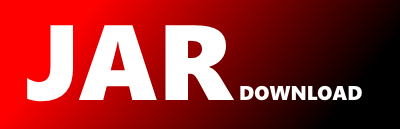
org.tentackle.wurblet.ModelComment Maven / Gradle / Ivy
// wurblet generated by Wurbelizer 2.0.6, see http://www.wurbelizer.org
package org.tentackle.wurblet;
import org.tentackle.buildsupport.*;
import java.util.*;
import java.io.*;
import org.tentackle.common.*;
import org.wurbelizer.wurbel.*;
import org.tentackle.model.*;
import org.wurbelizer.wurblet.*;
public class ModelComment extends ModelWurblet {
@Override
public void run() throws WurbelException {
super.run();
try {
// ----------------- begin wurblet code -----------------
/**
* Tentackle - http://www.tentackle.org
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
/**
* generate a comment to document the relations of the entity.
*
* usage: @wurblet ModelComment --model=
*
* is the mapping description
*
* Example: @wurblet relations Relations --model=$mapping
*/
// create comment for referencing relations
List referencingRelations = getEntity().getReferencingRelationsIncludingInherited();
List subEntities = getEntity().getSubEntities();
Set> compositePaths = getEntity().getTopSuperEntity().getCompositePaths();
List compositeRelations = new ArrayList<>();
for (Relation relation: getEntity().getRelationsIncludingInherited()) {
if (relation.isComposite()) {
compositeRelations.add(relation);
}
}
out.print(source[0]); // 43:2 = " /************************************..."
List refRels = new ArrayList<>(); // referencing relations without backlinks from components
for (Relation rel: referencingRelations) {
Relation foreignRelation = rel.getForeignRelation();
// don't print composite back-relations
if (foreignRelation == null || !foreignRelation.getEntity().equals(getEntity()) ||
!foreignRelation.isComposite()) {
refRels.add(rel);
}
}
if (refRels.isEmpty()) {
out.print(source[1]); // 59:2 = " * "
out.print(getEntity());
out.print(source[2]); // 60:20 = " is not referenced by any other entity"
}
else {
out.print(source[3]); // 64:2 = " * "
out.print(getEntity());
out.print(source[4]); // 65:20 = " is referenced by: *"
}
for (Relation rel: refRels) {
ModelCommentSupport.printReferencedBy(rel, " * ", out);
}
if (getEntity().isRootEntityAccordingToModel() ||
getEntity().getOptions().isRootEntity()) {
out.print(source[5]); // 75:2 = " * * * "
out.print(getEntity());
out.print(source[6]); // 78:20 = " is a root entity"
}
if (!compositeRelations.isEmpty()) {
out.print(source[7]); // 83:2 = " * * * "
out.print(getEntity());
out.print(source[8]); // 86:20 = " is a composite with the components:"
ModelCommentSupport.printComponents(compositeRelations, subEntities, " * ", out);
}
List outRels = new ArrayList<>();
Set components = new HashSet<>();
components.add(getEntity());
components.addAll(getEntity().getSuperEntities());
for (Entity component: getEntity().getAllComponents()) {
components.add(component);
components.addAll(component.getSuperEntities());
}
for (Entity component: components) {
for (Relation relation: component.getRelationsIncludingSubEntities()) {
if (!relation.isComposite() && !components.contains(relation.getForeignEntity())) {
outRels.add(relation);
}
}
}
if (outRels.isEmpty()) {
out.print(source[9]); // 108:2 = " * * * "
out.print(getEntity());
out.print(source[10]); // 111:20 = " is not referencing other entities"
}
else {
out.print(source[11]); // 115:2 = " * * * "
out.print(getEntity());
out.print(source[12]); // 118:20 = " is referencing the following entities:..."
ModelCommentSupport.printNonCompositeRelations(getEntity(), outRels, " * ", out);
}
if (getEntity().isComposite()) {
List deepRels = getEntity().getDeepReferencesToComponents();
if (deepRels.isEmpty()) {
out.print(source[13]); // 127:2 = " * * * Components of "
out.print(getEntity());
out.print(source[14]); // 130:34 = " are not deeply referenced"
}
else {
out.print(source[15]); // 134:2 = " * * * The following components ..."
out.print(getEntity());
out.print(source[16]); // 137:48 = " are deeply referenced: *"
ModelCommentSupport.printNonCompositeRelations(getEntity(), deepRels, " * ", out);
}
}
if (!compositePaths.isEmpty()) {
out.print(source[17]); // 145:2 = " * *"
Set roots = new TreeSet<>();
Set parents = new TreeSet<>();
for (List compositePath: compositePaths) {
roots.add(compositePath.get(0).getEntity());
parents.add(compositePath.get(compositePath.size() - 1).getEntity());
}
StringBuilder buf = new StringBuilder();
for (Entity parent: parents) {
if (buf.length() > 0) {
buf.append(", ");
}
buf.append(parent);
}
out.print(source[18]); // 162:2 = " * "
out.print(getEntity());
out.print(source[19]); // 163:20 = " is a component of: "
out.print(buf);
out.print(source[20]); // 163:47 = ""
buf = new StringBuilder();
for (Entity root: roots) {
if (buf.length() > 0) {
buf.append(", ");
}
buf.append(root);
}
out.print(source[21]); // 172:2 = " * and belongs to the root "
out.print(roots.size() > 1 ? "entities" : "entity");
out.print(source[22]); // 173:73 = ": "
out.print(buf);
out.print(source[23]); // 173:82 = ""
}
if (subEntities.isEmpty()) {
out.print(source[24]); // 179:2 = " * * * "
out.print(getEntity());
out.print(source[25]); // 182:20 = " is not extended"
}
else {
out.print(source[26]); // 186:2 = " * * * "
out.print(getEntity());
out.print(source[27]); // 189:20 = " is extended by:"
ModelCommentSupport.printSubEntities(subEntities, " * ", out);
}
out.print(source[28]); // 193:2 = " * ********************************..."
// ----------------- end wurblet code -----------------
}
catch (Exception ex) {
throw new WurbelException("wurblet " + this + " failed", ex);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy