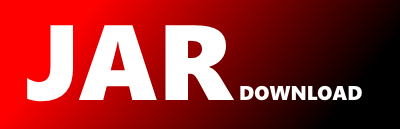
org.threadly.litesockets.utils.PortUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of litesockets Show documentation
Show all versions of litesockets Show documentation
A light weight socket library designed for highly concurrent applications. LiteSockets leverages threadly to make it easy to maintain concurrent connections while keeping threading on a per-client basis single threaded.
The newest version!
package org.threadly.litesockets.utils;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.net.ServerSocket;
import java.nio.channels.DatagramChannel;
/**
* Simple helper functions for dealing with ports.
*
* @author lwahlmeier
*
*/
public class PortUtils {
private PortUtils(){};
/**
* Returns an available TCP port.
*
* @return a free tcp port number.
*/
public static int findTCPPort() {
try {
ServerSocket s = new ServerSocket(0);
s.setReuseAddress(true);
int port = s.getLocalPort();
s.close();
return port;
} catch(IOException e) {
//We Dont Care
}
throw new RuntimeException("Could not find a port!!");
}
/**
* Returns an available UDP port.
*
* @return a free udp port number.
*/
public static int findUDPPort() {
try {
DatagramChannel channel = DatagramChannel.open();
channel.socket().bind(new InetSocketAddress("0.0.0.0", 0));
channel.socket().setReuseAddress(true);
int port = channel.socket().getLocalPort();
channel.close();
return port;
} catch(IOException e) {
//We Dont Care
}
throw new RuntimeException("Could not find a port!!");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy