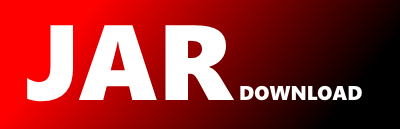
org.tensorflow.util.SaverDef Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proto Show documentation
Show all versions of proto Show documentation
Java API for TensorFlow protocol buffers.
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: tensorflow/core/protobuf/saver.proto
package org.tensorflow.util;
/**
*
* Protocol buffer representing the configuration of a Saver.
*
*
* Protobuf type {@code tensorflow.SaverDef}
*/
public final class SaverDef extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:tensorflow.SaverDef)
SaverDefOrBuilder {
// Use SaverDef.newBuilder() to construct.
private SaverDef(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private SaverDef() {
filenameTensorName_ = "";
saveTensorName_ = "";
restoreOpName_ = "";
maxToKeep_ = 0;
sharded_ = false;
keepCheckpointEveryNHours_ = 0F;
version_ = 0;
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private SaverDef(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 10: {
java.lang.String s = input.readStringRequireUtf8();
filenameTensorName_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
saveTensorName_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
restoreOpName_ = s;
break;
}
case 32: {
maxToKeep_ = input.readInt32();
break;
}
case 40: {
sharded_ = input.readBool();
break;
}
case 53: {
keepCheckpointEveryNHours_ = input.readFloat();
break;
}
case 56: {
int rawValue = input.readEnum();
version_ = rawValue;
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.util.SaverProtos.internal_static_tensorflow_SaverDef_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.util.SaverProtos.internal_static_tensorflow_SaverDef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.util.SaverDef.class, org.tensorflow.util.SaverDef.Builder.class);
}
/**
*
* A version number that identifies a different on-disk checkpoint format.
* Usually, each subclass of BaseSaverBuilder works with a particular
* version/format. However, it is possible that the same builder may be
* upgraded to support a newer checkpoint format in the future.
*
*
* Protobuf enum {@code tensorflow.SaverDef.CheckpointFormatVersion}
*/
public enum CheckpointFormatVersion
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Internal legacy format.
*
*
* LEGACY = 0;
*/
LEGACY(0),
/**
*
* Current format: tf.Saver() which works with tensorflow::table::Table.
*
*
* V1 = 1;
*/
V1(1),
/**
*
* Experimental format under development.
*
*
* V2 = 2;
*/
V2(2),
UNRECOGNIZED(-1),
;
/**
*
* Internal legacy format.
*
*
* LEGACY = 0;
*/
public static final int LEGACY_VALUE = 0;
/**
*
* Current format: tf.Saver() which works with tensorflow::table::Table.
*
*
* V1 = 1;
*/
public static final int V1_VALUE = 1;
/**
*
* Experimental format under development.
*
*
* V2 = 2;
*/
public static final int V2_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CheckpointFormatVersion valueOf(int value) {
return forNumber(value);
}
public static CheckpointFormatVersion forNumber(int value) {
switch (value) {
case 0: return LEGACY;
case 1: return V1;
case 2: return V2;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
CheckpointFormatVersion> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public CheckpointFormatVersion findValueByNumber(int number) {
return CheckpointFormatVersion.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.tensorflow.util.SaverDef.getDescriptor().getEnumTypes().get(0);
}
private static final CheckpointFormatVersion[] VALUES = values();
public static CheckpointFormatVersion valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private CheckpointFormatVersion(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:tensorflow.SaverDef.CheckpointFormatVersion)
}
public static final int FILENAME_TENSOR_NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object filenameTensorName_;
/**
*
* The name of the tensor in which to specify the filename when saving or
* restoring a model checkpoint.
*
*
* string filename_tensor_name = 1;
*/
public java.lang.String getFilenameTensorName() {
java.lang.Object ref = filenameTensorName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filenameTensorName_ = s;
return s;
}
}
/**
*
* The name of the tensor in which to specify the filename when saving or
* restoring a model checkpoint.
*
*
* string filename_tensor_name = 1;
*/
public com.google.protobuf.ByteString
getFilenameTensorNameBytes() {
java.lang.Object ref = filenameTensorName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filenameTensorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SAVE_TENSOR_NAME_FIELD_NUMBER = 2;
private volatile java.lang.Object saveTensorName_;
/**
*
* The operation to run when saving a model checkpoint.
*
*
* string save_tensor_name = 2;
*/
public java.lang.String getSaveTensorName() {
java.lang.Object ref = saveTensorName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
saveTensorName_ = s;
return s;
}
}
/**
*
* The operation to run when saving a model checkpoint.
*
*
* string save_tensor_name = 2;
*/
public com.google.protobuf.ByteString
getSaveTensorNameBytes() {
java.lang.Object ref = saveTensorName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
saveTensorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int RESTORE_OP_NAME_FIELD_NUMBER = 3;
private volatile java.lang.Object restoreOpName_;
/**
*
* The operation to run when restoring a model checkpoint.
*
*
* string restore_op_name = 3;
*/
public java.lang.String getRestoreOpName() {
java.lang.Object ref = restoreOpName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
restoreOpName_ = s;
return s;
}
}
/**
*
* The operation to run when restoring a model checkpoint.
*
*
* string restore_op_name = 3;
*/
public com.google.protobuf.ByteString
getRestoreOpNameBytes() {
java.lang.Object ref = restoreOpName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
restoreOpName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MAX_TO_KEEP_FIELD_NUMBER = 4;
private int maxToKeep_;
/**
*
* Maximum number of checkpoints to keep. If 0, no checkpoints are deleted.
*
*
* int32 max_to_keep = 4;
*/
public int getMaxToKeep() {
return maxToKeep_;
}
public static final int SHARDED_FIELD_NUMBER = 5;
private boolean sharded_;
/**
*
* Shard the save files, one per device that has Variable nodes.
*
*
* bool sharded = 5;
*/
public boolean getSharded() {
return sharded_;
}
public static final int KEEP_CHECKPOINT_EVERY_N_HOURS_FIELD_NUMBER = 6;
private float keepCheckpointEveryNHours_;
/**
*
* How often to keep an additional checkpoint. If not specified, only the last
* "max_to_keep" checkpoints are kept; if specified, in addition to keeping
* the last "max_to_keep" checkpoints, an additional checkpoint will be kept
* for every n hours of training.
*
*
* float keep_checkpoint_every_n_hours = 6;
*/
public float getKeepCheckpointEveryNHours() {
return keepCheckpointEveryNHours_;
}
public static final int VERSION_FIELD_NUMBER = 7;
private int version_;
/**
* .tensorflow.SaverDef.CheckpointFormatVersion version = 7;
*/
public int getVersionValue() {
return version_;
}
/**
* .tensorflow.SaverDef.CheckpointFormatVersion version = 7;
*/
public org.tensorflow.util.SaverDef.CheckpointFormatVersion getVersion() {
org.tensorflow.util.SaverDef.CheckpointFormatVersion result = org.tensorflow.util.SaverDef.CheckpointFormatVersion.valueOf(version_);
return result == null ? org.tensorflow.util.SaverDef.CheckpointFormatVersion.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getFilenameTensorNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, filenameTensorName_);
}
if (!getSaveTensorNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, saveTensorName_);
}
if (!getRestoreOpNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, restoreOpName_);
}
if (maxToKeep_ != 0) {
output.writeInt32(4, maxToKeep_);
}
if (sharded_ != false) {
output.writeBool(5, sharded_);
}
if (keepCheckpointEveryNHours_ != 0F) {
output.writeFloat(6, keepCheckpointEveryNHours_);
}
if (version_ != org.tensorflow.util.SaverDef.CheckpointFormatVersion.LEGACY.getNumber()) {
output.writeEnum(7, version_);
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getFilenameTensorNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, filenameTensorName_);
}
if (!getSaveTensorNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, saveTensorName_);
}
if (!getRestoreOpNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, restoreOpName_);
}
if (maxToKeep_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeInt32Size(4, maxToKeep_);
}
if (sharded_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(5, sharded_);
}
if (keepCheckpointEveryNHours_ != 0F) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(6, keepCheckpointEveryNHours_);
}
if (version_ != org.tensorflow.util.SaverDef.CheckpointFormatVersion.LEGACY.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(7, version_);
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.tensorflow.util.SaverDef)) {
return super.equals(obj);
}
org.tensorflow.util.SaverDef other = (org.tensorflow.util.SaverDef) obj;
boolean result = true;
result = result && getFilenameTensorName()
.equals(other.getFilenameTensorName());
result = result && getSaveTensorName()
.equals(other.getSaveTensorName());
result = result && getRestoreOpName()
.equals(other.getRestoreOpName());
result = result && (getMaxToKeep()
== other.getMaxToKeep());
result = result && (getSharded()
== other.getSharded());
result = result && (
java.lang.Float.floatToIntBits(getKeepCheckpointEveryNHours())
== java.lang.Float.floatToIntBits(
other.getKeepCheckpointEveryNHours()));
result = result && version_ == other.version_;
return result;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + FILENAME_TENSOR_NAME_FIELD_NUMBER;
hash = (53 * hash) + getFilenameTensorName().hashCode();
hash = (37 * hash) + SAVE_TENSOR_NAME_FIELD_NUMBER;
hash = (53 * hash) + getSaveTensorName().hashCode();
hash = (37 * hash) + RESTORE_OP_NAME_FIELD_NUMBER;
hash = (53 * hash) + getRestoreOpName().hashCode();
hash = (37 * hash) + MAX_TO_KEEP_FIELD_NUMBER;
hash = (53 * hash) + getMaxToKeep();
hash = (37 * hash) + SHARDED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSharded());
hash = (37 * hash) + KEEP_CHECKPOINT_EVERY_N_HOURS_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getKeepCheckpointEveryNHours());
hash = (37 * hash) + VERSION_FIELD_NUMBER;
hash = (53 * hash) + version_;
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.tensorflow.util.SaverDef parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.util.SaverDef parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.util.SaverDef parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.tensorflow.util.SaverDef parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.tensorflow.util.SaverDef parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.util.SaverDef parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.util.SaverDef parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static org.tensorflow.util.SaverDef parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.tensorflow.util.SaverDef parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static org.tensorflow.util.SaverDef parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.tensorflow.util.SaverDef prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Protocol buffer representing the configuration of a Saver.
*
*
* Protobuf type {@code tensorflow.SaverDef}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:tensorflow.SaverDef)
org.tensorflow.util.SaverDefOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.tensorflow.util.SaverProtos.internal_static_tensorflow_SaverDef_descriptor;
}
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.tensorflow.util.SaverProtos.internal_static_tensorflow_SaverDef_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.tensorflow.util.SaverDef.class, org.tensorflow.util.SaverDef.Builder.class);
}
// Construct using org.tensorflow.util.SaverDef.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
public Builder clear() {
super.clear();
filenameTensorName_ = "";
saveTensorName_ = "";
restoreOpName_ = "";
maxToKeep_ = 0;
sharded_ = false;
keepCheckpointEveryNHours_ = 0F;
version_ = 0;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.tensorflow.util.SaverProtos.internal_static_tensorflow_SaverDef_descriptor;
}
public org.tensorflow.util.SaverDef getDefaultInstanceForType() {
return org.tensorflow.util.SaverDef.getDefaultInstance();
}
public org.tensorflow.util.SaverDef build() {
org.tensorflow.util.SaverDef result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public org.tensorflow.util.SaverDef buildPartial() {
org.tensorflow.util.SaverDef result = new org.tensorflow.util.SaverDef(this);
result.filenameTensorName_ = filenameTensorName_;
result.saveTensorName_ = saveTensorName_;
result.restoreOpName_ = restoreOpName_;
result.maxToKeep_ = maxToKeep_;
result.sharded_ = sharded_;
result.keepCheckpointEveryNHours_ = keepCheckpointEveryNHours_;
result.version_ = version_;
onBuilt();
return result;
}
public Builder clone() {
return (Builder) super.clone();
}
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.setField(field, value);
}
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return (Builder) super.clearField(field);
}
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return (Builder) super.clearOneof(oneof);
}
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, Object value) {
return (Builder) super.setRepeatedField(field, index, value);
}
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
Object value) {
return (Builder) super.addRepeatedField(field, value);
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.tensorflow.util.SaverDef) {
return mergeFrom((org.tensorflow.util.SaverDef)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.tensorflow.util.SaverDef other) {
if (other == org.tensorflow.util.SaverDef.getDefaultInstance()) return this;
if (!other.getFilenameTensorName().isEmpty()) {
filenameTensorName_ = other.filenameTensorName_;
onChanged();
}
if (!other.getSaveTensorName().isEmpty()) {
saveTensorName_ = other.saveTensorName_;
onChanged();
}
if (!other.getRestoreOpName().isEmpty()) {
restoreOpName_ = other.restoreOpName_;
onChanged();
}
if (other.getMaxToKeep() != 0) {
setMaxToKeep(other.getMaxToKeep());
}
if (other.getSharded() != false) {
setSharded(other.getSharded());
}
if (other.getKeepCheckpointEveryNHours() != 0F) {
setKeepCheckpointEveryNHours(other.getKeepCheckpointEveryNHours());
}
if (other.version_ != 0) {
setVersionValue(other.getVersionValue());
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
org.tensorflow.util.SaverDef parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (org.tensorflow.util.SaverDef) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object filenameTensorName_ = "";
/**
*
* The name of the tensor in which to specify the filename when saving or
* restoring a model checkpoint.
*
*
* string filename_tensor_name = 1;
*/
public java.lang.String getFilenameTensorName() {
java.lang.Object ref = filenameTensorName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filenameTensorName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the tensor in which to specify the filename when saving or
* restoring a model checkpoint.
*
*
* string filename_tensor_name = 1;
*/
public com.google.protobuf.ByteString
getFilenameTensorNameBytes() {
java.lang.Object ref = filenameTensorName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filenameTensorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the tensor in which to specify the filename when saving or
* restoring a model checkpoint.
*
*
* string filename_tensor_name = 1;
*/
public Builder setFilenameTensorName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
filenameTensorName_ = value;
onChanged();
return this;
}
/**
*
* The name of the tensor in which to specify the filename when saving or
* restoring a model checkpoint.
*
*
* string filename_tensor_name = 1;
*/
public Builder clearFilenameTensorName() {
filenameTensorName_ = getDefaultInstance().getFilenameTensorName();
onChanged();
return this;
}
/**
*
* The name of the tensor in which to specify the filename when saving or
* restoring a model checkpoint.
*
*
* string filename_tensor_name = 1;
*/
public Builder setFilenameTensorNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
filenameTensorName_ = value;
onChanged();
return this;
}
private java.lang.Object saveTensorName_ = "";
/**
*
* The operation to run when saving a model checkpoint.
*
*
* string save_tensor_name = 2;
*/
public java.lang.String getSaveTensorName() {
java.lang.Object ref = saveTensorName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
saveTensorName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The operation to run when saving a model checkpoint.
*
*
* string save_tensor_name = 2;
*/
public com.google.protobuf.ByteString
getSaveTensorNameBytes() {
java.lang.Object ref = saveTensorName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
saveTensorName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The operation to run when saving a model checkpoint.
*
*
* string save_tensor_name = 2;
*/
public Builder setSaveTensorName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
saveTensorName_ = value;
onChanged();
return this;
}
/**
*
* The operation to run when saving a model checkpoint.
*
*
* string save_tensor_name = 2;
*/
public Builder clearSaveTensorName() {
saveTensorName_ = getDefaultInstance().getSaveTensorName();
onChanged();
return this;
}
/**
*
* The operation to run when saving a model checkpoint.
*
*
* string save_tensor_name = 2;
*/
public Builder setSaveTensorNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
saveTensorName_ = value;
onChanged();
return this;
}
private java.lang.Object restoreOpName_ = "";
/**
*
* The operation to run when restoring a model checkpoint.
*
*
* string restore_op_name = 3;
*/
public java.lang.String getRestoreOpName() {
java.lang.Object ref = restoreOpName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
restoreOpName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The operation to run when restoring a model checkpoint.
*
*
* string restore_op_name = 3;
*/
public com.google.protobuf.ByteString
getRestoreOpNameBytes() {
java.lang.Object ref = restoreOpName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
restoreOpName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The operation to run when restoring a model checkpoint.
*
*
* string restore_op_name = 3;
*/
public Builder setRestoreOpName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
restoreOpName_ = value;
onChanged();
return this;
}
/**
*
* The operation to run when restoring a model checkpoint.
*
*
* string restore_op_name = 3;
*/
public Builder clearRestoreOpName() {
restoreOpName_ = getDefaultInstance().getRestoreOpName();
onChanged();
return this;
}
/**
*
* The operation to run when restoring a model checkpoint.
*
*
* string restore_op_name = 3;
*/
public Builder setRestoreOpNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
restoreOpName_ = value;
onChanged();
return this;
}
private int maxToKeep_ ;
/**
*
* Maximum number of checkpoints to keep. If 0, no checkpoints are deleted.
*
*
* int32 max_to_keep = 4;
*/
public int getMaxToKeep() {
return maxToKeep_;
}
/**
*
* Maximum number of checkpoints to keep. If 0, no checkpoints are deleted.
*
*
* int32 max_to_keep = 4;
*/
public Builder setMaxToKeep(int value) {
maxToKeep_ = value;
onChanged();
return this;
}
/**
*
* Maximum number of checkpoints to keep. If 0, no checkpoints are deleted.
*
*
* int32 max_to_keep = 4;
*/
public Builder clearMaxToKeep() {
maxToKeep_ = 0;
onChanged();
return this;
}
private boolean sharded_ ;
/**
*
* Shard the save files, one per device that has Variable nodes.
*
*
* bool sharded = 5;
*/
public boolean getSharded() {
return sharded_;
}
/**
*
* Shard the save files, one per device that has Variable nodes.
*
*
* bool sharded = 5;
*/
public Builder setSharded(boolean value) {
sharded_ = value;
onChanged();
return this;
}
/**
*
* Shard the save files, one per device that has Variable nodes.
*
*
* bool sharded = 5;
*/
public Builder clearSharded() {
sharded_ = false;
onChanged();
return this;
}
private float keepCheckpointEveryNHours_ ;
/**
*
* How often to keep an additional checkpoint. If not specified, only the last
* "max_to_keep" checkpoints are kept; if specified, in addition to keeping
* the last "max_to_keep" checkpoints, an additional checkpoint will be kept
* for every n hours of training.
*
*
* float keep_checkpoint_every_n_hours = 6;
*/
public float getKeepCheckpointEveryNHours() {
return keepCheckpointEveryNHours_;
}
/**
*
* How often to keep an additional checkpoint. If not specified, only the last
* "max_to_keep" checkpoints are kept; if specified, in addition to keeping
* the last "max_to_keep" checkpoints, an additional checkpoint will be kept
* for every n hours of training.
*
*
* float keep_checkpoint_every_n_hours = 6;
*/
public Builder setKeepCheckpointEveryNHours(float value) {
keepCheckpointEveryNHours_ = value;
onChanged();
return this;
}
/**
*
* How often to keep an additional checkpoint. If not specified, only the last
* "max_to_keep" checkpoints are kept; if specified, in addition to keeping
* the last "max_to_keep" checkpoints, an additional checkpoint will be kept
* for every n hours of training.
*
*
* float keep_checkpoint_every_n_hours = 6;
*/
public Builder clearKeepCheckpointEveryNHours() {
keepCheckpointEveryNHours_ = 0F;
onChanged();
return this;
}
private int version_ = 0;
/**
* .tensorflow.SaverDef.CheckpointFormatVersion version = 7;
*/
public int getVersionValue() {
return version_;
}
/**
* .tensorflow.SaverDef.CheckpointFormatVersion version = 7;
*/
public Builder setVersionValue(int value) {
version_ = value;
onChanged();
return this;
}
/**
* .tensorflow.SaverDef.CheckpointFormatVersion version = 7;
*/
public org.tensorflow.util.SaverDef.CheckpointFormatVersion getVersion() {
org.tensorflow.util.SaverDef.CheckpointFormatVersion result = org.tensorflow.util.SaverDef.CheckpointFormatVersion.valueOf(version_);
return result == null ? org.tensorflow.util.SaverDef.CheckpointFormatVersion.UNRECOGNIZED : result;
}
/**
* .tensorflow.SaverDef.CheckpointFormatVersion version = 7;
*/
public Builder setVersion(org.tensorflow.util.SaverDef.CheckpointFormatVersion value) {
if (value == null) {
throw new NullPointerException();
}
version_ = value.getNumber();
onChanged();
return this;
}
/**
* .tensorflow.SaverDef.CheckpointFormatVersion version = 7;
*/
public Builder clearVersion() {
version_ = 0;
onChanged();
return this;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:tensorflow.SaverDef)
}
// @@protoc_insertion_point(class_scope:tensorflow.SaverDef)
private static final org.tensorflow.util.SaverDef DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.tensorflow.util.SaverDef();
}
public static org.tensorflow.util.SaverDef getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public SaverDef parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new SaverDef(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public org.tensorflow.util.SaverDef getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy