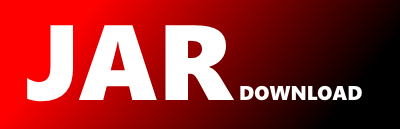
org.test4j.json.JSON Maven / Gradle / Ivy
package org.test4j.json;
import java.io.StringWriter;
import java.lang.reflect.Type;
import java.lang.reflect.TypeVariable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.test4j.json.decoder.IDecoder;
import org.test4j.json.decoder.base.DecoderFactory;
import org.test4j.json.encoder.JSONEncoder;
import org.test4j.json.helper.JSONArray;
import org.test4j.json.helper.JSONFeature;
import org.test4j.json.helper.JSONMap;
import org.test4j.json.helper.JSONObject;
import org.test4j.json.helper.JSONScanner;
import org.test4j.json.helper.JSONSingle;
/**
* json解码,编码工具类
*
* @author darui.wudr
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public final class JSON {
/**
* 将json字符串反序列为对象
*
* @param json
* @return
*/
public static final T toObject(String json) {
if (json == null) {
return null;
}
JSONObject jsonObject = JSONScanner.scnJSON(json);
Object o = toObject(jsonObject, new HashMap());
return (T) o;
}
public static final T toObject(String json, Type clazz) {
if (json == null) {
return null;
}
JSONObject jsonObject = JSONScanner.scnJSON(json);
Object o = toObject(jsonObject, clazz, new HashMap());
return (T) o;
}
/**
* 将json字符串反序列为对象
*
* @param
* @param json
* @param clazz
* @param decodingFeatures
* @return
*/
public static final T toObject(JSONObject json, Map references) {
if (json instanceof JSONArray) {
Object o = toObject(json, Object[].class, references);
return (T) o;
} else if (json instanceof JSONMap) {
Type toType = ((JSONMap) json).getClazzFromJSONFProp(HashMap.class);
Object o = toObject(json, toType, references);
return (T) o;
} else {
Object value = ((JSONSingle) json).toPrimitiveValue();
return (T) value;
}
}
public static final T toObject(JSONObject json, Type type, Map references) {
if (type == null || Object.class.equals(type) || type instanceof TypeVariable) {
return toObject(json, references);
} else {
IDecoder decoder = DecoderFactory.getDecoder(type);
Object obj = decoder.decode(json, type, references);
return (T) obj;
}
}
/**
* 将对象编码为json串
*
* @param object
* @return
*/
public static final String toJSON(Object object, JSONFeature... features) {
if (object == null) {
return "null";
}
int value = JSONFeature.getFeaturesMask(features);
String json = toJSON(object, value);
return json;
}
/**
* 将对象编码为json串
*
* @param object
* @param features
* @return
*/
public static final String toJSON(Object object, int features) {
if (object == null) {
return "null";
}
StringWriter writer = new StringWriter();
JSONEncoder encoder = JSONEncoder.get(object.getClass());
encoder.setFeatures(features);
List references = new ArrayList();
encoder.encode(object, writer, references);
String json = writer.toString();
return json;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy