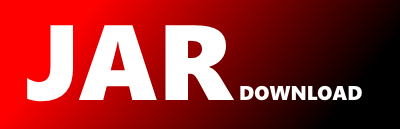
org.testtoolinterfaces.testresult.TestCaseResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of testresult Show documentation
Show all versions of testresult Show documentation
Test Result classes for Test Tool Interfaces
/**
*
*/
package org.testtoolinterfaces.testresult;
import java.util.ArrayList;
import java.util.Hashtable;
import org.testtoolinterfaces.testsuite.TestCase;
import org.testtoolinterfaces.utils.Trace;
/**
* @author Arjan Kranenburg
*
*/
public class TestCaseResult extends TestResult implements TestStepResultObserver
{
private TestCase myTestCase;
private Hashtable myPrepareResults;
private Hashtable myExecutionResults;
private Hashtable myRestoreResults;
private ArrayList myObserverCollection;
/**
* @param aTestCase
*/
public TestCaseResult(TestCase aTestCase)
{
super();
Trace.println(Trace.CONSTRUCTOR, "TestCaseResult( " + aTestCase + " )" );
myTestCase = aTestCase;
myPrepareResults = new Hashtable();
myExecutionResults = new Hashtable();
myRestoreResults = new Hashtable();
myObserverCollection = new ArrayList();
}
/**
* @param anInitializationResult
*/
public void addInitialization(TestStepResult anInitializationResult)
{
Trace.println(Trace.SETTER);
myPrepareResults.put( myPrepareResults.size(), anInitializationResult );
anInitializationResult.register(this);
notifyObservers();
}
/**
* @param anInitializationResult
*/
public void addExecution(TestStepResult anExecutionResult)
{
Trace.println(Trace.SETTER);
myExecutionResults.put( myExecutionResults.size(), anExecutionResult );
setResult(anExecutionResult.getResult());
anExecutionResult.register(this);
notifyObservers();
}
/**
* @param anInitializationResult
*/
public void addRestore(TestStepResult aRestoreResult)
{
Trace.println(Trace.SETTER);
myRestoreResults.put( myRestoreResults.size(), aRestoreResult );
aRestoreResult.register(this);
notifyObservers();
}
@Override
public void setExecutionPath(String anExecutionPath)
{
super.setExecutionPath(anExecutionPath);
for (TestStepResult result : myPrepareResults.values())
{
result.setExecutionPath(anExecutionPath + "." + this.getId());
}
for (TestStepResult result : myExecutionResults.values())
{
result.setExecutionPath(anExecutionPath + "." + this.getId());
}
for (TestStepResult result : myRestoreResults.values())
{
result.setExecutionPath(anExecutionPath + "." + this.getId());
}
}
/**
* @return the id of myTestCase
*/
public String getId()
{
Trace.println(Trace.GETTER);
return myTestCase.getId();
}
public int getSequenceNr()
{
Trace.println(Trace.GETTER);
return myTestCase.getSequenceNr();
}
public String getDescription()
{
Trace.println(Trace.GETTER);
return myTestCase.getDescription();
}
public ArrayList getRequirements()
{
Trace.println(Trace.GETTER);
return myTestCase.getRequirements();
}
public Hashtable getPrepareResults()
{
Trace.println(Trace.GETTER);
return myPrepareResults;
}
public Hashtable getExecutionResults()
{
Trace.println(Trace.GETTER);
return myExecutionResults;
}
public Hashtable getRestoreResults()
{
Trace.println(Trace.GETTER);
return myRestoreResults;
}
// Implementation of the Observer Pattern
protected void notifyObservers()
{
Trace.println(Trace.EXEC_PLUS);
for (TestCaseResultObserver observer : myObserverCollection)
{
observer.notify(this);
}
}
public void register( TestCaseResultObserver anObserver )
{
Trace.println(Trace.SETTER);
myObserverCollection.add(anObserver);
}
public void unRegisterObserver( TestCaseResultObserver anObserver )
{
Trace.println(Trace.SETTER);
myObserverCollection.remove( anObserver );
}
public void notify(TestStepResult aTestStepResult)
{
Trace.println(Trace.EXEC_UTIL);
notifyObservers();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy