperation();
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
com.google.protobuf.ByteString
getOperationBytes();
}
/**
* Protobuf type {@code tensorflow.SavedConstant}
*/
public static final class SavedConstant extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedConstant)
SavedConstantOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedConstant.newBuilder() to construct.
private SavedConstant(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedConstant() {
operation_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedConstant(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
operation_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConstant_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConstant_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedConstant.class, tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder.class);
}
public static final int OPERATION_FIELD_NUMBER = 1;
private volatile java.lang.Object operation_;
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
public java.lang.String getOperation() {
java.lang.Object ref = operation_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
operation_ = s;
return s;
}
}
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
public com.google.protobuf.ByteString
getOperationBytes() {
java.lang.Object ref = operation_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
operation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getOperationBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, operation_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getOperationBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, operation_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedConstant)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedConstant other = (tensorflow.SavedObjectGraphOuterClass.SavedConstant) obj;
boolean result = true;
result = result && getOperation()
.equals(other.getOperation());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + OPERATION_FIELD_NUMBER;
hash = (53 * hash) + getOperation().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedConstant prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code tensorflow.SavedConstant}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedConstant)
tensorflow.SavedObjectGraphOuterClass.SavedConstantOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConstant_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConstant_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedConstant.class, tensorflow.SavedObjectGraphOuterClass.SavedConstant.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedConstant.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
operation_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedConstant_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedConstant getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedConstant build() {
tensorflow.SavedObjectGraphOuterClass.SavedConstant result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedConstant buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedConstant result = new tensorflow.SavedObjectGraphOuterClass.SavedConstant(this);
result.operation_ = operation_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedConstant) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedConstant)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedConstant other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedConstant.getDefaultInstance()) return this;
if (!other.getOperation().isEmpty()) {
operation_ = other.operation_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedConstant parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedConstant) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object operation_ = "";
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
public java.lang.String getOperation() {
java.lang.Object ref = operation_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
operation_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
public com.google.protobuf.ByteString
getOperationBytes() {
java.lang.Object ref = operation_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
operation_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
public Builder setOperation(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
operation_ = value;
onChanged();
return this;
}
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
public Builder clearOperation() {
operation_ = getDefaultInstance().getOperation();
onChanged();
return this;
}
/**
*
* An Operation name for a ConstantOp in this SavedObjectGraph's MetaGraph.
*
*
* string operation = 1;
*/
public Builder setOperationBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
operation_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedConstant)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedConstant)
private static final tensorflow.SavedObjectGraphOuterClass.SavedConstant DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedConstant();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedConstant getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedConstant parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedConstant(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedConstant getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedVariableOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedVariable)
com.google.protobuf.MessageOrBuilder {
/**
* .tensorflow.DataType dtype = 1;
*/
int getDtypeValue();
/**
* .tensorflow.DataType dtype = 1;
*/
org.tensorflow.framework.DataType getDtype();
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
boolean hasShape();
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
org.tensorflow.framework.TensorShapeProto getShape();
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
org.tensorflow.framework.TensorShapeProtoOrBuilder getShapeOrBuilder();
/**
* bool trainable = 3;
*/
boolean getTrainable();
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
int getSynchronizationValue();
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
org.tensorflow.framework.VariableSynchronization getSynchronization();
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
int getAggregationValue();
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
org.tensorflow.framework.VariableAggregation getAggregation();
/**
* string name = 6;
*/
java.lang.String getName();
/**
* string name = 6;
*/
com.google.protobuf.ByteString
getNameBytes();
}
/**
*
* Represents a Variable that is initialized by loading the contents from the
* checkpoint.
*
*
* Protobuf type {@code tensorflow.SavedVariable}
*/
public static final class SavedVariable extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedVariable)
SavedVariableOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedVariable.newBuilder() to construct.
private SavedVariable(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedVariable() {
dtype_ = 0;
trainable_ = false;
synchronization_ = 0;
aggregation_ = 0;
name_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedVariable(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 8: {
int rawValue = input.readEnum();
dtype_ = rawValue;
break;
}
case 18: {
org.tensorflow.framework.TensorShapeProto.Builder subBuilder = null;
if (shape_ != null) {
subBuilder = shape_.toBuilder();
}
shape_ = input.readMessage(org.tensorflow.framework.TensorShapeProto.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(shape_);
shape_ = subBuilder.buildPartial();
}
break;
}
case 24: {
trainable_ = input.readBool();
break;
}
case 32: {
int rawValue = input.readEnum();
synchronization_ = rawValue;
break;
}
case 40: {
int rawValue = input.readEnum();
aggregation_ = rawValue;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedVariable_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedVariable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedVariable.class, tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder.class);
}
public static final int DTYPE_FIELD_NUMBER = 1;
private int dtype_;
/**
* .tensorflow.DataType dtype = 1;
*/
public int getDtypeValue() {
return dtype_;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public org.tensorflow.framework.DataType getDtype() {
org.tensorflow.framework.DataType result = org.tensorflow.framework.DataType.valueOf(dtype_);
return result == null ? org.tensorflow.framework.DataType.UNRECOGNIZED : result;
}
public static final int SHAPE_FIELD_NUMBER = 2;
private org.tensorflow.framework.TensorShapeProto shape_;
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public boolean hasShape() {
return shape_ != null;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProto getShape() {
return shape_ == null ? org.tensorflow.framework.TensorShapeProto.getDefaultInstance() : shape_;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProtoOrBuilder getShapeOrBuilder() {
return getShape();
}
public static final int TRAINABLE_FIELD_NUMBER = 3;
private boolean trainable_;
/**
* bool trainable = 3;
*/
public boolean getTrainable() {
return trainable_;
}
public static final int SYNCHRONIZATION_FIELD_NUMBER = 4;
private int synchronization_;
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
public int getSynchronizationValue() {
return synchronization_;
}
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
public org.tensorflow.framework.VariableSynchronization getSynchronization() {
org.tensorflow.framework.VariableSynchronization result = org.tensorflow.framework.VariableSynchronization.valueOf(synchronization_);
return result == null ? org.tensorflow.framework.VariableSynchronization.UNRECOGNIZED : result;
}
public static final int AGGREGATION_FIELD_NUMBER = 5;
private int aggregation_;
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
public int getAggregationValue() {
return aggregation_;
}
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
public org.tensorflow.framework.VariableAggregation getAggregation() {
org.tensorflow.framework.VariableAggregation result = org.tensorflow.framework.VariableAggregation.valueOf(aggregation_);
return result == null ? org.tensorflow.framework.VariableAggregation.UNRECOGNIZED : result;
}
public static final int NAME_FIELD_NUMBER = 6;
private volatile java.lang.Object name_;
/**
* string name = 6;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 6;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (dtype_ != org.tensorflow.framework.DataType.DT_INVALID.getNumber()) {
output.writeEnum(1, dtype_);
}
if (shape_ != null) {
output.writeMessage(2, getShape());
}
if (trainable_ != false) {
output.writeBool(3, trainable_);
}
if (synchronization_ != org.tensorflow.framework.VariableSynchronization.VARIABLE_SYNCHRONIZATION_AUTO.getNumber()) {
output.writeEnum(4, synchronization_);
}
if (aggregation_ != org.tensorflow.framework.VariableAggregation.VARIABLE_AGGREGATION_NONE.getNumber()) {
output.writeEnum(5, aggregation_);
}
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, name_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (dtype_ != org.tensorflow.framework.DataType.DT_INVALID.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, dtype_);
}
if (shape_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getShape());
}
if (trainable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, trainable_);
}
if (synchronization_ != org.tensorflow.framework.VariableSynchronization.VARIABLE_SYNCHRONIZATION_AUTO.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(4, synchronization_);
}
if (aggregation_ != org.tensorflow.framework.VariableAggregation.VARIABLE_AGGREGATION_NONE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(5, aggregation_);
}
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, name_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedVariable)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedVariable other = (tensorflow.SavedObjectGraphOuterClass.SavedVariable) obj;
boolean result = true;
result = result && dtype_ == other.dtype_;
result = result && (hasShape() == other.hasShape());
if (hasShape()) {
result = result && getShape()
.equals(other.getShape());
}
result = result && (getTrainable()
== other.getTrainable());
result = result && synchronization_ == other.synchronization_;
result = result && aggregation_ == other.aggregation_;
result = result && getName()
.equals(other.getName());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DTYPE_FIELD_NUMBER;
hash = (53 * hash) + dtype_;
if (hasShape()) {
hash = (37 * hash) + SHAPE_FIELD_NUMBER;
hash = (53 * hash) + getShape().hashCode();
}
hash = (37 * hash) + TRAINABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTrainable());
hash = (37 * hash) + SYNCHRONIZATION_FIELD_NUMBER;
hash = (53 * hash) + synchronization_;
hash = (37 * hash) + AGGREGATION_FIELD_NUMBER;
hash = (53 * hash) + aggregation_;
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedVariable prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents a Variable that is initialized by loading the contents from the
* checkpoint.
*
*
* Protobuf type {@code tensorflow.SavedVariable}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedVariable)
tensorflow.SavedObjectGraphOuterClass.SavedVariableOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedVariable_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedVariable_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedVariable.class, tensorflow.SavedObjectGraphOuterClass.SavedVariable.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedVariable.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
dtype_ = 0;
if (shapeBuilder_ == null) {
shape_ = null;
} else {
shape_ = null;
shapeBuilder_ = null;
}
trainable_ = false;
synchronization_ = 0;
aggregation_ = 0;
name_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedVariable_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedVariable getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedVariable build() {
tensorflow.SavedObjectGraphOuterClass.SavedVariable result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedVariable buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedVariable result = new tensorflow.SavedObjectGraphOuterClass.SavedVariable(this);
result.dtype_ = dtype_;
if (shapeBuilder_ == null) {
result.shape_ = shape_;
} else {
result.shape_ = shapeBuilder_.build();
}
result.trainable_ = trainable_;
result.synchronization_ = synchronization_;
result.aggregation_ = aggregation_;
result.name_ = name_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedVariable) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedVariable)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedVariable other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedVariable.getDefaultInstance()) return this;
if (other.dtype_ != 0) {
setDtypeValue(other.getDtypeValue());
}
if (other.hasShape()) {
mergeShape(other.getShape());
}
if (other.getTrainable() != false) {
setTrainable(other.getTrainable());
}
if (other.synchronization_ != 0) {
setSynchronizationValue(other.getSynchronizationValue());
}
if (other.aggregation_ != 0) {
setAggregationValue(other.getAggregationValue());
}
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedVariable parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedVariable) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int dtype_ = 0;
/**
* .tensorflow.DataType dtype = 1;
*/
public int getDtypeValue() {
return dtype_;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public Builder setDtypeValue(int value) {
dtype_ = value;
onChanged();
return this;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public org.tensorflow.framework.DataType getDtype() {
org.tensorflow.framework.DataType result = org.tensorflow.framework.DataType.valueOf(dtype_);
return result == null ? org.tensorflow.framework.DataType.UNRECOGNIZED : result;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public Builder setDtype(org.tensorflow.framework.DataType value) {
if (value == null) {
throw new NullPointerException();
}
dtype_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.DataType dtype = 1;
*/
public Builder clearDtype() {
dtype_ = 0;
onChanged();
return this;
}
private org.tensorflow.framework.TensorShapeProto shape_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.TensorShapeProto, org.tensorflow.framework.TensorShapeProto.Builder, org.tensorflow.framework.TensorShapeProtoOrBuilder> shapeBuilder_;
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public boolean hasShape() {
return shapeBuilder_ != null || shape_ != null;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProto getShape() {
if (shapeBuilder_ == null) {
return shape_ == null ? org.tensorflow.framework.TensorShapeProto.getDefaultInstance() : shape_;
} else {
return shapeBuilder_.getMessage();
}
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder setShape(org.tensorflow.framework.TensorShapeProto value) {
if (shapeBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
shape_ = value;
onChanged();
} else {
shapeBuilder_.setMessage(value);
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder setShape(
org.tensorflow.framework.TensorShapeProto.Builder builderForValue) {
if (shapeBuilder_ == null) {
shape_ = builderForValue.build();
onChanged();
} else {
shapeBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder mergeShape(org.tensorflow.framework.TensorShapeProto value) {
if (shapeBuilder_ == null) {
if (shape_ != null) {
shape_ =
org.tensorflow.framework.TensorShapeProto.newBuilder(shape_).mergeFrom(value).buildPartial();
} else {
shape_ = value;
}
onChanged();
} else {
shapeBuilder_.mergeFrom(value);
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public Builder clearShape() {
if (shapeBuilder_ == null) {
shape_ = null;
onChanged();
} else {
shape_ = null;
shapeBuilder_ = null;
}
return this;
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProto.Builder getShapeBuilder() {
onChanged();
return getShapeFieldBuilder().getBuilder();
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
public org.tensorflow.framework.TensorShapeProtoOrBuilder getShapeOrBuilder() {
if (shapeBuilder_ != null) {
return shapeBuilder_.getMessageOrBuilder();
} else {
return shape_ == null ?
org.tensorflow.framework.TensorShapeProto.getDefaultInstance() : shape_;
}
}
/**
* .tensorflow.TensorShapeProto shape = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.TensorShapeProto, org.tensorflow.framework.TensorShapeProto.Builder, org.tensorflow.framework.TensorShapeProtoOrBuilder>
getShapeFieldBuilder() {
if (shapeBuilder_ == null) {
shapeBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
org.tensorflow.framework.TensorShapeProto, org.tensorflow.framework.TensorShapeProto.Builder, org.tensorflow.framework.TensorShapeProtoOrBuilder>(
getShape(),
getParentForChildren(),
isClean());
shape_ = null;
}
return shapeBuilder_;
}
private boolean trainable_ ;
/**
* bool trainable = 3;
*/
public boolean getTrainable() {
return trainable_;
}
/**
* bool trainable = 3;
*/
public Builder setTrainable(boolean value) {
trainable_ = value;
onChanged();
return this;
}
/**
* bool trainable = 3;
*/
public Builder clearTrainable() {
trainable_ = false;
onChanged();
return this;
}
private int synchronization_ = 0;
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
public int getSynchronizationValue() {
return synchronization_;
}
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
public Builder setSynchronizationValue(int value) {
synchronization_ = value;
onChanged();
return this;
}
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
public org.tensorflow.framework.VariableSynchronization getSynchronization() {
org.tensorflow.framework.VariableSynchronization result = org.tensorflow.framework.VariableSynchronization.valueOf(synchronization_);
return result == null ? org.tensorflow.framework.VariableSynchronization.UNRECOGNIZED : result;
}
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
public Builder setSynchronization(org.tensorflow.framework.VariableSynchronization value) {
if (value == null) {
throw new NullPointerException();
}
synchronization_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.VariableSynchronization synchronization = 4;
*/
public Builder clearSynchronization() {
synchronization_ = 0;
onChanged();
return this;
}
private int aggregation_ = 0;
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
public int getAggregationValue() {
return aggregation_;
}
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
public Builder setAggregationValue(int value) {
aggregation_ = value;
onChanged();
return this;
}
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
public org.tensorflow.framework.VariableAggregation getAggregation() {
org.tensorflow.framework.VariableAggregation result = org.tensorflow.framework.VariableAggregation.valueOf(aggregation_);
return result == null ? org.tensorflow.framework.VariableAggregation.UNRECOGNIZED : result;
}
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
public Builder setAggregation(org.tensorflow.framework.VariableAggregation value) {
if (value == null) {
throw new NullPointerException();
}
aggregation_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.VariableAggregation aggregation = 5;
*/
public Builder clearAggregation() {
aggregation_ = 0;
onChanged();
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 6;
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 6;
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 6;
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 6;
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 6;
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedVariable)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedVariable)
private static final tensorflow.SavedObjectGraphOuterClass.SavedVariable DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedVariable();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedVariable getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedVariable parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedVariable(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedVariable getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface FunctionSpecOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.FunctionSpec)
com.google.protobuf.MessageOrBuilder {
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
boolean hasFullargspec();
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
tensorflow.Struct.StructuredValue getFullargspec();
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
tensorflow.Struct.StructuredValueOrBuilder getFullargspecOrBuilder();
/**
*
* Whether this represents a class method.
*
*
* bool is_method = 2;
*/
boolean getIsMethod();
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
boolean hasInputSignature();
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
tensorflow.Struct.StructuredValue getInputSignature();
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
tensorflow.Struct.StructuredValueOrBuilder getInputSignatureOrBuilder();
}
/**
*
* Represents `FunctionSpec` used in `Function`. This represents a
* function that has been wrapped as a TensorFlow `Function`.
*
*
* Protobuf type {@code tensorflow.FunctionSpec}
*/
public static final class FunctionSpec extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.FunctionSpec)
FunctionSpecOrBuilder {
private static final long serialVersionUID = 0L;
// Use FunctionSpec.newBuilder() to construct.
private FunctionSpec(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private FunctionSpec() {
isMethod_ = false;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private FunctionSpec(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
tensorflow.Struct.StructuredValue.Builder subBuilder = null;
if (fullargspec_ != null) {
subBuilder = fullargspec_.toBuilder();
}
fullargspec_ = input.readMessage(tensorflow.Struct.StructuredValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(fullargspec_);
fullargspec_ = subBuilder.buildPartial();
}
break;
}
case 16: {
isMethod_ = input.readBool();
break;
}
case 42: {
tensorflow.Struct.StructuredValue.Builder subBuilder = null;
if (inputSignature_ != null) {
subBuilder = inputSignature_.toBuilder();
}
inputSignature_ = input.readMessage(tensorflow.Struct.StructuredValue.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(inputSignature_);
inputSignature_ = subBuilder.buildPartial();
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_FunctionSpec_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_FunctionSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.FunctionSpec.class, tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder.class);
}
public static final int FULLARGSPEC_FIELD_NUMBER = 1;
private tensorflow.Struct.StructuredValue fullargspec_;
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public boolean hasFullargspec() {
return fullargspec_ != null;
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public tensorflow.Struct.StructuredValue getFullargspec() {
return fullargspec_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : fullargspec_;
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public tensorflow.Struct.StructuredValueOrBuilder getFullargspecOrBuilder() {
return getFullargspec();
}
public static final int IS_METHOD_FIELD_NUMBER = 2;
private boolean isMethod_;
/**
*
* Whether this represents a class method.
*
*
* bool is_method = 2;
*/
public boolean getIsMethod() {
return isMethod_;
}
public static final int INPUT_SIGNATURE_FIELD_NUMBER = 5;
private tensorflow.Struct.StructuredValue inputSignature_;
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public boolean hasInputSignature() {
return inputSignature_ != null;
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public tensorflow.Struct.StructuredValue getInputSignature() {
return inputSignature_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : inputSignature_;
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public tensorflow.Struct.StructuredValueOrBuilder getInputSignatureOrBuilder() {
return getInputSignature();
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (fullargspec_ != null) {
output.writeMessage(1, getFullargspec());
}
if (isMethod_ != false) {
output.writeBool(2, isMethod_);
}
if (inputSignature_ != null) {
output.writeMessage(5, getInputSignature());
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (fullargspec_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, getFullargspec());
}
if (isMethod_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, isMethod_);
}
if (inputSignature_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, getInputSignature());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.FunctionSpec)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.FunctionSpec other = (tensorflow.SavedObjectGraphOuterClass.FunctionSpec) obj;
boolean result = true;
result = result && (hasFullargspec() == other.hasFullargspec());
if (hasFullargspec()) {
result = result && getFullargspec()
.equals(other.getFullargspec());
}
result = result && (getIsMethod()
== other.getIsMethod());
result = result && (hasInputSignature() == other.hasInputSignature());
if (hasInputSignature()) {
result = result && getInputSignature()
.equals(other.getInputSignature());
}
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (hasFullargspec()) {
hash = (37 * hash) + FULLARGSPEC_FIELD_NUMBER;
hash = (53 * hash) + getFullargspec().hashCode();
}
hash = (37 * hash) + IS_METHOD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsMethod());
if (hasInputSignature()) {
hash = (37 * hash) + INPUT_SIGNATURE_FIELD_NUMBER;
hash = (53 * hash) + getInputSignature().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.FunctionSpec prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Represents `FunctionSpec` used in `Function`. This represents a
* function that has been wrapped as a TensorFlow `Function`.
*
*
* Protobuf type {@code tensorflow.FunctionSpec}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.FunctionSpec)
tensorflow.SavedObjectGraphOuterClass.FunctionSpecOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_FunctionSpec_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_FunctionSpec_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.FunctionSpec.class, tensorflow.SavedObjectGraphOuterClass.FunctionSpec.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.FunctionSpec.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
if (fullargspecBuilder_ == null) {
fullargspec_ = null;
} else {
fullargspec_ = null;
fullargspecBuilder_ = null;
}
isMethod_ = false;
if (inputSignatureBuilder_ == null) {
inputSignature_ = null;
} else {
inputSignature_ = null;
inputSignatureBuilder_ = null;
}
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_FunctionSpec_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.FunctionSpec getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.FunctionSpec.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.FunctionSpec build() {
tensorflow.SavedObjectGraphOuterClass.FunctionSpec result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.FunctionSpec buildPartial() {
tensorflow.SavedObjectGraphOuterClass.FunctionSpec result = new tensorflow.SavedObjectGraphOuterClass.FunctionSpec(this);
if (fullargspecBuilder_ == null) {
result.fullargspec_ = fullargspec_;
} else {
result.fullargspec_ = fullargspecBuilder_.build();
}
result.isMethod_ = isMethod_;
if (inputSignatureBuilder_ == null) {
result.inputSignature_ = inputSignature_;
} else {
result.inputSignature_ = inputSignatureBuilder_.build();
}
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.FunctionSpec) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.FunctionSpec)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.FunctionSpec other) {
if (other == tensorflow.SavedObjectGraphOuterClass.FunctionSpec.getDefaultInstance()) return this;
if (other.hasFullargspec()) {
mergeFullargspec(other.getFullargspec());
}
if (other.getIsMethod() != false) {
setIsMethod(other.getIsMethod());
}
if (other.hasInputSignature()) {
mergeInputSignature(other.getInputSignature());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.FunctionSpec parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.FunctionSpec) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private tensorflow.Struct.StructuredValue fullargspec_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder> fullargspecBuilder_;
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public boolean hasFullargspec() {
return fullargspecBuilder_ != null || fullargspec_ != null;
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public tensorflow.Struct.StructuredValue getFullargspec() {
if (fullargspecBuilder_ == null) {
return fullargspec_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : fullargspec_;
} else {
return fullargspecBuilder_.getMessage();
}
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public Builder setFullargspec(tensorflow.Struct.StructuredValue value) {
if (fullargspecBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
fullargspec_ = value;
onChanged();
} else {
fullargspecBuilder_.setMessage(value);
}
return this;
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public Builder setFullargspec(
tensorflow.Struct.StructuredValue.Builder builderForValue) {
if (fullargspecBuilder_ == null) {
fullargspec_ = builderForValue.build();
onChanged();
} else {
fullargspecBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public Builder mergeFullargspec(tensorflow.Struct.StructuredValue value) {
if (fullargspecBuilder_ == null) {
if (fullargspec_ != null) {
fullargspec_ =
tensorflow.Struct.StructuredValue.newBuilder(fullargspec_).mergeFrom(value).buildPartial();
} else {
fullargspec_ = value;
}
onChanged();
} else {
fullargspecBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public Builder clearFullargspec() {
if (fullargspecBuilder_ == null) {
fullargspec_ = null;
onChanged();
} else {
fullargspec_ = null;
fullargspecBuilder_ = null;
}
return this;
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public tensorflow.Struct.StructuredValue.Builder getFullargspecBuilder() {
onChanged();
return getFullargspecFieldBuilder().getBuilder();
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
public tensorflow.Struct.StructuredValueOrBuilder getFullargspecOrBuilder() {
if (fullargspecBuilder_ != null) {
return fullargspecBuilder_.getMessageOrBuilder();
} else {
return fullargspec_ == null ?
tensorflow.Struct.StructuredValue.getDefaultInstance() : fullargspec_;
}
}
/**
*
* Full arg spec from inspect.getfullargspec().
*
*
* .tensorflow.StructuredValue fullargspec = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>
getFullargspecFieldBuilder() {
if (fullargspecBuilder_ == null) {
fullargspecBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>(
getFullargspec(),
getParentForChildren(),
isClean());
fullargspec_ = null;
}
return fullargspecBuilder_;
}
private boolean isMethod_ ;
/**
*
* Whether this represents a class method.
*
*
* bool is_method = 2;
*/
public boolean getIsMethod() {
return isMethod_;
}
/**
*
* Whether this represents a class method.
*
*
* bool is_method = 2;
*/
public Builder setIsMethod(boolean value) {
isMethod_ = value;
onChanged();
return this;
}
/**
*
* Whether this represents a class method.
*
*
* bool is_method = 2;
*/
public Builder clearIsMethod() {
isMethod_ = false;
onChanged();
return this;
}
private tensorflow.Struct.StructuredValue inputSignature_ = null;
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder> inputSignatureBuilder_;
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public boolean hasInputSignature() {
return inputSignatureBuilder_ != null || inputSignature_ != null;
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public tensorflow.Struct.StructuredValue getInputSignature() {
if (inputSignatureBuilder_ == null) {
return inputSignature_ == null ? tensorflow.Struct.StructuredValue.getDefaultInstance() : inputSignature_;
} else {
return inputSignatureBuilder_.getMessage();
}
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public Builder setInputSignature(tensorflow.Struct.StructuredValue value) {
if (inputSignatureBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
inputSignature_ = value;
onChanged();
} else {
inputSignatureBuilder_.setMessage(value);
}
return this;
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public Builder setInputSignature(
tensorflow.Struct.StructuredValue.Builder builderForValue) {
if (inputSignatureBuilder_ == null) {
inputSignature_ = builderForValue.build();
onChanged();
} else {
inputSignatureBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public Builder mergeInputSignature(tensorflow.Struct.StructuredValue value) {
if (inputSignatureBuilder_ == null) {
if (inputSignature_ != null) {
inputSignature_ =
tensorflow.Struct.StructuredValue.newBuilder(inputSignature_).mergeFrom(value).buildPartial();
} else {
inputSignature_ = value;
}
onChanged();
} else {
inputSignatureBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public Builder clearInputSignature() {
if (inputSignatureBuilder_ == null) {
inputSignature_ = null;
onChanged();
} else {
inputSignature_ = null;
inputSignatureBuilder_ = null;
}
return this;
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public tensorflow.Struct.StructuredValue.Builder getInputSignatureBuilder() {
onChanged();
return getInputSignatureFieldBuilder().getBuilder();
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
public tensorflow.Struct.StructuredValueOrBuilder getInputSignatureOrBuilder() {
if (inputSignatureBuilder_ != null) {
return inputSignatureBuilder_.getMessageOrBuilder();
} else {
return inputSignature_ == null ?
tensorflow.Struct.StructuredValue.getDefaultInstance() : inputSignature_;
}
}
/**
*
* The input signature, if specified.
*
*
* .tensorflow.StructuredValue input_signature = 5;
*/
private com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>
getInputSignatureFieldBuilder() {
if (inputSignatureBuilder_ == null) {
inputSignatureBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
tensorflow.Struct.StructuredValue, tensorflow.Struct.StructuredValue.Builder, tensorflow.Struct.StructuredValueOrBuilder>(
getInputSignature(),
getParentForChildren(),
isClean());
inputSignature_ = null;
}
return inputSignatureBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.FunctionSpec)
}
// @@protoc_insertion_point(class_scope:tensorflow.FunctionSpec)
private static final tensorflow.SavedObjectGraphOuterClass.FunctionSpec DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.FunctionSpec();
}
public static tensorflow.SavedObjectGraphOuterClass.FunctionSpec getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public FunctionSpec parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new FunctionSpec(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.FunctionSpec getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SavedResourceOrBuilder extends
// @@protoc_insertion_point(interface_extends:tensorflow.SavedResource)
com.google.protobuf.MessageOrBuilder {
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
java.lang.String getDevice();
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
com.google.protobuf.ByteString
getDeviceBytes();
}
/**
*
* A SavedResource represents a TF object that holds state during its lifetime.
* An object of this type can have a reference to a:
* create_resource() and an initialize() function.
*
*
* Protobuf type {@code tensorflow.SavedResource}
*/
public static final class SavedResource extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SavedResource)
SavedResourceOrBuilder {
private static final long serialVersionUID = 0L;
// Use SavedResource.newBuilder() to construct.
private SavedResource(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SavedResource() {
device_ = "";
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private SavedResource(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!parseUnknownFieldProto3(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
device_ = s;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedResource_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedResource_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedResource.class, tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder.class);
}
public static final int DEVICE_FIELD_NUMBER = 1;
private volatile java.lang.Object device_;
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
public java.lang.String getDevice() {
java.lang.Object ref = device_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
device_ = s;
return s;
}
}
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
public com.google.protobuf.ByteString
getDeviceBytes() {
java.lang.Object ref = device_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
device_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getDeviceBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, device_);
}
unknownFields.writeTo(output);
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getDeviceBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, device_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof tensorflow.SavedObjectGraphOuterClass.SavedResource)) {
return super.equals(obj);
}
tensorflow.SavedObjectGraphOuterClass.SavedResource other = (tensorflow.SavedObjectGraphOuterClass.SavedResource) obj;
boolean result = true;
result = result && getDevice()
.equals(other.getDevice());
result = result && unknownFields.equals(other.unknownFields);
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DEVICE_FIELD_NUMBER;
hash = (53 * hash) + getDevice().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(tensorflow.SavedObjectGraphOuterClass.SavedResource prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* A SavedResource represents a TF object that holds state during its lifetime.
* An object of this type can have a reference to a:
* create_resource() and an initialize() function.
*
*
* Protobuf type {@code tensorflow.SavedResource}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SavedResource)
tensorflow.SavedObjectGraphOuterClass.SavedResourceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedResource_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedResource_fieldAccessorTable
.ensureFieldAccessorsInitialized(
tensorflow.SavedObjectGraphOuterClass.SavedResource.class, tensorflow.SavedObjectGraphOuterClass.SavedResource.Builder.class);
}
// Construct using tensorflow.SavedObjectGraphOuterClass.SavedResource.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
device_ = "";
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return tensorflow.SavedObjectGraphOuterClass.internal_static_tensorflow_SavedResource_descriptor;
}
public tensorflow.SavedObjectGraphOuterClass.SavedResource getDefaultInstanceForType() {
return tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance();
}
public tensorflow.SavedObjectGraphOuterClass.SavedResource build() {
tensorflow.SavedObjectGraphOuterClass.SavedResource result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public tensorflow.SavedObjectGraphOuterClass.SavedResource buildPartial() {
tensorflow.SavedObjectGraphOuterClass.SavedResource result = new tensorflow.SavedObjectGraphOuterClass.SavedResource(this);
result.device_ = device_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof tensorflow.SavedObjectGraphOuterClass.SavedResource) {
return mergeFrom((tensorflow.SavedObjectGraphOuterClass.SavedResource)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(tensorflow.SavedObjectGraphOuterClass.SavedResource other) {
if (other == tensorflow.SavedObjectGraphOuterClass.SavedResource.getDefaultInstance()) return this;
if (!other.getDevice().isEmpty()) {
device_ = other.device_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
tensorflow.SavedObjectGraphOuterClass.SavedResource parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (tensorflow.SavedObjectGraphOuterClass.SavedResource) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object device_ = "";
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
public java.lang.String getDevice() {
java.lang.Object ref = device_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
device_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
public com.google.protobuf.ByteString
getDeviceBytes() {
java.lang.Object ref = device_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
device_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
public Builder setDevice(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
device_ = value;
onChanged();
return this;
}
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
public Builder clearDevice() {
device_ = getDefaultInstance().getDevice();
onChanged();
return this;
}
/**
*
* A device specification indicating a required placement for the resource
* creation function, e.g. "CPU". An empty string allows the user to select a
* device.
*
*
* string device = 1;
*/
public Builder setDeviceBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
device_ = value;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFieldsProto3(unknownFields);
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:tensorflow.SavedResource)
}
// @@protoc_insertion_point(class_scope:tensorflow.SavedResource)
private static final tensorflow.SavedObjectGraphOuterClass.SavedResource DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new tensorflow.SavedObjectGraphOuterClass.SavedResource();
}
public static tensorflow.SavedObjectGraphOuterClass.SavedResource getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SavedResource parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SavedResource(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public tensorflow.SavedObjectGraphOuterClass.SavedResource getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedObjectGraph_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedObjectGraph_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedObjectGraph_ConcreteFunctionsEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedObjectGraph_ConcreteFunctionsEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedObject_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedObject_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedUserObject_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedUserObject_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedAsset_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedAsset_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedFunction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedFunction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedConcreteFunction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedConcreteFunction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedBareConcreteFunction_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedBareConcreteFunction_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedConstant_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedConstant_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedVariable_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedVariable_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_FunctionSpec_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_FunctionSpec_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_tensorflow_SavedResource_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_tensorflow_SavedResource_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n1tensorflow/core/protobuf/saved_object_" +
"graph.proto\022\ntensorflow\0325tensorflow/core" +
"/protobuf/trackable_object_graph.proto\032%" +
"tensorflow/core/protobuf/struct.proto\032,t" +
"ensorflow/core/framework/tensor_shape.pr" +
"oto\032%tensorflow/core/framework/types.pro" +
"to\032(tensorflow/core/framework/versions.p" +
"roto\032(tensorflow/core/framework/variable" +
".proto\"\350\001\n\020SavedObjectGraph\022&\n\005nodes\030\001 \003" +
"(\0132\027.tensorflow.SavedObject\022O\n\022concrete_" +
"functions\030\002 \003(\01323.tensorflow.SavedObject" +
"Graph.ConcreteFunctionsEntry\032[\n\026Concrete" +
"FunctionsEntry\022\013\n\003key\030\001 \001(\t\0220\n\005value\030\002 \001" +
"(\0132!.tensorflow.SavedConcreteFunction:\0028" +
"\001\"\275\004\n\013SavedObject\022R\n\010children\030\001 \003(\[email protected]" +
"nsorflow.TrackableObjectGraph.TrackableO" +
"bject.ObjectReference\022^\n\016slot_variables\030" +
"\003 \003(\0132F.tensorflow.TrackableObjectGraph." +
"TrackableObject.SlotVariableReference\0222\n" +
"\013user_object\030\004 \001(\0132\033.tensorflow.SavedUse" +
"rObjectH\000\022\'\n\005asset\030\005 \001(\0132\026.tensorflow.Sa" +
"vedAssetH\000\022-\n\010function\030\006 \001(\0132\031.tensorflo" +
"w.SavedFunctionH\000\022-\n\010variable\030\007 \001(\0132\031.te" +
"nsorflow.SavedVariableH\000\022G\n\026bare_concret" +
"e_function\030\010 \001(\0132%.tensorflow.SavedBareC" +
"oncreteFunctionH\000\022-\n\010constant\030\t \001(\0132\031.te" +
"nsorflow.SavedConstantH\000\022-\n\010resource\030\n \001" +
"(\0132\031.tensorflow.SavedResourceH\000B\006\n\004kindJ" +
"\004\010\002\020\003R\nattributes\"`\n\017SavedUserObject\022\022\n\n" +
"identifier\030\001 \001(\t\022\'\n\007version\030\002 \001(\0132\026.tens" +
"orflow.VersionDef\022\020\n\010metadata\030\003 \001(\t\"*\n\nS" +
"avedAsset\022\034\n\024asset_file_def_index\030\001 \001(\005\"" +
"\\\n\rSavedFunction\022\032\n\022concrete_functions\030\001" +
" \003(\t\022/\n\rfunction_spec\030\002 \001(\0132\030.tensorflow" +
".FunctionSpec\"\250\001\n\025SavedConcreteFunction\022" +
"\024\n\014bound_inputs\030\002 \003(\005\022B\n\035canonicalized_i" +
"nput_signature\030\003 \001(\0132\033.tensorflow.Struct" +
"uredValue\0225\n\020output_signature\030\004 \001(\0132\033.te" +
"nsorflow.StructuredValue\"|\n\031SavedBareCon" +
"creteFunction\022\036\n\026concrete_function_name\030" +
"\001 \001(\t\022\031\n\021argument_keywords\030\002 \003(\t\022$\n\034allo" +
"wed_positional_arguments\030\003 \001(\003\"\"\n\rSavedC" +
"onstant\022\021\n\toperation\030\001 \001(\t\"\366\001\n\rSavedVari" +
"able\022#\n\005dtype\030\001 \001(\0162\024.tensorflow.DataTyp" +
"e\022+\n\005shape\030\002 \001(\0132\034.tensorflow.TensorShap" +
"eProto\022\021\n\ttrainable\030\003 \001(\010\022<\n\017synchroniza" +
"tion\030\004 \001(\0162#.tensorflow.VariableSynchron" +
"ization\0224\n\013aggregation\030\005 \001(\0162\037.tensorflo" +
"w.VariableAggregation\022\014\n\004name\030\006 \001(\t\"\225\001\n\014" +
"FunctionSpec\0220\n\013fullargspec\030\001 \001(\0132\033.tens" +
"orflow.StructuredValue\022\021\n\tis_method\030\002 \001(" +
"\010\0224\n\017input_signature\030\005 \001(\0132\033.tensorflow." +
"StructuredValueJ\004\010\003\020\004J\004\010\004\020\005\"\037\n\rSavedReso" +
"urce\022\016\n\006device\030\001 \001(\tB\003\370\001\001b\006proto3"
};
com.google.protobuf.Descriptors.FileDescriptor.InternalDescriptorAssigner assigner =
new com.google.protobuf.Descriptors.FileDescriptor. InternalDescriptorAssigner() {
public com.google.protobuf.ExtensionRegistry assignDescriptors(
com.google.protobuf.Descriptors.FileDescriptor root) {
descriptor = root;
return null;
}
};
com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
tensorflow.TrackableObjectGraphOuterClass.getDescriptor(),
tensorflow.Struct.getDescriptor(),
org.tensorflow.framework.TensorShapeProtos.getDescriptor(),
org.tensorflow.framework.TypesProtos.getDescriptor(),
org.tensorflow.framework.VersionsProtos.getDescriptor(),
org.tensorflow.framework.VariableProtos.getDescriptor(),
}, assigner);
internal_static_tensorflow_SavedObjectGraph_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_tensorflow_SavedObjectGraph_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedObjectGraph_descriptor,
new java.lang.String[] { "Nodes", "ConcreteFunctions", });
internal_static_tensorflow_SavedObjectGraph_ConcreteFunctionsEntry_descriptor =
internal_static_tensorflow_SavedObjectGraph_descriptor.getNestedTypes().get(0);
internal_static_tensorflow_SavedObjectGraph_ConcreteFunctionsEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedObjectGraph_ConcreteFunctionsEntry_descriptor,
new java.lang.String[] { "Key", "Value", });
internal_static_tensorflow_SavedObject_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_tensorflow_SavedObject_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedObject_descriptor,
new java.lang.String[] { "Children", "SlotVariables", "UserObject", "Asset", "Function", "Variable", "BareConcreteFunction", "Constant", "Resource", "Kind", });
internal_static_tensorflow_SavedUserObject_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_tensorflow_SavedUserObject_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedUserObject_descriptor,
new java.lang.String[] { "Identifier", "Version", "Metadata", });
internal_static_tensorflow_SavedAsset_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_tensorflow_SavedAsset_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedAsset_descriptor,
new java.lang.String[] { "AssetFileDefIndex", });
internal_static_tensorflow_SavedFunction_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_tensorflow_SavedFunction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedFunction_descriptor,
new java.lang.String[] { "ConcreteFunctions", "FunctionSpec", });
internal_static_tensorflow_SavedConcreteFunction_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_tensorflow_SavedConcreteFunction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedConcreteFunction_descriptor,
new java.lang.String[] { "BoundInputs", "CanonicalizedInputSignature", "OutputSignature", });
internal_static_tensorflow_SavedBareConcreteFunction_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_tensorflow_SavedBareConcreteFunction_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedBareConcreteFunction_descriptor,
new java.lang.String[] { "ConcreteFunctionName", "ArgumentKeywords", "AllowedPositionalArguments", });
internal_static_tensorflow_SavedConstant_descriptor =
getDescriptor().getMessageTypes().get(7);
internal_static_tensorflow_SavedConstant_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedConstant_descriptor,
new java.lang.String[] { "Operation", });
internal_static_tensorflow_SavedVariable_descriptor =
getDescriptor().getMessageTypes().get(8);
internal_static_tensorflow_SavedVariable_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedVariable_descriptor,
new java.lang.String[] { "Dtype", "Shape", "Trainable", "Synchronization", "Aggregation", "Name", });
internal_static_tensorflow_FunctionSpec_descriptor =
getDescriptor().getMessageTypes().get(9);
internal_static_tensorflow_FunctionSpec_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_FunctionSpec_descriptor,
new java.lang.String[] { "Fullargspec", "IsMethod", "InputSignature", });
internal_static_tensorflow_SavedResource_descriptor =
getDescriptor().getMessageTypes().get(10);
internal_static_tensorflow_SavedResource_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_tensorflow_SavedResource_descriptor,
new java.lang.String[] { "Device", });
tensorflow.TrackableObjectGraphOuterClass.getDescriptor();
tensorflow.Struct.getDescriptor();
org.tensorflow.framework.TensorShapeProtos.getDescriptor();
org.tensorflow.framework.TypesProtos.getDescriptor();
org.tensorflow.framework.VersionsProtos.getDescriptor();
org.tensorflow.framework.VariableProtos.getDescriptor();
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy