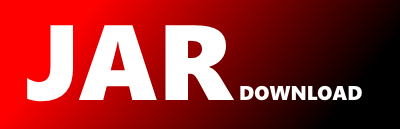
org.tentackle.ns.pdo.NumberRangePersistenceImpl Maven / Gradle / Ivy
/**
* Tentackle - http://www.tentackle.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.tentackle.ns.pdo;
import java.rmi.RemoteException;
import java.util.List;
import org.tentackle.log.Logger;
import org.tentackle.log.LoggerFactory;
import org.tentackle.misc.TrackedList;
import org.tentackle.ns.pdo.rmi.NumberRangeRemoteDelegate;
import org.tentackle.pdo.AbstractPersistentObject;
import org.tentackle.pdo.DomainContext;
import org.tentackle.pdo.ModificationEvent;
import org.tentackle.pdo.Pdo;
import org.tentackle.pdo.PdoCache;
import org.tentackle.pdo.PdoListener;
import org.tentackle.pdo.PdoTracker;
import org.tentackle.pdo.PersistenceException;
import org.tentackle.pdo.Persistent;
import org.tentackle.pdo.PersistentObjectClassVariables;
import org.tentackle.pdo.PersistentObjectService;
import org.tentackle.pdo.Session;
import org.tentackle.persist.PreparedStatementWrapper;
import org.tentackle.persist.ResultSetWrapper;
import org.tentackle.persist.StatementId;
import org.tentackle.sql.Backend;
/**
* Number Space persistence implementation.
*
* @author harald
*/
@PersistentObjectService(NumberRange.class)
public class NumberRangePersistenceImpl extends AbstractPersistentObject implements NumberRangePersistence {
private static final long serialVersionUID = -1178387730540795339L;
/**
* logger for this class.
*/
private static final Logger LOGGER = LoggerFactory.getLogger(NumberRangePersistenceImpl.class);
// @wurblet classVariables ClassVariables
// //GEN-BEGIN:classVariables
/** Variables common to all instances of NumberRangePersistenceImpl. */
@SuppressWarnings("unchecked")
public static final PersistentObjectClassVariables CLASSVARIABLES =
new PersistentObjectClassVariables<>(
NumberRange.class,
NumberRangePersistenceImpl.class,
"nrange",
null,
null);
@Override
public PersistentObjectClassVariables getClassVariables() {
return CLASSVARIABLES;
}
// //GEN-END:classVariables
// @wurblet fieldnames ColumnNames
// //GEN-BEGIN:fieldnames
/** database column name for 'numberPoolId'. */
public static final String CN_NUMBERPOOLID = "poolid";
/** database column name for 'begin'. */
public static final String CN_BEGIN = "rbegin";
/** database column name for 'end'. */
public static final String CN_END = "rend";
// //GEN-END:fieldnames
// @wurblet declare Declare
// //GEN-BEGIN:declare
/** ID of the number pool. */
@Persistent("ID of the number pool")
private long numberPoolId;
/** true if numberPoolId has been modified. */
private boolean numberPoolIdModified;
/** the last persisted value of numberPoolId. */
private long numberPoolIdPersisted;
/** begin of range. */
@Persistent("begin of range")
private long begin;
/** true if begin has been modified. */
private boolean beginModified;
/** the last persisted value of begin. */
private long beginPersisted;
/** end of range. */
@Persistent("end of range")
private long end;
/** true if end has been modified. */
private boolean endModified;
/** the last persisted value of end. */
private long endPersisted;
// //GEN-END:declare
/**
* Creates a number space.
*
* @param pdo the number space PDO
* @param context the domain context
*/
public NumberRangePersistenceImpl (NumberRange pdo, DomainContext context) {
super(pdo, context);
}
/**
* Creates a number space with a session only.
*
* @param pdo the number space PDO
* @param session the session
*/
public NumberRangePersistenceImpl(NumberRange pdo, Session session) {
super(pdo, session);
}
/**
* Creates a number space without domain context or session.
*
* @param pdo the number space PDO
*/
public NumberRangePersistenceImpl(NumberRange pdo) {
super(pdo);
}
/**
* Creates a number space without domain context or session.
*/
public NumberRangePersistenceImpl() {
super();
}
// @wurblet methods MethodsImpl
// //GEN-BEGIN:methods
@Override
public NumberRangeRemoteDelegate getRemoteDelegate() {
return (NumberRangeRemoteDelegate) super.getRemoteDelegate();
}
/**
* The root entity id is numberPoolId.
* @return the ID of the root entity
*/
@Override
public long getRootId() {
return numberPoolId;
}
/**
* The root entity class is NumberPool.
* @return the class ID of the root entity
*/
@Override
public int getRootClassId() {
return 6;
}
/**
* Overridden cause of "--tracked".
* @return true = setters check for modification
*/
@Override
public boolean isTracked() {
return true; // invoking isModified() is ok
}
/**
* Overridden cause of "--attracked".
*/
@Override
public void setModified(boolean modified) {
super.setModified(modified);
if (!modified) {
numberPoolIdModified = false;
numberPoolIdPersisted = numberPoolId;
beginModified = false;
beginPersisted = begin;
endModified = false;
endPersisted = end;
}
}
/**
* Overridden cause of "--fulltracked".
*/
@Override
public boolean differsPersisted() {
return super.differsPersisted()
|| numberPoolIdPersisted != numberPoolId
|| beginPersisted != begin
|| endPersisted != end
;
}
@Override
public void getFields(ResultSetWrapper rs) {
super.getFields(rs);
if (rs.configureSection(CLASSVARIABLES)) {
rs.configureColumn(CN_NUMBERPOOLID);
rs.configureColumn(CN_BEGIN);
rs.configureColumn(CN_END);
rs.configureColumn(CN_ID);
rs.configureColumn(CN_SERIAL);
}
if (rs.getRow() <= 0) {
throw new PersistenceException(getSession(), "no valid row");
}
numberPoolId = rs.getLong();
begin = rs.getLong();
end = rs.getLong();
setId(rs.getLong());
setSerial(rs.getLong());
}
@Override
public int setFields(PreparedStatementWrapper st) {
int ndx = super.setFields(st);
st.setLong(++ndx, numberPoolId);
st.setLong(++ndx, begin);
st.setLong(++ndx, end);
st.setLong(++ndx, getId());
st.setLong(++ndx, getSerial());
return ndx;
}
@Override
public String createInsertSql() {
return Backend.SQL_INSERT_INTO + getTableName() + Backend.SQL_LEFT_PARENTHESIS +
CN_NUMBERPOOLID + Backend.SQL_COMMA +
CN_BEGIN + Backend.SQL_COMMA +
CN_END + Backend.SQL_COMMA +
CN_ID + Backend.SQL_COMMA +
CN_SERIAL +
Backend.SQL_INSERT_VALUES +
Backend.SQL_PAR_COMMA +
Backend.SQL_PAR_COMMA +
Backend.SQL_PAR_COMMA +
Backend.SQL_PAR_COMMA +
Backend.SQL_PAR + Backend.SQL_RIGHT_PARENTHESIS;
}
@Override
public String createUpdateSql() {
return Backend.SQL_UPDATE + getTableName() + Backend.SQL_SET +
CN_NUMBERPOOLID + Backend.SQL_EQUAL_PAR_COMMA +
CN_BEGIN + Backend.SQL_EQUAL_PAR_COMMA +
CN_END + Backend.SQL_EQUAL_PAR_COMMA +
CN_SERIAL + Backend.SQL_EQUAL + CN_SERIAL + Backend.SQL_PLUS_ONE +
Backend.SQL_WHERE + CN_ID + Backend.SQL_EQUAL_PAR +
Backend.SQL_AND + CN_SERIAL + Backend.SQL_EQUAL_PAR;
}
/**
* Gets the attribute numberPoolId.
*
* @return ID of the number pool
*/
@Override
public long getNumberPoolId() {
return numberPoolId;
}
/**
* Sets the attribute numberPoolId.
*
* @param numberPoolId ID of the number pool
*/
private void setNumberPoolId(long numberPoolId) {
assertMutable();
if (this.numberPoolId != numberPoolId) {
setModified(true);
numberPoolIdModified = true;
firePropertyChange(AN_NUMBERPOOLID, this.numberPoolId, numberPoolId);
}
this.numberPoolId = numberPoolId;
}
/**
* Gets the modification state of numberPoolId.
*
* @return true if numberPoolId has been modified
*/
@Override
public boolean isNumberPoolIdModified() {
return numberPoolIdModified;
}
/**
* Gets the last persisted value of numberPoolId.
*
* @return the last persisted value
*/
@Override
public long getNumberPoolIdPersisted() {
return numberPoolIdPersisted;
}
/**
* Gets the attribute begin.
*
* @return begin of range
*/
@Override
public long getBegin() {
return begin;
}
/**
* Sets the attribute begin.
*
* @param begin begin of range
*/
@Override
public void setBegin(long begin) {
assertMutable();
if (this.begin != begin) {
setModified(true);
beginModified = true;
firePropertyChange(AN_BEGIN, this.begin, begin);
}
this.begin = begin;
}
/**
* Gets the modification state of begin.
*
* @return true if begin has been modified
*/
@Override
public boolean isBeginModified() {
return beginModified;
}
/**
* Gets the last persisted value of begin.
*
* @return the last persisted value
*/
@Override
public long getBeginPersisted() {
return beginPersisted;
}
/**
* Gets the attribute end.
*
* @return end of range
*/
@Override
public long getEnd() {
return end;
}
/**
* Sets the attribute end.
*
* @param end end of range
*/
@Override
public void setEnd(long end) {
assertMutable();
if (this.end != end) {
setModified(true);
endModified = true;
firePropertyChange(AN_END, this.end, end);
}
this.end = end;
}
/**
* Gets the modification state of end.
*
* @return true if end has been modified
*/
@Override
public boolean isEndModified() {
return endModified;
}
/**
* Gets the last persisted value of end.
*
* @return the last persisted value
*/
@Override
public long getEndPersisted() {
return endPersisted;
}
/**
* Updates the attributes in snapshot object.
* The snapshot object is assumed to be a clone of this object.
*
* @param snapshot the snapshot
*/
protected void createAttributesInSnapshot(NumberRangePersistenceImpl snapshot) {
super.createAttributesInSnapshot(snapshot);
}
/**
* Copies all attributes from a snapshot back to this object.
*
* @param snapshot the snapshot object
*/
protected void revertAttributesToSnapshot(NumberRangePersistenceImpl snapshot) {
super.revertAttributesToSnapshot(snapshot);
// ID of the number pool
numberPoolId = snapshot.numberPoolId;
numberPoolIdModified = snapshot.numberPoolIdModified;
numberPoolIdPersisted = snapshot.numberPoolIdPersisted;
// begin of range
begin = snapshot.begin;
beginModified = snapshot.beginModified;
beginPersisted = snapshot.beginPersisted;
// end of range
end = snapshot.end;
endModified = snapshot.endModified;
endPersisted = snapshot.endPersisted;
}
// //GEN-END:methods
// @wurblet relations PdoRelations
// //GEN-BEGIN:relations
/**
* # Relations for NumberRange:
*
* NumberRange: NumberPool numberPool via numberPoolId (numberPool)
*/
// NumberRange: NumberPool numberPool via numberPoolId (numberPool)
private transient NumberPool numberPool;
private transient boolean numberPoolLoaded;
/**
* Gets numberPool via numberPoolId.
*
* @return numberPool numberPool
*/
@Override
public NumberPool getNumberPool() {
if (!numberPoolLoaded) {
numberPool = numberPoolId == 0 ? null : on(NumberPool.class).select(numberPoolId);
numberPoolLoaded = true;
}
return numberPool;
}
/**
* Sets numberPool via numberPoolId.
*
* @param numberPool numberPool
*/
@Override
public void setNumberPool(NumberPool numberPool) {
assertMutable();
this.numberPool = numberPool;
setNumberPoolId(numberPool == null ? 0 : numberPool.getId());
numberPoolLoaded = true;
}
/**
* Sets the session in this and all related objects.
*
* @param session the session
*/
@Override
public void setSession(Session session) {
super.setSession(session);
session.applyTo(numberPool);
}
/**
* Sets the context in this and all related objects.
*
* @param context the domain context
*/
@Override
public void setDomainContext(DomainContext context) {
super.setDomainContext(context);
context.applyTo(numberPool);
}
// selects NumberPool: composite list of NumberRange numberRangeList via NumberRange#numberPoolId (numberRange)
// @wurblet selectByNumberPoolId PdoSelectList --tracked numberPoolId
// //GEN-END:relations
// //GEN-BEGIN:selectByNumberPoolId
private static final StatementId SELECT_BY_NUMBER_POOL_ID_STMT = new StatementId();
@Override
public TrackedList selectByNumberPoolId(long numberPoolId) {
if (getSession().isRemote()) {
// invoke remote method
try {
TrackedList list = getRemoteDelegate().selectByNumberPoolId(getDomainContext(), numberPoolId);
configureRemoteObjects(getDomainContext(), list);
return list;
}
catch (RemoteException e) {
throw PersistenceException.createFromRemoteException(this, e);
}
}
// else: local mode
PreparedStatementWrapper st = getPreparedStatement(SELECT_BY_NUMBER_POOL_ID_STMT,
() -> {
StringBuilder sql = createSelectAllInnerSql();
sql.append(Backend.SQL_AND);
sql.append(getColumnName(CN_NUMBERPOOLID));
sql.append(Backend.SQL_EQUAL_PAR);
getBackend().buildSelectSql(sql, false, 0, 0);
return sql.toString();
}
);
int ndx = 1;
st.setLong(ndx++, numberPoolId);
return executeTrackedListQuery(st);
}
// //GEN-END:selectByNumberPoolId
// @wurblet cache PdoCache --preload
// //GEN-BEGIN:cache
/** the cache. */
private static PdoCache cache;
/**
* Creates the cache singleton if it does not exist.
*
* @return the cache singleton
*/
private static synchronized PdoCache createCache() {
if (cache == null) {
cache = Pdo.createPdoCache(NumberRange.class, true, true, false);
PdoTracker.getInstance().addModificationListener(new PdoListener(NumberRange.class) {
@Override
public void dataChanged(ModificationEvent ev) {
cache.expire(ev.getSerial()); // delayed expiration
}
});
}
return cache;
}
@Override
public PdoCache getCache() {
return cache == null ? createCache() : cache;
}
@Override
public boolean isCountingModification(char modType) {
return true;
}
@Override
public boolean isReadAllowed() {
return true;
}
@Override
public boolean expireCache(long maxSerial) {
getCache().expire(maxSerial);
return true;
}
@Override
public NumberRange selectCachedOnly(long id) {
return getCache().select(getDomainContext(), id, false);
}
@Override
public NumberRange selectCached(long id) {
return getCache().select(getDomainContext(), id);
}
@Override
public List selectAllCached() {
return getCache().selectAll(getDomainContext());
}
// //GEN-END:cache
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy