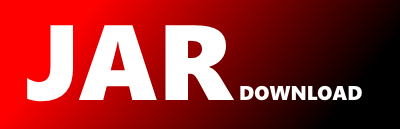
org.textmapper.templates.ast.TemplatesParser Maven / Gradle / Ivy
/**
* Copyright 2002-2016 Evgeny Gryaznov
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.textmapper.templates.ast;
import java.io.IOException;
import java.text.MessageFormat;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.textmapper.templates.ast.TemplatesLexer.ErrorReporter;
import org.textmapper.templates.ast.TemplatesLexer.Span;
import org.textmapper.templates.ast.TemplatesLexer.Tokens;
import org.textmapper.templates.ast.TemplatesTree.TextSource;
import org.textmapper.templates.bundle.IBundleEntity;
public class TemplatesParser {
public static class ParseException extends Exception {
private static final long serialVersionUID = 1L;
public ParseException() {
}
}
private final ErrorReporter reporter;
public TemplatesParser(ErrorReporter reporter) {
this.reporter = reporter;
}
private static final boolean DEBUG_SYNTAX = false;
TextSource source;
String templatePackage;
private int killEnds = -1;
private int rawText(int start, final int end) {
CharSequence buffer = source.getContents();
if (killEnds == start) {
while (start < end && (buffer.charAt(start) == '\t' || buffer.charAt(start) == ' '))
start++;
if (start < end && buffer.charAt(start) == '\r')
start++;
if (start < end && buffer.charAt(start) == '\n')
start++;
}
return start;
}
private void checkIsSpace(int start, int end, int line) {
String val = source.getText(rawText(start, end), end).trim();
if (val.length() > 0) {
reporter.error("Unknown text ignored: `" + val + "`", line, start, end);
}
}
private void applyElse(CompoundNode node, ElseIfNode elseNode, int offset, int endoffset, int line) {
if (elseNode == null) {
return;
}
if (node instanceof IfNode) {
((IfNode)node).applyElse(elseNode);
} else {
reporter.error("Unknown else node, instructions skipped", line, offset, endoffset);
}
}
private ExpressionNode createCollectionProcessor(ExpressionNode context, String instruction, String varName, ExpressionNode foreachExpr, TextSource source, int offset, int endoffset, int line) {
char first = instruction.charAt(0);
int kind = 0;
switch(first) {
case 'c':
if(instruction.equals("collect")) {
kind = CollectionProcessorNode.COLLECT;
} else if(instruction.equals("collectUnique")) {
kind = CollectionProcessorNode.COLLECTUNIQUE;
}
break;
case 'r':
if(instruction.equals("reject")) {
kind = CollectionProcessorNode.REJECT;
}
break;
case 'm':
if(instruction.equals("max")) {
kind = CollectionProcessorNode.MAX;
}
break;
case 's':
if(instruction.equals("select")) {
kind = CollectionProcessorNode.SELECT;
} else if(instruction.equals("sort")) {
kind = CollectionProcessorNode.SORT;
}
break;
case 'f':
if(instruction.equals("forAll")) {
kind = CollectionProcessorNode.FORALL;
}
break;
case 'e':
if(instruction.equals("exists")) {
kind = CollectionProcessorNode.EXISTS;
}
break;
case 'g':
if(instruction.equals("groupBy")) {
kind = CollectionProcessorNode.GROUPBY;
}
break;
}
if (kind == 0) {
reporter.error("unknown collection processing instruction: " + instruction, line, offset, endoffset);
return new ErrorNode(source, offset, endoffset);
}
return new CollectionProcessorNode(context, kind, varName, foreachExpr, source, offset, endoffset);
}
private Node createEscapedId(String escid, int offset, int endoffset) {
int sharp = escid.indexOf('#');
if (sharp >= 0) {
Integer index = new Integer(escid.substring(sharp+1));
escid = escid.substring(0, sharp);
return new IndexNode(new SelectNode(null,escid,source,offset,endoffset), new LiteralNode(index,source,offset,endoffset),source,offset,endoffset);
} else {
return new SelectNode(null,escid,source,offset,endoffset);
}
}
private void skipSpaces(int offset) {
killEnds = offset+1;
}
private void checkFqn(String templateName, int offset, int endoffset, int line) {
if (templateName.indexOf('.') >= 0 && templatePackage != null) {
reporter.error("template name should be simple identifier", line, offset, endoffset);
}
}
private static final int[] tmAction = TemplatesLexer.unpack_int(263,
"\ufffd\uffff\uffff\uffff\5\0\ufff5\uffff\uffed\uffff\1\0\3\0\4\0\uffff\uffff\0\0" +
"\31\0\30\0\26\0\27\0\uffff\uffff\uffe5\uffff\20\0\25\0\23\0\24\0\uffff\uffff\11\0" +
"\uffff\uffff\164\0\uffff\uffff\2\0\uffff\uffff\7\0\uffff\uffff\uffd7\uffff\71\0\73" +
"\0\uffff\uffff\uffff\uffff\121\0\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff" +
"\uffff\uffff\75\0\uffff\uffff\120\0\74\0\uffff\uffff\uffa1\uffff\uffff\uffff\uffff" +
"\uffff\uff99\uffff\uffff\uffff\161\0\uffff\uffff\uffff\uffff\uff7b\uffff\106\0\105" +
"\0\72\0\125\0\uff47\uffff\uff19\uffff\ufefd\uffff\ufee3\uffff\152\0\154\0\ufecd\uffff" +
"\37\0\17\0\uffff\uffff\41\0\ufec5\uffff\uffff\uffff\uffff\uffff\6\0\ufebb\uffff\uffff" +
"\uffff\ufe9d\uffff\ufe91\uffff\ufe5b\uffff\uffff\uffff\ufe53\uffff\uffff\uffff\ufe4b" +
"\uffff\uffff\uffff\uffff\uffff\ufe43\uffff\ufe3b\uffff\124\0\123\0\ufe35\uffff\uffff" +
"\uffff\156\0\ufe03\uffff\uffff\uffff\uffff\uffff\22\0\21\0\32\0\57\0\uffff\uffff" +
"\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff" +
"\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff" +
"\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\66\0\45\0\50\0\ufdfb" +
"\uffff\uffff\uffff\166\0\uffff\uffff\ufdf5\uffff\16\0\uffff\uffff\153\0\ufded\uffff" +
"\172\0\ufdcf\uffff\uffff\uffff\176\0\35\0\uffff\uffff\uffff\uffff\ufdc7\uffff\ufdc1" +
"\uffff\14\0\ufdbb\uffff\uffff\uffff\116\0\117\0\115\0\uffff\uffff\111\0\uffff\uffff" +
"\uffff\uffff\110\0\70\0\uffff\uffff\ufdb3\uffff\uffff\uffff\uffff\uffff\ufd7d\uffff" +
"\ufd4f\uffff\ufd21\uffff\ufcf3\uffff\ufcc5\uffff\ufc97\uffff\ufc69\uffff\ufc3b\uffff" +
"\ufc0d\uffff\141\0\ufbdf\uffff\ufbc1\uffff\ufba5\uffff\ufb89\uffff\ufb73\uffff\uffff" +
"\uffff\155\0\uffff\uffff\uffff\uffff\uffff\uffff\42\0\12\0\uffff\uffff\76\0\uffff" +
"\uffff\uffff\uffff\174\0\34\0\40\0\uffff\uffff\ufb5d\uffff\uffff\uffff\ufb53\uffff" +
"\uffff\uffff\202\0\uffff\uffff\uffff\uffff\uffff\uffff\113\0\uffff\uffff\157\0\104" +
"\0\ufb4d\uffff\uffff\uffff\ufb2f\uffff\uffff\uffff\65\0\uffff\uffff\uffff\uffff\13" +
"\0\uffff\uffff\44\0\ufb11\uffff\uffff\uffff\uffff\uffff\204\0\60\0\112\0\uffff\uffff" +
"\uffff\uffff\53\0\15\0\uffff\uffff\uffff\uffff\ufb09\uffff\uffff\uffff\uffff\uffff" +
"\uffff\uffff\151\0\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff\ufadb\uffff\uffff" +
"\uffff\uffff\uffff\55\0\54\0\52\0\107\0\114\0\uffff\uffff\100\0\ufad3\uffff\102\0" +
"\uffff\uffff\uffff\uffff\47\0\10\0\uffff\uffff\uffff\uffff\uffff\uffff\uffff\uffff" +
"\uffff\uffff\uffff\uffff\ufab5\uffff\56\0\uffff\uffff\101\0\103\0\46\0\61\0\uffff" +
"\uffff\51\0\uffff\uffff\uffff\uffff\ufffe\uffff\ufffe\uffff");
private static final int[] tmLalr = TemplatesLexer.unpack_int(1360,
"\1\0\uffff\uffff\5\0\uffff\uffff\0\0\163\0\uffff\uffff\ufffe\uffff\13\0\uffff\uffff" +
"\37\0\uffff\uffff\34\0\165\0\uffff\uffff\ufffe\uffff\1\0\uffff\uffff\5\0\uffff\uffff" +
"\0\0\162\0\uffff\uffff\ufffe\uffff\1\0\uffff\uffff\2\0\uffff\uffff\3\0\uffff\uffff" +
"\4\0\uffff\uffff\5\0\uffff\uffff\0\0\160\0\uffff\uffff\ufffe\uffff\57\0\uffff\uffff" +
"\66\0\uffff\uffff\30\0\67\0\36\0\67\0\44\0\67\0\45\0\67\0\46\0\67\0\47\0\67\0\50" +
"\0\67\0\51\0\67\0\52\0\67\0\55\0\67\0\56\0\67\0\60\0\67\0\61\0\67\0\62\0\67\0\63" +
"\0\67\0\64\0\67\0\65\0\67\0\67\0\67\0\70\0\67\0\72\0\67\0\73\0\67\0\74\0\67\0\75" +
"\0\67\0\77\0\67\0\uffff\uffff\ufffe\uffff\13\0\uffff\uffff\7\0\165\0\71\0\165\0\uffff" +
"\uffff\ufffe\uffff\7\0\uffff\uffff\10\0\uffff\uffff\11\0\uffff\uffff\20\0\uffff\uffff" +
"\32\0\uffff\uffff\33\0\uffff\uffff\40\0\uffff\uffff\41\0\uffff\uffff\43\0\uffff\uffff" +
"\47\0\uffff\uffff\53\0\uffff\uffff\55\0\uffff\uffff\57\0\uffff\uffff\56\0\201\0\uffff" +
"\uffff\ufffe\uffff\55\0\uffff\uffff\61\0\uffff\uffff\70\0\uffff\uffff\30\0\122\0" +
"\36\0\122\0\44\0\122\0\45\0\122\0\46\0\122\0\47\0\122\0\50\0\122\0\51\0\122\0\52" +
"\0\122\0\56\0\122\0\60\0\122\0\62\0\122\0\63\0\122\0\64\0\122\0\65\0\122\0\67\0\122" +
"\0\72\0\122\0\73\0\122\0\74\0\122\0\75\0\122\0\76\0\122\0\77\0\122\0\uffff\uffff" +
"\ufffe\uffff\46\0\uffff\uffff\47\0\uffff\uffff\50\0\uffff\uffff\51\0\uffff\uffff" +
"\52\0\uffff\uffff\72\0\uffff\uffff\73\0\uffff\uffff\74\0\uffff\uffff\75\0\uffff\uffff" +
"\30\0\137\0\36\0\137\0\44\0\137\0\45\0\137\0\56\0\137\0\60\0\137\0\62\0\137\0\63" +
"\0\137\0\64\0\137\0\65\0\137\0\67\0\137\0\76\0\137\0\77\0\137\0\uffff\uffff\ufffe" +
"\uffff\30\0\uffff\uffff\36\0\142\0\44\0\142\0\45\0\142\0\56\0\142\0\60\0\142\0\62" +
"\0\142\0\63\0\142\0\64\0\142\0\65\0\142\0\67\0\142\0\76\0\142\0\77\0\142\0\uffff" +
"\uffff\ufffe\uffff\65\0\uffff\uffff\67\0\uffff\uffff\36\0\145\0\44\0\145\0\45\0\145" +
"\0\56\0\145\0\60\0\145\0\62\0\145\0\63\0\145\0\64\0\145\0\76\0\145\0\77\0\145\0\uffff" +
"\uffff\ufffe\uffff\63\0\uffff\uffff\64\0\uffff\uffff\77\0\uffff\uffff\36\0\150\0" +
"\44\0\150\0\45\0\150\0\56\0\150\0\60\0\150\0\62\0\150\0\76\0\150\0\uffff\uffff\ufffe" +
"\uffff\62\0\uffff\uffff\44\0\33\0\45\0\33\0\uffff\uffff\ufffe\uffff\57\0\uffff\uffff" +
"\61\0\uffff\uffff\44\0\167\0\45\0\167\0\uffff\uffff\ufffe\uffff\7\0\uffff\uffff\10" +
"\0\uffff\uffff\11\0\uffff\uffff\20\0\uffff\uffff\32\0\uffff\uffff\33\0\uffff\uffff" +
"\40\0\uffff\uffff\41\0\uffff\uffff\43\0\uffff\uffff\47\0\uffff\uffff\53\0\uffff\uffff" +
"\55\0\uffff\uffff\57\0\uffff\uffff\60\0\201\0\uffff\uffff\ufffe\uffff\57\0\uffff" +
"\uffff\61\0\uffff\uffff\21\0\173\0\44\0\173\0\45\0\173\0\uffff\uffff\ufffe\uffff" +
"\57\0\uffff\uffff\30\0\67\0\36\0\67\0\44\0\67\0\45\0\67\0\46\0\67\0\47\0\67\0\50" +
"\0\67\0\51\0\67\0\52\0\67\0\55\0\67\0\56\0\67\0\60\0\67\0\61\0\67\0\62\0\67\0\63" +
"\0\67\0\64\0\67\0\65\0\67\0\67\0\67\0\70\0\67\0\72\0\67\0\73\0\67\0\74\0\67\0\75" +
"\0\67\0\76\0\67\0\77\0\67\0\uffff\uffff\ufffe\uffff\62\0\uffff\uffff\44\0\177\0\45" +
"\0\177\0\uffff\uffff\ufffe\uffff\62\0\uffff\uffff\44\0\63\0\45\0\63\0\uffff\uffff" +
"\ufffe\uffff\62\0\uffff\uffff\44\0\62\0\45\0\62\0\uffff\uffff\ufffe\uffff\62\0\uffff" +
"\uffff\44\0\36\0\45\0\36\0\uffff\uffff\ufffe\uffff\7\0\uffff\uffff\71\0\171\0\uffff" +
"\uffff\ufffe\uffff\57\0\uffff\uffff\66\0\uffff\uffff\71\0\uffff\uffff\76\0\uffff" +
"\uffff\30\0\67\0\46\0\67\0\47\0\67\0\50\0\67\0\51\0\67\0\52\0\67\0\55\0\67\0\56\0" +
"\67\0\61\0\67\0\62\0\67\0\63\0\67\0\64\0\67\0\65\0\67\0\67\0\67\0\70\0\67\0\72\0" +
"\67\0\73\0\67\0\74\0\67\0\75\0\67\0\77\0\67\0\uffff\uffff\ufffe\uffff\62\0\uffff" +
"\uffff\56\0\200\0\60\0\200\0\uffff\uffff\ufffe\uffff\7\0\uffff\uffff\60\0\171\0\uffff" +
"\uffff\ufffe\uffff\57\0\uffff\uffff\61\0\uffff\uffff\66\0\167\0\uffff\uffff\ufffe" +
"\uffff\7\0\uffff\uffff\10\0\uffff\uffff\11\0\uffff\uffff\20\0\uffff\uffff\32\0\uffff" +
"\uffff\33\0\uffff\uffff\40\0\uffff\uffff\41\0\uffff\uffff\43\0\uffff\uffff\47\0\uffff" +
"\uffff\53\0\uffff\uffff\55\0\uffff\uffff\57\0\uffff\uffff\60\0\201\0\uffff\uffff" +
"\ufffe\uffff\21\0\uffff\uffff\44\0\175\0\45\0\175\0\uffff\uffff\ufffe\uffff\7\0\uffff" +
"\uffff\60\0\207\0\uffff\uffff\ufffe\uffff\1\0\uffff\uffff\5\0\203\0\uffff\uffff\ufffe" +
"\uffff\62\0\uffff\uffff\60\0\170\0\71\0\170\0\uffff\uffff\ufffe\uffff\57\0\uffff" +
"\uffff\30\0\77\0\36\0\77\0\44\0\77\0\45\0\77\0\46\0\77\0\47\0\77\0\50\0\77\0\51\0" +
"\77\0\52\0\77\0\55\0\77\0\56\0\77\0\60\0\77\0\61\0\77\0\62\0\77\0\63\0\77\0\64\0" +
"\77\0\65\0\77\0\67\0\77\0\70\0\77\0\72\0\77\0\73\0\77\0\74\0\77\0\75\0\77\0\76\0" +
"\77\0\77\0\77\0\uffff\uffff\ufffe\uffff\46\0\131\0\47\0\131\0\50\0\uffff\uffff\51" +
"\0\uffff\uffff\52\0\uffff\uffff\72\0\131\0\73\0\131\0\74\0\131\0\75\0\131\0\30\0" +
"\131\0\36\0\131\0\44\0\131\0\45\0\131\0\56\0\131\0\60\0\131\0\62\0\131\0\63\0\131" +
"\0\64\0\131\0\65\0\131\0\67\0\131\0\76\0\131\0\77\0\131\0\uffff\uffff\ufffe\uffff" +
"\46\0\132\0\47\0\132\0\50\0\uffff\uffff\51\0\uffff\uffff\52\0\uffff\uffff\72\0\132" +
"\0\73\0\132\0\74\0\132\0\75\0\132\0\30\0\132\0\36\0\132\0\44\0\132\0\45\0\132\0\56" +
"\0\132\0\60\0\132\0\62\0\132\0\63\0\132\0\64\0\132\0\65\0\132\0\67\0\132\0\76\0\132" +
"\0\77\0\132\0\uffff\uffff\ufffe\uffff\46\0\126\0\47\0\126\0\50\0\126\0\51\0\126\0" +
"\52\0\126\0\72\0\126\0\73\0\126\0\74\0\126\0\75\0\126\0\30\0\126\0\36\0\126\0\44" +
"\0\126\0\45\0\126\0\56\0\126\0\60\0\126\0\62\0\126\0\63\0\126\0\64\0\126\0\65\0\126" +
"\0\67\0\126\0\76\0\126\0\77\0\126\0\uffff\uffff\ufffe\uffff\46\0\127\0\47\0\127\0" +
"\50\0\127\0\51\0\127\0\52\0\127\0\72\0\127\0\73\0\127\0\74\0\127\0\75\0\127\0\30" +
"\0\127\0\36\0\127\0\44\0\127\0\45\0\127\0\56\0\127\0\60\0\127\0\62\0\127\0\63\0\127" +
"\0\64\0\127\0\65\0\127\0\67\0\127\0\76\0\127\0\77\0\127\0\uffff\uffff\ufffe\uffff" +
"\46\0\130\0\47\0\130\0\50\0\130\0\51\0\130\0\52\0\130\0\72\0\130\0\73\0\130\0\74" +
"\0\130\0\75\0\130\0\30\0\130\0\36\0\130\0\44\0\130\0\45\0\130\0\56\0\130\0\60\0\130" +
"\0\62\0\130\0\63\0\130\0\64\0\130\0\65\0\130\0\67\0\130\0\76\0\130\0\77\0\130\0\uffff" +
"\uffff\ufffe\uffff\46\0\uffff\uffff\47\0\uffff\uffff\50\0\uffff\uffff\51\0\uffff" +
"\uffff\52\0\uffff\uffff\72\0\135\0\73\0\135\0\74\0\135\0\75\0\135\0\30\0\135\0\36" +
"\0\135\0\44\0\135\0\45\0\135\0\56\0\135\0\60\0\135\0\62\0\135\0\63\0\135\0\64\0\135" +
"\0\65\0\135\0\67\0\135\0\76\0\135\0\77\0\135\0\uffff\uffff\ufffe\uffff\46\0\uffff" +
"\uffff\47\0\uffff\uffff\50\0\uffff\uffff\51\0\uffff\uffff\52\0\uffff\uffff\72\0\136" +
"\0\73\0\136\0\74\0\136\0\75\0\136\0\30\0\136\0\36\0\136\0\44\0\136\0\45\0\136\0\56" +
"\0\136\0\60\0\136\0\62\0\136\0\63\0\136\0\64\0\136\0\65\0\136\0\67\0\136\0\76\0\136" +
"\0\77\0\136\0\uffff\uffff\ufffe\uffff\46\0\uffff\uffff\47\0\uffff\uffff\50\0\uffff" +
"\uffff\51\0\uffff\uffff\52\0\uffff\uffff\72\0\133\0\73\0\133\0\74\0\133\0\75\0\133" +
"\0\30\0\133\0\36\0\133\0\44\0\133\0\45\0\133\0\56\0\133\0\60\0\133\0\62\0\133\0\63" +
"\0\133\0\64\0\133\0\65\0\133\0\67\0\133\0\76\0\133\0\77\0\133\0\uffff\uffff\ufffe" +
"\uffff\46\0\uffff\uffff\47\0\uffff\uffff\50\0\uffff\uffff\51\0\uffff\uffff\52\0\uffff" +
"\uffff\72\0\134\0\73\0\134\0\74\0\134\0\75\0\134\0\30\0\134\0\36\0\134\0\44\0\134" +
"\0\45\0\134\0\56\0\134\0\60\0\134\0\62\0\134\0\63\0\134\0\64\0\134\0\65\0\134\0\67" +
"\0\134\0\76\0\134\0\77\0\134\0\uffff\uffff\ufffe\uffff\61\0\uffff\uffff\30\0\140" +
"\0\36\0\140\0\44\0\140\0\45\0\140\0\56\0\140\0\60\0\140\0\62\0\140\0\63\0\140\0\64" +
"\0\140\0\65\0\140\0\67\0\140\0\76\0\140\0\77\0\140\0\uffff\uffff\ufffe\uffff\30\0" +
"\uffff\uffff\36\0\143\0\44\0\143\0\45\0\143\0\56\0\143\0\60\0\143\0\62\0\143\0\63" +
"\0\143\0\64\0\143\0\65\0\143\0\67\0\143\0\76\0\143\0\77\0\143\0\uffff\uffff\ufffe" +
"\uffff\30\0\uffff\uffff\36\0\144\0\44\0\144\0\45\0\144\0\56\0\144\0\60\0\144\0\62" +
"\0\144\0\63\0\144\0\64\0\144\0\65\0\144\0\67\0\144\0\76\0\144\0\77\0\144\0\uffff" +
"\uffff\ufffe\uffff\63\0\146\0\64\0\146\0\36\0\146\0\44\0\146\0\45\0\146\0\56\0\146" +
"\0\60\0\146\0\62\0\146\0\76\0\146\0\77\0\146\0\uffff\uffff\ufffe\uffff\63\0\uffff" +
"\uffff\64\0\147\0\36\0\147\0\44\0\147\0\45\0\147\0\56\0\147\0\60\0\147\0\62\0\147" +
"\0\76\0\147\0\77\0\147\0\uffff\uffff\ufffe\uffff\36\0\uffff\uffff\62\0\uffff\uffff" +
"\44\0\205\0\45\0\205\0\uffff\uffff\ufffe\uffff\62\0\uffff\uffff\60\0\206\0\uffff" +
"\uffff\ufffe\uffff\7\0\uffff\uffff\10\0\uffff\uffff\11\0\uffff\uffff\20\0\uffff\uffff" +
"\32\0\uffff\uffff\33\0\uffff\uffff\40\0\uffff\uffff\41\0\uffff\uffff\43\0\uffff\uffff" +
"\47\0\uffff\uffff\53\0\uffff\uffff\55\0\uffff\uffff\57\0\uffff\uffff\60\0\201\0\uffff" +
"\uffff\ufffe\uffff\7\0\uffff\uffff\10\0\uffff\uffff\11\0\uffff\uffff\20\0\uffff\uffff" +
"\32\0\uffff\uffff\33\0\uffff\uffff\40\0\uffff\uffff\41\0\uffff\uffff\43\0\uffff\uffff" +
"\47\0\uffff\uffff\53\0\uffff\uffff\55\0\uffff\uffff\57\0\uffff\uffff\60\0\201\0\uffff" +
"\uffff\ufffe\uffff\62\0\uffff\uffff\44\0\43\0\45\0\43\0\uffff\uffff\ufffe\uffff\54" +
"\0\uffff\uffff\57\0\uffff\uffff\30\0\67\0\46\0\67\0\47\0\67\0\50\0\67\0\51\0\67\0" +
"\52\0\67\0\55\0\67\0\60\0\67\0\61\0\67\0\62\0\67\0\63\0\67\0\64\0\67\0\65\0\67\0" +
"\67\0\67\0\70\0\67\0\72\0\67\0\73\0\67\0\74\0\67\0\75\0\67\0\77\0\67\0\uffff\uffff" +
"\ufffe\uffff\62\0\uffff\uffff\44\0\64\0\45\0\64\0\uffff\uffff\ufffe\uffff\7\0\uffff" +
"\uffff\10\0\uffff\uffff\11\0\uffff\uffff\20\0\uffff\uffff\32\0\uffff\uffff\33\0\uffff" +
"\uffff\40\0\uffff\uffff\41\0\uffff\uffff\43\0\uffff\uffff\47\0\uffff\uffff\53\0\uffff" +
"\uffff\55\0\uffff\uffff\57\0\uffff\uffff\60\0\201\0\uffff\uffff\ufffe\uffff\36\0" +
"\uffff\uffff\44\0\205\0\45\0\205\0\uffff\uffff\ufffe\uffff");
private static final int[] lapg_sym_goto = TemplatesLexer.unpack_int(123,
"\0\0\2\0\22\0\37\0\54\0\71\0\111\0\116\0\221\0\305\0\372\0\377\0\u0101\0\u0103\0" +
"\u0107\0\u0109\0\u010e\0\u0142\0\u0148\0\u014d\0\u0152\0\u0152\0\u0158\0\u015a\0" +
"\u015a\0\u015d\0\u015d\0\u0191\0\u01c5\0\u01c6\0\u01cb\0\u01cd\0\u01ce\0\u0202\0" +
"\u0236\0\u023b\0\u026f\0\u027b\0\u0284\0\u028e\0\u02cc\0\u02d6\0\u02e0\0\u02ea\0" +
"\u031e\0\u031f\0\u0355\0\u0359\0\u0399\0\u03a3\0\u03aa\0\u03c0\0\u03c3\0\u03c6\0" +
"\u03c7\0\u03cc\0\u03cd\0\u03ce\0\u03d2\0\u03dc\0\u03e6\0\u03f0\0\u03fa\0\u03fe\0" +
"\u03ff\0\u03ff\0\u0404\0\u0405\0\u0406\0\u0408\0\u040a\0\u040c\0\u040e\0\u0410\0" +
"\u0412\0\u0414\0\u0416\0\u041c\0\u0425\0\u0432\0\u043f\0\u0444\0\u0445\0\u044b\0" +
"\u044c\0\u044d\0\u045a\0\u045c\0\u0469\0\u046a\0\u046c\0\u0479\0\u047e\0\u0480\0" +
"\u0485\0\u04b9\0\u04ed\0\u0521\0\u0523\0\u0526\0\u055a\0\u058e\0\u05c0\0\u05e9\0" +
"\u0610\0\u0637\0\u065c\0\u0671\0\u0685\0\u068b\0\u068c\0\u0691\0\u0692\0\u0694\0" +
"\u0696\0\u0698\0\u0699\0\u069a\0\u069b\0\u06a1\0\u06a2\0\u06a4\0\u06a5\0");
private static final int[] lapg_sym_from = TemplatesLexer.unpack_int(1701,
"\u0103\0\u0104\0\0\0\1\0\4\0\10\0\17\0\24\0\34\0\103\0\212\0\313\0\326\0\341\0\360" +
"\0\371\0\374\0\u0101\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341\0\360\0\371" +
"\0\374\0\u0101\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341\0\360\0\371\0\374" +
"\0\u0101\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341\0\360\0\371\0\374\0\u0101" +
"\0\0\0\1\0\4\0\10\0\17\0\24\0\34\0\103\0\276\0\313\0\326\0\341\0\360\0\371\0\374" +
"\0\u0101\0\103\0\326\0\341\0\371\0\u0101\0\16\0\26\0\32\0\40\0\41\0\43\0\44\0\45" +
"\0\46\0\47\0\51\0\54\0\56\0\57\0\60\0\61\0\106\0\111\0\112\0\125\0\142\0\143\0\144" +
"\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\156\0\157\0\160\0\161\0" +
"\162\0\163\0\164\0\165\0\171\0\172\0\201\0\204\0\210\0\211\0\221\0\223\0\224\0\231" +
"\0\264\0\270\0\277\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0" +
"\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111" +
"\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\157\0\160\0" +
"\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300" +
"\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0" +
"\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0\146" +
"\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\156\0\157\0\160\0\161\0\162\0\163\0" +
"\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310" +
"\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\165\0\346" +
"\0\361\0\3\0\55\0\325\0\346\0\32\0\165\0\346\0\361\0\165\0\346\0\16\0\32\0\165\0" +
"\346\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142" +
"\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0" +
"\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307" +
"\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\165" +
"\0\203\0\346\0\361\0\16\0\32\0\165\0\346\0\361\0\16\0\32\0\165\0\346\0\361\0\16\0" +
"\32\0\165\0\255\0\346\0\361\0\116\0\120\0\73\0\246\0\247\0\16\0\32\0\41\0\44\0\46" +
"\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0" +
"\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210" +
"\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0" +
"\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0" +
"\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155" +
"\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0" +
"\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354" +
"\0\356\0\361\0\30\0\16\0\32\0\165\0\346\0\361\0\271\0\372\0\3\0\16\0\32\0\41\0\44" +
"\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151" +
"\0\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0" +
"\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332" +
"\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57" +
"\0\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155" +
"\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0" +
"\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354" +
"\0\356\0\361\0\16\0\32\0\165\0\346\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56" +
"\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154" +
"\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0" +
"\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346" +
"\0\354\0\356\0\361\0\63\0\64\0\107\0\123\0\174\0\254\0\255\0\331\0\340\0\342\0\365" +
"\0\366\0\63\0\64\0\123\0\174\0\254\0\255\0\340\0\365\0\366\0\72\0\233\0\234\0\235" +
"\0\236\0\237\0\240\0\241\0\242\0\243\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57" +
"\0\60\0\61\0\72\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154" +
"\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0" +
"\231\0\233\0\234\0\235\0\236\0\237\0\240\0\241\0\242\0\243\0\264\0\270\0\300\0\305" +
"\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\72\0\233" +
"\0\234\0\235\0\236\0\237\0\240\0\241\0\242\0\243\0\72\0\233\0\234\0\235\0\236\0\237" +
"\0\240\0\241\0\242\0\243\0\72\0\233\0\234\0\235\0\236\0\237\0\240\0\241\0\242\0\243" +
"\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0" +
"\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0\164" +
"\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0" +
"\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\333\0\16\0\32\0\41\0" +
"\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\65\0\111\0\112\0\142\0\145\0\146\0\147\0" +
"\150\0\151\0\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201" +
"\0\204\0\207\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0" +
"\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\131\0\134\0\227\0\364\0\16" +
"\0\32\0\35\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\105\0\111\0\112\0\113\0" +
"\114\0\122\0\130\0\142\0\144\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155" +
"\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\175\0\201\0\204\0\210\0\221\0\224\0" +
"\230\0\231\0\232\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\333" +
"\0\335\0\343\0\345\0\346\0\354\0\356\0\361\0\135\0\177\0\256\0\263\0\274\0\306\0" +
"\334\0\336\0\367\0\370\0\65\0\105\0\113\0\122\0\175\0\232\0\245\0\100\0\115\0\117" +
"\0\121\0\123\0\124\0\131\0\133\0\135\0\214\0\227\0\271\0\273\0\306\0\317\0\320\0" +
"\331\0\340\0\342\0\344\0\365\0\367\0\75\0\250\0\251\0\75\0\250\0\251\0\74\0\35\0" +
"\130\0\261\0\272\0\302\0\74\0\65\0\130\0\215\0\272\0\302\0\72\0\233\0\234\0\235\0" +
"\236\0\237\0\240\0\241\0\242\0\243\0\72\0\233\0\234\0\235\0\236\0\237\0\240\0\241" +
"\0\242\0\243\0\72\0\233\0\234\0\235\0\236\0\237\0\240\0\241\0\242\0\243\0\72\0\233" +
"\0\234\0\235\0\236\0\237\0\240\0\241\0\242\0\243\0\130\0\252\0\272\0\302\0\75\0\16" +
"\0\32\0\165\0\346\0\361\0\0\0\0\0\0\0\4\0\0\0\4\0\0\0\4\0\3\0\55\0\0\0\4\0\105\0" +
"\175\0\125\0\171\0\10\0\34\0\1\0\10\0\24\0\313\0\360\0\374\0\63\0\64\0\123\0\174" +
"\0\254\0\255\0\340\0\365\0\366\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341\0" +
"\360\0\371\0\374\0\u0101\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341\0\360\0" +
"\371\0\374\0\u0101\0\16\0\32\0\165\0\346\0\361\0\115\0\26\0\40\0\47\0\106\0\144\0" +
"\156\0\203\0\113\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341\0\360\0\371\0\374" +
"\0\u0101\0\103\0\371\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341\0\360\0\371" +
"\0\374\0\u0101\0\276\0\276\0\326\0\1\0\10\0\17\0\24\0\34\0\103\0\313\0\326\0\341" +
"\0\360\0\371\0\374\0\u0101\0\16\0\32\0\165\0\346\0\361\0\271\0\372\0\103\0\326\0" +
"\341\0\371\0\u0101\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0" +
"\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\157\0\160\0\161" +
"\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0" +
"\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\16" +
"\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0\146" +
"\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0" +
"\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312" +
"\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0" +
"\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152" +
"\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0" +
"\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343" +
"\0\345\0\346\0\354\0\356\0\361\0\60\0\211\0\130\0\272\0\302\0\16\0\32\0\41\0\44\0" +
"\46\0\51\0\54\0\56\0\57\0\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151" +
"\0\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0" +
"\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332" +
"\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\56\0\57" +
"\0\60\0\61\0\111\0\112\0\142\0\145\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155" +
"\0\157\0\160\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0" +
"\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354" +
"\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\60\0\61\0\111\0\112\0\142\0\145" +
"\0\146\0\147\0\150\0\151\0\152\0\153\0\154\0\155\0\157\0\160\0\161\0\162\0\163\0" +
"\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310" +
"\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44" +
"\0\46\0\51\0\54\0\60\0\61\0\111\0\112\0\142\0\157\0\160\0\161\0\162\0\163\0\164\0" +
"\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312" +
"\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0" +
"\51\0\54\0\60\0\61\0\111\0\112\0\142\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0" +
"\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332" +
"\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\60\0\61" +
"\0\111\0\112\0\142\0\161\0\162\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0" +
"\231\0\264\0\270\0\300\0\305\0\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346" +
"\0\354\0\356\0\361\0\16\0\32\0\41\0\44\0\46\0\51\0\54\0\60\0\61\0\111\0\112\0\142" +
"\0\163\0\164\0\165\0\201\0\204\0\210\0\221\0\224\0\231\0\264\0\270\0\300\0\305\0" +
"\307\0\310\0\312\0\315\0\321\0\332\0\343\0\345\0\346\0\354\0\356\0\361\0\16\0\32" +
"\0\44\0\46\0\51\0\54\0\61\0\142\0\164\0\165\0\210\0\231\0\264\0\300\0\312\0\315\0" +
"\321\0\345\0\346\0\354\0\361\0\16\0\32\0\44\0\46\0\51\0\54\0\61\0\142\0\165\0\210" +
"\0\231\0\264\0\300\0\312\0\315\0\321\0\345\0\346\0\354\0\361\0\60\0\111\0\201\0\305" +
"\0\307\0\356\0\1\0\16\0\32\0\165\0\346\0\361\0\0\0\3\0\55\0\105\0\175\0\125\0\171" +
"\0\113\0\203\0\115\0\60\0\111\0\201\0\305\0\307\0\356\0\212\0\271\0\372\0\211\0");
private static final int[] lapg_sym_to = TemplatesLexer.unpack_int(1701,
"\u0105\0\u0106\0\2\0\12\0\2\0\12\0\12\0\12\0\12\0\12\0\275\0\12\0\12\0\12\0\12\0" +
"\12\0\12\0\12\0\13\0\13\0\13\0\13\0\13\0\13\0\13\0\13\0\13\0\13\0\13\0\13\0\13\0" +
"\14\0\14\0\14\0\14\0\14\0\14\0\14\0\14\0\14\0\14\0\14\0\14\0\14\0\15\0\15\0\15\0" +
"\15\0\15\0\15\0\15\0\15\0\15\0\15\0\15\0\15\0\15\0\3\0\16\0\3\0\32\0\16\0\16\0\32" +
"\0\165\0\325\0\16\0\346\0\361\0\16\0\165\0\16\0\361\0\166\0\166\0\166\0\166\0\166" +
"\0\35\0\104\0\35\0\104\0\114\0\116\0\35\0\120\0\35\0\104\0\35\0\35\0\114\0\114\0" +
"\130\0\35\0\104\0\114\0\114\0\213\0\35\0\230\0\104\0\114\0\114\0\114\0\114\0\114" +
"\0\114\0\114\0\114\0\114\0\104\0\114\0\114\0\114\0\114\0\114\0\35\0\35\0\213\0\257" +
"\0\114\0\114\0\35\0\272\0\114\0\302\0\114\0\35\0\35\0\114\0\330\0\35\0\333\0\114" +
"\0\114\0\35\0\35\0\35\0\114\0\114\0\35\0\35\0\35\0\114\0\35\0\36\0\36\0\36\0\36\0" +
"\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0" +
"\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0" +
"\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0\36\0" +
"\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0" +
"\37\0\37\0\37\0\37\0\37\0\37\0\37\0\244\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0" +
"\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0\37\0" +
"\37\0\37\0\37\0\37\0\37\0\40\0\40\0\40\0\40\0\40\0\25\0\25\0\345\0\345\0\107\0\254" +
"\0\254\0\254\0\255\0\366\0\41\0\41\0\41\0\41\0\41\0\42\0\42\0\42\0\42\0\42\0\42\0" +
"\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0" +
"\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0" +
"\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\42\0\43\0\43\0" +
"\43\0\264\0\43\0\43\0\44\0\44\0\44\0\44\0\44\0\45\0\45\0\45\0\45\0\45\0\46\0\46\0" +
"\46\0\312\0\46\0\46\0\207\0\210\0\156\0\156\0\156\0\47\0\47\0\47\0\47\0\47\0\47\0" +
"\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0" +
"\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0" +
"\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\47\0\50\0\50\0" +
"\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0" +
"\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0" +
"\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0\50\0" +
"\50\0\50\0\106\0\51\0\51\0\51\0\51\0\51\0\321\0\321\0\26\0\52\0\52\0\52\0\52\0\52" +
"\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52" +
"\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52" +
"\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\52\0\53" +
"\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53" +
"\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53" +
"\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53\0\53" +
"\0\53\0\53\0\53\0\54\0\54\0\54\0\54\0\54\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55" +
"\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55" +
"\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55" +
"\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\55\0\136\0\136\0\176\0" +
"\136\0\136\0\136\0\136\0\352\0\136\0\363\0\136\0\136\0\137\0\137\0\137\0\137\0\137" +
"\0\137\0\137\0\137\0\137\0\145\0\145\0\145\0\145\0\145\0\145\0\145\0\145\0\145\0" +
"\145\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\146\0\56\0\56\0\56" +
"\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56" +
"\0\56\0\56\0\56\0\56\0\56\0\56\0\146\0\146\0\146\0\146\0\146\0\146\0\146\0\146\0" +
"\146\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0\56\0" +
"\56\0\147\0\147\0\147\0\147\0\147\0\147\0\147\0\147\0\147\0\147\0\150\0\150\0\150" +
"\0\150\0\150\0\150\0\150\0\150\0\150\0\150\0\151\0\151\0\151\0\151\0\151\0\151\0" +
"\151\0\151\0\151\0\151\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57" +
"\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57" +
"\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57" +
"\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\57\0\354\0\60\0\60\0\60\0\60\0\60\0\60\0\60" +
"\0\60\0\60\0\60\0\60\0\142\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60" +
"\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\270\0\60\0\60\0\60\0\60\0\60" +
"\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\60\0\222" +
"\0\225\0\304\0\372\0\61\0\61\0\111\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0" +
"\171\0\61\0\61\0\201\0\111\0\211\0\111\0\61\0\231\0\61\0\61\0\61\0\61\0\61\0\61\0" +
"\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\171\0\61\0\61\0\61\0\61\0\61\0" +
"\305\0\61\0\307\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\61\0\111\0\356\0\61" +
"\0\61\0\61\0\61\0\61\0\61\0\226\0\262\0\314\0\316\0\324\0\335\0\355\0\357\0\375\0" +
"\376\0\143\0\172\0\172\0\172\0\172\0\172\0\172\0\164\0\204\0\164\0\164\0\164\0\164" +
"\0\223\0\224\0\164\0\277\0\164\0\164\0\223\0\164\0\164\0\343\0\164\0\164\0\164\0" +
"\164\0\164\0\164\0\161\0\161\0\161\0\162\0\162\0\162\0\157\0\112\0\216\0\315\0\216" +
"\0\216\0\160\0\144\0\217\0\300\0\217\0\217\0\152\0\152\0\152\0\152\0\152\0\152\0" +
"\152\0\152\0\152\0\152\0\153\0\153\0\153\0\153\0\153\0\153\0\153\0\153\0\153\0\153" +
"\0\154\0\154\0\154\0\154\0\154\0\154\0\154\0\154\0\154\0\154\0\155\0\155\0\155\0" +
"\155\0\155\0\155\0\155\0\155\0\155\0\155\0\220\0\310\0\220\0\220\0\163\0\62\0\62" +
"\0\62\0\62\0\62\0\u0103\0\4\0\5\0\31\0\6\0\6\0\7\0\7\0\27\0\27\0\10\0\10\0\173\0" +
"\173\0\214\0\214\0\33\0\110\0\17\0\34\0\103\0\341\0\371\0\u0101\0\140\0\141\0\212" +
"\0\260\0\311\0\313\0\360\0\373\0\374\0\20\0\20\0\102\0\20\0\102\0\102\0\20\0\347" +
"\0\102\0\20\0\102\0\20\0\102\0\21\0\21\0\21\0\21\0\21\0\21\0\21\0\21\0\21\0\21\0" +
"\21\0\21\0\21\0\63\0\63\0\63\0\63\0\63\0\205\0\105\0\113\0\122\0\175\0\232\0\245" +
"\0\265\0\202\0\22\0\22\0\22\0\22\0\22\0\22\0\22\0\22\0\22\0\22\0\22\0\22\0\22\0\167" +
"\0\377\0\23\0\23\0\23\0\23\0\23\0\23\0\23\0\23\0\23\0\23\0\23\0\23\0\23\0\326\0\327" +
"\0\350\0\24\0\24\0\24\0\24\0\24\0\24\0\24\0\24\0\24\0\24\0\24\0\24\0\24\0\64\0\64" +
"\0\64\0\64\0\64\0\322\0\322\0\170\0\351\0\362\0\170\0\u0102\0\65\0\65\0\65\0\65\0" +
"\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0" +
"\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0" +
"\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0\65\0" +
"\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0" +
"\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0" +
"\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0\66\0" +
"\66\0\66\0\66\0\66\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0" +
"\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0" +
"\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0" +
"\67\0\67\0\67\0\67\0\67\0\67\0\67\0\67\0\131\0\273\0\221\0\221\0\332\0\70\0\70\0" +
"\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0" +
"\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0" +
"\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0\70\0" +
"\70\0\70\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\126\0\127\0\71\0\71\0\71\0\71\0\71" +
"\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71" +
"\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71\0\71" +
"\0\71\0\71\0\71\0\71\0\71\0\71\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72" +
"\0\72\0\72\0\233\0\234\0\235\0\236\0\237\0\240\0\241\0\242\0\243\0\72\0\72\0\72\0" +
"\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0" +
"\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\72\0\73\0\73\0\73\0\73\0\73\0\73\0" +
"\73\0\73\0\73\0\73\0\73\0\73\0\246\0\247\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73" +
"\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73\0\73" +
"\0\73\0\73\0\73\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74" +
"\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74" +
"\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\74\0\75\0\75\0\75\0\75\0\75\0\75" +
"\0\75\0\75\0\75\0\75\0\75\0\75\0\250\0\251\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75" +
"\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75\0\75" +
"\0\75\0\76\0\76\0\115\0\76\0\76\0\76\0\76\0\132\0\76\0\132\0\200\0\76\0\252\0\76" +
"\0\76\0\132\0\267\0\76\0\301\0\303\0\76\0\76\0\320\0\76\0\132\0\132\0\337\0\76\0" +
"\76\0\76\0\353\0\364\0\76\0\76\0\76\0\132\0\76\0\77\0\77\0\77\0\77\0\77\0\77\0\77" +
"\0\77\0\253\0\77\0\77\0\77\0\77\0\77\0\77\0\77\0\77\0\77\0\77\0\77\0\77\0\100\0\100" +
"\0\117\0\121\0\123\0\124\0\135\0\227\0\100\0\271\0\306\0\317\0\331\0\340\0\342\0" +
"\344\0\365\0\100\0\367\0\100\0\133\0\133\0\133\0\133\0\133\0\133\0\u0104\0\101\0" +
"\101\0\101\0\101\0\101\0\11\0\30\0\125\0\174\0\261\0\215\0\256\0\203\0\266\0\206" +
"\0\134\0\177\0\263\0\334\0\336\0\370\0\276\0\323\0\u0100\0\274\0");
private static final int[] tmRuleLen = TemplatesLexer.unpack_int(136,
"\1\0\1\0\2\0\1\0\1\0\1\0\3\0\2\0\10\0\1\0\5\0\3\0\1\0\3\0\3\0\2\0\1\0\1\0\1\0\1\0" +
"\1\0\1\0\1\0\1\0\1\0\1\0\3\0\1\0\4\0\3\0\2\0\1\0\2\0\1\0\3\0\2\0\3\0\3\0\7\0\5\0" +
"\1\0\13\0\7\0\1\0\2\0\2\0\4\0\3\0\5\0\11\0\2\0\2\0\2\0\3\0\1\0\1\0\3\0\1\0\1\0\1" +
"\0\1\0\1\0\4\0\3\0\6\0\10\0\6\0\10\0\4\0\1\0\1\0\6\0\3\0\3\0\5\0\3\0\5\0\1\0\1\0" +
"\1\0\1\0\1\0\1\0\2\0\2\0\1\0\3\0\3\0\3\0\3\0\3\0\3\0\3\0\3\0\3\0\1\0\3\0\3\0\1\0" +
"\3\0\3\0\1\0\3\0\3\0\1\0\5\0\1\0\3\0\1\0\3\0\1\0\3\0\1\0\1\0\1\0\0\0\1\0\0\0\1\0" +
"\0\0\1\0\0\0\1\0\0\0\1\0\0\0\1\0\0\0\1\0\0\0\1\0\0\0\1\0\0\0\1\0\0\0");
private static final int[] tmRuleSymbol = TemplatesLexer.unpack_int(136,
"\102\0\103\0\103\0\104\0\104\0\104\0\105\0\105\0\106\0\107\0\110\0\111\0\112\0\112" +
"\0\113\0\114\0\114\0\115\0\115\0\116\0\116\0\116\0\116\0\116\0\116\0\116\0\117\0" +
"\120\0\120\0\120\0\120\0\120\0\121\0\122\0\122\0\123\0\124\0\125\0\126\0\126\0\126" +
"\0\127\0\127\0\130\0\130\0\130\0\131\0\132\0\133\0\133\0\133\0\133\0\134\0\135\0" +
"\135\0\136\0\136\0\136\0\136\0\136\0\136\0\136\0\136\0\136\0\136\0\136\0\136\0\136" +
"\0\136\0\136\0\136\0\137\0\140\0\140\0\140\0\141\0\141\0\142\0\142\0\142\0\143\0" +
"\143\0\144\0\144\0\144\0\145\0\145\0\145\0\145\0\145\0\145\0\145\0\145\0\145\0\145" +
"\0\146\0\146\0\146\0\147\0\147\0\147\0\150\0\150\0\150\0\151\0\151\0\152\0\152\0" +
"\153\0\153\0\154\0\154\0\155\0\156\0\157\0\157\0\160\0\160\0\161\0\161\0\162\0\162" +
"\0\163\0\163\0\164\0\164\0\165\0\165\0\166\0\166\0\167\0\167\0\170\0\170\0\171\0" +
"\171\0");
protected static final String[] tmSymbolNames = new String[] {
"eoi",
"any",
"escdollar",
"escid",
"escint",
"'${'",
"'$/'",
"identifier",
"icon",
"ccon",
"Lcall",
"Lcached",
"Lcase",
"Lend",
"Lelse",
"Leval",
"Lfalse",
"Lfor",
"Lfile",
"Lforeach",
"Lgrep",
"Lif",
"Lin",
"Limport",
"Lis",
"Lmap",
"Lnew",
"Lnull",
"Lquery",
"Lswitch",
"Lseparator",
"Ltemplate",
"Ltrue",
"Lself",
"Lassert",
"'{'",
"'}'",
"'-}'",
"'+'",
"'-'",
"'*'",
"'/'",
"'%'",
"'!'",
"'|'",
"'['",
"']'",
"'('",
"')'",
"'.'",
"','",
"'&&'",
"'||'",
"'=='",
"'='",
"'!='",
"'->'",
"'=>'",
"'<='",
"'>='",
"'<'",
"'>'",
"':'",
"'?'",
"_skip",
"error",
"input",
"definitions",
"definition",
"template_def",
"query_def",
"cached_flag",
"template_start",
"parameters",
"parameter_list",
"template_end",
"instructions",
"'[-]}'",
"instruction",
"simple_instruction",
"sentence",
"comma_expr",
"qualified_id",
"template_for_expr",
"template_arguments",
"control_instruction",
"else_clause",
"switch_instruction",
"case_list",
"one_case",
"control_start",
"control_sentence",
"separator_expr",
"control_end",
"primary_expression",
"closure",
"complex_data",
"map_entries",
"map_separator",
"bcon",
"unary_expression",
"binary_op",
"instanceof_expression",
"equality_expression",
"conditional_op",
"conditional_expression",
"assignment_expression",
"expression",
"expression_list",
"body",
"syntax_problem",
"definitionsopt",
"cached_flagopt",
"parametersopt",
"parameter_listopt",
"template_argumentsopt",
"template_for_expropt",
"comma_expropt",
"expression_listopt",
"anyopt",
"separator_expropt",
"map_entriesopt",
};
public interface Nonterminals extends Tokens {
// non-terminals
int input = 66;
int definitions = 67;
int definition = 68;
int template_def = 69;
int query_def = 70;
int cached_flag = 71;
int template_start = 72;
int parameters = 73;
int parameter_list = 74;
int template_end = 75;
int instructions = 76;
int LbrackMinusRbrackRbrace = 77;
int instruction = 78;
int simple_instruction = 79;
int sentence = 80;
int comma_expr = 81;
int qualified_id = 82;
int template_for_expr = 83;
int template_arguments = 84;
int control_instruction = 85;
int else_clause = 86;
int switch_instruction = 87;
int case_list = 88;
int one_case = 89;
int control_start = 90;
int control_sentence = 91;
int separator_expr = 92;
int control_end = 93;
int primary_expression = 94;
int closure = 95;
int complex_data = 96;
int map_entries = 97;
int map_separator = 98;
int bcon = 99;
int unary_expression = 100;
int binary_op = 101;
int instanceof_expression = 102;
int equality_expression = 103;
int conditional_op = 104;
int conditional_expression = 105;
int assignment_expression = 106;
int expression = 107;
int expression_list = 108;
int body = 109;
int syntax_problem = 110;
int definitionsopt = 111;
int cached_flagopt = 112;
int parametersopt = 113;
int parameter_listopt = 114;
int template_argumentsopt = 115;
int template_for_expropt = 116;
int comma_expropt = 117;
int expression_listopt = 118;
int anyopt = 119;
int separator_expropt = 120;
int map_entriesopt = 121;
}
/**
* -3-n Lookahead (state id)
* -2 Error
* -1 Shift
* 0..n Reduce (rule index)
*/
protected static int tmAction(int state, int symbol) {
int p;
if (tmAction[state] < -2) {
for (p = -tmAction[state] - 3; tmLalr[p] >= 0; p += 2) {
if (tmLalr[p] == symbol) {
break;
}
}
return tmLalr[p + 1];
}
return tmAction[state];
}
protected static int tmGoto(int state, int symbol) {
int min = lapg_sym_goto[symbol], max = lapg_sym_goto[symbol + 1] - 1;
int i, e;
while (min <= max) {
e = (min + max) >> 1;
i = lapg_sym_from[e];
if (i == state) {
return lapg_sym_to[e];
} else if (i < state) {
min = e + 1;
} else {
max = e - 1;
}
}
return -1;
}
protected int tmHead;
protected Span[] tmStack;
protected Span tmNext;
protected TemplatesLexer tmLexer;
private Object parse(TemplatesLexer lexer, int initialState, int finalState) throws IOException, ParseException {
tmLexer = lexer;
tmStack = new Span[1024];
tmHead = 0;
int tmShiftsAfterError = 4;
tmStack[0] = new Span();
tmStack[0].state = initialState;
tmNext = tmLexer.next();
while (tmStack[tmHead].state != finalState) {
int action = tmAction(tmStack[tmHead].state, tmNext.symbol);
if (action >= 0) {
reduce(action);
} else if (action == -1) {
shift();
tmShiftsAfterError++;
}
if (action == -2 || tmStack[tmHead].state == -1) {
if (restore()) {
if (tmShiftsAfterError >= 4) {
reporter.error(MessageFormat.format("syntax error before line {0}", tmLexer.getTokenLine()), tmNext.line, tmNext.offset, tmNext.endoffset);
}
if (tmShiftsAfterError <= 1) {
tmNext = tmLexer.next();
}
tmShiftsAfterError = 0;
continue;
}
if (tmHead < 0) {
tmHead = 0;
tmStack[0] = new Span();
tmStack[0].state = initialState;
}
break;
}
}
if (tmStack[tmHead].state != finalState) {
if (tmShiftsAfterError >= 4) {
reporter.error(MessageFormat.format("syntax error before line {0}",
tmLexer.getTokenLine()), tmNext.line, tmNext.offset, tmNext.endoffset);
}
throw new ParseException();
}
return tmStack[tmHead - 1].value;
}
protected boolean restore() {
if (tmNext.symbol == 0) {
return false;
}
while (tmHead >= 0 && tmGoto(tmStack[tmHead].state, 65) == -1) {
dispose(tmStack[tmHead]);
tmStack[tmHead] = null;
tmHead--;
}
if (tmHead >= 0) {
tmStack[++tmHead] = new Span();
tmStack[tmHead].symbol = 65;
tmStack[tmHead].value = null;
tmStack[tmHead].state = tmGoto(tmStack[tmHead - 1].state, 65);
tmStack[tmHead].line = tmNext.line;
tmStack[tmHead].offset = tmNext.offset;
tmStack[tmHead].endoffset = tmNext.endoffset;
return true;
}
return false;
}
protected void shift() throws IOException {
tmStack[++tmHead] = tmNext;
tmStack[tmHead].state = tmGoto(tmStack[tmHead - 1].state, tmNext.symbol);
if (DEBUG_SYNTAX) {
System.out.println(MessageFormat.format("shift: {0} ({1})", tmSymbolNames[tmNext.symbol], tmLexer.tokenText()));
}
if (tmStack[tmHead].state != -1 && tmNext.symbol != 0) {
tmNext = tmLexer.next();
}
}
protected void reduce(int rule) {
Span left = new Span();
left.value = (tmRuleLen[rule] != 0) ? tmStack[tmHead + 1 - tmRuleLen[rule]].value : null;
left.symbol = tmRuleSymbol[rule];
left.state = 0;
if (DEBUG_SYNTAX) {
System.out.println("reduce to " + tmSymbolNames[tmRuleSymbol[rule]]);
}
Span startsym = (tmRuleLen[rule] != 0) ? tmStack[tmHead + 1 - tmRuleLen[rule]] : tmNext;
left.line = startsym.line;
left.offset = startsym.offset;
left.endoffset = (tmRuleLen[rule] != 0) ? tmStack[tmHead].endoffset : tmNext.offset;
applyRule(left, rule, tmRuleLen[rule]);
for (int e = tmRuleLen[rule]; e > 0; e--) {
cleanup(tmStack[tmHead]);
tmStack[tmHead--] = null;
}
tmStack[++tmHead] = left;
tmStack[tmHead].state = tmGoto(tmStack[tmHead - 1].state, left.symbol);
}
@SuppressWarnings("unchecked")
protected void applyRule(Span tmLeft, int ruleIndex, int ruleLength) {
switch (ruleIndex) {
case 1: // definitions ::= definition
{ tmLeft.value = new ArrayList(); if (((IBundleEntity)tmStack[tmHead].value) != null) ((List)tmLeft.value).add(((IBundleEntity)tmStack[tmHead].value)); }
break;
case 2: // definitions ::= definitions definition
{ if (((IBundleEntity)tmStack[tmHead].value) != null) ((List)tmStack[tmHead - 1].value).add(((IBundleEntity)tmStack[tmHead].value)); }
break;
case 5: // definition ::= any
{ tmLeft.value = null; }
break;
case 6: // template_def ::= template_start instructions template_end
{ ((TemplateNode)tmStack[tmHead - 2].value).setInstructions(((ArrayList)tmStack[tmHead - 1].value)); }
break;
case 8: // query_def ::= '${' cached_flagopt Lquery qualified_id parametersopt '=' expression '}'
{ tmLeft.value = new QueryNode(((String)tmStack[tmHead - 4].value), ((List)tmStack[tmHead - 3].value), templatePackage, ((ExpressionNode)tmStack[tmHead - 1].value), ((Boolean)tmStack[tmHead - 6].value) != null, source, tmLeft.offset, tmLeft.endoffset); checkFqn(((String)tmStack[tmHead - 4].value), tmLeft.offset, tmLeft.endoffset, tmStack[tmHead - 7].line); }
break;
case 9: // cached_flag ::= Lcached
{ tmLeft.value = Boolean.TRUE; }
break;
case 10: // template_start ::= '${' Ltemplate qualified_id parametersopt '[-]}'
{ tmLeft.value = new TemplateNode(((String)tmStack[tmHead - 2].value), ((List)tmStack[tmHead - 1].value), templatePackage, source, tmLeft.offset, tmLeft.endoffset); checkFqn(((String)tmStack[tmHead - 2].value), tmLeft.offset, tmLeft.endoffset, tmStack[tmHead - 4].line); }
break;
case 11: // parameters ::= '(' parameter_listopt ')'
{ tmLeft.value = ((List)tmStack[tmHead - 1].value); }
break;
case 12: // parameter_list ::= identifier
{ tmLeft.value = new ArrayList(); ((List)tmLeft.value).add(new ParameterNode(((String)tmStack[tmHead].value), source, tmStack[tmHead].offset, tmLeft.endoffset)); }
break;
case 13: // parameter_list ::= parameter_list ',' identifier
{ ((List)tmStack[tmHead - 2].value).add(new ParameterNode(((String)tmStack[tmHead].value), source, tmStack[tmHead].offset, tmLeft.endoffset)); }
break;
case 15: // instructions ::= instructions instruction
{ if (((Node)tmStack[tmHead].value) != null) ((ArrayList)tmStack[tmHead - 1].value).add(((Node)tmStack[tmHead].value)); }
break;
case 16: // instructions ::= instruction
{ tmLeft.value = new ArrayList(); if (((Node)tmStack[tmHead].value)!=null) ((ArrayList)tmLeft.value).add(((Node)tmStack[tmHead].value)); }
break;
case 17: // '[-]}' ::= '-}'
{ skipSpaces(tmStack[tmHead].offset+1); }
break;
case 22: // instruction ::= escid
{ tmLeft.value = createEscapedId(((String)tmStack[tmHead].value), tmLeft.offset, tmLeft.endoffset); }
break;
case 23: // instruction ::= escint
{ tmLeft.value = new IndexNode(null, new LiteralNode(((Integer)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 24: // instruction ::= escdollar
{ tmLeft.value = new DollarNode(source, tmLeft.offset, tmLeft.endoffset); }
break;
case 25: // instruction ::= any
{ tmLeft.value = new TextNode(source, rawText(tmLeft.offset, tmLeft.endoffset), tmLeft.endoffset); }
break;
case 26: // simple_instruction ::= '${' sentence '[-]}'
{ tmLeft.value = ((Node)tmStack[tmHead - 1].value); }
break;
case 28: // sentence ::= Lcall qualified_id template_argumentsopt template_for_expropt
{ tmLeft.value = new CallTemplateNode(((String)tmStack[tmHead - 2].value), ((ArrayList)tmStack[tmHead - 1].value), ((ExpressionNode)tmStack[tmHead].value), templatePackage, true, source, tmLeft.offset, tmLeft.endoffset); }
break;
case 29: // sentence ::= Leval conditional_expression comma_expropt
{ tmLeft.value = new EvalNode(((ExpressionNode)tmStack[tmHead - 1].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 30: // sentence ::= Lassert expression
{ tmLeft.value = new AssertNode(((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 31: // sentence ::= syntax_problem
{ tmLeft.value = null; }
break;
case 32: // comma_expr ::= ',' conditional_expression
{ tmLeft.value = ((ExpressionNode)tmStack[tmHead].value); }
break;
case 34: // qualified_id ::= qualified_id '.' identifier
{ tmLeft.value = ((String)tmStack[tmHead - 2].value) + "." + ((String)tmStack[tmHead].value); }
break;
case 35: // template_for_expr ::= Lfor expression
{ tmLeft.value = ((ExpressionNode)tmStack[tmHead].value); }
break;
case 36: // template_arguments ::= '(' expression_listopt ')'
{ tmLeft.value = ((ArrayList)tmStack[tmHead - 1].value); }
break;
case 37: // control_instruction ::= control_start instructions else_clause
{ ((CompoundNode)tmStack[tmHead - 2].value).setInstructions(((ArrayList)tmStack[tmHead - 1].value)); applyElse(((CompoundNode)tmStack[tmHead - 2].value),((ElseIfNode)tmStack[tmHead].value), tmLeft.offset, tmLeft.endoffset, tmLeft.line); }
break;
case 38: // else_clause ::= '${' Lelse Lif expression '[-]}' instructions else_clause
{ tmLeft.value = new ElseIfNode(((ExpressionNode)tmStack[tmHead - 3].value), ((ArrayList)tmStack[tmHead - 1].value), ((ElseIfNode)tmStack[tmHead].value), source, tmStack[tmHead - 6].offset, tmStack[tmHead - 1].endoffset); }
break;
case 39: // else_clause ::= '${' Lelse '[-]}' instructions control_end
{ tmLeft.value = new ElseIfNode(null, ((ArrayList)tmStack[tmHead - 1].value), null, source, tmStack[tmHead - 4].offset, tmStack[tmHead - 1].endoffset); }
break;
case 40: // else_clause ::= control_end
{ tmLeft.value = null; }
break;
case 41: // switch_instruction ::= '${' Lswitch expression '[-]}' anyopt case_list '${' Lelse '[-]}' instructions control_end
{ tmLeft.value = new SwitchNode(((ExpressionNode)tmStack[tmHead - 8].value), ((ArrayList)tmStack[tmHead - 5].value), ((ArrayList)tmStack[tmHead - 1].value), source, tmLeft.offset,tmLeft.endoffset); checkIsSpace(tmStack[tmHead - 6].offset,tmStack[tmHead - 6].endoffset, tmStack[tmHead - 6].line); }
break;
case 42: // switch_instruction ::= '${' Lswitch expression '[-]}' anyopt case_list control_end
{ tmLeft.value = new SwitchNode(((ExpressionNode)tmStack[tmHead - 4].value), ((ArrayList)tmStack[tmHead - 1].value), ((ArrayList)null), source, tmLeft.offset,tmLeft.endoffset); checkIsSpace(tmStack[tmHead - 2].offset,tmStack[tmHead - 2].endoffset, tmStack[tmHead - 2].line); }
break;
case 43: // case_list ::= one_case
{ tmLeft.value = new ArrayList(); ((ArrayList)tmLeft.value).add(((CaseNode)tmStack[tmHead].value)); }
break;
case 44: // case_list ::= case_list one_case
{ ((ArrayList)tmStack[tmHead - 1].value).add(((CaseNode)tmStack[tmHead].value)); }
break;
case 45: // case_list ::= case_list instruction
{ CaseNode.add(((ArrayList)tmStack[tmHead - 1].value), ((Node)tmStack[tmHead].value)); }
break;
case 46: // one_case ::= '${' Lcase expression '[-]}'
{ tmLeft.value = new CaseNode(((ExpressionNode)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 47: // control_start ::= '${' control_sentence '[-]}'
{ tmLeft.value = ((CompoundNode)tmStack[tmHead - 1].value); }
break;
case 48: // control_sentence ::= Lforeach identifier Lin expression separator_expropt
{ tmLeft.value = new ForeachNode(((String)tmStack[tmHead - 3].value), ((ExpressionNode)tmStack[tmHead - 1].value), null, ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 49: // control_sentence ::= Lfor identifier Lin '[' conditional_expression ',' conditional_expression ']' separator_expropt
{ tmLeft.value = new ForeachNode(((String)tmStack[tmHead - 7].value), ((ExpressionNode)tmStack[tmHead - 4].value), ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 50: // control_sentence ::= Lif expression
{ tmLeft.value = new IfNode(((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 51: // control_sentence ::= Lfile expression
{ tmLeft.value = new FileNode(((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 52: // separator_expr ::= Lseparator expression
{ tmLeft.value = ((ExpressionNode)tmStack[tmHead].value); }
break;
case 55: // primary_expression ::= identifier
{ tmLeft.value = new SelectNode(null, ((String)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 56: // primary_expression ::= '(' expression ')'
{ tmLeft.value = new ParenthesesNode(((ExpressionNode)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 57: // primary_expression ::= icon
{ tmLeft.value = new LiteralNode(((Integer)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 58: // primary_expression ::= bcon
{ tmLeft.value = new LiteralNode(((Boolean)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 59: // primary_expression ::= ccon
{ tmLeft.value = new LiteralNode(((String)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 60: // primary_expression ::= Lself
{ tmLeft.value = new ThisNode(source, tmLeft.offset, tmLeft.endoffset); }
break;
case 61: // primary_expression ::= Lnull
{ tmLeft.value = new LiteralNode(null, source, tmLeft.offset, tmLeft.endoffset); }
break;
case 62: // primary_expression ::= identifier '(' expression_listopt ')'
{ tmLeft.value = new MethodCallNode(null, ((String)tmStack[tmHead - 3].value), ((ArrayList)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 63: // primary_expression ::= primary_expression '.' identifier
{ tmLeft.value = new SelectNode(((ExpressionNode)tmStack[tmHead - 2].value), ((String)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 64: // primary_expression ::= primary_expression '.' identifier '(' expression_listopt ')'
{ tmLeft.value = new MethodCallNode(((ExpressionNode)tmStack[tmHead - 5].value), ((String)tmStack[tmHead - 3].value), ((ArrayList)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 65: // primary_expression ::= primary_expression '.' identifier '(' identifier '|' expression ')'
{ tmLeft.value = createCollectionProcessor(((ExpressionNode)tmStack[tmHead - 7].value), ((String)tmStack[tmHead - 5].value), ((String)tmStack[tmHead - 3].value), ((ExpressionNode)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset, tmLeft.line); }
break;
case 66: // primary_expression ::= primary_expression '->' qualified_id '(' expression_listopt ')'
{ tmLeft.value = new CallTemplateNode(((String)tmStack[tmHead - 3].value), ((ArrayList)tmStack[tmHead - 1].value), ((ExpressionNode)tmStack[tmHead - 5].value), templatePackage, false, source, tmLeft.offset, tmLeft.endoffset); }
break;
case 67: // primary_expression ::= primary_expression '->' '(' expression ')' '(' expression_listopt ')'
{ tmLeft.value = new CallTemplateNode(((ExpressionNode)tmStack[tmHead - 4].value),((ArrayList)tmStack[tmHead - 1].value),((ExpressionNode)tmStack[tmHead - 7].value),templatePackage, source, tmLeft.offset, tmLeft.endoffset); }
break;
case 68: // primary_expression ::= primary_expression '[' expression ']'
{ tmLeft.value = new IndexNode(((ExpressionNode)tmStack[tmHead - 3].value), ((ExpressionNode)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 71: // closure ::= '{' cached_flagopt parameter_listopt '=>' expression '}'
{ tmLeft.value = new ClosureNode(((Boolean)tmStack[tmHead - 4].value) != null, ((List)tmStack[tmHead - 3].value), ((ExpressionNode)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 72: // complex_data ::= '[' expression_listopt ']'
{ tmLeft.value = new ListNode(((ArrayList)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 73: // complex_data ::= '[' map_entries ']'
{ tmLeft.value = new ConcreteMapNode(((Map)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 74: // complex_data ::= Lnew qualified_id '(' map_entriesopt ')'
{ tmLeft.value = new CreateClassNode(((String)tmStack[tmHead - 3].value), ((Map)tmStack[tmHead - 1].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 75: // map_entries ::= identifier map_separator conditional_expression
{ tmLeft.value = new LinkedHashMap(); ((Map)tmLeft.value).put(((String)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value)); }
break;
case 76: // map_entries ::= map_entries ',' identifier map_separator conditional_expression
{ ((Map)tmStack[tmHead - 4].value).put(((String)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value)); }
break;
case 80: // bcon ::= Ltrue
{ tmLeft.value = Boolean.TRUE; }
break;
case 81: // bcon ::= Lfalse
{ tmLeft.value = Boolean.FALSE; }
break;
case 83: // unary_expression ::= '!' unary_expression
{ tmLeft.value = new UnaryExpression(UnaryExpression.NOT, ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 84: // unary_expression ::= '-' unary_expression
{ tmLeft.value = new UnaryExpression(UnaryExpression.MINUS, ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 86: // binary_op ::= binary_op '*' binary_op
{ tmLeft.value = new ArithmeticNode(ArithmeticNode.MULT, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 87: // binary_op ::= binary_op '/' binary_op
{ tmLeft.value = new ArithmeticNode(ArithmeticNode.DIV, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 88: // binary_op ::= binary_op '%' binary_op
{ tmLeft.value = new ArithmeticNode(ArithmeticNode.REM, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 89: // binary_op ::= binary_op '+' binary_op
{ tmLeft.value = new ArithmeticNode(ArithmeticNode.PLUS, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 90: // binary_op ::= binary_op '-' binary_op
{ tmLeft.value = new ArithmeticNode(ArithmeticNode.MINUS, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 91: // binary_op ::= binary_op '<' binary_op
{ tmLeft.value = new ConditionalNode(ConditionalNode.LT, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 92: // binary_op ::= binary_op '>' binary_op
{ tmLeft.value = new ConditionalNode(ConditionalNode.GT, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 93: // binary_op ::= binary_op '<=' binary_op
{ tmLeft.value = new ConditionalNode(ConditionalNode.LE, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 94: // binary_op ::= binary_op '>=' binary_op
{ tmLeft.value = new ConditionalNode(ConditionalNode.GE, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 96: // instanceof_expression ::= instanceof_expression Lis qualified_id
{ tmLeft.value = new InstanceOfNode(((ExpressionNode)tmStack[tmHead - 2].value), ((String)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 97: // instanceof_expression ::= instanceof_expression Lis ccon
{ tmLeft.value = new InstanceOfNode(((ExpressionNode)tmStack[tmHead - 2].value), ((String)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 99: // equality_expression ::= equality_expression '==' instanceof_expression
{ tmLeft.value = new ConditionalNode(ConditionalNode.EQ, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 100: // equality_expression ::= equality_expression '!=' instanceof_expression
{ tmLeft.value = new ConditionalNode(ConditionalNode.NE, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 102: // conditional_op ::= conditional_op '&&' conditional_op
{ tmLeft.value = new ConditionalNode(ConditionalNode.AND, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 103: // conditional_op ::= conditional_op '||' conditional_op
{ tmLeft.value = new ConditionalNode(ConditionalNode.OR, ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 105: // conditional_expression ::= conditional_op '?' conditional_expression ':' conditional_expression
{ tmLeft.value = new TriplexNode(((ExpressionNode)tmStack[tmHead - 4].value), ((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 107: // assignment_expression ::= identifier '=' conditional_expression
{ tmLeft.value = new AssignNode(((String)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 109: // expression ::= expression ',' assignment_expression
{ tmLeft.value = new CommaNode(((ExpressionNode)tmStack[tmHead - 2].value), ((ExpressionNode)tmStack[tmHead].value), source, tmLeft.offset, tmLeft.endoffset); }
break;
case 110: // expression_list ::= conditional_expression
{ tmLeft.value = new ArrayList(); ((ArrayList)tmLeft.value).add(((ExpressionNode)tmStack[tmHead].value)); }
break;
case 111: // expression_list ::= expression_list ',' conditional_expression
{ ((ArrayList)tmStack[tmHead - 2].value).add(((ExpressionNode)tmStack[tmHead].value)); }
break;
case 112: // body ::= instructions
{
tmLeft.value = new TemplateNode("inline", null, templatePackage, source, tmLeft.offset, tmLeft.endoffset);
((TemplateNode)tmLeft.value).setInstructions(((ArrayList)tmStack[tmHead].value));
}
break;
}
}
/**
* disposes symbol dropped by error recovery mechanism
*/
protected void dispose(Span value) {
}
/**
* cleans node removed from the stack
*/
protected void cleanup(Span value) {
}
public List parseInput(TemplatesLexer lexer) throws IOException, ParseException {
return (List) parse(lexer, 0, 261);
}
public TemplateNode parseBody(TemplatesLexer lexer) throws IOException, ParseException {
return (TemplateNode) parse(lexer, 1, 262);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy