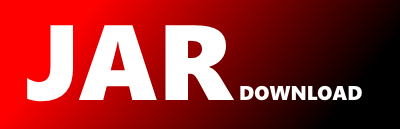
everything.Everything Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (1.0.0-dev)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package everything;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
/**
* This struct has a bunch of different fields
*/
@Generated(value = "Autogenerated by Thrift Compiler (1.0.0-dev)", date = "2016-02-10")
public class Everything implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("Everything");
private static final org.apache.thrift.protocol.TField STR_FIELD_DESC = new org.apache.thrift.protocol.TField("str", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField INT64_FIELD_DESC = new org.apache.thrift.protocol.TField("int64", org.apache.thrift.protocol.TType.I64, (short)2);
private static final org.apache.thrift.protocol.TField INT32_FIELD_DESC = new org.apache.thrift.protocol.TField("int32", org.apache.thrift.protocol.TType.I32, (short)3);
private static final org.apache.thrift.protocol.TField INT16_FIELD_DESC = new org.apache.thrift.protocol.TField("int16", org.apache.thrift.protocol.TType.I16, (short)4);
private static final org.apache.thrift.protocol.TField BITE_FIELD_DESC = new org.apache.thrift.protocol.TField("bite", org.apache.thrift.protocol.TType.BYTE, (short)5);
private static final org.apache.thrift.protocol.TField DBL_FIELD_DESC = new org.apache.thrift.protocol.TField("dbl", org.apache.thrift.protocol.TType.DOUBLE, (short)6);
private static final org.apache.thrift.protocol.TField BIN_FIELD_DESC = new org.apache.thrift.protocol.TField("bin", org.apache.thrift.protocol.TType.STRING, (short)7);
private static final org.apache.thrift.protocol.TField ENU_FIELD_DESC = new org.apache.thrift.protocol.TField("enu", org.apache.thrift.protocol.TType.I32, (short)8);
private static final org.apache.thrift.protocol.TField ONION_FIELD_DESC = new org.apache.thrift.protocol.TField("onion", org.apache.thrift.protocol.TType.STRUCT, (short)9);
private static final org.apache.thrift.protocol.TField STR_LIST_FIELD_DESC = new org.apache.thrift.protocol.TField("str_list", org.apache.thrift.protocol.TType.LIST, (short)10);
private static final org.apache.thrift.protocol.TField ENUM_LIST_FIELD_DESC = new org.apache.thrift.protocol.TField("enum_list", org.apache.thrift.protocol.TType.LIST, (short)11);
private static final org.apache.thrift.protocol.TField OBJ_LIST_FIELD_DESC = new org.apache.thrift.protocol.TField("obj_list", org.apache.thrift.protocol.TType.LIST, (short)12);
private static final org.apache.thrift.protocol.TField INT_LIST_LIST_FIELD_DESC = new org.apache.thrift.protocol.TField("int_list_list", org.apache.thrift.protocol.TType.LIST, (short)13);
private static final org.apache.thrift.protocol.TField STR_STR_MAP_FIELD_DESC = new org.apache.thrift.protocol.TField("str_str_map", org.apache.thrift.protocol.TType.MAP, (short)14);
private static final org.apache.thrift.protocol.TField INT_STR_MAP_FIELD_DESC = new org.apache.thrift.protocol.TField("int_str_map", org.apache.thrift.protocol.TType.MAP, (short)15);
private static final org.apache.thrift.protocol.TField INT_OBJ_MAP_FIELD_DESC = new org.apache.thrift.protocol.TField("int_obj_map", org.apache.thrift.protocol.TType.MAP, (short)16);
private static final org.apache.thrift.protocol.TField OBJ_FIELD_DESC = new org.apache.thrift.protocol.TField("obj", org.apache.thrift.protocol.TType.STRUCT, (short)17);
private static final org.apache.thrift.protocol.TField STR_SET_FIELD_DESC = new org.apache.thrift.protocol.TField("str_set", org.apache.thrift.protocol.TType.SET, (short)18);
private static final org.apache.thrift.protocol.TField OBJ_SET_FIELD_DESC = new org.apache.thrift.protocol.TField("obj_set", org.apache.thrift.protocol.TType.SET, (short)19);
private static final org.apache.thrift.protocol.TField SMORK_FIELD_DESC = new org.apache.thrift.protocol.TField("smork", org.apache.thrift.protocol.TType.STRUCT, (short)20);
private static final org.apache.thrift.protocol.TField ENUM_LIST_MAP_FIELD_DESC = new org.apache.thrift.protocol.TField("enum_list_map", org.apache.thrift.protocol.TType.MAP, (short)21);
private static final org.apache.thrift.protocol.TField REALLY_FIELD_DESC = new org.apache.thrift.protocol.TField("really", org.apache.thrift.protocol.TType.BOOL, (short)22);
private static final org.apache.thrift.protocol.TField EMPTY_FIELD_DESC = new org.apache.thrift.protocol.TField("empty", org.apache.thrift.protocol.TType.STRING, (short)23);
private static final org.apache.thrift.protocol.TField SOMEINT_FIELD_DESC = new org.apache.thrift.protocol.TField("someint", org.apache.thrift.protocol.TType.I32, (short)24);
private static final org.apache.thrift.protocol.TField SOMEOBJ_FIELD_DESC = new org.apache.thrift.protocol.TField("someobj", org.apache.thrift.protocol.TType.STRUCT, (short)25);
private static final org.apache.thrift.protocol.TField SOMEOBJ2_FIELD_DESC = new org.apache.thrift.protocol.TField("someobj2", org.apache.thrift.protocol.TType.STRUCT, (short)26);
private static final SchemeFactory STANDARD_SCHEME_FACTORY = new EverythingStandardSchemeFactory();
private static final SchemeFactory TUPLE_SCHEME_FACTORY = new EverythingTupleSchemeFactory();
public String str; // required
public long int64; // optional
public int int32; // required
public short int16; // required
public byte bite; // required
public double dbl; // required
public ByteBuffer bin; // required
/**
*
* @see Spinkle
*/
public Spinkle enu; // required
public Sprat onion; // required
public List str_list; // required
public List enum_list; // required
public List obj_list; // required
public List> int_list_list; // required
public Map str_str_map; // required
public Map int_str_map; // required
public Map int_obj_map; // required
public Spirfle obj; // required
public Set str_set; // required
public Set obj_set; // required
public another.Blotto smork; // required
public Map> enum_list_map; // required
public boolean really; // optional
public String empty; // required
public int someint; // required
public Sprat someobj; // required
public another.Blotto someobj2; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
STR((short)1, "str"),
INT64((short)2, "int64"),
INT32((short)3, "int32"),
INT16((short)4, "int16"),
BITE((short)5, "bite"),
DBL((short)6, "dbl"),
BIN((short)7, "bin"),
/**
*
* @see Spinkle
*/
ENU((short)8, "enu"),
ONION((short)9, "onion"),
STR_LIST((short)10, "str_list"),
ENUM_LIST((short)11, "enum_list"),
OBJ_LIST((short)12, "obj_list"),
INT_LIST_LIST((short)13, "int_list_list"),
STR_STR_MAP((short)14, "str_str_map"),
INT_STR_MAP((short)15, "int_str_map"),
INT_OBJ_MAP((short)16, "int_obj_map"),
OBJ((short)17, "obj"),
STR_SET((short)18, "str_set"),
OBJ_SET((short)19, "obj_set"),
SMORK((short)20, "smork"),
ENUM_LIST_MAP((short)21, "enum_list_map"),
REALLY((short)22, "really"),
EMPTY((short)23, "empty"),
SOMEINT((short)24, "someint"),
SOMEOBJ((short)25, "someobj"),
SOMEOBJ2((short)26, "someobj2");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // STR
return STR;
case 2: // INT64
return INT64;
case 3: // INT32
return INT32;
case 4: // INT16
return INT16;
case 5: // BITE
return BITE;
case 6: // DBL
return DBL;
case 7: // BIN
return BIN;
case 8: // ENU
return ENU;
case 9: // ONION
return ONION;
case 10: // STR_LIST
return STR_LIST;
case 11: // ENUM_LIST
return ENUM_LIST;
case 12: // OBJ_LIST
return OBJ_LIST;
case 13: // INT_LIST_LIST
return INT_LIST_LIST;
case 14: // STR_STR_MAP
return STR_STR_MAP;
case 15: // INT_STR_MAP
return INT_STR_MAP;
case 16: // INT_OBJ_MAP
return INT_OBJ_MAP;
case 17: // OBJ
return OBJ;
case 18: // STR_SET
return STR_SET;
case 19: // OBJ_SET
return OBJ_SET;
case 20: // SMORK
return SMORK;
case 21: // ENUM_LIST_MAP
return ENUM_LIST_MAP;
case 22: // REALLY
return REALLY;
case 23: // EMPTY
return EMPTY;
case 24: // SOMEINT
return SOMEINT;
case 25: // SOMEOBJ
return SOMEOBJ;
case 26: // SOMEOBJ2
return SOMEOBJ2;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __INT64_ISSET_ID = 0;
private static final int __INT32_ISSET_ID = 1;
private static final int __INT16_ISSET_ID = 2;
private static final int __BITE_ISSET_ID = 3;
private static final int __DBL_ISSET_ID = 4;
private static final int __REALLY_ISSET_ID = 5;
private static final int __SOMEINT_ISSET_ID = 6;
private byte __isset_bitfield = 0;
private static final _Fields optionals[] = {_Fields.INT64,_Fields.REALLY};
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.STR, new org.apache.thrift.meta_data.FieldMetaData("str", org.apache.thrift.TFieldRequirementType.REQUIRED,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.INT64, new org.apache.thrift.meta_data.FieldMetaData("int64", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I64)));
tmpMap.put(_Fields.INT32, new org.apache.thrift.meta_data.FieldMetaData("int32", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.INT16, new org.apache.thrift.meta_data.FieldMetaData("int16", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I16)));
tmpMap.put(_Fields.BITE, new org.apache.thrift.meta_data.FieldMetaData("bite", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BYTE)));
tmpMap.put(_Fields.DBL, new org.apache.thrift.meta_data.FieldMetaData("dbl", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.DOUBLE)));
tmpMap.put(_Fields.BIN, new org.apache.thrift.meta_data.FieldMetaData("bin", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING , true)));
tmpMap.put(_Fields.ENU, new org.apache.thrift.meta_data.FieldMetaData("enu", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Spinkle.class)));
tmpMap.put(_Fields.ONION, new org.apache.thrift.meta_data.FieldMetaData("onion", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Sprat.class)));
tmpMap.put(_Fields.STR_LIST, new org.apache.thrift.meta_data.FieldMetaData("str_list", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.ENUM_LIST, new org.apache.thrift.meta_data.FieldMetaData("enum_list", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Spinkle.class))));
tmpMap.put(_Fields.OBJ_LIST, new org.apache.thrift.meta_data.FieldMetaData("obj_list", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Spirfle.class))));
tmpMap.put(_Fields.INT_LIST_LIST, new org.apache.thrift.meta_data.FieldMetaData("int_list_list", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)))));
tmpMap.put(_Fields.STR_STR_MAP, new org.apache.thrift.meta_data.FieldMetaData("str_str_map", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING),
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.INT_STR_MAP, new org.apache.thrift.meta_data.FieldMetaData("int_str_map", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32),
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.INT_OBJ_MAP, new org.apache.thrift.meta_data.FieldMetaData("int_obj_map", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32),
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Spirfle.class))));
tmpMap.put(_Fields.OBJ, new org.apache.thrift.meta_data.FieldMetaData("obj", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Spirfle.class)));
tmpMap.put(_Fields.STR_SET, new org.apache.thrift.meta_data.FieldMetaData("str_set", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.SetMetaData(org.apache.thrift.protocol.TType.SET,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING))));
tmpMap.put(_Fields.OBJ_SET, new org.apache.thrift.meta_data.FieldMetaData("obj_set", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.SetMetaData(org.apache.thrift.protocol.TType.SET,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Spirfle.class))));
tmpMap.put(_Fields.SMORK, new org.apache.thrift.meta_data.FieldMetaData("smork", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, another.Blotto.class)));
tmpMap.put(_Fields.ENUM_LIST_MAP, new org.apache.thrift.meta_data.FieldMetaData("enum_list_map", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.MapMetaData(org.apache.thrift.protocol.TType.MAP,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Spinkle.class),
new org.apache.thrift.meta_data.ListMetaData(org.apache.thrift.protocol.TType.LIST,
new org.apache.thrift.meta_data.StructMetaData(org.apache.thrift.protocol.TType.STRUCT, Spirfle.class)))));
tmpMap.put(_Fields.REALLY, new org.apache.thrift.meta_data.FieldMetaData("really", org.apache.thrift.TFieldRequirementType.OPTIONAL,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.BOOL)));
tmpMap.put(_Fields.EMPTY, new org.apache.thrift.meta_data.FieldMetaData("empty", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.SOMEINT, new org.apache.thrift.meta_data.FieldMetaData("someint", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32 , "int32")));
tmpMap.put(_Fields.SOMEOBJ, new org.apache.thrift.meta_data.FieldMetaData("someobj", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "poig")));
tmpMap.put(_Fields.SOMEOBJ2, new org.apache.thrift.meta_data.FieldMetaData("someobj2", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "hammlegaff")));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(Everything.class, metaDataMap);
}
public Everything() {
this.str = "default";
this.really = true;
}
public Everything(
String str,
int int32,
short int16,
byte bite,
double dbl,
ByteBuffer bin,
Spinkle enu,
Sprat onion,
List str_list,
List enum_list,
List obj_list,
List> int_list_list,
Map str_str_map,
Map int_str_map,
Map int_obj_map,
Spirfle obj,
Set str_set,
Set obj_set,
another.Blotto smork,
Map> enum_list_map,
String empty,
int someint,
Sprat someobj,
another.Blotto someobj2)
{
this();
this.str = str;
this.int32 = int32;
setInt32IsSet(true);
this.int16 = int16;
setInt16IsSet(true);
this.bite = bite;
setBiteIsSet(true);
this.dbl = dbl;
setDblIsSet(true);
this.bin = org.apache.thrift.TBaseHelper.copyBinary(bin);
this.enu = enu;
this.onion = onion;
this.str_list = str_list;
this.enum_list = enum_list;
this.obj_list = obj_list;
this.int_list_list = int_list_list;
this.str_str_map = str_str_map;
this.int_str_map = int_str_map;
this.int_obj_map = int_obj_map;
this.obj = obj;
this.str_set = str_set;
this.obj_set = obj_set;
this.smork = smork;
this.enum_list_map = enum_list_map;
this.empty = empty;
this.someint = someint;
setSomeintIsSet(true);
this.someobj = someobj;
this.someobj2 = someobj2;
}
/**
* Performs a deep copy on other.
*/
public Everything(Everything other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetStr()) {
this.str = other.str;
}
this.int64 = other.int64;
this.int32 = other.int32;
this.int16 = other.int16;
this.bite = other.bite;
this.dbl = other.dbl;
if (other.isSetBin()) {
this.bin = org.apache.thrift.TBaseHelper.copyBinary(other.bin);
}
if (other.isSetEnu()) {
this.enu = other.enu;
}
if (other.isSetOnion()) {
this.onion = new Sprat(other.onion);
}
if (other.isSetStr_list()) {
List __this__str_list = new ArrayList(other.str_list);
this.str_list = __this__str_list;
}
if (other.isSetEnum_list()) {
List __this__enum_list = new ArrayList(other.enum_list.size());
for (Spinkle other_element : other.enum_list) {
__this__enum_list.add(other_element);
}
this.enum_list = __this__enum_list;
}
if (other.isSetObj_list()) {
List __this__obj_list = new ArrayList(other.obj_list.size());
for (Spirfle other_element : other.obj_list) {
__this__obj_list.add(new Spirfle(other_element));
}
this.obj_list = __this__obj_list;
}
if (other.isSetInt_list_list()) {
List> __this__int_list_list = new ArrayList>(other.int_list_list.size());
for (List other_element : other.int_list_list) {
List __this__int_list_list_copy = new ArrayList(other_element);
__this__int_list_list.add(__this__int_list_list_copy);
}
this.int_list_list = __this__int_list_list;
}
if (other.isSetStr_str_map()) {
Map __this__str_str_map = new HashMap(other.str_str_map);
this.str_str_map = __this__str_str_map;
}
if (other.isSetInt_str_map()) {
Map __this__int_str_map = new HashMap(other.int_str_map);
this.int_str_map = __this__int_str_map;
}
if (other.isSetInt_obj_map()) {
Map __this__int_obj_map = new HashMap(other.int_obj_map.size());
for (Map.Entry other_element : other.int_obj_map.entrySet()) {
Integer other_element_key = other_element.getKey();
Spirfle other_element_value = other_element.getValue();
Integer __this__int_obj_map_copy_key = other_element_key;
Spirfle __this__int_obj_map_copy_value = new Spirfle(other_element_value);
__this__int_obj_map.put(__this__int_obj_map_copy_key, __this__int_obj_map_copy_value);
}
this.int_obj_map = __this__int_obj_map;
}
if (other.isSetObj()) {
this.obj = new Spirfle(other.obj);
}
if (other.isSetStr_set()) {
Set __this__str_set = new HashSet(other.str_set);
this.str_set = __this__str_set;
}
if (other.isSetObj_set()) {
Set __this__obj_set = new HashSet(other.obj_set.size());
for (Spirfle other_element : other.obj_set) {
__this__obj_set.add(new Spirfle(other_element));
}
this.obj_set = __this__obj_set;
}
if (other.isSetSmork()) {
this.smork = new another.Blotto(other.smork);
}
if (other.isSetEnum_list_map()) {
Map> __this__enum_list_map = new HashMap>(other.enum_list_map.size());
for (Map.Entry> other_element : other.enum_list_map.entrySet()) {
Spinkle other_element_key = other_element.getKey();
List other_element_value = other_element.getValue();
Spinkle __this__enum_list_map_copy_key = other_element_key;
List __this__enum_list_map_copy_value = new ArrayList(other_element_value.size());
for (Spirfle other_element_value_element : other_element_value) {
__this__enum_list_map_copy_value.add(new Spirfle(other_element_value_element));
}
__this__enum_list_map.put(__this__enum_list_map_copy_key, __this__enum_list_map_copy_value);
}
this.enum_list_map = __this__enum_list_map;
}
this.really = other.really;
if (other.isSetEmpty()) {
this.empty = other.empty;
}
this.someint = other.someint;
if (other.isSetSomeobj()) {
this.someobj = other.someobj;
}
if (other.isSetSomeobj2()) {
this.someobj2 = other.someobj2;
}
}
public Everything deepCopy() {
return new Everything(this);
}
@Override
public void clear() {
this.str = "default";
setInt64IsSet(false);
this.int64 = 0;
setInt32IsSet(false);
this.int32 = 0;
setInt16IsSet(false);
this.int16 = 0;
setBiteIsSet(false);
this.bite = 0;
setDblIsSet(false);
this.dbl = 0.0;
this.bin = null;
this.enu = null;
this.onion = null;
this.str_list = null;
this.enum_list = null;
this.obj_list = null;
this.int_list_list = null;
this.str_str_map = null;
this.int_str_map = null;
this.int_obj_map = null;
this.obj = null;
this.str_set = null;
this.obj_set = null;
this.smork = null;
this.enum_list_map = null;
this.really = true;
this.empty = null;
setSomeintIsSet(false);
this.someint = 0;
this.someobj = null;
this.someobj2 = null;
}
public String getStr() {
return this.str;
}
public Everything setStr(String str) {
this.str = str;
return this;
}
public void unsetStr() {
this.str = null;
}
/** Returns true if field str is set (has been assigned a value) and false otherwise */
public boolean isSetStr() {
return this.str != null;
}
public void setStrIsSet(boolean value) {
if (!value) {
this.str = null;
}
}
public long getInt64() {
return this.int64;
}
public Everything setInt64(long int64) {
this.int64 = int64;
setInt64IsSet(true);
return this;
}
public void unsetInt64() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __INT64_ISSET_ID);
}
/** Returns true if field int64 is set (has been assigned a value) and false otherwise */
public boolean isSetInt64() {
return EncodingUtils.testBit(__isset_bitfield, __INT64_ISSET_ID);
}
public void setInt64IsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __INT64_ISSET_ID, value);
}
public int getInt32() {
return this.int32;
}
public Everything setInt32(int int32) {
this.int32 = int32;
setInt32IsSet(true);
return this;
}
public void unsetInt32() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __INT32_ISSET_ID);
}
/** Returns true if field int32 is set (has been assigned a value) and false otherwise */
public boolean isSetInt32() {
return EncodingUtils.testBit(__isset_bitfield, __INT32_ISSET_ID);
}
public void setInt32IsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __INT32_ISSET_ID, value);
}
public short getInt16() {
return this.int16;
}
public Everything setInt16(short int16) {
this.int16 = int16;
setInt16IsSet(true);
return this;
}
public void unsetInt16() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __INT16_ISSET_ID);
}
/** Returns true if field int16 is set (has been assigned a value) and false otherwise */
public boolean isSetInt16() {
return EncodingUtils.testBit(__isset_bitfield, __INT16_ISSET_ID);
}
public void setInt16IsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __INT16_ISSET_ID, value);
}
public byte getBite() {
return this.bite;
}
public Everything setBite(byte bite) {
this.bite = bite;
setBiteIsSet(true);
return this;
}
public void unsetBite() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __BITE_ISSET_ID);
}
/** Returns true if field bite is set (has been assigned a value) and false otherwise */
public boolean isSetBite() {
return EncodingUtils.testBit(__isset_bitfield, __BITE_ISSET_ID);
}
public void setBiteIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __BITE_ISSET_ID, value);
}
public double getDbl() {
return this.dbl;
}
public Everything setDbl(double dbl) {
this.dbl = dbl;
setDblIsSet(true);
return this;
}
public void unsetDbl() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __DBL_ISSET_ID);
}
/** Returns true if field dbl is set (has been assigned a value) and false otherwise */
public boolean isSetDbl() {
return EncodingUtils.testBit(__isset_bitfield, __DBL_ISSET_ID);
}
public void setDblIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __DBL_ISSET_ID, value);
}
public byte[] getBin() {
setBin(org.apache.thrift.TBaseHelper.rightSize(bin));
return bin == null ? null : bin.array();
}
public ByteBuffer bufferForBin() {
return org.apache.thrift.TBaseHelper.copyBinary(bin);
}
public Everything setBin(byte[] bin) {
this.bin = bin == null ? (ByteBuffer)null : ByteBuffer.wrap(Arrays.copyOf(bin, bin.length));
return this;
}
public Everything setBin(ByteBuffer bin) {
this.bin = org.apache.thrift.TBaseHelper.copyBinary(bin);
return this;
}
public void unsetBin() {
this.bin = null;
}
/** Returns true if field bin is set (has been assigned a value) and false otherwise */
public boolean isSetBin() {
return this.bin != null;
}
public void setBinIsSet(boolean value) {
if (!value) {
this.bin = null;
}
}
/**
*
* @see Spinkle
*/
public Spinkle getEnu() {
return this.enu;
}
/**
*
* @see Spinkle
*/
public Everything setEnu(Spinkle enu) {
this.enu = enu;
return this;
}
public void unsetEnu() {
this.enu = null;
}
/** Returns true if field enu is set (has been assigned a value) and false otherwise */
public boolean isSetEnu() {
return this.enu != null;
}
public void setEnuIsSet(boolean value) {
if (!value) {
this.enu = null;
}
}
public Sprat getOnion() {
return this.onion;
}
public Everything setOnion(Sprat onion) {
this.onion = onion;
return this;
}
public void unsetOnion() {
this.onion = null;
}
/** Returns true if field onion is set (has been assigned a value) and false otherwise */
public boolean isSetOnion() {
return this.onion != null;
}
public void setOnionIsSet(boolean value) {
if (!value) {
this.onion = null;
}
}
public int getStr_listSize() {
return (this.str_list == null) ? 0 : this.str_list.size();
}
public java.util.Iterator getStr_listIterator() {
return (this.str_list == null) ? null : this.str_list.iterator();
}
public void addToStr_list(String elem) {
if (this.str_list == null) {
this.str_list = new ArrayList();
}
this.str_list.add(elem);
}
public List getStr_list() {
return this.str_list;
}
public Everything setStr_list(List str_list) {
this.str_list = str_list;
return this;
}
public void unsetStr_list() {
this.str_list = null;
}
/** Returns true if field str_list is set (has been assigned a value) and false otherwise */
public boolean isSetStr_list() {
return this.str_list != null;
}
public void setStr_listIsSet(boolean value) {
if (!value) {
this.str_list = null;
}
}
public int getEnum_listSize() {
return (this.enum_list == null) ? 0 : this.enum_list.size();
}
public java.util.Iterator getEnum_listIterator() {
return (this.enum_list == null) ? null : this.enum_list.iterator();
}
public void addToEnum_list(Spinkle elem) {
if (this.enum_list == null) {
this.enum_list = new ArrayList();
}
this.enum_list.add(elem);
}
public List getEnum_list() {
return this.enum_list;
}
public Everything setEnum_list(List enum_list) {
this.enum_list = enum_list;
return this;
}
public void unsetEnum_list() {
this.enum_list = null;
}
/** Returns true if field enum_list is set (has been assigned a value) and false otherwise */
public boolean isSetEnum_list() {
return this.enum_list != null;
}
public void setEnum_listIsSet(boolean value) {
if (!value) {
this.enum_list = null;
}
}
public int getObj_listSize() {
return (this.obj_list == null) ? 0 : this.obj_list.size();
}
public java.util.Iterator getObj_listIterator() {
return (this.obj_list == null) ? null : this.obj_list.iterator();
}
public void addToObj_list(Spirfle elem) {
if (this.obj_list == null) {
this.obj_list = new ArrayList();
}
this.obj_list.add(elem);
}
public List getObj_list() {
return this.obj_list;
}
public Everything setObj_list(List obj_list) {
this.obj_list = obj_list;
return this;
}
public void unsetObj_list() {
this.obj_list = null;
}
/** Returns true if field obj_list is set (has been assigned a value) and false otherwise */
public boolean isSetObj_list() {
return this.obj_list != null;
}
public void setObj_listIsSet(boolean value) {
if (!value) {
this.obj_list = null;
}
}
public int getInt_list_listSize() {
return (this.int_list_list == null) ? 0 : this.int_list_list.size();
}
public java.util.Iterator> getInt_list_listIterator() {
return (this.int_list_list == null) ? null : this.int_list_list.iterator();
}
public void addToInt_list_list(List elem) {
if (this.int_list_list == null) {
this.int_list_list = new ArrayList>();
}
this.int_list_list.add(elem);
}
public List> getInt_list_list() {
return this.int_list_list;
}
public Everything setInt_list_list(List> int_list_list) {
this.int_list_list = int_list_list;
return this;
}
public void unsetInt_list_list() {
this.int_list_list = null;
}
/** Returns true if field int_list_list is set (has been assigned a value) and false otherwise */
public boolean isSetInt_list_list() {
return this.int_list_list != null;
}
public void setInt_list_listIsSet(boolean value) {
if (!value) {
this.int_list_list = null;
}
}
public int getStr_str_mapSize() {
return (this.str_str_map == null) ? 0 : this.str_str_map.size();
}
public void putToStr_str_map(String key, String val) {
if (this.str_str_map == null) {
this.str_str_map = new HashMap();
}
this.str_str_map.put(key, val);
}
public Map getStr_str_map() {
return this.str_str_map;
}
public Everything setStr_str_map(Map str_str_map) {
this.str_str_map = str_str_map;
return this;
}
public void unsetStr_str_map() {
this.str_str_map = null;
}
/** Returns true if field str_str_map is set (has been assigned a value) and false otherwise */
public boolean isSetStr_str_map() {
return this.str_str_map != null;
}
public void setStr_str_mapIsSet(boolean value) {
if (!value) {
this.str_str_map = null;
}
}
public int getInt_str_mapSize() {
return (this.int_str_map == null) ? 0 : this.int_str_map.size();
}
public void putToInt_str_map(int key, String val) {
if (this.int_str_map == null) {
this.int_str_map = new HashMap();
}
this.int_str_map.put(key, val);
}
public Map getInt_str_map() {
return this.int_str_map;
}
public Everything setInt_str_map(Map int_str_map) {
this.int_str_map = int_str_map;
return this;
}
public void unsetInt_str_map() {
this.int_str_map = null;
}
/** Returns true if field int_str_map is set (has been assigned a value) and false otherwise */
public boolean isSetInt_str_map() {
return this.int_str_map != null;
}
public void setInt_str_mapIsSet(boolean value) {
if (!value) {
this.int_str_map = null;
}
}
public int getInt_obj_mapSize() {
return (this.int_obj_map == null) ? 0 : this.int_obj_map.size();
}
public void putToInt_obj_map(int key, Spirfle val) {
if (this.int_obj_map == null) {
this.int_obj_map = new HashMap();
}
this.int_obj_map.put(key, val);
}
public Map getInt_obj_map() {
return this.int_obj_map;
}
public Everything setInt_obj_map(Map int_obj_map) {
this.int_obj_map = int_obj_map;
return this;
}
public void unsetInt_obj_map() {
this.int_obj_map = null;
}
/** Returns true if field int_obj_map is set (has been assigned a value) and false otherwise */
public boolean isSetInt_obj_map() {
return this.int_obj_map != null;
}
public void setInt_obj_mapIsSet(boolean value) {
if (!value) {
this.int_obj_map = null;
}
}
public Spirfle getObj() {
return this.obj;
}
public Everything setObj(Spirfle obj) {
this.obj = obj;
return this;
}
public void unsetObj() {
this.obj = null;
}
/** Returns true if field obj is set (has been assigned a value) and false otherwise */
public boolean isSetObj() {
return this.obj != null;
}
public void setObjIsSet(boolean value) {
if (!value) {
this.obj = null;
}
}
public int getStr_setSize() {
return (this.str_set == null) ? 0 : this.str_set.size();
}
public java.util.Iterator getStr_setIterator() {
return (this.str_set == null) ? null : this.str_set.iterator();
}
public void addToStr_set(String elem) {
if (this.str_set == null) {
this.str_set = new HashSet();
}
this.str_set.add(elem);
}
public Set getStr_set() {
return this.str_set;
}
public Everything setStr_set(Set str_set) {
this.str_set = str_set;
return this;
}
public void unsetStr_set() {
this.str_set = null;
}
/** Returns true if field str_set is set (has been assigned a value) and false otherwise */
public boolean isSetStr_set() {
return this.str_set != null;
}
public void setStr_setIsSet(boolean value) {
if (!value) {
this.str_set = null;
}
}
public int getObj_setSize() {
return (this.obj_set == null) ? 0 : this.obj_set.size();
}
public java.util.Iterator getObj_setIterator() {
return (this.obj_set == null) ? null : this.obj_set.iterator();
}
public void addToObj_set(Spirfle elem) {
if (this.obj_set == null) {
this.obj_set = new HashSet();
}
this.obj_set.add(elem);
}
public Set getObj_set() {
return this.obj_set;
}
public Everything setObj_set(Set obj_set) {
this.obj_set = obj_set;
return this;
}
public void unsetObj_set() {
this.obj_set = null;
}
/** Returns true if field obj_set is set (has been assigned a value) and false otherwise */
public boolean isSetObj_set() {
return this.obj_set != null;
}
public void setObj_setIsSet(boolean value) {
if (!value) {
this.obj_set = null;
}
}
public another.Blotto getSmork() {
return this.smork;
}
public Everything setSmork(another.Blotto smork) {
this.smork = smork;
return this;
}
public void unsetSmork() {
this.smork = null;
}
/** Returns true if field smork is set (has been assigned a value) and false otherwise */
public boolean isSetSmork() {
return this.smork != null;
}
public void setSmorkIsSet(boolean value) {
if (!value) {
this.smork = null;
}
}
public int getEnum_list_mapSize() {
return (this.enum_list_map == null) ? 0 : this.enum_list_map.size();
}
public void putToEnum_list_map(Spinkle key, List val) {
if (this.enum_list_map == null) {
this.enum_list_map = new HashMap>();
}
this.enum_list_map.put(key, val);
}
public Map> getEnum_list_map() {
return this.enum_list_map;
}
public Everything setEnum_list_map(Map> enum_list_map) {
this.enum_list_map = enum_list_map;
return this;
}
public void unsetEnum_list_map() {
this.enum_list_map = null;
}
/** Returns true if field enum_list_map is set (has been assigned a value) and false otherwise */
public boolean isSetEnum_list_map() {
return this.enum_list_map != null;
}
public void setEnum_list_mapIsSet(boolean value) {
if (!value) {
this.enum_list_map = null;
}
}
public boolean isReally() {
return this.really;
}
public Everything setReally(boolean really) {
this.really = really;
setReallyIsSet(true);
return this;
}
public void unsetReally() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __REALLY_ISSET_ID);
}
/** Returns true if field really is set (has been assigned a value) and false otherwise */
public boolean isSetReally() {
return EncodingUtils.testBit(__isset_bitfield, __REALLY_ISSET_ID);
}
public void setReallyIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __REALLY_ISSET_ID, value);
}
public String getEmpty() {
return this.empty;
}
public Everything setEmpty(String empty) {
this.empty = empty;
return this;
}
public void unsetEmpty() {
this.empty = null;
}
/** Returns true if field empty is set (has been assigned a value) and false otherwise */
public boolean isSetEmpty() {
return this.empty != null;
}
public void setEmptyIsSet(boolean value) {
if (!value) {
this.empty = null;
}
}
public int getSomeint() {
return this.someint;
}
public Everything setSomeint(int someint) {
this.someint = someint;
setSomeintIsSet(true);
return this;
}
public void unsetSomeint() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SOMEINT_ISSET_ID);
}
/** Returns true if field someint is set (has been assigned a value) and false otherwise */
public boolean isSetSomeint() {
return EncodingUtils.testBit(__isset_bitfield, __SOMEINT_ISSET_ID);
}
public void setSomeintIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SOMEINT_ISSET_ID, value);
}
public Sprat getSomeobj() {
return this.someobj;
}
public Everything setSomeobj(Sprat someobj) {
this.someobj = someobj;
return this;
}
public void unsetSomeobj() {
this.someobj = null;
}
/** Returns true if field someobj is set (has been assigned a value) and false otherwise */
public boolean isSetSomeobj() {
return this.someobj != null;
}
public void setSomeobjIsSet(boolean value) {
if (!value) {
this.someobj = null;
}
}
public another.Blotto getSomeobj2() {
return this.someobj2;
}
public Everything setSomeobj2(another.Blotto someobj2) {
this.someobj2 = someobj2;
return this;
}
public void unsetSomeobj2() {
this.someobj2 = null;
}
/** Returns true if field someobj2 is set (has been assigned a value) and false otherwise */
public boolean isSetSomeobj2() {
return this.someobj2 != null;
}
public void setSomeobj2IsSet(boolean value) {
if (!value) {
this.someobj2 = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case STR:
if (value == null) {
unsetStr();
} else {
setStr((String)value);
}
break;
case INT64:
if (value == null) {
unsetInt64();
} else {
setInt64((Long)value);
}
break;
case INT32:
if (value == null) {
unsetInt32();
} else {
setInt32((Integer)value);
}
break;
case INT16:
if (value == null) {
unsetInt16();
} else {
setInt16((Short)value);
}
break;
case BITE:
if (value == null) {
unsetBite();
} else {
setBite((Byte)value);
}
break;
case DBL:
if (value == null) {
unsetDbl();
} else {
setDbl((Double)value);
}
break;
case BIN:
if (value == null) {
unsetBin();
} else {
if (value instanceof byte[]) {
setBin((byte[])value);
} else {
setBin((ByteBuffer)value);
}
}
break;
case ENU:
if (value == null) {
unsetEnu();
} else {
setEnu((Spinkle)value);
}
break;
case ONION:
if (value == null) {
unsetOnion();
} else {
setOnion((Sprat)value);
}
break;
case STR_LIST:
if (value == null) {
unsetStr_list();
} else {
setStr_list((List)value);
}
break;
case ENUM_LIST:
if (value == null) {
unsetEnum_list();
} else {
setEnum_list((List)value);
}
break;
case OBJ_LIST:
if (value == null) {
unsetObj_list();
} else {
setObj_list((List)value);
}
break;
case INT_LIST_LIST:
if (value == null) {
unsetInt_list_list();
} else {
setInt_list_list((List>)value);
}
break;
case STR_STR_MAP:
if (value == null) {
unsetStr_str_map();
} else {
setStr_str_map((Map)value);
}
break;
case INT_STR_MAP:
if (value == null) {
unsetInt_str_map();
} else {
setInt_str_map((Map)value);
}
break;
case INT_OBJ_MAP:
if (value == null) {
unsetInt_obj_map();
} else {
setInt_obj_map((Map)value);
}
break;
case OBJ:
if (value == null) {
unsetObj();
} else {
setObj((Spirfle)value);
}
break;
case STR_SET:
if (value == null) {
unsetStr_set();
} else {
setStr_set((Set)value);
}
break;
case OBJ_SET:
if (value == null) {
unsetObj_set();
} else {
setObj_set((Set)value);
}
break;
case SMORK:
if (value == null) {
unsetSmork();
} else {
setSmork((another.Blotto)value);
}
break;
case ENUM_LIST_MAP:
if (value == null) {
unsetEnum_list_map();
} else {
setEnum_list_map((Map>)value);
}
break;
case REALLY:
if (value == null) {
unsetReally();
} else {
setReally((Boolean)value);
}
break;
case EMPTY:
if (value == null) {
unsetEmpty();
} else {
setEmpty((String)value);
}
break;
case SOMEINT:
if (value == null) {
unsetSomeint();
} else {
setSomeint((Integer)value);
}
break;
case SOMEOBJ:
if (value == null) {
unsetSomeobj();
} else {
setSomeobj((Sprat)value);
}
break;
case SOMEOBJ2:
if (value == null) {
unsetSomeobj2();
} else {
setSomeobj2((another.Blotto)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case STR:
return getStr();
case INT64:
return getInt64();
case INT32:
return getInt32();
case INT16:
return getInt16();
case BITE:
return getBite();
case DBL:
return getDbl();
case BIN:
return getBin();
case ENU:
return getEnu();
case ONION:
return getOnion();
case STR_LIST:
return getStr_list();
case ENUM_LIST:
return getEnum_list();
case OBJ_LIST:
return getObj_list();
case INT_LIST_LIST:
return getInt_list_list();
case STR_STR_MAP:
return getStr_str_map();
case INT_STR_MAP:
return getInt_str_map();
case INT_OBJ_MAP:
return getInt_obj_map();
case OBJ:
return getObj();
case STR_SET:
return getStr_set();
case OBJ_SET:
return getObj_set();
case SMORK:
return getSmork();
case ENUM_LIST_MAP:
return getEnum_list_map();
case REALLY:
return isReally();
case EMPTY:
return getEmpty();
case SOMEINT:
return getSomeint();
case SOMEOBJ:
return getSomeobj();
case SOMEOBJ2:
return getSomeobj2();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case STR:
return isSetStr();
case INT64:
return isSetInt64();
case INT32:
return isSetInt32();
case INT16:
return isSetInt16();
case BITE:
return isSetBite();
case DBL:
return isSetDbl();
case BIN:
return isSetBin();
case ENU:
return isSetEnu();
case ONION:
return isSetOnion();
case STR_LIST:
return isSetStr_list();
case ENUM_LIST:
return isSetEnum_list();
case OBJ_LIST:
return isSetObj_list();
case INT_LIST_LIST:
return isSetInt_list_list();
case STR_STR_MAP:
return isSetStr_str_map();
case INT_STR_MAP:
return isSetInt_str_map();
case INT_OBJ_MAP:
return isSetInt_obj_map();
case OBJ:
return isSetObj();
case STR_SET:
return isSetStr_set();
case OBJ_SET:
return isSetObj_set();
case SMORK:
return isSetSmork();
case ENUM_LIST_MAP:
return isSetEnum_list_map();
case REALLY:
return isSetReally();
case EMPTY:
return isSetEmpty();
case SOMEINT:
return isSetSomeint();
case SOMEOBJ:
return isSetSomeobj();
case SOMEOBJ2:
return isSetSomeobj2();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof Everything)
return this.equals((Everything)that);
return false;
}
public boolean equals(Everything that) {
if (that == null)
return false;
boolean this_present_str = true && this.isSetStr();
boolean that_present_str = true && that.isSetStr();
if (this_present_str || that_present_str) {
if (!(this_present_str && that_present_str))
return false;
if (!this.str.equals(that.str))
return false;
}
boolean this_present_int64 = true && this.isSetInt64();
boolean that_present_int64 = true && that.isSetInt64();
if (this_present_int64 || that_present_int64) {
if (!(this_present_int64 && that_present_int64))
return false;
if (this.int64 != that.int64)
return false;
}
boolean this_present_int32 = true;
boolean that_present_int32 = true;
if (this_present_int32 || that_present_int32) {
if (!(this_present_int32 && that_present_int32))
return false;
if (this.int32 != that.int32)
return false;
}
boolean this_present_int16 = true;
boolean that_present_int16 = true;
if (this_present_int16 || that_present_int16) {
if (!(this_present_int16 && that_present_int16))
return false;
if (this.int16 != that.int16)
return false;
}
boolean this_present_bite = true;
boolean that_present_bite = true;
if (this_present_bite || that_present_bite) {
if (!(this_present_bite && that_present_bite))
return false;
if (this.bite != that.bite)
return false;
}
boolean this_present_dbl = true;
boolean that_present_dbl = true;
if (this_present_dbl || that_present_dbl) {
if (!(this_present_dbl && that_present_dbl))
return false;
if (this.dbl != that.dbl)
return false;
}
boolean this_present_bin = true && this.isSetBin();
boolean that_present_bin = true && that.isSetBin();
if (this_present_bin || that_present_bin) {
if (!(this_present_bin && that_present_bin))
return false;
if (!this.bin.equals(that.bin))
return false;
}
boolean this_present_enu = true && this.isSetEnu();
boolean that_present_enu = true && that.isSetEnu();
if (this_present_enu || that_present_enu) {
if (!(this_present_enu && that_present_enu))
return false;
if (!this.enu.equals(that.enu))
return false;
}
boolean this_present_onion = true && this.isSetOnion();
boolean that_present_onion = true && that.isSetOnion();
if (this_present_onion || that_present_onion) {
if (!(this_present_onion && that_present_onion))
return false;
if (!this.onion.equals(that.onion))
return false;
}
boolean this_present_str_list = true && this.isSetStr_list();
boolean that_present_str_list = true && that.isSetStr_list();
if (this_present_str_list || that_present_str_list) {
if (!(this_present_str_list && that_present_str_list))
return false;
if (!this.str_list.equals(that.str_list))
return false;
}
boolean this_present_enum_list = true && this.isSetEnum_list();
boolean that_present_enum_list = true && that.isSetEnum_list();
if (this_present_enum_list || that_present_enum_list) {
if (!(this_present_enum_list && that_present_enum_list))
return false;
if (!this.enum_list.equals(that.enum_list))
return false;
}
boolean this_present_obj_list = true && this.isSetObj_list();
boolean that_present_obj_list = true && that.isSetObj_list();
if (this_present_obj_list || that_present_obj_list) {
if (!(this_present_obj_list && that_present_obj_list))
return false;
if (!this.obj_list.equals(that.obj_list))
return false;
}
boolean this_present_int_list_list = true && this.isSetInt_list_list();
boolean that_present_int_list_list = true && that.isSetInt_list_list();
if (this_present_int_list_list || that_present_int_list_list) {
if (!(this_present_int_list_list && that_present_int_list_list))
return false;
if (!this.int_list_list.equals(that.int_list_list))
return false;
}
boolean this_present_str_str_map = true && this.isSetStr_str_map();
boolean that_present_str_str_map = true && that.isSetStr_str_map();
if (this_present_str_str_map || that_present_str_str_map) {
if (!(this_present_str_str_map && that_present_str_str_map))
return false;
if (!this.str_str_map.equals(that.str_str_map))
return false;
}
boolean this_present_int_str_map = true && this.isSetInt_str_map();
boolean that_present_int_str_map = true && that.isSetInt_str_map();
if (this_present_int_str_map || that_present_int_str_map) {
if (!(this_present_int_str_map && that_present_int_str_map))
return false;
if (!this.int_str_map.equals(that.int_str_map))
return false;
}
boolean this_present_int_obj_map = true && this.isSetInt_obj_map();
boolean that_present_int_obj_map = true && that.isSetInt_obj_map();
if (this_present_int_obj_map || that_present_int_obj_map) {
if (!(this_present_int_obj_map && that_present_int_obj_map))
return false;
if (!this.int_obj_map.equals(that.int_obj_map))
return false;
}
boolean this_present_obj = true && this.isSetObj();
boolean that_present_obj = true && that.isSetObj();
if (this_present_obj || that_present_obj) {
if (!(this_present_obj && that_present_obj))
return false;
if (!this.obj.equals(that.obj))
return false;
}
boolean this_present_str_set = true && this.isSetStr_set();
boolean that_present_str_set = true && that.isSetStr_set();
if (this_present_str_set || that_present_str_set) {
if (!(this_present_str_set && that_present_str_set))
return false;
if (!this.str_set.equals(that.str_set))
return false;
}
boolean this_present_obj_set = true && this.isSetObj_set();
boolean that_present_obj_set = true && that.isSetObj_set();
if (this_present_obj_set || that_present_obj_set) {
if (!(this_present_obj_set && that_present_obj_set))
return false;
if (!this.obj_set.equals(that.obj_set))
return false;
}
boolean this_present_smork = true && this.isSetSmork();
boolean that_present_smork = true && that.isSetSmork();
if (this_present_smork || that_present_smork) {
if (!(this_present_smork && that_present_smork))
return false;
if (!this.smork.equals(that.smork))
return false;
}
boolean this_present_enum_list_map = true && this.isSetEnum_list_map();
boolean that_present_enum_list_map = true && that.isSetEnum_list_map();
if (this_present_enum_list_map || that_present_enum_list_map) {
if (!(this_present_enum_list_map && that_present_enum_list_map))
return false;
if (!this.enum_list_map.equals(that.enum_list_map))
return false;
}
boolean this_present_really = true && this.isSetReally();
boolean that_present_really = true && that.isSetReally();
if (this_present_really || that_present_really) {
if (!(this_present_really && that_present_really))
return false;
if (this.really != that.really)
return false;
}
boolean this_present_empty = true && this.isSetEmpty();
boolean that_present_empty = true && that.isSetEmpty();
if (this_present_empty || that_present_empty) {
if (!(this_present_empty && that_present_empty))
return false;
if (!this.empty.equals(that.empty))
return false;
}
boolean this_present_someint = true;
boolean that_present_someint = true;
if (this_present_someint || that_present_someint) {
if (!(this_present_someint && that_present_someint))
return false;
if (this.someint != that.someint)
return false;
}
boolean this_present_someobj = true && this.isSetSomeobj();
boolean that_present_someobj = true && that.isSetSomeobj();
if (this_present_someobj || that_present_someobj) {
if (!(this_present_someobj && that_present_someobj))
return false;
if (!this.someobj.equals(that.someobj))
return false;
}
boolean this_present_someobj2 = true && this.isSetSomeobj2();
boolean that_present_someobj2 = true && that.isSetSomeobj2();
if (this_present_someobj2 || that_present_someobj2) {
if (!(this_present_someobj2 && that_present_someobj2))
return false;
if (!this.someobj2.equals(that.someobj2))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy