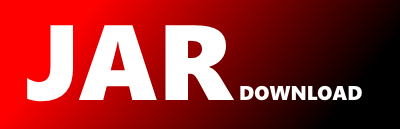
org.tentackle.dbms.StatementHistory Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tentackle-database Show documentation
Show all versions of tentackle-database Show documentation
Tentackle Low-Level DBMS Layer
/*
* Tentackle - https://tentackle.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.tentackle.dbms;
import org.tentackle.common.Timestamp;
import org.tentackle.log.Logger;
import org.tentackle.misc.TimeKeeper;
import java.util.List;
import java.util.Map;
import java.util.TreeSet;
/**
* History of statement execution.
*
* @author harald
*/
public class StatementHistory {
private static long logMinDurationMillis;
/**
* Sets the duration of statements to be logged as a warning.
*
* @param logMinDurationMillis the duration in milliseconds, 0 to disable (default)
*/
public static void setLogMinDurationMillis(long logMinDurationMillis) {
StatementHistory.logMinDurationMillis = logMinDurationMillis;
}
/**
* Gets the duration of statements to be logged as a warning.
*
* @return the duration in milliseconds
*/
public static long getLogMinDurationMillis() {
return logMinDurationMillis;
}
private static final Logger LOGGER = Logger.get(StatementHistory.class);
private final String txName; // transaction name if tx was running, else null
private final long executionStart; // start of execution in epochal milliseconds
private final TimeKeeper executionDuration; // SQL statement execution duration in nanoseconds
private final String sql; // the SQL statement
private final Map parameters; // execution parameters if prepared statement, else null
private final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy