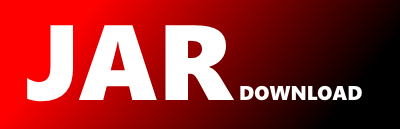
com.google.api.HttpRule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of management Show documentation
Show all versions of management Show documentation
The Things Network Handler API Client
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: google/api/http.proto
package com.google.api;
/**
*
* `HttpRule` defines the mapping of an RPC method to one or more HTTP REST API
* methods. The mapping determines what portions of the request message are
* populated from the path, query parameters, or body of the HTTP request. The
* mapping is typically specified as an `google.api.http` annotation, see
* "google/api/annotations.proto" for details.
* The mapping consists of a mandatory field specifying a path template and an
* optional `body` field specifying what data is represented in the HTTP request
* body. The field name for the path indicates the HTTP method. Example:
* ```
* package google.storage.v2;
* import "google/api/annotations.proto";
* service Storage {
* rpc CreateObject(CreateObjectRequest) returns (Object) {
* option (google.api.http) {
* post: "/v2/{bucket_name=buckets/*}/objects"
* body: "object"
* };
* };
* }
* ```
* Here `bucket_name` and `object` bind to fields of the request message
* `CreateObjectRequest`.
* The rules for mapping HTTP path, query parameters, and body fields
* to the request message are as follows:
* 1. The `body` field specifies either `*` or a field path, or is
* omitted. If omitted, it assumes there is no HTTP body.
* 2. Leaf fields (recursive expansion of nested messages in the
* request) can be classified into three types:
* (a) Matched in the URL template.
* (b) Covered by body (if body is `*`, everything except (a) fields;
* else everything under the body field)
* (c) All other fields.
* 3. URL query parameters found in the HTTP request are mapped to (c) fields.
* 4. Any body sent with an HTTP request can contain only (b) fields.
* The syntax of the path template is as follows:
* Template = "/" Segments [ Verb ] ;
* Segments = Segment { "/" Segment } ;
* Segment = "*" | "**" | LITERAL | Variable ;
* Variable = "{" FieldPath [ "=" Segments ] "}" ;
* FieldPath = IDENT { "." IDENT } ;
* Verb = ":" LITERAL ;
* `*` matches a single path component, `**` zero or more path components, and
* `LITERAL` a constant. A `Variable` can match an entire path as specified
* again by a template; this nested template must not contain further variables.
* If no template is given with a variable, it matches a single path component.
* The notation `{var}` is henceforth equivalent to `{var=*}`.
* Use CustomHttpPattern to specify any HTTP method that is not included in the
* pattern field, such as HEAD, or "*" to leave the HTTP method unspecified for
* a given URL path rule. The wild-card rule is useful for services that provide
* content to Web (HTML) clients.
*
*
* Protobuf type {@code google.api.HttpRule}
*/
public final class HttpRule extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:google.api.HttpRule)
HttpRuleOrBuilder {
// Use HttpRule.newBuilder() to construct.
private HttpRule(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private HttpRule() {
body_ = "";
additionalBindings_ = java.util.Collections.emptyList();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return com.google.protobuf.UnknownFieldSet.getDefaultInstance();
}
private HttpRule(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
int mutable_bitField0_ = 0;
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
default: {
if (!input.skipField(tag)) {
done = true;
}
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
patternCase_ = 2;
pattern_ = s;
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
patternCase_ = 3;
pattern_ = s;
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
patternCase_ = 4;
pattern_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
patternCase_ = 5;
pattern_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
patternCase_ = 6;
pattern_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
body_ = s;
break;
}
case 66: {
com.google.api.CustomHttpPattern.Builder subBuilder = null;
if (patternCase_ == 8) {
subBuilder = ((com.google.api.CustomHttpPattern) pattern_).toBuilder();
}
pattern_ =
input.readMessage(com.google.api.CustomHttpPattern.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((com.google.api.CustomHttpPattern) pattern_);
pattern_ = subBuilder.buildPartial();
}
patternCase_ = 8;
break;
}
case 90: {
if (!((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
additionalBindings_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000080;
}
additionalBindings_.add(input.readMessage(com.google.api.HttpRule.parser(), extensionRegistry));
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000080) == 0x00000080)) {
additionalBindings_ = java.util.Collections.unmodifiableList(additionalBindings_);
}
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.api.HttpProto.internal_static_google_api_HttpRule_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.api.HttpProto.internal_static_google_api_HttpRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.api.HttpRule.class, com.google.api.HttpRule.Builder.class);
}
private int bitField0_;
private int patternCase_ = 0;
private java.lang.Object pattern_;
public enum PatternCase
implements com.google.protobuf.Internal.EnumLite {
GET(2),
PUT(3),
POST(4),
DELETE(5),
PATCH(6),
CUSTOM(8),
PATTERN_NOT_SET(0);
private final int value;
private PatternCase(int value) {
this.value = value;
}
/**
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static PatternCase valueOf(int value) {
return forNumber(value);
}
public static PatternCase forNumber(int value) {
switch (value) {
case 2: return GET;
case 3: return PUT;
case 4: return POST;
case 5: return DELETE;
case 6: return PATCH;
case 8: return CUSTOM;
case 0: return PATTERN_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public PatternCase
getPatternCase() {
return PatternCase.forNumber(
patternCase_);
}
public static final int GET_FIELD_NUMBER = 2;
/**
*
* Used for listing and getting information about resources.
*
*
* optional string get = 2;
*/
public java.lang.String getGet() {
java.lang.Object ref = "";
if (patternCase_ == 2) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 2) {
pattern_ = s;
}
return s;
}
}
/**
*
* Used for listing and getting information about resources.
*
*
* optional string get = 2;
*/
public com.google.protobuf.ByteString
getGetBytes() {
java.lang.Object ref = "";
if (patternCase_ == 2) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 2) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PUT_FIELD_NUMBER = 3;
/**
*
* Used for updating a resource.
*
*
* optional string put = 3;
*/
public java.lang.String getPut() {
java.lang.Object ref = "";
if (patternCase_ == 3) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 3) {
pattern_ = s;
}
return s;
}
}
/**
*
* Used for updating a resource.
*
*
* optional string put = 3;
*/
public com.google.protobuf.ByteString
getPutBytes() {
java.lang.Object ref = "";
if (patternCase_ == 3) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 3) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int POST_FIELD_NUMBER = 4;
/**
*
* Used for creating a resource.
*
*
* optional string post = 4;
*/
public java.lang.String getPost() {
java.lang.Object ref = "";
if (patternCase_ == 4) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 4) {
pattern_ = s;
}
return s;
}
}
/**
*
* Used for creating a resource.
*
*
* optional string post = 4;
*/
public com.google.protobuf.ByteString
getPostBytes() {
java.lang.Object ref = "";
if (patternCase_ == 4) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 4) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DELETE_FIELD_NUMBER = 5;
/**
*
* Used for deleting a resource.
*
*
* optional string delete = 5;
*/
public java.lang.String getDelete() {
java.lang.Object ref = "";
if (patternCase_ == 5) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 5) {
pattern_ = s;
}
return s;
}
}
/**
*
* Used for deleting a resource.
*
*
* optional string delete = 5;
*/
public com.google.protobuf.ByteString
getDeleteBytes() {
java.lang.Object ref = "";
if (patternCase_ == 5) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 5) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int PATCH_FIELD_NUMBER = 6;
/**
*
* Used for updating a resource.
*
*
* optional string patch = 6;
*/
public java.lang.String getPatch() {
java.lang.Object ref = "";
if (patternCase_ == 6) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 6) {
pattern_ = s;
}
return s;
}
}
/**
*
* Used for updating a resource.
*
*
* optional string patch = 6;
*/
public com.google.protobuf.ByteString
getPatchBytes() {
java.lang.Object ref = "";
if (patternCase_ == 6) {
ref = pattern_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 6) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CUSTOM_FIELD_NUMBER = 8;
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public com.google.api.CustomHttpPattern getCustom() {
if (patternCase_ == 8) {
return (com.google.api.CustomHttpPattern) pattern_;
}
return com.google.api.CustomHttpPattern.getDefaultInstance();
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public com.google.api.CustomHttpPatternOrBuilder getCustomOrBuilder() {
if (patternCase_ == 8) {
return (com.google.api.CustomHttpPattern) pattern_;
}
return com.google.api.CustomHttpPattern.getDefaultInstance();
}
public static final int BODY_FIELD_NUMBER = 7;
private volatile java.lang.Object body_;
/**
*
* The name of the request field whose value is mapped to the HTTP body, or
* `*` for mapping all fields not captured by the path pattern to the HTTP
* body.
*
*
* optional string body = 7;
*/
public java.lang.String getBody() {
java.lang.Object ref = body_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
body_ = s;
return s;
}
}
/**
*
* The name of the request field whose value is mapped to the HTTP body, or
* `*` for mapping all fields not captured by the path pattern to the HTTP
* body.
*
*
* optional string body = 7;
*/
public com.google.protobuf.ByteString
getBodyBytes() {
java.lang.Object ref = body_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
body_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ADDITIONAL_BINDINGS_FIELD_NUMBER = 11;
private java.util.List additionalBindings_;
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public java.util.List getAdditionalBindingsList() {
return additionalBindings_;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public java.util.List extends com.google.api.HttpRuleOrBuilder>
getAdditionalBindingsOrBuilderList() {
return additionalBindings_;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public int getAdditionalBindingsCount() {
return additionalBindings_.size();
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public com.google.api.HttpRule getAdditionalBindings(int index) {
return additionalBindings_.get(index);
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public com.google.api.HttpRuleOrBuilder getAdditionalBindingsOrBuilder(
int index) {
return additionalBindings_.get(index);
}
private byte memoizedIsInitialized = -1;
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (patternCase_ == 2) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, pattern_);
}
if (patternCase_ == 3) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, pattern_);
}
if (patternCase_ == 4) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, pattern_);
}
if (patternCase_ == 5) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, pattern_);
}
if (patternCase_ == 6) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, pattern_);
}
if (!getBodyBytes().isEmpty()) {
com.google.protobuf.GeneratedMessage.writeString(output, 7, body_);
}
if (patternCase_ == 8) {
output.writeMessage(8, (com.google.api.CustomHttpPattern) pattern_);
}
for (int i = 0; i < additionalBindings_.size(); i++) {
output.writeMessage(11, additionalBindings_.get(i));
}
}
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (patternCase_ == 2) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, pattern_);
}
if (patternCase_ == 3) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, pattern_);
}
if (patternCase_ == 4) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, pattern_);
}
if (patternCase_ == 5) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, pattern_);
}
if (patternCase_ == 6) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(6, pattern_);
}
if (!getBodyBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(7, body_);
}
if (patternCase_ == 8) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, (com.google.api.CustomHttpPattern) pattern_);
}
for (int i = 0; i < additionalBindings_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(11, additionalBindings_.get(i));
}
memoizedSize = size;
return size;
}
private static final long serialVersionUID = 0L;
public static com.google.api.HttpRule parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.api.HttpRule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.api.HttpRule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static com.google.api.HttpRule parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static com.google.api.HttpRule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.api.HttpRule parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.api.HttpRule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static com.google.api.HttpRule parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static com.google.api.HttpRule parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static com.google.api.HttpRule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(com.google.api.HttpRule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* `HttpRule` defines the mapping of an RPC method to one or more HTTP REST API
* methods. The mapping determines what portions of the request message are
* populated from the path, query parameters, or body of the HTTP request. The
* mapping is typically specified as an `google.api.http` annotation, see
* "google/api/annotations.proto" for details.
* The mapping consists of a mandatory field specifying a path template and an
* optional `body` field specifying what data is represented in the HTTP request
* body. The field name for the path indicates the HTTP method. Example:
* ```
* package google.storage.v2;
* import "google/api/annotations.proto";
* service Storage {
* rpc CreateObject(CreateObjectRequest) returns (Object) {
* option (google.api.http) {
* post: "/v2/{bucket_name=buckets/*}/objects"
* body: "object"
* };
* };
* }
* ```
* Here `bucket_name` and `object` bind to fields of the request message
* `CreateObjectRequest`.
* The rules for mapping HTTP path, query parameters, and body fields
* to the request message are as follows:
* 1. The `body` field specifies either `*` or a field path, or is
* omitted. If omitted, it assumes there is no HTTP body.
* 2. Leaf fields (recursive expansion of nested messages in the
* request) can be classified into three types:
* (a) Matched in the URL template.
* (b) Covered by body (if body is `*`, everything except (a) fields;
* else everything under the body field)
* (c) All other fields.
* 3. URL query parameters found in the HTTP request are mapped to (c) fields.
* 4. Any body sent with an HTTP request can contain only (b) fields.
* The syntax of the path template is as follows:
* Template = "/" Segments [ Verb ] ;
* Segments = Segment { "/" Segment } ;
* Segment = "*" | "**" | LITERAL | Variable ;
* Variable = "{" FieldPath [ "=" Segments ] "}" ;
* FieldPath = IDENT { "." IDENT } ;
* Verb = ":" LITERAL ;
* `*` matches a single path component, `**` zero or more path components, and
* `LITERAL` a constant. A `Variable` can match an entire path as specified
* again by a template; this nested template must not contain further variables.
* If no template is given with a variable, it matches a single path component.
* The notation `{var}` is henceforth equivalent to `{var=*}`.
* Use CustomHttpPattern to specify any HTTP method that is not included in the
* pattern field, such as HEAD, or "*" to leave the HTTP method unspecified for
* a given URL path rule. The wild-card rule is useful for services that provide
* content to Web (HTML) clients.
*
*
* Protobuf type {@code google.api.HttpRule}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:google.api.HttpRule)
com.google.api.HttpRuleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return com.google.api.HttpProto.internal_static_google_api_HttpRule_descriptor;
}
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return com.google.api.HttpProto.internal_static_google_api_HttpRule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
com.google.api.HttpRule.class, com.google.api.HttpRule.Builder.class);
}
// Construct using com.google.api.HttpRule.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders) {
getAdditionalBindingsFieldBuilder();
}
}
public Builder clear() {
super.clear();
body_ = "";
if (additionalBindingsBuilder_ == null) {
additionalBindings_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
} else {
additionalBindingsBuilder_.clear();
}
patternCase_ = 0;
pattern_ = null;
return this;
}
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return com.google.api.HttpProto.internal_static_google_api_HttpRule_descriptor;
}
public com.google.api.HttpRule getDefaultInstanceForType() {
return com.google.api.HttpRule.getDefaultInstance();
}
public com.google.api.HttpRule build() {
com.google.api.HttpRule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
public com.google.api.HttpRule buildPartial() {
com.google.api.HttpRule result = new com.google.api.HttpRule(this);
int from_bitField0_ = bitField0_;
int to_bitField0_ = 0;
if (patternCase_ == 2) {
result.pattern_ = pattern_;
}
if (patternCase_ == 3) {
result.pattern_ = pattern_;
}
if (patternCase_ == 4) {
result.pattern_ = pattern_;
}
if (patternCase_ == 5) {
result.pattern_ = pattern_;
}
if (patternCase_ == 6) {
result.pattern_ = pattern_;
}
if (patternCase_ == 8) {
if (customBuilder_ == null) {
result.pattern_ = pattern_;
} else {
result.pattern_ = customBuilder_.build();
}
}
result.body_ = body_;
if (additionalBindingsBuilder_ == null) {
if (((bitField0_ & 0x00000080) == 0x00000080)) {
additionalBindings_ = java.util.Collections.unmodifiableList(additionalBindings_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.additionalBindings_ = additionalBindings_;
} else {
result.additionalBindings_ = additionalBindingsBuilder_.build();
}
result.bitField0_ = to_bitField0_;
result.patternCase_ = patternCase_;
onBuilt();
return result;
}
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof com.google.api.HttpRule) {
return mergeFrom((com.google.api.HttpRule)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(com.google.api.HttpRule other) {
if (other == com.google.api.HttpRule.getDefaultInstance()) return this;
if (!other.getBody().isEmpty()) {
body_ = other.body_;
onChanged();
}
if (additionalBindingsBuilder_ == null) {
if (!other.additionalBindings_.isEmpty()) {
if (additionalBindings_.isEmpty()) {
additionalBindings_ = other.additionalBindings_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureAdditionalBindingsIsMutable();
additionalBindings_.addAll(other.additionalBindings_);
}
onChanged();
}
} else {
if (!other.additionalBindings_.isEmpty()) {
if (additionalBindingsBuilder_.isEmpty()) {
additionalBindingsBuilder_.dispose();
additionalBindingsBuilder_ = null;
additionalBindings_ = other.additionalBindings_;
bitField0_ = (bitField0_ & ~0x00000080);
additionalBindingsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getAdditionalBindingsFieldBuilder() : null;
} else {
additionalBindingsBuilder_.addAllMessages(other.additionalBindings_);
}
}
}
switch (other.getPatternCase()) {
case GET: {
patternCase_ = 2;
pattern_ = other.pattern_;
onChanged();
break;
}
case PUT: {
patternCase_ = 3;
pattern_ = other.pattern_;
onChanged();
break;
}
case POST: {
patternCase_ = 4;
pattern_ = other.pattern_;
onChanged();
break;
}
case DELETE: {
patternCase_ = 5;
pattern_ = other.pattern_;
onChanged();
break;
}
case PATCH: {
patternCase_ = 6;
pattern_ = other.pattern_;
onChanged();
break;
}
case CUSTOM: {
mergeCustom(other.getCustom());
break;
}
case PATTERN_NOT_SET: {
break;
}
}
onChanged();
return this;
}
public final boolean isInitialized() {
return true;
}
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
com.google.api.HttpRule parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (com.google.api.HttpRule) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int patternCase_ = 0;
private java.lang.Object pattern_;
public PatternCase
getPatternCase() {
return PatternCase.forNumber(
patternCase_);
}
public Builder clearPattern() {
patternCase_ = 0;
pattern_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
*
* Used for listing and getting information about resources.
*
*
* optional string get = 2;
*/
public java.lang.String getGet() {
java.lang.Object ref = "";
if (patternCase_ == 2) {
ref = pattern_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 2) {
pattern_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used for listing and getting information about resources.
*
*
* optional string get = 2;
*/
public com.google.protobuf.ByteString
getGetBytes() {
java.lang.Object ref = "";
if (patternCase_ == 2) {
ref = pattern_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 2) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used for listing and getting information about resources.
*
*
* optional string get = 2;
*/
public Builder setGet(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
patternCase_ = 2;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for listing and getting information about resources.
*
*
* optional string get = 2;
*/
public Builder clearGet() {
if (patternCase_ == 2) {
patternCase_ = 0;
pattern_ = null;
onChanged();
}
return this;
}
/**
*
* Used for listing and getting information about resources.
*
*
* optional string get = 2;
*/
public Builder setGetBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
patternCase_ = 2;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for updating a resource.
*
*
* optional string put = 3;
*/
public java.lang.String getPut() {
java.lang.Object ref = "";
if (patternCase_ == 3) {
ref = pattern_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 3) {
pattern_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used for updating a resource.
*
*
* optional string put = 3;
*/
public com.google.protobuf.ByteString
getPutBytes() {
java.lang.Object ref = "";
if (patternCase_ == 3) {
ref = pattern_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 3) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used for updating a resource.
*
*
* optional string put = 3;
*/
public Builder setPut(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
patternCase_ = 3;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for updating a resource.
*
*
* optional string put = 3;
*/
public Builder clearPut() {
if (patternCase_ == 3) {
patternCase_ = 0;
pattern_ = null;
onChanged();
}
return this;
}
/**
*
* Used for updating a resource.
*
*
* optional string put = 3;
*/
public Builder setPutBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
patternCase_ = 3;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for creating a resource.
*
*
* optional string post = 4;
*/
public java.lang.String getPost() {
java.lang.Object ref = "";
if (patternCase_ == 4) {
ref = pattern_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 4) {
pattern_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used for creating a resource.
*
*
* optional string post = 4;
*/
public com.google.protobuf.ByteString
getPostBytes() {
java.lang.Object ref = "";
if (patternCase_ == 4) {
ref = pattern_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 4) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used for creating a resource.
*
*
* optional string post = 4;
*/
public Builder setPost(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
patternCase_ = 4;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for creating a resource.
*
*
* optional string post = 4;
*/
public Builder clearPost() {
if (patternCase_ == 4) {
patternCase_ = 0;
pattern_ = null;
onChanged();
}
return this;
}
/**
*
* Used for creating a resource.
*
*
* optional string post = 4;
*/
public Builder setPostBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
patternCase_ = 4;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for deleting a resource.
*
*
* optional string delete = 5;
*/
public java.lang.String getDelete() {
java.lang.Object ref = "";
if (patternCase_ == 5) {
ref = pattern_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 5) {
pattern_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used for deleting a resource.
*
*
* optional string delete = 5;
*/
public com.google.protobuf.ByteString
getDeleteBytes() {
java.lang.Object ref = "";
if (patternCase_ == 5) {
ref = pattern_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 5) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used for deleting a resource.
*
*
* optional string delete = 5;
*/
public Builder setDelete(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
patternCase_ = 5;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for deleting a resource.
*
*
* optional string delete = 5;
*/
public Builder clearDelete() {
if (patternCase_ == 5) {
patternCase_ = 0;
pattern_ = null;
onChanged();
}
return this;
}
/**
*
* Used for deleting a resource.
*
*
* optional string delete = 5;
*/
public Builder setDeleteBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
patternCase_ = 5;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for updating a resource.
*
*
* optional string patch = 6;
*/
public java.lang.String getPatch() {
java.lang.Object ref = "";
if (patternCase_ == 6) {
ref = pattern_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (patternCase_ == 6) {
pattern_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Used for updating a resource.
*
*
* optional string patch = 6;
*/
public com.google.protobuf.ByteString
getPatchBytes() {
java.lang.Object ref = "";
if (patternCase_ == 6) {
ref = pattern_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (patternCase_ == 6) {
pattern_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Used for updating a resource.
*
*
* optional string patch = 6;
*/
public Builder setPatch(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
patternCase_ = 6;
pattern_ = value;
onChanged();
return this;
}
/**
*
* Used for updating a resource.
*
*
* optional string patch = 6;
*/
public Builder clearPatch() {
if (patternCase_ == 6) {
patternCase_ = 0;
pattern_ = null;
onChanged();
}
return this;
}
/**
*
* Used for updating a resource.
*
*
* optional string patch = 6;
*/
public Builder setPatchBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
patternCase_ = 6;
pattern_ = value;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilder<
com.google.api.CustomHttpPattern, com.google.api.CustomHttpPattern.Builder, com.google.api.CustomHttpPatternOrBuilder> customBuilder_;
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public com.google.api.CustomHttpPattern getCustom() {
if (customBuilder_ == null) {
if (patternCase_ == 8) {
return (com.google.api.CustomHttpPattern) pattern_;
}
return com.google.api.CustomHttpPattern.getDefaultInstance();
} else {
if (patternCase_ == 8) {
return customBuilder_.getMessage();
}
return com.google.api.CustomHttpPattern.getDefaultInstance();
}
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public Builder setCustom(com.google.api.CustomHttpPattern value) {
if (customBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
pattern_ = value;
onChanged();
} else {
customBuilder_.setMessage(value);
}
patternCase_ = 8;
return this;
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public Builder setCustom(
com.google.api.CustomHttpPattern.Builder builderForValue) {
if (customBuilder_ == null) {
pattern_ = builderForValue.build();
onChanged();
} else {
customBuilder_.setMessage(builderForValue.build());
}
patternCase_ = 8;
return this;
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public Builder mergeCustom(com.google.api.CustomHttpPattern value) {
if (customBuilder_ == null) {
if (patternCase_ == 8 &&
pattern_ != com.google.api.CustomHttpPattern.getDefaultInstance()) {
pattern_ = com.google.api.CustomHttpPattern.newBuilder((com.google.api.CustomHttpPattern) pattern_)
.mergeFrom(value).buildPartial();
} else {
pattern_ = value;
}
onChanged();
} else {
if (patternCase_ == 8) {
customBuilder_.mergeFrom(value);
}
customBuilder_.setMessage(value);
}
patternCase_ = 8;
return this;
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public Builder clearCustom() {
if (customBuilder_ == null) {
if (patternCase_ == 8) {
patternCase_ = 0;
pattern_ = null;
onChanged();
}
} else {
if (patternCase_ == 8) {
patternCase_ = 0;
pattern_ = null;
}
customBuilder_.clear();
}
return this;
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public com.google.api.CustomHttpPattern.Builder getCustomBuilder() {
return getCustomFieldBuilder().getBuilder();
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
public com.google.api.CustomHttpPatternOrBuilder getCustomOrBuilder() {
if ((patternCase_ == 8) && (customBuilder_ != null)) {
return customBuilder_.getMessageOrBuilder();
} else {
if (patternCase_ == 8) {
return (com.google.api.CustomHttpPattern) pattern_;
}
return com.google.api.CustomHttpPattern.getDefaultInstance();
}
}
/**
*
* Custom pattern is used for defining custom verbs.
*
*
* optional .google.api.CustomHttpPattern custom = 8;
*/
private com.google.protobuf.SingleFieldBuilder<
com.google.api.CustomHttpPattern, com.google.api.CustomHttpPattern.Builder, com.google.api.CustomHttpPatternOrBuilder>
getCustomFieldBuilder() {
if (customBuilder_ == null) {
if (!(patternCase_ == 8)) {
pattern_ = com.google.api.CustomHttpPattern.getDefaultInstance();
}
customBuilder_ = new com.google.protobuf.SingleFieldBuilder<
com.google.api.CustomHttpPattern, com.google.api.CustomHttpPattern.Builder, com.google.api.CustomHttpPatternOrBuilder>(
(com.google.api.CustomHttpPattern) pattern_,
getParentForChildren(),
isClean());
pattern_ = null;
}
patternCase_ = 8;
onChanged();;
return customBuilder_;
}
private java.lang.Object body_ = "";
/**
*
* The name of the request field whose value is mapped to the HTTP body, or
* `*` for mapping all fields not captured by the path pattern to the HTTP
* body.
*
*
* optional string body = 7;
*/
public java.lang.String getBody() {
java.lang.Object ref = body_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
body_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The name of the request field whose value is mapped to the HTTP body, or
* `*` for mapping all fields not captured by the path pattern to the HTTP
* body.
*
*
* optional string body = 7;
*/
public com.google.protobuf.ByteString
getBodyBytes() {
java.lang.Object ref = body_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
body_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The name of the request field whose value is mapped to the HTTP body, or
* `*` for mapping all fields not captured by the path pattern to the HTTP
* body.
*
*
* optional string body = 7;
*/
public Builder setBody(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
body_ = value;
onChanged();
return this;
}
/**
*
* The name of the request field whose value is mapped to the HTTP body, or
* `*` for mapping all fields not captured by the path pattern to the HTTP
* body.
*
*
* optional string body = 7;
*/
public Builder clearBody() {
body_ = getDefaultInstance().getBody();
onChanged();
return this;
}
/**
*
* The name of the request field whose value is mapped to the HTTP body, or
* `*` for mapping all fields not captured by the path pattern to the HTTP
* body.
*
*
* optional string body = 7;
*/
public Builder setBodyBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
body_ = value;
onChanged();
return this;
}
private java.util.List additionalBindings_ =
java.util.Collections.emptyList();
private void ensureAdditionalBindingsIsMutable() {
if (!((bitField0_ & 0x00000080) == 0x00000080)) {
additionalBindings_ = new java.util.ArrayList(additionalBindings_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.api.HttpRule, com.google.api.HttpRule.Builder, com.google.api.HttpRuleOrBuilder> additionalBindingsBuilder_;
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public java.util.List getAdditionalBindingsList() {
if (additionalBindingsBuilder_ == null) {
return java.util.Collections.unmodifiableList(additionalBindings_);
} else {
return additionalBindingsBuilder_.getMessageList();
}
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public int getAdditionalBindingsCount() {
if (additionalBindingsBuilder_ == null) {
return additionalBindings_.size();
} else {
return additionalBindingsBuilder_.getCount();
}
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public com.google.api.HttpRule getAdditionalBindings(int index) {
if (additionalBindingsBuilder_ == null) {
return additionalBindings_.get(index);
} else {
return additionalBindingsBuilder_.getMessage(index);
}
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder setAdditionalBindings(
int index, com.google.api.HttpRule value) {
if (additionalBindingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalBindingsIsMutable();
additionalBindings_.set(index, value);
onChanged();
} else {
additionalBindingsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder setAdditionalBindings(
int index, com.google.api.HttpRule.Builder builderForValue) {
if (additionalBindingsBuilder_ == null) {
ensureAdditionalBindingsIsMutable();
additionalBindings_.set(index, builderForValue.build());
onChanged();
} else {
additionalBindingsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder addAdditionalBindings(com.google.api.HttpRule value) {
if (additionalBindingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalBindingsIsMutable();
additionalBindings_.add(value);
onChanged();
} else {
additionalBindingsBuilder_.addMessage(value);
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder addAdditionalBindings(
int index, com.google.api.HttpRule value) {
if (additionalBindingsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureAdditionalBindingsIsMutable();
additionalBindings_.add(index, value);
onChanged();
} else {
additionalBindingsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder addAdditionalBindings(
com.google.api.HttpRule.Builder builderForValue) {
if (additionalBindingsBuilder_ == null) {
ensureAdditionalBindingsIsMutable();
additionalBindings_.add(builderForValue.build());
onChanged();
} else {
additionalBindingsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder addAdditionalBindings(
int index, com.google.api.HttpRule.Builder builderForValue) {
if (additionalBindingsBuilder_ == null) {
ensureAdditionalBindingsIsMutable();
additionalBindings_.add(index, builderForValue.build());
onChanged();
} else {
additionalBindingsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder addAllAdditionalBindings(
java.lang.Iterable extends com.google.api.HttpRule> values) {
if (additionalBindingsBuilder_ == null) {
ensureAdditionalBindingsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, additionalBindings_);
onChanged();
} else {
additionalBindingsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder clearAdditionalBindings() {
if (additionalBindingsBuilder_ == null) {
additionalBindings_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
additionalBindingsBuilder_.clear();
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public Builder removeAdditionalBindings(int index) {
if (additionalBindingsBuilder_ == null) {
ensureAdditionalBindingsIsMutable();
additionalBindings_.remove(index);
onChanged();
} else {
additionalBindingsBuilder_.remove(index);
}
return this;
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public com.google.api.HttpRule.Builder getAdditionalBindingsBuilder(
int index) {
return getAdditionalBindingsFieldBuilder().getBuilder(index);
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public com.google.api.HttpRuleOrBuilder getAdditionalBindingsOrBuilder(
int index) {
if (additionalBindingsBuilder_ == null) {
return additionalBindings_.get(index); } else {
return additionalBindingsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public java.util.List extends com.google.api.HttpRuleOrBuilder>
getAdditionalBindingsOrBuilderList() {
if (additionalBindingsBuilder_ != null) {
return additionalBindingsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(additionalBindings_);
}
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public com.google.api.HttpRule.Builder addAdditionalBindingsBuilder() {
return getAdditionalBindingsFieldBuilder().addBuilder(
com.google.api.HttpRule.getDefaultInstance());
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public com.google.api.HttpRule.Builder addAdditionalBindingsBuilder(
int index) {
return getAdditionalBindingsFieldBuilder().addBuilder(
index, com.google.api.HttpRule.getDefaultInstance());
}
/**
*
* Additional HTTP bindings for the selector. Nested bindings must not
* specify a selector and must not contain additional bindings.
*
*
* repeated .google.api.HttpRule additional_bindings = 11;
*/
public java.util.List
getAdditionalBindingsBuilderList() {
return getAdditionalBindingsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
com.google.api.HttpRule, com.google.api.HttpRule.Builder, com.google.api.HttpRuleOrBuilder>
getAdditionalBindingsFieldBuilder() {
if (additionalBindingsBuilder_ == null) {
additionalBindingsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
com.google.api.HttpRule, com.google.api.HttpRule.Builder, com.google.api.HttpRuleOrBuilder>(
additionalBindings_,
((bitField0_ & 0x00000080) == 0x00000080),
getParentForChildren(),
isClean());
additionalBindings_ = null;
}
return additionalBindingsBuilder_;
}
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return this;
}
// @@protoc_insertion_point(builder_scope:google.api.HttpRule)
}
// @@protoc_insertion_point(class_scope:google.api.HttpRule)
private static final com.google.api.HttpRule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new com.google.api.HttpRule();
}
public static com.google.api.HttpRule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
public HttpRule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new HttpRule(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
public com.google.api.HttpRule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy