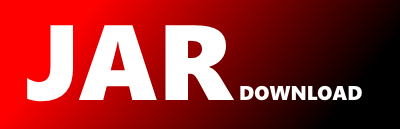
org.tentackle.wurblet.Methods Maven / Gradle / Ivy
// wurblet generated by Wurbelizer 2.0.6, see http://www.wurbelizer.org
package org.tentackle.wurblet;
import org.tentackle.buildsupport.*;
import java.util.*;
import java.io.*;
import org.tentackle.common.*;
import org.wurbelizer.wurbel.*;
import org.tentackle.model.*;
import org.wurbelizer.wurblet.*;
public class Methods extends ModelWurblet {
@Override
public void run() throws WurbelException {
super.run();
try {
// ----------------- begin wurblet code -----------------
/**
* Tentackle - http://www.tentackle.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
/**
* create a getter and setter methods for pdo interfaces.
* usage: @wurblet Methods --model= [--noudk]
*
* --noudk don't generate selectByUniqueDomainKey method definition
*
* Example: @wurblet methods Methods --model=$scripts/artikel.map
*/
boolean generateSelectByUDK = getOption("noudk") == null;
for (Attribute attr: getEntity().getAttributes()) {
if (attr.getOptions().getAccessScope() != AccessScope.PUBLIC ||
attr.getOptions().isFromSuper()) {
continue; // skip
}
if (attr.getOptions().isNoMethod()) {
out.print(source[0]); // 43:2 = " // no accessor methods for "
out.print(attr);
out.print(source[1]); // 45:37 = " // "
out.print(attr.getOptions().getComment());
out.print(source[2]); // 46:39 = ""
}
else {
String type = attr.getJavaType();
String methodSuffix = attr.getMethodNameSuffix();
String getter = attr.getGetterName();
String setter = attr.getSetterName();
if (attr.getOptions().isWriteOnly()) {
out.print(source[3]); // 55:2 = " // "
out.print(attr);
out.print(source[4]); // 57:13 = " is writeonly, no getter."
}
else {
out.print(source[5]); // 61:2 = " /** * Gets the attribute "
out.print(attr);
out.print(source[6]); // 64:32 = ". * * @return "
out.print(attr.getOptions().getComment());
out.print(source[7]); // 66:48 = " */ @Persistent("
out.print(BasicStringHelper.toDoubleQuotes(attr.getOptions().getComment()));
out.print(source[8]); // 68:83 = ")"
for (String annotation: attr.getOptions().getAnnotations()) {
out.print(source[9]); // 71:2 = " "
out.print(annotation);
out.print(source[10]); // 72:16 = ""
}
if (attr.getOptions().isBind()) {
out.print(source[11]); // 76:2 = " "
out.print(attr.getBindableAnnotation());
out.print(source[12]); // 77:34 = ""
}
if (attr.getOptions().isDomainKey()) {
out.print(source[13]); // 81:2 = " @DomainKey"
}
out.print(source[14]); // 85:2 = " "
out.print(type);
out.print(source[15]); // 86:10 = " "
out.print(getter);
out.print(source[16]); // 86:21 = "();"
}
if (attr.getOptions().isReadOnly()) {
out.print(source[17]); // 91:2 = " // "
out.print(attr);
out.print(source[18]); // 93:13 = " is readonly, no setter."
}
else {
// check to make setter for lazy or eager object relations hidden to the application
Relation rel = attr.getRelation();
if (rel == null || rel.getSelectionType() == SelectionType.ALWAYS) {
out.print(source[19]); // 100:2 = " /** * Sets the attribute "
out.print(attr);
out.print(source[20]); // 103:32 = ". * * @param "
out.print(attr.getJavaName());
out.print(source[21]); // 105:35 = " "
out.print(attr.getOptions().getComment());
out.print(source[22]); // 105:70 = " */"
if (attr.getOptions().isBind()) {
out.print(source[23]); // 109:2 = " @Bindable"
}
out.print(source[24]); // 113:2 = " void "
out.print(setter);
out.print(source[25]); // 114:17 = "("
out.print(type);
out.print(source[26]); // 114:26 = " "
out.print(attr.getJavaName());
out.print(source[27]); // 114:49 = ");"
}
}
if (getEntity().getOptions().getTrackType().isAttracked()) {
out.print(source[28]); // 119:2 = " /** * Gets the modification state ..."
out.print(attr);
out.print(source[29]); // 122:44 = ". * * @return true if modified ..."
out.print(methodSuffix);
out.print(source[30]); // 126:28 = "Modified();"
}
if (getEntity().getOptions().getTrackType() == TrackType.FULLTRACKED) {
out.print(source[31]); // 130:2 = " /** * Gets the last persisted valu..."
out.print(attr);
out.print(source[32]); // 133:46 = ". * * @return the last persisted v..."
out.print(attr.getJavaType());
out.print(source[33]); // 137:24 = " "
out.print(getter);
out.print(source[34]); // 137:35 = "Persisted();"
}
if (attr.getOptions().isWithTimezone()) {
out.print(source[35]); // 141:2 = " /** * Gets the database timezone f..."
out.print(attr);
out.print(source[36]); // 144:44 = ". * * @return the timezone configu..."
out.print(methodSuffix);
out.print(source[37]); // 148:30 = "TimezoneConfig();"
}
}
}
if (isPdo() && generateSelectByUDK) {
List udk = getEntity().getUniqueDomainKey();
if (!udk.isEmpty()) {
List superUdk = null;
if (getEntity().getSuperEntity() != null) {
superUdk = getEntity().getSuperEntity().getUniqueDomainKey();
}
if (superUdk == null || !udk.equals(superUdk)) {
out.print(source[38]); // 162:2 = " /** * Selects "
out.print(getEntity());
out.print(source[39]); // 165:28 = " by its unique domain key. *"
String returnType = isGenerified() ? "T" : getPdoClassName();
StringBuilder params = new StringBuilder();
for (Attribute attr: udk) {
appendCommaSeparated(params, attr.getJavaType());
params.append(' ').append(attr.getJavaName());
out.print(source[40]); // 173:2 = " * @param "
out.print(attr.getJavaName());
out.print(source[41]); // 174:35 = " "
out.print(attr.getOptions().getComment());
out.print(source[42]); // 174:70 = ""
}
out.print(source[43]); // 177:2 = " * @return the "
out.print(getEntity());
out.print(source[44]); // 178:33 = ", null if no such PDO */ "
out.print(returnType);
out.print(source[45]); // 180:16 = " selectByUniqueDomainKey("
out.print(params);
out.print(source[46]); // 180:51 = ");"
}
}
}
// ----------------- end wurblet code -----------------
}
catch (Exception ex) {
throw new WurbelException("wurblet " + this + " failed", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy