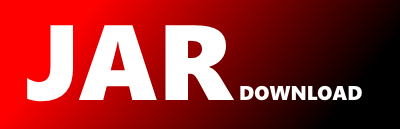
org.plasma.query.model.Expression Maven / Gradle / Ivy
//
/**
* PlasmaSDO™ License
*
* This is a community release of PlasmaSDO™, a dual-license
* Service Data Object (SDO) 2.1 implementation.
* This particular copy of the software is released under the
* version 2 of the GNU General Public License. PlasmaSDO™ was developed by
* TerraMeta Software, Inc.
*
* Copyright (c) 2013, TerraMeta Software, Inc. All rights reserved.
*
* General License information can be found below.
*
* This distribution may include materials developed by third
* parties. For license and attribution notices for these
* materials, please refer to the documentation that accompanies
* this distribution (see the "Licenses for Third-Party Components"
* appendix) or view the online documentation at
* .
*
*/
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-661
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2011.04.11 at 02:47:51 PM EDT
//
package org.plasma.query.model;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.jaxen.expr.BinaryExpr;
import org.jaxen.expr.EqualityExpr;
import org.jaxen.expr.LiteralExpr;
import org.jaxen.expr.LocationPath;
import org.jaxen.expr.LogicalExpr;
import org.jaxen.expr.NameStep;
import org.jaxen.expr.NumberExpr;
import org.jaxen.expr.RelationalExpr;
import org.plasma.query.visitor.DefaultQueryVisitor;
import org.plasma.query.visitor.QueryVisitor;
import org.plasma.query.visitor.Traversal;
/**
* Java class for Expression complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Expression">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element ref="{http://www.terrameta.org/plasma/query}Term" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Expression", propOrder = {
"terms"
})
@XmlRootElement(name = "Expression")
public class Expression implements org.plasma.query.Expression {
private final static String SYNTHETIC_PARENT_ID = "synthetic";
public final static String NOOP_EXPR_ID = "NO_OP";
private static transient Log log = LogFactory.getLog(Expression.class);
protected transient Expression parent;
@XmlAttribute
protected String id;
public Expression getParent() {
return this.parent;
}
@Override
public org.plasma.query.Expression and(org.plasma.query.Expression other) {
return this.cat(other, LogicalOperatorValues.AND);
}
@Override
public org.plasma.query.Expression or(org.plasma.query.Expression other) {
return this.cat(other, LogicalOperatorValues.OR);
}
@Override
public org.plasma.query.Expression group() {
// we may have been re-parented
Expression root = this;
while (root.getParent() != null) {
root = root.getParent();
}
root.getTerms().add(0,
new Term(new GroupOperator(GroupOperatorValues.RP_1)));
root.getTerms().add(
new Term(new GroupOperator(GroupOperatorValues.LP_1)));
return this;
}
public boolean isGroup() {
if (this.getTerms().size() > 0) {
GroupOperator left = this.getTerms().get(0).getGroupOperator();
if (left != null && left.getValue().ordinal() == GroupOperatorValues.RP_1.ordinal()) {
GroupOperator right = this.getTerms().get(this.getTerms().size()-1).getGroupOperator();
if (right != null && right.getValue().ordinal() == GroupOperatorValues.LP_1.ordinal()) {
return true;
}
}
}
return false;
}
private Expression cat(org.plasma.query.Expression other, LogicalOperatorValues oper) {
Expression result = null;
if (this.parent == null) {
Expression parent = findParent();
if (parent == null) {
if (log.isDebugEnabled())
log.debug("creating synthetic expr parent for concatination");
parent = new Expression();
parent.id = SYNTHETIC_PARENT_ID;
((Expression)other).parent = parent;
this.parent = parent;
parent.getTerms().add(new Term(this));
parent.getTerms().add(new Term(
new LogicalOperator(oper)));
parent.getTerms().add(new Term((Expression)other));
result = this.parent;
}
else {
if (log.isDebugEnabled())
log.debug("using existing synthetic expr parent for concatination");
parent.getTerms().add(new Term(
new LogicalOperator(oper)));
parent.getTerms().add(new Term((Expression)other));
((Expression)other).parent = parent;
result = parent;
}
}
else {
if (log.isDebugEnabled())
log.debug("using existing parent for concatination");
this.parent.getTerms().add(new Term(
new LogicalOperator(oper)));
this.parent.getTerms().add(new Term((Expression)other));
((Expression)other).parent = this.parent;
result = this.parent;
}
return result;
}
private Expression findParent() {
ExprFinder finder = new ExprFinder(SYNTHETIC_PARENT_ID);
this.accept(finder);
return finder.getResult();
}
@XmlElement(name = "Term")
protected List terms;
public Expression() {
super();
}
public Expression(Term term) {
this();
this.getTerms().add(term);
}
public Expression(Term t1, Term t2, Term t3) {
this();
this.getTerms().add(t1);
this.getTerms().add(t2);
this.getTerms().add(t3);
}
public Expression(Expression e1, Expression e2, Expression e3) {
this();
this.getTerms().add(new Term(e1));
this.getTerms().add(new Term(e2));
this.getTerms().add(new Term(e3));
}
public Expression(Expression[] exprs) {
this();
for (int i = 0; i < exprs.length; i++)
this.getTerms().add(new Term(exprs[i]));
}
public Expression(Property prop, ArithmeticOperator oper, Literal lit) {
this();
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(lit));
}
public Expression(Expression left, RelationalOperator oper, Expression right) {
this();
this.getTerms().add(new Term(left));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(right));
}
public Expression(Expression left, LogicalOperator oper, Expression right) {
this();
this.getTerms().add(new Term(left));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(right));
}
public Expression(GroupOperator right, Property prop, RelationalOperator oper, Literal lit) {
this();
this.getTerms().add(new Term(right));
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(lit));
}
public Expression(Property prop, RelationalOperator oper, Literal lit, GroupOperator left) {
this();
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(lit));
this.getTerms().add(new Term(left));
}
public Expression(GroupOperator right, Property prop, RelationalOperator oper, Literal lit, GroupOperator left) {
this();
this.getTerms().add(new Term(right));
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(lit));
this.getTerms().add(new Term(left));
}
public Expression(Property prop, RelationalOperator oper, Variable var) {
this();
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(var));
}
public Expression(Property prop, RelationalOperator oper, Literal lit) {
this();
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(lit));
}
public Expression(Property prop, WildcardOperator oper, Literal lit) {
this();
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(lit));
}
public Expression(Property prop, Literal min, Literal max) {
this();
Expression left = new Expression(prop,
new RelationalOperator(RelationalOperatorValues.GREATER_THAN_EQUALS),
min);
Expression right = new Expression(prop,
new RelationalOperator(RelationalOperatorValues.LESS_THAN_EQUALS),
max);
this.getTerms().add(new Term(left));
this.getTerms().add(new Term(new LogicalOperator(LogicalOperatorValues.AND)));
this.getTerms().add(new Term(right));
}
public Expression(Property prop, RelationalOperator oper, NullLiteral lit) {
this();
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(lit));
}
public Expression(Property prop, SubqueryOperator oper, Query query) {
this();
this.getTerms().add(new Term(prop));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(query));
}
public Expression(Property left, RelationalOperator oper, Property right) {
this();
this.getTerms().add(new Term(left));
this.getTerms().add(new Term(oper));
this.getTerms().add(new Term(right));
}
public Expression(RelationalOperator oper) {
this();
this.getTerms().add(new Term(oper));
}
public Expression(ArithmeticOperator oper) {
this();
this.getTerms().add(new Term(oper));
}
public Expression(LogicalOperatorValues oper) {
this();
this.getTerms().add(new Term(new LogicalOperator(oper)));
}
public Expression(GroupOperator oper) {
this();
this.getTerms().add(new Term(oper));
}
public Expression(Property prop) {
this();
this.getTerms().add(new Term(prop));
}
public Expression(Entity entity) {
this();
this.getTerms().add(new Term(entity));
}
/**
* Returns an expression where
* the given property is equal to to the given literal object. The given
* object is assumed to be a string literal, or can be converted to a
* string literal.
* @param prop the property
* @param object the literal
* @return the boolean expression
*/
public static Expression eq(String prop, Object object)
{
return Expression.eq(new Property(prop), Literal.valueOf(object));
}
/**
* Returns an expression where
* the given property is equal to to the given literal object. The given
* object is assumed to be a string literal, or can be converted to a
* string literal.
* @param prop the property
* @param path the multi-element property path
* @param object the literal
* @return the boolean expression
*/
public static Expression eq(String prop, String[] path, Object object)
{
return Expression.eq(new Property(prop, new Path(path)), Literal.valueOf(object));
}
/**
* Returns an expression where
* the given property is equal to to the given literal object. The given
* object is assumed to be a string literal, or can be converted to a
* string literal.
* @param prop the property
* @param path the single-element property path
* @param object the literal
* @return the boolean expression
*/
public static Expression eq(String prop, String path, Object object)
{
return Expression.eq(new Property(prop, new Path(path)), Literal.valueOf(object));
}
/**
* Returns an expression where
* the given property is equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression eq(Property prop, Literal literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.EQUALS), literal);
}
/**
* Returns an expression where
* the given property is equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression eq(Property prop, Object literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.EQUALS), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is equal to to the given null-literal.
* @param prop the property
* @param object the literal
* @return the boolean expression
*/
public static Expression eq(Property prop, NullLiteral literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.EQUALS), literal);
}
/**
* Returns an expression where
* the given property is not equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression ne(String prop, Object literal)
{
return Expression.ne(new Property(prop), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is not equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression ne(Property prop, Object literal)
{
return Expression.ne(prop, Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is not equal to to the given literal.
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression ne(String prop, String path, Object literal)
{
return Expression.ne(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is not equal to to the given literal.
* @param prop the property
* @param path the multi-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression ne(String prop, String[] path, Object literal)
{
return Expression.ne(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is not equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression ne(Property prop, Literal literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.NOT_EQUALS), literal);
}
/**
* Returns an expression where
* the given property is not equal to to the given null-literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression ne(Property prop, NullLiteral literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.NOT_EQUALS), literal);
}
/**
* Returns an expression where
* the given property is greated than to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression gt(String prop, Object literal)
{
return Expression.gt(new Property(prop), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is greated than to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression gt(Property prop, Object literal)
{
return Expression.gt(prop, Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is greated than to the given literal.
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression gt(String prop, String path, Object literal)
{
return Expression.gt(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is greated than to the given literal.
* @param prop the property
* @param path the multi-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression gt(String prop, String[] path, Object literal)
{
return Expression.gt(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is greater than to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression gt(Property prop, Literal literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.GREATER_THAN), literal);
}
/**
* Returns an expression where
* the given property is greater than or equalt to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression ge(String prop, Object literal)
{
return Expression.ge(new Property(prop), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is greater than or equal to to the given literal.
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression ge(String prop, String path, Object literal)
{
return Expression.ge(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is greater than or equal to to the given literal.
* @param prop the property
* @param path the multi-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression ge(String prop, String[] path, Object literal)
{
return Expression.ge(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is greater than or equal to to the given literal.
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression ge(Property prop, Literal literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.GREATER_THAN_EQUALS), literal);
}
/**
* Returns an expression where
* the given property is greater than or equal to to the given literal.
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression ge(Property prop, Object literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.GREATER_THAN_EQUALS), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression lt(Property prop, Object literal)
{
return Expression.lt(prop, Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression lt(String prop, Object literal)
{
return Expression.lt(new Property(prop), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than to the given literal.
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression lt(String prop, String path, Object literal)
{
return Expression.lt(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than to the given literal.
* @param prop the property
* @param path the multi-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression lt(String prop, String[] path, Object literal)
{
return Expression.lt(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression lt(Property prop, Literal literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.LESS_THAN), literal);
}
/**
* Returns an expression where
* the given property is less than or equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression le(String prop, Object literal)
{
return Expression.le(new Property(prop), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than or equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression le(Property prop, Object literal)
{
return Expression.le(prop, Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than or equal to to the given literal.
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression le(String prop, String path, Object literal)
{
return Expression.le(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than or equal to to the given literal.
* @param prop the property
* @param path the multi-element property path
* @param literal the literal
* @return the boolean expression
*/
public static Expression le(String prop, String[] path, Object literal)
{
return Expression.le(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns an expression where
* the given property is less than or equal to to the given literal.
* @param prop the property
* @param literal the literal
* @return the boolean expression
*/
public static Expression le(Property prop, Literal literal)
{
return new Expression(prop, new RelationalOperator(RelationalOperatorValues.LESS_THAN_EQUALS), literal);
}
/**
* Returns a wildcard expression "triad", where
* the given property is like the given literal, and the literal
* may contain any number of wildcards, the wildcard character being '*'
* @param prop the property
* @param literal the literal
* @return the wildcard expression
*/
public static Expression like(String prop, Object literal)
{
return Expression.like(new Property(prop), Literal.valueOf(literal));
}
/**
* Returns a wildcard expression "triad", where
* the given property is like the given literal, and the literal
* may contain any number of wildcards, the wildcard character being '*'
* @param prop the property
* @param literal the literal
* @return the wildcard expression
*/
public static Expression like(Property prop, Object literal)
{
return Expression.like(prop, Literal.valueOf(literal));
}
/**
* Returns a wildcard expression "triad", where
* the given property is like the given literal, and the literal
* may contain any number of wildcards, the wildcard character being '*'
* @param prop the property
* @param path the single-element property path
* @param literal the literal
* @return the wildcard expression
*/
public static Expression like(String prop, String path, Object literal)
{
return Expression.like(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns a wildcard expression "triad", where
* the given property is like the given literal, and the literal
* may contain any number of wildcards, the wildcard character being '*'
* @param prop the property
* @param path the multi-element property path
* @param literal the literal
* @return the wildcard expression
*/
public static Expression like(String prop, String[] path, Object literal)
{
return Expression.like(new Property(prop, new Path(path)), Literal.valueOf(literal));
}
/**
* Returns a wildcard expression "triad", where
* the given property is like the given literal, and the literal
* may contain any number of wildcards, the wildcard character being '*'
* @param prop the property
* @param literal the literal
* @return the wildcard expression
*/
public static Expression like(Property prop, Literal literal)
{
return new Expression(prop, new WildcardOperator(), literal);
}
/**
* Returns a 6 term expression, where
* the given property is greater than or equal to the given 'min' literal,
* and is less than or equal tothe given 'max' literal.
* @param prop the property
* @param min the minimum literal
* @param max the maximum literal
* @return the expression
*/
public static Expression between(Property prop, Literal min, Literal max)
{
return new Expression(prop, min, max);
}
/**
* Returns a 6 term expression, where
* the given property is greater than or equal to the given 'min' literal,
* and is less than or equal tothe given 'max' literal.
* @param prop the property
* @param min the minimum literal
* @param max the maximum literal
* @return the expression
*/
public static Expression between(String prop, Object min, Object max)
{
return new Expression(new Property(prop), Literal.valueOf(min), Literal.valueOf(max));
}
/**
* Returns a 6 term expression, where
* the given property is greater than or equal to the given 'min' literal,
* and is less than or equal tothe given 'max' literal.
* @param prop the property
* @param min the minimum literal
* @param max the maximum literal
* @return the expression
*/
public static Expression between(Property prop, Object min, Object max)
{
return new Expression(prop, Literal.valueOf(min), Literal.valueOf(max));
}
/**
* Returns a 6 term expression, where
* the given property is greater than or equal to the given 'min' literal,
* and is less than or equal tothe given 'max' literal.
* @param prop the property
* @param path the property path
* @param min the minimum literal
* @param max the maximum literal
* @return the expression
*/
public static Expression between(String prop, String[] path, Object min, Object max)
{
return new Expression(new Property(prop, new Path(path)), Literal.valueOf(min), Literal.valueOf(max));
}
/**
* Returns a subquery expression, where
* the given property is found within the given subquery results collection.
* @param prop the property
* @param subquery the subquery
* @return the subquery expression
*/
public static Expression in(Property prop, Query subquery)
{
return new Expression(prop, new SubqueryOperator(SubqueryOperatorValues.IN), subquery);
}
/**
* Returns a subquery expression, where
* the given property is found within the given subquery results collection.
* @param prop the property
* @param subquery the subquery
* @return the subquery expression
*/
public static Expression in(String prop, Query subquery)
{
return new Expression(new Property(prop), new SubqueryOperator(SubqueryOperatorValues.IN), subquery);
}
/**
* Returns a subquery expression, where
* the given property is found within the given subquery results collection.
* @param prop the property
* @param path the path
* @param subquery the subquery
* @return the subquery expression
*/
public static Expression in(String prop, String[] path, Query subquery)
{
return new Expression(new Property(prop, new Path(path)), new SubqueryOperator(SubqueryOperatorValues.IN), subquery);
}
/**
* Returns a subquery expression, where
* the given property is not found within the given subquery results collection.
* @param prop the property
* @param subquery the subquery
* @return the subquery expression
*/
public static Expression notIn(Property prop, Query subquery)
{
return new Expression(prop, new SubqueryOperator(SubqueryOperatorValues.NOT_IN), subquery);
}
public static Expression exists(Property prop, Query subquery)
{
return new Expression(prop, new SubqueryOperator(SubqueryOperatorValues.EXISTS), subquery);
}
/**
* Returns a simple 1-term expression containing a boolean
* operator, AND.
* @return the simple expression
*/
public static Expression and()
{
return new Expression(LogicalOperatorValues.AND);
}
/**
* Returns a simple 1-term expression containing a boolean
* operator, OR.
* @return the simple expression
*/
public static Expression or()
{
return new Expression(LogicalOperatorValues.OR);
}
/**
* Returns a simple 1-term expression containing a group
* operator, ).
* @return the simple expression
*/
public static Expression left()
{
return new Expression(new GroupOperator(")"));
}
/**
* Returns a simple 1-term expression containing a group
* operator, (.
* @return the simple expression
*/
public static Expression right()
{
return new Expression(new GroupOperator("("));
}
/**
* Factory method supporting Jaxen XAPTH relational expressions.
* @param expr a Jaxen XAPTH relational expression
* @return
*/
public static Expression valueOf(RelationalExpr expr) {
LocationPath left = (LocationPath)expr.getLHS();
NameStep name = (NameStep)left.getSteps().get(0);
Literal literal = null;
if (expr.getRHS() instanceof NumberExpr) {
NumberExpr num = ((NumberExpr)expr.getRHS());
literal = Literal.valueOf(num.getNumber());
}
else if (expr.getRHS() instanceof LiteralExpr) {
LiteralExpr lit = ((LiteralExpr)expr.getRHS());
literal = Literal.valueOf(lit.getLiteral());
}
else {
literal = Literal.valueOf(
expr.getRHS().toString());
}
return new Expression(
new Property(name.getLocalName()),
new RelationalOperator(expr.getOperator()),
literal);
}
/**
* Factory method supporting Jaxen XAPTH logical expressions.
* @param expr a Jaxen XAPTH logical expression
* @return
*/
public static Expression valueOf(LogicalExpr expr) {
return booleanExprFor(expr);
}
public static Expression valueOf(EqualityExpr expr) {
return booleanExprFor(expr);
}
public static Expression noOp() {
Expression noOp = new Expression();
noOp.id = Expression.NOOP_EXPR_ID;
return noOp;
}
public boolean isNoOp() {
return this.id != null && this.id.equals(Expression.NOOP_EXPR_ID);
}
private static Expression booleanExprFor(BinaryExpr expr) {
LocationPath left = (LocationPath)expr.getLHS();
NameStep name = (NameStep)left.getSteps().get(0);
Literal literal = null;
if (expr.getRHS() instanceof NumberExpr) {
NumberExpr num = ((NumberExpr)expr.getRHS());
literal = Literal.valueOf(num.getNumber());
}
else if (expr.getRHS() instanceof LiteralExpr) {
LiteralExpr lit = ((LiteralExpr)expr.getRHS());
literal = Literal.valueOf(lit.getLiteral());
}
else {
literal = Literal.valueOf(
expr.getRHS().toString());
}
return new Expression(
new Property(name.getLocalName()),
RelationalOperator.valueOf(expr.getOperator()),
literal);
}
/**
* Gets the value of the terms property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the terms property.
*
*
* For example, to add a new item, do as follows:
*
* getTerms().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Term }
*
*
*/
public List getTerms() {
if (terms == null) {
terms = new ArrayList();
}
return this.terms;
}
public void accept(QueryVisitor visitor)
{
visitor.start(this);
if (visitor.getContext().getTraversal().ordinal() == Traversal.CONTINUE.ordinal())
for (int i = 0; i < this.getTerms().size(); i++)
this.getTerms().get(i).accept(visitor);
visitor.end(this);
}
class ExprFinder extends DefaultQueryVisitor {
private String exprId;
private Expression result;
@SuppressWarnings("unused")
private ExprFinder() {}
public ExprFinder(String exprId) {
this.exprId = exprId;
}
public void start(Expression expression) {
if (this.exprId.equals(expression.id))
this.result = expression;
}
public Expression getResult() {
return result;
}
}
}