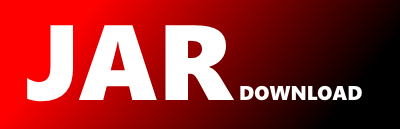
org.testng.internal.Systematiser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testng Show documentation
Show all versions of testng Show documentation
Testing framework for Java
package org.testng.internal;
import java.util.Arrays;
import java.util.Comparator;
import java.util.Objects;
import org.testng.ITestNGMethod;
/** Helps determine how should {@link ITestNGMethod} be ordered by TestNG. */
public final class Systematiser {
private static final Comparator COMPARE_INSTANCES =
Comparator.comparingInt(ITestNGMethod::getPriority)
.thenComparing(method -> method.getRealClass().getName())
.thenComparing(ITestNGMethod::getMethodName)
.thenComparing(Object::toString)
.thenComparing(
method -> {
IParameterInfo paramsInfo = method.getFactoryMethodParamsInfo();
// TODO: avoid toString in parameter comparison
return paramsInfo == null ? "" : Arrays.toString(paramsInfo.getParameters());
})
.thenComparingInt(method -> Objects.hashCode(method.getInstance()));
private Systematiser() {
// Utility class. Defeat instantiation.
}
/**
* @return - A {@link Comparator} that helps TestNG sort {@link ITestNGMethod}s in a specific
* order. Currently the following two orders are supported :
*
* - Based on the name of methods
*
- Based on the
toString()
implementation that resides within the test
* class
*
*/
public static Comparator getComparator() {
Comparator comparator;
String text = RuntimeBehavior.orderMethodsBasedOn();
Order order = Order.parse(text);
switch (order) {
case METHOD_NAMES:
comparator =
new Comparator() {
@Override
public int compare(ITestNGMethod o1, ITestNGMethod o2) {
String n1 = o1.getMethodName();
String n2 = o2.getMethodName();
return n1.compareTo(n2);
}
@Override
public String toString() {
return "Method_Names";
}
};
break;
case NONE:
// Disables sorting by providing a dummy comparator which always regards two elements as
// equal.
comparator =
new Comparator() {
@Override
public int compare(ITestNGMethod o1, ITestNGMethod o2) {
return 0;
}
@Override
public String toString() {
return "No_Sorting";
}
};
break;
case INSTANCES:
default:
comparator =
new Comparator() {
@Override
public int compare(ITestNGMethod o1, ITestNGMethod o2) {
return COMPARE_INSTANCES.compare(o1, o2);
}
@Override
public String toString() {
return "Instance_Names";
}
};
}
return comparator;
}
enum Order {
METHOD_NAMES("methods"),
INSTANCES("instances"),
NONE("none");
Order(String value) {
this.value = value;
}
private final String value;
public String getValue() {
return value;
}
public static Order parse(String value) {
if (value == null || value.trim().isEmpty()) {
return INSTANCES;
}
for (Order each : values()) {
if (each.getValue().equalsIgnoreCase(value)) {
return each;
}
}
return INSTANCES;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy