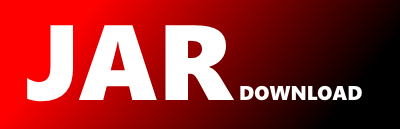
org.tentackle.fx.rdc.PdoTreeItem Maven / Gradle / Ivy
Show all versions of tentackle-fx-rdc Show documentation
/*
* Tentackle - http://www.tentackle.org.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
*/
package org.tentackle.fx.rdc;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import javafx.collections.ObservableList;
import javafx.scene.control.TreeItem;
import org.tentackle.pdo.PersistentDomainObject;
/**
* Tree item for a PDO.
*
* @author harald
* @param the pdo type
*/
public class PdoTreeItem> extends TreeItem {
private T pdo;
private GuiProvider provider;
private boolean childrenLoaded;
/**
* Creates a tree item for a PDO.
*
* @param pdo the pdo
*/
public PdoTreeItem(T pdo) {
super(pdo);
this.pdo = pdo;
}
/**
* Returns the PDO represented by this item.
*
* @return the PDO
*/
public T getPdo() {
return pdo;
}
/**
* Sets the pdo.
* Can be used to reload the pdo, for example.
*
* @param pdo the pdo
*/
public void setPdo(T pdo) {
setValue(pdo);
this.pdo = pdo;
provider = null; // force reload
}
@Override
public boolean isLeaf() {
return !getGuiProvider().providesTreeChildObjects();
}
@Override
public ObservableList> getChildren() {
if (!childrenLoaded) {
childrenLoaded = true;
List> childList = new ArrayList<>();
PdoTreeItem parentItem = getParentPdoItem();
for (PersistentDomainObject child:
getGuiProvider().getTreeChildObjects(parentItem == null ? null : parentItem.getPdo())) {
@SuppressWarnings("unchecked")
TreeItem treeItem = GuiProviderFactory.getInstance().createGuiProvider(child).createTreeItem();
childList.add(treeItem);
}
super.getChildren().setAll(childList);
}
return super.getChildren();
}
/**
* Gets the parent PdoTreeItem.
*
* @param the parent PDO type
* @return the parent, null if no parent or parent is no PdoTreeItem
*/
@SuppressWarnings("unchecked")
public
> PdoTreeItem
getParentPdoItem() {
TreeItem> parent = getParent();
return parent instanceof PdoTreeItem ? (PdoTreeItem) parent : null;
}
/**
* Gets the parent item's pdo.
*
* @param
the parent PDO type
* @param parentPdoClass the entity class of the parent PDO
* @return the parent pdo, null if no parent or parent is no PdoTreeItem or not pointing to an instance of {@code parentPdoClass}
*/
@SuppressWarnings({"unchecked", "rawtypes"})
public
> P getParentPdo(Class
parentPdoClass) {
TreeItem> parent = getParent();
if (parent instanceof PdoTreeItem) {
PersistentDomainObject parentPdo = ((PdoTreeItem) parent).getPdo();
if (parentPdo != null && parentPdoClass.isAssignableFrom(parentPdo.getClass())) {
return (P) parentPdo;
}
}
return null;
}
/**
* Lazily gets the GUI provider for the PDO.
*
* @return the provider, never null
*/
public GuiProvider getGuiProvider() {
if (provider == null) {
provider = GuiProviderFactory.getInstance().createGuiProvider(pdo);
}
return provider;
}
/**
* Expands this and all child tree items.
*/
public void expand() {
if (isExpanded()) {
collapse();
}
expand(new HashSet<>());
}
/**
* Collapses this and all child tree items.
*/
public void collapse() {
collapse(new HashSet<>());
}
/**
* Recursively expands given item and all child items.
*
* @param pdoSet holds already expanded PDOs to detect recursion loops
*/
protected void expand(Set pdoSet) {
if (getGuiProvider().providesTreeChildObjects()) {
if (pdoSet.add(getPdo())) {
setExpanded(true);
for (TreeItem childItem : getChildren()) {
((PdoTreeItem) childItem).expand(pdoSet);
}
}
}
}
/**
* Recursively collapses given item and all child items.
*
* @param pdoSet holds already collapsed PDOs to detect recursion loops
*/
protected void collapse(Set pdoSet) {
if (pdoSet.add(getPdo())) {
setExpanded(false);
for (TreeItem childItem : getChildren()) {
((PdoTreeItem) childItem).collapse(pdoSet);
}
}
}
}