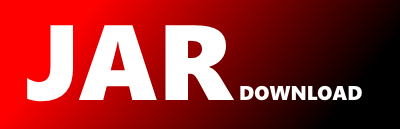
org.tentackle.fx.rdc.PdoEditor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tentackle-fx-rdc Show documentation
Show all versions of tentackle-fx-rdc Show documentation
Rich Desktop Client based on FX
/*
* Tentackle - http://www.tentackle.org.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
*/
package org.tentackle.fx.rdc;
import org.tentackle.fx.AbstractFxController;
import org.tentackle.fx.FxUtilities;
import org.tentackle.pdo.DomainContext;
import org.tentackle.pdo.DomainContextProvider;
import org.tentackle.pdo.PdoHolder;
import org.tentackle.pdo.PersistentDomainObject;
import org.tentackle.validate.ValidationMapper;
import org.tentackle.validate.ValidationUtilities;
import java.util.NavigableSet;
import java.util.TreeSet;
/**
* FxController to edit a PDO.
*
* @author harald
* @param the pdo type
*/
public abstract class PdoEditor> extends AbstractFxController
implements PdoHolder, DomainContextProvider {
/**
* Requests the initial focus after stage is shown.
*/
public abstract void requestInitialFocus();
@Override
public DomainContext getDomainContext() {
T pdo = getPdo();
return pdo == null ? null : pdo.getDomainContext();
}
/**
* Returns whether the user has sufficient permissions to view the PDO.
*
* @return true if allowed
*/
public boolean isViewAllowed() {
return getPdo().isViewAllowed();
}
/**
* Returns whether the user has sufficient permissions to edit the PDO.
*
* @return true if allowed
*/
public boolean isEditAllowed() {
return getPdo().isEditAllowed();
}
/**
* Returns whether the user is allowed to edit the PDO and the PDO is removable.
*
* @return true if remove allowed
*/
public boolean isRemoveAllowed() {
return isEditAllowed() && getPdo().isRemovable();
}
/**
* Returns whether the user is allowed to create a new PDO.
*
* @return true if creation allowed
*/
public boolean isNewAllowed() {
return isEditAllowed();
}
/**
* Gets the validation root path.
* By default, the server returns validation results with a validation path that starts with the entity baseclassname
* (the first character in lowercase). If the bound PDO variable of the editor uses a different name,
* a {@link ValidationMapper} is necessary to map the results back to the form.
* This method is provided to reduce the amount of application code.
*
* @return the validation root path, default is null
* @see #getValidationMappers()
*/
public String getValidationPath() {
return null;
}
/**
* Gets the validation mappers to map the validation results to the controls.
* If {@link #getValidationPath()} returns a non-null value, a set with a default mapper
* will be created.
*
* @return the mappers, null if none
*/
public NavigableSet getValidationMappers() {
String validationPath = getValidationPath();
if (validationPath != null) {
NavigableSet validationMappers = new TreeSet<>();
validationMappers.add(new ValidationMapper(ValidationUtilities.getInstance().getDefaultValidationPath(getPdo()), getBinder(), validationPath, null));
return validationMappers;
}
return null;
}
/**
* Validates the form before the PDO get saved.
* This can be used for validations that are not covered by the persistence model,
* for example password confirmation.
*
* @return true if ok
*/
public boolean validateForm() {
return true;
}
/**
* Prints the current PDO.
*/
public void print() {
// the default implementation just prints the contents of this editor
FxUtilities.getInstance().print(getView());
}
/**
* Set the changeable property of the editor's view.
*
* @param changeable true if changeable
*/
public void setChangeable(boolean changeable) {
getContainer().setChangeable(changeable);
}
/**
* Get the changeable property of the editor's view.
*
* @return true if changeable
*/
public boolean isChangeable() {
return getContainer().isChangeable();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy