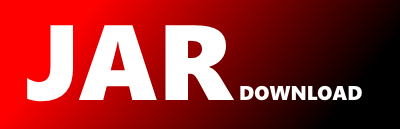
org.theta4j.webapi.Theta.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of theta-web-api Show documentation
Show all versions of theta-web-api Show documentation
Client implementation of RICOH THETA API.
The newest version!
/*
* Copyright (C) 2022 theta4j project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.theta4j.webapi
import org.theta4j.osc.*
import org.theta4j.osc.OSCClient.Companion.createWithDigestAuthentication
import java.net.URI
class Theta private constructor(private val oscClient: OSCClient) {
companion object {
private const val DEFAULT_ENDPOINT = "http://192.168.1.1"
private const val INTERNAL_ENDPOINT = "http:127.0.0.1:8080"
fun create(): Theta = create(DEFAULT_ENDPOINT)
fun create(endpoint: String): Theta = Theta(OSCClient.create(endpoint))
fun create(endpoint: String, username: String, password: String): Theta {
val oscClient = createWithDigestAuthentication(endpoint, username, password)
return Theta(oscClient)
}
fun createForPlugin(): Theta = Theta(OSCClient.create(INTERNAL_ENDPOINT))
}
suspend fun info(): ThetaInfo = oscClient.info(ThetaInfo.serializer())
suspend fun state(): OSCState = oscClient.state(ThetaState.serializer())
suspend fun checkForUpdates(fingerprint: String): String = oscClient.checkForUpdates(fingerprint)
suspend fun commandStatus(response: CommandResponse): CommandResponse = oscClient.commandStatus(response)
suspend fun await(response: CommandResponse): CommandResponse = oscClient.await(response)
suspend fun getOption(option: Option): T = oscClient.getOption(option)
suspend fun getOption(option: ArrayOption): List = oscClient.getOption(option)
suspend fun getOptions(vararg options: Option<*>): OptionSet = oscClient.getOptions(*options)
suspend fun getOptions(options: Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy