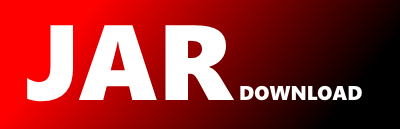
org.tentackle.swing.rdc.AboutDialog Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tentackle-swing-rdc Show documentation
Show all versions of tentackle-swing-rdc Show documentation
Rich Desktop Client based on Tentackle Swing
/**
* Tentackle - http://www.tentackle.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
// Created on February 18, 2004, 12:34 PM
package org.tentackle.swing.rdc;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.text.MessageFormat;
import java.util.StringTokenizer;
import javax.swing.Icon;
import javax.swing.ImageIcon;
/**
* About dialog.
*
* Displays the application's logo (icon), versioning information
* and a memory/GC-button.
*
* @author harald
*/
public class AboutDialog extends org.tentackle.swing.FormDialog {
private static final long serialVersionUID = -3397099233126775727L;
private Thread gcUpdater; // update GC-Button thread
private final String text; // the text
private Icon logo; // logo icon
/**
* Creates an about dialog.
*
* @param text the application text, description
* @param logo the icon logo, null = default icon
*/
public AboutDialog(String text, Icon logo) {
this.text = text;
this.logo = logo;
if (logo == null) {
this.logo = new ImageIcon(AboutDialog.class.getResource("/org/tentackle/swing/rdc/images/loginLogo.png"));
}
setup();
}
/**
* initialization code common to all constructors
*/
private void setup() {
initComponents();
applicationTextArea.setText(text);
tentackleVersionLabel.setText("Tentackle " + org.tentackle.common.Version.RELEASE);
javaVersionLabel.setText("Java " + System.getProperty("java.version"));
Dimension size = imageLabel.getPreferredSize();
int width = size.width;
int height = size.height;
layeredPane.setPreferredSize(size);
imageLabel.setBounds(0, 0, width, height);
logoPanel.setBounds(0, 0, width, height);
int logoWidth = logo.getIconWidth();
int logoHeight = logo.getIconHeight();
logoLabel.setIcon(logo);
logoLabel.setBounds((width/2 - logoWidth)/2, (height - logoHeight)/2, logoWidth, logoHeight);
// the first word of "text" becomes part of the dialog title
StringTokenizer stok = new StringTokenizer(text);
if (stok.hasMoreTokens()) {
setTitle(MessageFormat.format(RdcSwingRdcBundle.getString("ABOUT {0}"), stok.nextToken()));
}
gcUpdater = new Thread("AboutDialog GC Info Updater") {
@Override
public void run() {
while (!isInterrupted()) {
try {
sleep(1000);
}
catch (InterruptedException ex) {
return; // stop thread
}
EventQueue.invokeLater(() -> gcButton.refresh());
}
}
};
pack();
gcUpdater.start();
}
/** This method is called from within the constructor to
* initialize the form.
* WARNING: Do NOT modify this code. The content of this method is
* always regenerated by the Form Editor.
*/
// //GEN-BEGIN:initComponents
private void initComponents() {
layeredPane = new javax.swing.JLayeredPane();
imageLabel = new javax.swing.JLabel();
infoPanel = new org.tentackle.swing.FormPanel();
applicationTextArea = new org.tentackle.swing.FormTextArea();
tentackleVersionLabel = new javax.swing.JLabel();
javaVersionLabel = new javax.swing.JLabel();
gcButton = new org.tentackle.swing.GCButton();
closeButton = new org.tentackle.swing.FormButton();
jLabel1 = new org.tentackle.swing.FormLabel();
logoPanel = new org.tentackle.swing.FormPanel();
logoLabel = new javax.swing.JLabel();
setAutoPosition(true);
addWindowListener(new java.awt.event.WindowAdapter() {
public void windowClosing(java.awt.event.WindowEvent evt) {
formWindowClosing(evt);
}
});
imageLabel.setIcon(new javax.swing.ImageIcon(getClass().getResource("/org/tentackle/swing/rdc/images/login.png"))); // NOI18N
layeredPane.add(imageLabel);
imageLabel.setBounds(0, 0, 500, 350);
infoPanel.setOpaque(false);
applicationTextArea.setChangeable(false);
applicationTextArea.setForeground(java.awt.Color.orange);
applicationTextArea.setFont(new java.awt.Font("Dialog", 1, 12)); // NOI18N
tentackleVersionLabel.setForeground(new java.awt.Color(204, 204, 255));
tentackleVersionLabel.setText("Tentackle Build xxx");
javaVersionLabel.setForeground(new java.awt.Color(204, 204, 255));
javaVersionLabel.setText("java xxx");
gcButton.setBorder(null);
gcButton.setForeground(new java.awt.Color(204, 204, 255));
gcButton.setText("? / ? M");
gcButton.setBorderPainted(false);
gcButton.setFocusable(false);
gcButton.setFont(new java.awt.Font("Dialog", 0, 12)); // NOI18N
gcButton.setName("gc"); // NOI18N
closeButton.setBackground(new java.awt.Color(204, 204, 255));
closeButton.setText(RdcSwingRdcBundle.getTranslation("CLOSE")); // NOI18N
closeButton.setMargin(new java.awt.Insets(1, 4, 1, 4));
closeButton.setName("close"); // NOI18N
closeButton.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(java.awt.event.ActionEvent evt) {
closeButtonActionPerformed(evt);
}
});
jLabel1.setForeground(new java.awt.Color(204, 204, 255));
jLabel1.setText(RdcSwingRdcBundle.getTranslation("MEMORY:")); // NOI18N
javax.swing.GroupLayout infoPanelLayout = new javax.swing.GroupLayout(infoPanel);
infoPanel.setLayout(infoPanelLayout);
infoPanelLayout.setHorizontalGroup(
infoPanelLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(infoPanelLayout.createSequentialGroup()
.addContainerGap(276, Short.MAX_VALUE)
.addGroup(infoPanelLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, infoPanelLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addComponent(tentackleVersionLabel)
.addComponent(applicationTextArea, javax.swing.GroupLayout.PREFERRED_SIZE, 212, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(javaVersionLabel)
.addGroup(infoPanelLayout.createSequentialGroup()
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(gcButton, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)))
.addComponent(closeButton, javax.swing.GroupLayout.Alignment.TRAILING, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addContainerGap())
);
infoPanelLayout.setVerticalGroup(
infoPanelLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(javax.swing.GroupLayout.Alignment.TRAILING, infoPanelLayout.createSequentialGroup()
.addContainerGap(98, Short.MAX_VALUE)
.addComponent(applicationTextArea, javax.swing.GroupLayout.PREFERRED_SIZE, 97, javax.swing.GroupLayout.PREFERRED_SIZE)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(tentackleVersionLabel)
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)
.addComponent(javaVersionLabel)
.addGap(7, 7, 7)
.addGroup(infoPanelLayout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(jLabel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(gcButton, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(56, 56, 56)
.addComponent(closeButton, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap())
);
layeredPane.add(infoPanel);
infoPanel.setBounds(0, 0, 500, 350);
layeredPane.setLayer(infoPanel, javax.swing.JLayeredPane.PALETTE_LAYER);
logoPanel.setOpaque(false);
logoPanel.setLayout(null);
logoLabel.setFont(new java.awt.Font("SansSerif", 1, 12)); // NOI18N
logoPanel.add(logoLabel);
logoLabel.setBounds(45, 60, 140, 200);
layeredPane.add(logoPanel);
logoPanel.setBounds(0, 0, 500, 350);
layeredPane.setLayer(logoPanel, javax.swing.JLayeredPane.MODAL_LAYER);
getContentPane().add(layeredPane, java.awt.BorderLayout.CENTER);
}// //GEN-END:initComponents
private void closeButtonActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_closeButtonActionPerformed
dispose();
}//GEN-LAST:event_closeButtonActionPerformed
private void formWindowClosing(java.awt.event.WindowEvent evt) {//GEN-FIRST:event_formWindowClosing
gcUpdater.interrupt();
}//GEN-LAST:event_formWindowClosing
// Variables declaration - do not modify//GEN-BEGIN:variables
private org.tentackle.swing.FormTextArea applicationTextArea;
private org.tentackle.swing.FormButton closeButton;
private org.tentackle.swing.GCButton gcButton;
private javax.swing.JLabel imageLabel;
private org.tentackle.swing.FormPanel infoPanel;
private org.tentackle.swing.FormLabel jLabel1;
private javax.swing.JLabel javaVersionLabel;
private javax.swing.JLayeredPane layeredPane;
private javax.swing.JLabel logoLabel;
private org.tentackle.swing.FormPanel logoPanel;
private javax.swing.JLabel tentackleVersionLabel;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy