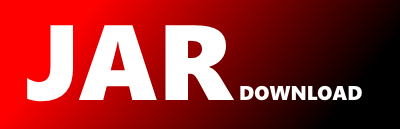
org.thymeleaf.standard.StandardDialect Maven / Gradle / Ivy
/*
* =============================================================================
*
* Copyright (c) 2011, The THYMELEAF team (http://www.thymeleaf.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* =============================================================================
*/
package org.thymeleaf.standard;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Set;
import org.thymeleaf.Standards;
import org.thymeleaf.dialect.AbstractXHTMLEnabledDialect;
import org.thymeleaf.doctype.DocTypeIdentifier;
import org.thymeleaf.doctype.resolution.ClassLoaderDocTypeResolutionEntry;
import org.thymeleaf.doctype.resolution.IDocTypeResolutionEntry;
import org.thymeleaf.doctype.translation.DocTypeTranslation;
import org.thymeleaf.doctype.translation.IDocTypeTranslation;
import org.thymeleaf.processor.attr.IAttrProcessor;
import org.thymeleaf.processor.tag.ITagProcessor;
import org.thymeleaf.processor.value.IValueProcessor;
import org.thymeleaf.standard.expression.ognl.StandardOGNLExpressionEvaluator;
import org.thymeleaf.standard.processor.attr.StandardAltTitleAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardAttrAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardAttrappendAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardAttrprependAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardClassappendAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardConditionalFixedValueAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardDOMEventAttributeModifierAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardEachAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardFragmentAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardIfAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardIncludeAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardInlineAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardLangXmlLangAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardObjectAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardRemoveAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardSingleNonRemovableAttributeModifierAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardSingleRemovableAttributeModifierAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardTextAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardUnlessAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardUtextAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardWithAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardXmlBaseAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardXmlLangAttrProcessor;
import org.thymeleaf.standard.processor.attr.StandardXmlSpaceAttrProcessor;
import org.thymeleaf.standard.processor.value.IStandardValueProcessor;
import org.thymeleaf.standard.processor.value.StandardValueProcessor;
import org.thymeleaf.standard.processor.value.link.IStandardLinkValueProcessor;
import org.thymeleaf.standard.processor.value.link.StandardLinkValueProcessor;
import org.thymeleaf.standard.processor.value.literal.IStandardLiteralValueProcessor;
import org.thymeleaf.standard.processor.value.literal.StandardLiteralValueProcessor;
import org.thymeleaf.standard.processor.value.message.IStandardMessageValueProcessor;
import org.thymeleaf.standard.processor.value.message.StandardMessageValueProcessor;
import org.thymeleaf.standard.processor.value.variable.IStandardVariableValueProcessor;
import org.thymeleaf.standard.processor.value.variable.StandardVariableValueProcessor;
/**
*
* The Standard Dialect, default implementation of {@link org.thymeleaf.dialect.IDialect}.
*
*
* - Prefix: th
* - Lenient: false
* - Attribute processors:
*
* - {@link StandardAltTitleAttrProcessor}
* - {@link StandardAttrAttrProcessor}
* - {@link StandardAttrappendAttrProcessor}
* - {@link StandardAttrprependAttrProcessor}
* - {@link StandardClassappendAttrProcessor}
* - {@link StandardConditionalFixedValueAttrProcessor}
* - {@link StandardDOMEventAttributeModifierAttrProcessor}
* - {@link StandardEachAttrProcessor}
* - {@link StandardFragmentAttrProcessor}
* - {@link StandardObjectAttrProcessor}
* - {@link StandardIfAttrProcessor}
* - {@link StandardInlineAttrProcessor}
* - {@link StandardUnlessAttrProcessor}
* - {@link StandardIncludeAttrProcessor}
* - {@link StandardLangXmlLangAttrProcessor}
* - {@link StandardRemoveAttrProcessor}
* - {@link StandardSingleNonRemovableAttributeModifierAttrProcessor}
* - {@link StandardSingleRemovableAttributeModifierAttrProcessor}
* - {@link StandardTextAttrProcessor}
* - {@link StandardUtextAttrProcessor}
* - {@link StandardWithAttrProcessor}
* - {@link StandardXmlBaseAttrProcessor}
* - {@link StandardXmlLangAttrProcessor}
* - {@link StandardXmlSpaceAttrProcessor}
*
*
* - Tag processors: none
* - Value processors:
*
* - {@link StandardLiteralValueProcessor}
* - {@link StandardValueProcessor}
* - {@link StandardVariableValueProcessor} with {@link StandardOGNLExpressionEvaluator} evaluator
* - {@link StandardMessageValueProcessor}
* - {@link StandardLinkValueProcessor}
*
*
* - DOCTYPE translations:
* - DOCTYPE resolution entries:
*
*
* @author Daniel Fernández
*
* @since 1.0
*
*/
public class StandardDialect extends AbstractXHTMLEnabledDialect {
public static final String PREFIX = "th";
public static final boolean LENIENT = false;
public static final DocTypeIdentifier XHTML1_STRICT_THYMELEAF1_SYSTEMID =
DocTypeIdentifier.forValue("http://www.thymeleaf.org/dtd/xhtml1-strict-thymeleaf-1.dtd");
public static final DocTypeIdentifier XHTML1_TRANSITIONAL_THYMELEAF1_SYSTEMID =
DocTypeIdentifier.forValue("http://www.thymeleaf.org/dtd/xhtml1-transitional-thymeleaf-1.dtd");
public static final DocTypeIdentifier XHTML1_FRAMESET_THYMELEAF1_SYSTEMID =
DocTypeIdentifier.forValue("http://www.thymeleaf.org/dtd/xhtml1-frameset-thymeleaf-1.dtd");
public static final DocTypeIdentifier XHTML11_THYMELEAF1_SYSTEMID =
DocTypeIdentifier.forValue("http://www.thymeleaf.org/dtd/xhtml11-thymeleaf-1.dtd");
public static final IDocTypeResolutionEntry XHTML1_STRICT_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY =
new ClassLoaderDocTypeResolutionEntry(
DocTypeIdentifier.NONE, // PUBLICID
XHTML1_STRICT_THYMELEAF1_SYSTEMID, // SYSTEMID
"org/thymeleaf/dtd/thymeleaf/xhtml1-strict-thymeleaf-1.dtd"); // CLASS-LOADER-RESOLVABLE RESOURCE
public static final IDocTypeResolutionEntry XHTML1_TRANSITIONAL_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY =
new ClassLoaderDocTypeResolutionEntry(
DocTypeIdentifier.NONE, // PUBLICID
XHTML1_TRANSITIONAL_THYMELEAF1_SYSTEMID, // SYSTEMID
"org/thymeleaf/dtd/thymeleaf/xhtml1-transitional-thymeleaf-1.dtd"); // CLASS-LOADER-RESOLVABLE RESOURCE
public static final IDocTypeResolutionEntry XHTML1_FRAMESET_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY =
new ClassLoaderDocTypeResolutionEntry(
DocTypeIdentifier.NONE, // PUBLICID
XHTML1_FRAMESET_THYMELEAF1_SYSTEMID, // SYSTEMID
"org/thymeleaf/dtd/thymeleaf/xhtml1-frameset-thymeleaf-1.dtd"); // CLASS-LOADER-RESOLVABLE RESOURCE
public static final IDocTypeResolutionEntry XHTML11_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY =
new ClassLoaderDocTypeResolutionEntry(
DocTypeIdentifier.NONE, // PUBLICID
XHTML11_THYMELEAF1_SYSTEMID, // SYSTEMID
"org/thymeleaf/dtd/thymeleaf/xhtml11-thymeleaf-1.dtd"); // CLASS-LOADER-RESOLVABLE RESOURCE
public static final Set DOC_TYPE_RESOLUTION_ENTRIES;
public static final IDocTypeTranslation XHTML1_STRICT_DOC_TYPE_TRANSLATION =
new DocTypeTranslation(
DocTypeIdentifier.NONE, // PUBLICID
XHTML1_STRICT_THYMELEAF1_SYSTEMID, // SYSTEMID
Standards.XHTML_1_STRICT_PUBLICID,
Standards.XHTML_1_STRICT_SYSTEMID);
public static final IDocTypeTranslation XHTML1_TRANSITIONAL_DOC_TYPE_TRANSLATION =
new DocTypeTranslation(
DocTypeIdentifier.NONE, // PUBLICID
XHTML1_TRANSITIONAL_THYMELEAF1_SYSTEMID, // SYSTEMID
Standards.XHTML_1_TRANSITIONAL_PUBLICID,
Standards.XHTML_1_TRANSITIONAL_SYSTEMID);
public static final IDocTypeTranslation XHTML1_FRAMESET_DOC_TYPE_TRANSLATION =
new DocTypeTranslation(
DocTypeIdentifier.NONE, // PUBLICID
XHTML1_FRAMESET_THYMELEAF1_SYSTEMID, // SYSTEMID
Standards.XHTML_1_FRAMESET_PUBLICID,
Standards.XHTML_1_FRAMESET_SYSTEMID);
public static final IDocTypeTranslation XHTML11_DOC_TYPE_TRANSLATION =
new DocTypeTranslation(
DocTypeIdentifier.NONE, // PUBLICID
XHTML11_THYMELEAF1_SYSTEMID, // SYSTEMID
Standards.XHTML_11_PUBLICID,
Standards.XHTML_11_SYSTEMID);
public static final Set DOC_TYPE_TRANSLATIONS =
Collections.unmodifiableSet(
new LinkedHashSet(
Arrays.asList(new IDocTypeTranslation[] {
XHTML1_STRICT_DOC_TYPE_TRANSLATION,
XHTML1_TRANSITIONAL_DOC_TYPE_TRANSLATION,
XHTML1_FRAMESET_DOC_TYPE_TRANSLATION,
XHTML11_DOC_TYPE_TRANSLATION
})));
public static final Set ATTR_ELEMENT_PROCESSORS =
Collections.unmodifiableSet(
new LinkedHashSet(
Arrays.asList(new IAttrProcessor[] {
new StandardAltTitleAttrProcessor(),
new StandardAttrAttrProcessor(),
new StandardAttrappendAttrProcessor(),
new StandardAttrprependAttrProcessor(),
new StandardClassappendAttrProcessor(),
new StandardConditionalFixedValueAttrProcessor(),
new StandardDOMEventAttributeModifierAttrProcessor(),
new StandardEachAttrProcessor(),
new StandardFragmentAttrProcessor(),
new StandardObjectAttrProcessor(),
new StandardIfAttrProcessor(),
new StandardInlineAttrProcessor(),
new StandardUnlessAttrProcessor(),
new StandardIncludeAttrProcessor(),
new StandardLangXmlLangAttrProcessor(),
new StandardRemoveAttrProcessor(),
new StandardSingleNonRemovableAttributeModifierAttrProcessor(),
new StandardSingleRemovableAttributeModifierAttrProcessor(),
new StandardTextAttrProcessor(),
new StandardUtextAttrProcessor(),
new StandardWithAttrProcessor(),
new StandardXmlBaseAttrProcessor(),
new StandardXmlLangAttrProcessor(),
new StandardXmlSpaceAttrProcessor()})));
public static final Set TAG_ELEMENT_PROCESSORS =
Collections.unmodifiableSet(
new LinkedHashSet(
Arrays.asList(new ITagProcessor[0])));
public static final Set VALUE_PROCESSORS =
Collections.unmodifiableSet(
new LinkedHashSet(
Arrays.asList(new IValueProcessor[] {
new StandardLiteralValueProcessor(),
new StandardValueProcessor(),
new StandardVariableValueProcessor(StandardOGNLExpressionEvaluator.INSTANCE),
new StandardMessageValueProcessor(),
new StandardLinkValueProcessor()})));
static {
final Set newDocTypeResolutionEntries = new LinkedHashSet();
newDocTypeResolutionEntries.add(XHTML1_STRICT_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY);
newDocTypeResolutionEntries.add(XHTML1_TRANSITIONAL_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY);
newDocTypeResolutionEntries.add(XHTML1_FRAMESET_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY);
newDocTypeResolutionEntries.add(XHTML11_THYMELEAF_1_DOC_TYPE_RESOLUTION_ENTRY);
DOC_TYPE_RESOLUTION_ENTRIES = Collections.unmodifiableSet(newDocTypeResolutionEntries);
}
public StandardDialect() {
super();
}
public String getPrefix() {
return PREFIX;
}
public boolean isLenient() {
return LENIENT;
}
@Override
public Set getDocTypeTranslations() {
final Set docTypeTranslations = new LinkedHashSet();
docTypeTranslations.addAll(DOC_TYPE_TRANSLATIONS);
final Set additionalDocTypeTranslations = getAdditionalDocTypeTranslations();
if (additionalDocTypeTranslations != null) {
docTypeTranslations.addAll(additionalDocTypeTranslations);
}
return Collections.unmodifiableSet(docTypeTranslations);
}
protected Set getAdditionalDocTypeTranslations() {
return null;
}
@Override
public Set getSpecificDocTypeResolutionEntries() {
final Set docTypeResolutionEntries = new LinkedHashSet();
docTypeResolutionEntries.addAll(DOC_TYPE_RESOLUTION_ENTRIES);
final Set additionalDocTypeResolutionEntries = getAdditionalDocTypeResolutionEntries();
if (additionalDocTypeResolutionEntries != null) {
docTypeResolutionEntries.addAll(additionalDocTypeResolutionEntries);
}
return Collections.unmodifiableSet(docTypeResolutionEntries);
}
protected Set getAdditionalDocTypeResolutionEntries() {
return null;
}
@Override
public Set getAttrProcessors() {
final List attrProcessors = new ArrayList();
final Set additionalAttrProcessors = getAdditionalAttrProcessors();
final Set> removedAttrProcessors = getRemovedAttrProcessors();
for (final IAttrProcessor attrProcessor : ATTR_ELEMENT_PROCESSORS) {
if (removedAttrProcessors == null || !removedAttrProcessors.contains(attrProcessor.getClass())) {
attrProcessors.add(attrProcessor);
}
}
if (additionalAttrProcessors != null) {
attrProcessors.addAll(additionalAttrProcessors);
}
return Collections.unmodifiableSet(new LinkedHashSet(attrProcessors));
}
protected Set getAdditionalAttrProcessors() {
return null;
}
protected Set> getRemovedAttrProcessors() {
return null;
}
@Override
public Set getTagProcessors() {
final List tagProcessors = new ArrayList();
final Set additionalTagProcessors = getAdditionalTagProcessors();
final Set> removedTagProcessors = getRemovedTagProcessors();
for (final ITagProcessor tagProcessor : TAG_ELEMENT_PROCESSORS) {
if (removedTagProcessors == null || !removedTagProcessors.contains(tagProcessor.getClass())) {
tagProcessors.add(tagProcessor);
}
}
if (additionalTagProcessors != null) {
tagProcessors.addAll(additionalTagProcessors);
}
return Collections.unmodifiableSet(new LinkedHashSet(tagProcessors));
}
protected Set getAdditionalTagProcessors() {
return null;
}
protected Set> getRemovedTagProcessors() {
return null;
}
@Override
public Set getValueProcessors() {
final Set valueProcessors = new LinkedHashSet();
valueProcessors.add(getStandardValueProcessor());
valueProcessors.add(getStandardLinkValueProcessor());
valueProcessors.add(getStandardLiteralValueProcessor());
valueProcessors.add(getStandardMessageValueProcessor());
valueProcessors.add(getStandardVariableValueProcessor());
return Collections.unmodifiableSet(valueProcessors);
}
protected IStandardValueProcessor getStandardValueProcessor() {
return new StandardValueProcessor();
}
protected IStandardLinkValueProcessor getStandardLinkValueProcessor() {
return new StandardLinkValueProcessor();
}
protected IStandardLiteralValueProcessor getStandardLiteralValueProcessor() {
return new StandardLiteralValueProcessor();
}
protected IStandardMessageValueProcessor getStandardMessageValueProcessor() {
return new StandardMessageValueProcessor();
}
protected IStandardVariableValueProcessor getStandardVariableValueProcessor() {
return new StandardVariableValueProcessor(StandardOGNLExpressionEvaluator.INSTANCE);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy