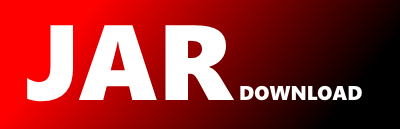
org.tentackle.persist.wurblet.RemoteMethod Maven / Gradle / Ivy
// wurblet generated by Wurbelizer 21.6.0.0, see https://wurbelizer.org
package org.tentackle.persist.wurblet;
import org.tentackle.wurblet.*;
import org.tentackle.buildsupport.*;
import java.util.*;
import java.io.*;
import org.tentackle.common.*;
import org.wurbelizer.wurbel.*;
import org.tentackle.sql.*;
import org.tentackle.model.*;
import org.wurbelizer.wurblet.*;
/**
* ({@code @wurblet}) Generates the remote delegate invocation code.
*
* In order for this wurblet to work, the method must be annotated with @RemoteMethod.
*
* usage:
* @wurblet <tag> RemoteMethod [--this] [--nocontext] [--instance=<object>]
*
* arguments:
*
* - --this: add the PDO itself to the method parameters.
* - --nocontext: don't pass the DomainContext.
* - --instance=<object>: object to invoke the method on in the remote implementation.
*
* For more options, see {@link DbModelWurblet}.
*/
public class RemoteMethod extends DbModelWurblet {
public RemoteMethod() {
setConfiguration("missingModelOk");
}
@Override
public void run() throws WurbelException {
super.run();
try {
wurbel();
}
catch (Throwable t) {
if (t instanceof WurbelException) {
throw (WurbelException) t;
}
throw new WurbelException("wurblet " + this + " failed", t);
}
}
// ----------------- begin wurblet code -----------------
private void wurbel() throws WurbelException {
String instanceName = null;
boolean addThis = false;
boolean noContext = false;
for (String arg: getOptionArgs()) {
if (arg.startsWith("instance=")) {
instanceName = arg.substring(9);
}
else if (arg.equals("this")) {
addThis = true;
}
else if (arg.equals("nocontext")) {
noContext = true;
}
}
String methodName = getMethodName();
String className = getClassName();
if (className == null) {
throw new WurbelException("can't determine ");
}
String packageName = getPackageName();
if (packageName == null) {
throw new WurbelException("can't determine ");
}
boolean isPdo = false;
String pdoClassName = null;
try {
pdoClassName = getPdoClassName();
isPdo = true;
}
catch (WurbelException wx) {
// no pdo
}
if (isRemote()) {
// create includes
RemoteIncludes genInc = new RemoteIncludes(this);
PrintStream implOut = genInc.getImplStream();
PrintStream remoteOut = genInc.getRemoteStream();
// load the info file
String infoName = (packageName + "." + className + "." + methodName).replace('.', '/') +
RemoteMethodInfo.INFO_FILE_EXTENSION;
RemoteMethodInfo info = null;
try {
info = RemoteMethodInfo.readInfo(getContainer().getAnalyzeFile(infoName));
}
catch (IOException ex) {
if (getContainer().getAnalyzeFile(AnalyzeProcessor.COMPILE_ERROR_LOG).exists()) {
genInc.discard();
throw new WurbelDiscardException("reading info '" + infoName + "' failed", ex);
}
else {
throw new WurbelException("reading info '" + infoName + "' failed (@RemoteMethod annotation missing?)", ex);
}
}
RemoteMethodHelper helper = new RemoteMethodHelper(info, pdoClassName, addThis);
boolean returningObject = helper.isReturningObject();
boolean returningCollection = helper.isReturningCollection();
boolean returningCursor = helper.isReturningCursor();
boolean returningVoid = helper.isReturningVoid();
// get the real method name
methodName = helper.getMethodName();
if (helper.isStaticMethod()) {
throw new WurbelException("static methods cannot be --remote");
}
// generate strings
String iparms = helper.getInvocationParameterString();
String riparms = helper.getRemoteInvocationParameterString();
String dparms = helper.getDeclarationParameterString();
if (isPdo && !addThis && !noContext) {
if (iparms.length() > 0) {
iparms = "getDomainContext(), " + iparms;
}
else {
iparms = "getDomainContext()";
}
if (dparms.length() > 0) {
dparms = "DomainContext context, " + dparms;
}
else {
dparms = "DomainContext context";
}
}
String returnType = helper.getReturnType();
String genType = helper.getGenericReturnType();
String firstName = helper.getFirstName();
boolean firstIsDb = helper.isFirstInstanceOfDb();
boolean firstIsDomainContext = helper.isFirstInstanceOfDomainContext();
String remoteDelegateName = (pdoClassName != null ? pdoClassName : className) + "RemoteDelegate";
String imethod = "getRemoteDelegate()";
String setDomainContext = "setDomainContext(" + firstName + ")";
String apply = null;
if (returningObject) {
apply = "configureRemoteObject(getDomainContext(), obj)";
}
else if (returningCollection) {
apply = "configureRemoteObjects(getDomainContext(), list)";
}
// always use a fresh pdo because we don't know what the app is really doing with it
String dbObject = "newInstance(" + (firstIsDomainContext ? firstName : "context") + ")";
if (!isPdo || noContext) {
dbObject = "dbObject";
}
if (addThis) {
dbObject = "obj";
}
else if (instanceName != null) {
dbObject = instanceName;
}
out.print(source[0]); // 151:2 = " if (getSession().isRemote()) { ..."
if (returningObject) {
String poType = isGenerified() ? "P" : getClassName();
remoteOut.print(source[1]); // 160:16 = " "
remoteOut.print(returnType);
remoteOut.print(source[2]); // 161:16 = " "
remoteOut.print(methodName);
remoteOut.print(source[3]); // 161:31 = "("
remoteOut.print(dparms);
remoteOut.print(source[4]); // 161:42 = ") throws RemoteException;"
implOut.print(source[5]); // 162:14 = " @Override public "
implOut.print(returnType);
implOut.print(source[6]); // 165:23 = " "
implOut.print(methodName);
implOut.print(source[7]); // 165:38 = "("
implOut.print(dparms);
implOut.print(source[8]); // 165:49 = ") throws RemoteException { try {"
if (firstIsDomainContext) {
implOut.print(source[9]); // 169:2 = " "
implOut.print(setDomainContext);
implOut.print(source[10]); // 170:26 = ";"
}
if (addThis) {
implOut.print(source[11]); // 174:2 = " (("
implOut.print(poType);
implOut.print(source[12]); // 175:18 = ") obj.getPersistenceDelegate()).setOverl..."
}
for (String statement: helper.getUpdateDbInParametersStatements()) {
implOut.print(source[13]); // 180:2 = " "
implOut.print(statement);
implOut.print(source[14]); // 181:19 = ";"
}
implOut.print(source[15]); // 184:2 = " return "
implOut.print(dbObject);
implOut.print(source[16]); // 185:25 = "."
implOut.print(methodName);
implOut.print(source[17]); // 185:40 = "("
implOut.print(riparms);
implOut.print(source[18]); // 185:52 = "); } catch (Exception e) { ..."
out.print(source[19]); // 191:6 = " "
out.print(returnType);
out.print(source[20]); // 192:22 = " obj = "
out.print(imethod);
out.print(source[21]); // 192:40 = "."
out.print(methodName);
out.print(source[22]); // 192:55 = "("
out.print(iparms);
out.print(source[23]); // 192:66 = "); "
out.print(apply);
out.print(source[24]); // 193:17 = "; return obj;"
}
else if (returningCollection) {
remoteOut.print(source[25]); // 201:16 = " "
remoteOut.print(returnType);
remoteOut.print(source[26]); // 202:16 = " "
remoteOut.print(methodName);
remoteOut.print(source[27]); // 202:31 = "("
remoteOut.print(dparms);
remoteOut.print(source[28]); // 202:42 = ") throws RemoteException;"
implOut.print(source[29]); // 203:14 = " @Override public "
implOut.print(returnType);
implOut.print(source[30]); // 206:23 = " "
implOut.print(methodName);
implOut.print(source[31]); // 206:38 = "("
implOut.print(dparms);
implOut.print(source[32]); // 206:49 = ") throws RemoteException { try {"
if (firstIsDomainContext) {
implOut.print(source[33]); // 210:2 = " "
implOut.print(setDomainContext);
implOut.print(source[34]); // 211:26 = ";"
}
if (addThis) {
implOut.print(source[35]); // 215:2 = " obj.setSession(getSession());"
}
for (String statement: helper.getUpdateDbInParametersStatements()) {
implOut.print(source[36]); // 220:2 = " "
implOut.print(statement);
implOut.print(source[37]); // 221:19 = ";"
}
implOut.print(source[38]); // 224:2 = " return "
implOut.print(dbObject);
implOut.print(source[39]); // 225:25 = "."
implOut.print(methodName);
implOut.print(source[40]); // 225:40 = "("
implOut.print(riparms);
implOut.print(source[41]); // 225:52 = "); } catch (Exception e) { ..."
out.print(source[42]); // 231:6 = " "
out.print(returnType);
out.print(source[43]); // 232:22 = " list = "
out.print(imethod);
out.print(source[44]); // 232:41 = "."
out.print(methodName);
out.print(source[45]); // 232:56 = "("
out.print(iparms);
out.print(source[46]); // 232:67 = "); "
out.print(apply);
out.print(source[47]); // 233:17 = "; return list;"
}
else if (returningCursor) {
remoteOut.print(source[48]); // 241:16 = " RemoteResultSetCursor"
remoteOut.print(genType);
remoteOut.print(source[49]); // 242:34 = " "
remoteOut.print(methodName);
remoteOut.print(source[50]); // 242:49 = "("
remoteOut.print(dparms);
remoteOut.print(source[51]); // 242:60 = ") throws RemoteException;"
implOut.print(source[52]); // 243:14 = " @Override public RemoteResultSetCur..."
implOut.print(genType);
implOut.print(source[53]); // 246:41 = " "
implOut.print(methodName);
implOut.print(source[54]); // 246:56 = "("
implOut.print(dparms);
implOut.print(source[55]); // 246:67 = ") throws RemoteException { try {"
if (firstIsDomainContext) {
implOut.print(source[56]); // 250:2 = " "
implOut.print(setDomainContext);
implOut.print(source[57]); // 251:26 = ";"
}
if (addThis) {
implOut.print(source[58]); // 255:2 = " obj.setSession(getSession());"
}
for (String statement: helper.getUpdateDbInParametersStatements()) {
implOut.print(source[59]); // 260:2 = " "
implOut.print(statement);
implOut.print(source[60]); // 261:19 = ";"
}
implOut.print(source[61]); // 264:2 = " return createRemoteResultSetCursor..."
implOut.print(dbObject);
implOut.print(source[62]); // 265:61 = "."
implOut.print(methodName);
implOut.print(source[63]); // 265:76 = "("
implOut.print(riparms);
implOut.print(source[64]); // 265:88 = ")); } catch (Exception e) { ..."
if (isPdo) {
out.print(source[65]); // 274:2 = " return new ResultSetCursor<>(get..."
out.print(imethod);
out.print(source[66]); // 276:32 = "."
out.print(methodName);
out.print(source[67]); // 276:47 = "("
out.print(iparms);
out.print(source[68]); // 276:58 = "));"
}
else {
out.print(source[69]); // 280:2 = " return new ResultSetCursor<>(get..."
out.print(imethod);
out.print(source[70]); // 282:32 = "."
out.print(methodName);
out.print(source[71]); // 282:47 = "("
out.print(iparms);
out.print(source[72]); // 282:58 = "));"
}
}
else if (returningVoid) {
remoteOut.print(source[73]); // 289:16 = " void "
remoteOut.print(methodName);
remoteOut.print(source[74]); // 290:21 = "("
remoteOut.print(dparms);
remoteOut.print(source[75]); // 290:32 = ") throws RemoteException;"
implOut.print(source[76]); // 291:14 = " @Override public void "
implOut.print(methodName);
implOut.print(source[77]); // 294:28 = "("
implOut.print(dparms);
implOut.print(source[78]); // 294:39 = ") throws RemoteException { try {"
if (firstIsDomainContext) {
implOut.print(source[79]); // 298:2 = " "
implOut.print(setDomainContext);
implOut.print(source[80]); // 299:26 = ";"
}
if (addThis) {
implOut.print(source[81]); // 303:2 = " obj.setSession(getSession());"
}
for (String statement: helper.getUpdateDbInParametersStatements()) {
implOut.print(source[82]); // 308:2 = " "
implOut.print(statement);
implOut.print(source[83]); // 309:19 = ";"
}
implOut.print(source[84]); // 312:2 = " "
implOut.print(dbObject);
implOut.print(source[85]); // 313:18 = "."
implOut.print(methodName);
implOut.print(source[86]); // 313:33 = "("
implOut.print(riparms);
implOut.print(source[87]); // 313:45 = "); } catch (Exception e) { ..."
out.print(source[88]); // 319:6 = " "
out.print(imethod);
out.print(source[89]); // 320:19 = "."
out.print(methodName);
out.print(source[90]); // 320:34 = "("
out.print(iparms);
out.print(source[91]); // 320:45 = "); return;"
}
else {
remoteOut.print(source[92]); // 328:16 = " "
remoteOut.print(returnType);
remoteOut.print(source[93]); // 329:16 = " "
remoteOut.print(methodName);
remoteOut.print(source[94]); // 329:31 = "("
remoteOut.print(dparms);
remoteOut.print(source[95]); // 329:42 = ") throws RemoteException;"
implOut.print(source[96]); // 330:14 = " @Override public "
implOut.print(returnType);
implOut.print(source[97]); // 333:23 = " "
implOut.print(methodName);
implOut.print(source[98]); // 333:38 = "("
implOut.print(dparms);
implOut.print(source[99]); // 333:49 = ") throws RemoteException { try {"
if (firstIsDomainContext) {
implOut.print(source[100]); // 337:2 = " "
implOut.print(setDomainContext);
implOut.print(source[101]); // 338:26 = ";"
}
if (addThis) {
implOut.print(source[102]); // 342:2 = " obj.setSession(getSession());"
}
for (String statement: helper.getUpdateDbInParametersStatements()) {
implOut.print(source[103]); // 347:2 = " "
implOut.print(statement);
implOut.print(source[104]); // 348:19 = ";"
}
implOut.print(source[105]); // 351:2 = " return "
implOut.print(dbObject);
implOut.print(source[106]); // 352:25 = "."
implOut.print(methodName);
implOut.print(source[107]); // 352:40 = "("
implOut.print(riparms);
implOut.print(source[108]); // 352:52 = "); } catch (Exception e) { ..."
out.print(source[109]); // 358:6 = " return "
out.print(imethod);
out.print(source[110]); // 359:26 = "."
out.print(methodName);
out.print(source[111]); // 359:41 = "("
out.print(iparms);
out.print(source[112]); // 359:52 = ");"
}
out.print(source[113]); // 362:2 = " } catch (RemoteException e) ..."
}
else {
out.print(source[114]); // 371:2 = " // remote code generation disabled"
}
}
// ----------------- end wurblet code -----------------
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy