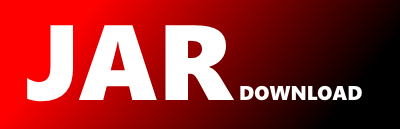
org.terrier.matching.QueryResultSet Maven / Gradle / Ivy
The newest version!
/*
* Terrier - Terabyte Retriever
* Webpage: http://terrier.org
* Contact: terrier{a.}dcs.gla.ac.uk
* University of Glasgow - School of Computing Science
* http://www.gla.ac.uk/
*
* The contents of this file are subject to the Mozilla Public License
* Version 1.1 (the "License"); you may not use this file except in
* compliance with the License. You may obtain a copy of the License at
* http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS"
* basis, WITHOUT WARRANTY OF ANY KIND, either express or implied. See
* the License for the specific language governing rights and limitations
* under the License.
*
* The Original Code is QueryResultSet.java.
*
* The Original Code is Copyright (C) 2004-2020 the University of Glasgow.
* All Rights Reserved.
*
* Contributor(s):
* Vassilis Plachouras (original author)
* Craig Macdonald
*/
package org.terrier.matching;
import gnu.trove.TObjectIntHashMap;
import java.util.Arrays;
//import org.apache.log4j.Logger;
/**
* A result set for a given query. This result set is created for
* a given number of documents, usually the number of retrieved
* documents for a query. Initially, it is created by cropping
* an instance of the CollectionResultSet, that is used in the
* Matching classes.
*
* This class has support for adding metadata as well.
* @author Vassilis Plachouras, Craig Macdonald
*/
public class QueryResultSet extends CollectionResultSet {
private static final long serialVersionUID = 1L;
/**
* The logger used
*/
//private static Logger logger = Logger.getLogger(QueryResultSet.class);
/**
* The structure that holds the metadata about the results.
* It maps the metadata name, a string, to an array index of
* the metadata array that contains the particular metadata.
*/
protected TObjectIntHashMap metaMap;
/** The structure holding the metadata. Each column is associated with a
* named metavalue type, as known by metaMap. Each row is for a given document. */
protected String[][] metadata;
/**
* A default constructor for the result set with a given
* number of documents.
* @param numberOfDocuments the number of documents contained in the result set.
*/
public QueryResultSet(final int numberOfDocuments) {
super(numberOfDocuments);
metaMap = new TObjectIntHashMap();
metadata = new String[0][];
}
public QueryResultSet(int[] docids, double[] ds, short[] occurrences) {
super(docids, ds, occurrences);
metaMap = new TObjectIntHashMap();
metadata = new String[0][];
}
/**
* Initialises the arrays prior of retrieval. Only the first time it is called,
* it will allocate memory for the arrays.
*/
public void initialise() {
super.initialise();
metaMap.clear();
metadata = new String[0][];
}
/**
* Adds a metadata value for a given document
* @param name the name of the metadata type. For example, it can be url for adding the URLs of documents.
* @param index the position in the resultset array of the given document
* @param value the metadata value.
*/
public void addMetaItem(final String name, final int index, final String value) {
int place = addMetaType(name);
metadata[place][index] = value;
}
/**
* Adds the metadata values for all the documents in the result set.
* The length of the metadata array values should be equal to the
* length of the docids array. The array must be sorted in the same
* way as the resultset (descending score)
*
* @param name the name of the metadata type. For example, it can be url for adding the URLs of documents.
* @param values the metadata values.
*/
public void addMetaItems(final String name, final String[] values) {
int place = addMetaType(name);
metadata[place] = values;
}
/**
* Gets a metadata value for a given document. If the requested
* metadata information is not specified, then we return null.
* @param name the name of the metadata type.
* @param index the postition in the array
* @return a string with the metadata information, or null of the metadata is not available.
*/
public String getMetaItem(final String name, final int index) {
if (!metaMap.containsKey(name))
return null;
return metadata[metaMap.get(name)][index];
}
/**
* Gets the metadata information for all documents. If the requested
* metadata information is not specified, then we return null.
* @param name the name of the metadata type.
* @return an array of strings with the metadata information, or null of the metadata is not available.
*/
public String[] getMetaItems(final String name) {
if (!metaMap.containsKey(name))
return null;
return metadata[metaMap.get(name)];
}
/** returns all metadata, indexed first by metadata type, then by rank.
* The order of keys is as in getMetaKeys()
*/
public String[][] allMetaItems() {
return metadata;
}
/** does the metaindex have a particular type of metadata? */
@Override
public boolean hasMetaItems(String name) {
return metaMap.containsKey(name);
}
/** {@inheritDoc} */
@Override
public String[] getMetaKeys() {
String[] rtr = metaMap.keys(new String[metaMap.size()]);
Arrays.sort(rtr, (String o1, String o2) -> metaMap.get(o1) - metaMap.get(o2));
return rtr;
}
/** Get the metadata index for the given name
* @param name The name of the metadata type to add on
* @return Integer of the place in the metadata array it should be stored in */
protected int addMetaType(final String name) {
if (! metaMap.containsKey(name)) {
String [][] tmp = metadata;
int length = tmp.length;
metadata = new String[length+1][resultSize];
for(int i=0;i)metaMap.clone();
resultSet.metadata = new String[metadata.length][length1];
for(int i=0;ipositions in the resultset that
* should be saved.
* @param positions int[] the list of elements in the current
* list that should be kept.
* @return a subset of the current result set specified by
* the list.
*/
public ResultSet getResultSet(final int[] positions) {
int NewSize = positions.length;
//if (logger.isDebugEnabled()){
// logger.debug("New results size is "+NewSize);
//}
QueryResultSet resultSet = makeNewResultSet(NewSize);
resultSet.setExactResultSize(this.exactResultSize);
int newDocids[] = resultSet.getDocids();
double newScores[] = resultSet.getScores();
short newOccurs[] = resultSet.getOccurrences();
resultSet.metaMap = (TObjectIntHashMap)metaMap.clone();
int metaLength = metadata.length;
resultSet.metadata = new String[metaLength][];
String[][] tmpMeta = resultSet.metadata;
int thisPosition;
for(int j=0;j 0)
throw new UnsupportedOperationException("sorting with metadata doesnt work");
super.sort();
}
@Override
public void sort(int topDocs) {
if (metaMap.size() > 0)
throw new UnsupportedOperationException("sorting with metadata doesnt work");
super.sort(topDocs);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy