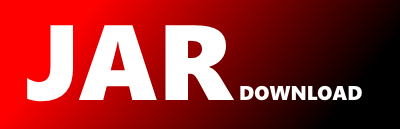
org.plasma.text.lang3gl.java.SDOInterfaceFactory Maven / Gradle / Ivy
/**
* PlasmaSDO™ License
*
* This is a community release of PlasmaSDO™, a dual-license
* Service Data Object (SDO) 2.1 implementation.
* This particular copy of the software is released under the
* version 2 of the GNU General Public License. PlasmaSDO™ was developed by
* TerraMeta Software, Inc.
*
* Copyright (c) 2013, TerraMeta Software, Inc. All rights reserved.
*
* General License information can be found below.
*
* This distribution may include materials developed by third
* parties. For license and attribution notices for these
* materials, please refer to the documentation that accompanies
* this distribution (see the "Licenses for Third-Party Components"
* appendix) or view the online documentation at
* .
*
*/
package org.plasma.text.lang3gl.java;
import java.util.Map;
import java.util.TreeMap;
import org.plasma.config.InterfaceProvisioning;
import org.plasma.config.Namespace;
import org.plasma.config.PlasmaConfig;
import org.plasma.metamodel.Class;
import org.plasma.metamodel.ClassRef;
import org.plasma.metamodel.Package;
import org.plasma.metamodel.Property;
import org.plasma.sdo.PlasmaDataObject;
import org.plasma.text.TextBuilder;
import org.plasma.text.TextProvisioningException;
import org.plasma.text.lang3gl.ClassNameResolver;
import org.plasma.text.lang3gl.InterfaceFactory;
import org.plasma.text.lang3gl.Lang3GLContext;
public class SDOInterfaceFactory extends SDODefaultFactory
implements InterfaceFactory {
public SDOInterfaceFactory(Lang3GLContext context) {
super(context);
}
public String createContent(Package pkg, Class clss) {
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
buf.append(this.createPackageDeclaration(pkg));
buf.append(LINE_SEP);
buf.append(this.createThirdPartyImportDeclarations(pkg, clss));
buf.append(LINE_SEP);
buf.append(this.createSDOInterfaceReferenceImportDeclarations(pkg, clss));
buf.append(LINE_SEP);
buf.append(LINE_SEP);
buf.append(this.createTypeDeclaration(pkg, clss));
buf.append(LINE_SEP);
buf.append(this.beginBody());
buf.append(LINE_SEP);
buf.append(this.createStaticFieldDeclarations(pkg, clss));
buf.append(LINE_SEP);
buf.append(this.createMethodDeclarations(clss));
for (Property field : clss.getProperties()) {
buf.append(LINE_SEP);
buf.append(this.createMethodDeclarations(clss, field));
}
buf.append(LINE_SEP);
buf.append(this.endBody());
return buf.toString();
}
protected String createThirdPartyImportDeclarations(Package pkg, Class clss) {
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
// FIXME: add array/list accessor collection config option
//if (!hasOnlySingilarFields(clss)) {
// buf.append(LINE_SEP);
// buf.append(this.createImportDeclaration(pkg, clss, List.class.getName()));
//}
return buf.toString();
}
protected String createTypeDeclaration(Package pkg, Class clss) {
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
String javadoc = createTypeDeclarationJavadoc(pkg, clss);
buf.append(javadoc);
SDOInterfaceNameResolver interfaceResolver = new SDOInterfaceNameResolver();
buf.append(LINE_SEP);
buf.append("public interface ");
buf.append(interfaceResolver.getName(clss));
buf.append(" extends ");
if (clss.getSuperClasses() != null && clss.getSuperClasses().size() > 0) {
int i = 0;
for (ClassRef ref : clss.getSuperClasses()) {
if (i > 0)
buf.append(", ");
buf.append(ref.getName());
i++;
}
}
else {
// always extends DO so we can cast from its impl to any generated interface
buf.append(PlasmaDataObject.class.getSimpleName());
}
return buf.toString();
}
private String createTypeDeclarationJavadoc(Package pkg, Class clss) {
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
buf.append("/**"); // begin javadoc
// add formatted doc from UML if exists
// always put model definition first so it appears
// on package summary line for class
String docs = getWrappedDocmentations(clss.getDocumentations(), 0);
if (docs.trim().length() > 0) {
buf.append(docs);
// if we have model docs, set up the next section w/a "header"
buf.append(newline(0));
buf.append(" * ");
}
buf.append(newline(0));
buf.append(" * Generated interface representing the domain model entity ");
buf.append(clss.getName());
buf.append(". This SDO interface directly reflects the");
buf.append(newline(0));
buf.append(" * class (single or multiple) inheritance lattice of the source domain model(s) ");
buf.append(" and is part of namespace ");
buf.append(clss.getUri());
buf.append(" defined within the Configuration.");
// data store mapping
if (clss.getAlias() != null && clss.getAlias().getPhysicalName() != null) {
buf.append(newline(0));
buf.append(" *");
buf.append(newline(0));
buf.append(" * ");
buf.append(newline(0));
buf.append(" * Data Store Mapping:");
buf.append(newline(0));
buf.append(" * Corresponds to the physical data store entity ");
buf.append(clss.getAlias().getPhysicalName());
buf.append(".");
buf.append(newline(0));
buf.append(" * ");
buf.append(newline(0));
buf.append(" *");
}
// add @see items for referenced classes
Map classMap = new TreeMap();
if (clss.getSuperClasses() != null && clss.getSuperClasses().size() > 0)
this.collectProvisioningSuperclasses(pkg, clss, classMap);
//for interfaces we have definitions for all methods generated
// based on local fields, not fields from superclasses
collectProvisioningClasses(pkg, clss, classMap);
for (Class refClass : classMap.values()) {
Namespace sdoNamespace = PlasmaConfig.getInstance().getSDONamespaceByURI(refClass.getUri());
String packageName = sdoNamespace.getProvisioning().getPackageName();
String packageQualifiedName = packageName + "." + refClass.getName();
buf.append(newline(0));
buf.append(" * @see ");
buf.append(packageQualifiedName);
buf.append(" ");
buf.append(refClass.getName());
}
buf.append(newline(0));
buf.append(" */"); // end javadoc
return buf.toString();
}
protected String createStaticFieldDeclarations(Package pkg, Class clss) {
InterfaceProvisioning interfaceProvisioning = PlasmaConfig.getInstance().getSDOInterfaceProvisioning(pkg.getUri());
if (interfaceProvisioning == null)
interfaceProvisioning = this.globalInterfaceProvisioning;
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
// the namespace URI
buf.appendln(1, "/** The SDO namespace URI associated with the Type for this class. */");
buf.appendln(1, "public static final String NAMESPACE_URI = \"");
buf.append(clss.getUri());
buf.append("\";");
buf.append(LINE_SEP);
//the entity name
buf.appendln(1, "/** The entity or Type logical name associated with this class. */");
buf.appendln(1, "public static final String TYPE_NAME_");
buf.append(toConstantName(clss.getName()));
buf.append(" = \"");
buf.append(clss.getName());
buf.append("\";");
buf.appendln(1, "");
switch (interfaceProvisioning.getPropertyNameStyle()) {
case ENUMS:
switch(interfaceProvisioning.getEnumSource()) {
case DERIVED:
// the static enums
buf.appendln(1, "/** The declared logical property names for this Type. */");
buf.appendln(1, "public static enum PROPERTY {");
int enumCount = 0;
for (Property field : clss.getProperties()) {
if (enumCount > 0)
buf.append(",");
buf.append(this.newline(2));
String javadoc = createStaticFieldDeclarationJavadoc(clss, field, 2);
buf.append(javadoc);
buf.append(this.newline(2));
buf.append(field.getName());
enumCount++;
}
buf.appendln(1, "}");
break;
case EXTERNAL: // noop
break;
default:
throw new TextProvisioningException("unexpected enum source, " + interfaceProvisioning.getEnumSource());
}
break;
case CONSTANTS:
// static constants
buf.appendln(1, "");
for (Property field : clss.getProperties()) {
String javadoc = createStaticFieldDeclarationJavadoc(clss, field, 1);
buf.appendln(1, javadoc);
buf.appendln(1, "public static final String ");
buf.append(toConstantName(field.getName()));
buf.append(" = \"");
buf.append(field.getName());
buf.append("\";");
}
buf.appendln(1, "");
break;
default:
}
return buf.toString();
}
private String createStaticFieldDeclarationJavadoc(Class clss, Property field, int indent)
{
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
buf.append(this.newline(indent));
buf.append("/**"); // begin javadoc
// add formatted doc from UML if exists
// always put model definition first so it appears
// on package summary line for class
String docs = getWrappedDocmentations(field.getDocumentations(), indent);
if (docs.trim().length() > 0) {
buf.append(docs);
buf.append(newline(indent));
buf.append(" * ");
buf.append(newline(indent));
buf.append(" *");
}
buf.append(newline(indent));
buf.append(" * Represents the logical Property ");
buf.append(field.getName());
buf.append(" which is part of the Type ");
buf.append(clss.getName());
buf.append(".");
// data store mapping
if (clss.getAlias() != null && clss.getAlias().getPhysicalName() != null &&
field.getAlias() != null && field.getAlias().getPhysicalName() != null) {
buf.append(this.newline(indent));
buf.append(" *");
buf.append(this.newline(indent));
buf.append(" * ");
buf.append(this.newline(indent));
buf.append(" * Data Store Mapping:");
buf.append(this.newline(indent));
buf.append(" * Corresponds to the physical data store element ");
buf.append(clss.getAlias().getPhysicalName() + "." + field.getAlias().getPhysicalName());
buf.append(".");
}
buf.append(this.newline(indent));
buf.append(" */"); // end javadoc
return buf.toString();
}
protected String createMethodDeclarations(Class clss) {
// TODO Auto-generated method stub
return "";
}
protected String createMethodDeclarations(Class clss, Property field) {
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
MetaClassInfo typeClassName = this.getTypeClassName(field.getType());
buf.append(LINE_SEP);
createIsSetDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
buf.append(LINE_SEP);
createUnsetterDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
if (field.getType() instanceof ClassRef) {
Class targetClass = this.context.findClass((ClassRef)field.getType());
if (!targetClass.isAbstract()) {
buf.append(LINE_SEP);
createCreatorDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
}
else {
buf.append(LINE_SEP);
createCreatorByAbstractClassDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
}
}
if (!field.isMany()) {
buf.append(LINE_SEP);
createSingularGetterDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
buf.append(LINE_SEP);
createSingularSetterDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
}
else {
buf.append(LINE_SEP);
createManyGetterDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
buf.append(LINE_SEP);
createManyIndexGetterDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
buf.append(LINE_SEP);
createManyCountDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
buf.append(LINE_SEP);
createManySetterDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
buf.append(LINE_SEP);
createManyAdderDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
buf.append(LINE_SEP);
createManyRemoverDeclaration(null, clss, field, typeClassName, buf);
buf.append(";");
}
return buf.toString();
}
public String createFileName(Class clss, Package pkg) {
SDOInterfaceNameResolver interfaceResolver = new SDOInterfaceNameResolver();
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
buf.append(interfaceResolver.getName(clss));
buf.append(".java");
return buf.toString();
}
protected String createSDOInterfaceReferenceImportDeclarations(Package pkg, Class clss) {
TextBuilder buf = new TextBuilder(LINE_SEP, this.context.getIndentationToken());
// for interfaces we extend our superclasses, so need to reference them
// FIXME: only 1 level though
ClassNameResolver resolver = new SDOInterfaceNameResolver();
Map nameMap = new TreeMap();
if (clss.getSuperClasses() != null && clss.getSuperClasses().size() > 0)
this.collectSuperclassNames(pkg, clss, nameMap, resolver);
else // it extends DataObject, so import it
nameMap.put(PlasmaDataObject.class.getName(), PlasmaDataObject.class.getName());
//for interfaces we have definitions for all methods generated
// based on local fields, not fields from superclasses
collectDataClassNames(pkg, clss, nameMap, resolver);
collectReferenceClassNames(pkg, clss, nameMap, resolver);
for (String name : nameMap.values()) {
if (name.startsWith("java.lang."))
continue;
buf.append(LINE_SEP);
buf.append("import ");
buf.append(name);
buf.append(";");
}
return buf.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy