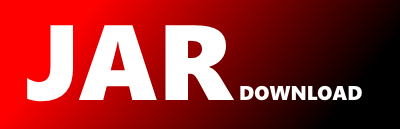
org.tentackle.sql.BackendPreparedStatement Maven / Gradle / Ivy
/*
* Tentackle - http://www.tentackle.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.tentackle.sql;
import java.math.BigDecimal;
import java.sql.Date;
import java.sql.Time;
import java.sql.Timestamp;
import java.util.Calendar;
/**
* Just an interface to decouple dependency from PreparedStatementWrapper.
*
* @author harald
*/
public interface BackendPreparedStatement {
/**
* Sets the designated parameter to the given Java Boolean
value.
* The driver converts this
* to an SQL BIT
or BOOLEAN
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param b the parameter value, null if the value should be set to SQL NULL
*/
void setBoolean(int p, Boolean b);
/**
* Sets the designated parameter to the given Java boolean
value.
* The driver converts this
* to an SQL BIT
or BOOLEAN
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param b the parameter value
*/
void setBoolean(int p, boolean b);
/**
* Sets the designated parameter to the given Java Byte
value.
* The driver converts this
* to an SQL TINYINT
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param b the parameter value, null if the value should be set to SQL NULL
*/
void setByte(int p, Byte b);
/**
* Sets the designated parameter to the given Java byte
value.
* The driver converts this
* to an SQL TINYINT
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param b the parameter value
*/
void setByte(int p, byte b);
/**
* Sets the designated parameter to the given Java char
value.
* The driver converts this
* to an SQL VARCHAR
* when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param c the parameter value
*/
void setChar(int p, char c);
/**
* Sets the designated parameter to the given Java Character
value.
* The driver converts this
* to an SQL VARCHAR
* when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param c the parameter value, null if the value should be set to SQL NULL
*/
void setCharacter(int p, Character c);
/**
* Sets the designated parameter to the given Java Character
value.
* The driver converts this
* to an SQL VARCHAR
* when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param c the parameter value, null if the value should be set to SQL NULL
* @param mapNull true if null values should be mapped to BLANK, else SQL NULL
*/
void setCharacter(int p, Character c, boolean mapNull);
/**
* Sets the designated parameter to the given java.sql.Date
value
* using the default time zone of the virtual machine that is running
* the application.
* The driver converts this
* to an SQL DATE
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param d the parameter value, null if the value should be set to SQL NULL
*/
void setDate(int p, Date d);
/**
* Sets the designated parameter to the given java.sql.Date
value
* using the default time zone of the virtual machine that is running
* the application.
* The driver converts this
* to an SQL DATE
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param d the parameter value
* @param mapNull to map null values to 1.1.1970 (epochal time zero), else SQL NULL
*/
void setDate(int p, Date d, boolean mapNull);
/**
* Sets the designated parameter to the given java.sql.Date
value
* using the default time zone of the virtual machine that is running
* the application.
* The driver converts this
* to an SQL DATE
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param d the parameter value
* @param timezone the calendar providing the timezone configuration
*/
void setDate(int p, Date d, Calendar timezone);
/**
* Sets the designated parameter to the given java.sql.Date
value
* using the default time zone of the virtual machine that is running
* the application.
* The driver converts this
* to an SQL DATE
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param d the parameter value
* @param timezone the calendar providing the timezone configuration
* @param mapNull to map null values to 1.1.1970 (epochal time zero), else SQL NULL
*/
void setDate(int p, Date d, Calendar timezone, boolean mapNull);
/**
* Sets the designated parameter to the given Java Double
value.
* The driver converts this
* to an SQL DOUBLE
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param d the parameter value, null if the value should be set to SQL NULL
*/
void setDouble(int p, Double d);
/**
* Sets the designated parameter to the given Java double
value.
* The driver converts this
* to an SQL DOUBLE
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param d the parameter value
*/
void setDouble(int p, double d);
/**
* Sets the designated parameter to the given Java Float
value.
* The driver converts this
* to an SQL REAL
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param f the parameter value, null if the value should be set to SQL NULL
*/
void setFloat(int p, Float f);
/**
* Sets the designated parameter to the given Java float
value.
* The driver converts this
* to an SQL REAL
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param f the parameter value
*/
void setFloat(int p, float f);
/**
* Sets the designated parameter to the given Java int
value.
* The driver converts this
* to an SQL INTEGER
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param i the parameter value
*/
void setInt(int p, int i);
/**
* Sets the designated parameter to the given Java Integer
value.
* The driver converts this
* to an SQL INTEGER
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param i the parameter value, null if the value should be set to SQL NULL
*/
void setInteger(int p, Integer i);
/**
* Sets the designated parameter to the given Java Long
value.
* The driver converts this
* to an SQL BIGINT
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param l the parameter value, null if the value should be set to SQL NULL
*/
void setLong(int p, Long l);
/**
* Sets the designated parameter to the given Java long
value.
* The driver converts this
* to an SQL BIGINT
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param l the parameter value
*/
void setLong(int p, long l);
/**
* Sets the designated parameter to SQL NULL
.
*
* @param pos the first parameter is 1, the second is 2, ...
* @param type the SQL type code defined in java.sql.Types
*/
void setNull(int pos, int type);
/**
* Sets the designated parameter to the given Java Short
value.
* The driver converts this
* to an SQL SMALLINT
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param s the parameter value, null if the value should be set to SQL NULL
*/
void setShort(int p, Short s);
/**
* Sets the designated parameter to the given Java short
value.
* The driver converts this
* to an SQL SMALLINT
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param s the parameter value
*/
void setShort(int p, short s);
/**
* Sets the designated parameter to the given Java String
value.
* The driver converts this
* to an SQL VARCHAR
or LONGVARCHAR
value
* (depending on the argument's
* size relative to the driver's limits on VARCHAR
values)
* when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param s the parameter value, null if the value should be set to SQL NULL
*/
void setString(int p, String s);
/**
* Sets the designated parameter to the given Java String
value.
* The driver converts this
* to an SQL VARCHAR
or LONGVARCHAR
value
* (depending on the argument's
* size relative to the driver's limits on VARCHAR
values)
* when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param s the parameter value
* @param mapNull true if null values should be mapped to the empty string, else SQL NULL
*/
void setString(int p, String s, boolean mapNull);
/**
* Sets the designated parameter to the given java.sql.Time
value.
* The driver converts this
* to an SQL TIME
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param t the parameter value, null if the value should be set to SQL NULL
*/
void setTime(int p, Time t);
/**
* Sets the designated parameter to the given java.sql.Time
value.
* The driver converts this
* to an SQL TIME
value when it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param t the parameter value, null if the value should be set to SQL NULL
* @param timezone the calendar providing the timezone configuration
*/
void setTime(int p, Time t, Calendar timezone);
/**
* Sets the designated parameter to the given java.sql.Timestamp
value.
* The driver
* converts this to an SQL TIMESTAMP
value when it sends it to the
* database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param ts the parameter value, null if the value should be set to SQL NULL
*/
void setTimestamp(int p, Timestamp ts);
/**
* Sets the designated parameter to the given java.sql.Timestamp
value.
* The driver
* converts this to an SQL TIMESTAMP
value when it sends it to the
* database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param ts the parameter value
* @param mapNull to map null values to 1.1.1970 00:00:00.000 (epochal time zero),
* else SQL NULL
*/
void setTimestamp(int p, Timestamp ts, boolean mapNull);
/**
* Sets the designated parameter to the given java.sql.Timestamp
value.
* The driver
* converts this to an SQL TIMESTAMP
value when it sends it to the
* database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param ts the parameter value
* @param timezone the calendar providing the timezone configuration
* else SQL NULL
*/
void setTimestamp(int p, Timestamp ts, Calendar timezone);
/**
* Sets the designated parameter to the given java.sql.Timestamp
value.
* The driver
* converts this to an SQL TIMESTAMP
value when it sends it to the
* database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param ts the parameter value
* @param timezone the calendar providing the timezone configuration
* @param mapNull to map null values to 1.1.1970 00:00:00.000 (epochal time zero),
* else SQL NULL
*/
void setTimestamp(int p, Timestamp ts, Calendar timezone, boolean mapNull);
/**
* Sets the designated parameter to the given java.math.BigDecimal
value.
* The driver converts this to an SQL NUMERIC
value when
* it sends it to the database.
*
* @param p the first parameter is 1, the second is 2, ...
* @param d the parameter value, null if the value should be set to SQL NULL
*/
void setBigDecimal(int p, BigDecimal d);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy