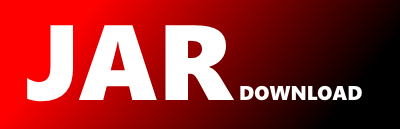
org.plasma.sdo.access.provider.jdo.Property Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-2
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2013.12.19 at 03:35:27 PM EST
//
package org.plasma.sdo.access.provider.jdo;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice minOccurs="0">
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}array"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}collection"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}map"/>
* </choice>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}join" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}embedded" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}element" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}key" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}value" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}order" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}column" maxOccurs="unbounded" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}foreign-key" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}index" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}unique" minOccurs="0"/>
* <element ref="{http://java.sun.com/xml/ns/jdo/jdo}extension" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attGroup ref="{http://java.sun.com/xml/ns/jdo/jdo}attlist.property"/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"map",
"collection",
"array",
"join",
"embeddedElement",
"element",
"key",
"value",
"order",
"columns",
"foreignKey",
"index",
"uniqueElement",
"extensions"
})
@XmlRootElement(name = "property")
public class Property {
protected Map map;
protected Collection collection;
protected Array array;
protected Join join;
@XmlElement(name = "embedded")
protected Embedded embeddedElement;
protected Element element;
protected Key key;
protected Value value;
protected Order order;
@XmlElement(name = "column")
protected List columns;
@XmlElement(name = "foreign-key")
protected ForeignKey foreignKey;
protected Index index;
@XmlElement(name = "unique")
protected Unique uniqueElement;
@XmlElement(name = "extension")
protected List extensions;
@XmlAttribute(name = "name", required = true)
@XmlSchemaType(name = "anySimpleType")
protected String name;
@XmlAttribute(name = "persistence-modifier")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String persistenceModifier;
@XmlAttribute(name = "default-fetch-group")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String defaultFetchGroup;
@XmlAttribute(name = "load-fetch-group")
@XmlSchemaType(name = "anySimpleType")
protected String loadFetchGroup;
@XmlAttribute(name = "null-value")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String nullValue;
@XmlAttribute(name = "dependent")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String dependent;
@XmlAttribute(name = "embedded")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String embedded;
@XmlAttribute(name = "primary-key")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String primaryKey;
@XmlAttribute(name = "value-strategy")
@XmlSchemaType(name = "anySimpleType")
protected String valueStrategy;
@XmlAttribute(name = "sequence")
@XmlSchemaType(name = "anySimpleType")
protected String sequence;
@XmlAttribute(name = "serialized")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String serialized;
@XmlAttribute(name = "field-type")
@XmlSchemaType(name = "anySimpleType")
protected String fieldType;
@XmlAttribute(name = "table")
@XmlSchemaType(name = "anySimpleType")
protected String table;
@XmlAttribute(name = "column")
@XmlSchemaType(name = "anySimpleType")
protected String column;
@XmlAttribute(name = "delete-action")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String deleteAction;
@XmlAttribute(name = "indexed")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String indexed;
@XmlAttribute(name = "unique")
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
protected String unique;
@XmlAttribute(name = "mapped-by")
@XmlSchemaType(name = "anySimpleType")
protected String mappedBy;
@XmlAttribute(name = "recursion-depth")
@XmlSchemaType(name = "anySimpleType")
protected String recursionDepth;
@XmlAttribute(name = "field-name")
@XmlSchemaType(name = "anySimpleType")
protected String fieldName;
/**
* Gets the value of the map property.
*
* @return
* possible object is
* {@link Map }
*
*/
public Map getMap() {
return map;
}
/**
* Sets the value of the map property.
*
* @param value
* allowed object is
* {@link Map }
*
*/
public void setMap(Map value) {
this.map = value;
}
/**
* Gets the value of the collection property.
*
* @return
* possible object is
* {@link Collection }
*
*/
public Collection getCollection() {
return collection;
}
/**
* Sets the value of the collection property.
*
* @param value
* allowed object is
* {@link Collection }
*
*/
public void setCollection(Collection value) {
this.collection = value;
}
/**
* Gets the value of the array property.
*
* @return
* possible object is
* {@link Array }
*
*/
public Array getArray() {
return array;
}
/**
* Sets the value of the array property.
*
* @param value
* allowed object is
* {@link Array }
*
*/
public void setArray(Array value) {
this.array = value;
}
/**
* Gets the value of the join property.
*
* @return
* possible object is
* {@link Join }
*
*/
public Join getJoin() {
return join;
}
/**
* Sets the value of the join property.
*
* @param value
* allowed object is
* {@link Join }
*
*/
public void setJoin(Join value) {
this.join = value;
}
/**
* Gets the value of the embeddedElement property.
*
* @return
* possible object is
* {@link Embedded }
*
*/
public Embedded getEmbeddedElement() {
return embeddedElement;
}
/**
* Sets the value of the embeddedElement property.
*
* @param value
* allowed object is
* {@link Embedded }
*
*/
public void setEmbeddedElement(Embedded value) {
this.embeddedElement = value;
}
/**
* Gets the value of the element property.
*
* @return
* possible object is
* {@link Element }
*
*/
public Element getElement() {
return element;
}
/**
* Sets the value of the element property.
*
* @param value
* allowed object is
* {@link Element }
*
*/
public void setElement(Element value) {
this.element = value;
}
/**
* Gets the value of the key property.
*
* @return
* possible object is
* {@link Key }
*
*/
public Key getKey() {
return key;
}
/**
* Sets the value of the key property.
*
* @param value
* allowed object is
* {@link Key }
*
*/
public void setKey(Key value) {
this.key = value;
}
/**
* Gets the value of the value property.
*
* @return
* possible object is
* {@link Value }
*
*/
public Value getValue() {
return value;
}
/**
* Sets the value of the value property.
*
* @param value
* allowed object is
* {@link Value }
*
*/
public void setValue(Value value) {
this.value = value;
}
/**
* Gets the value of the order property.
*
* @return
* possible object is
* {@link Order }
*
*/
public Order getOrder() {
return order;
}
/**
* Sets the value of the order property.
*
* @param value
* allowed object is
* {@link Order }
*
*/
public void setOrder(Order value) {
this.order = value;
}
/**
* Gets the value of the columns property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the columns property.
*
*
* For example, to add a new item, do as follows:
*
* getColumns().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Column }
*
*
*/
public List getColumns() {
if (columns == null) {
columns = new ArrayList();
}
return this.columns;
}
/**
* Gets the value of the foreignKey property.
*
* @return
* possible object is
* {@link ForeignKey }
*
*/
public ForeignKey getForeignKey() {
return foreignKey;
}
/**
* Sets the value of the foreignKey property.
*
* @param value
* allowed object is
* {@link ForeignKey }
*
*/
public void setForeignKey(ForeignKey value) {
this.foreignKey = value;
}
/**
* Gets the value of the index property.
*
* @return
* possible object is
* {@link Index }
*
*/
public Index getIndex() {
return index;
}
/**
* Sets the value of the index property.
*
* @param value
* allowed object is
* {@link Index }
*
*/
public void setIndex(Index value) {
this.index = value;
}
/**
* Gets the value of the uniqueElement property.
*
* @return
* possible object is
* {@link Unique }
*
*/
public Unique getUniqueElement() {
return uniqueElement;
}
/**
* Sets the value of the uniqueElement property.
*
* @param value
* allowed object is
* {@link Unique }
*
*/
public void setUniqueElement(Unique value) {
this.uniqueElement = value;
}
/**
* Gets the value of the extensions property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the extensions property.
*
*
* For example, to add a new item, do as follows:
*
* getExtensions().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Extension }
*
*
*/
public List getExtensions() {
if (extensions == null) {
extensions = new ArrayList();
}
return this.extensions;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the persistenceModifier property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPersistenceModifier() {
return persistenceModifier;
}
/**
* Sets the value of the persistenceModifier property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPersistenceModifier(String value) {
this.persistenceModifier = value;
}
/**
* Gets the value of the defaultFetchGroup property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDefaultFetchGroup() {
return defaultFetchGroup;
}
/**
* Sets the value of the defaultFetchGroup property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDefaultFetchGroup(String value) {
this.defaultFetchGroup = value;
}
/**
* Gets the value of the loadFetchGroup property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLoadFetchGroup() {
return loadFetchGroup;
}
/**
* Sets the value of the loadFetchGroup property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLoadFetchGroup(String value) {
this.loadFetchGroup = value;
}
/**
* Gets the value of the nullValue property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNullValue() {
if (nullValue == null) {
return "none";
} else {
return nullValue;
}
}
/**
* Sets the value of the nullValue property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNullValue(String value) {
this.nullValue = value;
}
/**
* Gets the value of the dependent property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDependent() {
return dependent;
}
/**
* Sets the value of the dependent property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDependent(String value) {
this.dependent = value;
}
/**
* Gets the value of the embedded property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getEmbedded() {
return embedded;
}
/**
* Sets the value of the embedded property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setEmbedded(String value) {
this.embedded = value;
}
/**
* Gets the value of the primaryKey property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPrimaryKey() {
if (primaryKey == null) {
return "false";
} else {
return primaryKey;
}
}
/**
* Sets the value of the primaryKey property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPrimaryKey(String value) {
this.primaryKey = value;
}
/**
* Gets the value of the valueStrategy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getValueStrategy() {
return valueStrategy;
}
/**
* Sets the value of the valueStrategy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setValueStrategy(String value) {
this.valueStrategy = value;
}
/**
* Gets the value of the sequence property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSequence() {
return sequence;
}
/**
* Sets the value of the sequence property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSequence(String value) {
this.sequence = value;
}
/**
* Gets the value of the serialized property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSerialized() {
return serialized;
}
/**
* Sets the value of the serialized property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSerialized(String value) {
this.serialized = value;
}
/**
* Gets the value of the fieldType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFieldType() {
return fieldType;
}
/**
* Sets the value of the fieldType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFieldType(String value) {
this.fieldType = value;
}
/**
* Gets the value of the table property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTable() {
return table;
}
/**
* Sets the value of the table property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTable(String value) {
this.table = value;
}
/**
* Gets the value of the column property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getColumn() {
return column;
}
/**
* Sets the value of the column property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setColumn(String value) {
this.column = value;
}
/**
* Gets the value of the deleteAction property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDeleteAction() {
return deleteAction;
}
/**
* Sets the value of the deleteAction property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDeleteAction(String value) {
this.deleteAction = value;
}
/**
* Gets the value of the indexed property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIndexed() {
return indexed;
}
/**
* Sets the value of the indexed property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIndexed(String value) {
this.indexed = value;
}
/**
* Gets the value of the unique property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUnique() {
return unique;
}
/**
* Sets the value of the unique property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUnique(String value) {
this.unique = value;
}
/**
* Gets the value of the mappedBy property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getMappedBy() {
return mappedBy;
}
/**
* Sets the value of the mappedBy property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setMappedBy(String value) {
this.mappedBy = value;
}
/**
* Gets the value of the recursionDepth property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRecursionDepth() {
return recursionDepth;
}
/**
* Sets the value of the recursionDepth property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRecursionDepth(String value) {
this.recursionDepth = value;
}
/**
* Gets the value of the fieldName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFieldName() {
return fieldName;
}
/**
* Sets the value of the fieldName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFieldName(String value) {
this.fieldName = value;
}
}