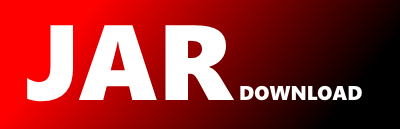
org.mvel2.execution.ExecutionEntry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tbel Show documentation
Show all versions of tbel Show documentation
TBEL is a powerful expression language for ThingsBoard platform user-defined functions.
Original implementation is based on MVEL.
package org.mvel2.execution;
import java.util.Map;
public class ExecutionEntry implements Map.Entry {
public final K key;
public V value;
public ExecutionEntry(K key, V value) {
this.key = key;
this.value = value;
}
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return value;
}
@Override
public V setValue(V value) {
V oldValue = this.value;
this.value = value;
return oldValue;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (!(o instanceof Map.Entry)) return false;
Map.Entry, ?> entry = (Map.Entry, ?>) o;
return (key == null ? entry.getKey() == null : key.equals(entry.getKey())) &&
(value == null ? entry.getValue() == null : value.equals(entry.getValue()));
}
@Override
public int hashCode() {
return (key == null ? 0 : key.hashCode()) ^ (value == null ? 0 : value.hashCode());
}
@Override
public String toString() {
return key + "=" + value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy