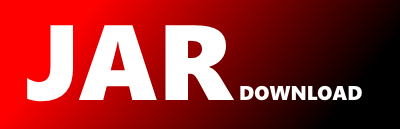
org.tentackle.fx.rdc.admin.SessionsView Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tentackle-fx-rdc Show documentation
Show all versions of tentackle-fx-rdc Show documentation
Rich Desktop Client based on FX
/*
* Tentackle - http://www.tentackle.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the DFree Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.tentackle.fx.rdc.admin;
import javafx.fxml.FXML;
import javafx.scene.control.SelectionMode;
import org.tentackle.bind.Bindable;
import org.tentackle.fx.AbstractFxController;
import org.tentackle.fx.Fx;
import org.tentackle.fx.FxControllerService;
import org.tentackle.fx.FxFactory;
import org.tentackle.fx.component.FxButton;
import org.tentackle.fx.component.FxTableView;
import org.tentackle.fx.container.FxHBox;
import org.tentackle.fx.rdc.RdcFactory;
import org.tentackle.fx.rdc.security.SecurityDialogFactory;
import org.tentackle.fx.rdc.table.TablePopup;
import org.tentackle.fx.table.TableConfiguration;
import org.tentackle.pdo.AdminExtension;
import org.tentackle.pdo.AdminExtension.SessionData;
import org.tentackle.pdo.DomainContext;
import org.tentackle.pdo.Pdo;
import org.tentackle.pdo.PdoRemoteSession;
import java.net.URL;
import java.text.MessageFormat;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.ResourceBundle;
import java.util.Set;
/**
* View showing all sessions logged in to the middle tier.
* Allows killing sessions as well.
*
* @author harald
*/
@FxControllerService
public class SessionsView extends AbstractFxController {
@Bindable
private List sessions;
@FXML
private FxTableView sessionsNode;
@FXML
private FxHBox buttonBox;
@FXML
private FxButton securityButton;
@FXML
private FxButton refreshButton;
@FXML
private FxButton killButton;
private ResourceBundle resources;
private AdminExtension adminExt;
private TablePopup popup;
@Override
@SuppressWarnings("unchecked")
public void initialize(URL location, ResourceBundle resources) {
this.resources = resources;
securityButton.setGraphic(Fx.createImageView("security"));
securityButton.setOnAction(e -> security());
refreshButton.setGraphic(Fx.createImageView("reload"));
refreshButton.setOnAction(e -> refresh());
killButton.setGraphic(Fx.createImageView("cancel"));
killButton.setOnAction(e -> kill());
killButton.disableProperty().bind(sessionsNode.getSelectionModel().selectedItemProperty().isNull());
popup = RdcFactory.getInstance().createTablePopup(sessionsNode, "Sessions", false, resources.getString("Sessions"));
TableConfiguration config = createTableConfiguration();
config.getBinder().bind();
config.configure(sessionsNode);
sessionsNode.getSelectionModel().setSelectionMode(SelectionMode.MULTIPLE);
}
@Override
public void configure() {
DomainContext context = Pdo.createDomainContext();
if (context.getSession().isRemote()) { // needs check to run tests
PdoRemoteSession remoteSession = (PdoRemoteSession) context.getSession().getRemoteSession();
adminExt = remoteSession.getExtension(context, AdminExtension.class);
popup.loadPreferences();
securityButton.setDisable(!SecurityDialogFactory.getInstance().isDialogAllowed(context));
refresh();
}
}
/**
* Gets the button box.
* Used to add additional buttons, for example to close the stage.
*
* @return the button box
*/
public FxHBox getButtonBox() {
return buttonBox;
}
/**
* Retrieves all sessions and refreshes the table view.
*/
private void refresh() {
sessions = adminExt.getSessions();
sessionsNode.updateView();
}
/**
* Kills all marked user sessions.
*/
private void kill() {
Set toKill = new LinkedHashSet<>();
// include all sessions of a group, even if not all are selected
for (SessionData session : sessionsNode.getSelectionModel().getSelectedItems()) {
toKill.add(session);
if (session.getSessionGroup() != 0) {
int row = 0;
for (SessionData otherSession: sessions) {
if (otherSession.getSessionGroup() == session.getSessionGroup()) {
toKill.add(otherSession);
sessionsNode.getSelectionModel().select(row, null);
}
row++;
}
}
}
if (!toKill.isEmpty()) {
String msg = toKill.size() > 1 ?
MessageFormat.format(resources.getString("really kill {0} sessions?"), toKill.size()) :
MessageFormat.format(resources.getString("really kill session {0}?"), toKill.iterator().next().getSessionNumber());
if (Fx.question(msg, false)) {
for (SessionData session : toKill) {
adminExt.kill(session.getUserId(), session.getSessionGroup(),
session.getApplicationName(), session.getApplicationId());
}
refresh();
}
}
}
/**
* Shows the security dialog.
*/
private void security() {
SecurityDialogFactory.getInstance().showDialog(AdminExtension.class);
}
private TableConfiguration createTableConfiguration() {
TableConfiguration config = FxFactory.getInstance().createTableConfiguration(SessionData.class, null);
config.addColumn("userId", resources.getString("user-ID"));
config.addColumn("userName", resources.getString("user"));
config.addColumn("applicationName", resources.getString("application"));
config.addColumn("applicationId", resources.getString("appl.-ID"));
config.addColumn("clientVersionInfo", resources.getString("version"));
config.addColumn("locale", resources.getString("locale"));
config.addColumn("timeZone", resources.getString("timezone"));
config.addColumn("vmInfo", resources.getString("VM"));
config.addColumn("osInfo", resources.getString("OS"));
config.addColumn("hostInfo", resources.getString("hostname"));
config.addColumn("clientHost", resources.getString("client"));
config.addColumn("since", resources.getString("since"));
config.addColumn("sessionNumber", resources.getString("session-no."));
config.addColumn("sessionGroup", resources.getString("group-no."));
config.addColumn("options", resources.getString("options"));
return config;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy