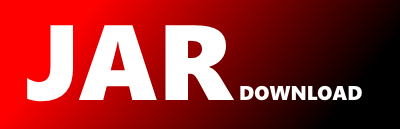
org.tentackle.persist.wurblet.PdoCache Maven / Gradle / Ivy
// wurblet generated by Wurbelizer 21.6.0.0, see https://wurbelizer.org
package org.tentackle.persist.wurblet;
import org.tentackle.wurblet.*;
import org.tentackle.buildsupport.*;
import java.util.*;
import java.io.*;
import org.tentackle.common.*;
import org.wurbelizer.wurbel.*;
import org.tentackle.sql.*;
import org.tentackle.model.*;
import org.wurbelizer.wurblet.*;
/**
* ({@code @wurblet}) Generate code for setting up a PdoCache for an entity.
*
* usage:
* @wurblet <tag> PdoCache
* [--secure] [--preload] [--mutable] [--udk]
* [--strategy=LRU|LFU|FORGET] [--maxsize=<n>] [--keepquota=<p>] [--configure=<method>]
* [index1] [index2] ...
*
* arguments:
*
* - --secure: if cache must check read permission for each access (default is no check).
* - --preload: the first access will load all entities at once.
* - --mutable: generates a non-shared cache, i.e. mutable PDOs (by default a shared readonly cache is generated).
* - --udk: add index for the unique domain key.
* - --strategy=LRU|LFU|FORGET: the caching strategy (default is FORGET).
* - --maxsize=<n>: the cache size (if not preloaded).
* - --keepquota=<p>: percentage of entries to keep when applying caching strategy. Default is 50.
* - --configure=<method>: an optional method to further configure the cache.
* - --index<n>: additional unique indexes. By default, only the ID-index will be created.
*
* For more options, see {@link DbModelWurblet}.
*/
public class PdoCache extends DbModelWurblet {
@Override
public void run() throws WurbelException {
super.run();
try {
wurbel();
}
catch (Throwable t) {
if (t instanceof WurbelException) {
throw (WurbelException) t;
}
throw new WurbelException("wurblet " + this + " failed", t);
}
}
// ----------------- begin wurblet code -----------------
private void wurbel() throws ModelException, WurbelException {
// main class
String pdoName = getPdoClassName();
boolean isAbstract = getEntity().isAbstract();
String pdoType = isAbstract ? "T" : pdoName;
boolean secure = false;
boolean preload = false;
boolean mutable = false;
boolean delayed = true;
boolean genudk = false;
String strategy = null;
Integer maxSize = null;
Integer keepQuota = null;
String configure = null;
for (String arg: getOptionArgs()) {
if (arg.equals("preload")) {
preload = true;
}
else if (arg.equals("secure")) {
secure = true;
}
else if (arg.equals("mutable")) {
mutable = true;
}
else if (arg.equals("immediate")) {
delayed = false;
}
else if (arg.equals("udk")) {
genudk = true;
}
else if (arg.startsWith("strategy=")) {
strategy = arg.substring(9).toUpperCase(Locale.ROOT);
}
else if (arg.startsWith("maxsize=")) {
maxSize = Integer.valueOf(arg.substring(8));
}
else if (arg.startsWith("keepquota=")) {
keepQuota = Integer.valueOf(arg.substring(10));
}
else if (arg.startsWith("configure=")) {
configure = arg.substring(10);
}
}
if (secure && !getEntity().isRootEntity()) {
throw new WurbelException(getEntity() + " is not a root-entity");
}
if (getEntity().getInheritanceType() == InheritanceType.PLAIN) {
throw new WurbelException("only concrete (leaf) entity types can be cached within a PLAIN inheritance hierarchy");
}
List udk = null;
String udkType = null;
String udkName = null;
String udkSuffix = null;
if (genudk) {
udk = getEntity().getUniqueDomainKey();
if (!udk.isEmpty()) {
if (udk.size() == 1) {
udkType = getNonPrimitiveJavaType(udk.get(0));
udkName = udk.get(0).getName();
udkSuffix = udk.get(0).getMethodNameSuffix();
}
else {
udkType = getEntity().getName() + "UDK";
udkName = "udk";
udkSuffix = "UniqueDomainKey";
udkType = getEntity().getName() + "." + udkType;
}
}
else {
udk = null;
genudk = false;
}
}
StringBuilder listenNames = new StringBuilder();
if (getEntity().getInheritanceType() == InheritanceType.MULTI) {
for (Entity leaf : getEntity().getLeafEntities()) {
if (!listenNames.isEmpty()) {
listenNames.append(", ");
}
listenNames.append(leaf.getName()).append(".class");
}
}
else {
listenNames.append(pdoName).append(".class");
}
out.print(source[0]); // 119:2 = " /** Holder of the PDO cache singleton...."
if (isAbstract) {
out.print(source[1]); // 124:2 = " private static final PdoCache exte..."
out.print(pdoName);
out.print(source[2]); // 125:55 = ">> CACHE = createCache();"
for (WurbletArgument key: getExpressionArguments()) {
String name = key.getMethodArgumentName();
String type = getNonPrimitiveJavaType(key.getAttribute());
out.print(source[3]); // 130:2 = " private static final PdoCacheIndex..."
out.print(pdoName);
out.print(source[4]); // 131:60 = ">, "
out.print(type);
out.print(source[5]); // 131:73 = "> "
out.print(name.toUpperCase(Locale.ROOT) + "_INDEX");
out.print(source[6]); // 131:119 = " = create"
out.print(StringHelper.firstToUpper(name));
out.print(source[7]); // 131:163 = "Index();"
}
if (genudk) {
out.print(source[8]); // 136:2 = " private static final PdoCacheIndex..."
out.print(pdoName);
out.print(source[9]); // 137:60 = ">, "
out.print(udkType);
out.print(source[10]); // 137:76 = "> UDK_INDEX = createUdkIndex();"
}
out.print(source[11]); // 140:2 = " @SuppressWarnings("unchecked") ..."
out.print(pdoName);
out.print(source[12]); // 143:41 = "> PdoCache createCache() { P..."
out.print(pdoName);
out.print(source[13]); // 144:70 = ".class, "
out.print(preload ? "true" : "false");
out.print(source[14]); // 144:108 = ", "
out.print(mutable ? "false" : "true");
out.print(source[15]); // 144:140 = ", "
out.print(secure ? "true" : "false");
out.print(source[16]); // 144:171 = "); Pdo.listen(cache::expire, "
out.print(listenNames);
out.print(source[17]); // 145:47 = ");"
if (strategy != null) {
out.print(source[18]); // 148:2 = " cache.setStrategy(PdoCacheStrategy..."
out.print(strategy);
out.print(source[19]); // 149:53 = ");"
}
if (maxSize != null) {
out.print(source[20]); // 153:2 = " cache.setMaxSize("
out.print(maxSize);
out.print(source[21]); // 154:34 = ");"
}
if (keepQuota != null) {
out.print(source[22]); // 158:2 = " cache.setKeepQuota("
out.print(keepQuota);
out.print(source[23]); // 159:38 = ");"
}
if (configure != null) {
out.print(source[24]); // 163:2 = " "
out.print(configure);
out.print(source[25]); // 164:19 = "(cache);"
}
out.print(source[26]); // 167:2 = " return cache; }"
for (WurbletArgument key: getExpressionArguments()) {
String name = key.getMethodArgumentName();
String type = getNonPrimitiveJavaType(key.getAttribute());
String suffix = Character.toUpperCase(name.charAt(0)) + name.substring(1);
if (isRemote()) {
RemoteIncludes genInc = new RemoteIncludes(this);
PrintStream implOut = genInc.getImplStream();
PrintStream remoteOut = genInc.getRemoteStream();
remoteOut.print(source[27]); // 180:16 = " T selectBy"
remoteOut.print(suffix);
remoteOut.print(source[28]); // 181:22 = "ForCache(DomainContext context, "
remoteOut.print(type);
remoteOut.print(source[29]); // 181:62 = " "
remoteOut.print(name);
remoteOut.print(source[30]); // 181:71 = ") throws RemoteException;"
implOut.print(source[31]); // 182:14 = " @Override public T selectBy"
implOut.print(suffix);
implOut.print(source[32]); // 185:29 = "ForCache(DomainContext context, "
implOut.print(type);
implOut.print(source[33]); // 185:69 = " "
implOut.print(name);
implOut.print(source[34]); // 185:78 = ") throws RemoteException { try { ..."
implOut.print(suffix);
implOut.print(source[35]); // 187:58 = "("
implOut.print(name);
implOut.print(source[36]); // 187:67 = "); } catch (RuntimeException e) ..."
out.print(source[37]); // 193:6 = " private static > PdoCacheIndex create"
out.print(StringHelper.firstToUpper(name));
out.print(source[40]); // 195:114 = "Index() { return new PdoCacheIndex..."
out.print(pdoName);
out.print(source[41]); // 196:45 = ":"
out.print(name);
out.print(source[42]); // 196:54 = "") { @Override @Suppress..."
out.print(type);
out.print(source[43]); // 199:55 = " "
out.print(name);
out.print(source[44]); // 199:64 = ") { return (X) Pdo.create("
out.print(pdoName);
out.print(source[45]); // 200:43 = ".class, context).selectBy"
out.print(suffix);
out.print(source[46]); // 200:78 = "ForCache("
out.print(name);
out.print(source[47]); // 200:95 = "); } @Override p..."
out.print(type);
out.print(source[48]); // 203:23 = " extract(X pdo) { return pdo.g..."
out.print(suffix);
out.print(source[49]); // 204:34 = "(); } }; }"
} // end isRemote
else {
out.print(source[50]); // 211:2 = " private static > PdoCacheIndex create"
out.print(StringHelper.firstToUpper(name));
out.print(source[53]); // 213:114 = "Index() { return new PdoCacheIndex..."
out.print(pdoName);
out.print(source[54]); // 214:45 = ":"
out.print(name);
out.print(source[55]); // 214:54 = "") { @Override @Suppress..."
out.print(type);
out.print(source[56]); // 217:55 = " "
out.print(name);
out.print(source[57]); // 217:64 = ") { return (X) Pdo.create("
out.print(pdoName);
out.print(source[58]); // 218:43 = ".class, context).findBy"
out.print(suffix);
out.print(source[59]); // 218:76 = "("
out.print(name);
out.print(source[60]); // 218:85 = "); } @Override p..."
out.print(type);
out.print(source[61]); // 221:23 = " extract(X pdo) { return pdo.g..."
out.print(suffix);
out.print(source[62]); // 222:34 = "(); } }; }"
}
}
if (genudk) {
out.print(source[63]); // 231:2 = " private static > PdoCacheIndex createUdkIndex() { return new Pd..."
out.print(pdoName);
out.print(source[66]); // 234:45 = ":UDK") { @Override @Supp..."
out.print(udkType);
out.print(source[67]); // 237:58 = " "
out.print(udkName);
out.print(source[68]); // 237:70 = ") {"
if (isRemote()) {
RemoteIncludes genInc = new RemoteIncludes(this);
PrintStream implOut = genInc.getImplStream();
PrintStream remoteOut = genInc.getRemoteStream();
remoteOut.print(source[69]); // 244:16 = " T selectByUniqueDomainKeyForCache(Doma..."
remoteOut.print(udkType);
remoteOut.print(source[70]); // 245:70 = " "
remoteOut.print(udkName);
remoteOut.print(source[71]); // 245:82 = ") throws RemoteException;"
implOut.print(source[72]); // 246:14 = " @Override public T selectByUniqueDo..."
implOut.print(udkType);
implOut.print(source[73]); // 249:77 = " "
implOut.print(udkName);
implOut.print(source[74]); // 249:89 = ") throws RemoteException { try { ..."
implOut.print(udkName);
implOut.print(source[75]); // 251:75 = "); } catch (RuntimeException e) ..."
out.print(source[76]); // 257:6 = " return (X) Pdo.create("
out.print(pdoName);
out.print(source[77]); // 258:43 = ".class, context).selectByUniqueDomainKey..."
out.print(udkName);
out.print(source[78]); // 258:103 = ");"
}
else {
out.print(source[79]); // 262:2 = " return (X) Pdo.create("
out.print(pdoName);
out.print(source[80]); // 263:43 = ".class, context).selectByUniqueDomainKey..."
out.print(udkName);
out.print(source[81]); // 263:95 = ");"
}
out.print(source[82]); // 266:2 = " } @Override publ..."
out.print(udkType);
out.print(source[83]); // 269:26 = " extract(X pdo) { return pdo.g..."
out.print(udkSuffix);
out.print(source[84]); // 270:37 = "(); } }; }"
}
out.print(source[85]); // 276:2 = " }"
} // end isAbstract() ----------------------------------------------------------------
else {
out.print(source[86]); // 283:2 = " private static final PdoCache<"
out.print(pdoName);
out.print(source[87]); // 284:45 = "> CACHE = createCache();"
for (WurbletArgument key: getExpressionArguments()) {
String name = key.getMethodArgumentName();
String type = getNonPrimitiveJavaType(key.getAttribute());
out.print(source[88]); // 289:2 = " private static final PdoCacheIndex<"
out.print(pdoName);
out.print(source[89]); // 290:50 = ", "
out.print(type);
out.print(source[90]); // 290:60 = "> "
out.print(name.toUpperCase(Locale.ROOT) + "_INDEX");
out.print(source[91]); // 290:106 = " = create"
out.print(StringHelper.firstToUpper(name));
out.print(source[92]); // 290:150 = "Index();"
}
if (genudk) {
out.print(source[93]); // 295:2 = " private static final PdoCacheIndex<"
out.print(pdoName);
out.print(source[94]); // 296:50 = ", "
out.print(udkType);
out.print(source[95]); // 296:63 = "> UDK_INDEX = createUdkIndex();"
}
out.print(source[96]); // 299:2 = " private static PdoCache<"
out.print(pdoName);
out.print(source[97]); // 301:39 = "> createCache() { PdoCache<"
out.print(pdoName);
out.print(source[98]); // 302:26 = "> cache = Pdo.createPdoCache("
out.print(pdoName);
out.print(source[99]); // 302:66 = ".class, "
out.print(preload ? "true" : "false");
out.print(source[100]); // 302:104 = ", "
out.print(mutable ? "false" : "true");
out.print(source[101]); // 302:136 = ", "
out.print(secure ? "true" : "false");
out.print(source[102]); // 302:167 = "); Pdo.listen(cache::expire, "
out.print(listenNames);
out.print(source[103]); // 303:47 = ");"
if (strategy != null) {
out.print(source[104]); // 306:2 = " cache.setStrategy(PdoCacheStrategy..."
out.print(strategy);
out.print(source[105]); // 307:53 = ");"
}
if (maxSize != null) {
out.print(source[106]); // 311:2 = " cache.setMaxSize("
out.print(maxSize);
out.print(source[107]); // 312:34 = ");"
}
if (keepQuota != null) {
out.print(source[108]); // 316:2 = " cache.setKeepQuota("
out.print(keepQuota);
out.print(source[109]); // 317:38 = ");"
}
if (configure != null) {
out.print(source[110]); // 321:2 = " "
out.print(configure);
out.print(source[111]); // 322:19 = "(cache);"
}
out.print(source[112]); // 325:2 = " return cache; }"
for (WurbletArgument key: getExpressionArguments()) {
String name = key.getMethodArgumentName();
String type = getNonPrimitiveJavaType(key.getAttribute());
String suffix = Character.toUpperCase(name.charAt(0)) + name.substring(1);
if (isRemote()) {
RemoteIncludes genInc = new RemoteIncludes(this);
PrintStream implOut = genInc.getImplStream();
PrintStream remoteOut = genInc.getRemoteStream();
remoteOut.print(source[113]); // 338:16 = " "
remoteOut.print(pdoType);
remoteOut.print(source[114]); // 339:13 = " selectBy"
remoteOut.print(suffix);
remoteOut.print(source[115]); // 339:32 = "ForCache(DomainContext context, "
remoteOut.print(type);
remoteOut.print(source[116]); // 339:72 = " "
remoteOut.print(name);
remoteOut.print(source[117]); // 339:81 = ") throws RemoteException;"
implOut.print(source[118]); // 340:14 = " @Override public "
implOut.print(pdoType);
implOut.print(source[119]); // 343:20 = " selectBy"
implOut.print(suffix);
implOut.print(source[120]); // 343:39 = "ForCache(DomainContext context, "
implOut.print(type);
implOut.print(source[121]); // 343:79 = " "
implOut.print(name);
implOut.print(source[122]); // 343:88 = ") throws RemoteException { try { ..."
implOut.print(suffix);
implOut.print(source[123]); // 345:58 = "("
implOut.print(name);
implOut.print(source[124]); // 345:67 = "); } catch (RuntimeException e) ..."
out.print(source[125]); // 351:6 = " private static PdoCacheIndex<"
out.print(pdoName);
out.print(source[126]); // 353:44 = ", "
out.print(type);
out.print(source[127]); // 353:54 = "> create"
out.print(StringHelper.firstToUpper(name));
out.print(source[128]); // 353:97 = "Index() { return new PdoCacheIndex..."
out.print(pdoName);
out.print(source[129]); // 354:45 = ":"
out.print(name);
out.print(source[130]); // 354:54 = "") { @Override public "
out.print(pdoName);
out.print(source[131]); // 356:26 = " select(DomainContext context, "
out.print(type);
out.print(source[132]); // 356:65 = " "
out.print(name);
out.print(source[133]); // 356:74 = ") { return Pdo.create("
out.print(pdoName);
out.print(source[134]); // 357:39 = ".class, context).selectBy"
out.print(suffix);
out.print(source[135]); // 357:74 = "ForCache("
out.print(name);
out.print(source[136]); // 357:91 = "); } @Override p..."
out.print(type);
out.print(source[137]); // 360:23 = " extract("
out.print(pdoName);
out.print(source[138]); // 360:43 = " pdo) { return pdo.get"
out.print(suffix);
out.print(source[139]); // 361:34 = "(); } }; }"
} // end isRemote
else {
out.print(source[140]); // 368:2 = " private static PdoCacheIndex<"
out.print(pdoName);
out.print(source[141]); // 370:44 = ", "
out.print(type);
out.print(source[142]); // 370:54 = "> create"
out.print(StringHelper.firstToUpper(name));
out.print(source[143]); // 370:97 = "Index() { return new PdoCacheIndex..."
out.print(pdoName);
out.print(source[144]); // 371:45 = ":"
out.print(name);
out.print(source[145]); // 371:54 = "") { @Override public "
out.print(pdoName);
out.print(source[146]); // 373:26 = " select(DomainContext context, "
out.print(type);
out.print(source[147]); // 373:65 = " "
out.print(name);
out.print(source[148]); // 373:74 = ") { return Pdo.create("
out.print(pdoName);
out.print(source[149]); // 374:39 = ".class, context).findBy"
out.print(suffix);
out.print(source[150]); // 374:72 = "("
out.print(name);
out.print(source[151]); // 374:81 = "); } @Override p..."
out.print(type);
out.print(source[152]); // 377:23 = " extract("
out.print(pdoName);
out.print(source[153]); // 377:43 = " pdo) { return pdo.get"
out.print(suffix);
out.print(source[154]); // 378:34 = "(); } }; }"
}
}
if (genudk) {
out.print(source[155]); // 387:2 = " private static PdoCacheIndex<"
out.print(pdoName);
out.print(source[156]); // 389:44 = ", "
out.print(udkType);
out.print(source[157]); // 389:57 = "> createUdkIndex() { return new Pd..."
out.print(pdoName);
out.print(source[158]); // 390:45 = ":UDK") { @Override publi..."
out.print(pdoName);
out.print(source[159]); // 392:26 = " select(DomainContext context, "
out.print(udkType);
out.print(source[160]); // 392:68 = " "
out.print(udkName);
out.print(source[161]); // 392:80 = ") {"
if (isRemote()) {
RemoteIncludes genInc = new RemoteIncludes(this);
PrintStream implOut = genInc.getImplStream();
PrintStream remoteOut = genInc.getRemoteStream();
remoteOut.print(source[162]); // 399:16 = " "
remoteOut.print(pdoType);
remoteOut.print(source[163]); // 400:13 = " selectByUniqueDomainKeyForCache(DomainC..."
remoteOut.print(udkType);
remoteOut.print(source[164]); // 400:80 = " "
remoteOut.print(udkName);
remoteOut.print(source[165]); // 400:92 = ") throws RemoteException;"
implOut.print(source[166]); // 401:14 = " @Override public "
implOut.print(pdoType);
implOut.print(source[167]); // 404:20 = " selectByUniqueDomainKeyForCache(DomainC..."
implOut.print(udkType);
implOut.print(source[168]); // 404:87 = " "
implOut.print(udkName);
implOut.print(source[169]); // 404:99 = ") throws RemoteException { try { ..."
implOut.print(udkName);
implOut.print(source[170]); // 406:75 = "); } catch (RuntimeException e) ..."
out.print(source[171]); // 412:6 = " return Pdo.create("
out.print(pdoName);
out.print(source[172]); // 413:39 = ".class, context).selectByUniqueDomainKey..."
out.print(udkName);
out.print(source[173]); // 413:99 = ");"
}
else {
out.print(source[174]); // 417:2 = " return Pdo.create("
out.print(pdoName);
out.print(source[175]); // 418:39 = ".class, context).selectByUniqueDomainKey..."
out.print(udkName);
out.print(source[176]); // 418:91 = ");"
}
out.print(source[177]); // 421:2 = " } @Override publ..."
out.print(udkType);
out.print(source[178]); // 424:26 = " extract("
out.print(pdoName);
out.print(source[179]); // 424:46 = " pdo) { return pdo.get"
out.print(udkSuffix);
out.print(source[180]); // 425:37 = "(); } }; }"
}
out.print(source[181]); // 431:2 = " }"
}
String filterBegin = "";
String filterEnd = "";
if (getEntity().isAbstract()) {
filterBegin = "filterByClassId(";
filterEnd = ")";
if (getEntity().getSuperEntity() == null) {
out.print(source[182]); // 444:2 = " private boolean isValidClassId(T pdo)..."
}
else {
out.print(source[183]); // 464:2 = " private boolean isValidClassId(T pdo)..."
}
}
out.print(source[184]); // 481:2 = " @Override"
if (isAbstract) {
out.print(source[185]); // 486:2 = " @SuppressWarnings("unchecked")"
}
out.print(source[186]); // 490:2 = " public PdoCache<"
out.print(pdoType);
out.print(source[187]); // 491:29 = "> getCache() {"
if (isAbstract) {
out.print(source[188]); // 494:2 = " return (PdoCache<"
out.print(pdoType);
out.print(source[189]); // 495:32 = ">) CacheHolder.CACHE;"
}
else {
out.print(source[190]); // 499:2 = " return CacheHolder.CACHE;"
}
out.print(source[191]); // 503:2 = " } @Override public boolean isCoun..."
out.print(pdoType);
out.print(source[192]); // 523:20 = " selectCachedOnly(long id) { return ..."
out.print(filterBegin);
out.print(source[193]); // 524:26 = "getCache().select(getDomainContext(), id..."
out.print(filterEnd);
out.print(source[194]); // 524:87 = "; } @Override public "
out.print(pdoType);
out.print(source[195]); // 528:20 = " selectCached(long id) { return "
out.print(filterBegin);
out.print(source[196]); // 529:26 = "getCache().select(getDomainContext(), id..."
out.print(filterEnd);
out.print(source[197]); // 529:80 = "; }"
for (WurbletArgument key: getExpressionArguments()) {
String name = key.getMethodArgumentName();
String type = getNonPrimitiveJavaType(key.getAttribute());
String suffix = Character.toUpperCase(name.charAt(0)) + name.substring(1);
String indexCast = getEntity().isAbstract() ? ("(PdoCacheIndex) ") : "";
String indexName = "CacheHolder." + name.toUpperCase(Locale.ROOT) + "_INDEX";
String indexMethod = "getCacheIndex" + StringHelper.firstToUpper(name);
out.print(source[198]); // 539:2 = " /** * Gets the index for "
out.print(name);
out.print(source[199]); // 542:32 = ". * * @return the index for "
out.print(type);
out.print(source[200]); // 544:36 = " "
out.print(name);
out.print(source[201]); // 544:45 = " */"
if (isAbstract) {
out.print(source[202]); // 548:2 = " @SuppressWarnings("unchecked")"
}
out.print(source[203]); // 552:2 = " protected PdoCacheIndex<"
out.print(pdoType);
out.print(source[204]); // 553:37 = ", "
out.print(type);
out.print(source[205]); // 553:47 = "> "
out.print(indexMethod);
out.print(source[206]); // 553:64 = "() { return "
out.print(indexCast);
out.print(indexName);
out.print(source[207]); // 554:37 = "; } /** * Selects from cache by ..."
out.print(name);
out.print(source[208]); // 558:35 = " but does not load from db if not in cac..."
out.print(name);
out.print(source[209]); // 560:21 = " the unique key * @return the pdo, nu..."
out.print(pdoType);
out.print(source[210]); // 564:20 = " selectCachedOnlyBy"
out.print(suffix);
out.print(source[211]); // 564:49 = "("
out.print(type);
out.print(source[212]); // 564:58 = " "
out.print(name);
out.print(source[213]); // 564:67 = ") { return "
out.print(filterBegin);
out.print(source[214]); // 565:26 = "getCache().select("
out.print(indexMethod);
out.print(source[215]); // 565:59 = "(), getDomainContext(), "
out.print(name);
out.print(source[216]); // 565:91 = ", false)"
out.print(filterEnd);
out.print(source[217]); // 565:112 = "; } /** * Selects via cache by "
out.print(name);
out.print(source[218]); // 569:34 = ". * * @param "
out.print(name);
out.print(source[219]); // 571:21 = " the unique key * @return the pdo, nu..."
out.print(pdoType);
out.print(source[220]); // 575:20 = " selectCachedBy"
out.print(suffix);
out.print(source[221]); // 575:45 = "("
out.print(type);
out.print(source[222]); // 575:54 = " "
out.print(name);
out.print(source[223]); // 575:63 = ") { return "
out.print(filterBegin);
out.print(source[224]); // 576:26 = "getCache().select("
out.print(indexMethod);
out.print(source[225]); // 576:59 = "(), getDomainContext(), "
out.print(name);
out.print(source[226]); // 576:91 = ")"
out.print(filterEnd);
out.print(source[227]); // 576:105 = "; } /** * Selects via remote cac..."
out.print(name);
out.print(source[228]); // 580:41 = ", if session is remote. * * @param..."
out.print(name);
out.print(source[229]); // 582:21 = " the unique key * @return the pdo, nu..."
out.print(pdoType);
out.print(source[230]); // 586:20 = " selectBy"
out.print(suffix);
out.print(source[231]); // 586:39 = "ForCache("
out.print(type);
out.print(source[232]); // 586:56 = " "
out.print(name);
out.print(source[233]); // 586:65 = ") { "
out.print(pdoType);
out.print(source[234]); // 587:15 = " obj; if (getSession().isRemote()) {..."
out.print(suffix);
out.print(source[235]); // 591:52 = "ForCache(context, "
out.print(name);
out.print(source[236]); // 591:78 = "); configureRemoteObject(context..."
out.print(suffix);
out.print(source[237]); // 599:33 = "("
out.print(name);
out.print(source[238]); // 599:42 = "); } return "
out.print(filterBegin);
out.print(source[239]); // 601:26 = "obj"
out.print(filterEnd);
out.print(source[240]); // 601:42 = "; }"
}
if (genudk) {
String indexCast = isAbstract ? ("(PdoCacheIndex) ") : "";
String indexMethod = "getCacheIndexUdk";
out.print(source[241]); // 609:2 = " /** * Gets the index for the uniqu..."
out.print(udkType);
out.print(source[242]); // 614:39 = " "
out.print(udkName);
out.print(source[243]); // 614:51 = " */"
if (isAbstract) {
out.print(source[244]); // 618:2 = " @SuppressWarnings("unchecked")"
}
out.print(source[245]); // 622:2 = " protected PdoCacheIndex<"
out.print(pdoType);
out.print(source[246]); // 623:37 = ", "
out.print(udkType);
out.print(source[247]); // 623:50 = "> "
out.print(indexMethod);
out.print(source[248]); // 623:67 = "() { return "
out.print(indexCast);
out.print(source[249]); // 624:24 = "CacheHolder.UDK_INDEX; } /** * S..."
out.print(udkName);
out.print(source[250]); // 630:24 = " the unique domain key * @return the ..."
out.print(pdoType);
out.print(source[251]); // 634:20 = " selectCachedOnlyByUniqueDomainKey("
out.print(udkType);
out.print(source[252]); // 634:66 = " "
out.print(udkName);
out.print(source[253]); // 634:78 = ") { return "
out.print(filterBegin);
out.print(source[254]); // 635:26 = "getCache().select("
out.print(indexMethod);
out.print(source[255]); // 635:59 = "(), getDomainContext(), "
out.print(udkName);
out.print(source[256]); // 635:94 = ", false)"
out.print(filterEnd);
out.print(source[257]); // 635:115 = "; } /** * Selects via cache by u..."
out.print(udkName);
out.print(source[258]); // 641:24 = " the unique key * @return the pdo, nu..."
out.print(pdoType);
out.print(source[259]); // 645:20 = " selectCachedByUniqueDomainKey("
out.print(udkType);
out.print(source[260]); // 645:62 = " "
out.print(udkName);
out.print(source[261]); // 645:74 = ") { return "
out.print(filterBegin);
out.print(source[262]); // 646:26 = "getCache().select("
out.print(indexMethod);
out.print(source[263]); // 646:59 = "(), getDomainContext(), "
out.print(udkName);
out.print(source[264]); // 646:94 = ")"
out.print(filterEnd);
out.print(source[265]); // 646:108 = "; } /** * Selects via remote cac..."
out.print(udkName);
out.print(source[266]); // 652:24 = " the unique key * @return the pdo, nu..."
out.print(pdoType);
out.print(source[267]); // 656:20 = " selectByUniqueDomainKeyForCache("
out.print(udkType);
out.print(source[268]); // 656:64 = " "
out.print(udkName);
out.print(source[269]); // 656:76 = ") { "
out.print(pdoType);
out.print(source[270]); // 657:15 = " obj; if (getSession().isRemote()) {..."
out.print(udkName);
out.print(source[271]); // 661:86 = "); configureRemoteObject(context..."
out.print(udkName);
out.print(source[272]); // 669:50 = "); } return "
out.print(filterBegin);
out.print(source[273]); // 671:26 = "obj"
out.print(filterEnd);
out.print(source[274]); // 671:42 = "; }"
}
out.print(source[275]); // 676:2 = " @Override public List<"
out.print(pdoType);
out.print(source[276]); // 679:25 = "> selectAllCached() { return "
out.print(filterBegin);
out.print(source[277]); // 680:26 = "getCache().selectAll(getDomainContext())..."
out.print(filterEnd);
out.print(source[278]); // 680:79 = "; }"
}
// ----------------- end wurblet code -----------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy