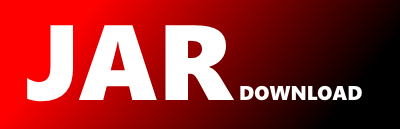
everything.Spirfle Maven / Gradle / Ivy
/**
* Autogenerated by Thrift Compiler (1.0.0-dev)
*
* DO NOT EDIT UNLESS YOU ARE SURE THAT YOU KNOW WHAT YOU ARE DOING
* @generated
*/
package everything;
import org.apache.thrift.scheme.IScheme;
import org.apache.thrift.scheme.SchemeFactory;
import org.apache.thrift.scheme.StandardScheme;
import org.apache.thrift.scheme.TupleScheme;
import org.apache.thrift.protocol.TTupleProtocol;
import org.apache.thrift.protocol.TProtocolException;
import org.apache.thrift.EncodingUtils;
import org.apache.thrift.TException;
import org.apache.thrift.async.AsyncMethodCallback;
import org.apache.thrift.server.AbstractNonblockingServer.*;
import java.util.List;
import java.util.ArrayList;
import java.util.Map;
import java.util.HashMap;
import java.util.EnumMap;
import java.util.Set;
import java.util.HashSet;
import java.util.EnumSet;
import java.util.Collections;
import java.util.BitSet;
import java.nio.ByteBuffer;
import java.util.Arrays;
import javax.annotation.Generated;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
@SuppressWarnings({"cast", "rawtypes", "serial", "unchecked", "unused"})
@Generated(value = "Autogenerated by Thrift Compiler (1.0.0-dev)", date = "2016-02-10")
public class Spirfle implements org.apache.thrift.TBase, java.io.Serializable, Cloneable, Comparable {
private static final org.apache.thrift.protocol.TStruct STRUCT_DESC = new org.apache.thrift.protocol.TStruct("Spirfle");
private static final org.apache.thrift.protocol.TField GIFFLE_FIELD_DESC = new org.apache.thrift.protocol.TField("giffle", org.apache.thrift.protocol.TType.STRING, (short)1);
private static final org.apache.thrift.protocol.TField FLAR_FIELD_DESC = new org.apache.thrift.protocol.TField("flar", org.apache.thrift.protocol.TType.I32, (short)2);
private static final org.apache.thrift.protocol.TField SPINKLE_FIELD_DESC = new org.apache.thrift.protocol.TField("spinkle", org.apache.thrift.protocol.TType.I32, (short)3);
private static final org.apache.thrift.protocol.TField SPOOT_FIELD_DESC = new org.apache.thrift.protocol.TField("spoot", org.apache.thrift.protocol.TType.I32, (short)4);
private static final org.apache.thrift.protocol.TField SPRAT_FIELD_DESC = new org.apache.thrift.protocol.TField("sprat", org.apache.thrift.protocol.TType.STRUCT, (short)5);
private static final org.apache.thrift.protocol.TField BLOTTO_FIELD_DESC = new org.apache.thrift.protocol.TField("blotto", org.apache.thrift.protocol.TType.STRUCT, (short)6);
private static final SchemeFactory STANDARD_SCHEME_FACTORY = new SpirfleStandardSchemeFactory();
private static final SchemeFactory TUPLE_SCHEME_FACTORY = new SpirfleTupleSchemeFactory();
public String giffle; // required
public int flar; // required
/**
*
* @see Spinkle
*/
public Spinkle spinkle; // required
public int spoot; // required
public Sprat sprat; // required
public another.Blotto blotto; // required
/** The set of fields this struct contains, along with convenience methods for finding and manipulating them. */
public enum _Fields implements org.apache.thrift.TFieldIdEnum {
GIFFLE((short)1, "giffle"),
FLAR((short)2, "flar"),
/**
*
* @see Spinkle
*/
SPINKLE((short)3, "spinkle"),
SPOOT((short)4, "spoot"),
SPRAT((short)5, "sprat"),
BLOTTO((short)6, "blotto");
private static final Map byName = new HashMap();
static {
for (_Fields field : EnumSet.allOf(_Fields.class)) {
byName.put(field.getFieldName(), field);
}
}
/**
* Find the _Fields constant that matches fieldId, or null if its not found.
*/
public static _Fields findByThriftId(int fieldId) {
switch(fieldId) {
case 1: // GIFFLE
return GIFFLE;
case 2: // FLAR
return FLAR;
case 3: // SPINKLE
return SPINKLE;
case 4: // SPOOT
return SPOOT;
case 5: // SPRAT
return SPRAT;
case 6: // BLOTTO
return BLOTTO;
default:
return null;
}
}
/**
* Find the _Fields constant that matches fieldId, throwing an exception
* if it is not found.
*/
public static _Fields findByThriftIdOrThrow(int fieldId) {
_Fields fields = findByThriftId(fieldId);
if (fields == null) throw new IllegalArgumentException("Field " + fieldId + " doesn't exist!");
return fields;
}
/**
* Find the _Fields constant that matches name, or null if its not found.
*/
public static _Fields findByName(String name) {
return byName.get(name);
}
private final short _thriftId;
private final String _fieldName;
_Fields(short thriftId, String fieldName) {
_thriftId = thriftId;
_fieldName = fieldName;
}
public short getThriftFieldId() {
return _thriftId;
}
public String getFieldName() {
return _fieldName;
}
}
// isset id assignments
private static final int __FLAR_ISSET_ID = 0;
private static final int __SPOOT_ISSET_ID = 1;
private byte __isset_bitfield = 0;
public static final Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> metaDataMap;
static {
Map<_Fields, org.apache.thrift.meta_data.FieldMetaData> tmpMap = new EnumMap<_Fields, org.apache.thrift.meta_data.FieldMetaData>(_Fields.class);
tmpMap.put(_Fields.GIFFLE, new org.apache.thrift.meta_data.FieldMetaData("giffle", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRING)));
tmpMap.put(_Fields.FLAR, new org.apache.thrift.meta_data.FieldMetaData("flar", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32)));
tmpMap.put(_Fields.SPINKLE, new org.apache.thrift.meta_data.FieldMetaData("spinkle", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.EnumMetaData(org.apache.thrift.protocol.TType.ENUM, Spinkle.class)));
tmpMap.put(_Fields.SPOOT, new org.apache.thrift.meta_data.FieldMetaData("spoot", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.I32 , "dukk")));
tmpMap.put(_Fields.SPRAT, new org.apache.thrift.meta_data.FieldMetaData("sprat", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "poig")));
tmpMap.put(_Fields.BLOTTO, new org.apache.thrift.meta_data.FieldMetaData("blotto", org.apache.thrift.TFieldRequirementType.DEFAULT,
new org.apache.thrift.meta_data.FieldValueMetaData(org.apache.thrift.protocol.TType.STRUCT , "hammlegaff")));
metaDataMap = Collections.unmodifiableMap(tmpMap);
org.apache.thrift.meta_data.FieldMetaData.addStructMetaDataMap(Spirfle.class, metaDataMap);
}
public Spirfle() {
}
public Spirfle(
String giffle,
int flar,
Spinkle spinkle,
int spoot,
Sprat sprat,
another.Blotto blotto)
{
this();
this.giffle = giffle;
this.flar = flar;
setFlarIsSet(true);
this.spinkle = spinkle;
this.spoot = spoot;
setSpootIsSet(true);
this.sprat = sprat;
this.blotto = blotto;
}
/**
* Performs a deep copy on other.
*/
public Spirfle(Spirfle other) {
__isset_bitfield = other.__isset_bitfield;
if (other.isSetGiffle()) {
this.giffle = other.giffle;
}
this.flar = other.flar;
if (other.isSetSpinkle()) {
this.spinkle = other.spinkle;
}
this.spoot = other.spoot;
if (other.isSetSprat()) {
this.sprat = other.sprat;
}
if (other.isSetBlotto()) {
this.blotto = other.blotto;
}
}
public Spirfle deepCopy() {
return new Spirfle(this);
}
@Override
public void clear() {
this.giffle = null;
setFlarIsSet(false);
this.flar = 0;
this.spinkle = null;
setSpootIsSet(false);
this.spoot = 0;
this.sprat = null;
this.blotto = null;
}
public String getGiffle() {
return this.giffle;
}
public Spirfle setGiffle(String giffle) {
this.giffle = giffle;
return this;
}
public void unsetGiffle() {
this.giffle = null;
}
/** Returns true if field giffle is set (has been assigned a value) and false otherwise */
public boolean isSetGiffle() {
return this.giffle != null;
}
public void setGiffleIsSet(boolean value) {
if (!value) {
this.giffle = null;
}
}
public int getFlar() {
return this.flar;
}
public Spirfle setFlar(int flar) {
this.flar = flar;
setFlarIsSet(true);
return this;
}
public void unsetFlar() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __FLAR_ISSET_ID);
}
/** Returns true if field flar is set (has been assigned a value) and false otherwise */
public boolean isSetFlar() {
return EncodingUtils.testBit(__isset_bitfield, __FLAR_ISSET_ID);
}
public void setFlarIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __FLAR_ISSET_ID, value);
}
/**
*
* @see Spinkle
*/
public Spinkle getSpinkle() {
return this.spinkle;
}
/**
*
* @see Spinkle
*/
public Spirfle setSpinkle(Spinkle spinkle) {
this.spinkle = spinkle;
return this;
}
public void unsetSpinkle() {
this.spinkle = null;
}
/** Returns true if field spinkle is set (has been assigned a value) and false otherwise */
public boolean isSetSpinkle() {
return this.spinkle != null;
}
public void setSpinkleIsSet(boolean value) {
if (!value) {
this.spinkle = null;
}
}
public int getSpoot() {
return this.spoot;
}
public Spirfle setSpoot(int spoot) {
this.spoot = spoot;
setSpootIsSet(true);
return this;
}
public void unsetSpoot() {
__isset_bitfield = EncodingUtils.clearBit(__isset_bitfield, __SPOOT_ISSET_ID);
}
/** Returns true if field spoot is set (has been assigned a value) and false otherwise */
public boolean isSetSpoot() {
return EncodingUtils.testBit(__isset_bitfield, __SPOOT_ISSET_ID);
}
public void setSpootIsSet(boolean value) {
__isset_bitfield = EncodingUtils.setBit(__isset_bitfield, __SPOOT_ISSET_ID, value);
}
public Sprat getSprat() {
return this.sprat;
}
public Spirfle setSprat(Sprat sprat) {
this.sprat = sprat;
return this;
}
public void unsetSprat() {
this.sprat = null;
}
/** Returns true if field sprat is set (has been assigned a value) and false otherwise */
public boolean isSetSprat() {
return this.sprat != null;
}
public void setSpratIsSet(boolean value) {
if (!value) {
this.sprat = null;
}
}
public another.Blotto getBlotto() {
return this.blotto;
}
public Spirfle setBlotto(another.Blotto blotto) {
this.blotto = blotto;
return this;
}
public void unsetBlotto() {
this.blotto = null;
}
/** Returns true if field blotto is set (has been assigned a value) and false otherwise */
public boolean isSetBlotto() {
return this.blotto != null;
}
public void setBlottoIsSet(boolean value) {
if (!value) {
this.blotto = null;
}
}
public void setFieldValue(_Fields field, Object value) {
switch (field) {
case GIFFLE:
if (value == null) {
unsetGiffle();
} else {
setGiffle((String)value);
}
break;
case FLAR:
if (value == null) {
unsetFlar();
} else {
setFlar((Integer)value);
}
break;
case SPINKLE:
if (value == null) {
unsetSpinkle();
} else {
setSpinkle((Spinkle)value);
}
break;
case SPOOT:
if (value == null) {
unsetSpoot();
} else {
setSpoot((Integer)value);
}
break;
case SPRAT:
if (value == null) {
unsetSprat();
} else {
setSprat((Sprat)value);
}
break;
case BLOTTO:
if (value == null) {
unsetBlotto();
} else {
setBlotto((another.Blotto)value);
}
break;
}
}
public Object getFieldValue(_Fields field) {
switch (field) {
case GIFFLE:
return getGiffle();
case FLAR:
return getFlar();
case SPINKLE:
return getSpinkle();
case SPOOT:
return getSpoot();
case SPRAT:
return getSprat();
case BLOTTO:
return getBlotto();
}
throw new IllegalStateException();
}
/** Returns true if field corresponding to fieldID is set (has been assigned a value) and false otherwise */
public boolean isSet(_Fields field) {
if (field == null) {
throw new IllegalArgumentException();
}
switch (field) {
case GIFFLE:
return isSetGiffle();
case FLAR:
return isSetFlar();
case SPINKLE:
return isSetSpinkle();
case SPOOT:
return isSetSpoot();
case SPRAT:
return isSetSprat();
case BLOTTO:
return isSetBlotto();
}
throw new IllegalStateException();
}
@Override
public boolean equals(Object that) {
if (that == null)
return false;
if (that instanceof Spirfle)
return this.equals((Spirfle)that);
return false;
}
public boolean equals(Spirfle that) {
if (that == null)
return false;
boolean this_present_giffle = true && this.isSetGiffle();
boolean that_present_giffle = true && that.isSetGiffle();
if (this_present_giffle || that_present_giffle) {
if (!(this_present_giffle && that_present_giffle))
return false;
if (!this.giffle.equals(that.giffle))
return false;
}
boolean this_present_flar = true;
boolean that_present_flar = true;
if (this_present_flar || that_present_flar) {
if (!(this_present_flar && that_present_flar))
return false;
if (this.flar != that.flar)
return false;
}
boolean this_present_spinkle = true && this.isSetSpinkle();
boolean that_present_spinkle = true && that.isSetSpinkle();
if (this_present_spinkle || that_present_spinkle) {
if (!(this_present_spinkle && that_present_spinkle))
return false;
if (!this.spinkle.equals(that.spinkle))
return false;
}
boolean this_present_spoot = true;
boolean that_present_spoot = true;
if (this_present_spoot || that_present_spoot) {
if (!(this_present_spoot && that_present_spoot))
return false;
if (this.spoot != that.spoot)
return false;
}
boolean this_present_sprat = true && this.isSetSprat();
boolean that_present_sprat = true && that.isSetSprat();
if (this_present_sprat || that_present_sprat) {
if (!(this_present_sprat && that_present_sprat))
return false;
if (!this.sprat.equals(that.sprat))
return false;
}
boolean this_present_blotto = true && this.isSetBlotto();
boolean that_present_blotto = true && that.isSetBlotto();
if (this_present_blotto || that_present_blotto) {
if (!(this_present_blotto && that_present_blotto))
return false;
if (!this.blotto.equals(that.blotto))
return false;
}
return true;
}
@Override
public int hashCode() {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy