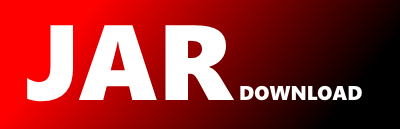
org.tentackle.fx.table.TableColumnConfiguration Maven / Gradle / Ivy
/*
* Tentackle - https://tentackle.org.
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
*/
package org.tentackle.fx.table;
import javafx.geometry.Pos;
import javafx.scene.control.TableColumn;
import javafx.scene.control.TreeTableColumn;
import org.tentackle.fx.FxComponent;
import org.tentackle.fx.bind.FxTableBinding;
import java.lang.reflect.Type;
import java.text.DateFormat;
import java.text.DecimalFormat;
import java.time.format.DateTimeFormatter;
/**
* Configuration for a table or treetable column.
*
* @param type of the objects contained within the table's or treetable's items list
* @param type of the content in all cells in this column
*
* @author harald
*/
public interface TableColumnConfiguration {
/**
* Gets the table configuration.
*
* @return the table configuration
*/
TableConfiguration getTableConfiguration();
/**
* Gets the column name.
* This is usually the binding path.
* The name is also used as a key to the table preferences.
*
* @return the name of the column
*/
String getName();
/**
* Gets the column name displayed in the column header.
*
* @return the display name of the column
*/
String getDisplayedName();
/**
* Sets the column name displayed in the column header.
*
* @param displayName the display name of the column
*/
void setDisplayedName(String displayName);
/**
* Determines the class for a given column.
*
* @return the column class
*/
Class getType();
/**
* Sets the column type.
*
* @param type the type
*/
void setType(Class type);
/**
* Sets the generic type.
*
* @param genericType the generic type
*/
void setGenericType(Type genericType);
/**
* Gets the generic type.
*
* @return the generic type
*/
Type getGenericType();
/**
* Defines the format for numeric or date/time-types.
* If null is returned a default format will be used according to the column class.
*
* @return the format pattern or null if default
*/
String getPattern();
/**
* Sets the formatting string.
*
* @param pattern the format pattern
*/
void setPattern(String pattern);
/**
* Gets the number format.
*
* @return the number format, null if default
*/
DecimalFormat getNumberFormat();
/**
* Gets the date format.
*
* @return the date format, null if default
*/
DateFormat getDateFormat();
/**
* Gets the date or time formatter.
*
* @return the formatter, null if default
*/
DateTimeFormatter getDateTimeFormatter();
/**
* Defines the alignment of the column.
*
* @return the alignment or null if default
*/
Pos getAlignment();
/**
* Sets the alignment within the cell.
*
* @param alignment the alignment
*/
void setAlignment(Pos alignment);
/**
* Defines the "blankzero" attribute of the column.
*
* @return true if blank zeros, false if not, null if use default from editor
*/
Boolean isBlankZero();
/**
* Sets whether to blank out numeric zero values.
*
* @param blankZero true if blank zeros, false if not, null if use default from editor
*/
void setBlankZero(Boolean blankZero);
/**
* Sets whether a numeric field is unsigned or signed.
*
* @param unsigned true if unsigned, null if use default from editor
*/
void setUnsigned(Boolean unsigned);
/**
* Returns whether a numeric field is unsigned or signed.
*
* @return true if unsigned, null if use default from editor
*/
Boolean isUnsigned();
/**
* Sets allowed characters.
*
* @param validChars the valid characters, null if use default from editor
*/
void setValidChars(String validChars);
/**
* Gets allowed characters.
*
* @return the valid characters, null if use default from editor
*/
String getValidChars();
/**
* Sets invalid characters.
*
* @param invalidChars the invalid characters, null if use default from editor
*/
void setInvalidChars(String invalidChars);
/**
* Gets invalid characters.
*
* @return the invalid characters, null if use default from editor
*/
String getInvalidChars();
/**
* Gets the autoselect flag.
*
* @return true if autoselect, false if not, null if use default from editor
*/
Boolean isAutoSelect();
/**
* Sets the autoselect feature.
*
* @param autoSelect true if autoselect, false if not, null if use default from editor
*/
void setAutoSelect(Boolean autoSelect);
/**
* Gets the maximum columns for text cell editors.
*
* @return the max columns, null if not defined
*/
Integer getMaxColumns();
/**
* Sets the max columns.
*
* @param maxColumns the max columns, null if not defined
*/
void setMaxColumns(Integer maxColumns);
/**
* Gets the scale for fractional numeric cell editors.
*
* @return the scale, null if not defined
*/
Integer getScale();
/**
* Sets the numeric scale.
*
* @param scale the scale, null if not defined
*/
void setScale(Integer scale);
/**
* Gets the case conversion.
*
* @return true = convert to uppercase, false = lowercase, null = no conversion (default)
*/
Boolean getCaseConversion();
/**
* Sets the case conversion.
*
* @param caseConversion true = convert to uppercase, false = lowercase, null = no conversion (default)
*/
void setCaseConversion(Boolean caseConversion);
/**
* Determines whether the column is summable.
*
* @return true if column is summable
*/
boolean isSummable();
/**
* Sets whether the column is summable.
*
* @param summable {@link Boolean#TRUE} if summable, {@link Boolean#FALSE} if not, NULL if numeric type
*/
void setSummable(Boolean summable);
/**
* Returns whether the column is summable.
*
* @return {@link Boolean#TRUE} if summable, {@link Boolean#FALSE} if not, NULL if numeric type
*/
Boolean getSummable();
/**
* Returns whether the column is editable.
*
* @return {@link Boolean#TRUE} if editable, {@link Boolean#FALSE} if not, NULL if depends on table configuration
*/
Boolean getEditable();
/**
* Sets whether the column is editable.
*
* @param editable {@link Boolean#TRUE} if editable, {@link Boolean#FALSE} if not, NULL if depends on table configuration
*/
void setEditable(Boolean editable);
/**
* Returns whether column is editable.
*
* @return true if editable, the default is false
*/
boolean isEditable();
/**
* Gets the editor component.
*
* @return the editor, null if use default from {@link TableCellType#getEditor()}
*/
FxComponent getEditor();
/**
* Sets the editor component.
*
* @param editor the editor, null if use default from {@link TableCellType#getEditor()}
*/
void setEditor(FxComponent editor);
/**
* Gets the binding for this column.
*
* @return the binding, null if none
*/
FxTableBinding getBinding();
/**
* Sets the binding.
*
* @param binding the binding
*/
void setBinding(FxTableBinding binding);
/**
* Gets or creates the table column.
* Used if configuration applies to a {@link javafx.scene.control.TableView}.
*
* @return the table column, never null
*/
TableColumn getTableColumn();
/**
* Gets or creates the tree table column.
* Used if configuration applies to a {@link javafx.scene.control.TreeTableView}.
*
* @return the tree table column, never null
*/
TreeTableColumn getTreeTableColumn();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy