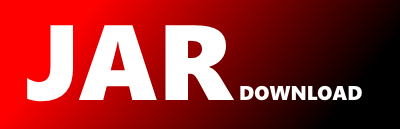
org.mvel2.SandboxedParserConfiguration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tbel Show documentation
Show all versions of tbel Show documentation
TBEL is a powerful expression language for ThingsBoard platform user-defined functions.
Original implementation is based on MVEL.
package org.mvel2;
import org.mvel2.compiler.AbstractParser;
import org.mvel2.util.TriFunction;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.function.Function;
import java.util.stream.Collectors;
public class SandboxedParserConfiguration extends ParserConfiguration {
private final Map, Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy